The `main.cpp` file is the starting point of a C++ program, where the execution begins, and typically includes the `main` function that returns an integer.
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
The Structure of a C++ Program
What is main()?
The `main()` function is the heart of every C++ program. It's where the execution begins and is essential for any standalone C++ application. The syntax of the `main()` function is simple:
int main() {
// code here
return 0;
}
The `main()` function must always return an integer value, which typically indicates if the program executed successfully or encountered an error.
Basic Syntax of main.cpp
A standard `main.cpp` setup includes headers for libraries, function declarations, and, of course, the main function. Here’s the breakdown:
-
Headers: This is where you include necessary libraries. For instance, if you need to use input and output functions, you would include the iostream library.
-
Function Declarations: These are optional but can help organize your code, especially in larger projects.
-
Main Function: As discussed, this function holds your primary logic.
Consider a simple `main.cpp` example:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
In this example, the `#include <iostream>` directive brings in standard input-output features, allowing the program to output text to the console.
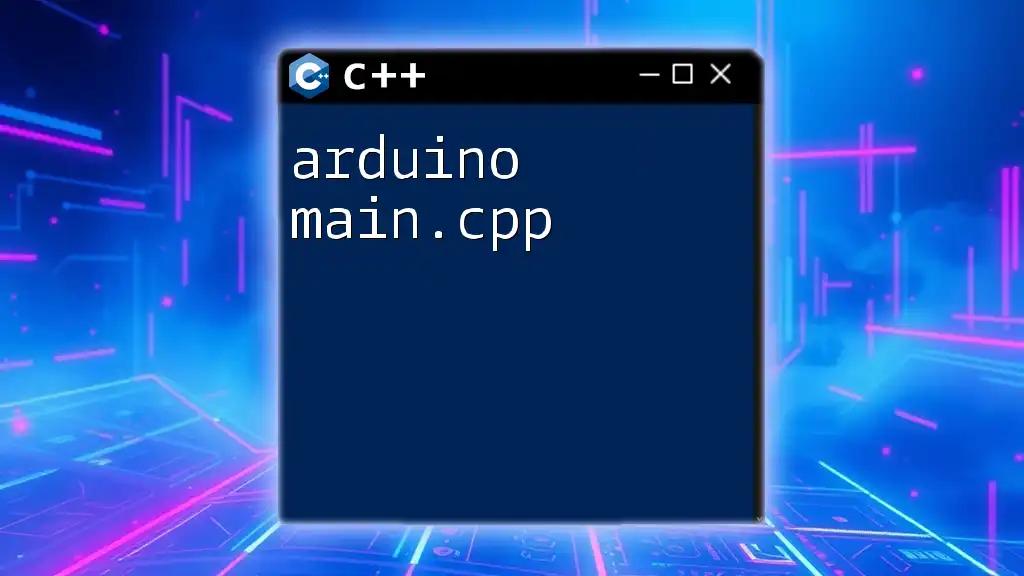
The Role of the `return` Statement
Understanding Return Types
Every C++ function, including `main()`, has a return type. The `main()` function typically returns an integer. Returning `0` signifies that the program completed successfully. If you return a non-zero value, it signals an error condition.
int main() {
// Successful execution
return 0;
}
This simple return mechanism can help other processes or scripts that execute your program understand if it completed successfully or if there were issues.
