The `switch` statement in C++ allows you to execute different blocks of code based on the value of a variable, making it a cleaner alternative to multiple `if-else` statements.
#include <iostream>
using namespace std;
int main() {
int day = 3;
switch (day) {
case 1: cout << "Monday"; break;
case 2: cout << "Tuesday"; break;
case 3: cout << "Wednesday"; break;
case 4: cout << "Thursday"; break;
case 5: cout << "Friday"; break;
default: cout << "Weekend"; break;
}
return 0;
}
Understanding the Switch Syntax in C++
The switch statement is a fundamental control structure in C++. It allows developers to select one of many code blocks to execute based on the value of an expression. This structure enhances code readability and efficiency, particularly when dealing with multiple conditions.
The basic structure of a switch statement is as follows:
switch (expression) {
case constant1:
// Code to execute if expression equals constant1
break;
case constant2:
// Code to execute if expression equals constant2
break;
default:
// Code to execute if expression doesn't match any case
}
In this syntax:
- Switch Expression: The expression evaluated once, determining which case to execute.
- Case Labels: Each `case` is followed by a specific constant value. When the switch expression matches a case label, the corresponding block of code executes.
- Break Statements: These statements terminate the switch case. If omitted, execution will "fall through" to the next case, which is often not the desired behavior.
- Default Case: The `default` case executes if none of the other cases match, providing a catch-all scenario.
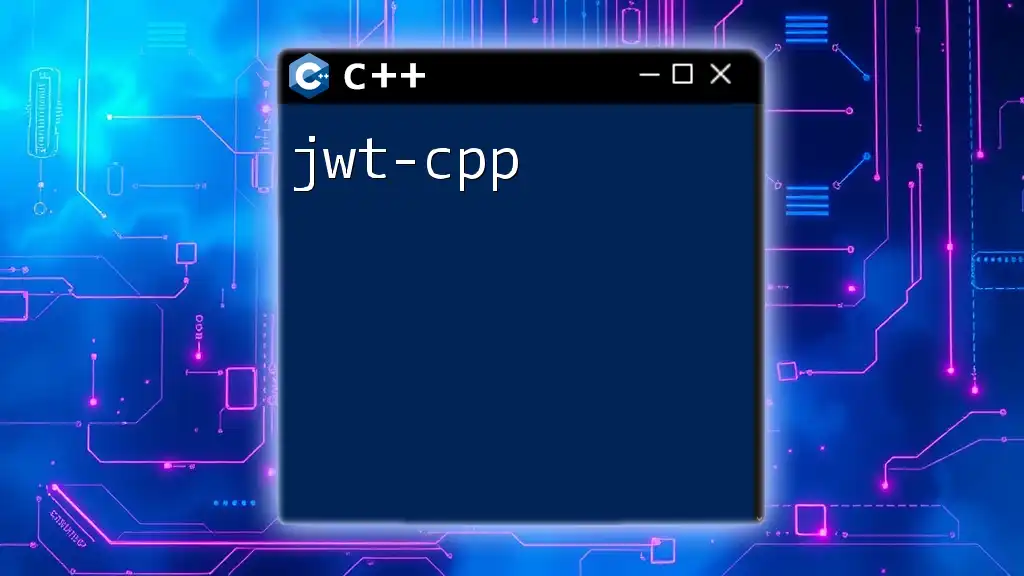
Detailed Breakdown of Switch Statement Components
Switch Expression
The switch expression can be of integral types like `int`, `char`, or `enum`. It’s critical to understand that the expression is evaluated only once when entering the switch. This characteristic distinguishes the switch statement from ``if-else`` constructs, where conditions may be evaluated multiple times.
int value = 2;
switch (value) {
// ...
