"Foundation C++" refers to the essential principles and commands of C++ programming that serve as the building blocks for writing efficient and effective code.
Here's a simple code snippet demonstrating the basics of using a class in C++:
#include <iostream>
class Example {
public:
void display() {
std::cout << "Hello, Foundation C++!" << std::endl;
}
};
int main() {
Example ex;
ex.display();
return 0;
}
Getting Started with C++
Setting Up Your Environment
To begin your journey in foundation C++, setting up an effective development environment is crucial. Consider using an Integrated Development Environment (IDE) or text editor that supports C++. Some popular choices are:
- Visual Studio: A robust IDE that provides extensive tools for C++.
- Code::Blocks: A free, open-source IDE that's flexible and easy to use.
- CLion: A commercial IDE that offers intelligent code assistance.
Installing a C++ compiler is equally important. For Windows, you might use MinGW, while macOS users can utilize Xcode command line tools. On Linux, GCC is often pre-installed or easily installable via package managers.
Writing Your First C++ Program
Once your environment is set, you can write your first C++ program. Every C++ program starts with the main() function, which serves as the entry point. The structure typically includes headers and possibly namespaces.
Here’s how the classic "Hello, World!" program looks:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
In this snippet:
- `#include <iostream>` imports the Input/Output stream library essential for using `std::cout`.
- The `main()` function encapsulates the program logic.
- `std::cout` prints the string to the console, and `std::endl` signifies the end of the line.
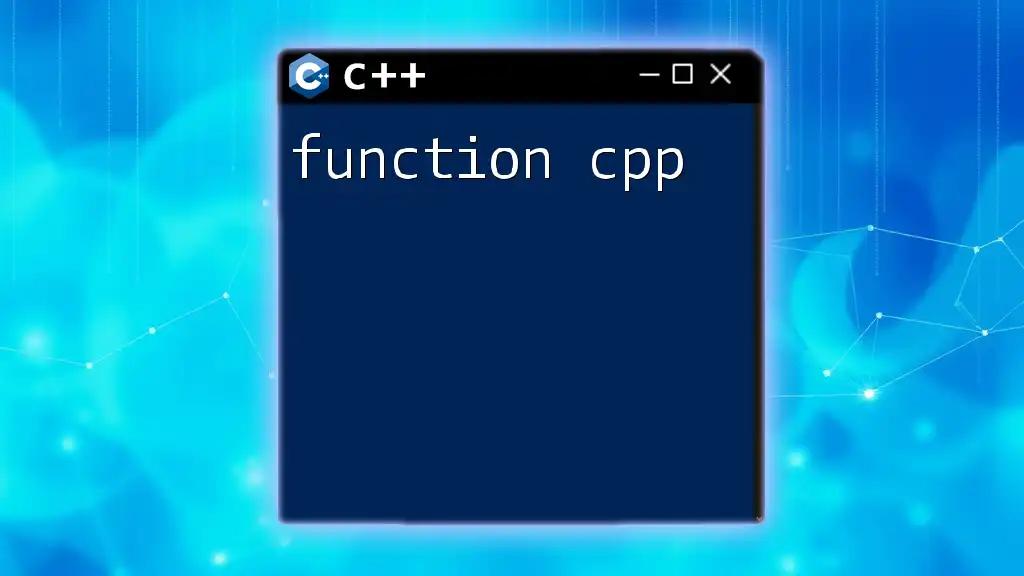
Basic Syntax in C++
Variables and Data Types
In C++, variables store data that can be manipulated. Familiarize yourself with primitive data types such as:
- int: used for integers
- float: used for decimal numbers
- char: used for single characters
- bool: used for boolean values (true/false)
Here’s a simple example:
int age = 25;
float height = 5.9;
char grade = 'A';
In this example, we define three variables of different types and assign them values.
