In C++, the `.hpp` file is typically used for declaring function prototypes and class definitions, while the `.cpp` file contains the actual implementation of those functions and methods.
Here's a simple example demonstrating the use of both file types:
Header file: example.hpp
#ifndef EXAMPLE_HPP
#define EXAMPLE_HPP
void greet();
#endif // EXAMPLE_HPP
Source file: example.cpp
#include <iostream>
#include "example.hpp"
void greet() {
std::cout << "Hello, World!" << std::endl;
}
In this example, `example.hpp` declares the `greet` function, and `example.cpp` provides its implementation.
Understanding C++ File Extensions
What is a .cpp File?
A .cpp file is a crucial component of C++ programming that contains the actual source code for a program. It is the place where the implementation of functions, classes, and algorithms are written. In essence, .cpp files serve as the backbone of C++ applications, holding the executable logic that runs when the program is executed.
For example, consider the following minimal .cpp file:
#include <iostream>
int main() {
std::cout << "Hello, World!";
return 0;
}
In this snippet, the `main` function is defined, which is the entry point of any C++ program, and `std::cout` is used for output. This simple program showcases core C++ syntax, demonstrating how .cpp files directly execute the logic of a program.
What is a .hpp File?
On the other hand, a .hpp file is commonly known as a header file. This file plays a pivotal role in declaring the interfaces for functions, classes, and constants without implementing them. Header files are essential for achieving modularity and separation of concerns in your C++ programs.
A basic example of a .hpp file would look like this:
#ifndef MYHEADER_HPP
#define MYHEADER_HPP
void printMessage();
#endif
In this example, the header file contains a function prototype for `printMessage`. It is surrounded by include guards (`#ifndef`, `#define`, and `#endif`), which help prevent multiple inclusions of the same header file, avoiding potential compilation errors.
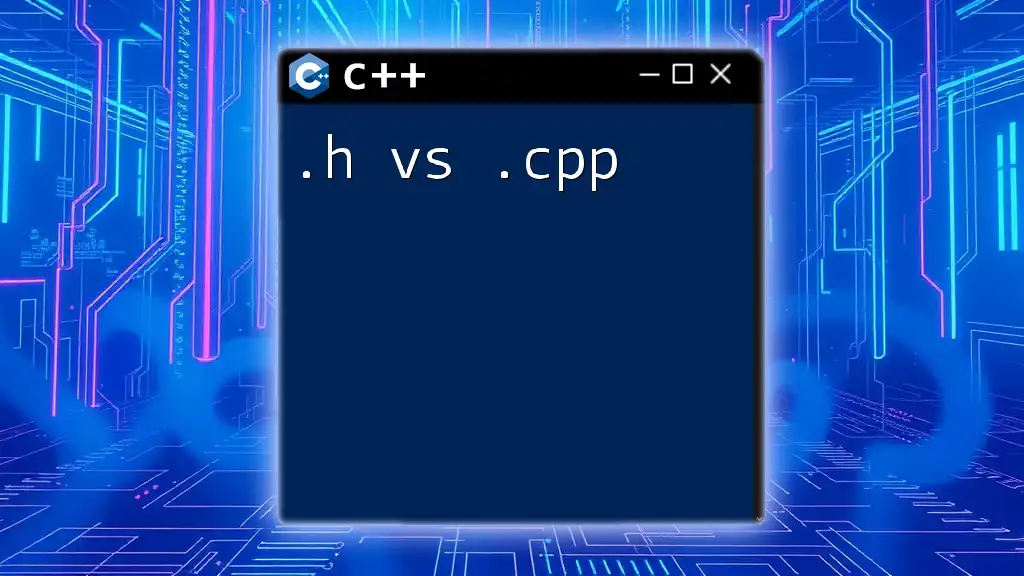
.hpp vs .cpp: Key Differences
Role in C++ Programming
When comparing .hpp vs .cpp, the most crucial distinction lies in their roles:
-
.cpp Files: These are implementation files that house the actual logic of your code. Functions defined in .cpp files are compiled to produce the executable binary. They may include one or more .hpp files to access declarations.
-
.hpp Files: These are declaration files that outline the structure of your program. They provide details about function prototypes, constants, and classes that will be implemented later in .cpp files. They act as a bridge, allowing different parts of a program to communicate.
