Boost C++ Libraries is a collection of peer-reviewed, portable C++ source libraries that facilitate functionality like smart pointers, multithreading, and regular expressions, enhancing productivity for C++ developers.
Here's a simple example of using Boost to create a smart pointer:
#include <boost/shared_ptr.hpp>
#include <iostream>
class MyClass {
public:
void display() {
std::cout << "Hello from MyClass!" << std::endl;
}
};
int main() {
boost::shared_ptr<MyClass> ptr(new MyClass());
ptr->display();
return 0;
}
What is Boost C++?
Boost is a collection of peer-reviewed, portable C++ libraries that offer a wide range of functionality. It originated in the late 1990s with the intent to provide a standard set of libraries for C++ programmers. Over the years, Boost has grown tremendously and is now widely recognized as one of the largest and most versatile C++ libraries available.
Boost libraries are known for their high quality and efficiency, which sets them apart from many other libraries. They often serve as the foundation for forthcoming features that eventually make their way into the C++ Standard Library. Utilizing Boost C++ can vastly enhance productivity while maintaining high performance in programming tasks.
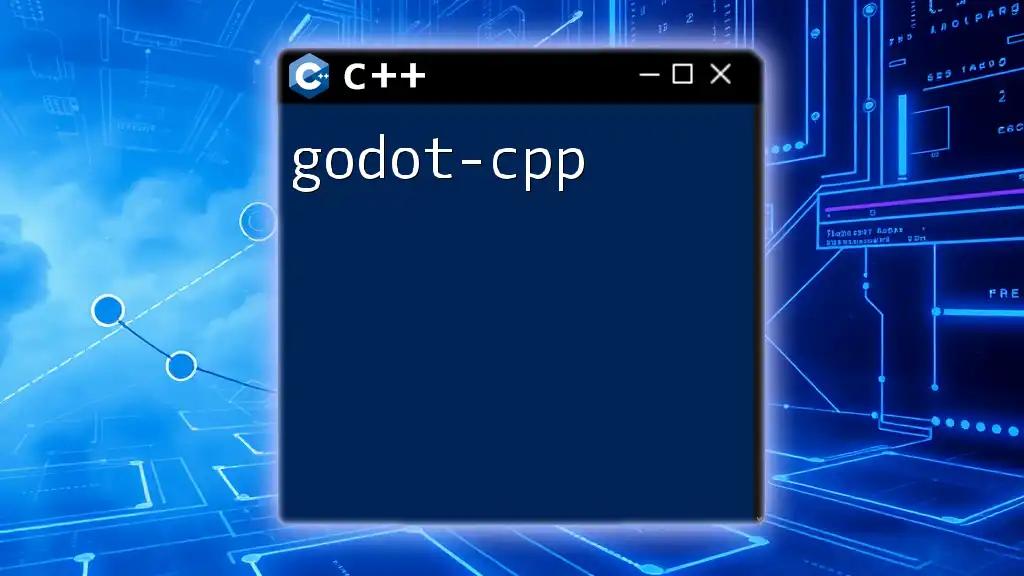
Why Use Boost C++?
Key Benefits
Boost provides numerous advantages that make it a valuable asset to any C++ developer:
- Code Reusability: With a vast selection of libraries, developers can leverage existing solutions instead of writing code from scratch.
- Enhanced Performance: Boost libraries are optimized for performance, often outperforming homegrown solutions.
- Extensive Library Support: Boost covers multiple domains, including algorithms, file handling, networking, and more, thus minimizing the need for external dependencies.
Comparison with Standard Libraries
While the C++ Standard Library covers many essential functionalities, it does have limitations. For instance, Boost offers libraries that are not included in the standard API. In cases where advanced data structures or algorithmic implementations are needed, Boost becomes the go-to choice.

Getting Started with Boost C++
Installation Guide
Installing Boost is straightforward but may vary based on your operating system.
For Windows
- Download the latest version from the Boost website.
- Extract the files to your desired directory.
- Optionally, you can use the Boost installer for a more automated setup.
For Linux
Use the package manager to install Boost with a simple command:
sudo apt-get install libboost-all-dev
For macOS
If you're using Homebrew, simply run:
brew install boost
Setting Up Your Environment
After installation, you'll need to configure your development environment. This includes linking Boost libraries in your IDE of choice, such as Visual Studio or Eclipse. Ensure you set the compiler option to recognize Boost headers to avoid any compatibility issues.
