In C++, you can define a simple function that adds two numbers and returns the result using the following syntax:
int add(int a, int b) {
return a + b;
}
Importance of C++ in Programming
C++ is a powerful, general-purpose programming language that has become a staple in various fields of software development. Its object-oriented nature allows developers to create complex systems efficiently and effectively. Some key areas where C++ is widely utilized include:
- Game Programming: Many popular game engines and games are built using C++ due to its performance and real-time processing capabilities.
- Systems Programming: Operating systems and system applications frequently leverage C++ for their speed and direct hardware interaction.
- Application Development: From desktop applications to high-performance applications in finance, C++ remains a strong choice.
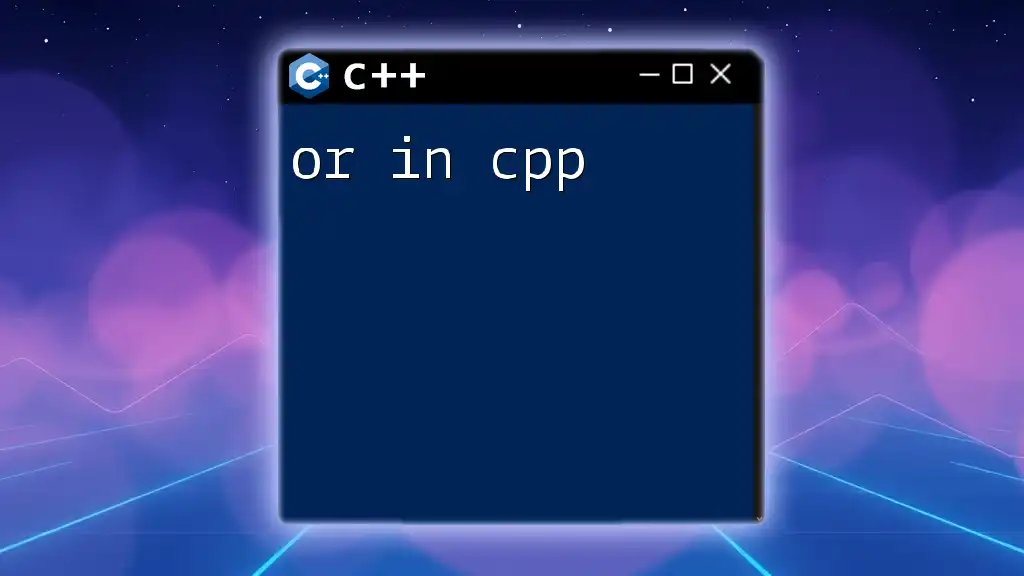
Purpose of This Guide
In this article, we aim to provide a clear and concise understanding of the "in" command in C++, focusing on its usage in taking input from users and other sources. By the end of this guide, you’ll have a solid foundation to handle input operations effectively within your C++ programs.
