"TCP CPP" refers to the utilization of C++ programming to implement TCP (Transmission Control Protocol) communication, enabling reliable data transmission over networks. Here's a simple code snippet demonstrating a basic TCP client connection using C++:
#include <iostream>
#include <string>
#include <sys/socket.h>
#include <arpa/inet.h>
#include <unistd.h>
int main() {
int sock = socket(AF_INET, SOCK_STREAM, 0);
struct sockaddr_in server_addr;
server_addr.sin_family = AF_INET;
server_addr.sin_port = htons(8080);
inet_pton(AF_INET, "127.0.0.1", &server_addr.sin_addr);
if (connect(sock, (struct sockaddr *)&server_addr, sizeof(server_addr)) != -1) {
std::cout << "Connected to server!" << std::endl;
} else {
std::cerr << "Connection failed!" << std::endl;
}
close(sock);
return 0;
}
What is TCP?
Transmission Control Protocol (TCP) is a fundamental communication protocol used across the Internet. Unlike its counterpart, UDP (User Datagram Protocol), TCP is connection-oriented, meaning it establishes a reliable connection between the sender and receiver before data transmission begins. Key characteristics of TCP include:
- Reliability: TCP ensures that data sent from the sender arrives at the receiver without errors and in the correct order.
- Flow Control: TCP manages the data flow between the sender and receiver to prevent overwhelming the receiver with too much data at once.
- Congestion Control: TCP adjusts the data transmission rate based on network conditions to avoid congestion.
Understanding the role of TCP in networking is vital for efficient C++ programming, especially when creating networked applications.
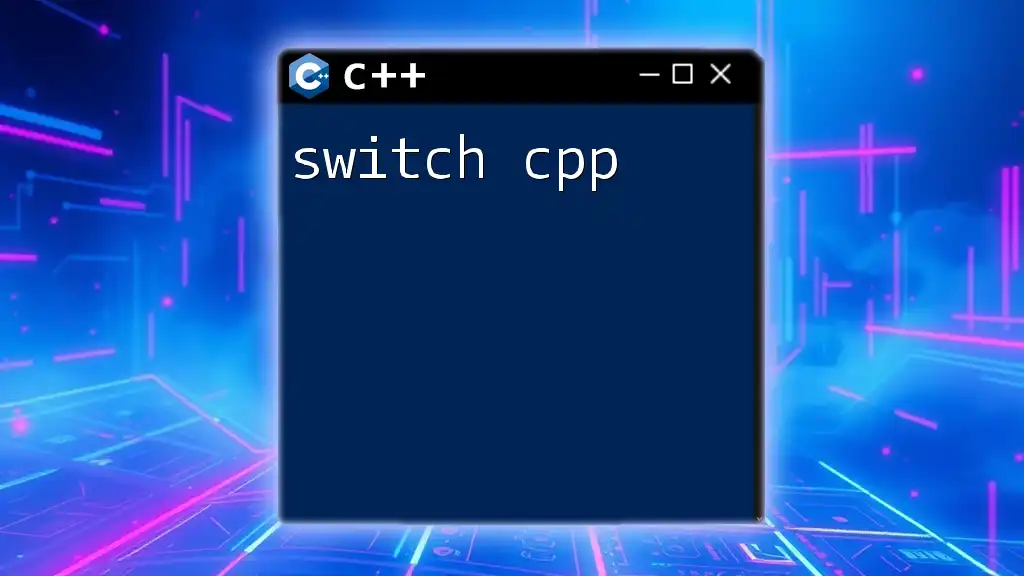
Overview of C++ in Networking
C++ is a powerful language for network programming due to its performance and flexibility. Libraries such as `<sys/socket.h>`, `<netinet/in.h>`, `<arpa/inet.h>`, and `<unistd.h>` provide necessary APIs for building TCP client and server applications. In C++, you can take advantage of object-oriented programming principles to create modular and reusable networking components.