}
Case Labels in C++
Case labels define the specific values that the switch expression may match. Each case ends with a `break` statement to prevent execution from falling through to subsequent cases:
switch (variable) {
case 1:
// Handle case 1
break;
case 2:
// Handle case 2
break;
}
Key point: You can group multiple case labels that execute the same code block without additional breaks.
switch (variable) {
case 1:
case 2:
// This code executes for both case 1 and case 2
break;
}
Default Case in C++
The `default` case is optional but highly recommended to ensure comprehensive handling of unexpected values. It acts as a safety net, executing when none of the specified cases match:
switch (value) {
case 1:
// Handle case 1
break;
default:
// Handle unexpected values
}
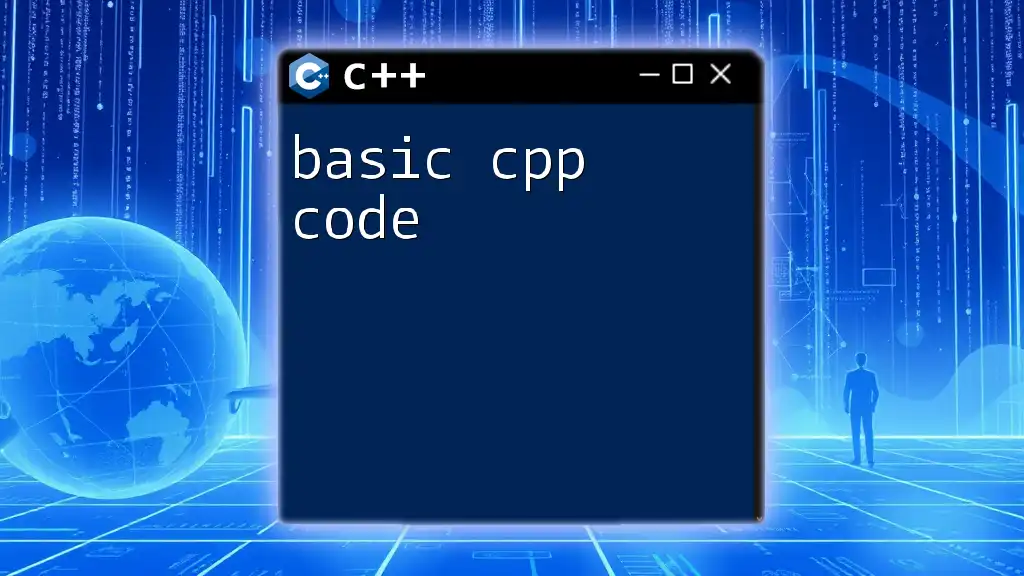
Switch Statement Example in C++
Let’s illustrate a switch statement with a simple example such as responding to user input in a console application:
#include <iostream>
using namespace std;
int main() {
int day;
cout << "Enter a number between 1 and 7: ";
cin >> day;
switch (day) {
case 1:
cout << "Monday";
break;
case 2:
cout << "Tuesday";
break;
case 3:
cout << "Wednesday";
break;
case 4:
cout << "Thursday";
break;
case 5:
cout << "Friday";
break;
case 6:
cout << "Saturday";
break;
case 7:
cout << "Sunday";
break;
default:
cout << "Invalid input!";
}
return 0;
}
In this example, the code takes an integer input representing a day of the week and uses the switch statement to display the corresponding day name. If an invalid number is entered, the default case handles the error.
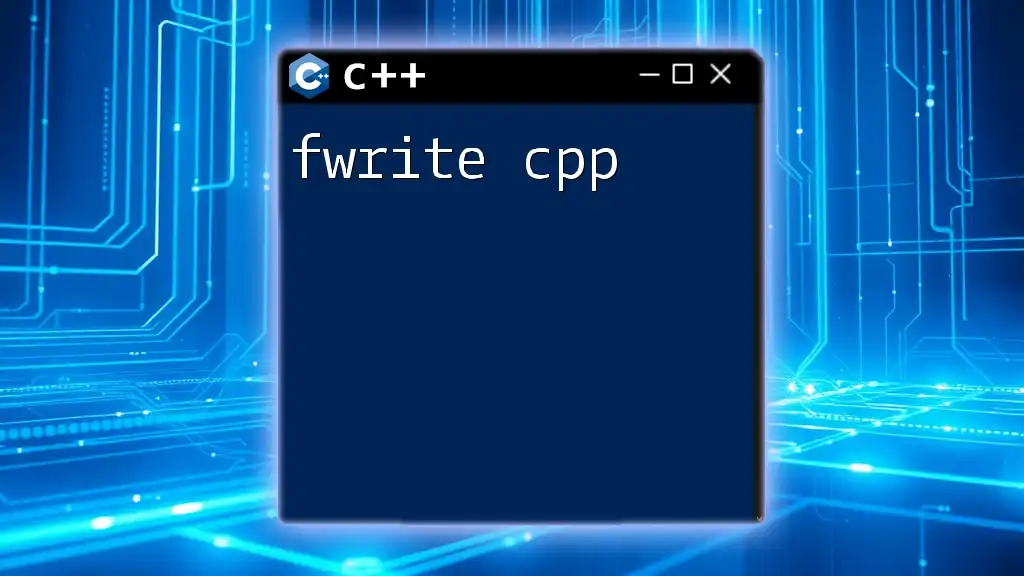
Real-World Applications of Switch Statements
