C++ file streams provide a way to read from and write to files using the `ifstream` and `ofstream` classes, allowing for efficient data management in applications.
Here's a simple example of how to write to and read from a file using C++ file streams:
#include <iostream>
#include <fstream>
#include <string>
int main() {
// Writing to a file
std::ofstream outFile("example.txt");
outFile << "Hello, C++ File Stream!" << std::endl;
outFile.close();
// Reading from a file
std::ifstream inFile("example.txt");
std::string line;
if (inFile.is_open()) {
while (std::getline(inFile, line)) {
std::cout << line << std::endl;
}
inFile.close();
}
return 0;
}
Understanding File Streams in C++
What are File Streams?
File streams in C++ are essential tools for performing input and output operations on files. They enable programmers to read data from files and write data to files, effectively allowing for persistent data storage beyond the program's lifetime. Understanding how to effectively use file streams is critical for any C++ developer looking to manage file-based data efficiently.
Types of File Streams
C++ offers three primary types of file streams that serve different purposes:
ifstream (Input File Stream): This stream is designed for reading data from files. It allows you to open a file and extract information stored within it, making it suitable for applications that require data retrieval.
ofstream (Output File Stream): This stream is used for writing data to files. It allows you to create new files or overwrite existing files, making it vital for applications that generate output data.
fstream (Input/Output File Stream): This versatile stream can handle both input and output operations. It allows you to read from and write to the same file, making it exceptionally useful for tasks that need to modify existing data without needing to close and reopen the file.
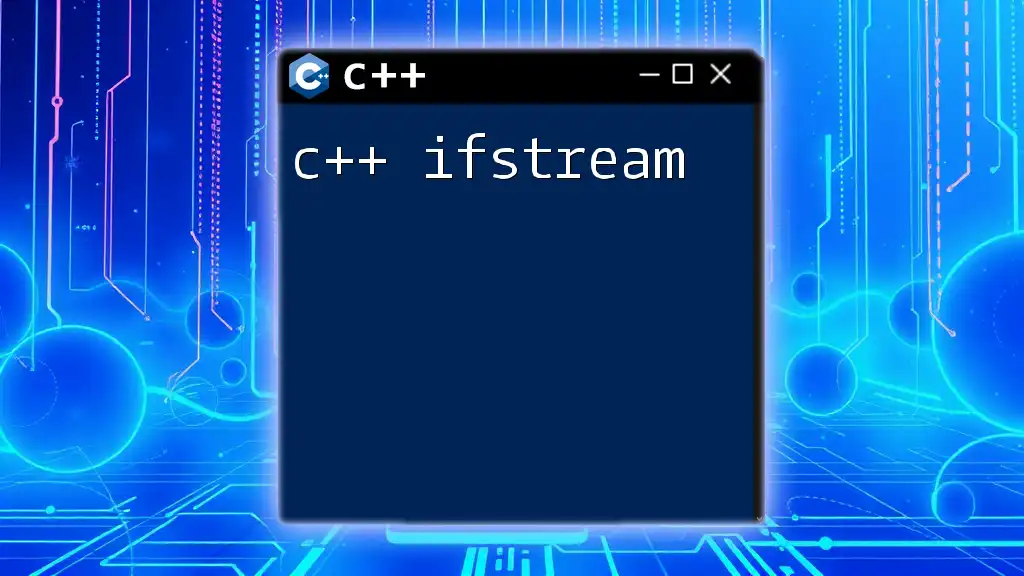
Setting Up File Streams in C++
Including the fstream Library
To begin using file streams in C++, you first need to include the `fstream` library. This library provides access to the ifstream, ofstream, and fstream classes, which are essential for file operations.
#include <fstream>
using namespace std;
Creating File Stream Objects
Creating file stream objects is straightforward. You simply define an object of the appropriate stream type, specifying the filename when necessary. Below is an example of how to create objects for reading and writing to files:
ifstream inputFile("input.txt");
ofstream outputFile("output.txt");
fstream fileStream("data.txt", ios::in | ios::out | ios::app);
Here, `inputFile` is for reading from "input.txt", `outputFile` is for writing to "output.txt", and `fileStream` is configured for both reading and appending data to "data.txt".