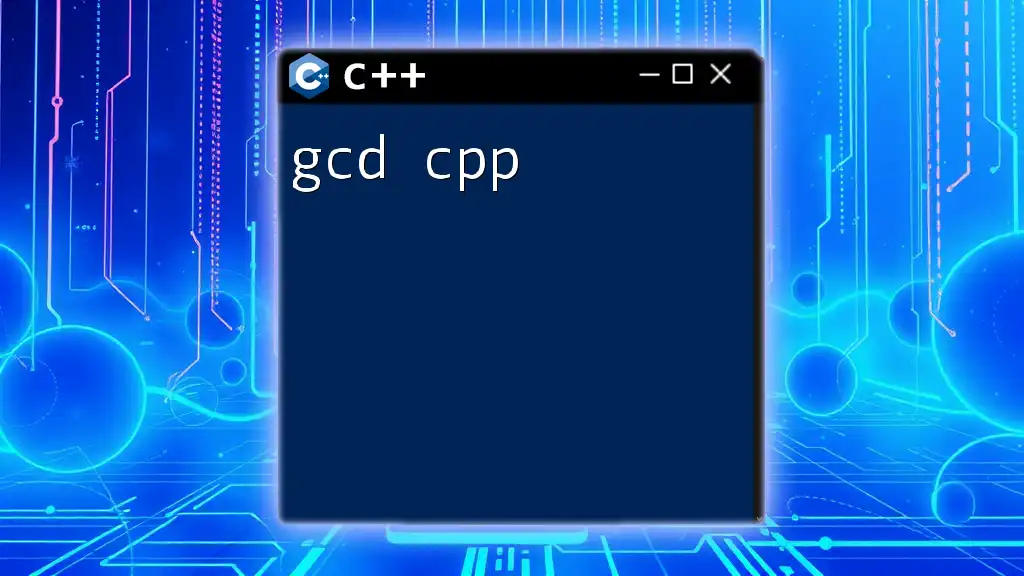
TCP vs. UDP
When working with network protocols, it’s essential to understand the differences between TCP and UDP:
- TCP is connection-oriented, meaning it establishes a connection and guarantees delivery of packets. It is best suited for applications that require reliability, such as web browsing, file transfers, and email.
- UDP, on the other hand, is connectionless and does not guarantee delivery, order, or error checking. This makes it suitable for applications where speed is crucial, like video streaming or online gaming.
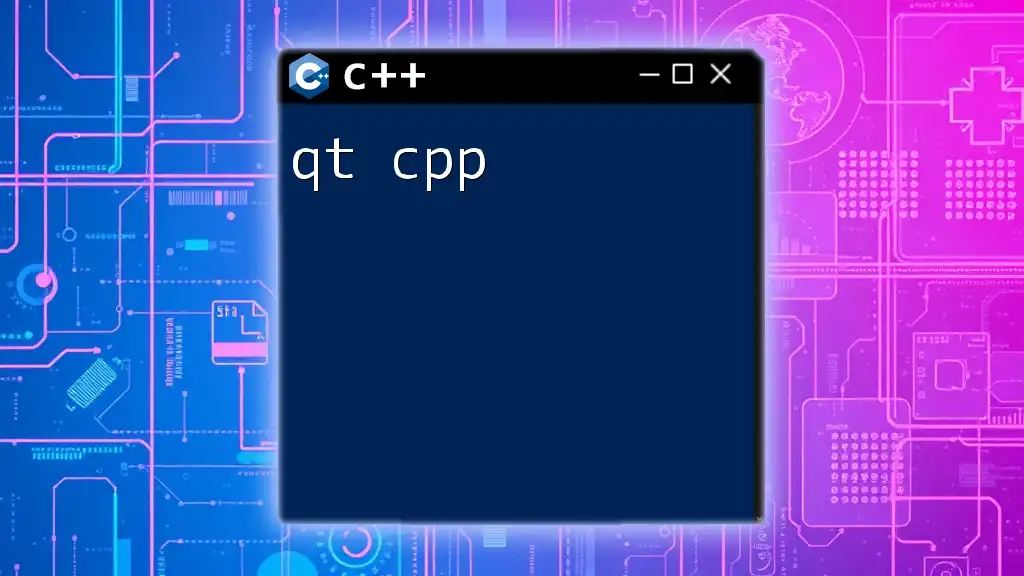
TCP Connection Lifecycle
The lifecycle of a TCP connection can be broken down into three main stages:
-
Connection Establishment: This involves the three-way handshake, where the client and server exchange SYN and ACK packets to establish a connection.
-
Data Transfer: Once the connection is established, data can be transmitted in both directions until one end signals that it has completed sending data.