Utilizing Switch Statements in Menu Selection
A prominent application of switch statements is in building console-based menu systems, where user inputs dictate which actions to perform:
#include <iostream>
using namespace std;
int main() {
int option;
cout << "Choose an option:\n1. Add\n2. Subtract\n3. Multiply\n4. Exit\n";
cin >> option;
switch (option) {
case 1:
cout << "Addition selected.";
break;
case 2:
cout << "Subtraction selected.";
break;
case 3:
cout << "Multiplication selected.";
break;
case 4:
cout << "Exiting.";
break;
default:
cout << "Invalid option.";
}
return 0;
}
In this scenario, the switch statement manages user interaction, invoking the appropriate action based on input.
Error Handling with Switch Statements
Switch can effectively simplify error handling in applications, allowing for a clear and organized method to address various error types. For instance, consider handling specific error codes returned from functions:
int errorCode = 2;
switch (errorCode) {
case 0:
cout << "No error.";
break;
case 1:
cout << "File not found.";
break;
case 2:
cout << "Access denied.";
break;
default:
cout << "Unknown error.";
}
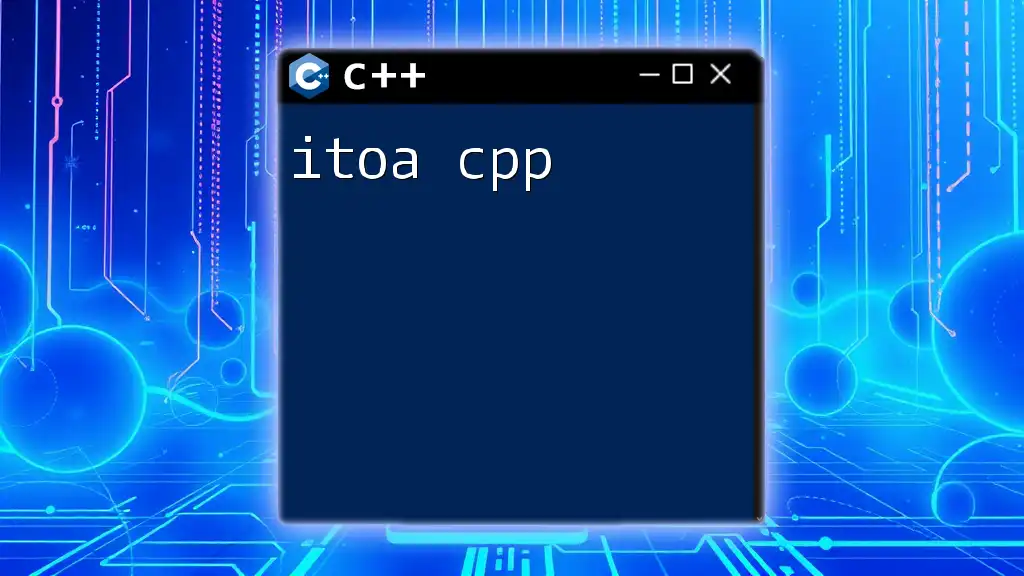
Best Practices for Using Switch in C++
When to Prefer Switch Over If-Else
- Readability: Complex conditionals with many branches are more readable with switch.
- Performance: Switch may provide faster execution since the compiler can optimize constant expressions leading to fewer comparisons.
Common Pitfalls to Avoid
- Omitting Break Statements: This error can lead to unintended behaviors, allowing multiple cases to execute sequentially, commonly referred to as "fall-through".
