A behavior tree in C++ is a hierarchical model used in AI for organizing complex behaviors in a modular and reusable manner.
Here’s a simple code snippet demonstrating a basic behavior tree structure in C++:
#include <iostream>
#include <memory>
class Node {
public:
virtual bool tick() = 0; // Each node should implement this method
};
class ActionNode : public Node {
public:
bool tick() override {
std::cout << "Action executed!" << std::endl;
return true; // Indicates success
}
};
class SelectorNode : public Node {
std::shared_ptr<Node> child1;
std::shared_ptr<Node> child2;
public:
SelectorNode(std::shared_ptr<Node> c1, std::shared_ptr<Node> c2)
: child1(c1), child2(c2) {}
bool tick() override {
return child1->tick() || child2->tick(); // Executes child nodes in order
}
};
int main() {
std::shared_ptr<Node> actionNode = std::make_shared<ActionNode>();
std::shared_ptr<Node> selectorNode = std::make_shared<SelectorNode>(actionNode, actionNode);
selectorNode->tick(); // Running the behavior tree
return 0;
}
Understanding Behavior Trees
What is a Behavior Tree?
A behavior tree is a hierarchical structure used in artificial intelligence that provides a clear and efficient way of managing complex behaviors in entities, especially in games. Its design allows for a modular approach, enabling developers to create reusable behaviors. Unlike finite state machines, which can become convoluted with numerous states and transitions, behavior trees divide behaviors into smaller, manageable pieces that can be combined in various ways.
Structure of Behavior Trees
Behavior trees consist of several key elements:
- Nodes are the building blocks of behavior trees. They can be broadly categorized into:
- Leaf Nodes: These perform actions or checks and provide the direct behavior of the entity.
- Composite Nodes: These manage collections of other nodes and define control flow.
- Types of Nodes include:
- Decorator Nodes: They add conditions or modify the behavior of other nodes. For instance, a decorator might restrict an action to only occur under a certain condition.
- Sequence Nodes: These nodes execute child nodes in order, stopping if any child fails.
- Selector Nodes: Also known as "fallback" nodes, these evaluate child nodes until one succeeds.
Execution Order
The execution order of nodes is critical in behavior trees. When a tree is ticked (evaluated), the root node is processed. If it is a composite node, it determines which child node to execute next based on its type (sequence or selector), eventually leading to leaf nodes performing actions or returning success or failure.
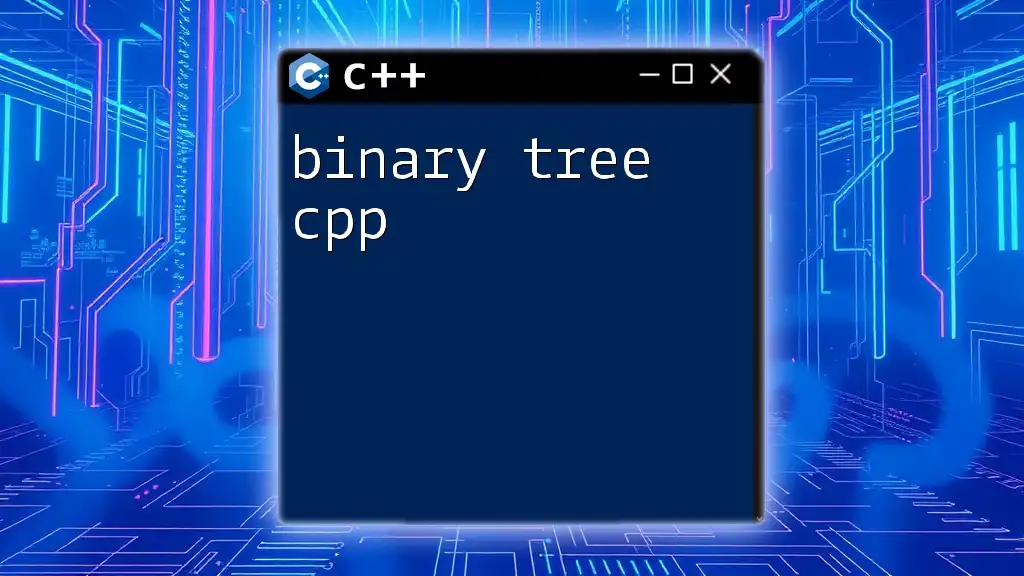
Setting Up Your C++ Environment
Required Tools and Libraries
To begin implementing behavior trees in C++, you will need a suitable development environment. Consider using Visual Studio or Code::Blocks for coding. Additionally, leveraging libraries like BehaviorTree.CPP can simplify your implementation, allowing you to focus on designing behavior rather than the nitty-gritty of the data structure.
