Kobold.cpp is a C++ library designed for creating and managing interactive dialogue systems in game development.
Here’s a simple example of how to use kobold.cpp to initiate a dialogue:
#include <kobold.h>
int main() {
KoboldDialogue dialogue;
dialogue.addLine("Hello, traveler! What brings you here?");
dialogue.addLine("I seek adventure!");
dialogue.start();
return 0;
}
What is kobold.cpp?
kobold.cpp is a robust library designed specifically for simplifying and enhancing C++ development. It provides a set of commands and functionalities that streamline common programming tasks, making it easier for developers to focus on building efficient and maintainable applications. With its origins rooted in open-source principles, kobold.cpp has established itself as a powerful tool for both novice and experienced programmers looking to boost their productivity.
Why Use kobold.cpp?
Leveraging kobold.cpp in your development process comes with numerous benefits:
- Increased Efficiency: By utilizing a range of pre-built functionalities, developers can drastically reduce the time spent on routine tasks.
- User-Friendly Commands: The commands offered by kobold.cpp are designed to be intuitive, making them easy to learn and use, even for those new to C++.
- Modular Design: kobold.cpp supports a modular architecture, allowing developers to integrate it seamlessly into various projects without the hassle of heavy dependencies.
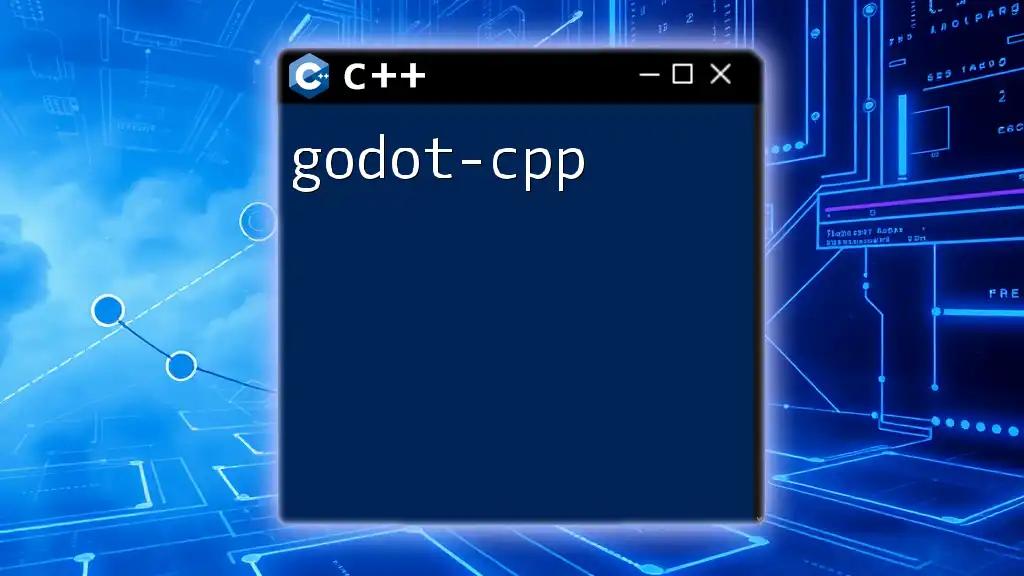
Installation Requirements
To get started with kobold.cpp, you need to ensure that your system meets a few basic requirements. This library is compatible with most modern operating systems, including:
- Windows
- macOS
- Linux
Steps to Install kobold.cpp
- Download the Library: Visit the official kobold.cpp repository on GitHub and download the latest release.
- Extract Files: Once downloaded, extract the files to a directory where you plan to develop.
- Add to Your C++ Project: Include the kobold.cpp headers in your C++ project.
#include "kobold.h" // Adjust the path based on where you placed the kobold.cpp files.
