In C++, the `pass` command is not a standard feature, but if you meant to discuss passing parameters to functions, here is a succinct explanation along with a code snippet:
In C++, you can pass arguments to a function by value or by reference to manipulate the data within the function.
#include <iostream>
void displayNumber(int num) { // Pass by value
std::cout << "The number is: " << num << std::endl;
}
void increment(int& num) { // Pass by reference
num++;
}
int main() {
int myNumber = 5;
displayNumber(myNumber); // Passing by value
increment(myNumber); // Passing by reference
displayNumber(myNumber); // Output will reflect the increment
return 0;
}
What Does "Pass" Mean in C++?
In the context of C++, "pass" refers to how data is transmitted to functions. When a function is called, it often requires input in the form of parameters. Understanding the different methods for passing data is crucial for effective coding. The three primary methods are passing by value, passing by reference, and passing by pointer, each with its own set of characteristics and implications for program behavior.
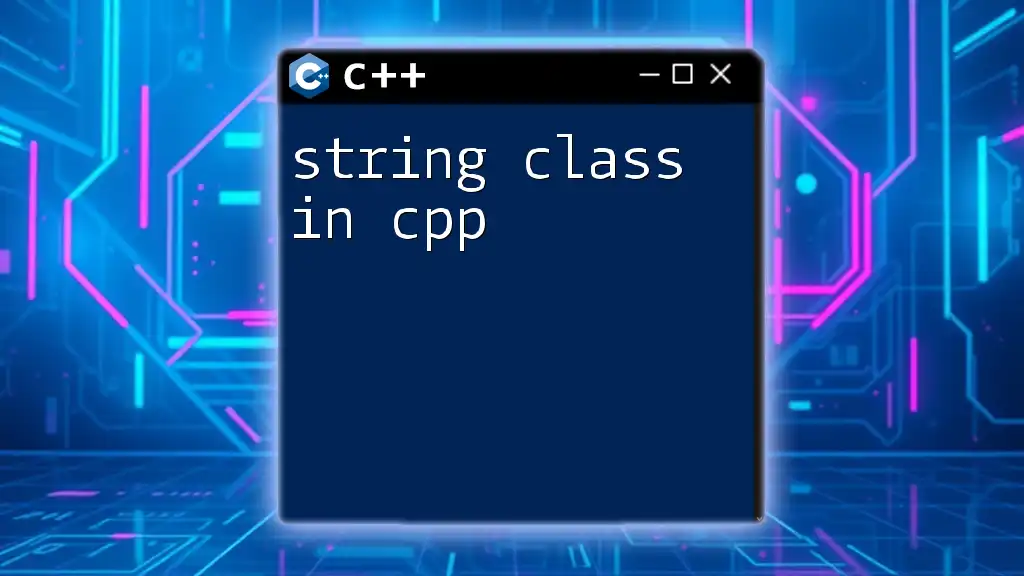
Types of Passing in C++
Pass by Value
Pass by value is the most straightforward method where a copy of the data is created for the function. When the function modifies the parameter, the changes do not affect the original variable.
Advantages:
- Safety: The original data cannot be modified unintentionally, reducing side effects.
- Easier to reason about the state of variables since changes are localized.
Disadvantages:
- Performance issue: For large data structures, copying can slow down performance and consume additional memory.
void modifyValue(int num) {
num = num + 10;
std::cout << "Inside function: " << num << std::endl; // Outputs modified num
}
int main() {
int original = 5;
modifyValue(original);
std::cout << "In main: " << original << std::endl; // Outputs the original value
return 0;
}
In this example, the variable `original` remains unchanged when passed to `modifyValue`, illustrating the concept of pass by value.
Pass by Reference
In pass by reference, instead of passing a copy, you pass a reference to the original variable. This means changes made within the function affect the original variable directly.
Advantages:
- Performance: No overhead from copying data, especially beneficial for large structures.
- Direct access: Offers the ability to modify the original variable.
Disadvantages:
- Risk of side effects: Accidental modifications can lead to bugs that are hard to trace.
void modifyReference(int &num) {
num = num + 10;
std::cout << "Inside function: " << num << std::endl; // Outputs modified num
}
int main() {
int original = 5;
modifyReference(original);
std::cout << "In main: " << original << std::endl; // Outputs modified value
return 0;
}
This example clearly shows that `original` is modified by the function, making it essential to use pass by reference judiciously.
Pass by Pointer
Pass by pointer involves passing the address of a variable, which allows the function to access and modify the variable directly.