Creating Your First Behavior Tree
Creating a simple behavior tree is a great way to familiarize yourself with the concepts. Let’s say we want to design a basic behavior tree for a Non-Playable Character (NPC) that decides whether to patrol or chase a player.
- Define the NPC behavior: Start by deciding the conditions under which the NPC will stop patrolling.
- Use the BehaviorTree.CPP library to set up your nodes. Below is an example of a basic behavior tree structure.
// Code snippet for a simple behavior tree node
class MyAction : public BT::ActionNode {
public:
MyAction(const std::string& name) : BT::ActionNode(name) {}
BT::NodeStatus tick() override {
// Action implementation
return BT::NodeStatus::SUCCESS;
}
};
In this code snippet, we define a custom action node that can be used in our behavior tree.
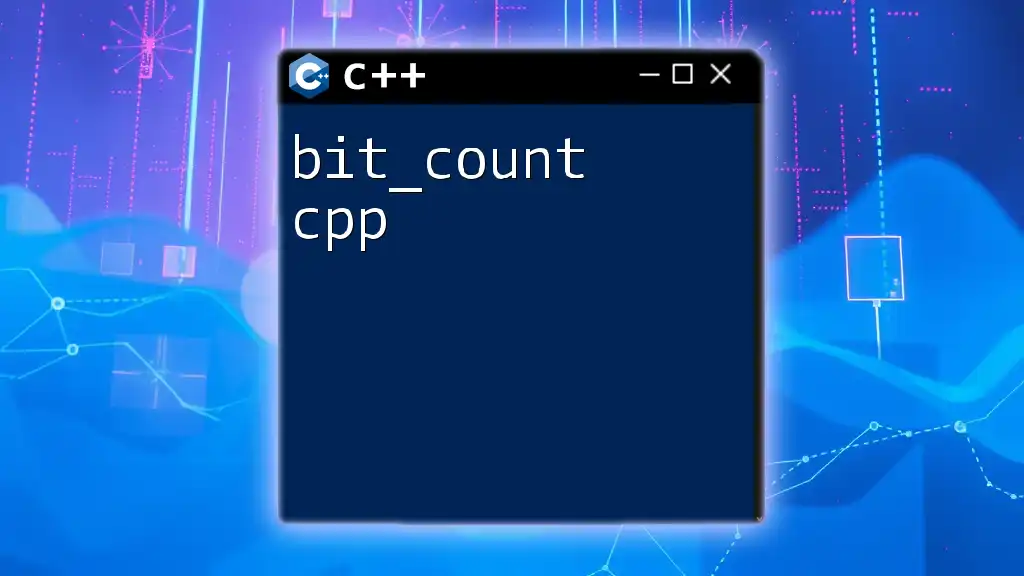
Implementing Behavior Trees in C++
Basic Node Implementation
Creating Custom Nodes
To enrich your behavior tree, you will likely want to implement custom nodes. This allows you to define specific actions and conditions tailored to your game's needs. An example of a custom condition node looks like this:
class MyCondition : public BT::ConditionNode {
public:
MyCondition(const std::string& name) : BT::ConditionNode(name) {}
BT::NodeStatus tick() override {
// Condition logic
return BT::NodeStatus::FAILURE;
}
};
This simple `MyCondition` class checks a condition every time the tree ticks. You can adjust the logic to meet your game requirements.
