The `strdup` function in C++ is used to create a duplicate of a string by allocating sufficient memory for it and copying the content from the source string.
#include <cstring>
#include <iostream>
int main() {
const char* original = "Hello, World!";
char* duplicate = strdup(original);
std::cout << "Duplicate String: " << duplicate << std::endl;
free(duplicate); // Always remember to free the allocated memory
return 0;
}
What is `strdup`?
The `strdup` function is a utility in C that allows for the duplication of C-style strings. Defined in `<cstring>`, it allocates sufficient memory to store a copy of an input string and then copies that string into the newly allocated memory. This function is particularly useful when you need a separate instance of a string that can be modified without affecting the original.
In C++, while there are better alternatives available for string manipulation, understanding `strdup` is valuable for memory management and for dealing with C-style strings. Moreover, it highlights the difference between automatic and manual memory management in C and C++.
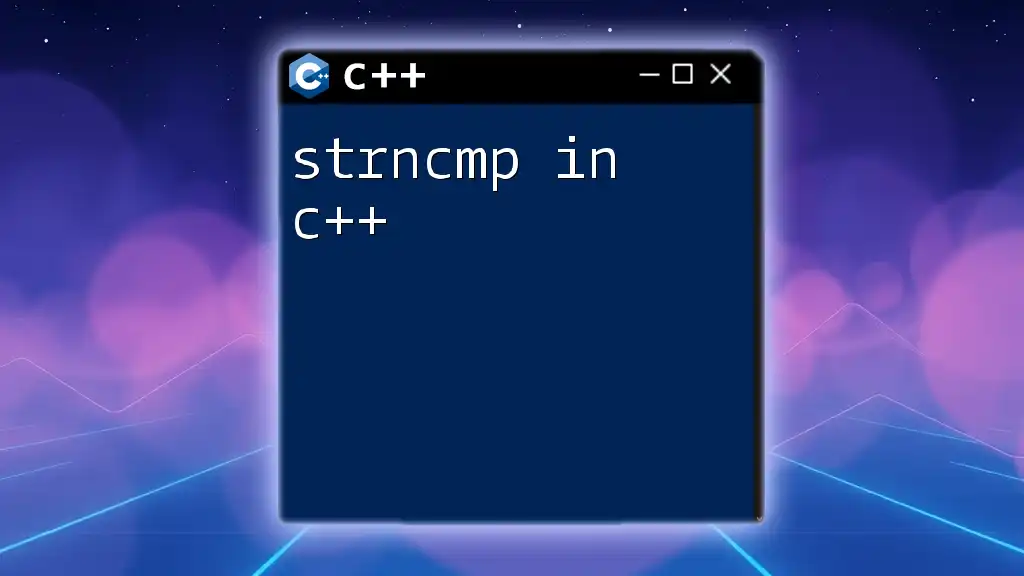
Syntax of `strdup`
The syntax for using `strdup` is straightforward:
char* strdup(const char* str);
Parameters and Return Values
- Parameter: A pointer to a constant character string (`const char* str`) which is the string to be duplicated.
- Return: A pointer to the duplicated string. If the input string is `NULL`, the function returns `NULL`. If memory allocation fails, a `NULL` pointer is also returned.
This return value necessitates proper error checking to avoid dereferencing a null pointer later in your code.
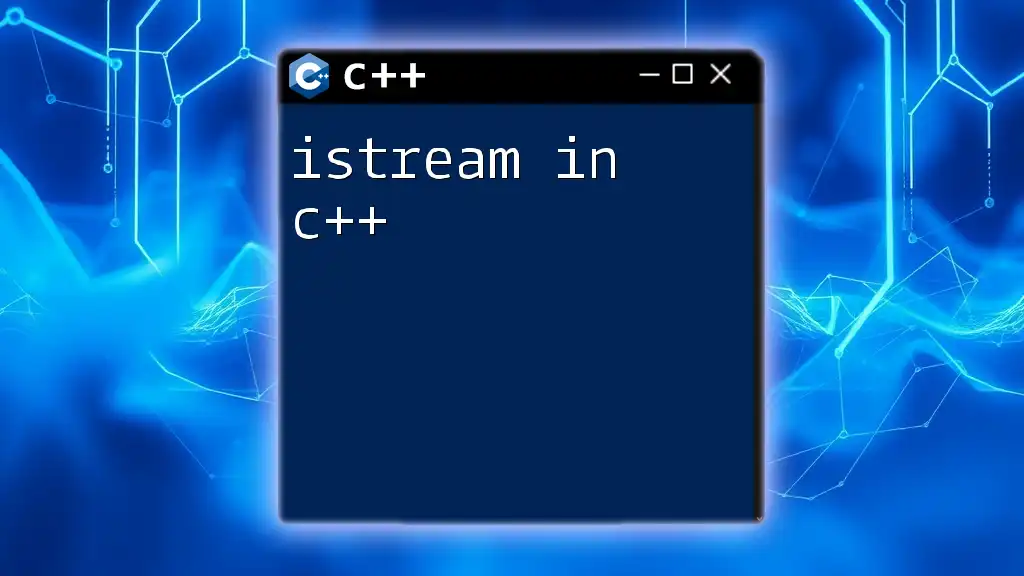
How `strdup` Works
When you call `strdup`, the function does the following:
- Memory Allocation: It allocates enough memory to hold the new string, including the null terminator.
- String Copying: The input string is copied into the newly allocated space, character by character.
- Return Pointer: A pointer to the beginning of this new string is returned.
It’s important to note that this duplicates the string in a new location in memory, preventing unintentional modification to the original string when the copied string is edited.