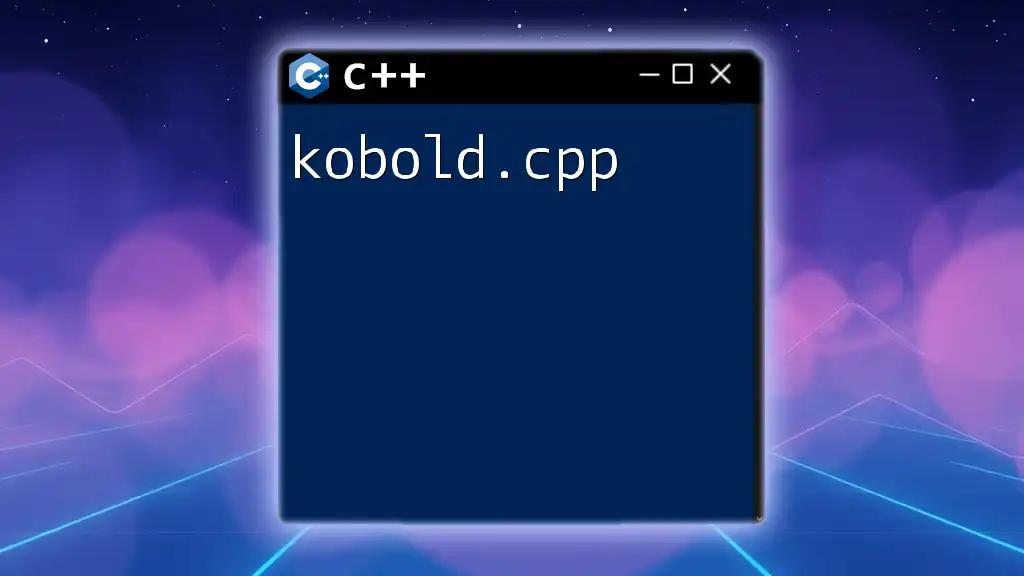
Key Features of Boost C++
Smart Pointers
One of the standout features of Boost is its implementation of smart pointers. Smart pointers help manage the memory of dynamically allocated objects, reducing the risk of memory leaks:
#include <boost/shared_ptr.hpp>
#include <iostream>
void useSmartPointer() {
boost::shared_ptr<int> ptr(new int(10));
std::cout << "Value: " << *ptr << std::endl;
}
In this example, `boost::shared_ptr` automatically manages the memory allocated for the integer. When the last reference to the pointer goes out of scope, the memory is automatically freed.
Boost.Asio for Networking
Boost.Asio is a powerful library for network programming and asynchronous I/O. It enables developers to write scalable and efficient network applications.
Here's an example of a simple TCP server using Boost.Asio:
#include <boost/asio.hpp>
void simpleServer() {
boost::asio::io_context io;
boost::asio::ip::tcp::acceptor acceptor(io, {boost::asio::ip::tcp::v4(), 12345});
boost::asio::ip::tcp::socket socket(io);
acceptor.accept(socket); // Accept a connection
// Additional server code...
}
This snippet shows how easily you can create a network service without having to manage low-level socket details.
Boost.Filesystem
This library simplifies file and directory manipulation. It provides a portable way to handle paths and operations seamlessly across different operating systems.
Here's an example of traversing a directory with Boost.Filesystem:
#include <boost/filesystem.hpp>
#include <iostream>
void listDirectory(const std::string& path) {
boost::filesystem::path dir(path);
for (const auto& entry : boost::filesystem::directory_iterator(dir)) {
std::cout << entry.path() << std::endl;
}
}
In this function, specifying a directory path allows you to list all files and directories within the target directory effortlessly.

Advanced Boost Libraries
Boost.Bind
Boost.Bind simplifies the handling of function calls and callbacks, whether for regular functions or class methods. This library can enhance code readability and reusability.
Example using Boost.Bind with a member function:
#include <boost/bind.hpp>
#include <iostream>
class MyClass {
public:
void show(int x) {
std::cout << "Value: " << x << std::endl;
}
};
void useBind() {
MyClass obj;
auto boundFunction = boost::bind(&MyClass::show, &obj, _1);
boundFunction(5);
}
In this example, `boost::bind` creates a callable object that is bound to a specific instance of `MyClass`, showcasing a simple way to manage callbacks.
Boost.Regex
Boost.Regex provides a robust framework for working with regular expressions in C++. It offers extensive regex capabilities that make pattern matching efficient.
Here's a code snippet demonstrating pattern matching:
#include <boost/regex.hpp>
#include <iostream>
void regexExample() {
std::string s = "Boost C++ Libraries";
boost::regex expr("Boost");
std::cout << boost::regex_search(s, expr) << std::endl; // Output: 1 (true)
}
This snippet verifies whether the substring "Boost" exists within the string `s`, returning a boolean value accordingly.
