The `do while` loop in C++ executes a block of code at least once and then continues to execute it as long as a specified condition remains true.
#include <iostream>
using namespace std;
int main() {
int count = 0;
do {
cout << "Count is: " << count << endl;
count++;
} while (count < 5);
return 0;
}
Understanding the Basics of Loops
What is a Loop?
A loop is a fundamental concept in programming that allows a block of code to be executed repeatedly for a specified number of times or under certain conditions. The ability to automate repetitive tasks is crucial in programming, and loops serve as a powerful tool to achieve this.
Types of Loops in C++
C++ provides several types of loops that cater to different needs:
- for Loop: Best used when the number of iterations is known beforehand.
- while Loop: Suitable when the number of iterations is not predetermined but depends on a condition.
- do while Loop: A unique loop that guarantees at least one execution of the loop body regardless of the condition.
Understanding when to use each loop type is key to writing efficient and readable code.
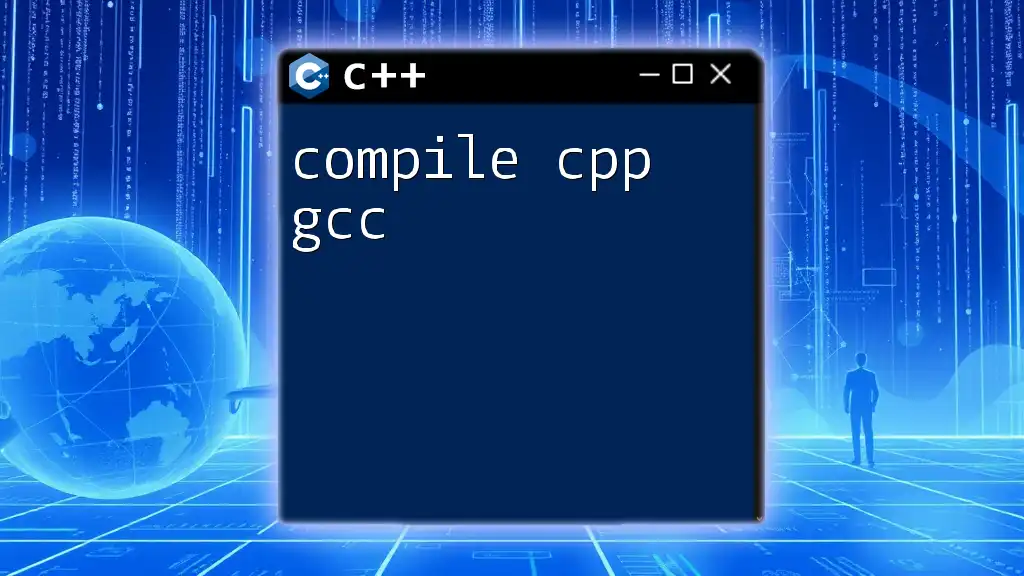
The "do while" Loop Explained
Definition of "do while"
The "do while" loop in C++ is designed to execute a block of code once before checking a specified condition. This characteristic sets it apart from a standard while loop, which checks its condition before executing the code block.
Syntax of "do while"
The basic syntax of a "do while" loop is as follows:
do {
// Code to be executed
} while (condition);
How "do while" Works
The execution flow of a "do while" loop can be broken down into clear steps:
- Execution of the Code Block: The code inside the `do` block is executed first.
- Condition Evaluation: After executing the block, the condition defined in the `while` statement is evaluated.
- Iteration Control: If the condition evaluates to true, the loop executes again. If false, the loop terminates.
This process ensures that the loop's body runs at least once, which can be particularly useful in scenarios where user interaction is required.
