The `ostream` is a class in C++ used for output stream operations, allowing you to send data to output devices, such as the console or files.
Here's a simple example of using `ostream` to print a message to the console:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl; // Using ostream to print to console
return 0;
}
What is ostream?
`ostream` is a class in C++ that represents output streams. It is a part of the C++ Standard Library and serves as an essential component for handling output operations in a straightforward manner. The primary function of `ostream` is to allow developers to output data to various destinations, such as the console, files, or other output devices.
Why Use ostream?
Utilizing `ostream` in C++ brings several benefits:
-
Ease of Use: `ostream` provides a user-friendly interface for outputting data, allowing developers to write simpler and more readable code.
-
Type Safety: The functions in `ostream` are type-safe, meaning they can handle a variety of data types without implicit conversions or type errors.
-
Stream Manipulation: With `ostream`, you can control the format of your output using various manipulators.
The ostream Class
The `ostream` class is defined within the `<ostream>` header file. All output streams in C++ are derived from `ostream`, including the standard output stream `std::cout`. The class contains various member functions and overloaded operators to support output operations.
To create an `ostream` object, you typically don’t need to instantiate the class directly, as the C++ standard library provides pre-defined instances like `std::cout` which can be used directly for output operations.
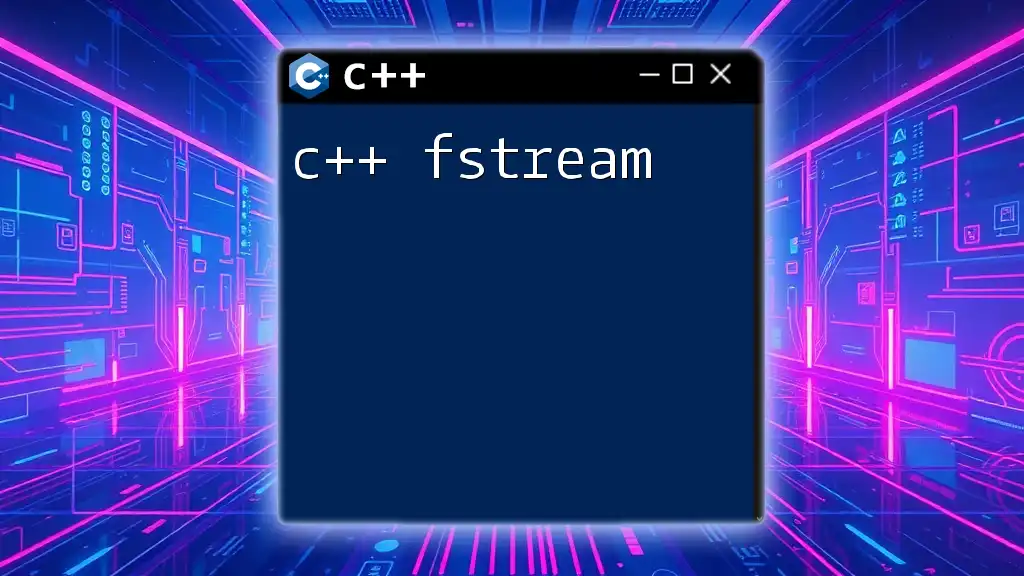
Standard Output Stream
Using std::cout
The most common use of `ostream` in C++ is through `std::cout`, which is the standard output stream representing the console. You can use `std::cout` to display output messages and data values.
Here’s a simple example:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
In this code snippet, `std::cout` is used to output the string "Hello, World!" to the console, followed by `std::endl`, which inserts a newline and flushes the output buffer.
Additional Output Manipulators
std::endl
The `std::endl` manipulator is used with `ostream` to insert a newline character and flush the output buffer. Flushing ensures that all buffered output is written to the console or file. While `std::endl` is useful, it can be more computationally expensive than simply using `\n` for new lines in performance-sensitive applications.
std::flush
Similar to `std::endl`, `std::flush` flushes the output buffer but does not insert a new line. This can be handy when you want to ensure output is displayed immediately without adding an extra newline.
Formatting Output
std::setw and std::setprecision
C++ also allows you to format the output in more controlled ways. For example, you can specify the width of the output and precision for floating-point numbers using the `iomanip` manipulator.
Here’s how you can achieve that:
#include <iostream>
#include <iomanip>
int main() {
double value = 3.14159;
std::cout << std::setprecision(2) << std::fixed << value << std::endl;
return 0;
}
This example uses `std::setprecision(2)` in combination with `std::fixed` to format the double value to two decimal places.