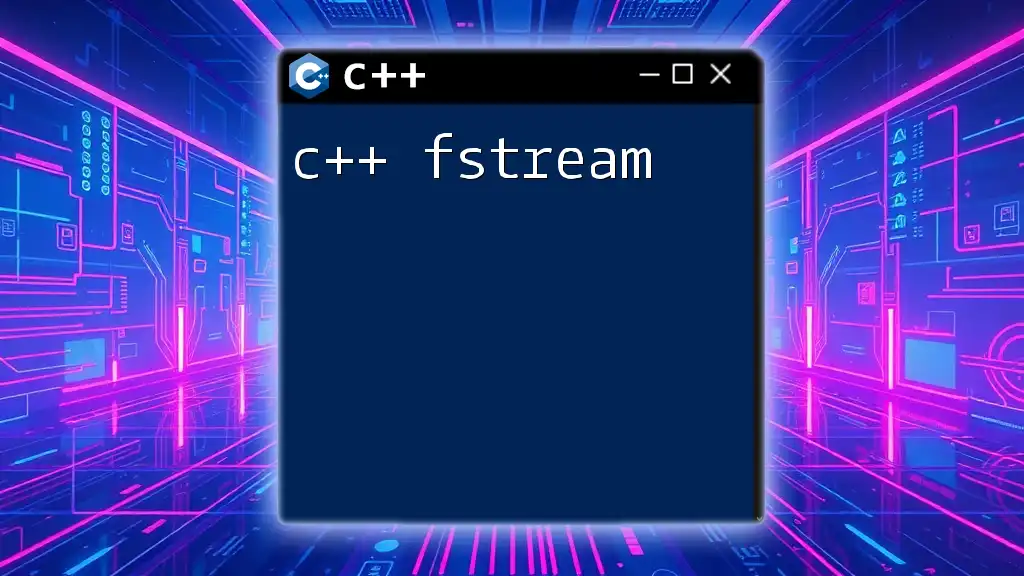
Reading from Files
Using ifstream for Input
To read data from a file using `ifstream`, you first need to open the file. After opening the file, you can employ various methods to extract data.
Common methods for reading include:
- getline(): This method reads a line from the file until it encounters a newline character.
- The >> operator: This operator allows you to extract formatted data directly from the file for various data types.
It's crucial to verify whether the file opened successfully before attempting to read data. You can do this by checking if the `ifstream` object evaluates to `true`.
Example of Reading Data from a File
Here is a simple example of reading from a file:
ifstream inputFile("data.txt");
if (!inputFile) {
cerr << "Error opening file!" << endl;
return 1;
}
string line;
while (getline(inputFile, line)) {
cout << line << endl; // Output the content
}
inputFile.close();
This code first checks if "data.txt" opened correctly. If it did, the program reads each line and outputs it to the console.