User Input and Output
For interacting with users, C++ provides `cin` for input and `cout` for output. You can prompt users for input as shown below:
int age;
std::cout << "Enter your age: ";
std::cin >> age;
std::cout << "You are " << age << " years old." << std::endl;
Operators in C++
C++ includes a variety of operators that allow you to perform operations on variables. Key types are:
- Arithmetic Operators: `+`, `-`, `*`, `/`
- Comparison Operators: `==`, `!=`, `>`, `<`
- Logical Operators: `&&`, `||`, `!`
Here’s a practical example using comparison:
int a = 5, b = 10;
if(a < b) {
std::cout << "a is less than b" << std::endl;
}
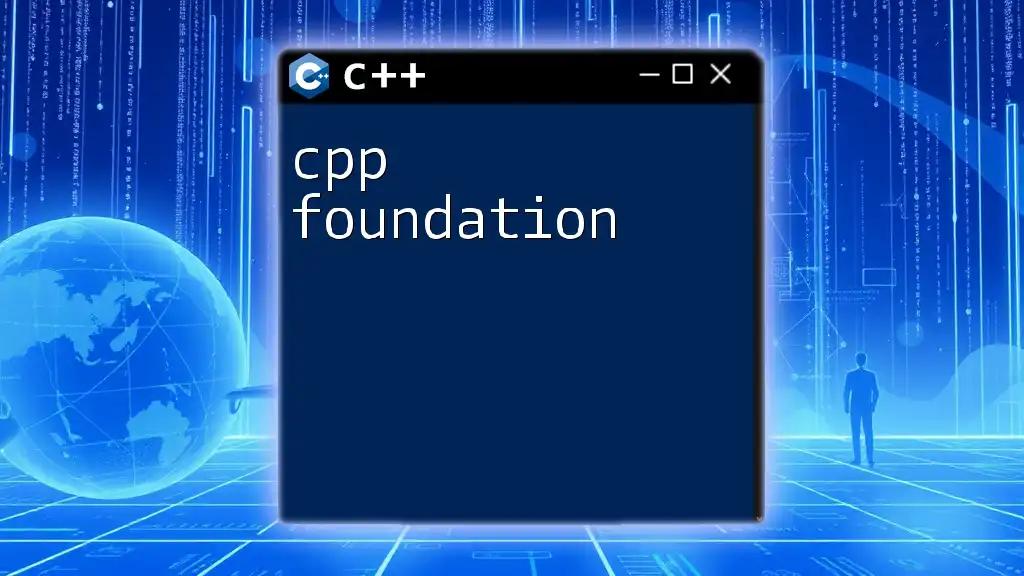
Control Structures
Conditional Statements
Control the flow of your program with conditional statements such as `if`, `else if`, and `else`. You can also implement a switch statement for multiple conditions.
For example:
int option = 2;
switch(option) {
case 1:
std::cout << "Option 1 selected.";
break;
case 2:
std::cout << "Option 2 selected.";
break;
default:
std::cout << "Invalid option.";
}
Loops in C++
Loops allow you to execute a block of code multiple times efficiently. The primary types include for, while, and do while loops.
Here’s a simple iteration using a `for` loop:
for(int i = 0; i < 5; i++) {
std::cout << "Iteration: " << i << std::endl;
}
This loop will print "Iteration: 0" through "Iteration: 4".
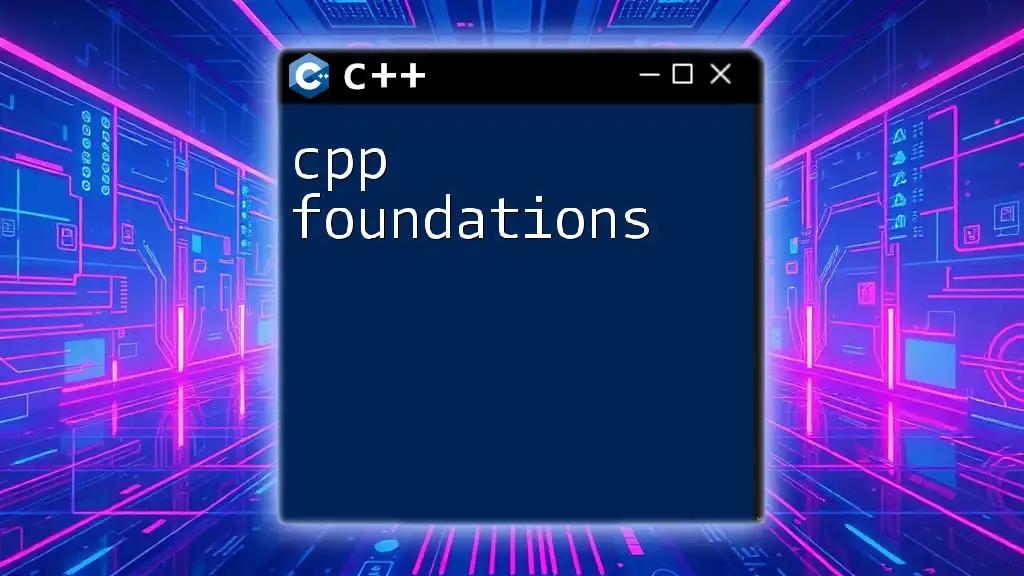
Functions in C++
Defining and Calling Functions
Functions encapsulate code for reusability. Here's the basic syntax for defining a function:
int add(int a, int b) {
return a + b;
}
To call the function:
int result = add(5, 3);
std::cout << "The sum is: " << result << std::endl;
Function Overloading
C++ allows you to define multiple functions with the same name but different parameters. This is known as function overloading.