-
Connection Termination: Finally, either side can terminate the connection through a process that ensures all data has been transmitted and acknowledged.
Understanding this lifecycle is imperative for implementing robust TCP C++ applications.
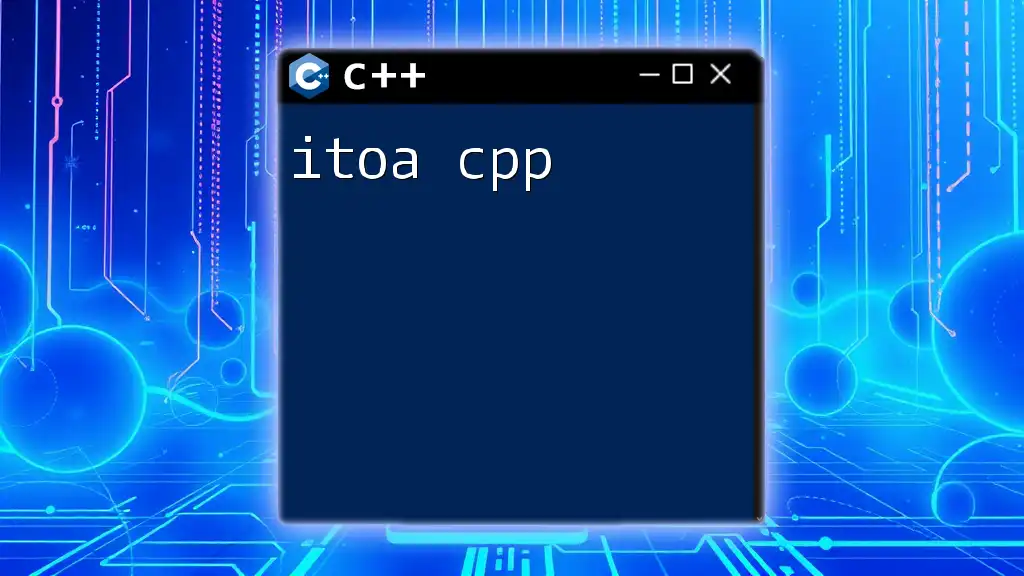
Setting Up Your C++ Environment for TCP Programming
Necessary Libraries
When programming in C++, you’ll primarily use the following libraries for TCP communication:
- `<sys/socket.h>`: Provides definitions for sockets.
- `<netinet/in.h>`: Contains constants and structures needed for Internet domain addresses.
- `<arpa/inet.h>`: Allows conversions between binary and text representations of IP addresses.
- `<unistd.h>`: Provides access to the POSIX operating system API, including methods for closing sockets.
To link these libraries in your project, ensure your compiler is set up accordingly.
Choosing a C++ Compiler
For TCP programming, choosing a reliable C++ compiler is crucial. Some popular options are:
- GCC (GNU Compiler Collection): Widely used, especially in Linux environments.
- Microsoft Visual C++: Ideal for Windows applications.
- Clang: Known for its excellent performance and robustness.
Ensure that your compiler supports the necessary libraries to seamlessly build your TCP applications.