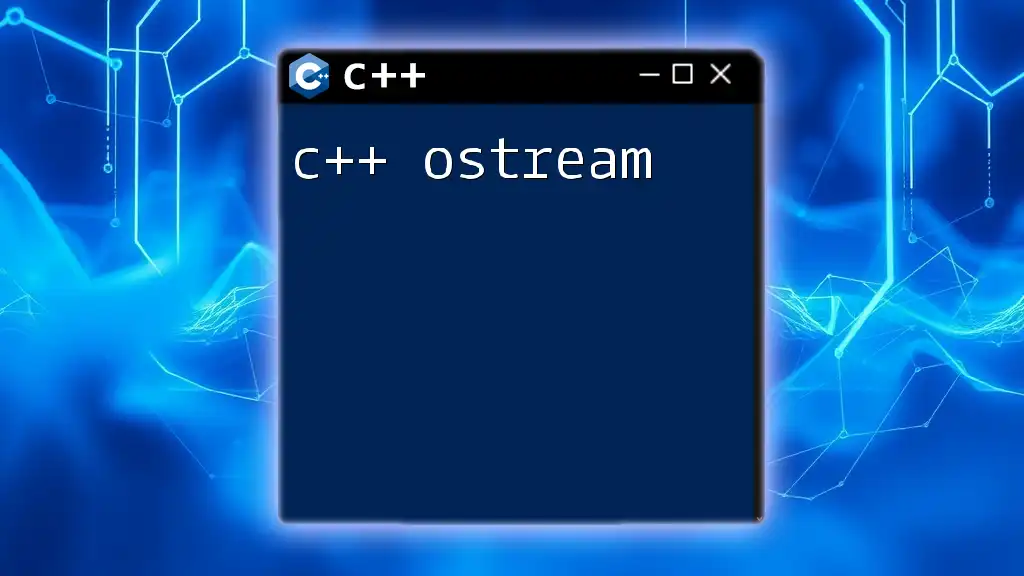
Writing to Files
Using ofstream for Output
When it comes to writing data to a file, the `ofstream` class plays a crucial role. You need to ensure that the target file is open, and then you can begin writing data.
Used methods for writing include:
- The << operator: Utilized for outputting text, similar to how you would print to the console.
- Control on whether to append or overwrite data by choosing the appropriate flags while opening the file.
Make sure to handle errors by checking if the `ofstream` object is valid after attempting to open the file.
Example of Writing Data to a File
Here is an example demonstrating how to write to a file:
ofstream outputFile("output.txt");
if (!outputFile) {
cerr << "Error opening file!" << endl;
return 1;
}
outputFile << "Hello, World!" << endl;
outputFile.close();
In this snippet, "output.txt" is checked to ensure it opened correctly, and a simple string is written to the file before closing the stream.
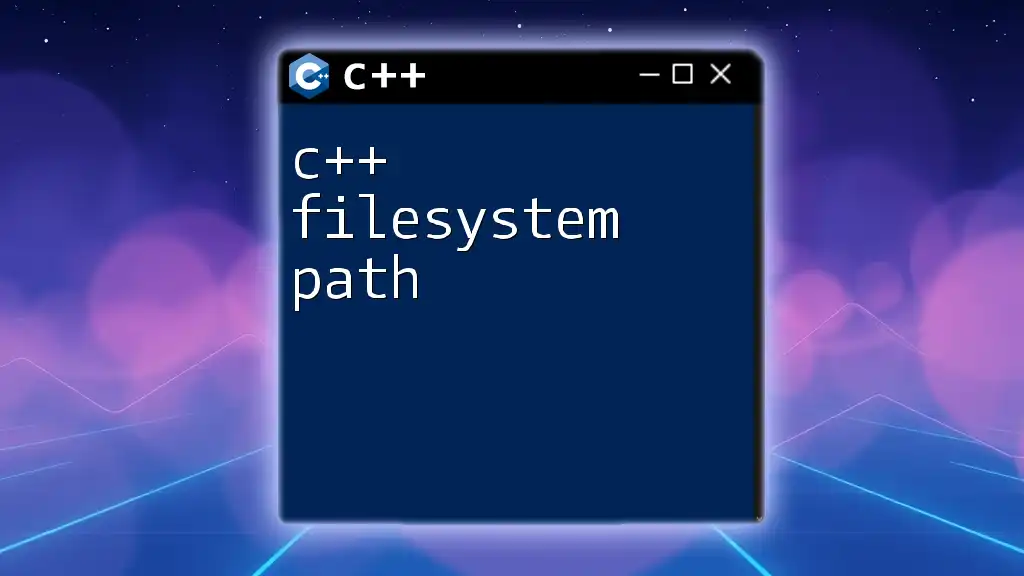
Working with fstream
Using fstream for Input/Output Operations
The `fstream` class allows you to perform both input and output operations on the same file without the need to close and reopen it. This is particularly useful when you need to modify existing data or maintain the state of a file while reading and writing.
