The `strcmp` function in C++ is used to compare two C-style strings and returns an integer indicating their relationship: 0 if they are equal, a negative value if the first string is less than the second, and a positive value if the first string is greater.
#include <iostream>
#include <cstring>
int main() {
const char* str1 = "Hello";
const char* str2 = "World";
int result = strcmp(str1, str2);
if (result == 0) {
std::cout << "Strings are equal." << std::endl;
} else if (result < 0) {
std::cout << "str1 is less than str2." << std::endl;
} else {
std::cout << "str1 is greater than str2." << std::endl;
}
return 0;
}
Understanding the Basics of strcmp
What is strcmp?
`strcmp` is a standard library function in C++ that plays a crucial role in comparing two C-style strings. It helps determine the lexicographical order of the strings being compared. Understanding how to use `strcmp` effectively is fundamental for many programming scenarios, especially when working with strings that are not encapsulated as `std::string`.
Syntax of strcmp
The syntax for `strcmp` is straightforward:
int strcmp(const char* str1, const char* str2);
-
Parameters:
- `str1`: This is the first string to be compared.
- `str2`: This is the second string to be compared.
-
Return Value: The function returns an integer:
- Less than 0: `str1` is less than `str2`.
- Equal to 0: The strings are equal.
- Greater than 0: `str1` is greater than `str2`.
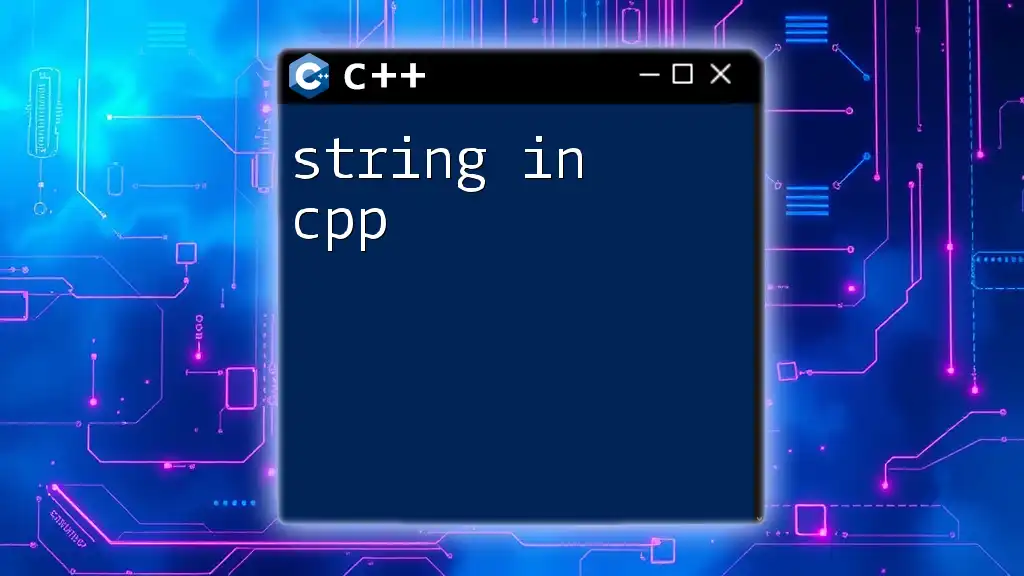
How strcmp Works
Character by Character Comparison
When `strcmp` is called, it compares the strings character by character. It starts from the first character of each string and continues until it finds a character that differs or reaches the end of one of the strings.
The comparison is based on the ASCII values of the characters. For example, the ASCII value of 'a' (97) is less than 'b' (98), so "apple" would be considered less than "banana".
Return Values of strcmp
Understanding the return values of `strcmp` is essential for using it effectively:
-
Return Value < 0: This indicates that `str1` is lexicographically less than `str2`. For example, `strcmp("apple", "banana")` would return a negative number.
-
Return Value = 0: This indicates that both strings are equal. For instance, `strcmp("test", "test")` will return 0.
-
Return Value > 0: This indicates that `str1` is greater than `str2`. For instance, `strcmp("zebra", "apple")` would return a positive value.