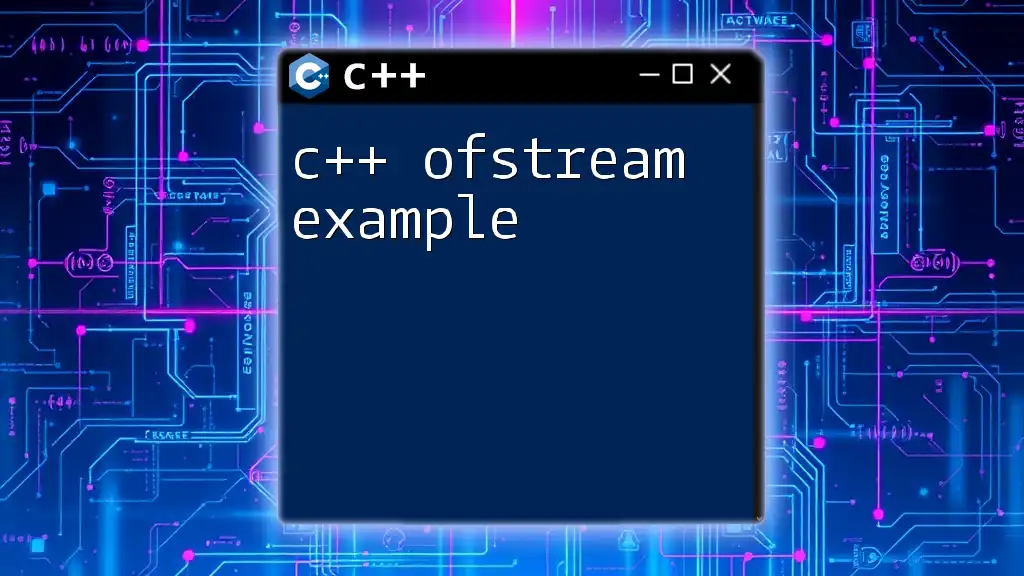
Customizing Your Output Stream
Creating Custom Stream Buffers
Custom stream buffers allow you to customize how data is outputted. You can derive from `std::streambuf` to create your own stream buffer class and then associate it with an `ostream` object, enabling specific formatting or logging functionalities.
Overloading Operators with ostream
You can extend the functionality of `ostream` by overloading the output operator (`<<`) for custom types. This is particularly useful when you want to print user-defined objects directly to the console or output stream.
Here’s an example of how to overload the `<<` operator:
#include <iostream>
class Point {
public:
int x, y;
Point(int x, int y) : x(x), y(y) {}
friend std::ostream& operator<<(std::ostream& os, const Point& point);
};
std::ostream& operator<<(std::ostream& os, const Point& point) {
os << "(" << point.x << ", " << point.y << ")";
return os;
}
int main() {
Point p(10, 20);
std::cout << p << std::endl;
return 0;
}
In this code, the `Point` class has an overloaded `<<` operator, allowing you to output instances of `Point` directly using `std::cout`.
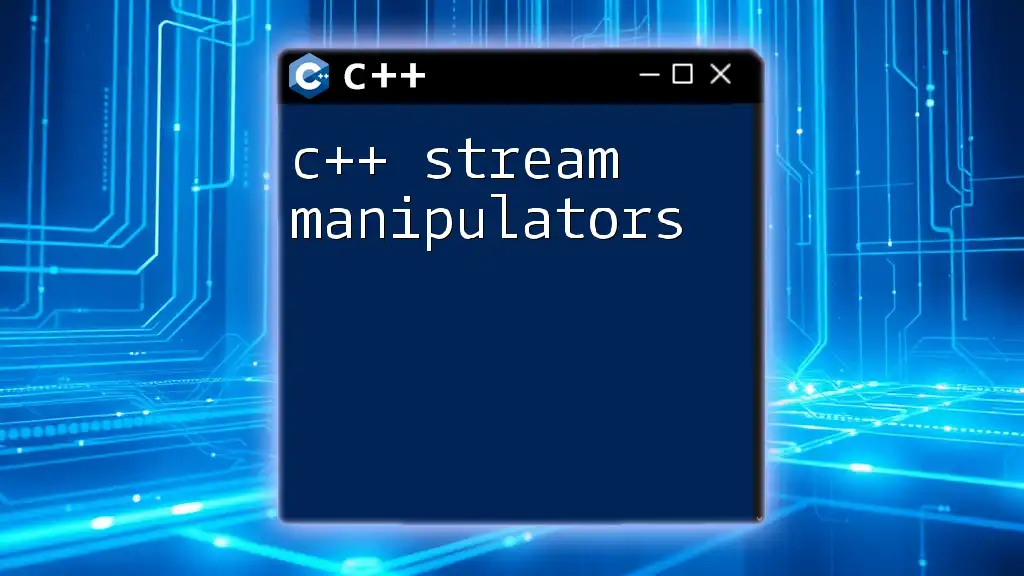
Advanced Features of ostream
Handling Different Data Types
The `ostream` class can output various data types, such as integers, floating-point numbers, and strings directly. It infers the type from the argument passed, simplifying the output process.
Chaining Output with ostream
One of the powerful features of `ostream` is the ability to chain output operations into a single statement. This allows you to combine multiple outputs into a single line of code:
#include <iostream>
int main() {
std::cout << "Year: " << 2023 << ", Value: " << 3.14 << std::endl;
return 0;
}
The above example demonstrates how multiple outputs can be concatenated seamlessly.