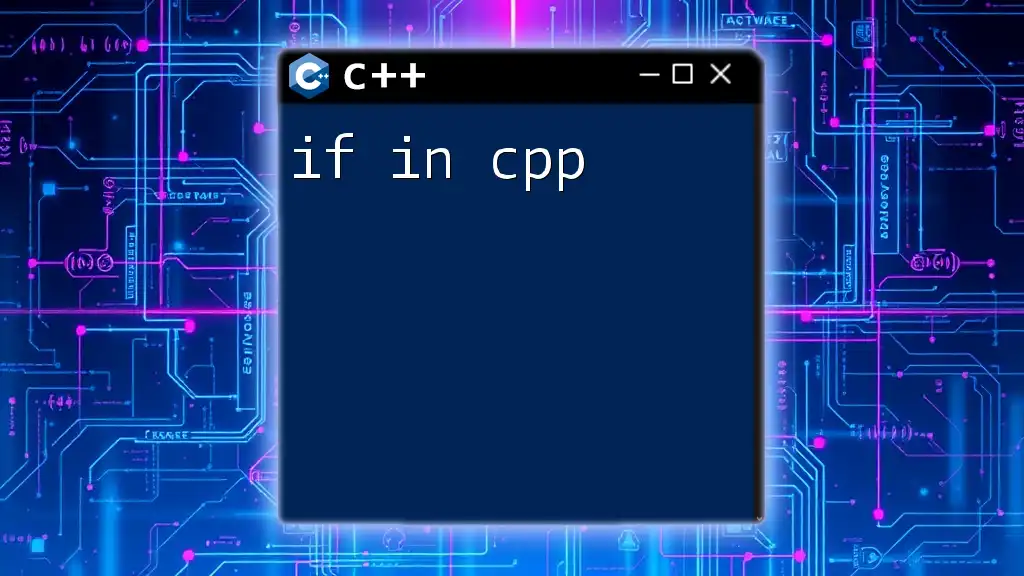
Understanding Input/Output Operations in C++
The Role of Input/Output in Programming
Input and output operations are crucial for any program, as they facilitate interaction between the program and the user. When a program requires user input to function, it must have a robust method for capturing this data. Effectively handling input not only improves user experience but also enhances the reliability of software.
Overview of Standard Input and Output Streams
In C++, there are standard streams to facilitate input and output operations. The two primary streams are:
- `cin`: This stream takes input from the standard input device, typically the keyboard.
- `cout`: This stream outputs data to the standard output device, usually the console.
Code Snippet: Basic Input and Output
Below is a simple example demonstrating how to use `cin` and `cout` in a C++ program:
#include <iostream>
using namespace std;
int main() {
int number;
cout << "Enter a number: ";
cin >> number; // Taking user input
cout << "You entered: " << number << endl; // Displaying output
return 0;
}
In the above example, the program prompts the user for a number and then displays it back to the console. This basic structure introduces concepts of input and output in C++.
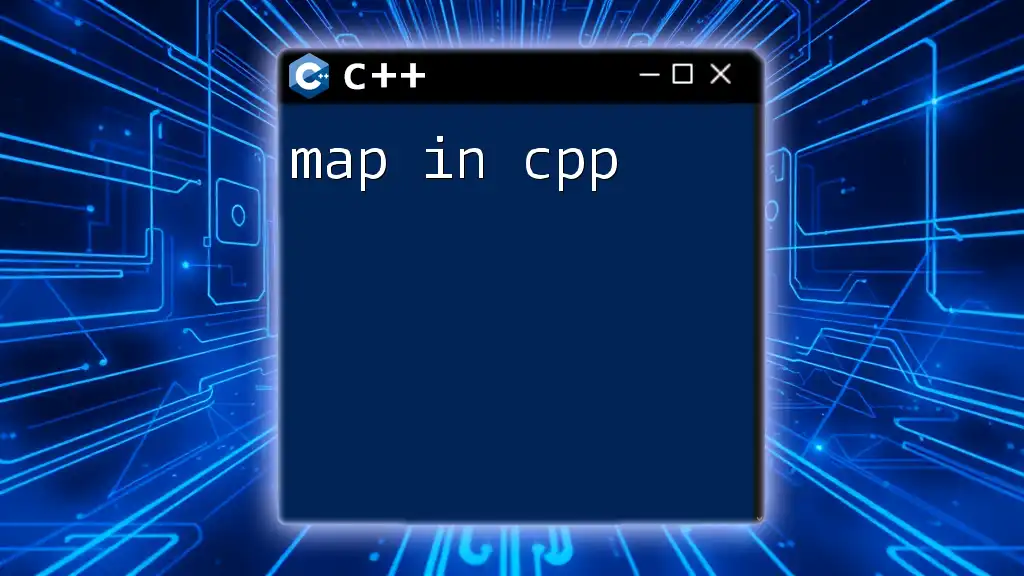
The Concept of "in" with Streams
What Does "in" Represent?