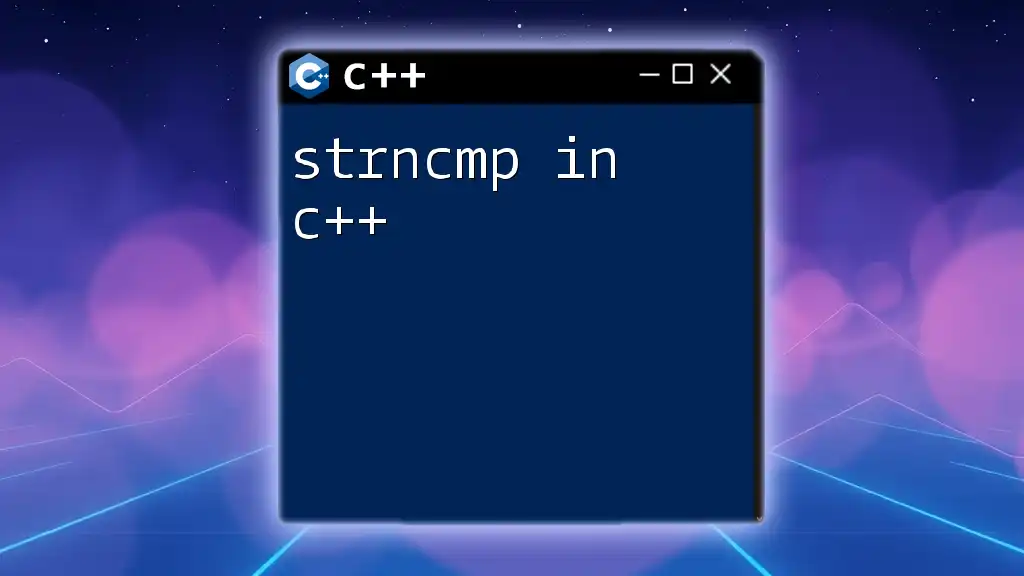
Practical Usage of strcmp in C++
Basic Examples
Here's a simple example that demonstrates the basic usage of `strcmp`:
#include <iostream>
#include <cstring> // Required for strcmp
int main() {
const char* str1 = "apple";
const char* str2 = "banana";
int result = strcmp(str1, str2);
if (result < 0) {
std::cout << "str1 is less than str2" << std::endl;
} else if (result > 0) {
std::cout << "str1 is greater than str2" << std::endl;
} else {
std::cout << "str1 is equal to str2" << std::endl;
}
return 0;
}
Comparing Strings in Conditional Statements
`strcmp` is frequently used in conditional statements to make decisions based on string comparisons. Here is how you might use it:
if (strcmp(str1, str2) == 0) {
// Strings are equal
std::cout << "The strings are equal." << std::endl;
} else {
// Strings are not equal
std::cout << "The strings are not equal." << std::endl;
}
Handling User Input
You can also use `strcmp` with user input to make dynamic comparisons. Here is an example of how to do this:
char input[100];
std::cout << "Enter a string: ";
std::cin >> input;
if (strcmp(input, "test") == 0) {
std::cout << "You entered 'test'" << std::endl;
}
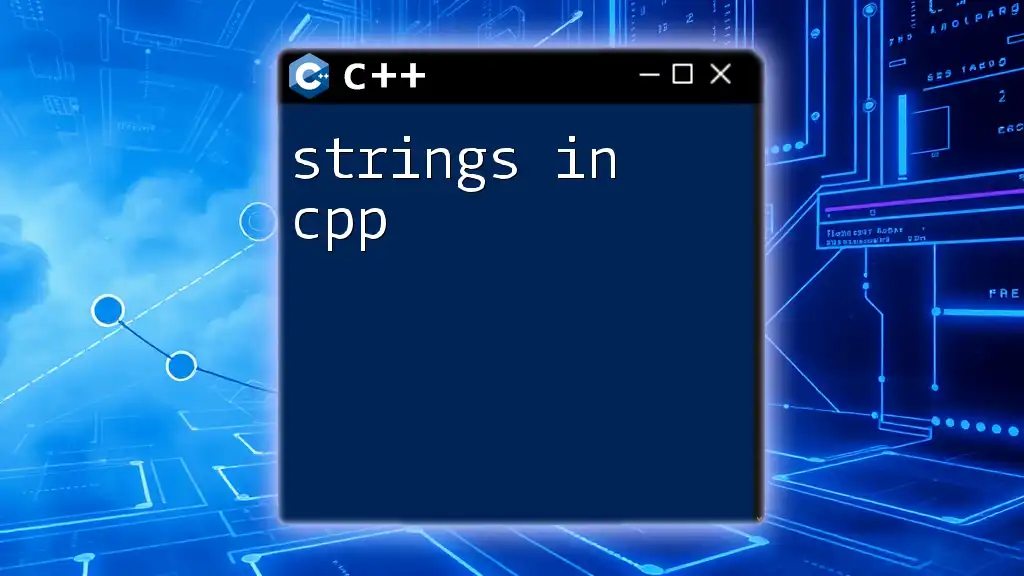
Common Pitfalls with strcmp
Case Sensitivity
One important aspect of `strcmp` is case sensitivity. For instance, `strcmp("Hello", "hello")` would not consider these strings equal due to the difference in the uppercase and lowercase letters. If you need to perform a case-insensitive comparison, consider using `strcasecmp`, which is available on POSIX systems.
