To sort a string in C++, you can utilize the `std::sort` function from the Standard Library, which rearranges the characters in the string in ascending order.
Here’s a concise example:
#include <iostream>
#include <algorithm>
int main() {
std::string str = "hello";
std::sort(str.begin(), str.end());
std::cout << str; // Output: ehllo
return 0;
}
Understanding Strings in C++
What is a String in C++?
In C++, a string is a sequence of characters that can represent text. The C++ Standard Library provides the `std::string` class, which makes it easy to create and manipulate strings. Unlike C-style strings, which are just arrays of characters ending with a null character, the `std::string` class handles various operations and memory management internally, reducing the complexity for developers.
Why Sort Strings?
Sorting strings is a fundamental operation in many applications. Whether for organizing user input, processing data before displaying it, or enhancing search functionality, sorting strings can significantly improve performance and usability. Understanding how to sort strings in C++ can help you write cleaner and more efficient code, and is an essential skill for any C++ programmer.
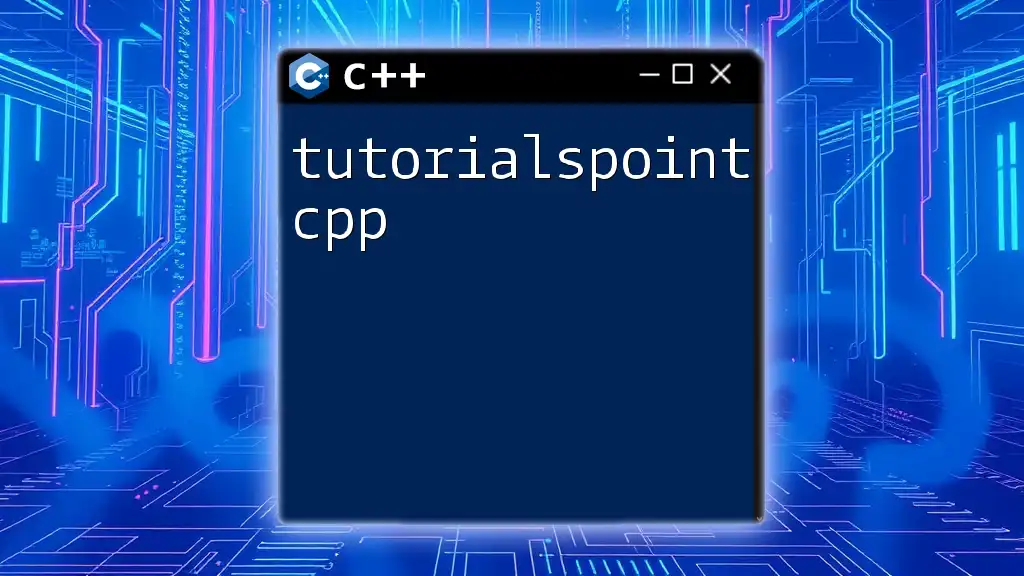
Methods to Sort a String in C++
Using the Standard Template Library (STL)
Sorting a String with `std::sort()`
The Standard Template Library (STL) in C++ offers powerful tools for sorting. The `std::sort()` function is a versatile function that can sort arrays, vectors, and strings. It sorts elements in ascending order by default.
Here’s how to sort a string using `std::sort()`:
#include <iostream>
#include <algorithm>
#include <string>
int main() {
std::string str = "cba";
std::sort(str.begin(), str.end());
std::cout << "Sorted string: " << str << std::endl;
return 0;
}
In this example, the string "cba" is sorted to "abc". The `std::sort()` function modifies the string in-place, meaning that the original string is changed directly. This function requires the `<algorithm>` header, so be sure to include it in your program.
Sorting a String in Reverse Order
Sorting strings in reverse order can be done using a custom comparator with `std::sort()`. By providing the function with a comparator that specifies the desired order, you can easily sort in descending order.
