The "gpu.cpp" file typically refers to a C++ source file that may contain implementations for GPU programming, often utilizing libraries like CUDA to leverage the parallel processing capabilities of graphics cards.
Here's a simple code snippet demonstrating how to allocate and initialize memory on a GPU using CUDA in a C++ context:
#include <iostream>
#include <cuda.h>
__global__ void kernelFunction(float *d_out) {
int idx = threadIdx.x;
d_out[idx] = idx * 1.0f; // simple operation
}
int main() {
float *d_out;
cudaMalloc((void**)&d_out, 256 * sizeof(float)); // Allocate memory on GPU
kernelFunction<<<1, 256>>>(d_out); // Launch kernel
cudaFree(d_out); // Free GPU memory
return 0;
}
Understanding GPU Programming
What is GPU Programming?
GPU programming refers to the development of software that enables the use of Graphics Processing Units (GPUs) to perform computation tasks traditionally handled by CPUs. The primary purpose of this programming paradigm is to leverage the parallel processing capabilities of modern GPUs, allowing for significant enhancements in performance for suitable tasks, such as machine learning, scientific simulations, and graphic rendering.
Benefits of GPU Programming
The advantages of GPU programming are manifold. First and foremost, it provides substantial performance improvements due to the ability of GPUs to execute thousands of threads simultaneously in parallel. This capability makes GPUs particularly well-suited for tasks that can be divided into smaller, concurrent operations.
Common applications include:
- Machine Learning: Speeding up training times for models.
- Scientific Computation: Executing large simulations faster.
- Graphics and Visualization: Rendering images and videos with high efficiency.
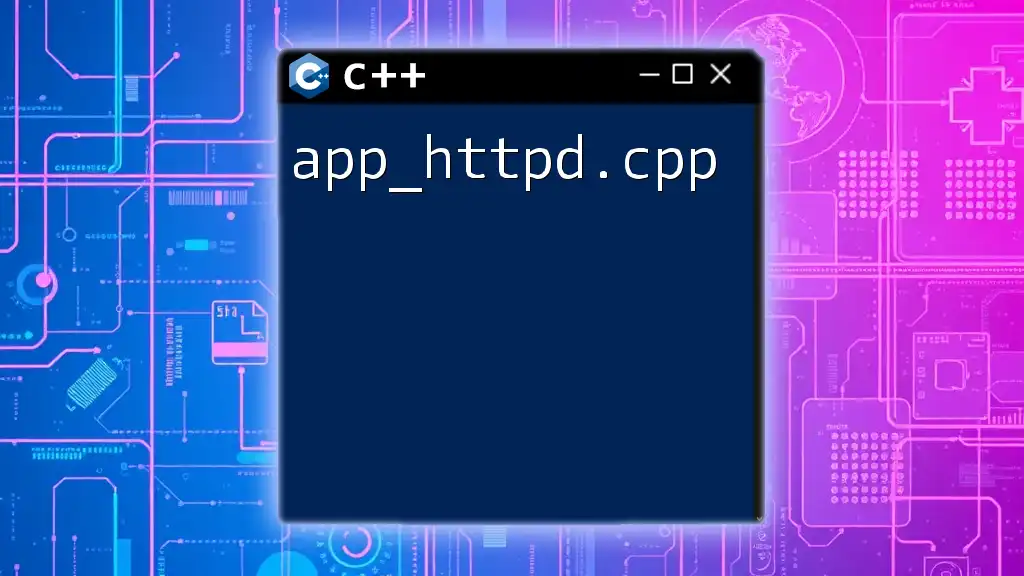
Exploring `gpu.cpp`
What is `gpu.cpp`?
`gpu.cpp` refers to a specific file extension that typically contains C++ code tailored for GPU programming. This file often serves as a primary source for CUDA (Compute Unified Device Architecture) code, which allows developers to write programs that utilize NVIDIA GPU hardware.
In the broader context, `gpu.cpp` can be used within various environments that support GPU operations. It functions as a bridge between the software and the GPU hardware, making it essential for developers aiming to harness GPU power in their applications.
Structure of `gpu.cpp`
The structure of a typical `gpu.cpp` file includes several key components:
- Function Declarations: Defining the individual computational tasks that the GPU will execute.