Structure and Content
Typical contents of a .cpp file focus on function definitions, variable initialization, and main program logic. Conversely, .hpp files contain declarations, such as:
- Function prototypes
- Class definitions
- Macros
- Type definitions
An organized structure promotes better maintenance and readability.
Compilation Process
Understanding the compilation process is critical in .hpp vs .cpp discussions. The compiler processes .cpp files by translating them into executable machine code. When a .cpp file includes a .hpp file, the compiler first processes the declarations in the header before proceeding to the function definitions in the source file.
For example, consider the simplistic compilation command:
g++ main.cpp helper.cpp -o output
Here, both `main.cpp` and `helper.cpp` may reference `helper.hpp`. The compiler handles the translation in accordance with the dependencies established in the includes.
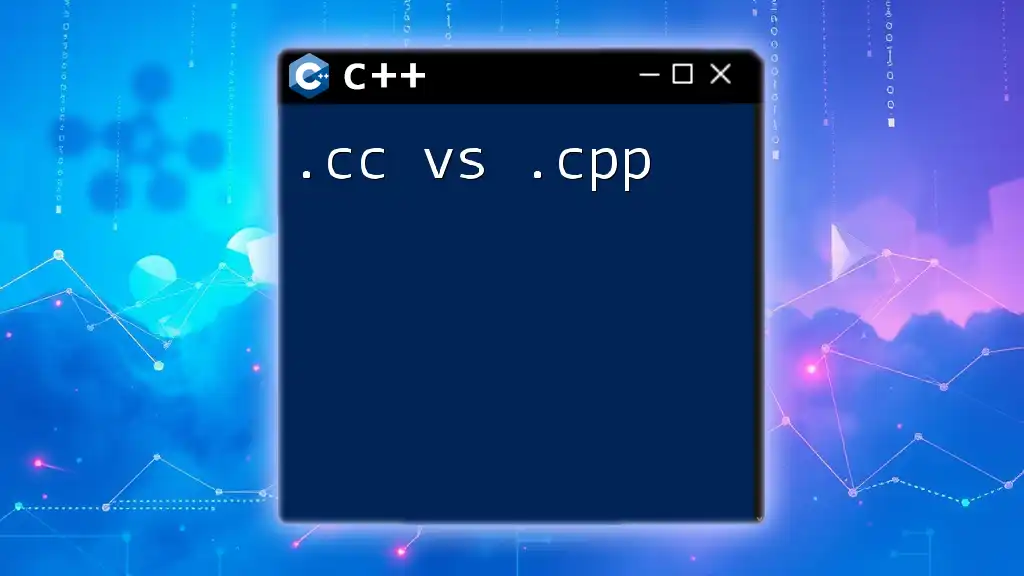
When to Use Each File Type
Best Practices for .cpp Files
You should create a .cpp file whenever you are defining the implementation of any functionalities. A good structure for your .cpp file includes:
- Including necessary header files
- Defining the `main()` function as an entry point
- Implementing functions declared in the corresponding .hpp files
Best Practices for .hpp Files
.hpp files are best suited for declaring functions, classes, and other constructs that need to be accessed from multiple source files. Key practices include:
- Using include guards to avoid multiple definitions.
- Keeping declarations concise and relevant to their purpose.
- Including only necessary headers to reduce dependencies.
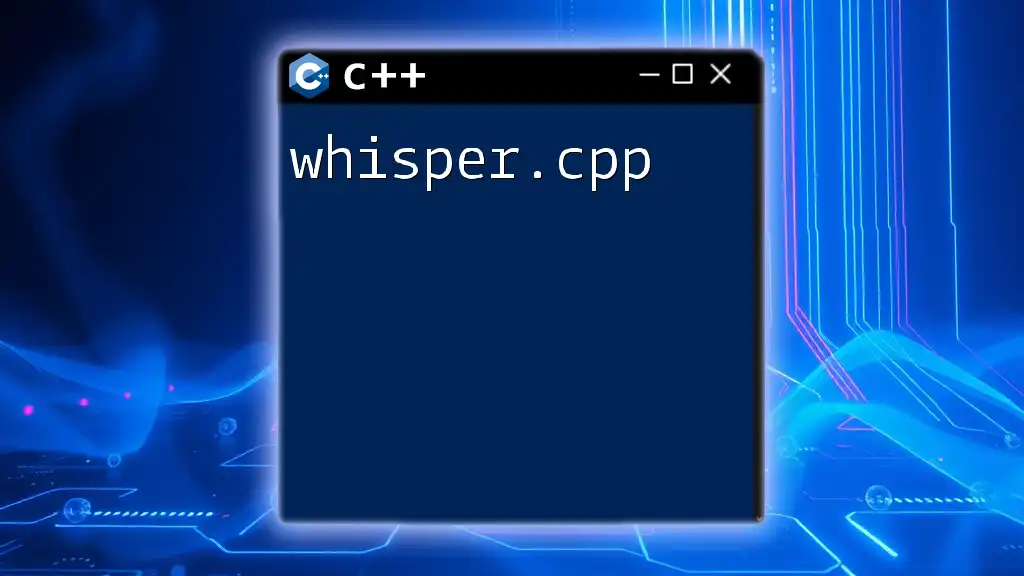
Example Project: Utilizing .hpp and .cpp
Project Overview
Let’s consider a simple C++ project: a calculator with basic arithmetic operations. This project will demonstrate how to utilize both .hpp and .cpp files effectively.