#include <iostream>
#include <algorithm>
#include <string>
int main() {
std::string str = "cba";
std::sort(str.begin(), str.end(), std::greater<char>());
std::cout << "Sorted string (descending): " << str << std::endl;
return 0;
}
Here, `std::greater<char>()` is utilized to sort the string in descending order, resulting in "cba". Using custom comparators allows for flexible sorting logic, empowering developers to tailor their sorting algorithms to specific needs.
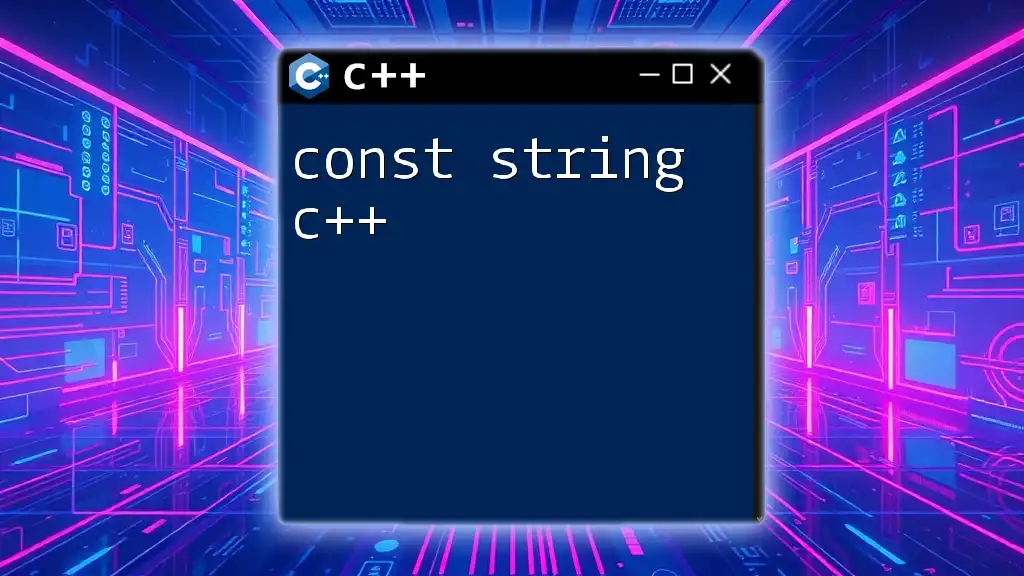
Manual String Sorting without STL
Implementing Bubble Sort
Bubble sort is a straightforward algorithm that repeatedly steps through the string to be sorted, compares adjacent elements, and swaps them if they are in the wrong order. This process is repeated until the string is sorted.
Here is how you can implement bubble sort for a string:
#include <iostream>
#include <string>
void bubbleSort(std::string &str) {
for (int i = 0; i < str.length() - 1; ++i)
for (int j = 0; j < str.length() - i - 1; ++j)
if (str[j] > str[j + 1])
std::swap(str[j], str[j + 1]);
}
int main() {
std::string str = "cba";
bubbleSort(str);
std::cout << "Sorted string using Bubble Sort: " << str << std::endl;
return 0;
}
In this code, the `bubbleSort` function iteratively compares adjacent pairs of characters, swapping them until the entire string is sorted. The efficiency of bubble sort can be a downside for larger datasets, as its time complexity is \(O(n^2)\) in the worst case.
Implementing Selection Sort
Selection sort is another simple sorting algorithm that divides the list into two parts: the sorted part and the unsorted part. It repeatedly selects the smallest (or largest, depending on sorting order) element from the unsorted part and moves it to the sorted part.