- Variable Definitions: Establishing the data types and variables needed for the operations.
- Libraries and Dependencies: Including necessary headers that provide GPU functionalities, such as `cuda_runtime.h` for CUDA applications.
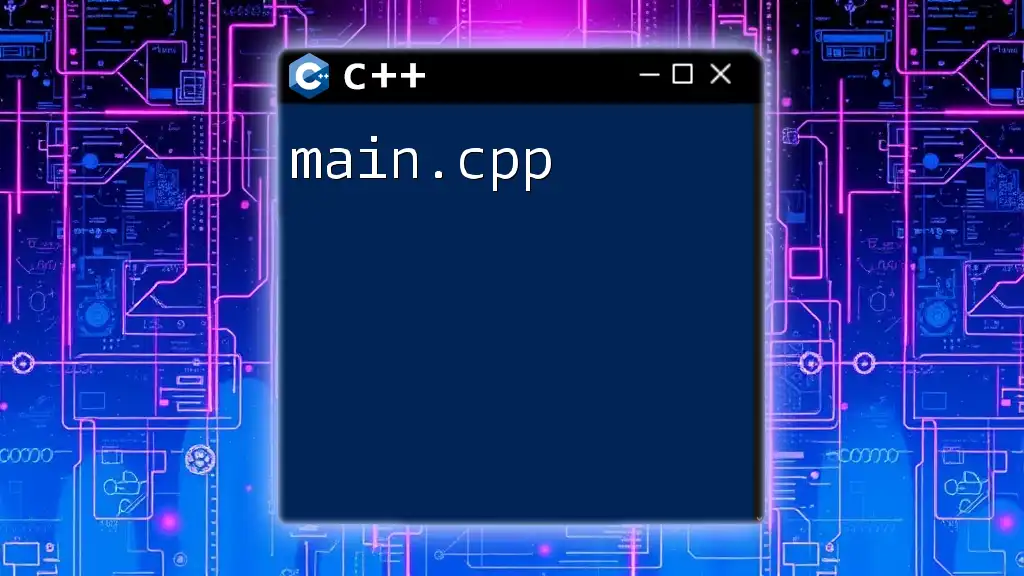
Setting Up Your Development Environment
Required Software and Tools
To successfully launch `gpu.cpp` applications, specific software and tools must be installed. Here is how you can set up your environment:
- Operating Systems: Linux and Windows are commonly used; however, ensure your system meets the hardware requirements for GPU programming.
- C++ Compilers: Use GCC or MSVC with NVIDIA's CUDA toolkit installed to enable GPU programming capabilities.
- Integrated Development Environments (IDEs): Some popular choices include Visual Studio, CLion, and Eclipse with CUDA support.
Getting Started with Your First `gpu.cpp`
Writing your first `gpu.cpp` file can be an exciting venture. Here’s a basic guide to get you started:
- Create a new file named `gpu.cpp`.
- Include necessary libraries, like `cuda_runtime.h`.
- Define your kernel function, which contains the code that will run on the GPU.
- Launch the kernel from your `main` function.
Here’s a simple example:
#include <iostream>
#include <cuda_runtime.h>
__global__ void simpleKernel() {
printf("Hello from GPU!\n");
}
int main() {
simpleKernel<<<1, 10>>>();
cudaDeviceSynchronize();
return 0;
}
In this example:
- The `global` keyword indicates that `simpleKernel` is a function that will be executed on the GPU.
- The `<<<1, 10>>>` specifies that we are launching one block of 10 threads.
- The `cudaDeviceSynchronize()` function ensures that the program waits for the GPU to finish executing before proceeding.