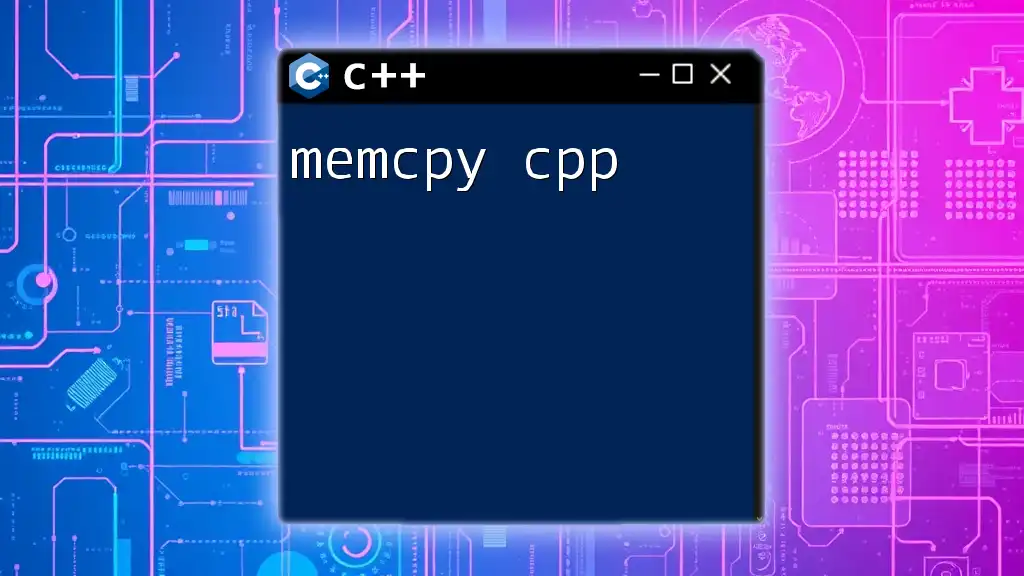
Writing a Simple TCP Client in C++
Creating the Client Socket
To initiate a connection to a TCP server, you first need to create a socket. Here's a simple TCP client socket creation code snippet:
#include <sys/socket.h>
#include <netinet/in.h>
#include <arpa/inet.h>
#include <unistd.h>
#include <iostream>
int main() {
int client_socket = socket(AF_INET, SOCK_STREAM, 0);
if (client_socket < 0) {
std::cerr << "Error creating socket." << std::endl;
return -1;
}
// Additional code will go here
}
The `socket()` function takes three parameters: the address family (`AF_INET` for IPv4), the socket type (`SOCK_STREAM` for TCP connections), and the protocol (0 for IP).
Connecting to the Server
After successfully creating the socket, the next step is to connect to the server. Here's how this can be done:
struct sockaddr_in server_address;
server_address.sin_family = AF_INET;
server_address.sin_port = htons(8080); // Port number
inet_pton(AF_INET, "127.0.0.1", &server_address.sin_addr); // IP address
if (connect(client_socket, (struct sockaddr*)&server_address, sizeof(server_address)) < 0) {
std::cerr << "Error connecting to server." << std::endl;
return -1;
}
In this example, `htons()` converts the port number from host byte order to network byte order. The `inet_pton()` function converts the IP address provided in string format to a binary format understood by the network.
Sending and Receiving Data
Once connected, send and receive data using the `send()` and `recv()` functions:
const char* message = "Hello, Server!";
send(client_socket, message, strlen(message), 0);
char buffer[1024];
int bytes_received = recv(client_socket, buffer, sizeof(buffer), 0);
if (bytes_received > 0) {
buffer[bytes_received] = '\0';
std::cout << "Message from server: " << buffer << std::endl;
}
The `send()` function sends the message to the server, while `recv()` receives data from the server. Ensure to handle the buffer correctly by terminating the string after reading.
Closing the Connection
Finally, gracefully terminate the connection with the following code:
close(client_socket);
This step ensures that all resources related to the socket are freed, preventing memory leaks or resource exhaustion.
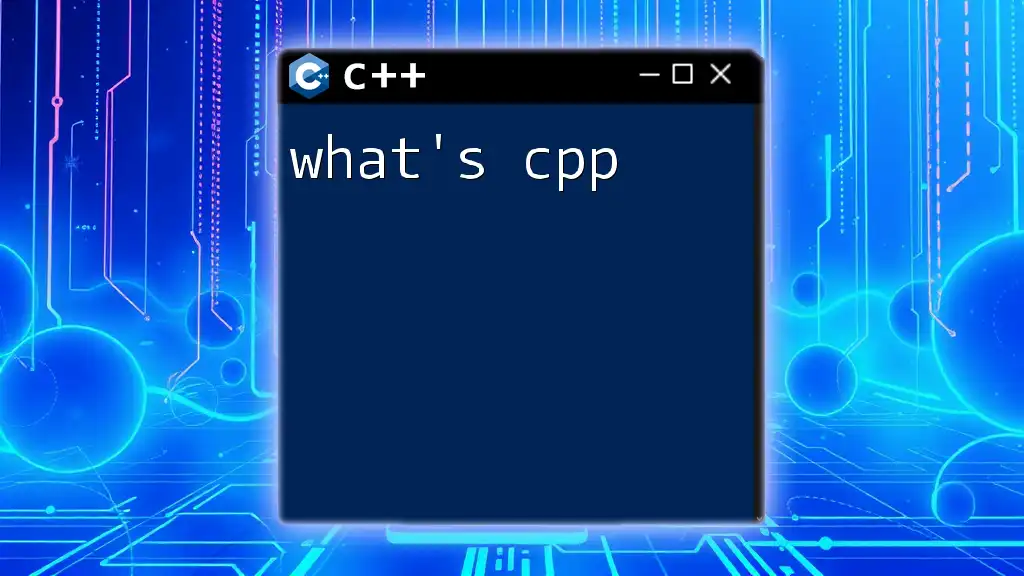