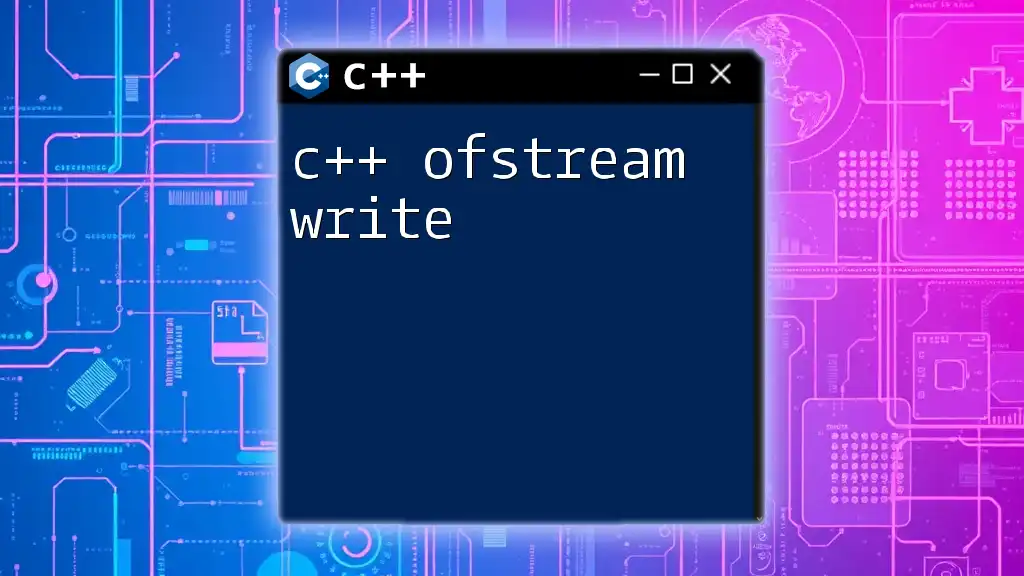
Error Handling in ostream
Checking for Stream Errors
C++ provides methods to check if an output operation was successful. The `fail()` method can determine if a stream operation encountered an error. This is particularly useful for ensuring the robustness of your program by confirming that output operations succeed as intended.
Here’s how you can check for errors:
#include <iostream>
int main() {
std::cout << "Output stream error check." << std::endl;
if (std::cout.fail()) {
std::cerr << "Output failed!" << std::endl;
}
return 0;
}
In this example, if the output to `std::cout` fails, an error message will be displayed via `std::cerr`, which is used for outputting error messages.
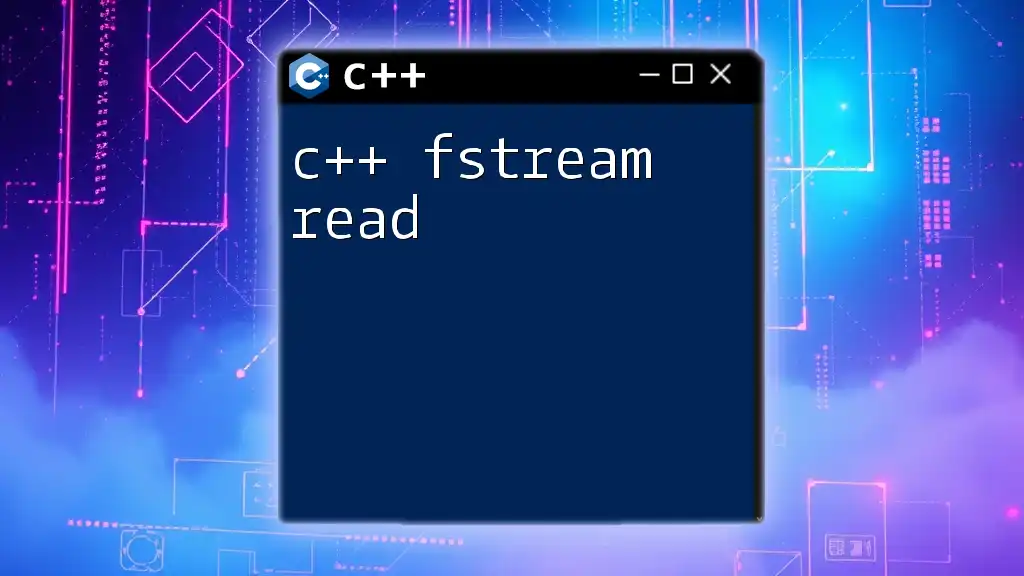
Conclusion
In summary, `c++ ostream` is a fundamental aspect of output operations in C++. It provides a powerful yet simple interface for displaying data and allows for customization through techniques like operator overloading and manipulators. Leveraging `ostream` effectively enhances the clarity and functionality of your C++ applications.
For those eager to delve deeper into the world of C++ I/O, further learning resources, and community support can immensely benefit your journey in mastering not just `ostream` but the entirety of C++.