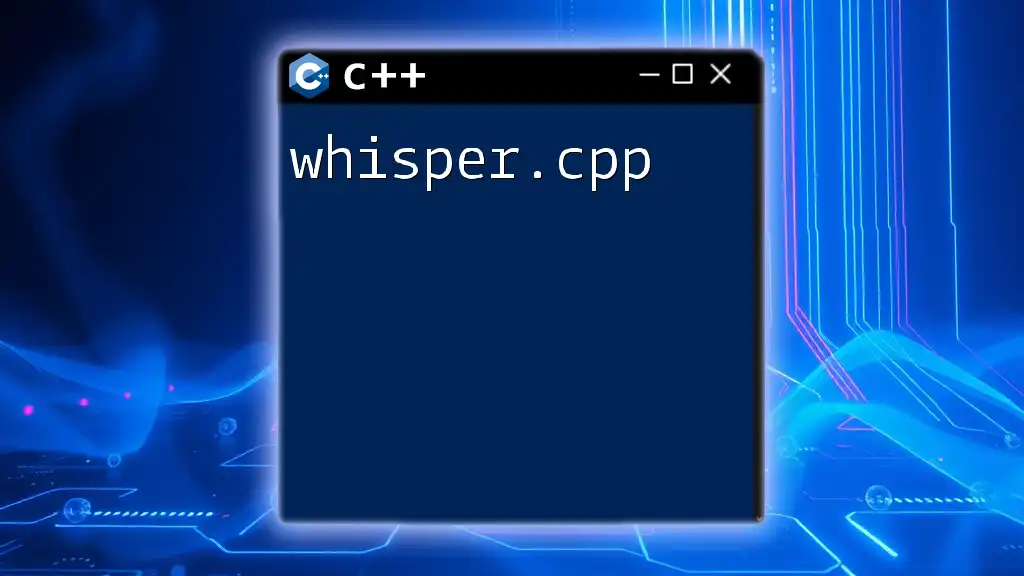
Core Concepts of `gpu.cpp`
Memory Management in GPU
Understanding memory management is crucial for effective GPU programming. GPU memory is split between:
- Device Memory: Memory located on the GPU. It is faster but needs to be explicitly managed by the programmer.
- Host Memory: Traditional RAM located on the CPU which is slower to access for GPU operations.
To utilize GPU memory effectively, developers must allocate and deallocate memory using functions like `cudaMalloc` and `cudaFree`.
Data Transfer Between Host and Device
Data transfer between the host and device is one of the crucial aspects of GPU programming. The process involves moving data from the main RAM to the GPU’s device memory and vice versa.
Here’s an example of how to copy data from host to device:
float *d_a;
cudaMalloc(&d_a, size); // Allocate device memory
cudaMemcpy(d_a, h_a, size, cudaMemcpyHostToDevice); // Copy data from host to device
Understanding the different types of memory transfer (`cudaMemcpyHostToDevice` and `cudaMemcpyDeviceToHost`) is essential for efficient data management.
Kernel Functions
Kernel functions are the backbone of GPU programming. They define the operations executed on the GPU. Writing and launching kernel functions is straightforward:
__global__ void addKernel(int *c, const int *a, const int *b) {
int idx = threadIdx.x;
c[idx] = a[idx] + b[idx];
}
In this kernel, each thread computes a sum of two arrays. The `threadIdx` built-in variable provides the unique index of the thread currently executing.
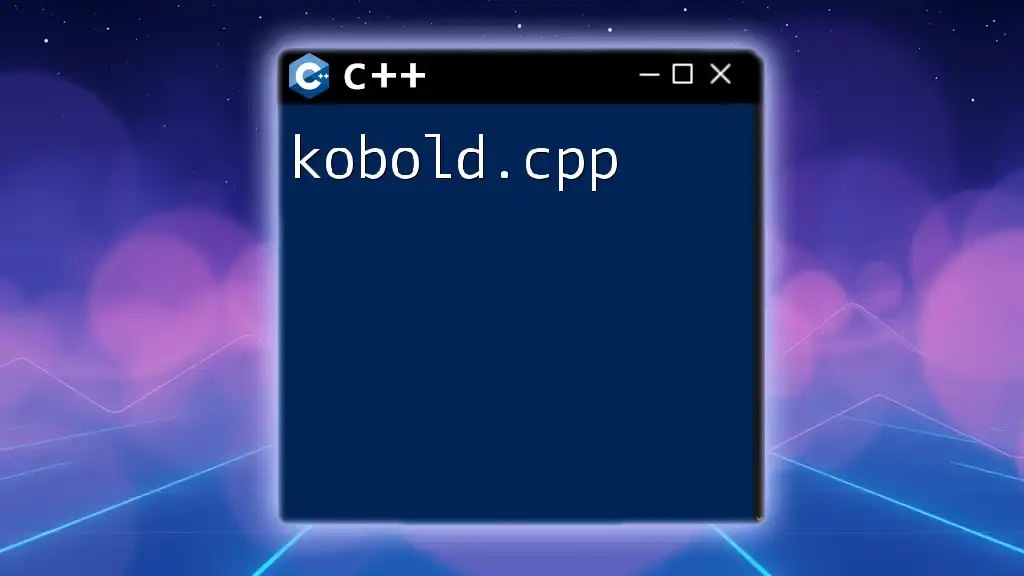
Advanced Topics in `gpu.cpp`
Optimization Techniques
Optimizing GPU code can yield significant performance improvements. Some common optimization strategies include:
- Memory Coalescing: Ensuring that memory accesses from threads are contiguous, improving memory throughput.
