C++ streaming refers to the process of reading from and writing to input and output streams, typically using the standard library's `iostream` classes to handle data flow efficiently.
Here’s an example of cpp streaming in action:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl; // Output to console
int number;
std::cin >> number; // Input from console
std::cout << "You entered: " << number << std::endl;
return 0;
}
What is CPP Streaming?
CPP streaming refers to the process of reading and writing data in C++ using a system of input and output streams. This concept is fundamental in C++ programming, allowing developers to interact with data efficiently, whether from user input, files, or other sources.
Historically, C++ began with simpler forms of input and output inherited from C. The introduction of streams in C++ allowed more sophisticated and flexible ways to handle data. This evolution has made C++ an adaptable language for various programming tasks, from console applications to complex data processing.

Understanding the Basics of C++ Streams
What Are Streams?
In C++, a stream is essentially a sequence of characters that can be read or written. The C++ Standard Library provides a robust framework for handling streams, making it easier for programmers to manage data flow without getting bogged down by the underlying complexities.
Fundamental Classes of C++ Streams
C++ provides several key stream classes, primarily:
- `istream`: This class is used for input operations.
- `ostream`: This class is dedicated to output operations.
- `fstream`: This is a combination class that supports both input and output file operations.
The C++ Standard Input and Output Streams
C++ offers several standard streams for user interaction:
- `cin`: The standard input stream, used to read data from the keyboard.
- `cout`: The standard output stream, used to send data to the console.
- `cerr`: The standard error stream, used to output error messages.
- `clog`: Also used for outputting logging information, but it is buffered.
Here’s a simple example that demonstrates the use of `cout`:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
This code outputs "Hello, World!" to the console by utilizing the `cout` stream.
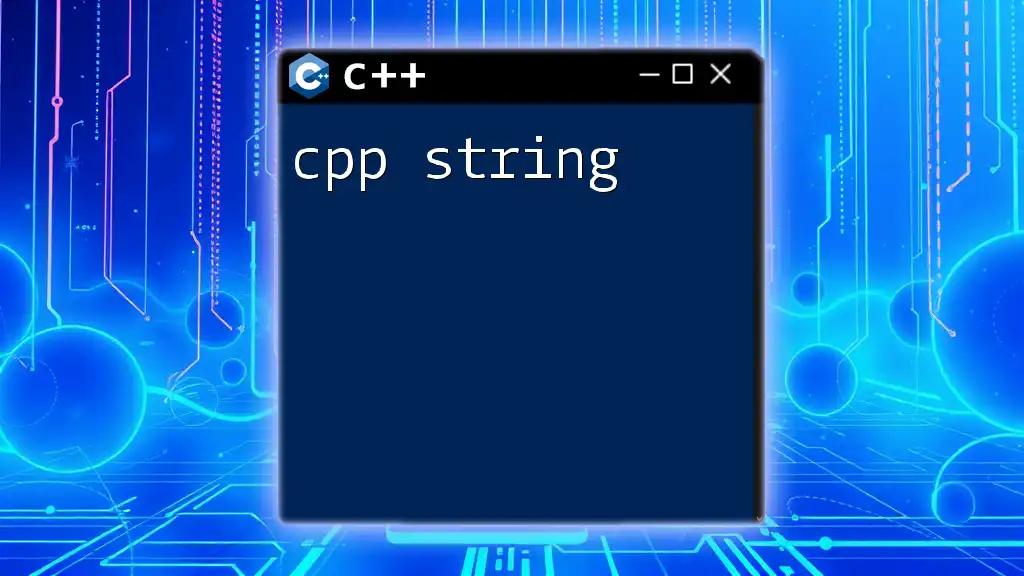
Working with Input and Output Streams
Reading from Input Streams
In C++, data can be read from input streams using the `>>` operator or functions like `getline()`. The `>>` operator is often used for reading formatted input:
int age;
std::cout << "Enter your age: ";
std::cin >> age;
For reading whole lines of text, especially those containing spaces, `getline()` is more appropriate:
std::string name;
std::cout << "Enter your name: ";
std::getline(std::cin, name);
Writing to Output Streams
You can send strings, numbers, and other data types to output streams using the `<<` operator. Here’s an example that illustrates both text and number output:
int number = 42;
std::cout << "The answer is: " << number << std::endl;
C++ also allows formatting output with manipulators. For instance, `std::setw` and `std::setprecision()` can help format numbers to a desired width or number of decimal places, respectively. For example:
#include <iostream>
#include <iomanip>
int main() {
double pi = 3.14159;
std::cout << "Pi to 2 decimal places: " << std::fixed << std::setprecision(2) << pi << std::endl;
return 0;
}
Error Handling in Streams
Error handling is critical when dealing with streams, especially for user input and file operations. Streams can enter various error states, which can be managed using the methods:
- `fail()`: Checks if the last I/O operation failed.