Advantages:
- Flexibility: Functions can check for null pointers, allowing them to handle optional parameters gracefully.
- Direct manipulation: Similar to reference passing but with explicit control over memory.
Disadvantages:
- Complexity: Increased chance of errors through pointer arithmetic or dereferencing issues.
- Memory management: The possibility of memory leaks and dangling pointers if not handled correctly.
void modifyPointer(int *numPtr) {
*numPtr = *numPtr + 10; // Dereferencing the pointer
std::cout << "Inside function: " << *numPtr << std::endl; // Outputs modified value
}
int main() {
int original = 5;
modifyPointer(&original); // Passing address of original
std::cout << "In main: " << original << std::endl; // Outputs modified value
return 0;
}
In this case, the function modifies the actual integer pointed to by `numPtr`, demonstrating the effectiveness of passing by pointer.
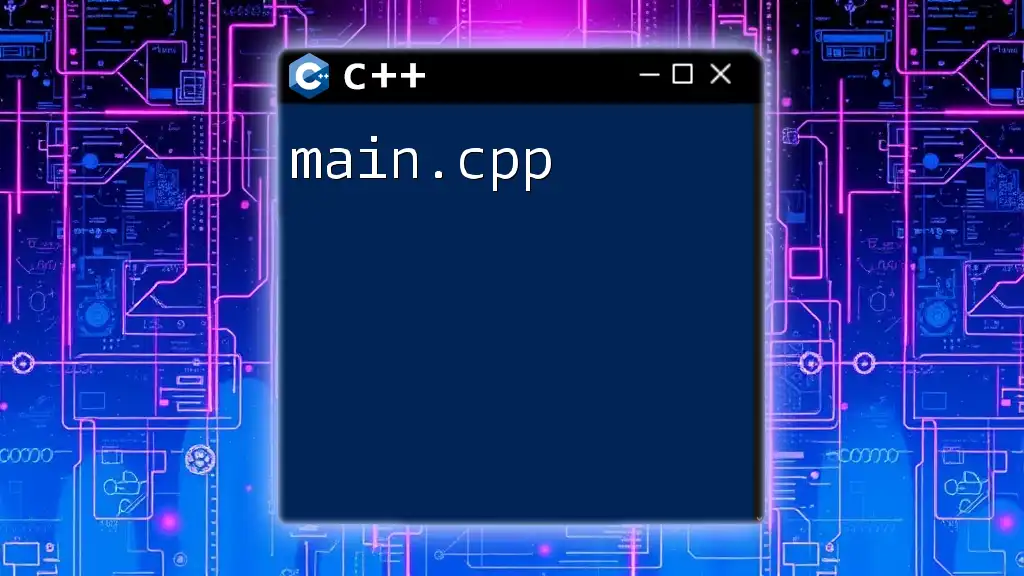
The Role of Const in Passing Arguments
Using `const` in parameter definitions can prevent unintended modifications to the arguments. This is particularly important in contexts where maintainability and safety are key concerns.
Passing by Value with Const
When passing by value, you can still use `const` to indicate that the value should not be modified within the function. For example:
void displayValue(const int num) {
std::cout << num << std::endl;
}
This preserves the safety of pass by value while ensuring that the function does not alter the input.
Passing by Reference with Const
Using `const` with pass by reference ensures that the referred variable is not susceptible to modification, providing the benefits of performance while maintaining safety.
void displayReference(const int &num) {
std::cout << num << std::endl;
}
Here, the input can be large without copy overhead, and it remains untouched during the function's execution.
Passing by Pointer with Const
When using pointers, `const` can be applied to either the pointer itself or the value pointed to, preventing modifications.
void displayPointer(const int *numPtr) {
std::cout << *numPtr << std::endl;
}
This technique allows functions to safely access data without the risk of altering the original data.