- Loop Unrolling: Increasing performance by reducing the overhead of branching in loops.
- Shared Memory Usage: Leveraging fast shared memory for frequently accessed data within blocks.
Profiling and Debugging
Profiling tools are available to help analyze and improve the performance of GPU applications. NVIDIA’s Nsight Systems or Nsight Compute helps to visualize application performance and identify bottlenecks.
Debugging GPU code can be more challenging than CPU code due to the parallel nature of execution. However, tools like CUDA-GDB can aid developers in stepping through code, examining variables, and identifying issues.
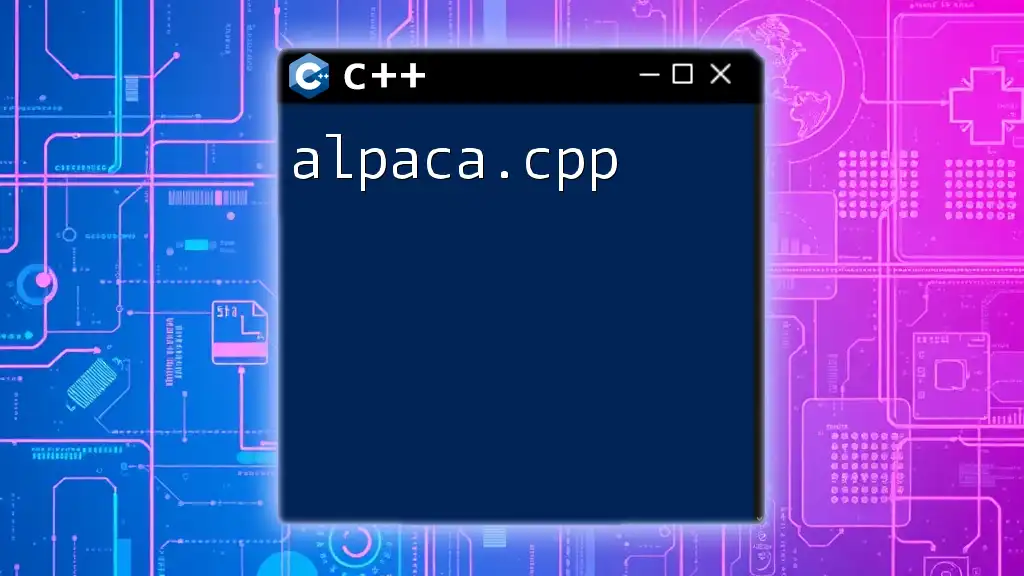
Best Practices for Writing Efficient `gpu.cpp`
Code Readability and Maintenance
Writing clear and maintainable code is critical in ensuring long-term project success. This includes:
- Using descriptive variable names.
- Breaking complex kernels into smaller, more manageable functions.
- Adding comments to clarify the purpose and behavior of code blocks.
Error Handling
Dealing with errors effectively is vital in GPU programming. Common errors include out-of-bounds memory accesses and CUDA function call failures. Checking returned error codes after CUDA API calls is a good practice:
cudaError_t err = cudaMemcpy(d_a, h_a, size, cudaMemcpyHostToDevice);
if (err != cudaSuccess) {
std::cerr << "CUDA error: " << cudaGetErrorString(err) << std::endl;
}
Being vigilant about error checking can save time during the debugging process.