- `eof()`: Identifies if the End of File (EOF) has been reached.
- `bad()`: Indicates if a non-recoverable stream error has occurred.
Here’s an example showing how to handle errors in user input:
int number;
std::cout << "Enter a number: ";
std::cin >> number;
if (std::cin.fail()) {
std::cerr << "Invalid input!" << std::endl;
std::cin.clear(); // Clear the error state
std::cin.ignore(std::numeric_limits<std::streamsize>::max(), '\n'); // Discard invalid input
}
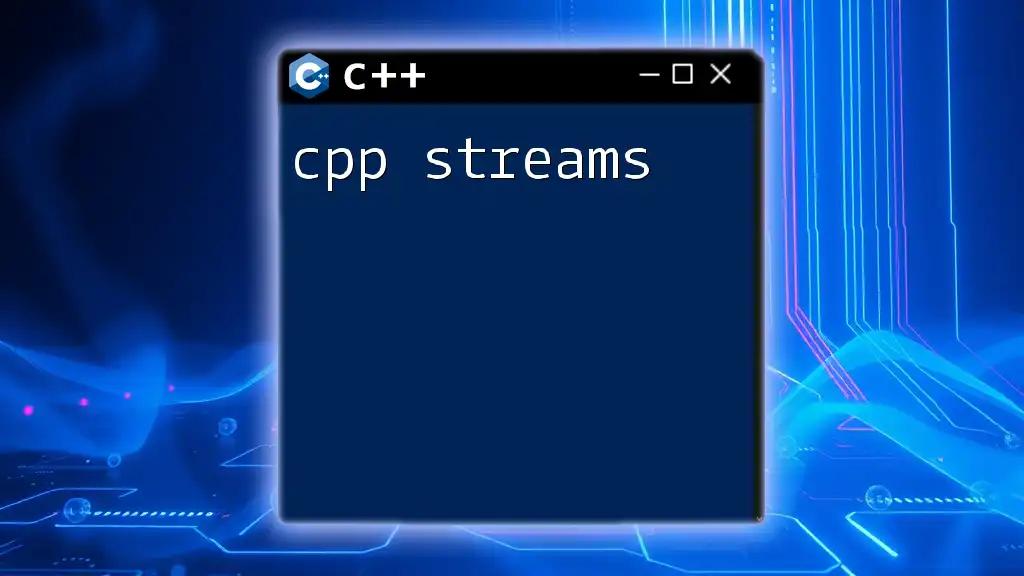
File Streams in C++
Introduction to File Streams
File streams are crucial for any application that requires persistent data storage. Unlike standard streams, file streams allow you to read from and write to files on disk.
Using fstream for File Operations
The `fstream` class can be used to manipulate files seamlessly. It allows you to open files in various modes - input, output, or both.
To open a file and read from it, you can use:
std::ifstream inputFile("data.txt");
std::string line;
while (std::getline(inputFile, line)) {
std::cout << line << std::endl;
}
inputFile.close();
This snippet demonstrates reading a text file line by line and printing each line to the console. Always ensure to close the file to free resources.
Writing to files can be achieved similarly using `ofstream`:
std::ofstream outputFile("output.txt");
outputFile << "Writing to a file!" << std::endl;
outputFile.close();
This example opens `output.txt` and writes a simple message to it. If the file does not exist, it will be created.