Writing a Simple TCP Server in C++
Creating the Server Socket
Creating a TCP server involves listening for incoming connections. Here’s how you can create a server socket:
int server_socket = socket(AF_INET, SOCK_STREAM, 0);
if (server_socket < 0) {
std::cerr << "Error creating socket." << std::endl;
return -1;
}
Binding to an Address
Once the socket is created, bind it to a specific IP address and port:
struct sockaddr_in server_address;
server_address.sin_family = AF_INET;
server_address.sin_port = htons(8080);
server_address.sin_addr.s_addr = INADDR_ANY; // Bind to any available interface
if (bind(server_socket, (struct sockaddr*)&server_address, sizeof(server_address)) < 0) {
std::cerr << "Error binding to port." << std::endl;
return -1;
}
The `bind()` function connects the socket to the specified address and port, allowing it to listen for incoming connections.
Listening for Connections
To listen for incoming client connections, you can use the `listen()` function:
listen(server_socket, 5); // 5 is the maximum length of the queue for pending connections
Accepting Client Connections
Once your server is listening, you can accept a client connection:
int client_socket = accept(server_socket, nullptr, nullptr);
if (client_socket < 0) {
std::cerr << "Error accepting connection." << std::endl;
return -1;
}
The `accept()` function blocks until a client connects, returning a new socket to communicate with that client.
Handling Client Communication
You can handle data exchange with the connected client as shown below:
char buffer[1024];
int bytes_received = recv(client_socket, buffer, sizeof(buffer), 0);
if (bytes_received > 0) {
buffer[bytes_received] = '\0'; // Null-terminate the string
std::cout << "Message from client: " << buffer << std::endl;
const char* response = "Hello from server!";
send(client_socket, response, strlen(response), 0);
}
Closing the Connection
As with the client, make sure to close the server and client sockets:
close(client_socket);
close(server_socket);
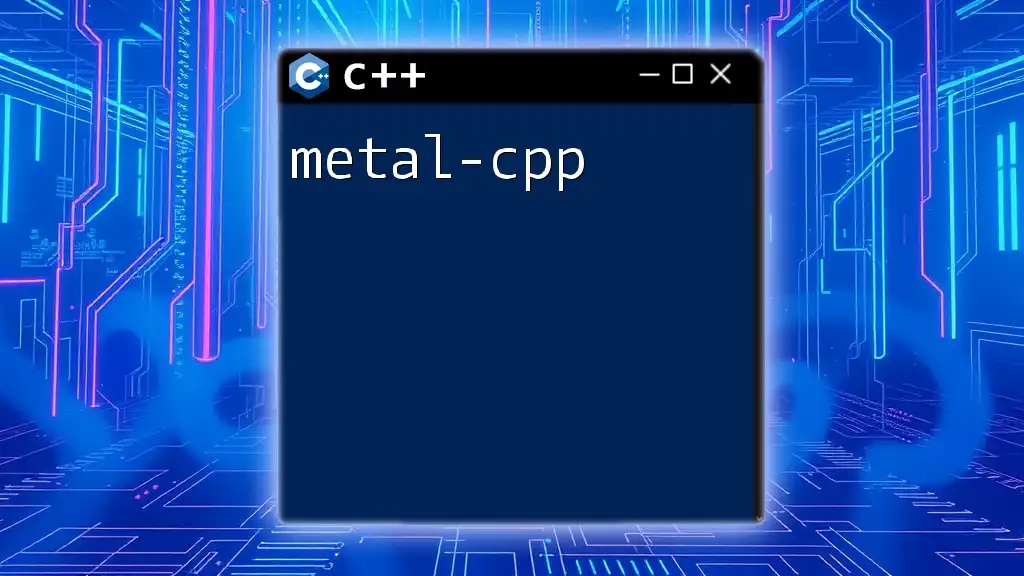
Error Handling in TCP C++
Common Socket Errors
When working with sockets, errors can occur at various stages. Here are some common issues to look out for:
- Socket Creation Failure: When creating a socket, check if the return value is less than 0.
