Whisper.cpp streaming allows for real-time audio transcription using the Whisper model's streaming capabilities, enabling low-latency processing of incoming audio data.
#include <whisper.h>
// Stream audio and transcribe using Whisper
WhisperModel model("path_to_model/whisper.bin");
AudioStream stream("input_audio.wav");
while (stream.isOpen()) {
auto chunk = stream.readNextChunk();
auto transcription = model.transcribe(chunk);
std::cout << transcription << std::endl; // Output transcription
}
Understanding Whisper.cpp
Whisper.cpp is a powerful C++ library designed specifically for handling streaming tasks efficiently. The scope of whisper.cpp is primarily focused on audio processing and streaming, making it a valuable tool for developers engaged in creating high-performance applications in this domain.
The significance of C++ in streaming applications cannot be overstated. C++ offers superior performance and fine-grained control over system resources, enabling developers to create applications capable of handling large volumes of data with minimal latency. This makes C++ an ideal choice for applications that require real-time audio streaming.
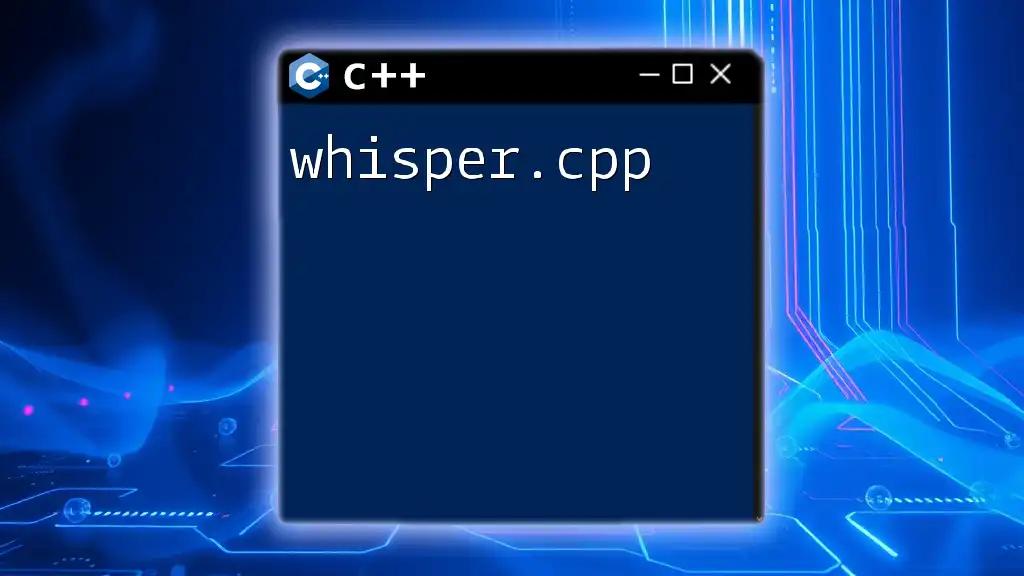
Setting Up Your Environment
Before diving into whisper.cpp streaming, ensuring that your development environment is correctly set up is crucial for success.
Prerequisites
To develop applications utilizing whisper.cpp, you will need:
- A modern C++ compiler (e.g., GCC, Clang, or MSVC)
- The CMake build system
- Essential audio libraries (e.g., PortAudio or OpenAL)
- Basic knowledge of C++ programming
Installing Whisper.cpp
To install whisper.cpp, follow these steps:
-
Clone the Repository: Navigate to your terminal and use git to clone the whisper.cpp repository.
git clone https://github.com/your-repo/whisper.cpp.git
-
Navigate to the Directory:
cd whisper.cpp
-
Build the Library:
cmake . make
These steps will prepare whisper.cpp for use in your projects.