For example:
int add(int a, int b) {
return a + b;
}
double add(double a, double b) {
return a + b;
}
The appropriate version of `add` will be called based on the types of arguments passed.
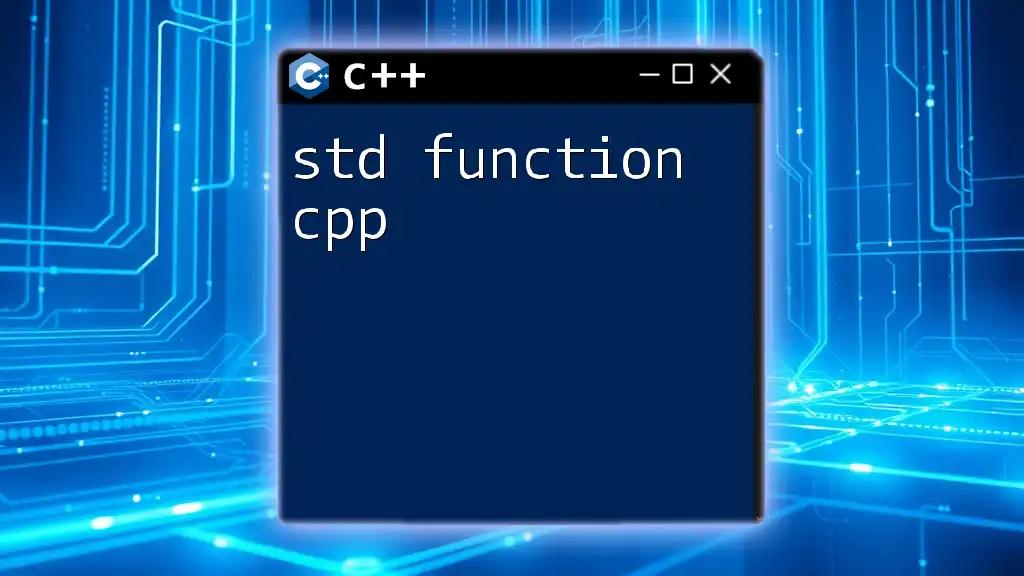
Object-Oriented Programming in C++
Introduction to OOP Concepts
Foundation C++ revolves around the principles of Object-Oriented Programming (OOP). Key concepts include:
- Classes and Objects: A class is a blueprint; an object is an instance of a class.
- Encapsulation: Restricting direct access to some of an object’s components.
- Inheritance: Creating new classes from existing ones.
- Polymorphism: Allowing objects to be treated as instances of their parent class.
Creating a Class
Creating a class in C++ is straightforward:
class Dog {
public:
void bark() {
std::cout << "Woof!" << std::endl;
}
};
Using Objects
After defining a class, you can create objects:
Dog myDog;
myDog.bark();
This will output "Woof!" to the console.