- Connection Errors: Errors during the `connect()` or `accept()` functions can indicate issues with your server setup or network configuration.
Implementing Error Handling Strategies
To improve robustness, implement error handling using `errno` to retrieve error codes and `strerror()` to convert them into readable messages:
#include <cstring> // For strerror
if (connect(client_socket, ... ) < 0) {
std::cerr << "Connect error: " << strerror(errno) << std::endl;
}
This approach helps pinpoint issues when they arise.
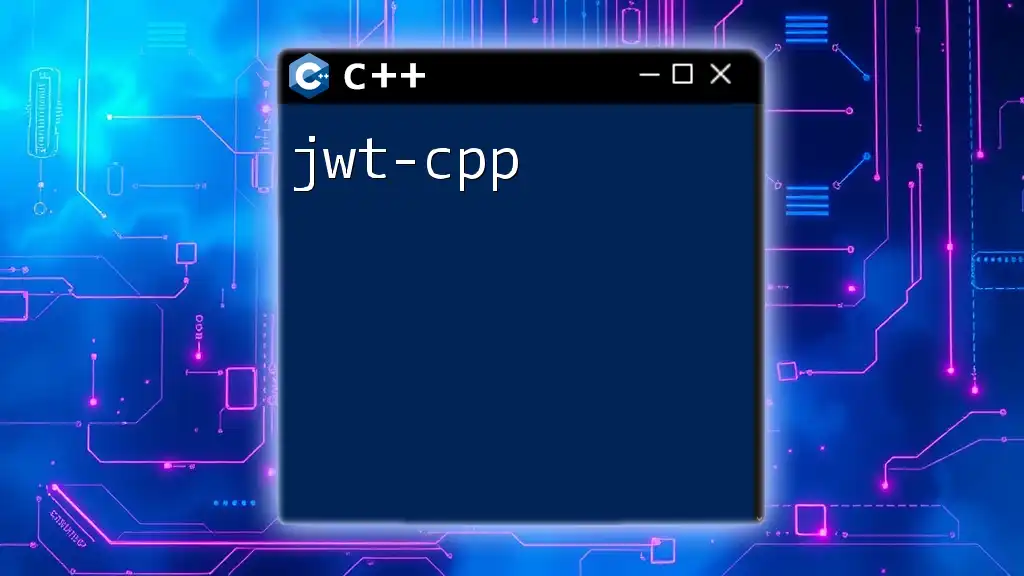
Advanced Topics in TCP C++ Programming
Asynchronous TCP Socket Programming
For more advanced applications, consider using asynchronous socket programming techniques. By employing non-blocking sockets, you can manage multiple connections without blocking calls, improving the responsiveness of your application.
Using functions such as `select()` or `poll()`, you can monitor multiple file descriptors to see if they are ready for I/O operations.
TCP Performance Optimization Techniques
Optimizing TCP applications can lead to significant performance gains. Consider the following techniques:
-
Nagle’s Algorithm: Typically enabled by default, it groups small packets together, reducing network congestion. However, for interactive applications, it might be beneficial to disable it.
-
Window Scaling: For high-latency networks, TCP window scaling allows for better utilization of bandwidth by increasing the window size.
Secure TCP Communication
Security is crucial in networking. Implementing SSL/TLS with C++ can help you secure your TCP connections. Libraries such as OpenSSL or Boost.Beast assist with integrating these security measures into your applications.