// Example Code Snippet for Initialization
#include "whisper.h"
// Setup initialization
Whisper whisper;
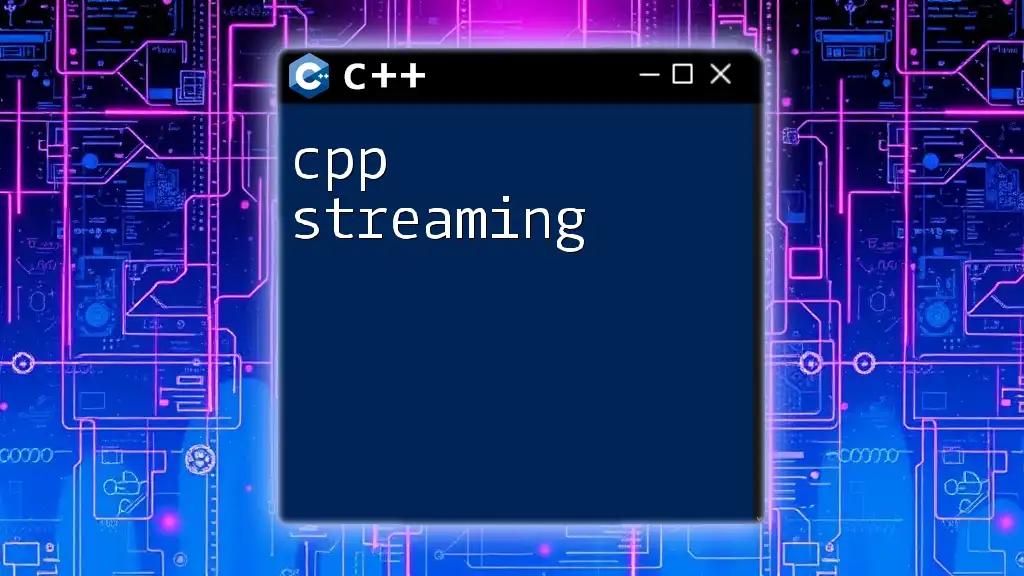
Streaming Basics
Streaming refers to the process of transmitting audio or video data in real-time. In the context of whisper.cpp streaming, this involves efficiently handling continuous audio data for applications such as music playback, broadcasting, or live performances.
C++ plays a pivotal role in streaming due to its performance optimizations. Features such as direct memory access and close-to-hardware functionality empower developers to minimize delays in audio signal processing, resulting in smooth and uninterrupted audio streams.

Stream Management in Whisper.cpp
Creating a Stream
At the heart of whisper.cpp streaming is the ability to create a stream. Here's how you can create a streaming instance using whisper.cpp:
// Example Code Snippet for Creating Stream
Stream stream = whisper.createStream("input.wav");
This line initializes a stream that reads from the specified audio file.
Managing Input and Output Streams
Effective stream management entails controlling both input and output. Whisper.cpp allows you to handle incoming and outgoing data seamlessly.
For instance, to ensure that your streams are synchronized and running smoothly, you should regularly monitor data flow. This includes checking the health of the stream, ensuring it is not disrupted, and handling errors gracefully. To read audio data dynamically, consider the following example:
while (stream.isAlive()) {
auto chunk = stream.readNextChunk();
processAudio(chunk);
}
This code snippet highlights how to read and process audio data in chunks, promoting efficient streaming functionality.
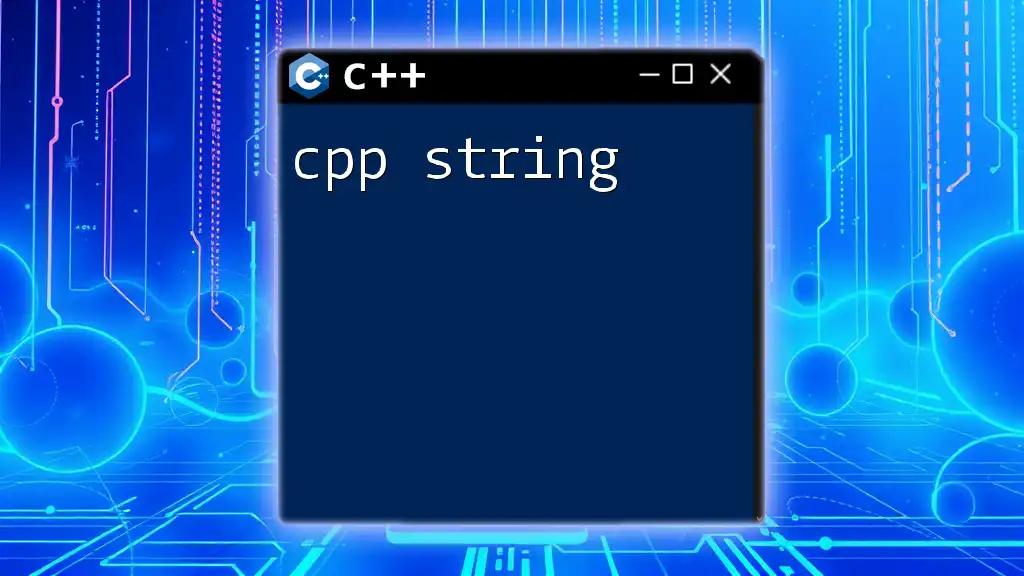
Implementing Whisper Streaming
Basic Streaming Implementation
To implement a basic application using whisper.cpp, follow this sequence:
- Initialize the Whisper Instance.
