The "Hello, World!" program in C++ is a simple demonstration of the language syntax, showcasing how to output text to the console.
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
The Structure of a C++ Hello World Program
What is a C++ Hello World Program?
The Hello World program is often the first piece of code that a beginner encounters when learning a new programming language. It serves as a simple introduction to basic syntax and the structure of a program. Historically, the Hello World program signifies the beginning of a programmer's journey, illustrating how to print text to the screen. In C++, it sets the foundation for more complex concepts to be built upon.
Basic Components of C++ Code
Understanding the basic structures in C++ is critical to writing effective code. Each program typically consists of the following elements:
- Header Files: These provide necessary functionalities to the program. For example, `#include <iostream>` allows the program to perform input and output operations.
- Main Function: Every C++ program must have a `main` function where the execution starts.
- Statements and Expressions: The core actions and calculations that the program performs.
Before diving into the code, one should be familiar with the syntax of a simple C++ program, which begins with the include directive and the `main` function.
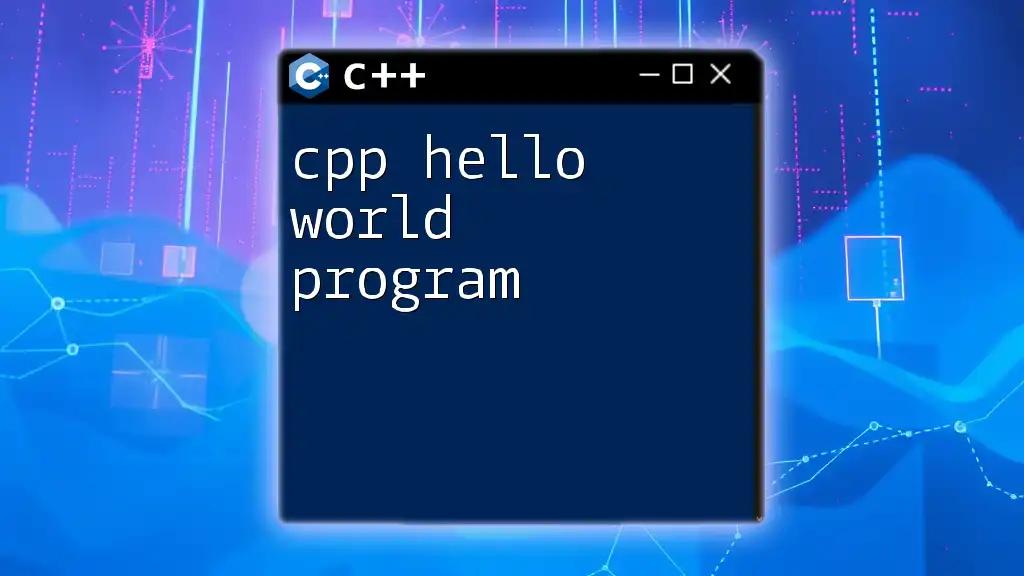
Writing Your First Hello World Program in C++
Step-by-step Code Snippet
Here’s a simple implementation of a Hello World program in C++:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Let’s break down this code:
- Line 1: The directive `#include <iostream>` tells the compiler to include the standard input-output stream library necessary for using `std::cout`.
- Line 2: The `int main()` function is the entry point for any C++ program. It returns an integer value to the operating system.
- Line 3: The statement `std::cout << "Hello, World!" << std::endl;` prints the string "Hello, World!" to the console. `std::cout` is used for output, and `std::endl` adds a newline and flushes the output buffer.
- Line 4: The `return 0;` statement indicates that the program has completed successfully.
Compiling and Running the Hello World Program
To see your Hello World program in action, you need to compile and run it. Here’s how to do this step-by-step:
-
Choose an IDE or Text Editor: Popular choices for writing C++ code are Code::Blocks, Visual Studio, or using a simple text editor along with the command line.
-
Saving Your Code: Save your code in a file named `hello.cpp`.
-
Compiling the Code: If you are using a terminal, you can compile the code using the following command:
g++ hello.cpp -o hello
-
Running the Program: After successful compilation, you run your program with this command:
./hello
By following these steps, you should see "Hello, World!" printed on the console.