Here’s how you can implement selection sort for a string:
#include <iostream>
#include <string>
void selectionSort(std::string &str) {
for (int i = 0; i < str.length() - 1; ++i) {
int minIndex = i;
for (int j = i + 1; j < str.length(); ++j)
if (str[j] < str[minIndex])
minIndex = j;
std::swap(str[i], str[minIndex]);
}
}
int main() {
std::string str = "cba";
selectionSort(str);
std::cout << "Sorted string using Selection Sort: " << str << std::endl;
return 0;
}
In this example, the `selectionSort` function finds the smallest character in the unsorted part and swaps it with the first unsorted character, effectively sorting the string in ascending order. Like bubble sort, selection sort has a worst-case time complexity of \(O(n^2)\).
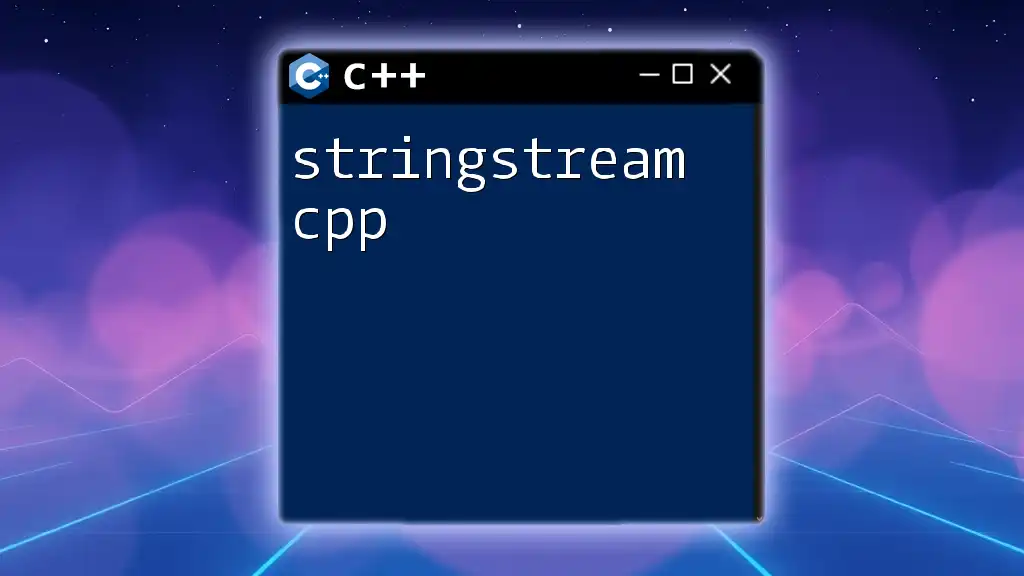
Practical Applications of Sorted Strings
Searching Sorted Strings
Once strings are sorted, they can be used with search algorithms, such as binary search. Searching through a sorted string is significantly more efficient than searching through an unsorted string. By implementing sorting prior to searching, you can greatly reduce the overall time required for data retrieval.
Storing Sorted Strings
Storing strings in a sorted order can enhance data organization. For applications like databases or file systems that require quick access to data, keeping strings sorted can improve retrieval times and efficiency.