- Create an Input Stream from an audio source.
- Process the Stream using a loop that reads chunks of data.
- Output to an Audio Device for playback.
The general flow maintains a consistent audio experience, allowing developers to focus on enhancing, rather than troubleshooting, their applications.
Working with Audio Data
When streaming audio, it's vital to understand the structure of audio data. Common formats like PCM, WAV, or MP3 can be handled using whisper.cpp, enabling developers to manage various types of audio sources effectively.
The management of different audio data types involves understanding how to decode and transform signals while ensuring minimized latency and preserving audio quality. With whisper.cpp, developers can work with raw audio bytes or higher-level abstractions, depending on the established requirements.
// Example Code Snippet: Processing Audio Data
void processAudio(const AudioChunk& chunk) {
// Handle audio processing logic
}
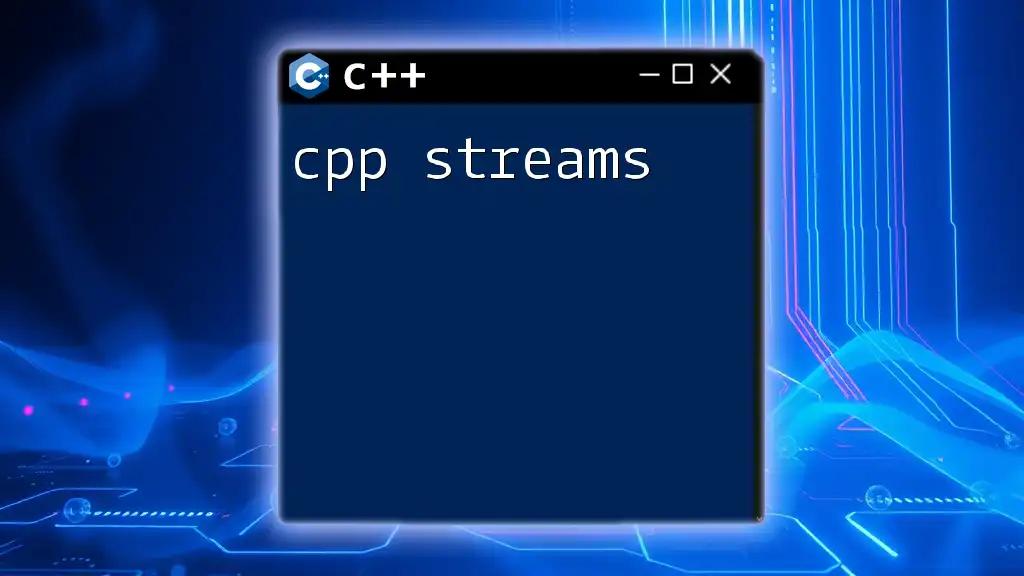
Advanced Streaming Techniques
Handling Latency Issues
Latency remains a primary concern in streaming applications. In whisper.cpp, you can identify and mitigate latency problems by employing techniques such as buffering and asynchronous reading/writing.
By implementing buffers before processing audio chunks, developers can ensure that incoming data is queued, preventing skips or interruptions. Furthermore, asynchronous operations can help you execute background tasks, allowing the main thread to focus on processing audio.