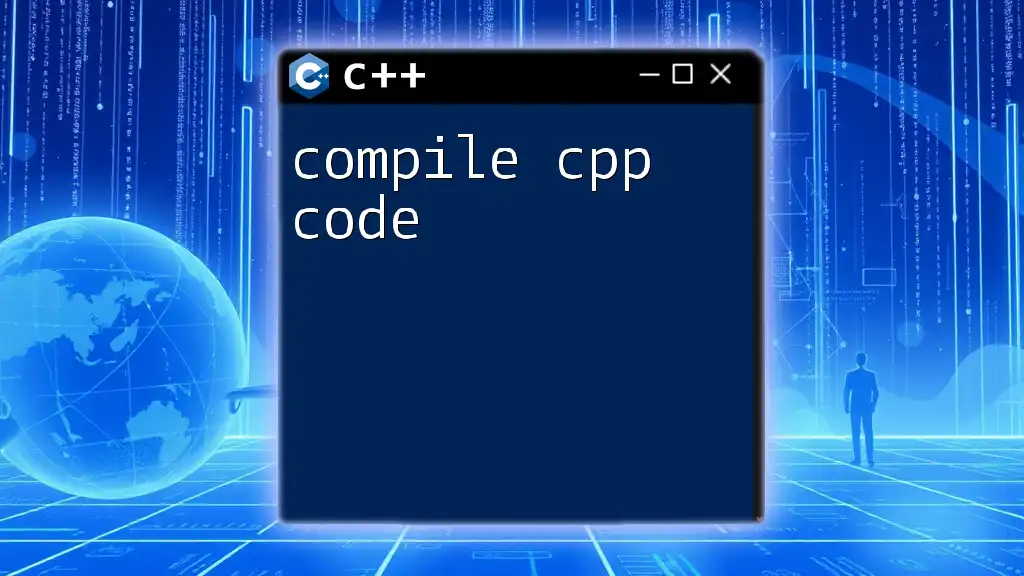
Practical Use Cases for "do while"
Common Scenarios to Use "do while"
The "do while" loop shines in situations where an operation must occur at least once before any condition is checked. It is especially beneficial for:
- User Input Validation: For scenarios where you need to guarantee that the user provides a valid input before proceeding.
- Menu-Driven Programs: Where a menu must be displayed and options processed repeatedly until the user decides to exit.
Example: User Input Validation
Consider the following example that prompts a user to enter a positive number. This example effectively demonstrates the utility of the "do while" loop:
#include <iostream>
using namespace std;
int main() {
int number;
do {
cout << "Enter a positive number: ";
cin >> number;
} while (number <= 0); // The loop continues until a positive number is entered
cout << "You entered: " << number << endl;
return 0;
}
In this code, the program ensures that the user must enter a positive number before proceeding, highlighting how the "do while" loop provides an efficient solution for input validation.
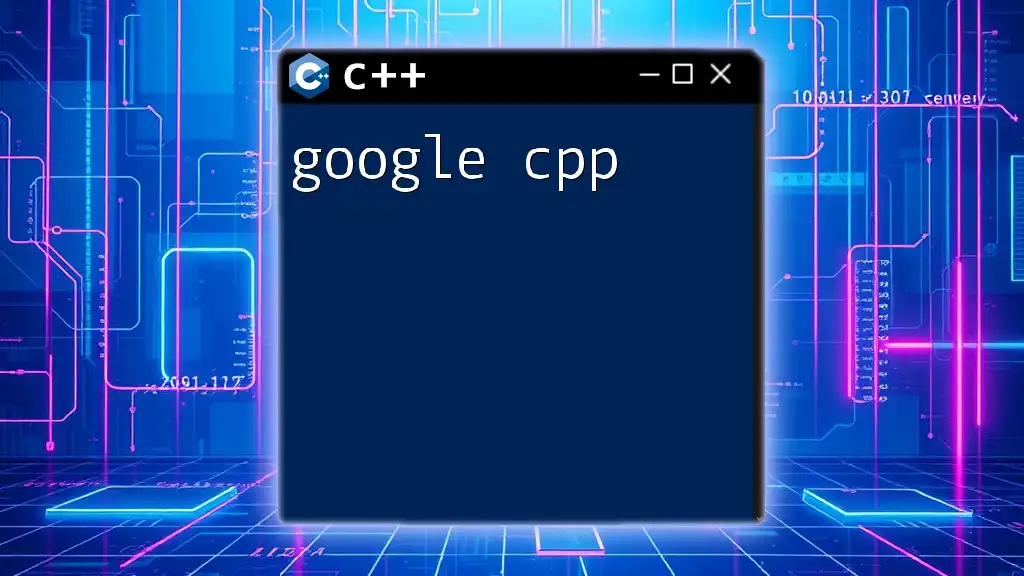
Advantages and Disadvantages of Using "do while"
Advantages
One of the main advantages of using a "do while" loop is its guarantee of executing the code block at least once. This feature makes it simple and effective for user input scenarios where user interaction is expected. Additionally, the structure is straightforward, which can enhance readability in certain contexts.