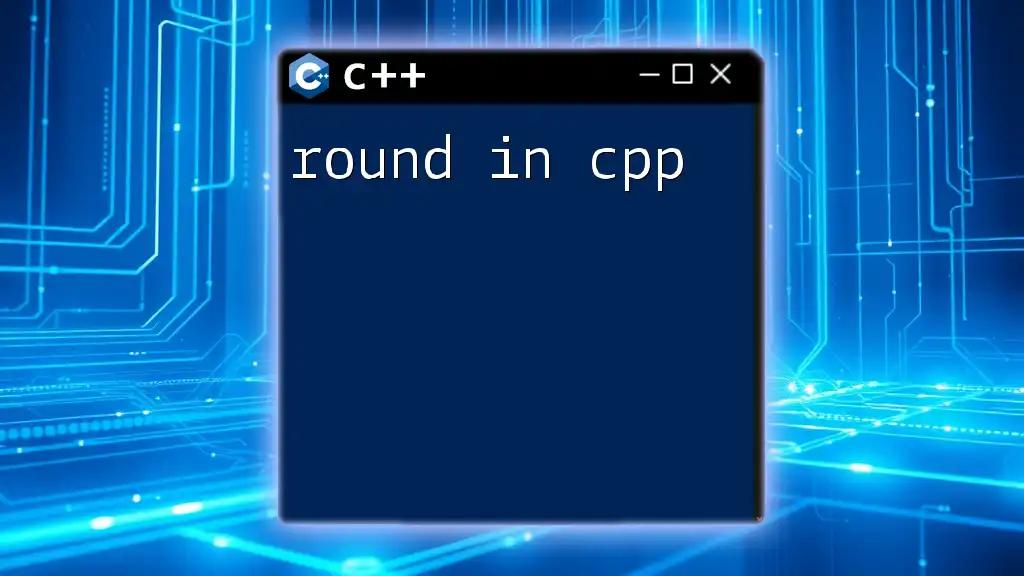
Advanced C++ Concepts
Pointers and References
Pointers store memory addresses and enable dynamic memory management. They can be tricky but are essential for understanding C++ deeply.
Here’s how to declare and use a pointer:
int a = 10;
int* p = &a; // p now holds the address of a
std::cout << *p << std::endl; // Outputs the value of a
Memory Management
Managing memory effectively is crucial, especially in larger applications. Use `new` to allocate memory and `delete` to free it:
int* arr = new int[10]; // Dynamically allocate an array of 10 integers
delete[] arr; // Release memory
Exception Handling
C++ uses `try`, `catch`, and `throw` to manage exceptions. This allows your program to handle errors gracefully. Here’s a basic example:
try {
throw std::runtime_error("Error occurred");
} catch (const std::exception& e) {
std::cout << e.what() << std::endl; // Outputs "Error occurred"
}
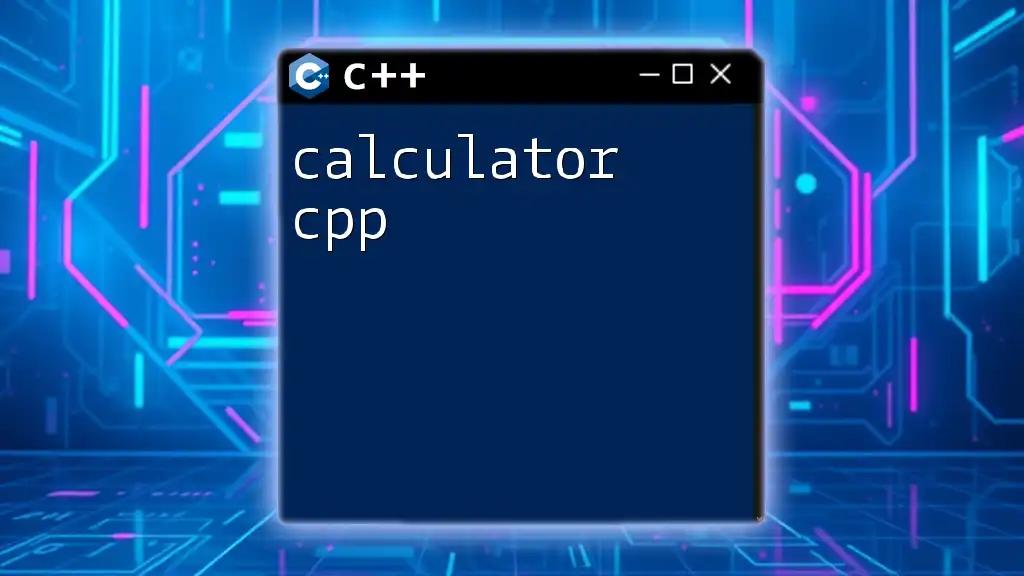
Best Practices in C++
To excel in foundation C++, adhere to best practices:
- Code Readability: Use clear naming conventions & maintain consistent formatting.
- Proper Use of Comments: Document complex sections of code to aid understanding.
- Optimization Techniques: Focus on efficient algorithms and structures.