Optimizing Performance
To achieve higher efficiency with whisper.cpp streaming:
- Profile your application: Use performance profiling tools to identify bottlenecks.
- Use low-latency audio APIs: Libraries such as PortAudio can significantly improve performance.
- Tune buffer sizes: Adjusting buffer sizes helps manage how much data is processed at a time, striking a balance between latency and throughput.
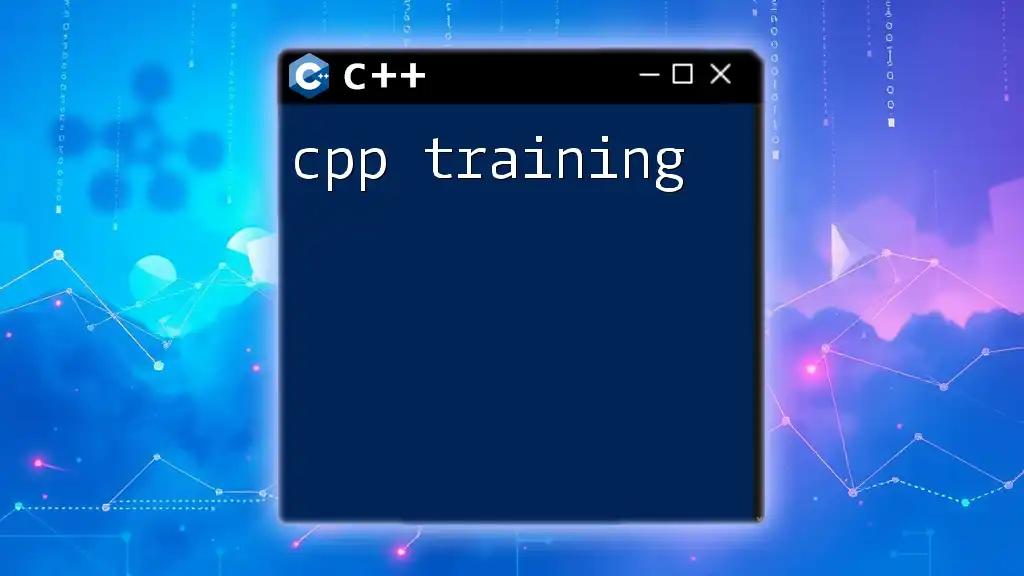
Error Handling and Debugging
Common Errors in Whisper.cpp
Errors in whisper.cpp streaming can manifest in various ways, ranging from buffer overflows to file read errors. Here are some typical issues you may encounter:
- Stream Initialization Errors: Often due to file path issues or unsupported formats.
- Data Underflows: Occur when the audio buffer is being read too quickly.
Debugging Techniques
Developers can adopt several strategies to debug whisper.cpp applications, including:
- Implementing comprehensive logging to trace the operation flow.
- Using assertions to validate the correctness of data.
- Taking advantage of multi-threaded debugging tools for examining concurrency issues.