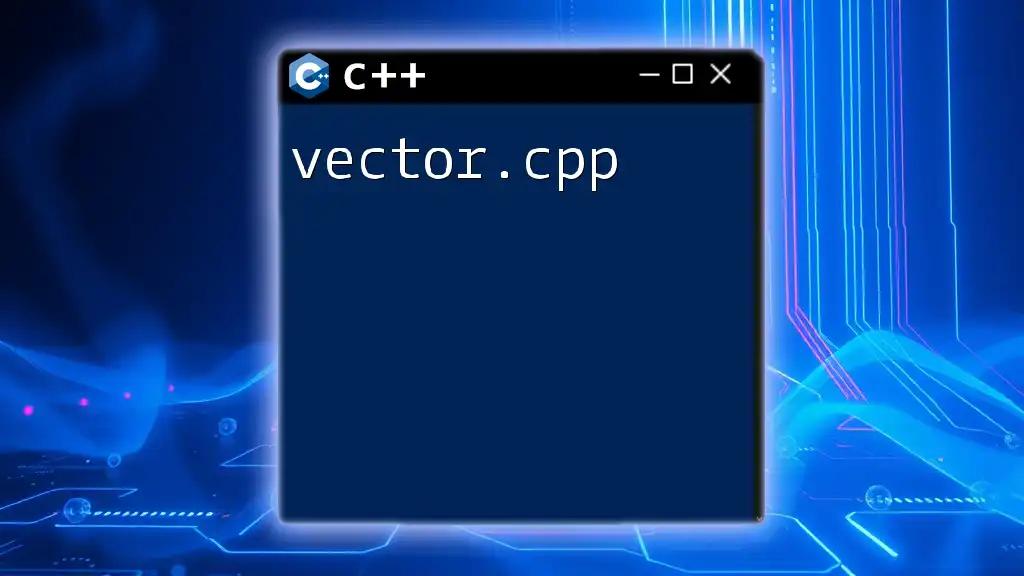
Basic Setup
Once you have installed kobold.cpp, initializing it in your C++ environment is straightforward. Here’s a simple example to demonstrate how to set it up:
#include "kobold.h"
int main() {
kobold::initialize(); // Initialize kobold.cpp
// Your application logic here.
return 0;
}
This snippet shows the basic setup, allowing your application to use the functions provided by kobold.cpp right away.
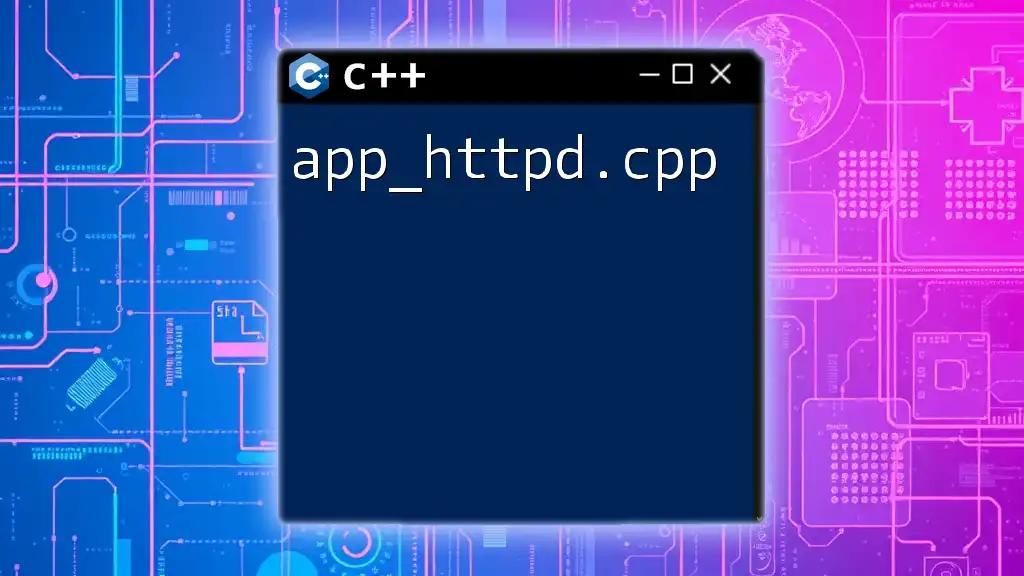
Understanding Key Components of kobold.cpp
Core Features
kobold.cpp offers several core features designed to enhance your programming experience:
- Logging: Easily log messages and errors for debugging.
- Data Handling: Simplified methods for processing data structures.
- Configuration Management: Effortlessly manage application settings through built-in support for configuration files.
Each feature is aimed at reducing boilerplate code, allowing developers to focus on functionality.
Configuration Options
Configuring kobold.cpp is simple and essential for optimal usage. You can customize various settings through configuration files, typically formatted in JSON or XML. Here’s an example JSON configuration:
{
"logging_level": "debug",
"output_directory": "/path/to/output"
}
Refer to the kobold.cpp documentation for a comprehensive list of configurable options to tailor your experience based on your project's needs.