Composing Behavior Trees
Using Composite Nodes
When composing your behavior tree, you will start to see how sequences and selectors interact with your defined nodes. Here’s an example of how to create a sequence node that first checks the NPC's health and then chooses to attack if the health is below a certain threshold:
auto root = std::make_shared<BT::Sequence>("MainSequence");
root->addChild(std::make_shared<MyCondition>("IsPlayerNearby"));
root->addChild(std::make_shared<MyAction>("PerformAttack"));
In this snippet, `IsPlayerNearby` is a condition that determines if the action `PerformAttack` can occur. The sequence executes in order, stopping if `IsPlayerNearby` fails.
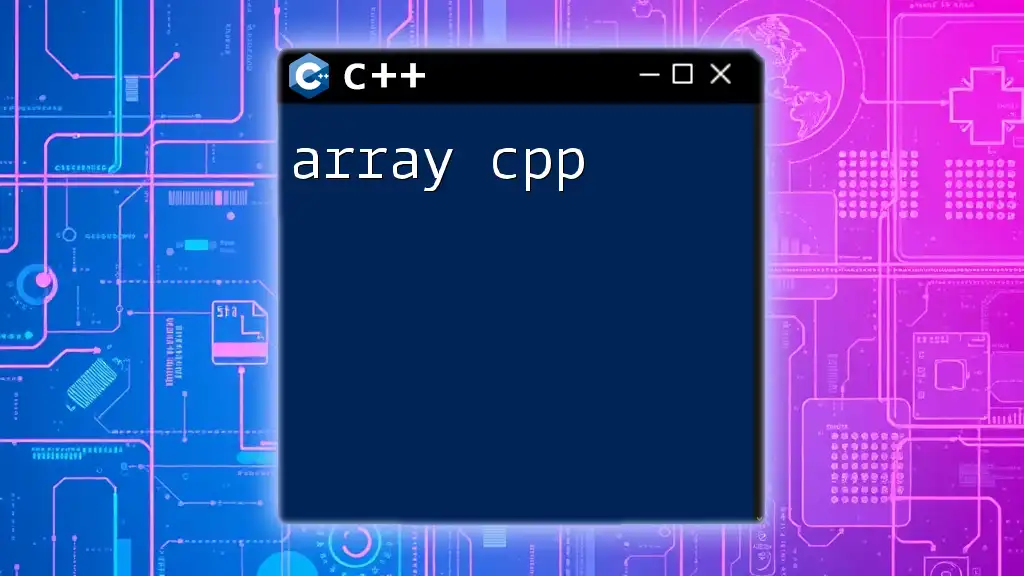
Debugging and Testing Behavior Trees
Common Issues and Solutions
Debugging behavior trees can be tricky given their hierarchical nature. Common issues include incorrect node execution order, which can often be solved by carefully reviewing and testing each node. Utilize logging to trace the flow of execution and ensure that conditions and actions are behaving as expected.
Visualization Tools
Visualizing your behavior trees can greatly enhance understanding and debugging. Some software offers graphical representations of behavior trees, making it easier to spot errors and refine behavior.
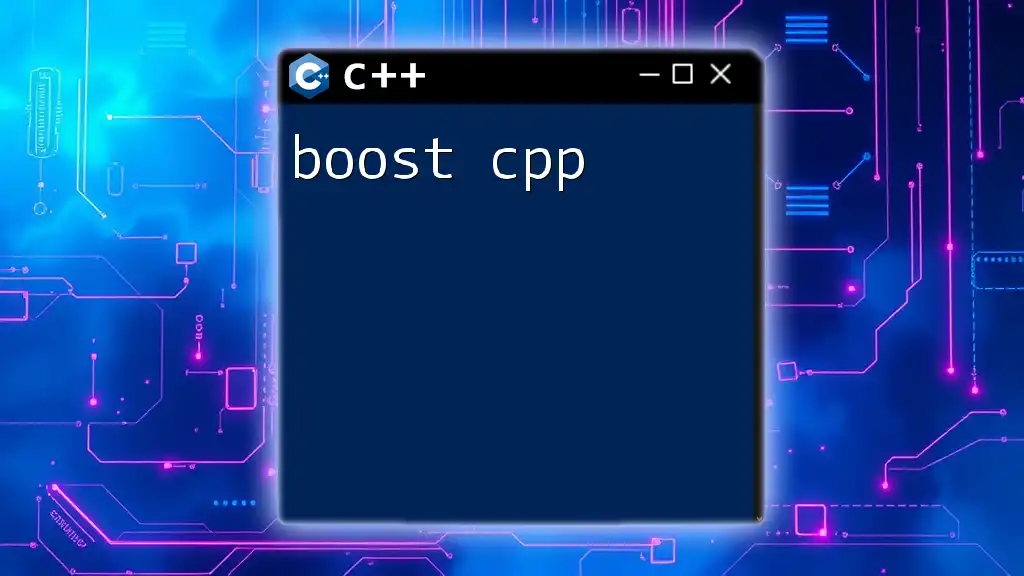
Best Practices for Behavior Trees
Design Principles
When designing behavior trees, keep nodes as small and focused as possible. Each node should ideally perform a single responsibility, enhancing both clarity and reusability. This modularity allows for easier updates and adaptations as your game's requirements evolve.