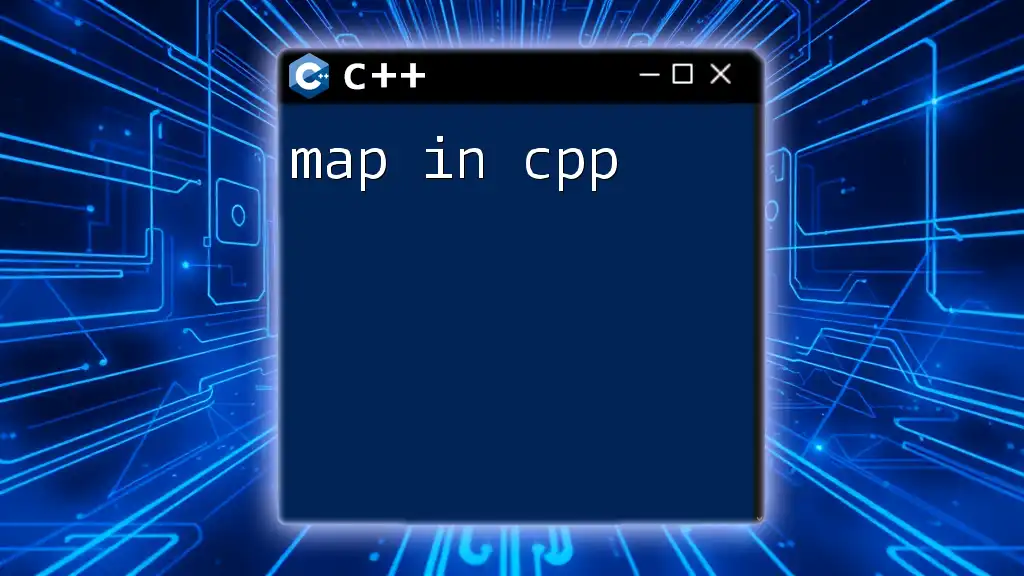
Variadic Functions: Passing a Variable Number of Arguments
Sometimes, a function may need to accept a variable number of arguments. This is accomplished using variadic functions, which enable flexible argument lists.
Definition and Explanation: Variadic functions utilize the `<cstdarg>` header to handle a series of arguments of potentially different types.
Code Example:
#include <iostream>
#include <cstdarg>
void printNumbers(int count, ...) {
va_list args;
va_start(args, count);
for (int i = 0; i < count; ++i) {
std::cout << va_arg(args, int) << " ";
}
va_end(args);
}
int main() {
printNumbers(5, 1, 2, 3, 4, 5);
return 0;
}
This function can print an arbitrary number of integers, demonstrating the flexibility offered by variadic functions.
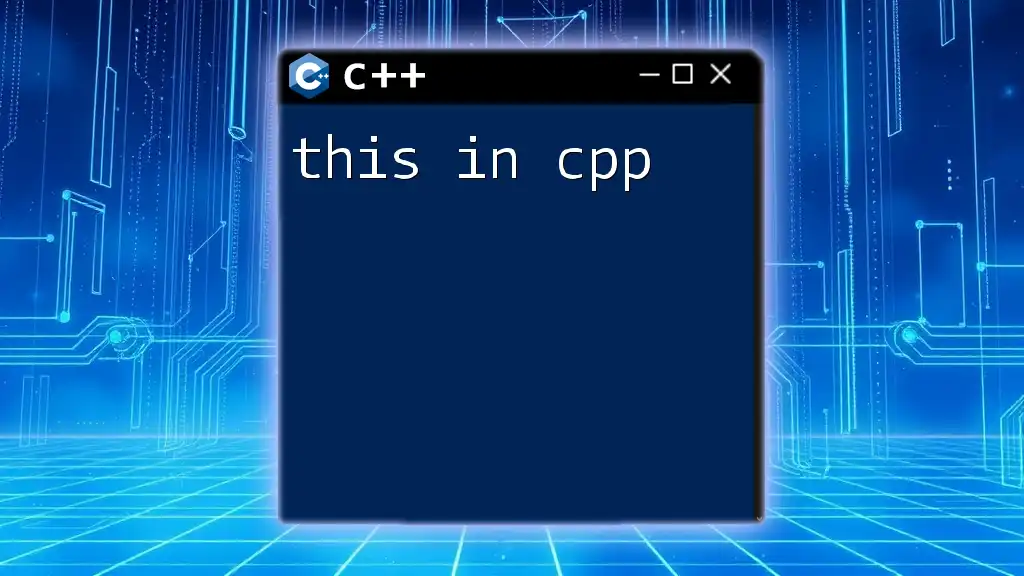
Best Practices for Passing in C++
When deciding how to pass data to functions, consider the following best practices:
- Choose the Right Method: The choice between values, references, and pointers should involve considerations regarding performance, safety, and the intended function behavior.
- Avoid Common Pitfalls: Memory leaks and dangling pointers are common issues associated with pointer usage. Always ensure that memory is adequately managed.
- Utilize `std::move` for Efficiency: For objects that are costly to copy, consider using `std::move` with rvalue references to transfer ownership without the cost of copying.