Example of Reading and Writing with fstream
Here is an example of how you can read from and write to a file using `fstream`:
fstream fileStream("example.txt", ios::in | ios::out | ios::app);
if (!fileStream) {
cerr << "Error opening file!" << endl;
return 1;
}
string line;
while (getline(fileStream, line)) {
cout << line << endl; // Reading existing content
}
fileStream << "Appending this line to the file." << endl; // Writing new content
fileStream.close();
In this example, the program reads existing content from "example.txt" and then appends a new line to it before closing the stream.
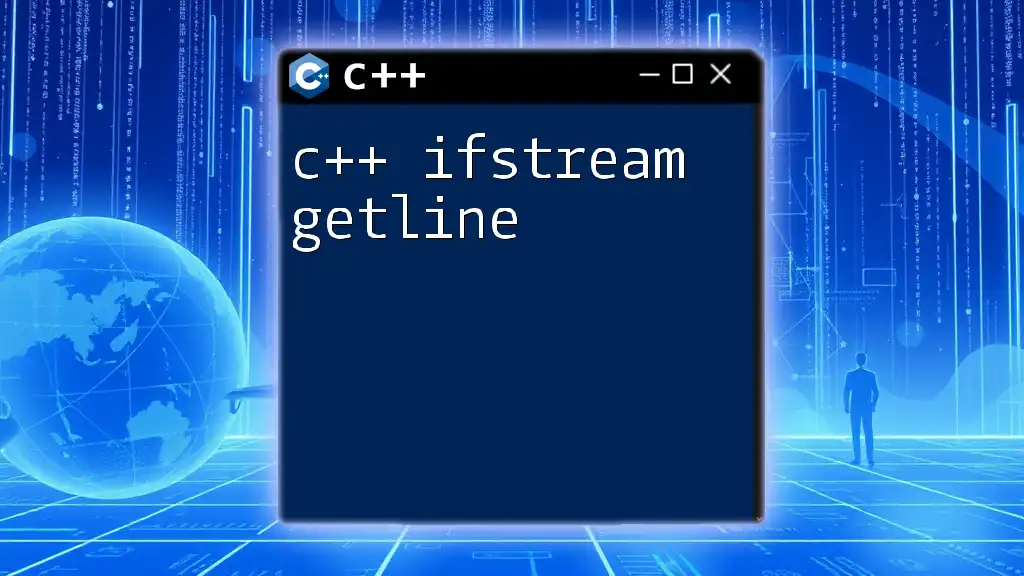
File Stream Manipulators
Overview of Common Manipulators
C++ provides manipulators to control output formatting. Some common manipulators include:
- std::endl: Used to insert a newline and flush the output buffer.
- std::setw: Controls the width of the output for better column alignment.
- std::setprecision: Sets the number of decimal places for floating-point numbers.
Using Manipulators in File Output
You can use manipulators within your file output operations for enhanced formatting. Here's an example:
outputFile << fixed << setprecision(2) << 123.456; // Writing a float with precision
This line ensures that when writing a floating-point number to the file, it is formatted to two decimal places.
