In C++, a `while true` loop creates an infinite loop that continuously executes the code within its block until explicitly broken out of, typically using a `break` statement.
while (true) {
// Your code here
if (someCondition) {
break; // Exit the loop if someCondition is met
}
}
Understanding Infinite Loops
An infinite loop is a loop that continues to execute indefinitely, meaning it doesn’t have a termination condition that causes it to exit. While generally considered a programming flaw, infinite loops can serve specific purposes when applied correctly. A common instance is using them to keep a program running until a specific event occurs—such as monitoring input or controlling a service.
Scenarios Where Infinite Loops are Useful
Infinite loops are essential in several programming contexts, such as:
- Monitoring systems: Where a service needs to consistently check for data or events.
- Event-driven architectures: To handle user interactions for graphical user interfaces (GUIs).
- Server applications: For continuously accepting connections from clients.
However, coding with infinite loops requires caution, as they can lead to performance bottlenecks or application crashes if not properly managed.
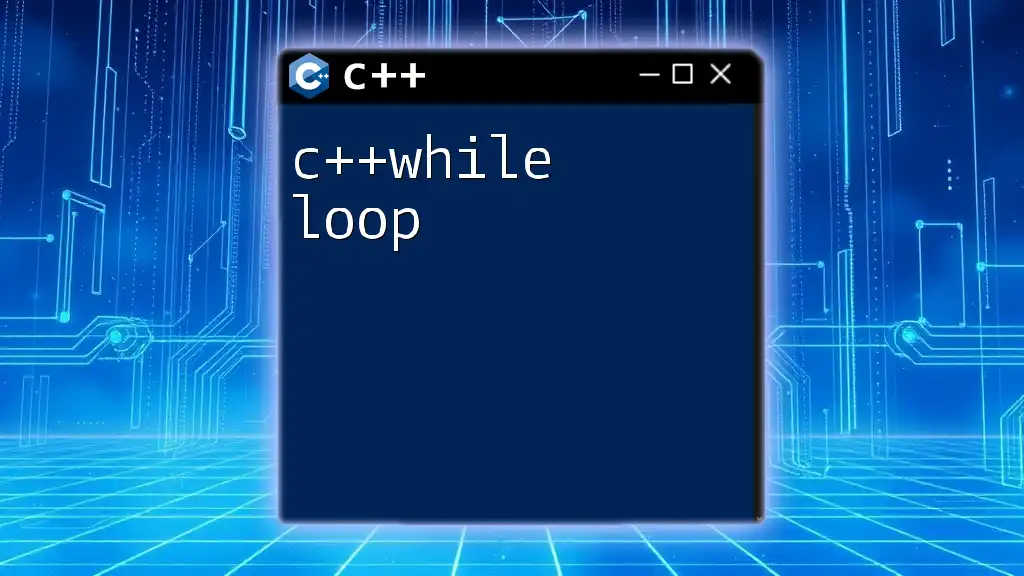
The "while true" Statement in C++
What is "while true"?
In C++, the statement `while (true)` creates a loop that will run continuously since the condition provided (`true`) is always satisfied. The syntax follows a basic structure:
while (true) {
// Your code here
}
This simplicity forms the backbone of many applications where persistent execution is required.
Basic Structure of a "while true" Loop
Here’s a straightforward example of how a "while true" loop can be implemented:
while (true) {
std::cout << "This loop will run indefinitely." << std::endl;
}
In this case, the program outputs a message repeatedly without stopping, demonstrating the perpetual nature of this construct.