Null Pointers and String Safety
Another potential issue arises when null pointers are passed to `strcmp`. If `str1` or `str2` is null, it can lead to undefined behavior and may cause your program to crash. Always ensure that both strings are valid before calling `strcmp`.
Buffer Overflows
When working with C-style strings, be mindful of buffer overflows. Buffer overflows can occur if the string is not null-terminated or if you exceed the boundaries of your allocated array. Always make sure your strings are properly terminated.
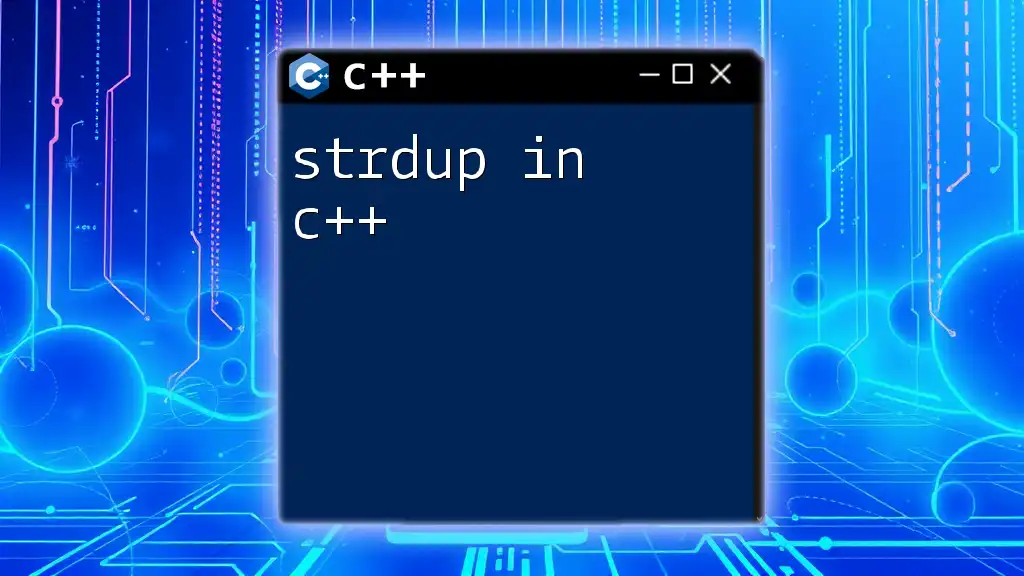
Alternatives to strcmp in C++
Using std::string instead of C-style Strings
In modern C++ programming, it is often preferable to use `std::string` instead of C-style strings for storing and manipulating strings. `std::string` provides operator overloading that allows for direct comparisons:
std::string str1 = "hello";
std::string str2 = "world";
if (str1 == str2) {
// Strings are equal
std::cout << "The strings are equal." << std::endl;
}
This approach eliminates many common pitfalls associated with C-style strings, such as buffer overflows and manual memory management.
Overview of Other Comparison Functions
In addition to `strcmp`, there are other comparison functions available in C++. For instance, `strncmp` allows you to specify the number of characters to compare, which can be useful in certain situations. Additionally, `strcoll` can be used for locale-aware string comparison.