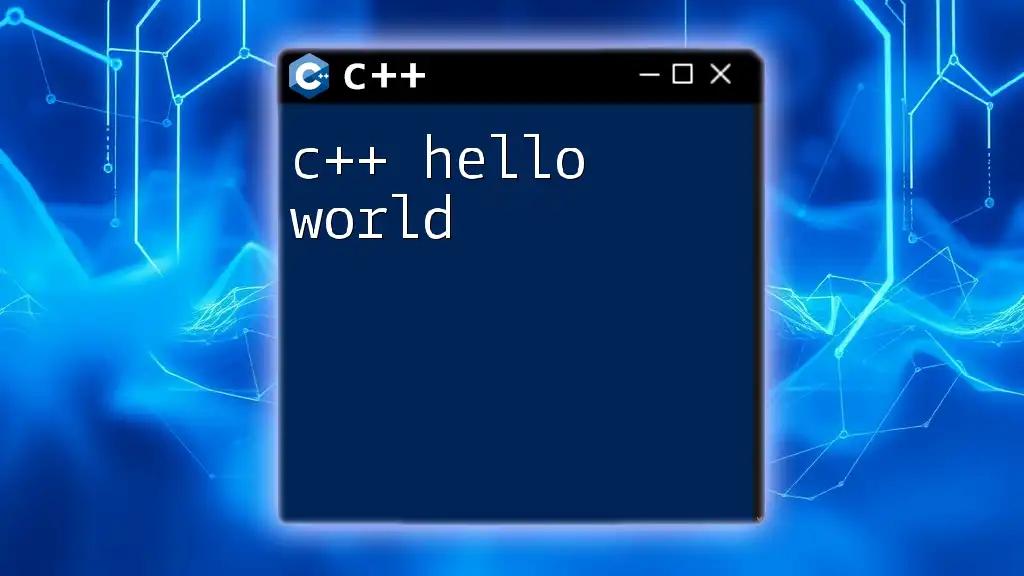
Common Errors and Troubleshooting
Common Mistakes in C++ Hello World Code
Even a simple Hello World program can lead to common mistakes:
- Missing `#include <iostream>`: Forgetting to include this header results in compilation errors related to undefined symbols.
- Incorrect usage of `std::cout` or `std::endl`: Typographical errors or forgetting to use the `std::` namespace will lead to errors.
- Not returning an integer from `main`: Omitting the return statement can cause warnings or affect the program's exit status.
Debugging Tips
Learning how to debug effectively is essential:
- Reading Compiler Errors: Begin by reviewing any error messages provided by the compiler. They often include the line number and a description of the issue.
- Checking Syntax and Structure: Ensure you've followed C++ syntax rules. Every statement must end with a semicolon.
- Using Online Resources: Websites like Stack Overflow or C++ tutorials often provide solutions and discussions on common issues.
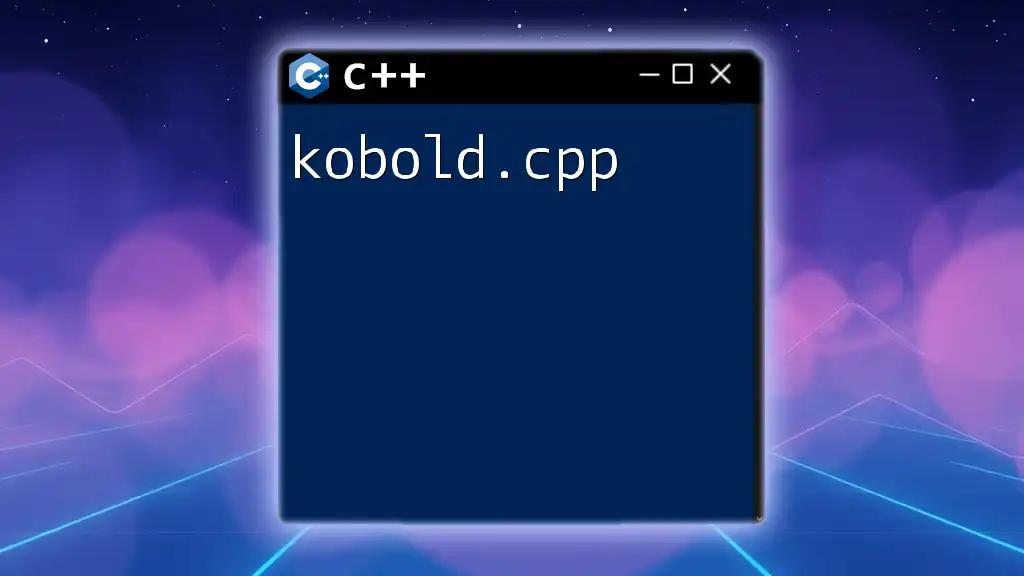
Variants of Hello World in C++
Modifying the Output
Changing the output is a fun way to experiment with your program:
std::cout << "Hello, C++!" << std::endl;
You can modify the print statement to display different messages.
If you'd like to print "Hello, World!" multiple times, you can use a loop, such as:
for(int i = 0; i < 5; ++i) {
std::cout << "Hello, World!" << std::endl;
}
This code snippet demonstrates how to utilize loops in C++, providing a simple introduction to repetitive tasks within your code.