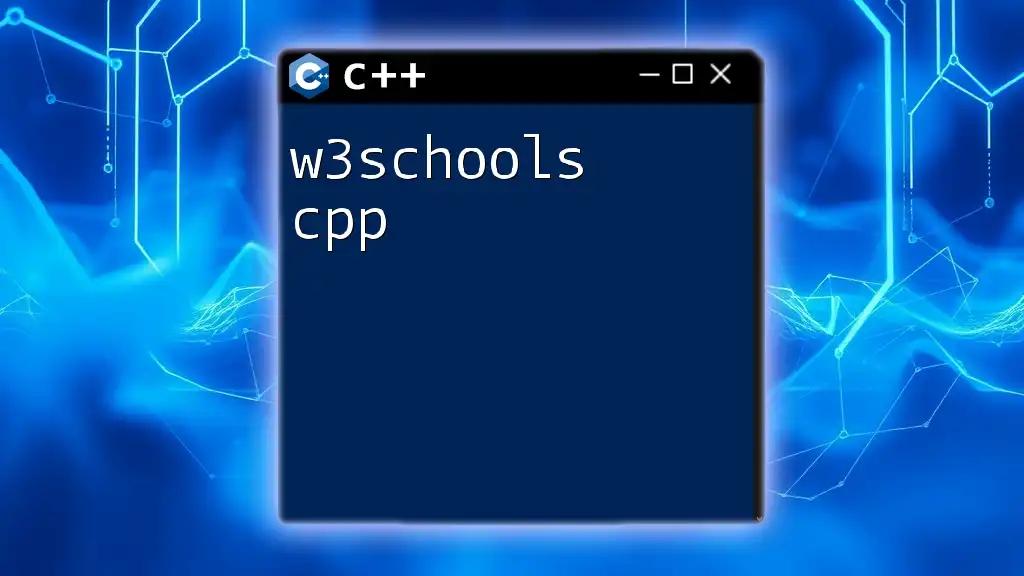
Working with kobold.cpp Commands
Basic Commands Overview
At the heart of kobold.cpp are several fundamental commands that facilitate various tasks. For example, logging messages can be done with:
kobold::log("This is a log message");
This command provides a clear and straightforward way to output logs without manually handling complex logging systems.
Advanced Command Usage
As you delve deeper into kobold.cpp, you can leverage advanced commands to enhance your applications further. For instance, to handle data processing with ease:
auto result = kobold::processData(inputData);
In this example, `processData` would automatically handle common data manipulation tasks, allowing you to skip writing repetitive code.
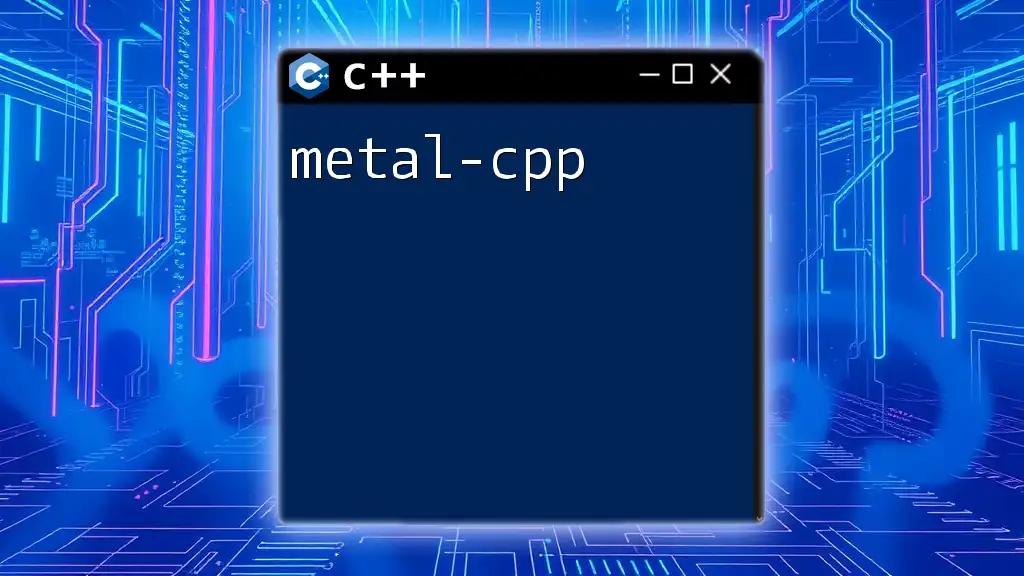
Example Applications of kobold.cpp
Creating a Simple Application Using kobold.cpp
Building a simple application is a great way to see kobold.cpp in action. Below is a short guide to creating a console application that logs user input:
#include "kobold.h"
#include <iostream>
int main() {
kobold::initialize();
std::string userInput;
std::cout << "Enter a message: ";
std::getline(std::cin, userInput);
kobold::log(userInput); // Log the user's input
return 0;
}
This application initializes kobold.cpp, prompts the user for input, and logs the message. It's a great starting point for building more complex programs.