Disadvantages
However, there are some potential drawbacks to consider. A common pitfall exists in creating infinite loops when conditions are not adequately handled. Moreover, the "do while" loop is less frequently used compared to "for" and "while" loops, which could result in a lack of familiarity for some programmers.
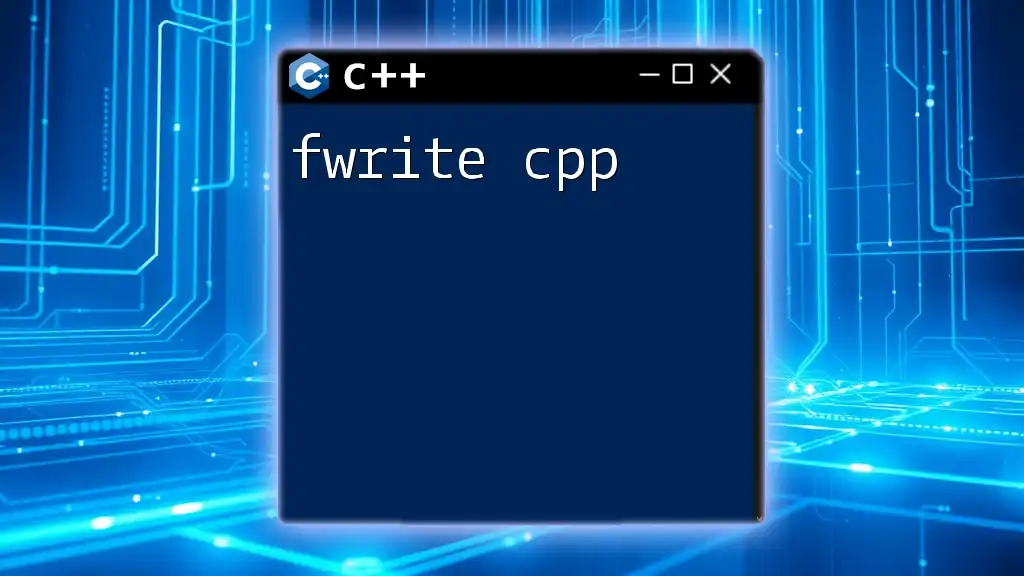
Best Practices for Using "do while" in C++
When utilizing the "do while" loop, consider these best practices:
- Clear Exit Conditions: Always ensure that your conditions are well-defined to prevent infinite loops. For example, validate user inputs continuously until the correct format is achieved.
- Avoid Uninitialized Variables: Initialize loop control variables before entering the loop to prevent unexpected behavior.
- Documentation: Comment your code where necessary to explain the purpose of the loop, especially if it contains complex conditions.