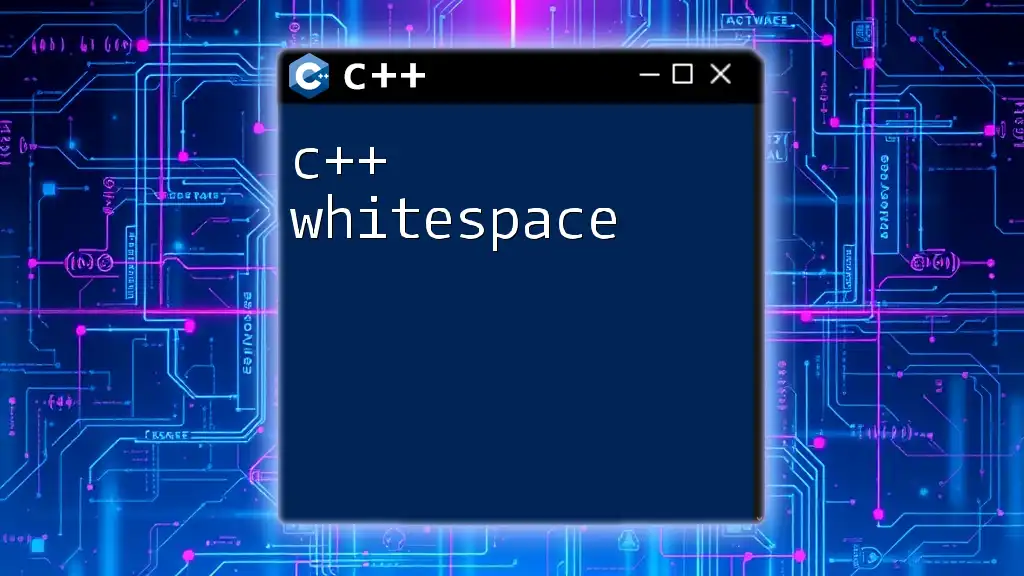
Controlling Flow within a "while true" Loop
Managing the flow within an infinite loop is crucial to prevent unwanted behaviors.
Breaking out of the Loop
To exit a "while true" loop gracefully, you can use the `break` statement.
while (true) {
std::cout << "Press 0 to exit the loop." << std::endl;
int input;
std::cin >> input;
if (input == 0) {
break; // Exit the loop when user presses 0
}
}
In this example, the loop continuously prompts the user for input and only exits when the user enters `0`.
Using the `continue` Statement
The `continue` statement can be useful if you want to skip the current iteration and move to the next one without exiting the loop entirely.
while (true) {
int number;
std::cout << "Enter a positive number (negative to skip): ";
std::cin >> number;
if (number < 0) {
continue; // Skip the rest of the loop for negative numbers
}
std::cout << "You entered: " << number << std::endl;
}
Here, if a user enters a negative number, the loop skips the output statement and continues to prompt for input, efficiently managing user interaction.
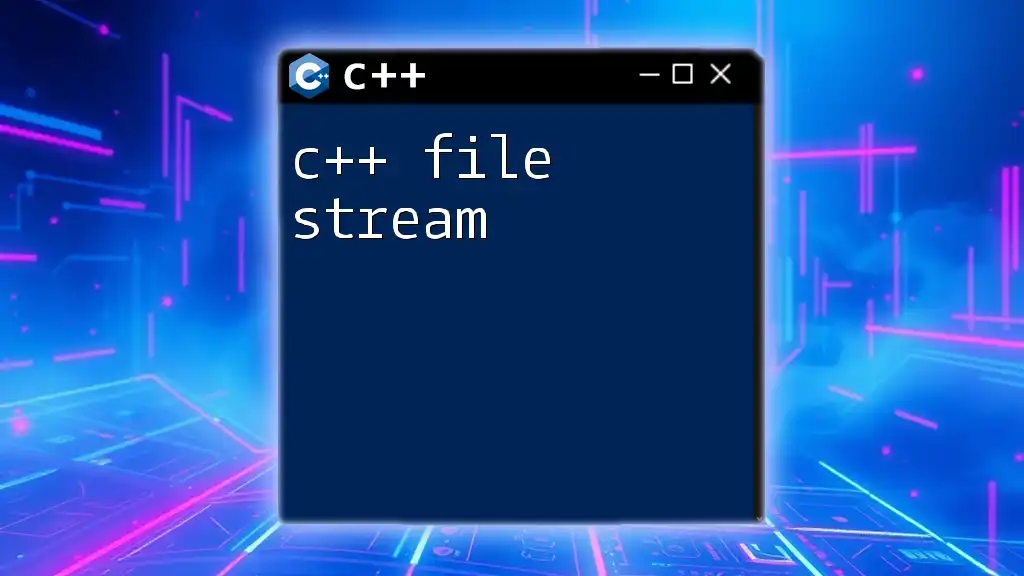
Practical Applications of "while true"
Monitoring Systems and Daemons
"while true" loops are incredibly useful in server applications that need to accept and process incoming connections continuously.