Integrating kobold.cpp with Other Libraries
Integrating kobold.cpp with other C++ libraries allows you to create a more robust application architecture. For example, if you're working with a library like Boost, you can combine their functionalities seamlessly:
#include <boost/algorithm/string.hpp>
#include "kobold.h"
int main() {
kobold::initialize();
std::string input = "hello, world!";
boost::to_upper(input); // Convert to uppercase using Boost
kobold::log(input); // Log the transformed input
return 0;
}
This snippet demonstrates how to take advantage of both libraries, showcasing the flexibility of kobold.cpp in your projects.
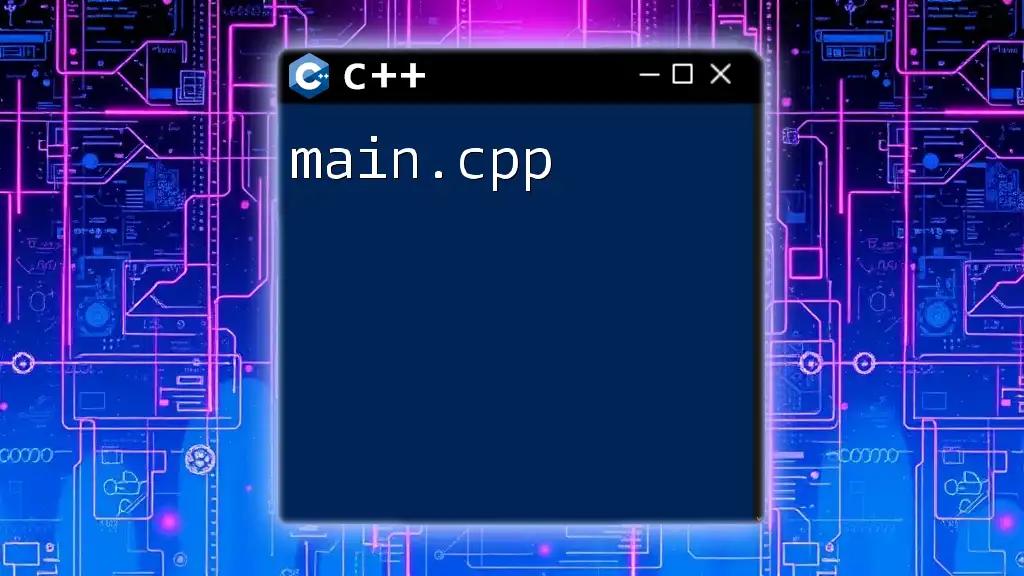
Troubleshooting and Common Issues
Common Problems in Using kobold.cpp
As with any library, users may encounter issues. Common problems include:
- Compilation Errors: Often related to missing headers or incorrect paths.
- Configuration Issues: Misconfigured settings can lead to unexpected behavior in your application.
FAQs
Q: How do I report bugs in kobold.cpp?
A: You can report bugs through the issue tracker on the kobold.cpp GitHub repository.
Q: Are there any community resources I can leverage?
A: Yes, the kobold.cpp community is active on forums such as Stack Overflow and Reddit, providing ample resources and support.