// Example Code Snippet for Debugging
if (stream.hasError()) {
logError(stream.getError());
}
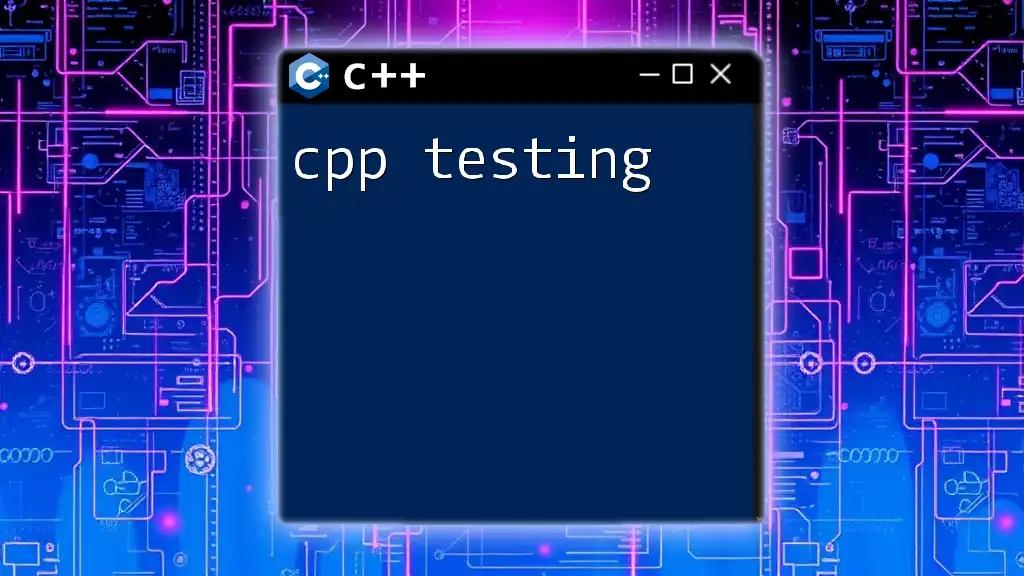
Use Cases and Applications
Real-Time Streaming Applications
Whisper.cpp is particularly well-suited for a range of real-time streaming applications. Examples include:
- Live Streaming for podcasts or radio stations.
- Interactive Gaming where audio plays a crucial role.
- Virtual Concerts, providing users access to live performances directly.
Case Study: Implementing Whisper in a Project
Consider a project where you develop a live music streaming application. By integrating whisper.cpp into your audio processing pipeline, you can ensure smooth audio delivery, allowing musicians to interact with their audience in real-time while maintaining high audio fidelity.
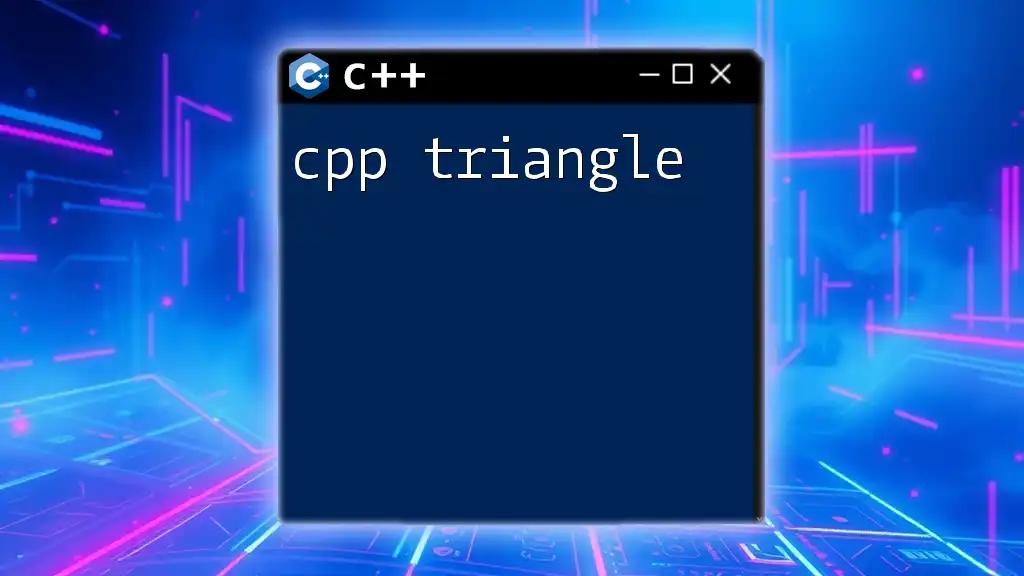
Conclusion
In this guide, we have explored the intricacies of whisper.cpp streaming. We have discussed its features, setup procedures, management strategies, and implemented techniques. As you venture into creating your own streaming applications, remember that whisper.cpp provides a rich toolbox for high-performance audio processing.
With the continual advancements in streaming technology, C++ remains a robust choice for developers looking to build responsive, efficient, and scalable applications.