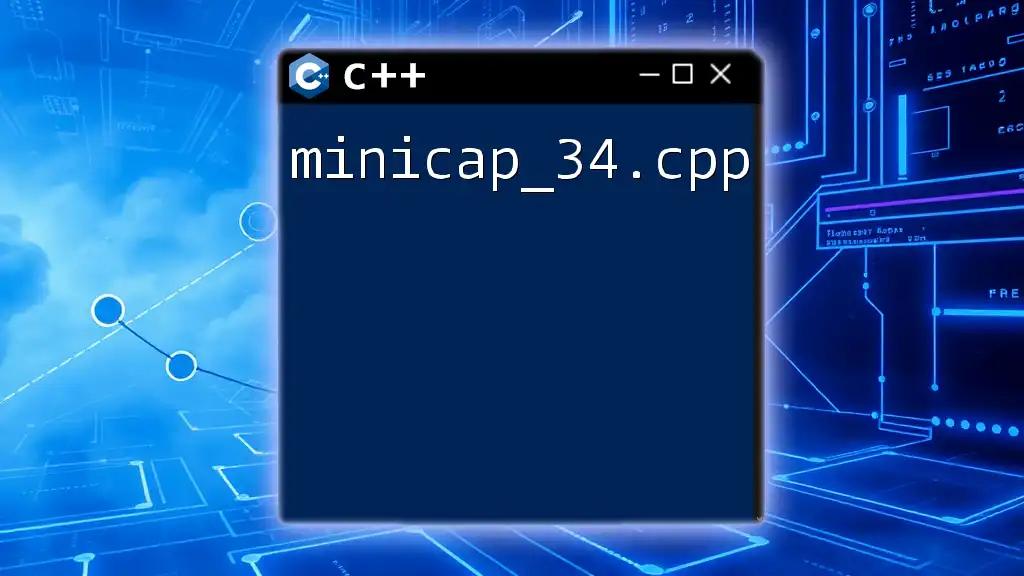
Input and Output in main.cpp
Using std::cout
To display output on the console, you utilize `std::cout`. This standard output stream allows you to print text and variables to the terminal. For instance:
std::cout << "This is a message!" << std::endl;
This line outputs the string “This is a message!” to the console and then moves to the next line.
Getting User Input with std::cin
Input is handled using `std::cin`. This allows your program to receive user data. Here's how to capture user input:
int age;
std::cout << "Enter your age: ";
std::cin >> age;
In this snippet, the program prompts the user and waits for them to enter their age, which is stored in the `age` variable.
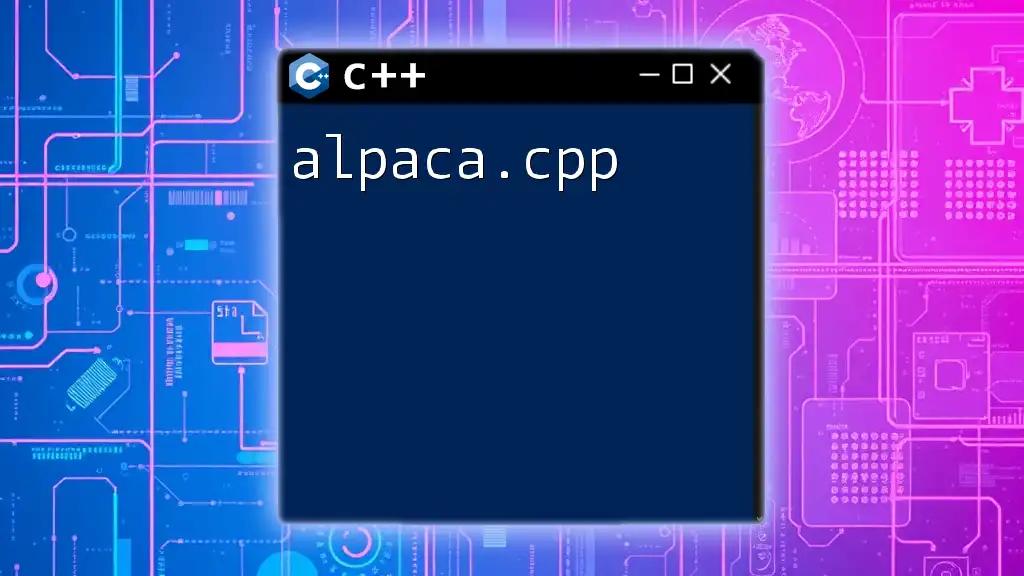
Command Line Arguments in main.cpp
What are Command Line Arguments?
Command line arguments enable your program to accept additional inputs when executed from a command line interface. The signature of the `main()` function can be expanded to accommodate these arguments:
int main(int argc, char* argv[]) {
// code here
}
Here, `argc` represents the number of arguments passed, while `argv` is an array of C-strings representing the actual arguments.