while (true) {
// Code to accept incoming connections
acceptConnection();
}
In this scenario, the server remains operational, waiting for requests from clients. The loop ensures it’s always ready to handle new connections as they arrive.
Event Listeners in GUI Applications
In GUI programming, an infinite loop can manage incoming events.
while (true) {
// Wait for user input events
processEvents();
}
This structure allows the application to respond to user inputs like clicks and keystrokes, ensuring a responsive user experience.
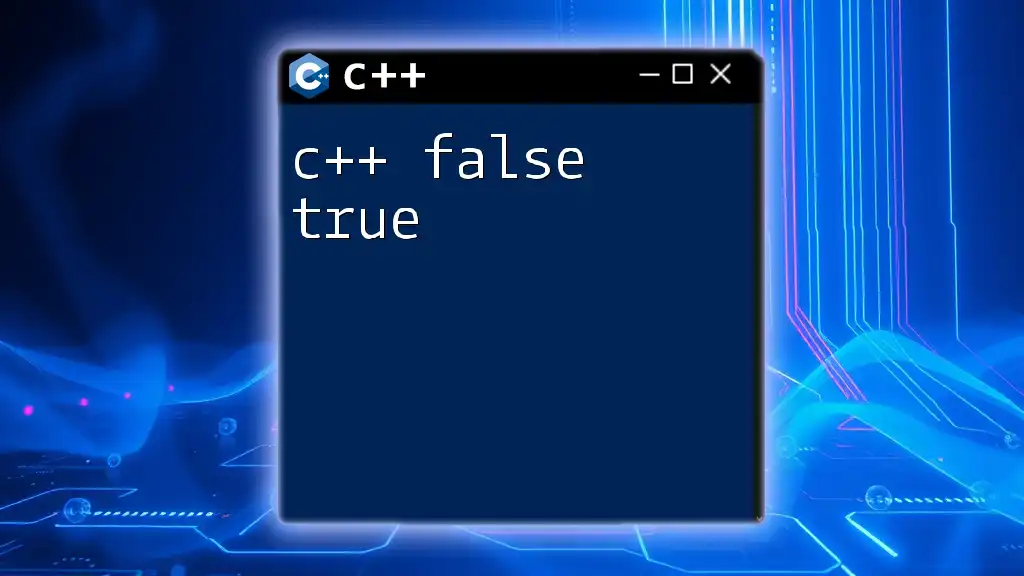
Best Practices for Using "while true"
Avoiding Infinite Loops
While `while true` can be powerful, it’s essential to set clear conditions under which the loop exits. Developers should always assess the risk of creating uncontrolled infinite loops that could affect application performance.
Memory Management
Memory management is critical when working within a "while true" loop. Always ensure that resources are appropriately allocated and freed to avoid memory leaks or exhaustion.
For instance, if you're dynamically allocating objects within the loop, make sure to deallocate them when they are no longer needed.