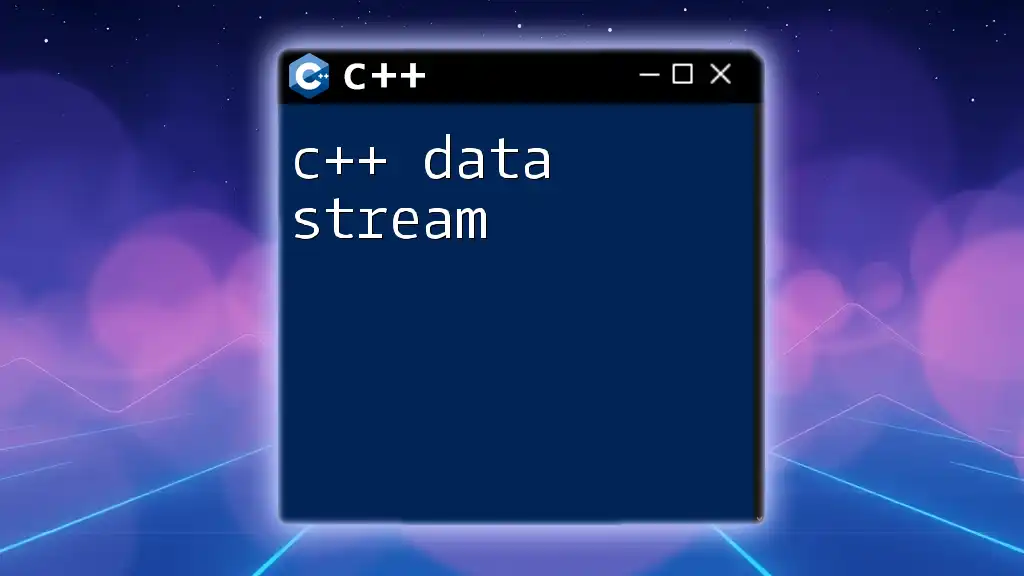
Best Practices for File Handling
Ensuring Proper File Closure
Always ensure that files are properly closed after operations. This helps to avoid data corruption and resource leaks. Using RAII (Resource Acquisition Is Initialization) can help with automatic management of resources.
Error Handling and Debugging
Anticipating errors during file operations is crucial. Always check whether the file stream is valid and handle errors gracefully by providing meaningful feedback to the user. Common issues include file not found, permissions error, and disk space limitations.
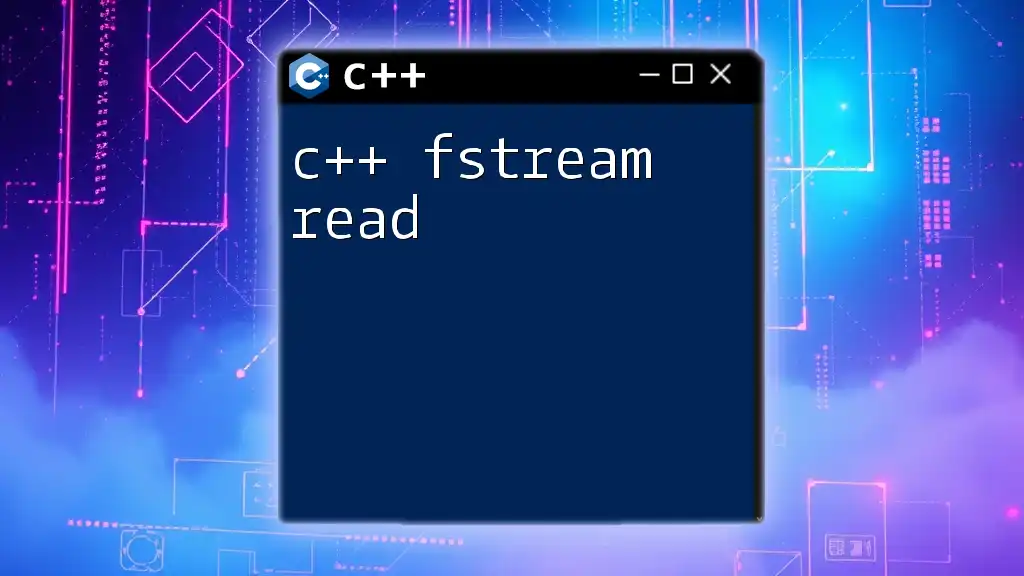
Conclusion
Recap of Key Points
Understanding how C++ file streams work is essential for managing file input and output efficiently. By mastering ifstream, ofstream, and fstream, you can complete a variety of tasks related to file handling in your applications.
Call to Action
Now that you have an overview of using C++ file streams, take some time to practice your skills. Experiment with reading and writing data through different streams, and feel free to explore additional resources to expand your knowledge further.
Additional Resources
Look for documentation, tutorials, and coding communities dedicated to C++ file streams to deepen your expertise and address any questions that arise as you continue your programming journey.