Performance Considerations
While behavior trees provide flexibility, it’s essential to remain mindful of performance. Inefficient nodes can lead to performance degradation, particularly in complex scenes with many entities. Always look for opportunities to optimize node logic and branch pruning.
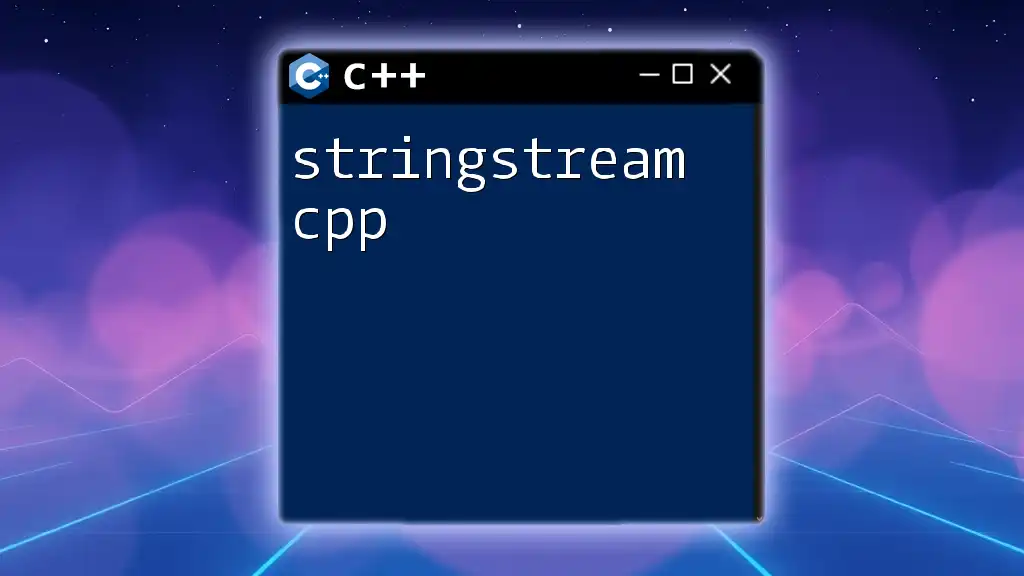
Real-World Applications of Behavior Trees
Case Study: Game AI
Behavior trees are prevalent in game AI design, notably in titles like Halo and Fortnite, where NPCs must exhibit adaptable and realistic behaviors. Each NPC’s behavior can be intricately constructed using behavior trees, allowing for smooth interaction with players. As an example, you might implement a chasing and evading sequence that reacts to player proximity dynamically.
Robotics and Automation
Beyond gaming, behavior trees can be applied in robotics for managing complex tasks. For example, using behavior trees in a robotic arm can effectively coordinate multiple tasks based on environmental conditions and sensor input. This high-level overview demonstrates the versatility of behavior trees across various domains.