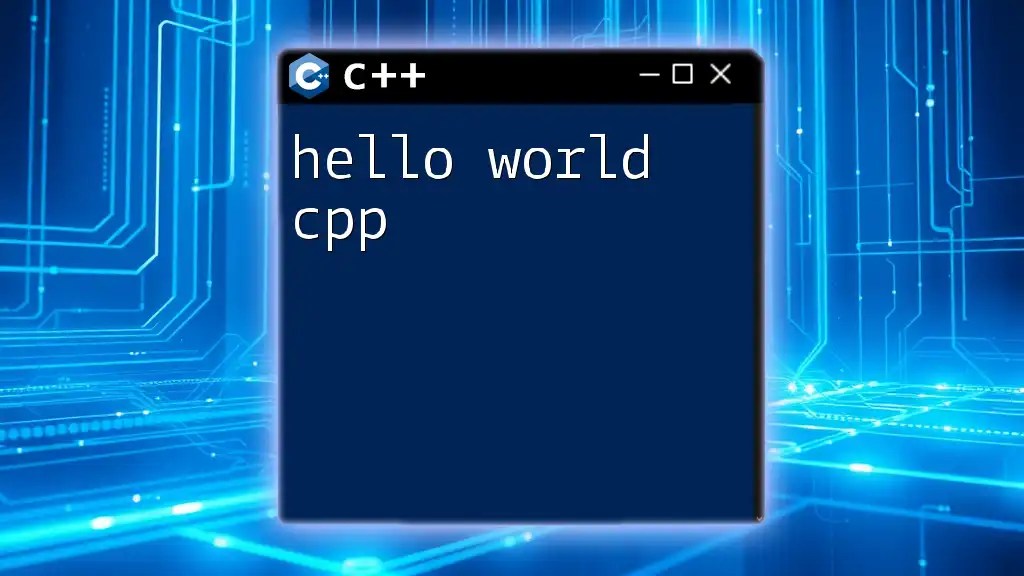
Comparison: "do while" vs. "while" Loops
Similarities
Both the "do while" and "while" loops serve the purpose of repeating a block of code based on the evaluation of conditions. They are integral to controlling the flow of execution in C++ programs.
Differences
The main difference lies in their execution timing:
- A "do while" loop executes its body at least once before checking the loop's condition.
- A "while" loop checks the condition first, which may lead to zero executions if the condition evaluates to false initially.
Example Comparison
To illustrate these differences, consider the following code snippets:
// A "do while" loop
int i = 0;
do {
cout << i << endl;
i++;
} while (i < 5);
// A "while" loop
int j = 0;
while (j < 5) {
cout << j << endl;
j++;
}
In the above examples, the "do while" loop will output `0` through `4`, as it guarantees the initial execution. Meanwhile, the "while" loop will achieve the same result, but it functions differently, confirming beforehand that `j` is less than `5`.
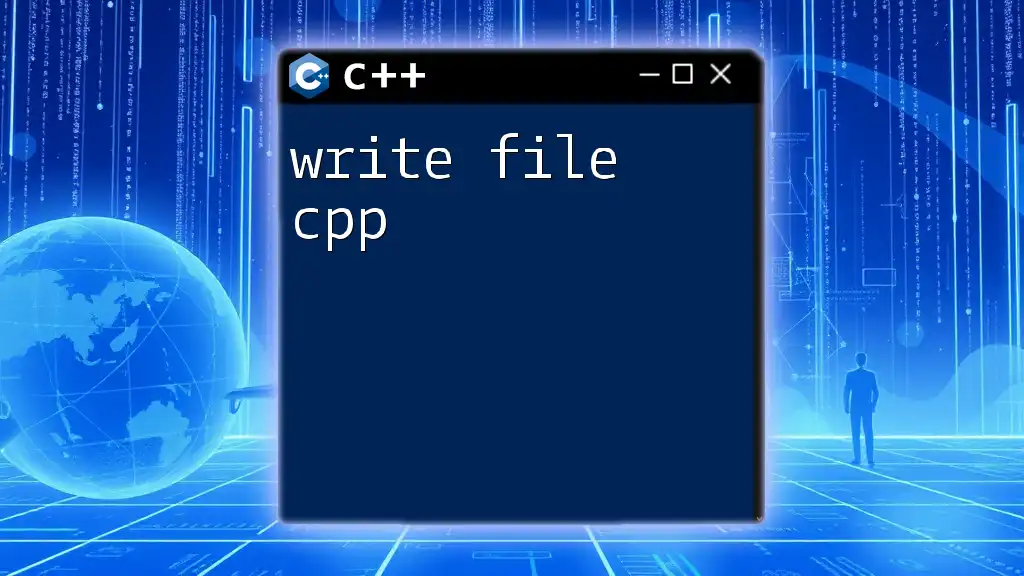
Common Mistakes to Avoid with "do while"
When using the "do while" loop, it’s essential to avoid common mistakes such as:
- Forgetting to Initialize Loop Variables: Such neglect can lead to unexpected behaviors and logical errors in your program.