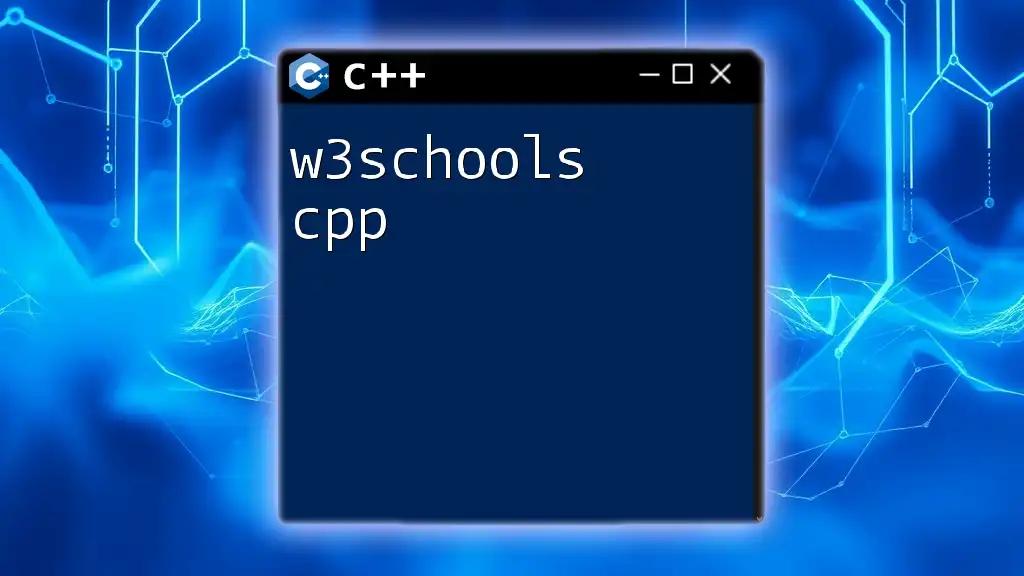
Troubleshooting Common Boost Issues
Language and Compiler Compatibility
Boost libraries are designed to be compatible with various C++ standards. However, developers should ensure that the installed Boost version aligns well with the compiler being used. Always consult the documentation for version compatibility.
Linking Errors
If you encounter linking errors during compilation, it’s often due to missing library paths or improperly configured project settings. Ensure that your development environment properly references the Boost library paths and adjust the project settings to include the necessary libraries.

Best Practices for Using Boost C++
Version Control
Keeping your Boost libraries up to date is crucial for accessing the latest features and optimizations. Monitoring the Boost release announcements can help you stay updated.
Documentation and Community Resources
Engaging with the community can enhance your learning experience. Utilize the official Boost documentation as well as online forums and tutorials to address queries and share knowledge.

Conclusion
Boost C++ libraries offer a treasure trove of functionalities that can significantly enhance your C++ programming experience. From smart pointers to networking solutions, Boost provides reliable tools to tackle complex tasks efficiently. Development in C++ can be more productive and enjoyable with the integration of Boost. Dive in, explore, and leverage these libraries to boost your C++ skills further. Consider joining our platform for specialized tutorials and insights into mastering the art of C++ programming!
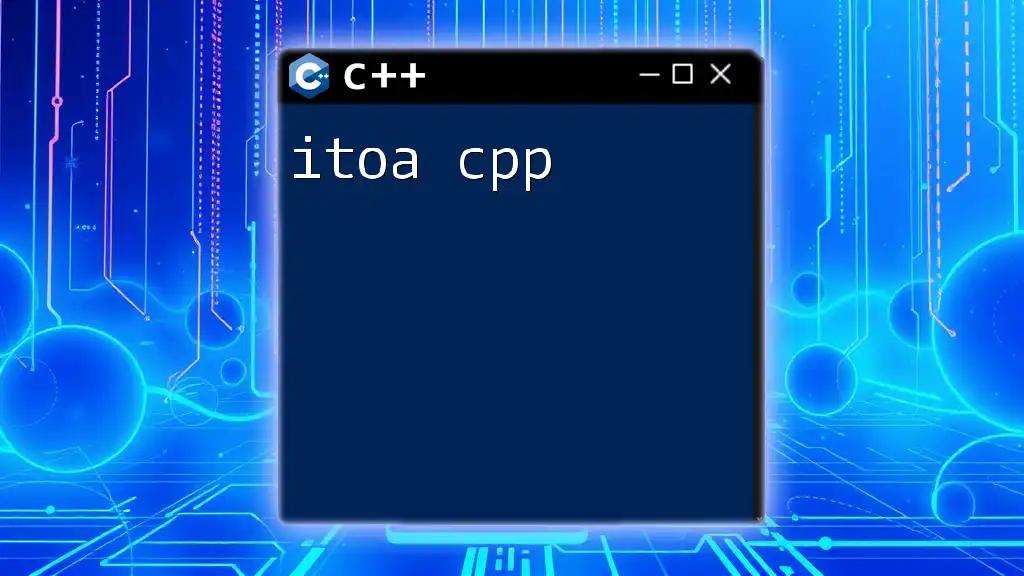
Additional Resources
To deepen your understanding, be sure to explore the official Boost C++ documentation and check out additional courses and tutorials that can help you get the most out of this incredible set of libraries.