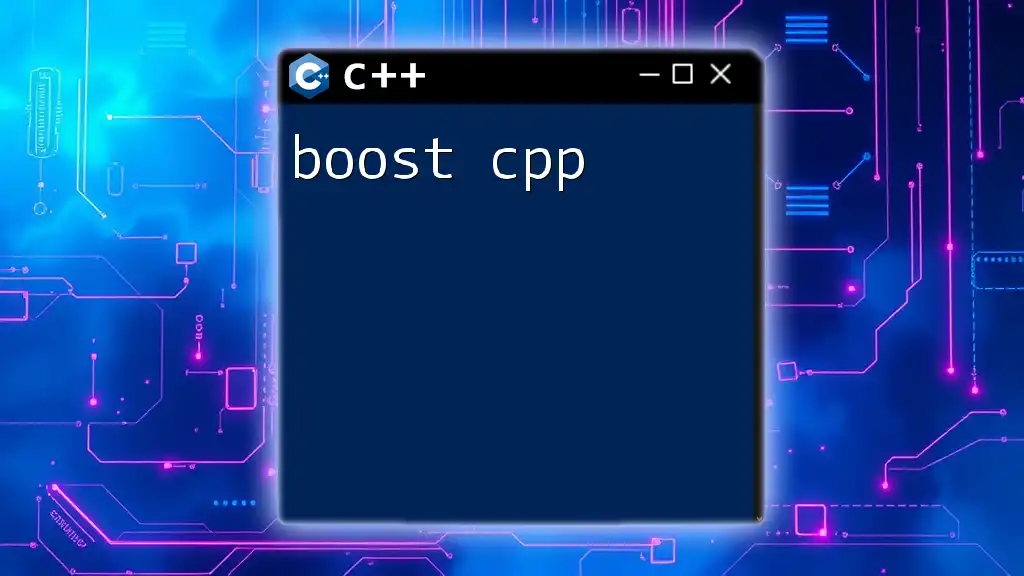
Real-world Examples and Use Cases
Chat Application Example
A simple TCP chat application can illustrate the practical use of TCP in networking. The server listens for incoming connections, and clients can send and receive messages to and from each other seamlessly.
File Transfer Protocol
Implementing a basic file transfer application using TCP involves creating a client-server model where clients can request file downloads from the server. This showcases the TCP reliability and flow control capabilities, ensuring complete and accurate file transfers.
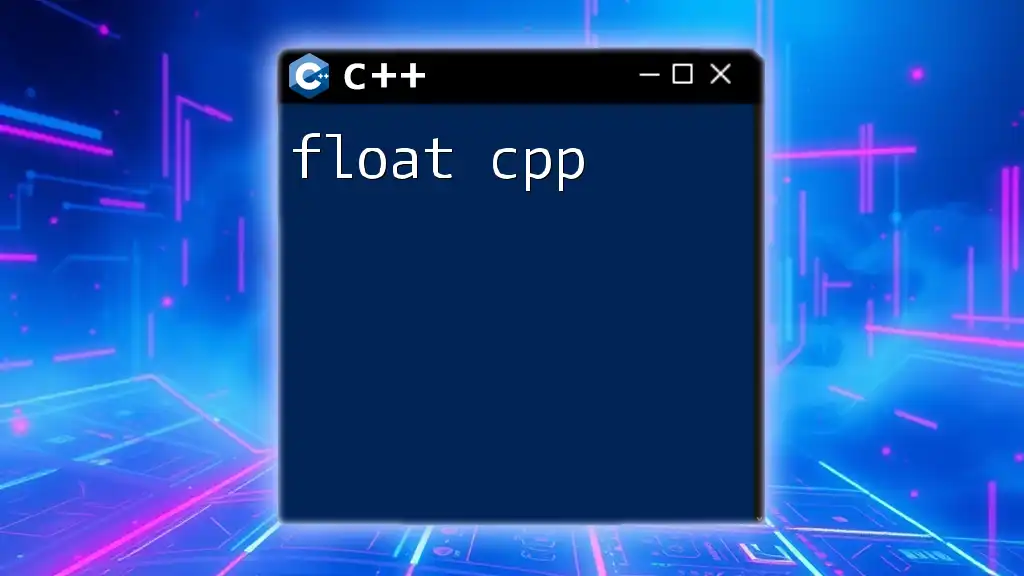
Best Practices for TCP C++ Programming
Code Organization and Modularity
Writing clean and modular code is essential. Organize your program into distinct functions or classes to separate concerns, making it easier to maintain or extend.
Testing and Debugging Techniques
Utilize tools like `gdb` for debugging your C++ applications. Additionally, comprehensive unit tests can help ensure the reliability of your TCP implementations.