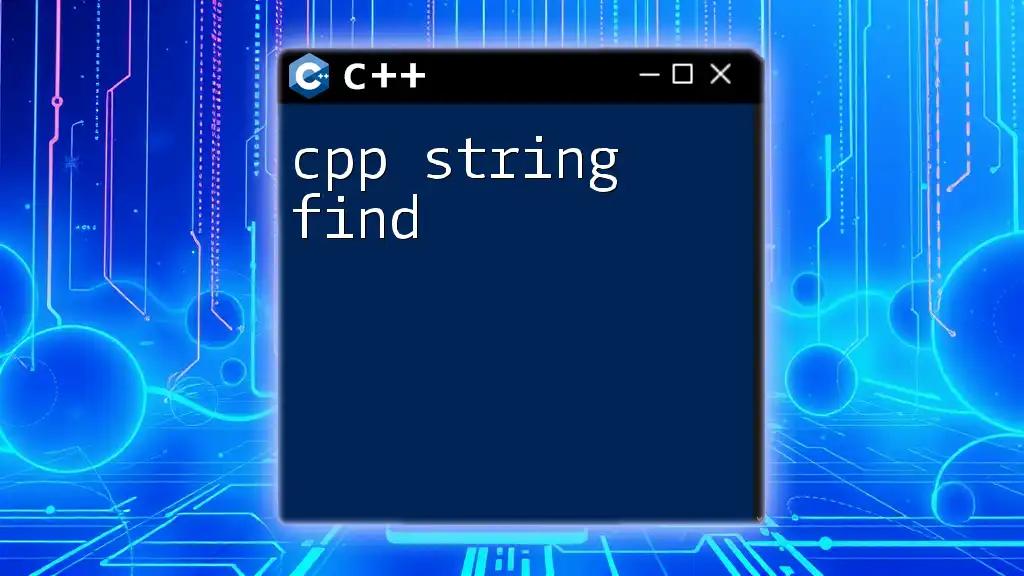
Additional Resources
For those eager to delve deeper into C++ and whisper.cpp, considering exploring relevant documentation, community forums, and contributing to open-source projects. Engaging with a community can foster learning and help address common challenges encountered in audio streaming development.
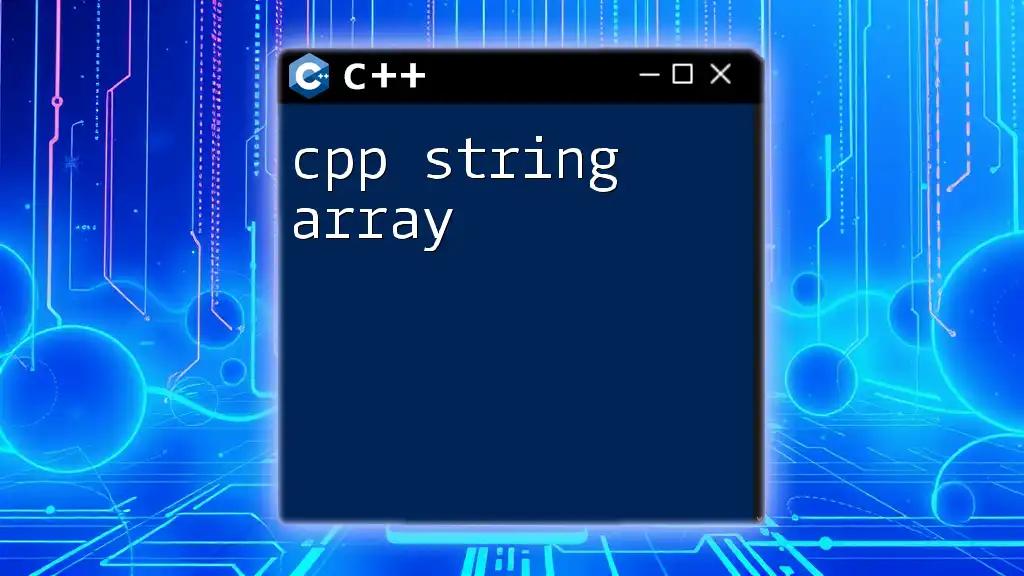
Call to Action
Join our community focused on C++ programming and whisper.cpp to access more tutorials, sessions, and discussions. Together, let’s empower each other to harness the potential of C++ in streaming!