Binary search in C++ is an efficient algorithm that finds the position of a target value within a sorted array by repeatedly dividing the search interval in half.
Here’s a simple implementation of binary search in C++:
#include <iostream>
#include <vector>
int binarySearch(const std::vector<int>& arr, int target) {
int left = 0, right = arr.size() - 1;
while (left <= right) {
int mid = left + (right - left) / 2;
if (arr[mid] == target) return mid; // Target found
if (arr[mid] < target) left = mid + 1; // Search right half
else right = mid - 1; // Search left half
}
return -1; // Target not found
}
int main() {
std::vector<int> arr = {1, 2, 3, 4, 5, 6, 7, 8, 9};
int target = 5;
int result = binarySearch(arr, target);
std::cout << "Target found at index: " << result << std::endl;
return 0;
}
Understanding the Binary Search Algorithm in C++
How Binary Search Works
Binary search operates on a sorted array and finds the position of a target value within that array. The central concept is that binary search narrows the search space by repeatedly dividing it in half. Here’s how it works step-by-step:
- Precondition: The array must be sorted. If the array is not sorted, binary search will not function correctly.
- Initial Bounds: Start with two pointers, `left` and `right`, indicating the starting and ending indices of the array.
- Midpoint Calculation: Calculate the middle index as `mid = left + (right - left) / 2`.
- Comparison:
- If the middle element equals the target value, the search is complete.
- If the target value is greater than the middle element, adjust the `left` pointer to `mid + 1`.
- If it is smaller, adjust the `right` pointer to `mid - 1`.
- Repeat: The process continues until the element is found or until `left` exceeds `right`, indicating the element is not present in the array.
The binary search can be implemented in two ways: iteratively or recursively.
Complexity Analysis of Binary Search
When analyzing binary search, it is essential to mention its efficiency in terms of time and space complexity:
- Time Complexity: O(log n)
- The logarithmic time complexity arises because with each iteration, the search space is reduced by half.
- Space Complexity: O(1) for the iterative version and O(log n) for the recursive version due to call stack usage.
When compared to linear search, which has a time complexity of O(n), binary search is significantly more efficient for large datasets, reinforcing its importance in algorithm development.

Implementing Binary Search in C++
Setting Up the C++ Environment
Before diving into code, ensure your C++ environment is ready. You can use any IDE such as Code::Blocks, Visual Studio, or even online compilers like Replit. For binary search, you will generally need the following libraries:
#include <iostream>
using namespace std;
Writing the Binary Search Algorithm in C++
Iterative Approach
The iterative approach to binary search uses a loop to reduce the search space. Below is the implementation:
int binarySearch(int arr[], int n, int x) {
int left = 0, right = n - 1;
while (left <= right) {
int mid = left + (right - left) / 2;
if (arr[mid] == x) {
return mid; // Element found
}
if (arr[mid] < x) {
left = mid + 1; // Adjust search to the right half
} else {
right = mid - 1; // Adjust search to the left half
}
}
return -1; // Element not found
}
Explanation:
- The while loop continues until `left` is less than or equal to `right`.
- If the middle element matches the target (`x`), its index is returned.
- Depending on whether `x` is greater or smaller than the middle element, the appropriate bounds are adjusted.
Recursive Approach
Using recursion, the binary search can be elegantly expressed. Here is the code:
int binarySearchRecursive(int arr[], int left, int right, int x) {
if (right >= left) {
int mid = left + (right - left) / 2;
if (arr[mid] == x) return mid; // Element found
if (arr[mid] > x) return binarySearchRecursive(arr, left, mid - 1, x);
return binarySearchRecursive(arr, mid + 1, right, x);
}
return -1; // Element not found
}
Explanation:
- The recursive function continues until the element is found or the search space is exhausted.
- The key difference from the iterative method is that recursion handles the function's repetitive calls implicitly.