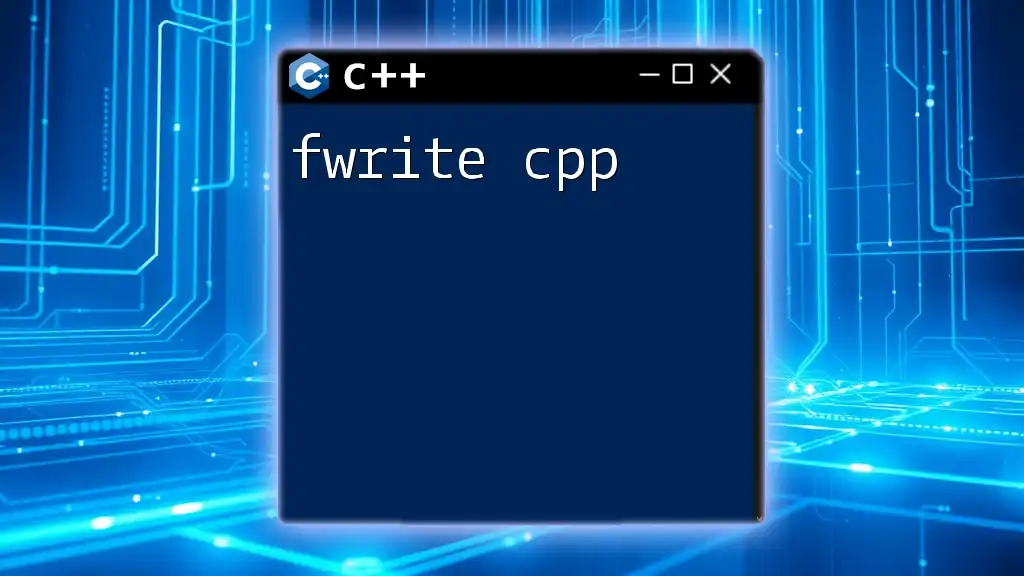
Conclusion
In this guide, we covered the fundamentals of TCP programming in C++, including socket creation, connecting to servers, handling data transfer, and advanced topics like performance optimization and secure communications. Understanding these concepts is key for anyone looking to build robust networked applications.
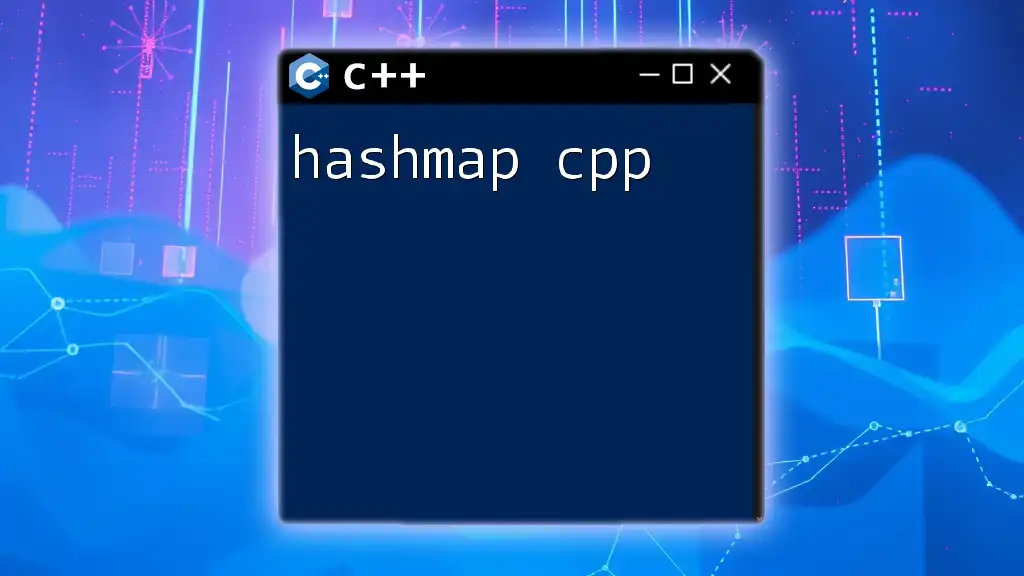
Additional Resources
To further enhance your understanding, explore online tutorials, official documentation, and recommended readings about C++ networking. Engaging with communities dedicated to C++ programming can also provide valuable insights and support as you continue your journey into TCP programming.