When we refer to the "in" command in C++, we typically discuss the input aspect associated with the `cin` stream. This command is critical for obtaining data from the user or external sources, enabling the program to function dynamically based on user interaction.
Differences Between Input Methods
There are multiple ways to capture input in C++, each serving different contexts:
cin vs. ifstream
- `cin`: This is used for standard input, typically from the keyboard.
- `ifstream`: An input file stream allows reading data from files. This is particularly useful when dealing with large datasets or when input needs to be collected from a file instead of via user interaction.
Code Snippet: Using ifstream for File Input
Here’s an example of how to read numbers from a file using `ifstream`:
#include <iostream>
#include <fstream>
using namespace std;
int main() {
ifstream inputFile("data.txt"); // Opening a file named 'data.txt'
int number;
if (inputFile.is_open()) {
while (inputFile >> number) { // Reading numbers one by one from the file
cout << "Read number: " << number << endl; // Display each number read
}
inputFile.close(); // Closing the file
} else {
cout << "Unable to open file." << endl; // Error handling
}
return 0;
}
In this snippet, the program opens a file named `data.txt` and reads integers until it reaches the end of the file, outputting each number. If the file cannot be opened, it alerts the user.
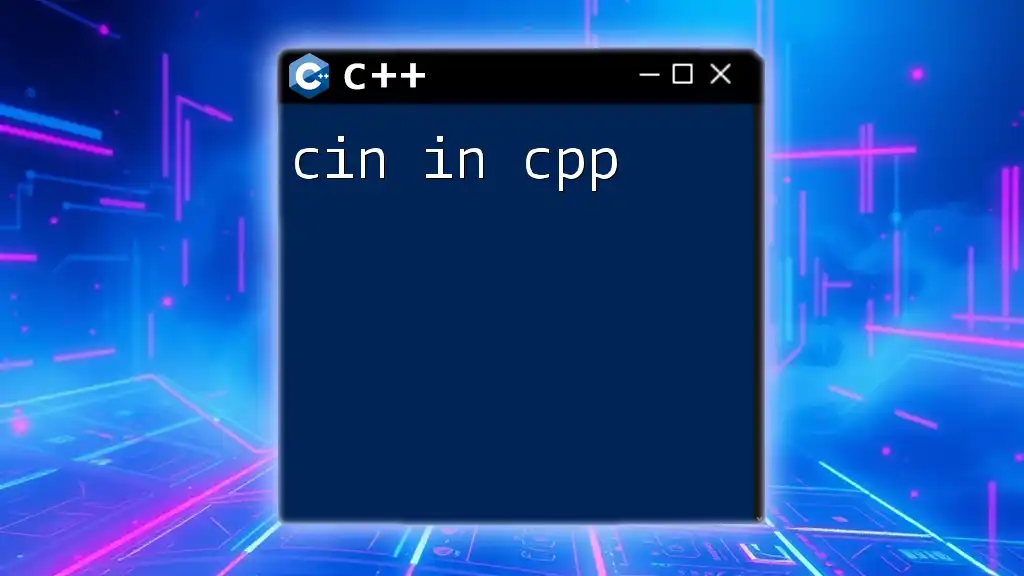
Practical Implementations of "in" Command
Reading User Input
One of the primary uses of the "in" command in C++ is to read user input effectively. The flexibility of `cin` enables the reading of various data types directly
Using cin for Different Data Types
C++ allows you to read integers, floating-point numbers, strings, and other types using `cin`. The data type you specify will determine how C++ interprets the input.
Code Snippet: Using cin with Different Types
Consider the following example that captures multiple types of input:
#include <iostream>
#include <string>
using namespace std;
int main() {
int age;
double height;
string name;
cout << "Enter your name: ";
cin >> name; // Reading a string
cout << "Enter your age: ";
cin >> age; // Reading an integer
cout << "Enter your height (in meters): ";
cin >> height; // Reading a floating-point number
cout << "Hello " << name << ", you are " << age << " years old and " << height << "m tall." << endl;
return 0;
}
In this program, we read a user's name, age, and height, demonstrating the versatility of the `cin` command.