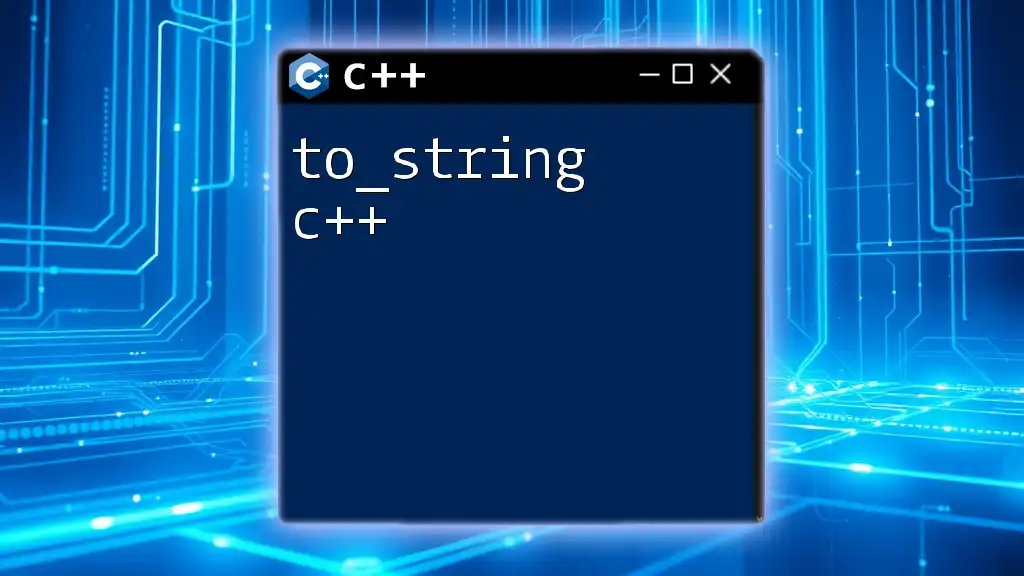
Conclusion
Understanding how to sort a string in C++ is an essential skill that not only enhances your programming capabilities but also allows for more efficient data handling in your applications. Whether utilizing the powerful tools provided by the Standard Template Library (STL) or implementing manual sorting algorithms like bubble sort or selection sort, mastering these concepts opens many possibilities for organizing and processing text in your C++ programs.
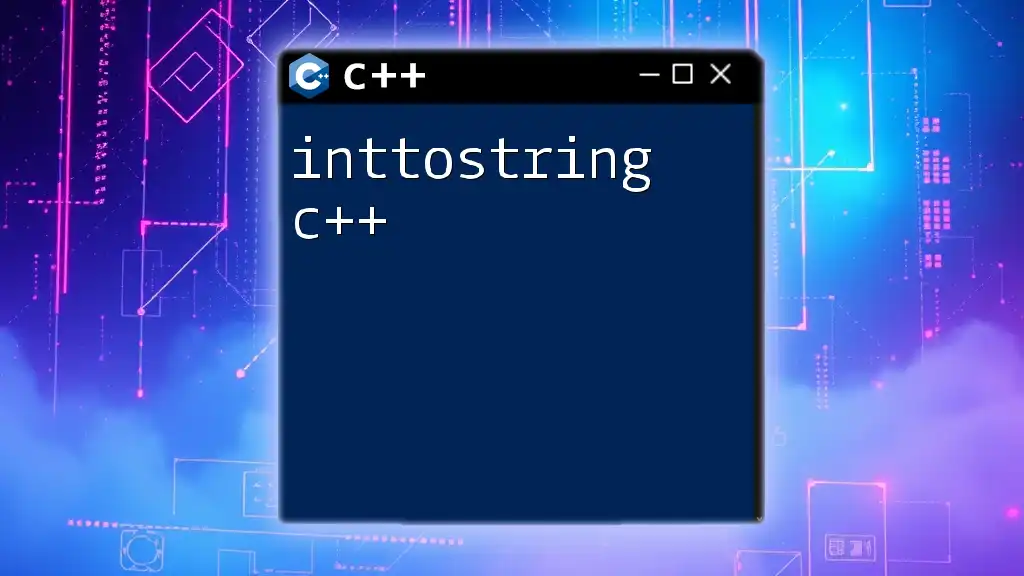
FAQs
What is the time complexity of sorting a string in C++?
The time complexity of sorting a string using `std::sort()` is \(O(n \log n)\) on average. In contrast, manual sorting methods like bubble sort and selection sort have a time complexity of \(O(n^2)\), making them less efficient for larger strings.
Can I sort strings without using STL?
Yes, you can sort strings without using STL by implementing manual sorting algorithms, such as bubble sort or selection sort, as shown in the examples above.
Is it more efficient to sort strings or store them in sorted order?
Storing strings in a sorted order can improve retrieval efficiency, especially for search operations. However, maintaining a sorted order every time you insert or delete strings can add overhead. Evaluating whether to sort or maintain sorted states depends on the specific use case and the frequency of insertions versus searches.