- Too Many Conditions: If your switch statement contains excessive cases, it may be beneficial to reconsider or refactor your approach for maintainability.
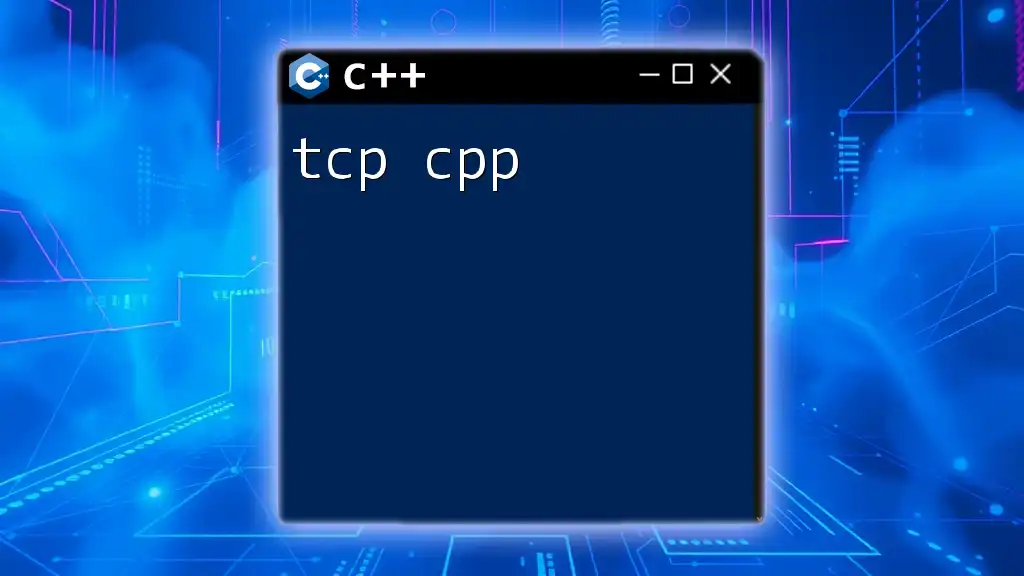
Switch Case in C++ Examples
Example 1: Basic Arithmetic Operations
A switch statement efficiently handles various arithmetic operations:
#include <iostream>
using namespace std;
int main() {
char operation;
double num1, num2;
cout << "Enter operator (+, -, *, /): ";
cin >> operation;
cout << "Enter two numbers: ";
cin >> num1 >> num2;
switch (operation) {
case '+':
cout << "Result: " << num1 + num2;
break;
case '-':
cout << "Result: " << num1 - num2;
break;
case '*':
cout << "Result: " << num1 * num2;
break;
case '/':
if (num2 != 0)
cout << "Result: " << num1 / num2;
else
cout << "Error: Division by zero.";
break;
default:
cout << "Invalid operator.";
}
return 0;
}
In this example, the switch handles four arithmetic operations based on user input, demonstrating clarity and versatility.
Example 2: Advanced Weather Application
A switch case can manage multiple weather conditions in an application:
#include <iostream>
using namespace std;
int main() {
int weather;
cout << "Enter the weather condition (1: Sunny, 2: Rainy, 3: Cloudy): ";
cin >> weather;
switch (weather) {
case 1:
cout << "It's a beautiful day!";
break;
case 2:
cout << "Don't forget your umbrella.";
break;
case 3:
cout << "Perfect weather for a walk.";
break;
default:
cout << "Unknown weather condition.";
}
return 0;
}
Here, the switch statement allows easy modification of actions based on the weather condition, maintaining a clean structure.