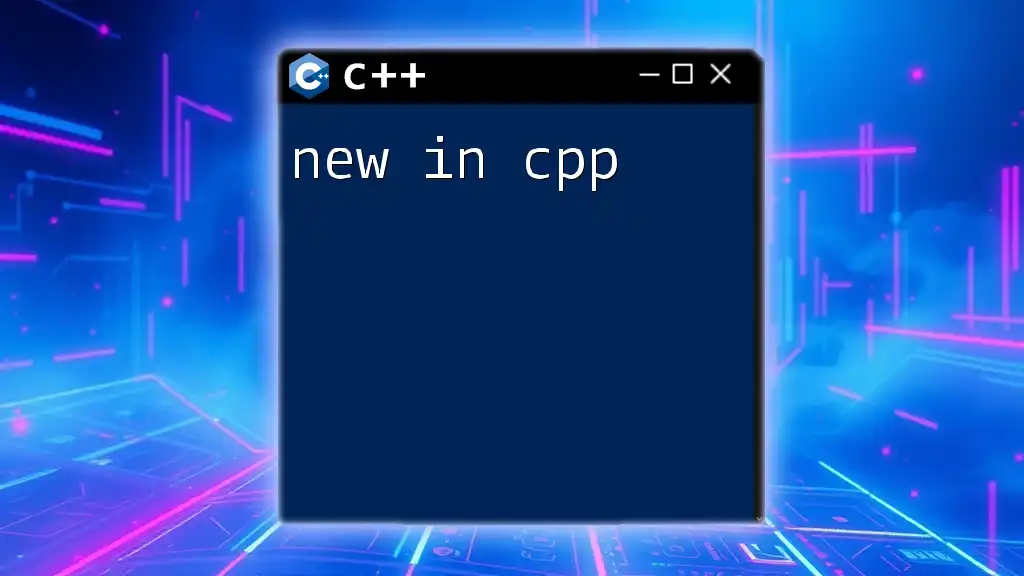
Error Handling with "in"
Importance of Validating Input
Validating user input is essential in programming. An unvalidated input can lead to unexpected behavior, program crashes, or security vulnerabilities. Handling errors gracefully improves the robustness of your code.
Techniques for Error Checking
Using cin.fail() to Detect Errors
The `fail()` method is a helpful tool that indicates whether the last input operation encountered an error. Using it, you can implement error handling to ensure that your program receives valid input.
Code Snippet: Validating User Input
Here’s how to implement input validation using `cin.fail()`:
#include <iostream>
using namespace std;
int main() {
int number;
cout << "Enter a number: ";
while (!(cin >> number)) { // Check for input validity
cin.clear(); // clear input buffer
cin.ignore(numeric_limits<streamsize>::max(), '\n'); // ignore bad input
cout << "Invalid input. Please enter an integer: "; // Prompt user again
}
cout << "You entered: " << number << endl; // Valid input reached
return 0;
}
In this example, if a user provides invalid input (e.g., inputting a letter instead of a number), the program will clear the input buffer and prompt the user again until valid input is received.