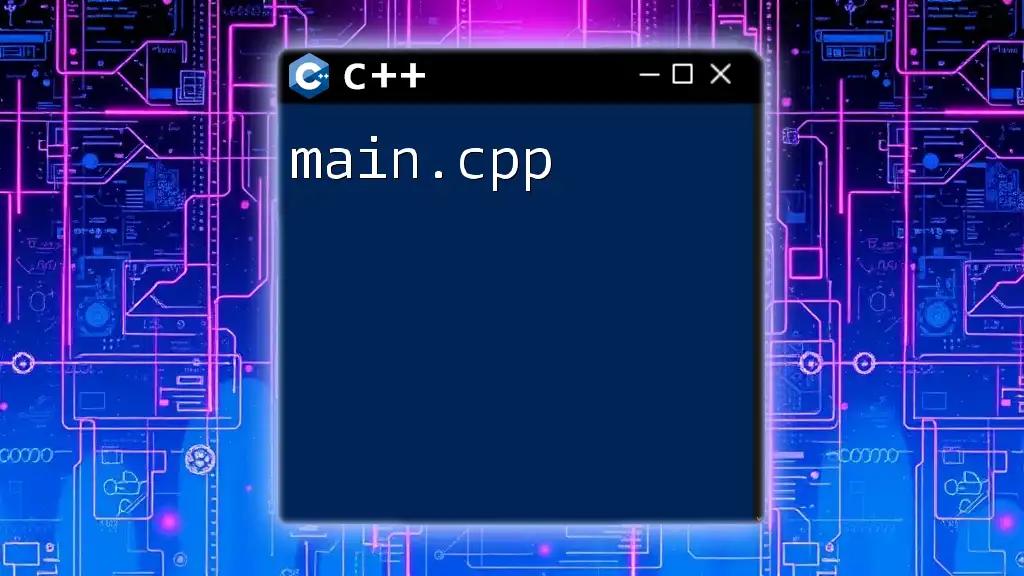
Conclusion
Mastering foundation C++ is essential for aspiring programmers and software developers. Key concepts such as variables, control structures, functions, classes, and advanced topics form the backbone of C++. With practice and application, you can acquire the skills needed to write robust C++ programs.
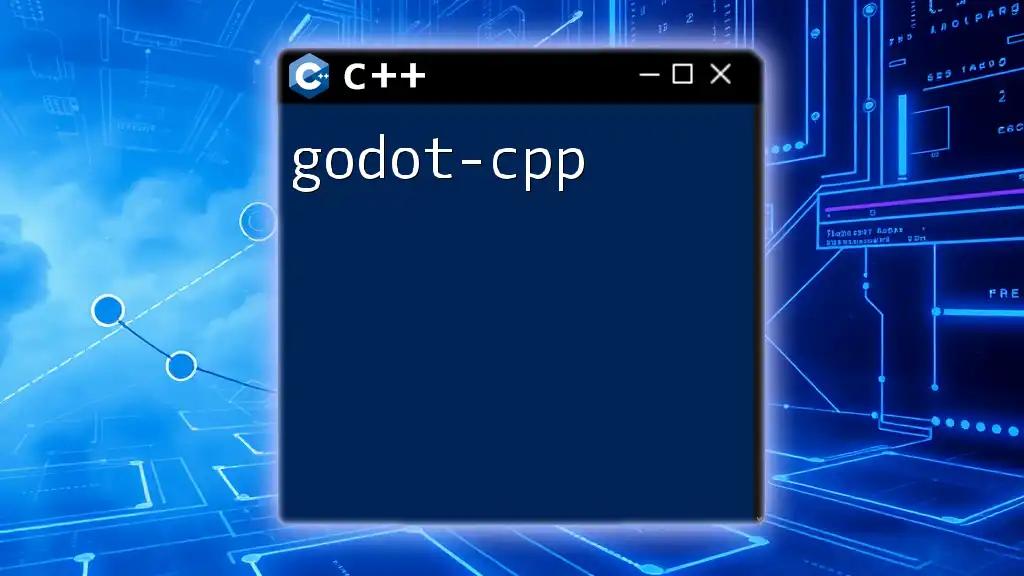
Additional Resources
To further enhance your understanding, explore recommended books and online courses dedicated to C++. Joining community forums or online groups can also provide additional support and practice opportunities, and don't overlook challenge websites for honing your skills.