Creating a Function for Hello World
Turning the Hello World output into a function demonstrates the importance of modular programming:
void printHello() {
std::cout << "Hello, World!" << std::endl;
}
int main() {
printHello();
return 0;
}
By using a function, you can call `printHello()` multiple times without duplicating the code, illustrating the value of creating reusable components.
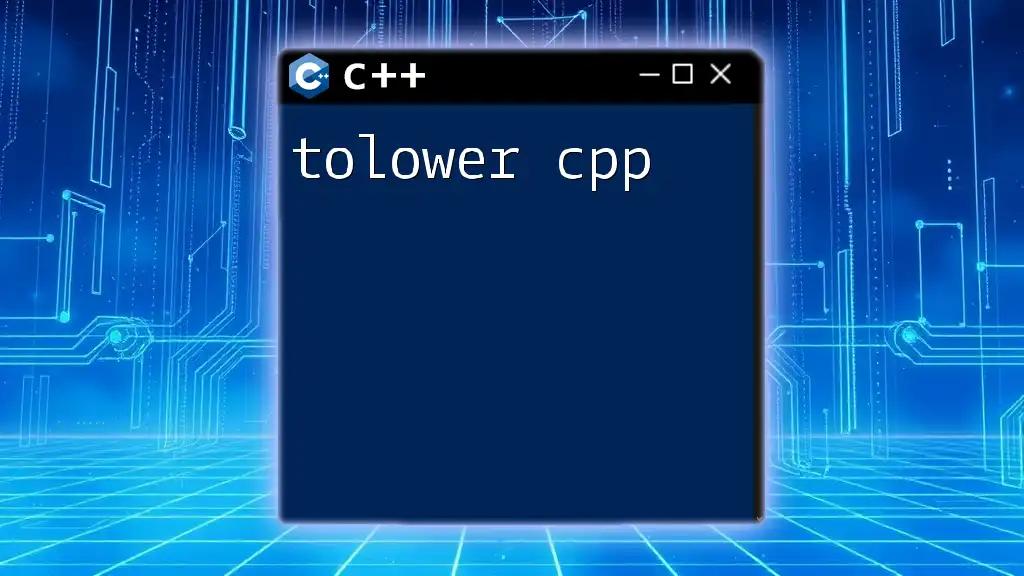
Conclusion
The Hello World program serves as a fundamental building block in learning C++. Grasping its structure and the underlying concepts paves the way for more complex programming skills. This all-important first step not only helps you understand the syntax but also instills confidence as you move into tackling more intricate C++ projects.
As you progress, remember that each error is an opportunity for learning, and every new line of code brings you one step closer to mastery. Dive deeper into the world of C++, explore its vast features, and don’t hesitate to seek help from reputable resources as you advance.
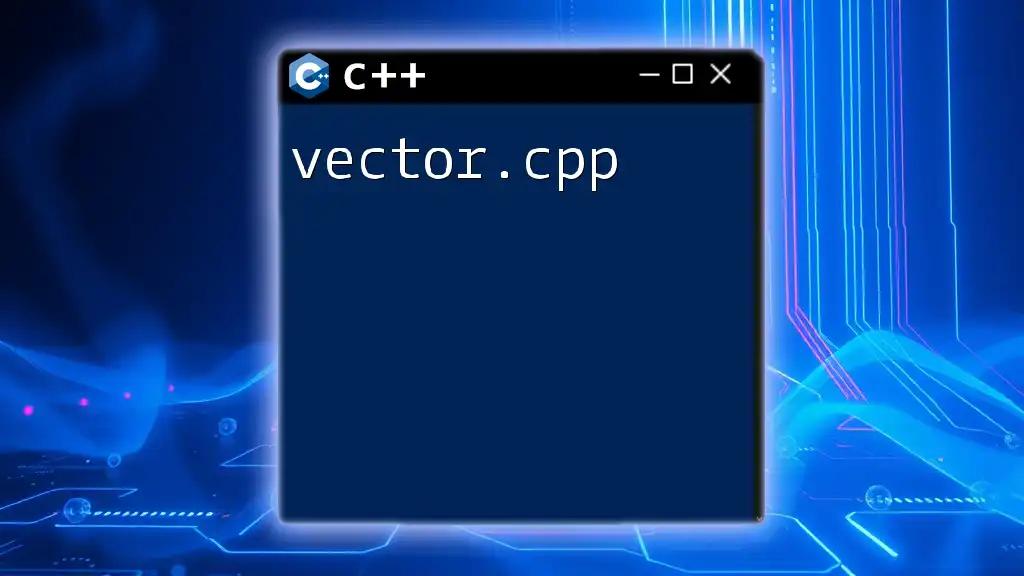
Additional Resources
To further your journey in C++, consider exploring recommended books, websites, and courses that provide a plethora of knowledge. Engaging with community forums and discussion groups will also offer invaluable insights and peer support as you enhance your programming skills. Now, let the adventure in C++ programming begin!