- Incorrectly Forming Exit Conditions: Be meticulous with your conditions to avoid infinite loops that hinder program performance.
- Failing to Validate User Inputs: Always handle user inputs properly to ensure program stability.
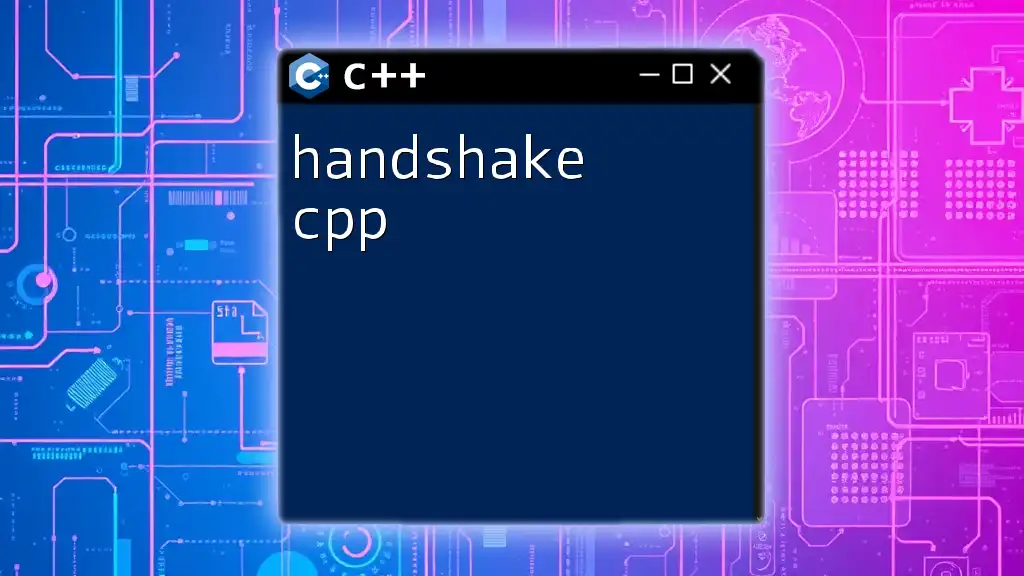
Conclusion
Understanding the "do while" loop is essential for any C++ programmer. This loop offers a unique way to ensure that your code executes at least once, which is invaluable in scenarios demanding user interaction or validation. Through careful implementation and awareness of potential pitfalls, you can leverage the "do while" loop to improve your coding efficacy.