Example using Command Line Arguments
To see command line arguments in action, consider the following example:
int main(int argc, char* argv[]) {
if (argc > 1) {
std::cout << "Hello, " << argv[1] << "!" << std::endl;
}
return 0;
}
In this case, if a user runs the program like this:
./myprogram John
The output would be “Hello, John!” This allows for more dynamic interactions based on user input from the command line.
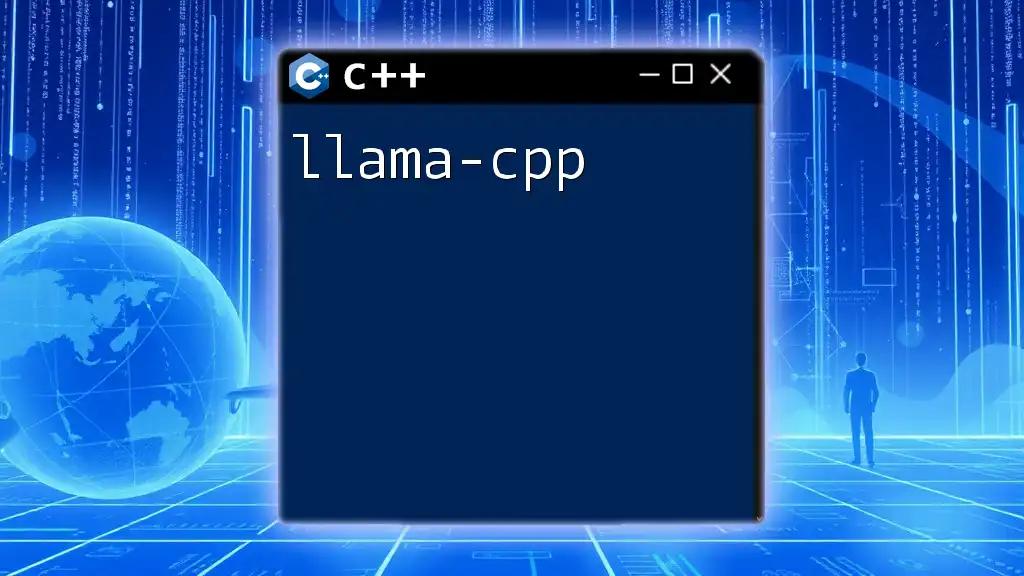
Creating a Complete `main.cpp` Example
Step-by-step Development
Let’s walk through building a more complex program, a simple calculator. This will allow us to see various aspects of `main.cpp` in action.
Code Example for a Simple Calculator
The following code illustrates a simple calculator that can add, subtract, multiply, or divide two numbers:
#include <iostream>
int main() {
int a, b;
char op;
std::cout << "Enter first number: ";
std::cin >> a;
std::cout << "Enter second number: ";
std::cin >> b;
std::cout << "Enter operator (+, -, *, /): ";
std::cin >> op;
switch (op) {
case '+':
std::cout << "Result: " << a + b << std::endl;
break;
case '-':
std::cout << "Result: " << a - b << std::endl;
break;
case '*':
std::cout << "Result: " << a * b << std::endl;
break;
case '/':
if (b != 0) {
std::cout << "Result: " << a / b << std::endl;
} else {
std::cout << "Error: Division by zero is not allowed." << std::endl;
}
break;
default:
std::cout << "Invalid operator" << std::endl;
}
return 0;
}
In this example, the program takes two numbers and an operator as input. It validates the operator and performs the respective operation, displaying the result or error message accordingly.