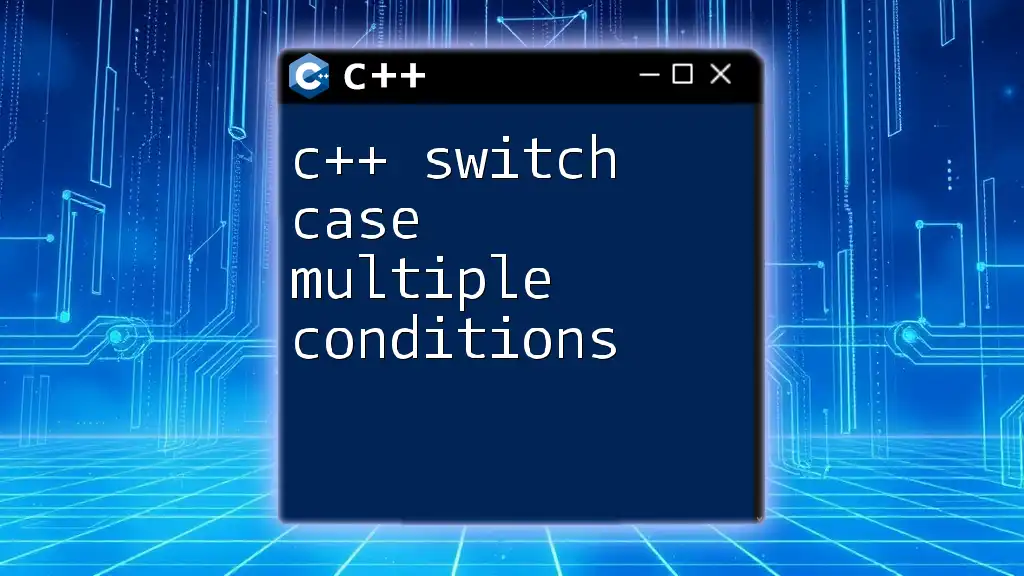
Conclusion
The switch statement in C++ is a powerful tool that can improve code clarity and efficiency, especially when facing multiple conditional branches. By using switch statements effectively, developers can manage complex logic with ease. Practicing the implementation of switch cases in various scenarios will solidify understanding and promote good coding habits.
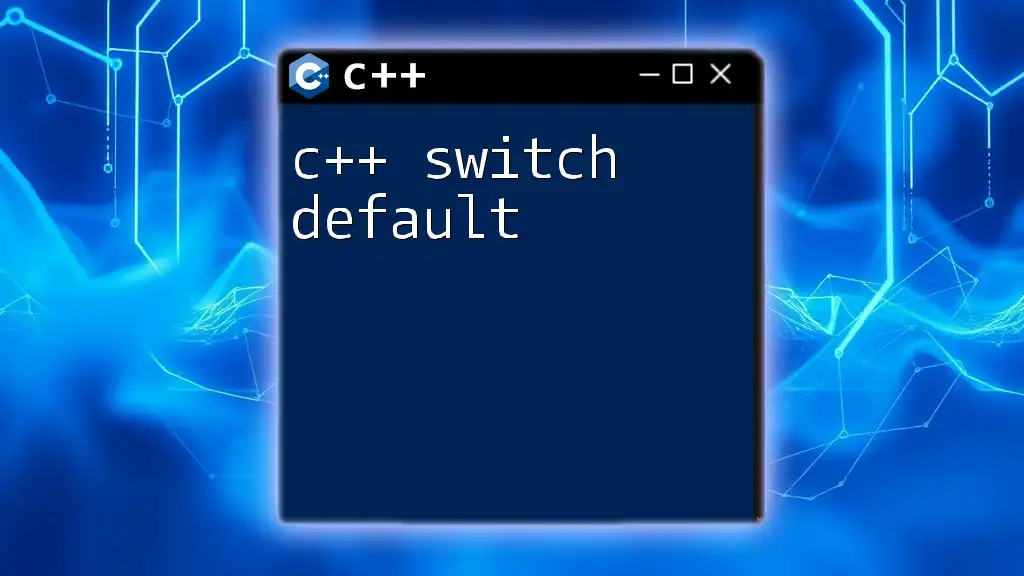
Frequently Asked Questions
What is the difference between switch and if-else in C++?
The switch statement is best for handling multiple discrete values of a single variable, while `if-else` is more flexible for complex conditions and ranges.
Can switch statements handle strings in C++?
No, C++ switch statements cannot handle strings directly. They accept integral types like integers, characters, or enumerated types.
Is there a limit to the number of case labels in a switch statement?
Technically, the number of case labels is constrained only by memory, but it’s advisable to limit case labels for maintainability.