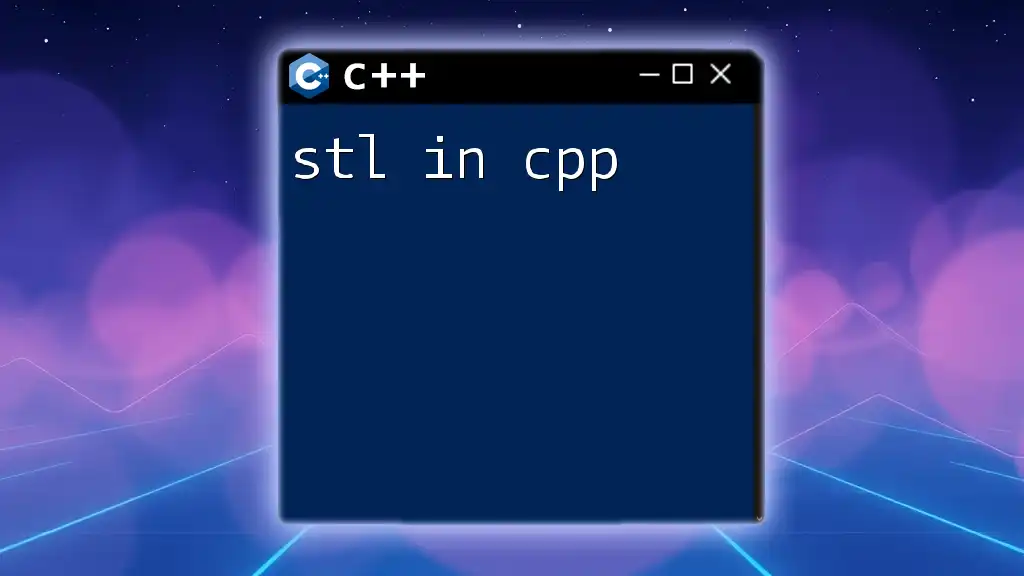
Conclusion
In this guide, we explored the "in" command in C++ and how it relates to user input through the `cin` stream and file input using `ifstream`. You learned about the importance of input handling, various methods to read different data types, and the necessity of validating user input to create reliable programs.
While this article provides a foundation, C++ offers much more in the realm of input and output operations. For further development and nuanced techniques, consider exploring additional resources and courses dedicated to C++ programming.
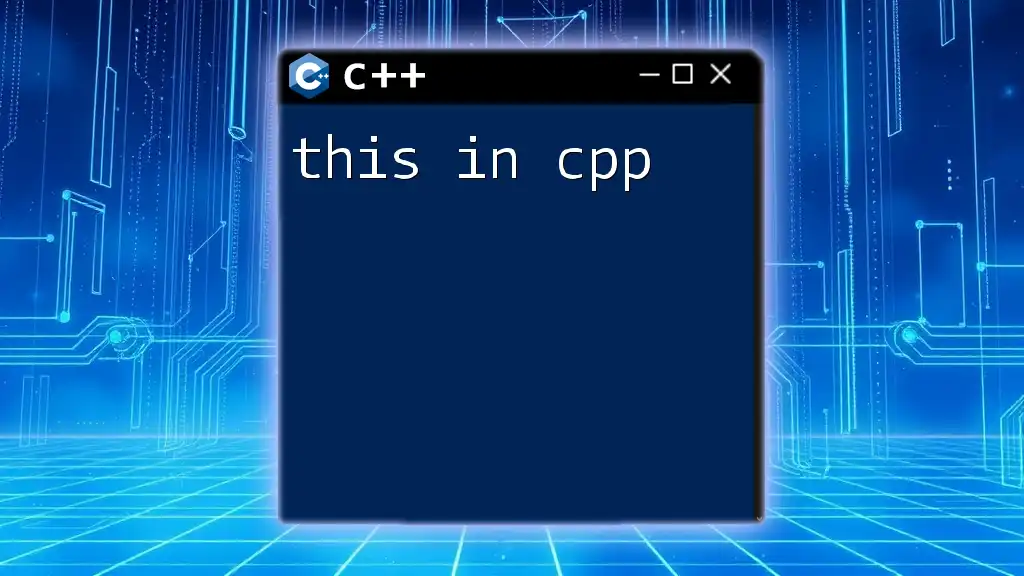
Call to Action
Join our community to receive more tips and tutorials tailored for C++ enthusiasts. Share your experiences or challenges with handling input in C++, and consider signing up for our courses that focus on enhancing your understanding and expertise in C++ programming!