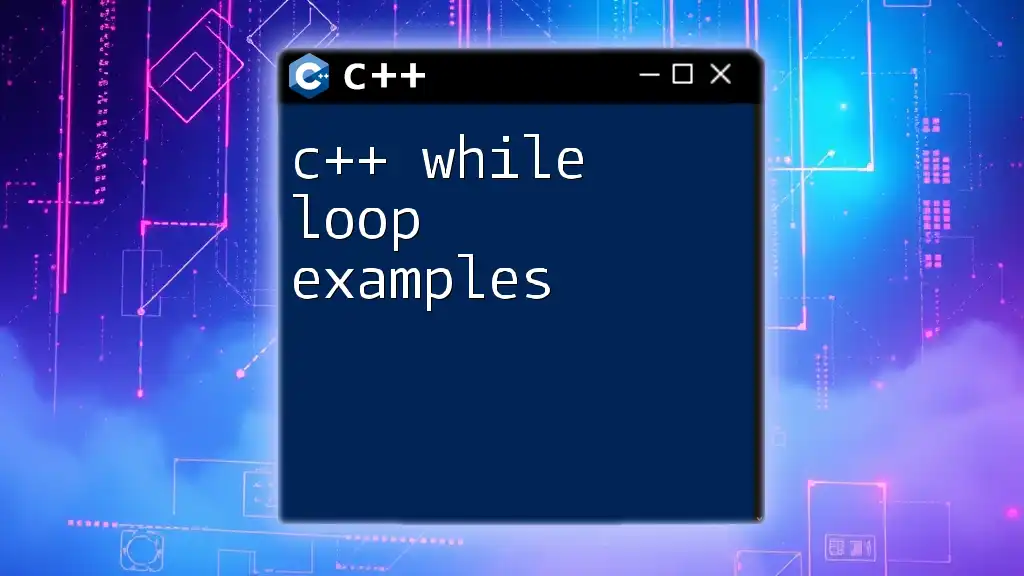
Debugging "while true" Loops
Debugging infinite loops can be challenging but necessary. Here are some techniques:
- Using print statements: Regularly outputting relevant variables can help track where the loop is executing.
- Using a debugger: Stepping through loop iterations allows you to observe the program's flow in real-time.
For example:
while (true) {
std::cout << "Looping..." << std::endl; // For debugging
}
This will print "Looping..." indefinitely, providing insight into whether your code reaches certain parts of your loop.
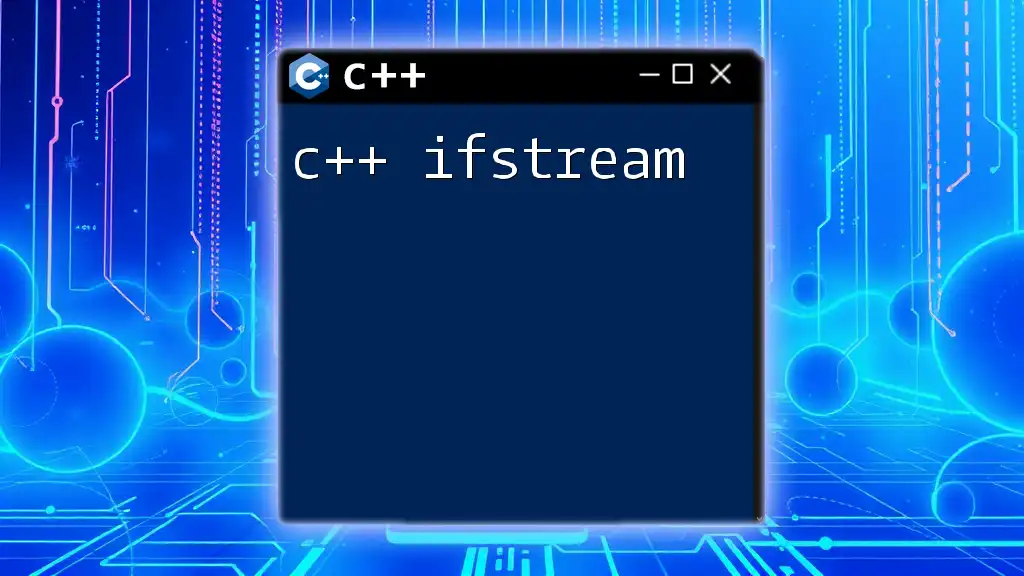
Conclusion
The "while true" construct in C++ is a fundamental building block for many programming tasks. Used judiciously, it provides a powerful way to facilitate continuous operations in software applications. Understanding how to control and debug such loops can enhance your capability as a programmer and ensure that you build efficient, reliable applications.
As you continue to explore C++, take the opportunity to experiment with these examples to gain a deeper understanding of how to wield "while true" in your projects.