A binary tree in C++ is a hierarchical data structure where each node has at most two children, typically referred to as the left and right child.
Here's a simple code snippet demonstrating the structure of a binary tree in C++:
#include <iostream>
struct Node {
int data;
Node* left;
Node* right;
Node(int value) : data(value), left(nullptr), right(nullptr) {}
};
int main() {
Node* root = new Node(1);
root->left = new Node(2);
root->right = new Node(3);
std::cout << "Root: " << root->data << std::endl; // Output: Root: 1
return 0;
}
Understanding Binary Trees
What is a Binary Tree?
A binary tree is a data structure that consists of nodes, where each node has at most two children referred to as the left child and the right child. Binary trees are fundamental in computer science and are enhanced versions of single linked lists that allow efficient data organization and retrieval.
Applications of binary trees are vast:
- They’re used to implement various search algorithms, such as binary search trees, where efficient searching can be performed in logarithmic time.
- Binary trees play a crucial role in various data storage methods, like databases, which utilize tree structures for indexing and quick lookup.
Characteristics of Binary Trees
Understanding the characteristics of binary trees can better equip you for actual implementation.
Node Structure
Each node in a binary tree is typically structured with the following elements:
- A data field which stores the value.
- A pointer/reference to its left child.
- A pointer/reference to its right child.
Types of Binary Trees
- Full Binary Tree: Every node other than the leaves has two children.
- Complete Binary Tree: All levels are fully filled, except possibly the last level, which is filled from left to right.
- Perfect Binary Tree: All nodes have two children, and all leaf nodes are at the same level.
- Balanced Binary Tree: The height of the tree is minimized, ensuring that left and right subtrees' heights differ by at most one.
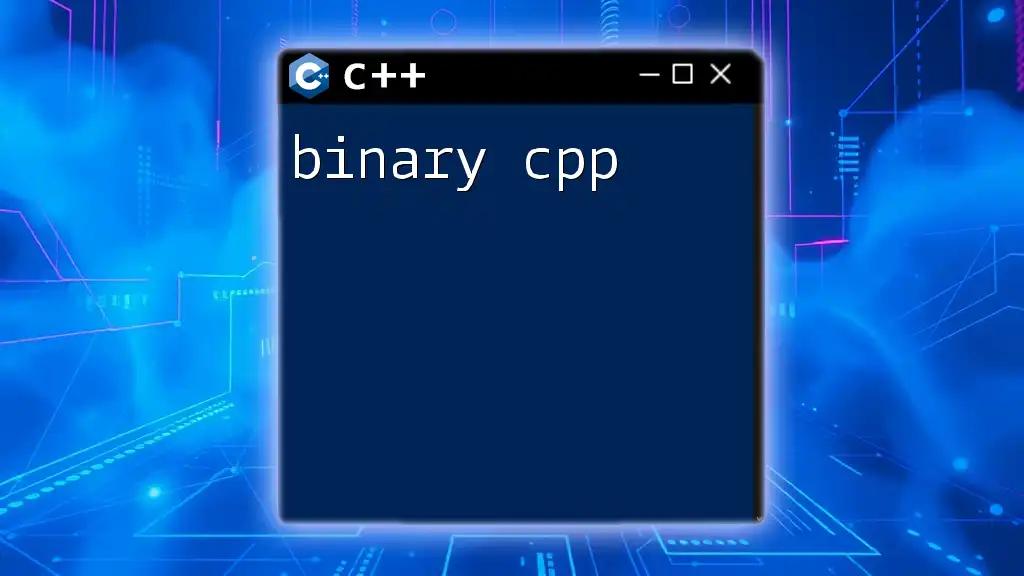
Implementing a Binary Tree in C++
Setting Up the Environment
To start working with binary trees in C++, you’ll need a development environment that supports C++. Popular choices include Visual Studio, Code::Blocks, and Eclipse. Ensure you have a compatible C++ compiler, such as g++, installed and properly configured.
Basic Structure of Binary Tree Node
To define a simple binary tree node in C++, you can use the following structure, which encapsulates the basic elements discussed:
struct Node {
int data;
Node* left;
Node* right;
Node(int val) : data(val), left(nullptr), right(nullptr) {}
};
This basic Node class enables the creation of a binary tree, where `data` is the value stored in the node, and `left` and `right` point to the child nodes.

Creating a Binary Tree
Inserting Elements
To construct a binary tree, you must implement an insert function. This function ensures that all values are inserted according to binary search tree properties. Here’s a concise insert function:
Node* insert(Node* root, int val) {
if (root == nullptr) {
return new Node(val);
}
if (val < root->data) {
root->left = insert(root->left, val);
} else {
root->right = insert(root->right, val);
}
return root;
}
In this example, if the `root` is `nullptr`, a new node is created. The function checks whether to traverse left or right based on the value being inserted.
Traversing the Binary Tree
Traversal enables exploration of the binary tree and can be done using various methods. Here are the main traversal types:
- In-order Traversal: Left, root, right sequence.
- Pre-order Traversal: Root, left, right sequence.
- Post-order Traversal: Left, right, root sequence.
An example of in-order traversal implementation is as follows:
void inOrder(Node* root) {
if (root != nullptr) {
inOrder(root->left);
std::cout << root->data << " ";
inOrder(root->right);
}
}
This method recursively visits the left subtree, processed the root, and finally visits the right subtree, resulting in sorted order for binary search trees.