Step-by-step Guide
Step 1: Create the calculator.hpp file with declarations.
#ifndef CALCULATOR_HPP
#define CALCULATOR_HPP
int add(int a, int b);
int subtract(int a, int b);
#endif
Step 2: Create the corresponding calculator.cpp file with implementations.
#include "calculator.hpp"
int add(int a, int b) {
return a + b;
}
int subtract(int a, int b) {
return a - b;
}
Step 3: Write the main program (main.cpp) that uses the calculator methods.
#include <iostream>
#include "calculator.hpp"
int main() {
std::cout << "Sum: " << add(5, 3) << std::endl;
std::cout << "Difference: " << subtract(5, 3) << std::endl;
return 0;
}
This project clearly separates declarations from implementations, thus following best practices in C++ development.
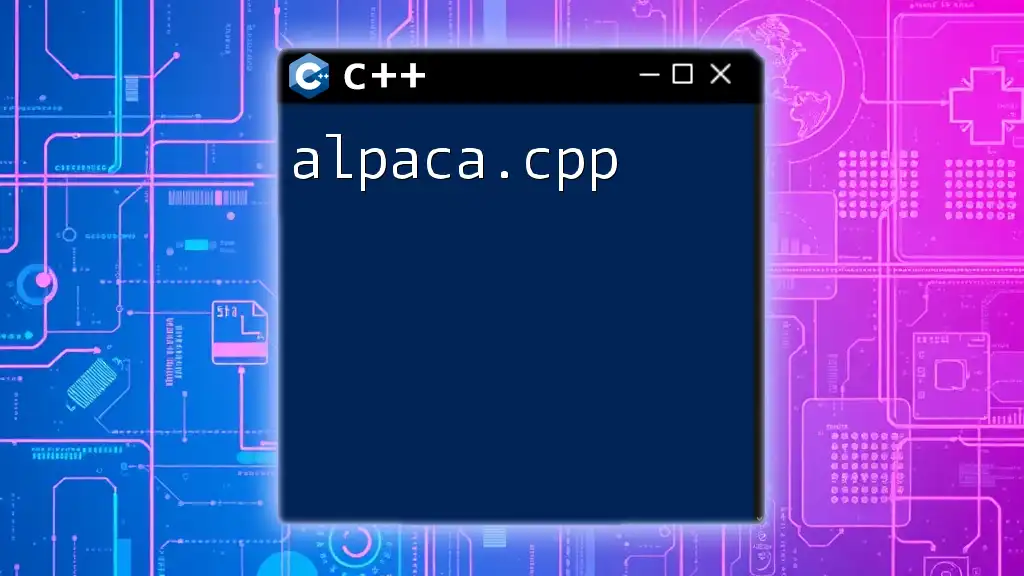
Common Mistakes to Avoid
Misunderstanding File Purpose
One common error is confusing the roles of .hpp and .cpp files. Remember that .hpp files should only contain declarations, while .cpp files should contain the implementation. This understanding is crucial to maintain modular and clean code.
Neglecting Include Guards
Many beginners overlook the importance of include guards in .hpp files. Without them, including a header file multiple times can lead to redefinition errors. Always ensure that your header files are properly guarded.