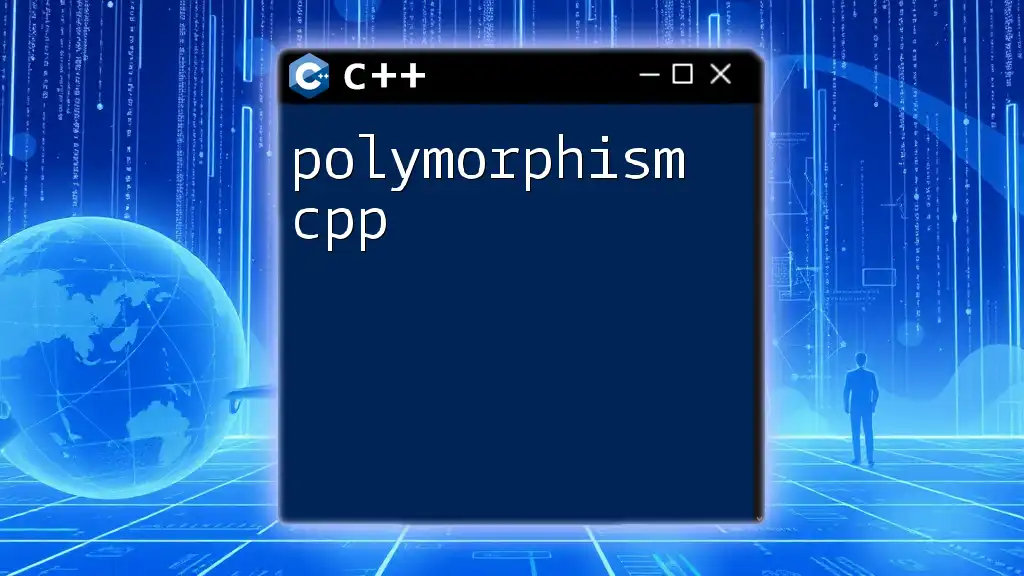
Additional Resources
For those looking to dive deeper into C++ loops, consider exploring further reading materials or practicing on online coding platforms that allow you to experiment with various loop constructs.
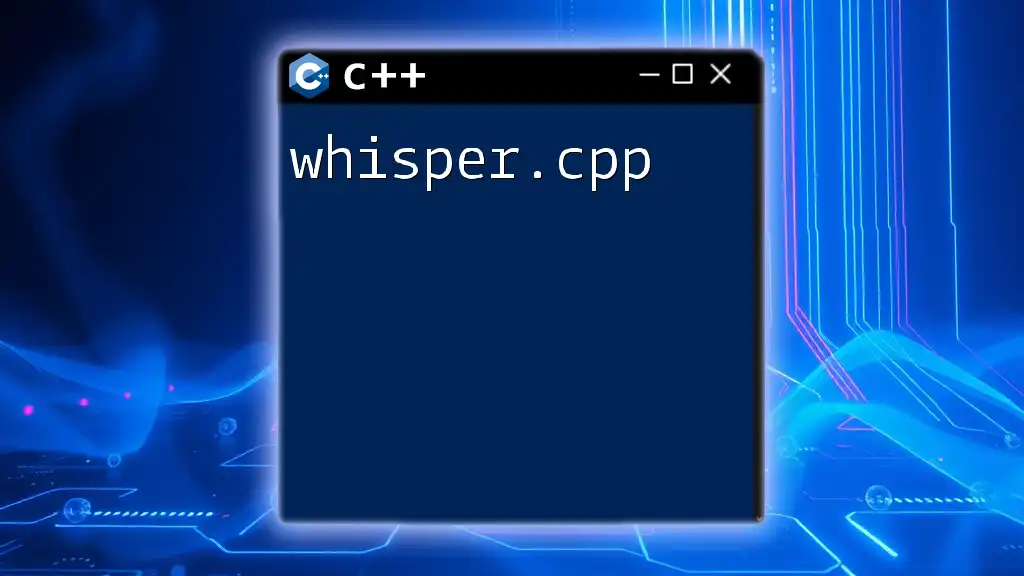
Call to Action
Now that you have a comprehensive understanding of the "do while" loop in C++, it's time to start experimenting! Implement "do while" loops in your projects to validate inputs, create clear user interfaces, and enhance overall program interactivity. Don’t hesitate to share your experiences or any questions you might have in the comments section!