Binary Tree Operations
Searching for an Element
Searching within a binary tree can be accomplished using a recursive approach as shown in this search function:
bool search(Node* root, int val) {
if (root == nullptr) return false;
if (root->data == val) return true;
return (val < root->data) ? search(root->left, val) : search(root->right, val);
}
In this function, if the node is `nullptr`, it returns `false`. If the node contains the search value, it returns `true`. Otherwise, it continues to search left or right based on the value.
Deleting Nodes
Deleting nodes requires special consideration as it can affect the tree structure. The `deleteNode` function below explains how to remove a node while maintaining binary tree properties.
Node* deleteNode(Node* root, int val) {
if (root == nullptr) return nullptr;
if (val < root->data) {
root->left = deleteNode(root->left, val);
} else if (val > root->data) {
root->right = deleteNode(root->right, val);
} else {
if (root->left == nullptr) return root->right;
else if (root->right == nullptr) return root->left;
Node* temp = minValueNode(root->right);
root->data = temp->data;
root->right = deleteNode(root->right, temp->data);
}
return root;
}
This function first locates the target node, then handles scenarios based on its children, ensuring the replacement of deleted nodes follows binary tree rules.
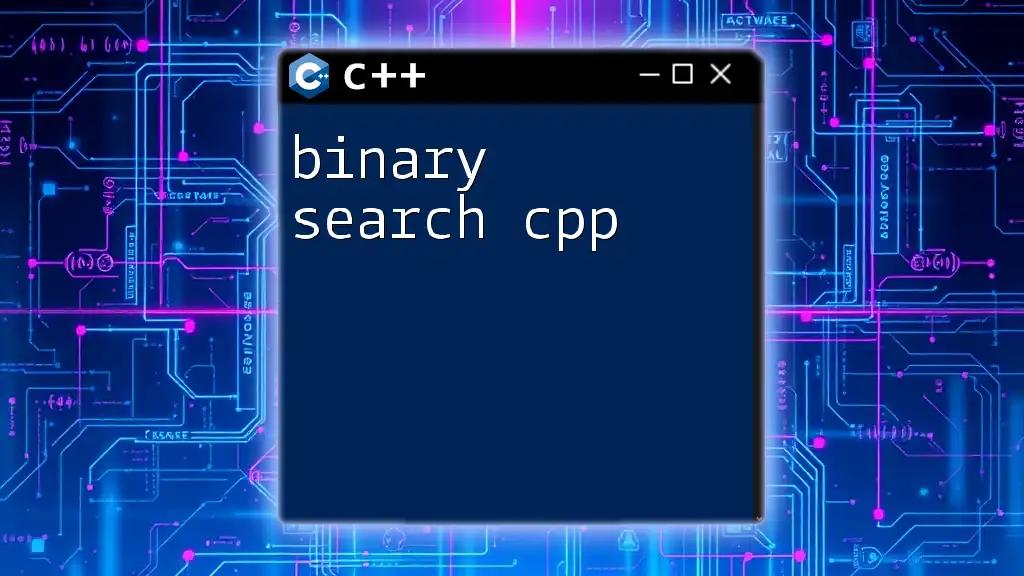
Common Problems and Solutions
Balancing the Binary Tree
Maintaining a balanced binary tree is crucial for efficient operations. A balanced tree has a minimal height, ensuring that the time complexity of search and insertion operations remains logarithmic.
To implement balancing techniques, you can utilize structures like AVL Trees or Red-Black Trees, which automatically maintain balance during insertions and deletions through rotations.
Handling Edge Cases
Handling edge cases is vital in robust binary tree implementations. For instance, when you attempt operations on a null tree:
if (root == nullptr) {
std::cout << "Tree is empty!";
return;
}
This check ensures that your program does not attempt to access properties of a null reference, leading to safer operations.

Advanced Concepts
Binary Tree vs. Binary Search Tree
While both binary trees and binary search trees (BSTs) are used to store hierarchical data, the key difference lies in how they organize their nodes. In a binary search tree, for any given node, values in the left subtree are less than the node's value, while values in the right subtree are greater. This structure enhances searching and sorting operations significantly compared to a general binary tree.
Conclusion
In summary, the efficient manipulation of binary trees in C++ relies on understanding their structure, operations, and common pitfalls. Implementing binary trees equips developers with the foundational knowledge needed for more complex data structures. Practicing these concepts will solidify your understanding and greatly enhance your programming skills in C++.