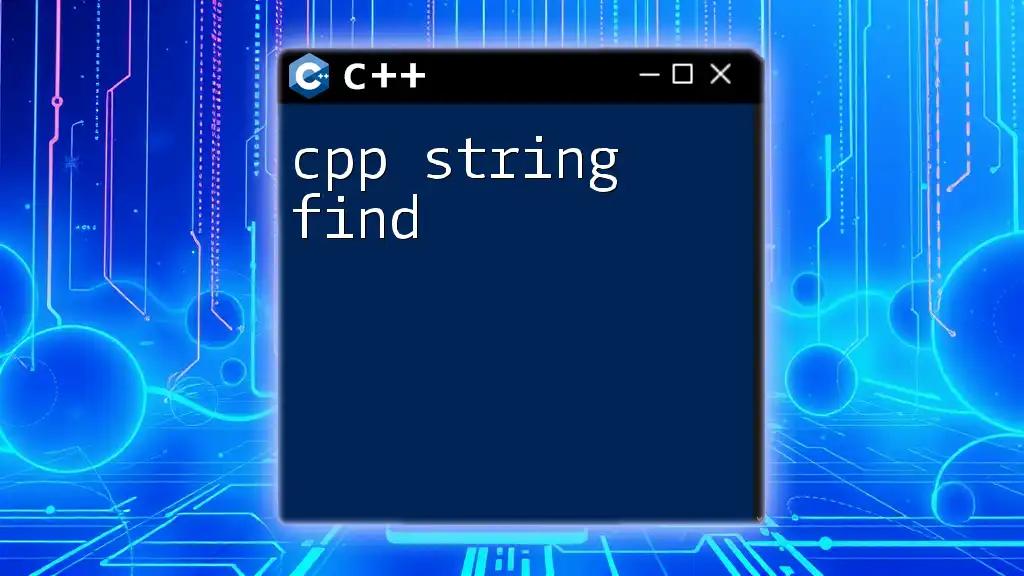
Advanced Streaming Topics
String Streams in C++
In addition to file streams, C++ provides string streams, which allow you to manipulate strings as if they were streams of data. The classes to note are `stringstream`, `istringstream`, and `ostringstream`.
String streams are particularly useful for formatting strings or converting data types. Here’s a brief example using `stringstream`:
std::stringstream ss;
ss << "Value: " << 42;
std::string output = ss.str();
std::cout << output << std::endl;
This code snippet creates a formatted string from a numeric value and outputs it.
Custom Stream Buffers
For more advanced usage, C++ allows the creation of custom stream buffers by inheriting from the `std::streambuf` class. This capability means you can define exactly how data is read from or written to a specific source or destination, introducing a new level of flexibility.
Stream Manipulation Functions
C++ also provides a variety of stream manipulators that ease formatting tasks. For instance, `std::setw` can set the width of the next output field, and `std::setfill` can specify a character used for padding within that field. Practical examples could involve aligning output for tabular data presentations.
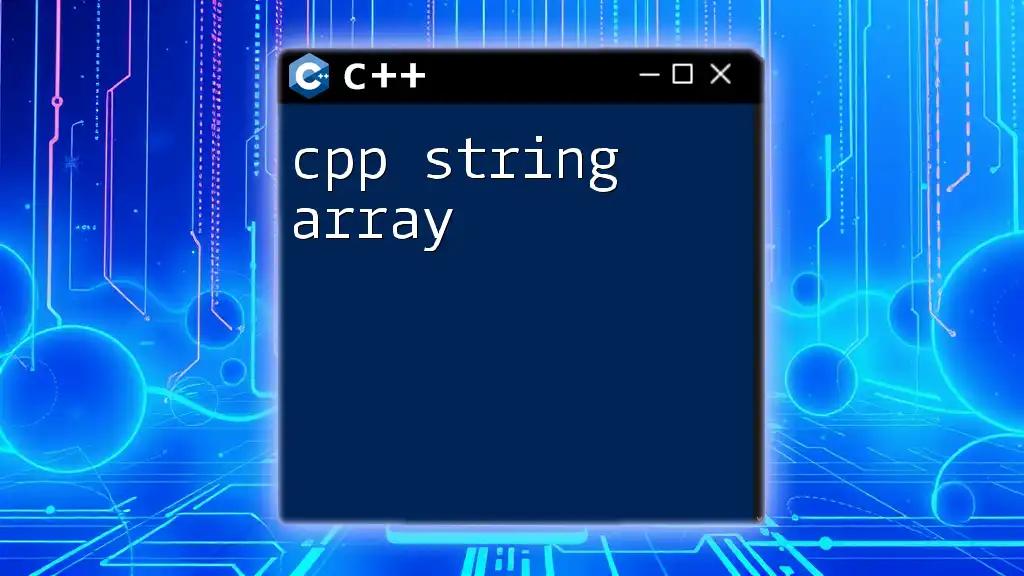
Best Practices for C++ Streaming
Performance Considerations
When dealing with larger files or high-frequency data, you should consider the performance implications of buffered vs. unbuffered streams. Buffering can significantly boost I/O performance, as data operations are grouped, reducing the number of read/write calls to disk.
Avoiding Common Pitfalls
A few careful practices can save you from common mistakes:
- Always check the state of your streams before and after operations. Use error-checking methods liberally.
- Close your files after operations to ensure that resources are released properly and files are not locked unnecessarily.
- Understand the difference between manipulating data directly versus manipulating the representation of that data through streams. Knowing when to use which is key to mastering CPP streaming.