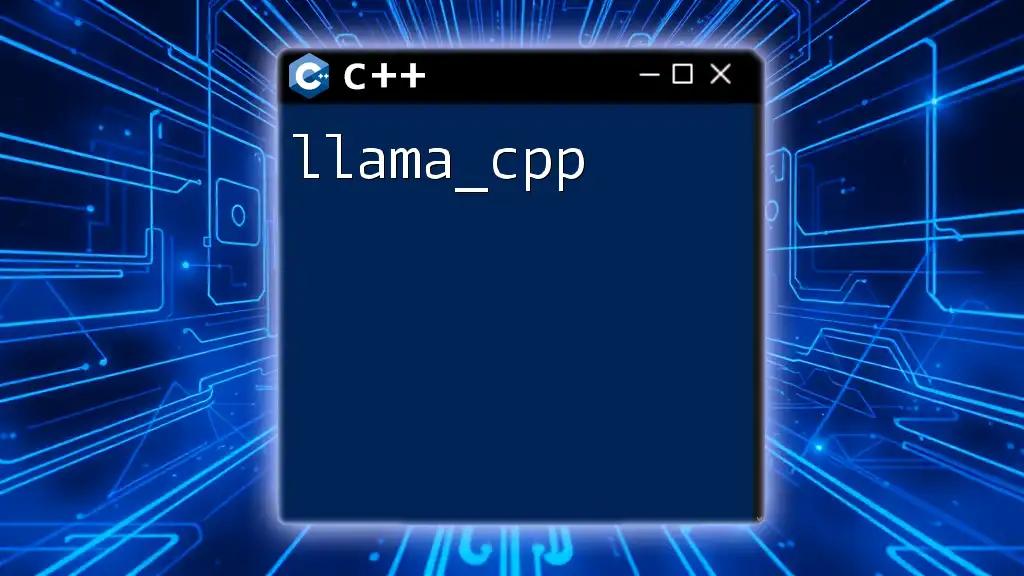
Common Mistakes to Avoid in main.cpp
Debugging Tips
- Missing Return Statement: Ensure to return a value at the end of `main()`. Omitting this can cause unexpected behavior when the program is executed.
- Incorrect Use of Headers: Missing necessary headers will lead to compilation errors. Always include what you need.
- Mishandling Input/Output: Make sure to prompt users clearly and validate inputs when necessary.
Develop a habit of testing thoroughly to catch these errors early in your coding journey.
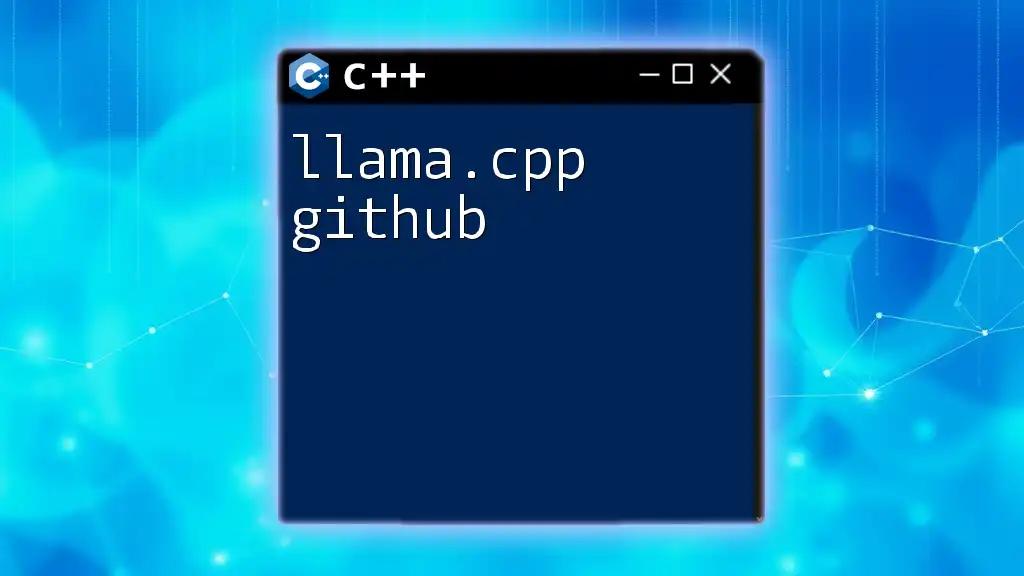
Best Practices for Writing main.cpp
Code Readability
Maintaining a clear code structure improves maintainability and collaboration. Use consistent naming conventions, and ensure your code is logically organized.
Comments and Documentation
Helpful comments can guide others (and yourself) when revisiting the code later. Always document your functions and the purpose of your main logic, especially for complex tasks.