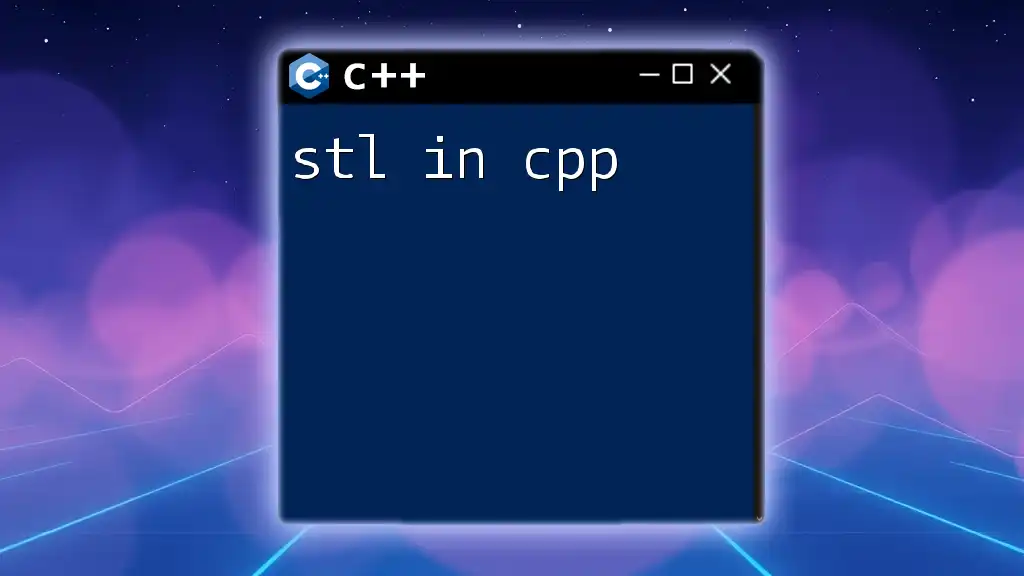
Conclusion
In conclusion, `strcmp` is a powerful function in C++ for comparing C-style strings, and it is important to understand its behavior, usage, and some common pitfalls. Practice using `strcmp` in various scenarios to strengthen your grasp of string comparisons. While `strcmp` is effective, consider leveraging `std::string` for a safer and more flexible approach in modern C++ programming.
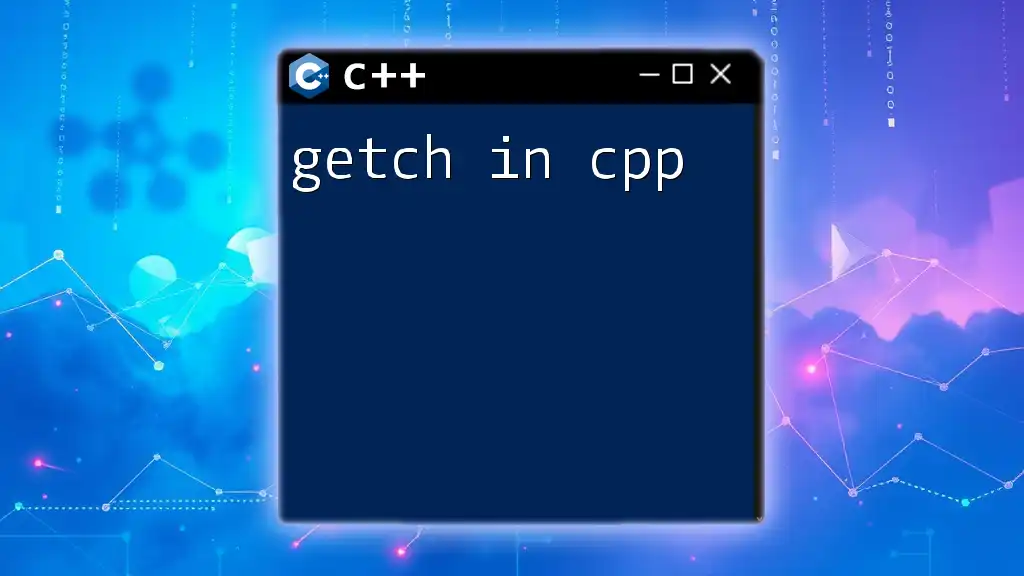
Frequently Asked Questions (FAQ)
What is the difference between strcmp and strcasecmp?
The primary difference between `strcmp` and `strcasecmp` is that `strcasecmp` performs a case-insensitive comparison of strings, while `strcmp` is case-sensitive.
Can strcmp be used with std::string?
`strcmp` is designed for C-style strings (null-terminated character arrays). Using `strcmp` directly with `std::string` can lead to issues since `std::string` does not provide null termination. Instead, use the `==` operator for comparisons.
What happens if I compare uninitialized strings with strcmp?
Comparing uninitialized strings with `strcmp` leads to undefined behavior, which can cause crashes or garbage output. Always initialize your strings before comparing them with `strcmp`.