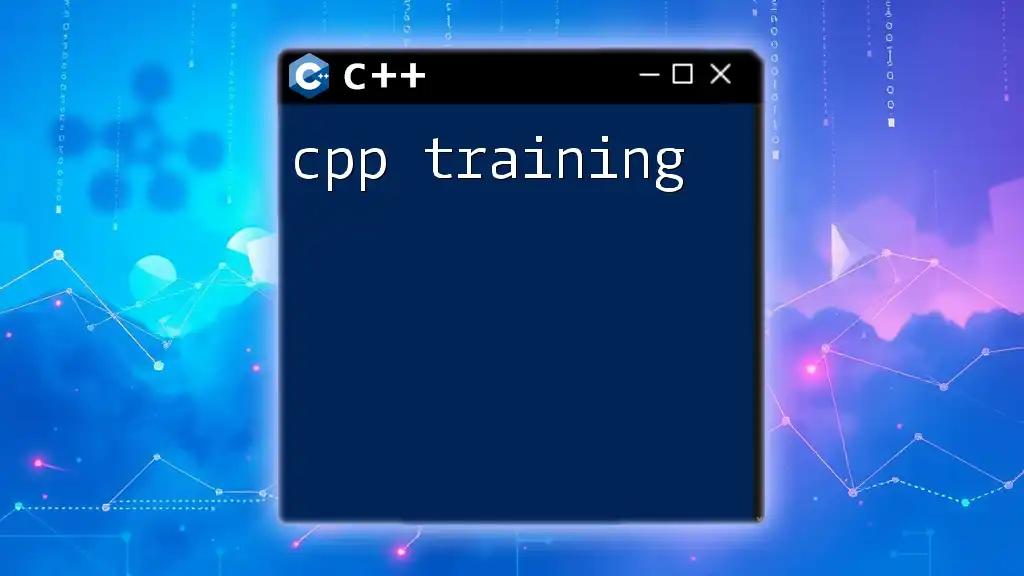
Conclusion
In summary, mastering CPP streaming opens doors to effective data handling in C++. The importance of understanding streams, handling input/output operations, and learning built-in classes cannot be overstated. By adhering to best practices, you set yourself up for success in crafting robust and efficient C++ applications.
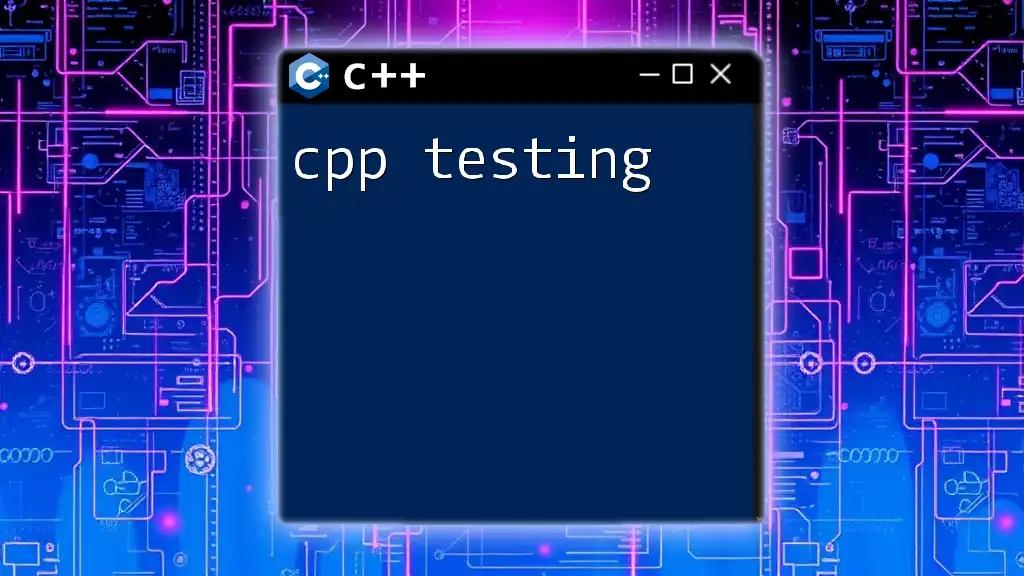
FAQs About CPP Streaming
What is the difference between `cin` and `getline()`?
`cin` reads input until a whitespace character is found, while `getline()` reads an entire line, including spaces, until a newline character is encountered.
How do I handle errors when reading from files?
You can check whether a stream is in a fail state using the `fail()` method and respond accordingly.
Can I use C++ streams for network programming?
Yes, while C++ streams are typically used for file and console I/O, they can also be adapted for network communication by creating custom stream classes that interface with socket operations.