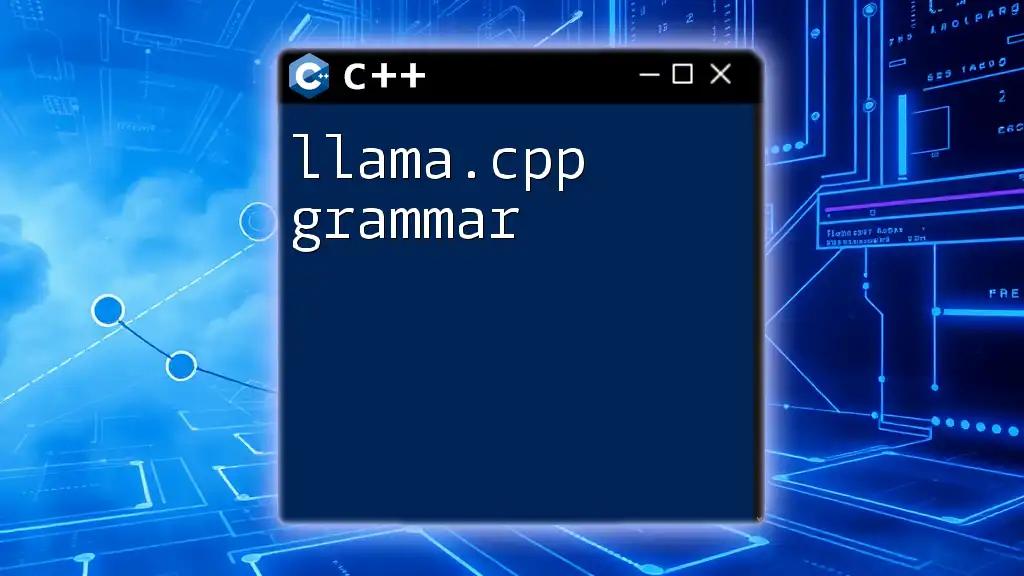
Conclusion
In summary, understanding main.cpp and the `main()` function is fundamental for anyone venturing into C++ programming. This article covered everything from basic syntax to handling user input, command line arguments, and creating complete applications. The more you practice writing and refining your main.cpp files, the more proficient you will become in C++. Explore and experiment with new functions, and don't hesitate to seek further resources as needed.
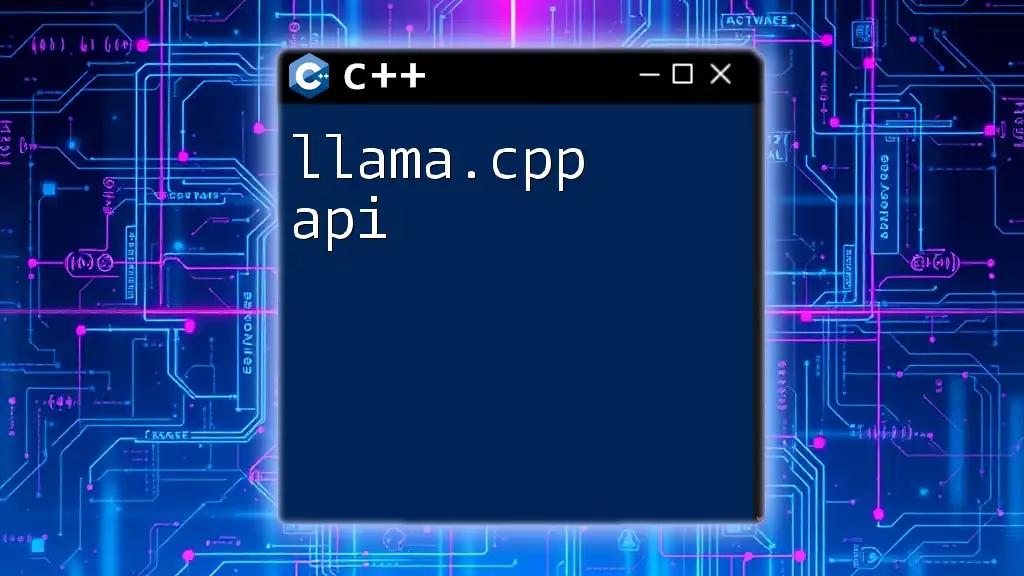
FAQs about main.cpp
Feel free to look out for common queries related to main.cpp, including misunderstandings about its structure, typical errors, and additional best practices. Engaging with these topics will solidify your understanding of this essential part of C++ programming.