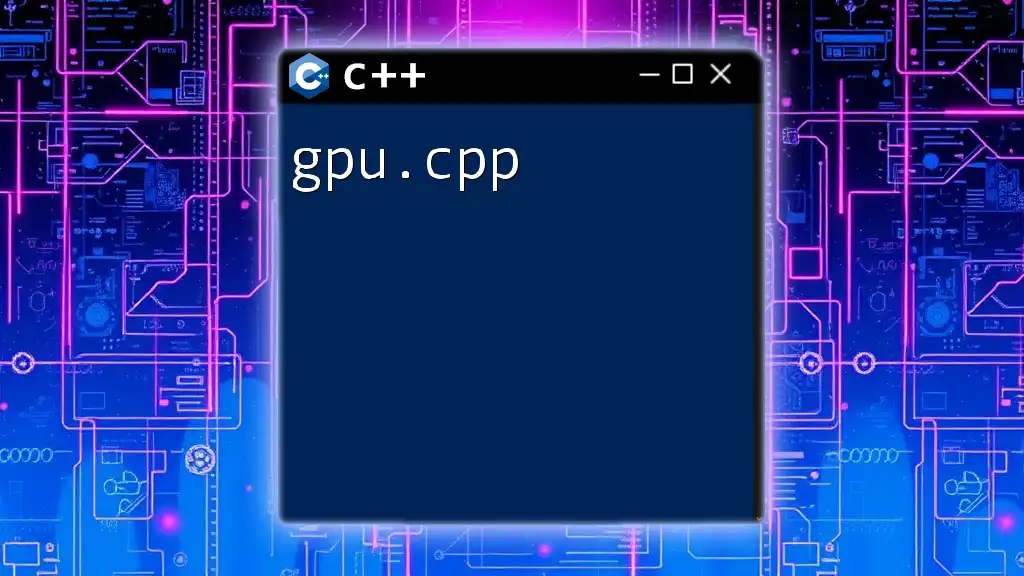
Conclusion
In conclusion, understanding the distinctions between .hpp vs .cpp is fundamental to mastering C++. .hpp files serve as declarations that facilitate modular programming, while .cpp files realize the logic required for execution. By implementing best practices, you can enhance both the maintainability and clarity of your C++ projects and elevate your programming skills.
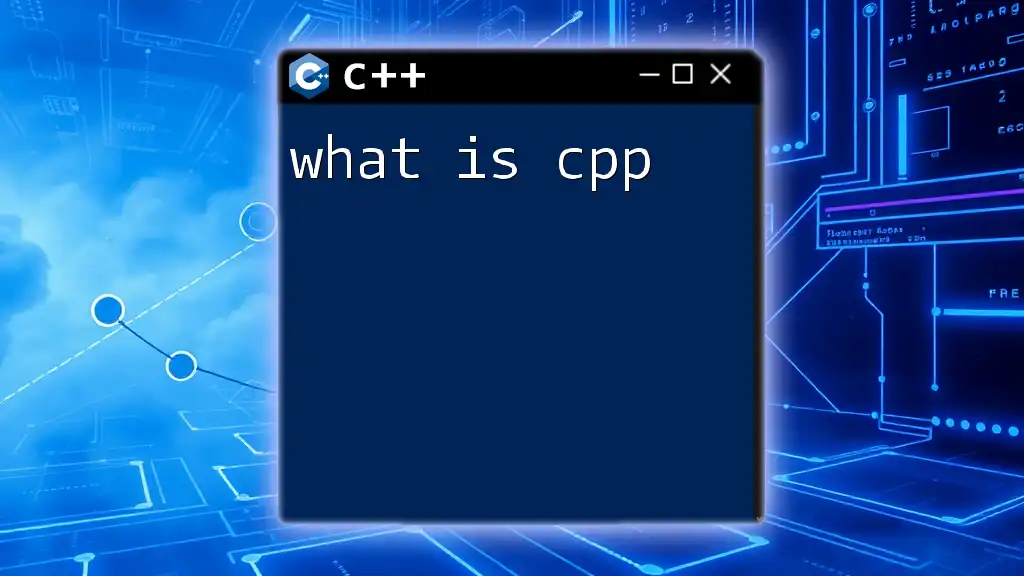
Call to Action
I encourage you to try working on your own sample projects using .hpp and .cpp files. Experimenting with these concepts in real scenarios will solidify your understanding and improve your C++ skills. For more resources and tutorials, stay tuned with us!