Example Use Cases of Binary Search in C++
Searching in a Sorted Array
Binary search is commonly used to locate an item in a sorted array. The target value is compared to the middle element, thereby allowing efficient searches, especially in large datasets. Always ensure that the array is sorted before implementing binary search.
Finding the First or Last Occurrence of an Element
Sometimes, you may want to find not just any occurrence of an element but specifically the first or last. Below are simplified concepts to implement these functionalities:
-
Find the First Occurrence: Adapt the binary search algorithm to continue searching in the left half even if the middle element matches the target.
-
Find the Last Occurrence: Similarly, if the element is found at the midpoint, search the right half for potential further occurrences.
Application in Real-World Scenarios
Binary search is used across various domains, including:
- Databases: Efficiently retrieving records from sorted indexes.
- Searching Algorithms: Foundation for many advanced algorithms, including those in game design (e.g., locating assets quickly).
Understanding when to use binary search versus other searching techniques is crucial for effective programming.
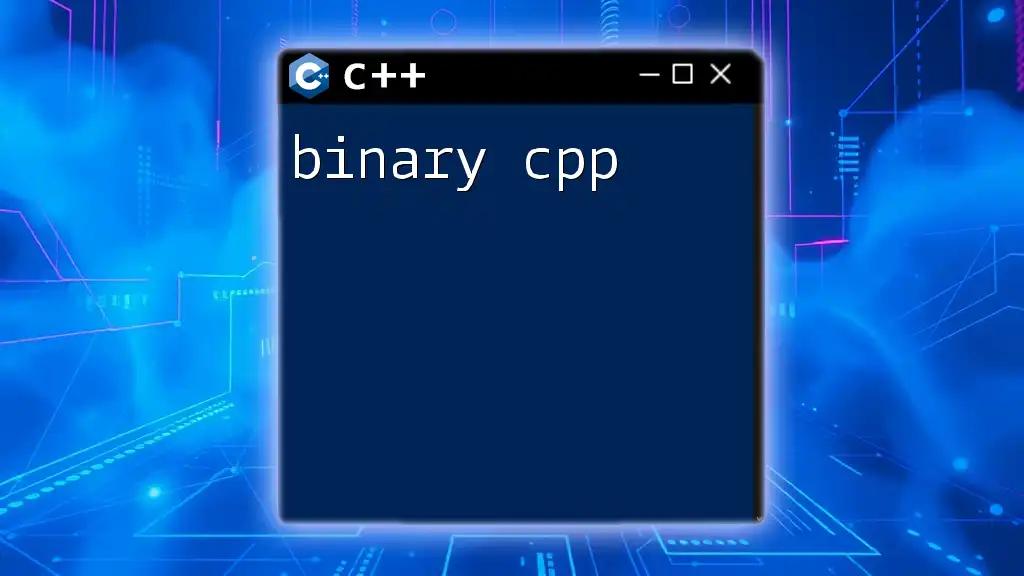
Troubleshooting Common Issues in Binary Search
Handling Edge Cases
When implementing binary search, consider these common scenarios:
- Element Not Present: Ensure your function returns a clear indicator (usually -1) when the target is not found.
- Empty Array: The search should handle an empty array gracefully.
- Single-Element Array: Ensure that the edge cases where the array has only one element are correctly processed.
Debugging Tips for Binary Search Implementation
Effective debugging can save time and effort. Here are a few tips:
- Visualize the Search Process: Write out the pseudocode and manually trace through an example to ensure you understand each step.
- Common Pitfalls:
- Incorrectly handling the `left` and `right` indices can cause infinite loops or missed elements.
- Not accounting for integer overflow when calculating the midpoint.

Conclusion: Mastering Binary Search in C++
In summary, binary search is a powerful algorithm that leverages sorted data to find elements efficiently. The insights into its implementation, time complexity, and use cases offer a strong foundation for mastering this algorithm in C++.
As you enhance your C++ skills, practicing these implementations and exploring variations will deepen your understanding and proficiency. Continue to challenge yourself with exercises and engage with communities to solidify your knowledge.
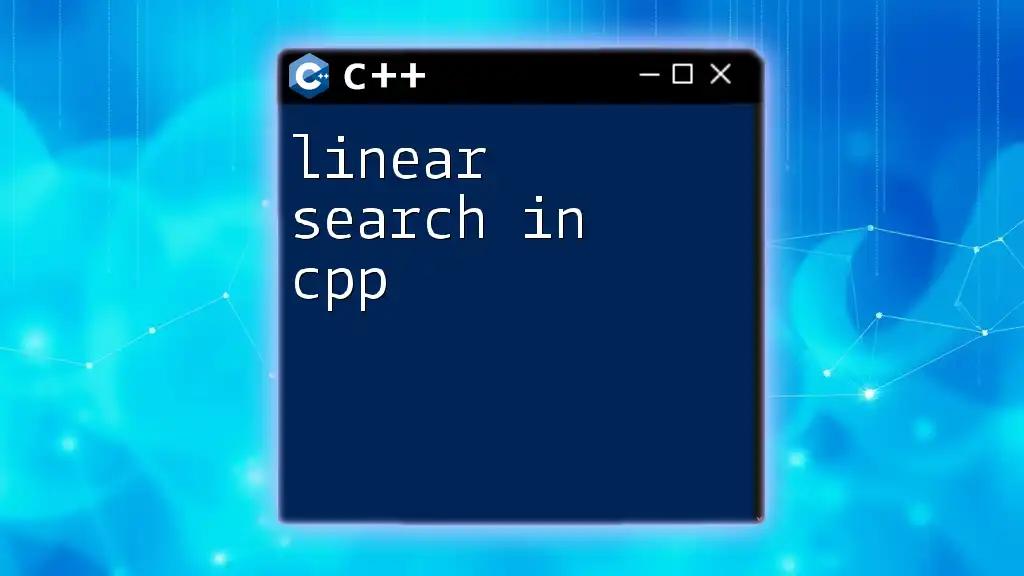
Additional Resources
Books and Online Courses
For further learning, consider resources such as:
- "Introduction to Algorithms" by Cormen et al.
- Online platforms like Coursera, Udemy, and edX that offer algorithm courses focusing on searching methods.
Community and Support
Engaging with coding communities such as Stack Overflow, Reddit's r/programming, or Discord servers can provide valuable insights and support as you delve deeper into binary search and other algorithms.
By embracing these resources and continuing your practice, you will gain mastery in binary search and its applications in C++.