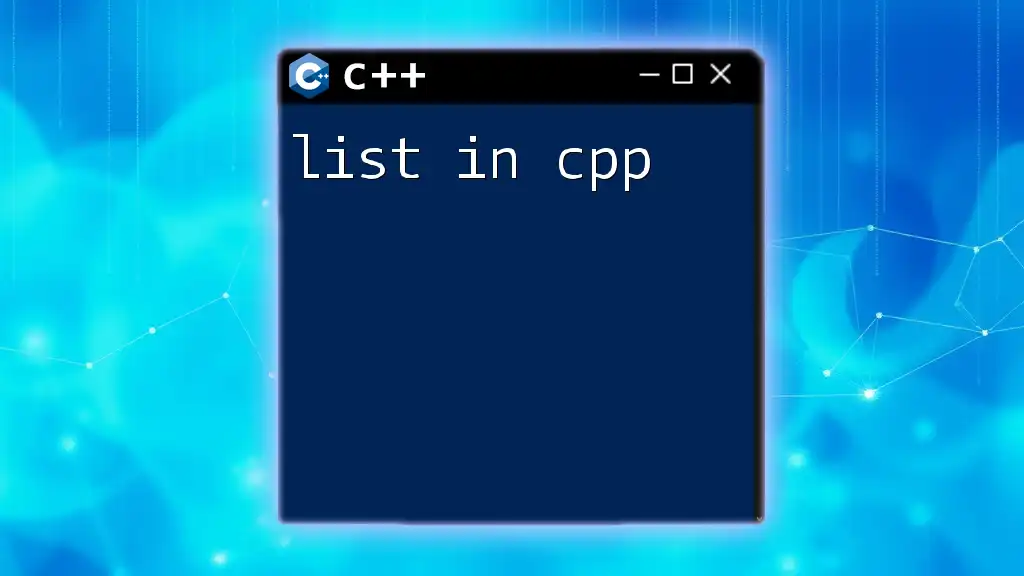
Conclusion
Understanding how to pass in CPP is fundamental to writing efficient and maintainable code. Each passing technique has its benefits and limitations, and mastering them will empower you to select the most effective approach for your functions. As you gain familiarity with these concepts, you'll be better equipped to tackle more advanced C++ topics and develop robust applications.
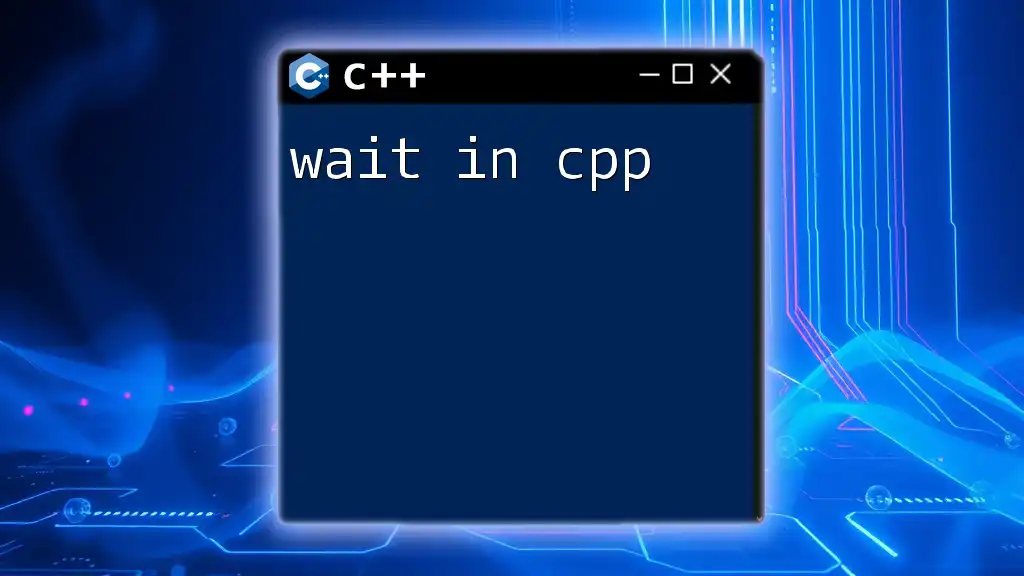
Call to Action
Stay tuned for more C++ tips and tricks to enhance your coding skills! If you have personal experiences or challenges related to passing arguments in C++, share them in the comments section below. Happy coding!