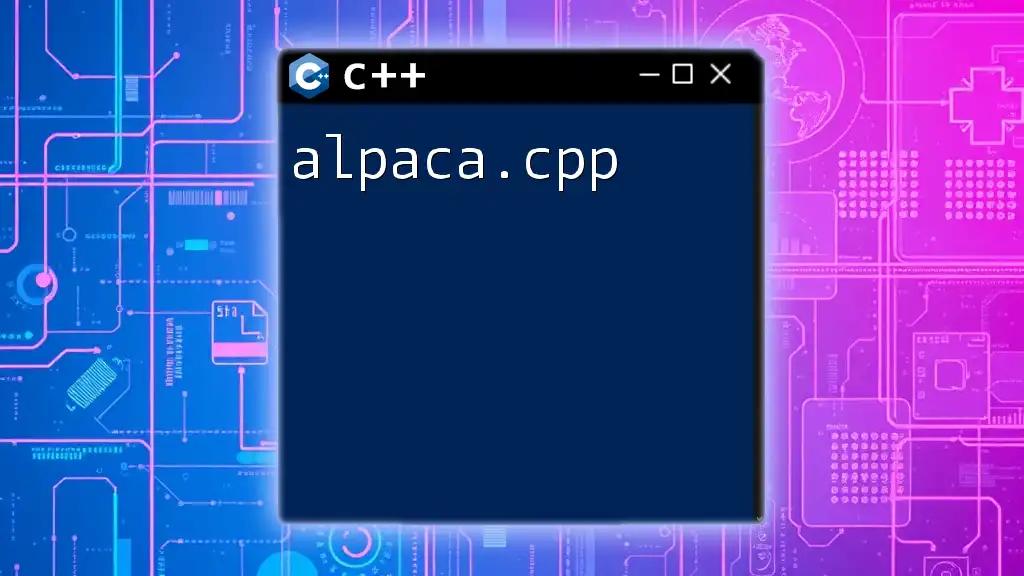
Conclusion
In summary, kobold.cpp offers robust tools designed to improve C++ development practices. By integrating its features into your projects, you can streamline coding workflows, debug effortlessly, and enhance overall productivity.
Getting Involved with the kobold.cpp Community
Engaging with the kobold.cpp community is highly encouraged. Join forums, contribute to discussions, or even participate in development on GitHub.
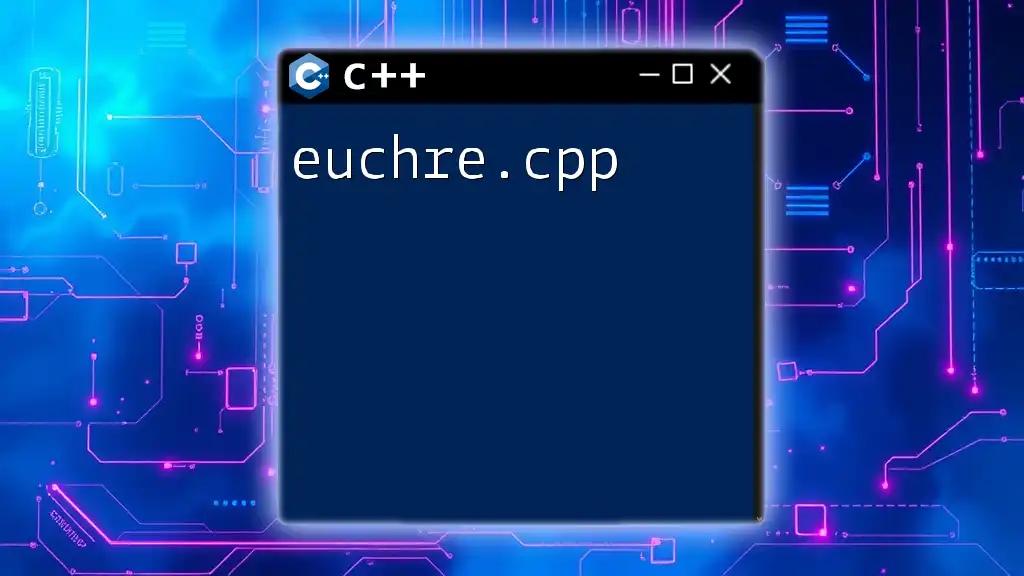
Additional Resources
Recommended Reading
If you're eager to learn more about kobold.cpp and enhance your C++ skills, consider visiting:
- The official documentation for kobold.cpp.
- Online C++ programming courses.
- Books focusing on modern C++ practices.
Tools and Libraries for C++ Development
To take your projects to the next level, explore popular development tools and libraries that complement kobold.cpp, such as:
- CMake for build management.
- gtest for unit testing.
- Boost for additional standard libraries.
By utilizing these resources and tools, you'll not only enhance your productivity but also your mastery of C++ development with kobold.cpp.