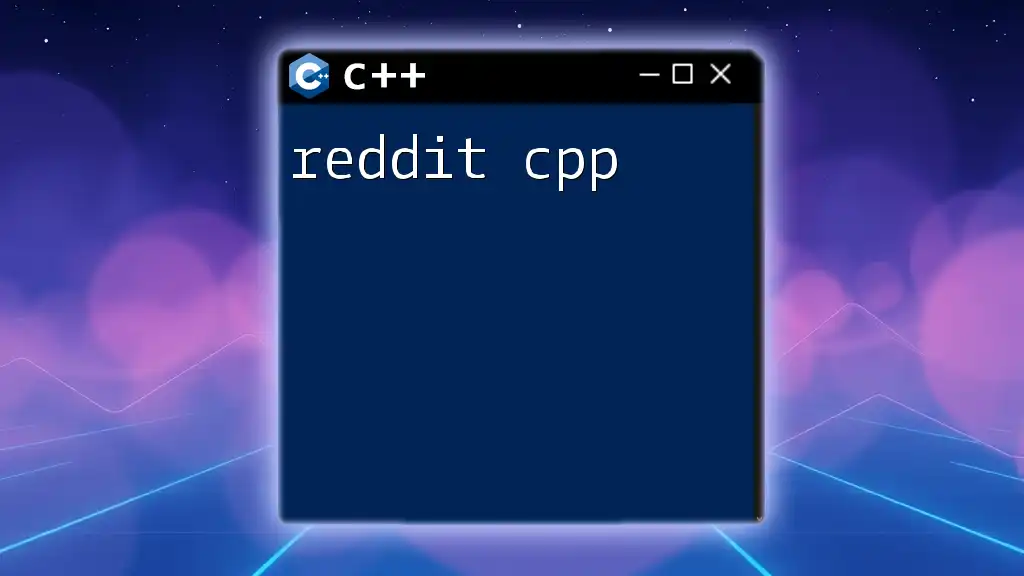
Additional Resources
For further understanding and practice with switch statements as part of C++, consider exploring additional resources and tutorials to sharpen your skills. These materials will deep dive into more complex implementations and best practices.
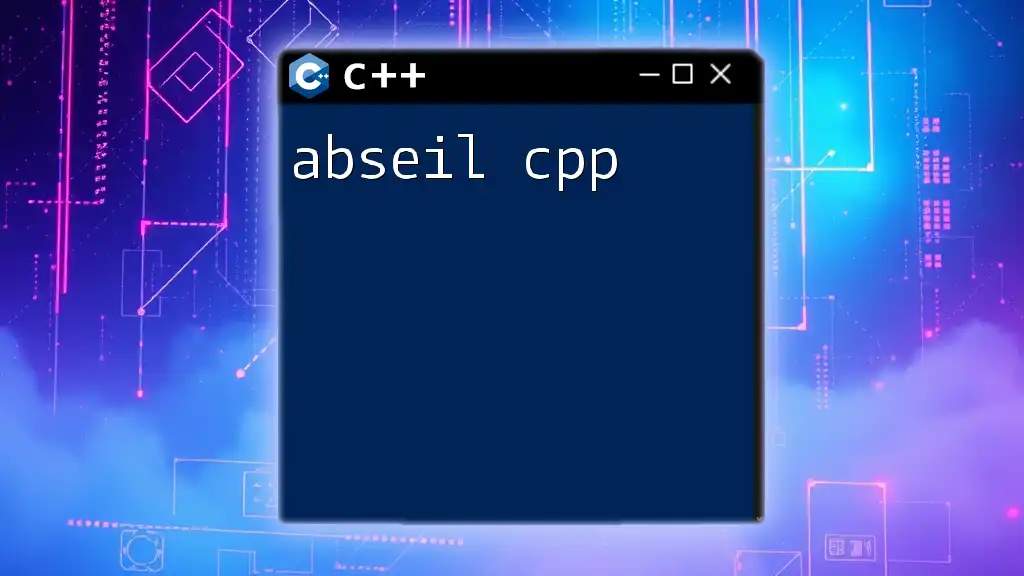
Call to Action
Ready to enhance your C++ skills? Enroll in our specialized courses for a deeper dive into `switch cpp`, along with a host of other essential programming concepts. Subscribe for more expert insights and tips!