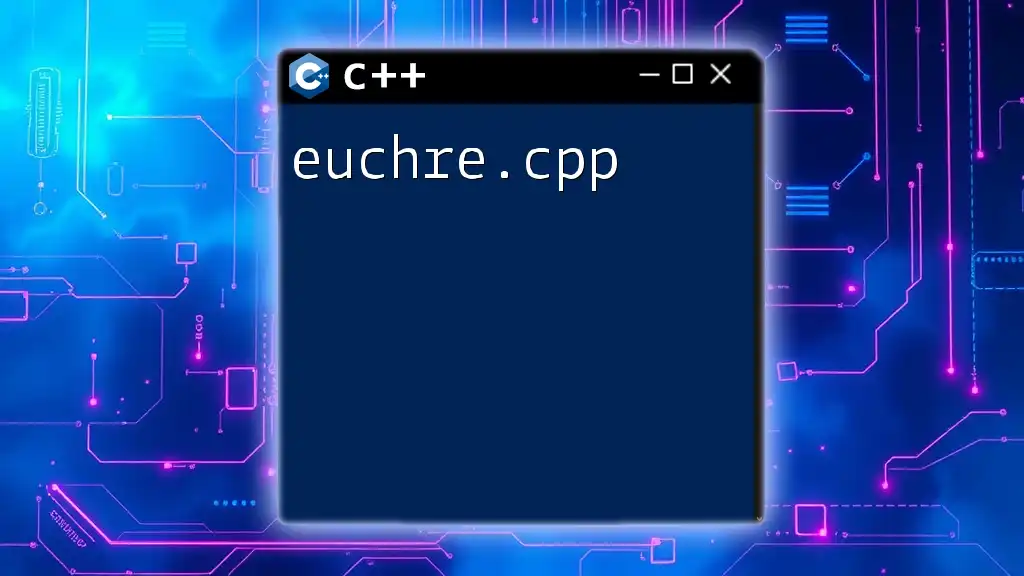
Conclusion
This guide has provided a comprehensive overview of `gpu.cpp` and its role in GPU programming. From the initial understanding of GPU versus CPU capabilities to advanced optimization techniques, there is much to explore and implement. Now, with the foundational knowledge presented here, you are encouraged to experiment with `gpu.cpp`, harness its potential, and push the boundaries of what is achievable with GPU computing.
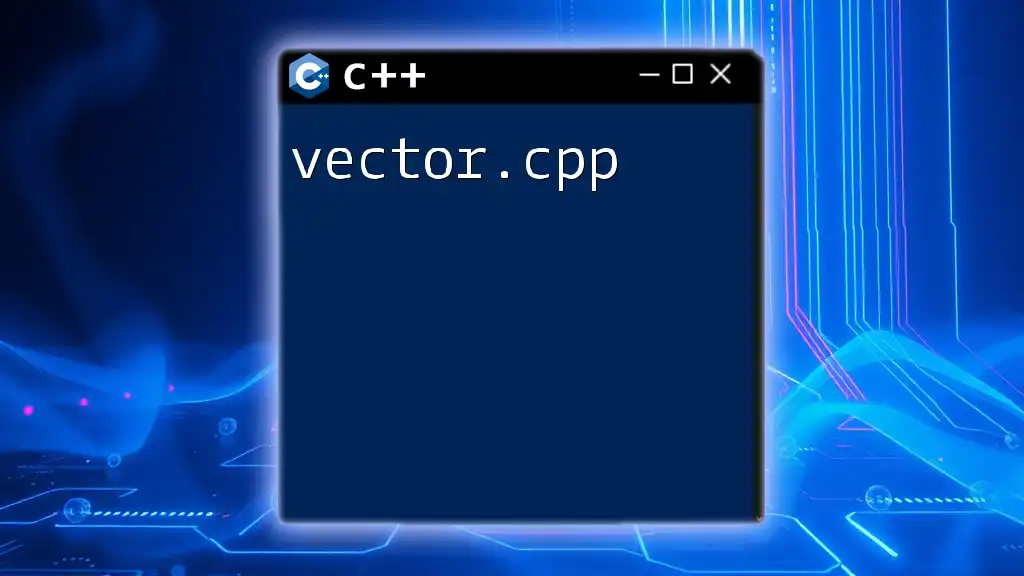
Additional Resources
To further your learning in GPU programming, explore recommended books, websites, forums, and tutorials that can provide deeper insights and hands-on experiences. Engaging with the community can also help you overcome challenges and share knowledge with other enthusiasts.