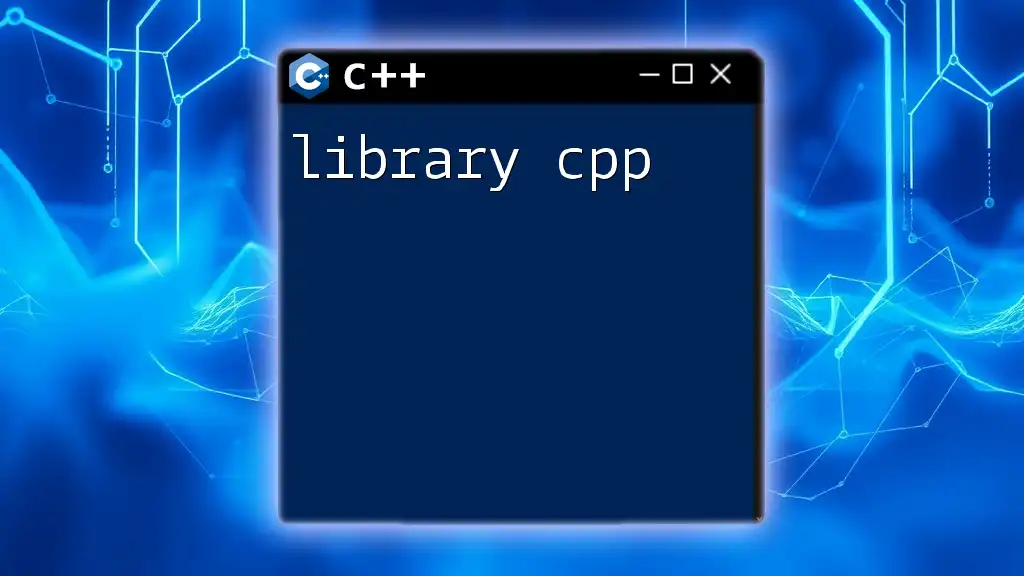
Conclusion
Behavior trees offer a powerful paradigm for managing complex behaviors, especially in gaming and AI contexts. By understanding their structure and execution flow, you can create sophisticated, modular behaviors that are easy to manage and extend. Experimentation with behavior trees will reveal their capabilities and enhance your projects' intelligence.
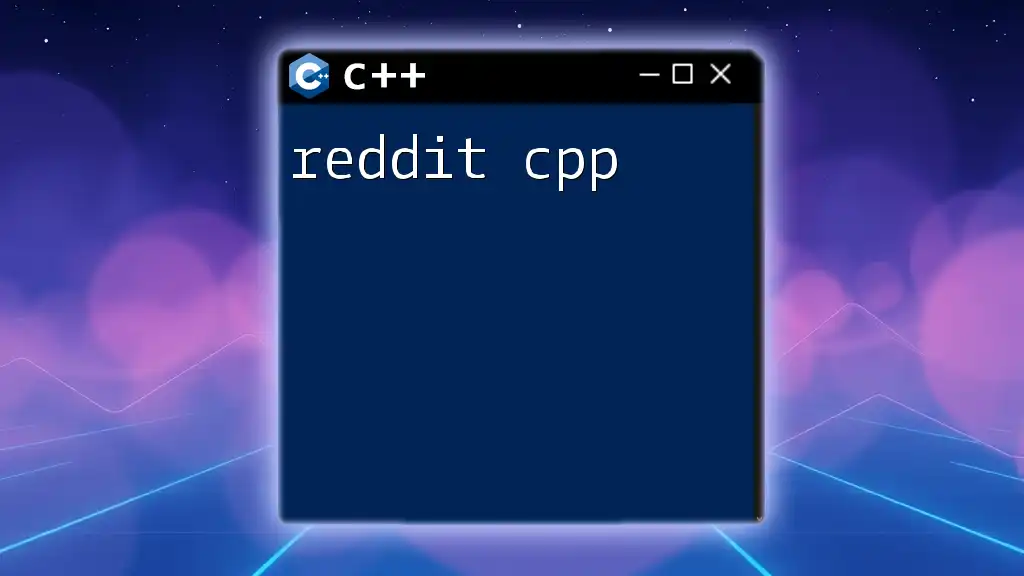
Additional Resources
To deepen your knowledge, consider exploring recommended books on game AI design and visiting online resources dedicated to C++ programming. Participating in community forums will also connect you with fellow developers eager to share insights on behavior trees and AI design.