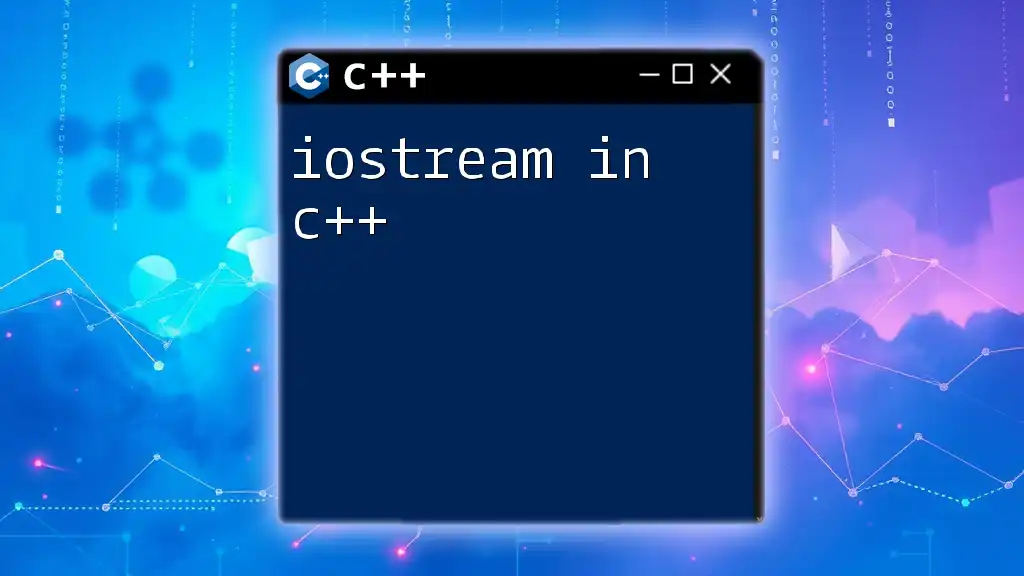
Practical Use Cases
Using `strdup` with C-style Strings
Here’s a simple implementation of `strdup` in a C++ program:
#include <iostream>
#include <cstring>
int main() {
const char* original = "Hello, World!";
char* copy = strdup(original);
if (copy) {
std::cout << "Original: " << original << std::endl;
std::cout << "Copy: " << copy << std::endl;
free(copy); // Important to free the allocated memory
} else {
std::cerr << "Memory allocation failed!" << std::endl;
}
return 0;
}
In this example, we duplicate the string "Hello, World!" and print both the original and the copy. After using the duplicated string, it’s crucial to release the allocated memory using `free()` to prevent memory leaks. Neglecting to do so can lead to significant memory issues in larger applications.
Common Mistakes When Using `strdup`
One common mistake developers make is forgetting to free the memory allocated by `strdup`, leading to a memory leak. Always ensure that you free the memory once it is no longer needed.
Additionally, handle inputs carefully. If you pass a `NULL` string to `strdup`, you must check for this condition because dereferencing a `NULL` pointer afterward will cause undefined behavior.
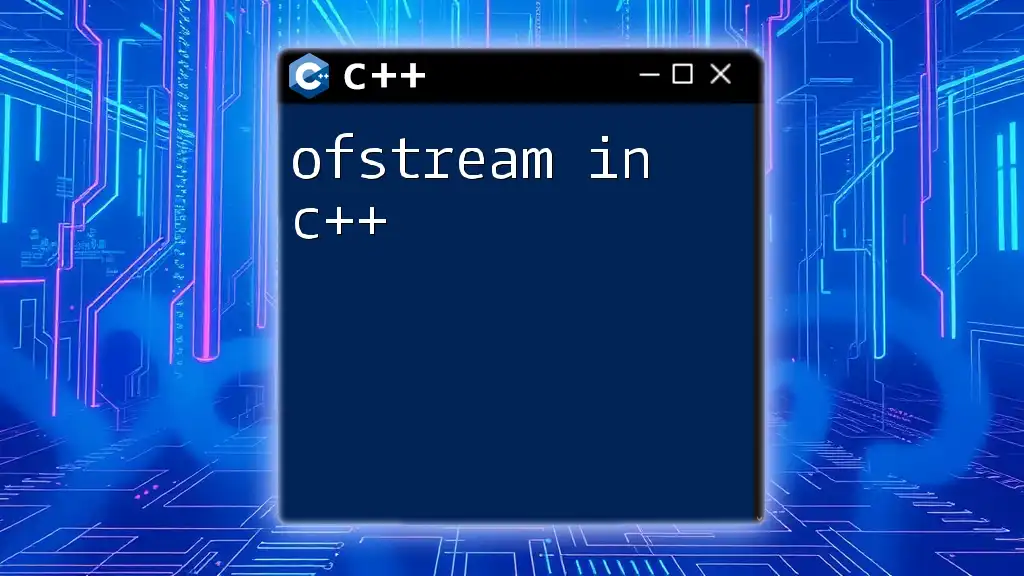
Alternatives to `strdup` in C++
Using `std::string`
While `strdup` is beneficial for C-style strings, C++ offers the more robust `std::string` class, which handles memory automatically. Here’s how you can accomplish the same result with `std::string`:
#include <iostream>
#include <string>
int main() {
std::string original = "Hello, World!";
std::string copy = original; // Automatic handling of memory
std::cout << "Original: " << original << std::endl;
std::cout << "Copy: " << copy << std::endl;
// No need to free memory as std::string handles it automatically
return 0;
}
Using `std::string` simplifies string manipulation significantly. You don't need to worry about manually allocating or deallocating memory.
Other String Functions
Other C-style functions such as `strcpy`, `strlen`, and `strcat` are often used in conjunction with `strdup`. However, these too require careful memory management and handling `NULL` pointers. In contrast, `std::string` provides a safer and more manageable option for string operations in C++.
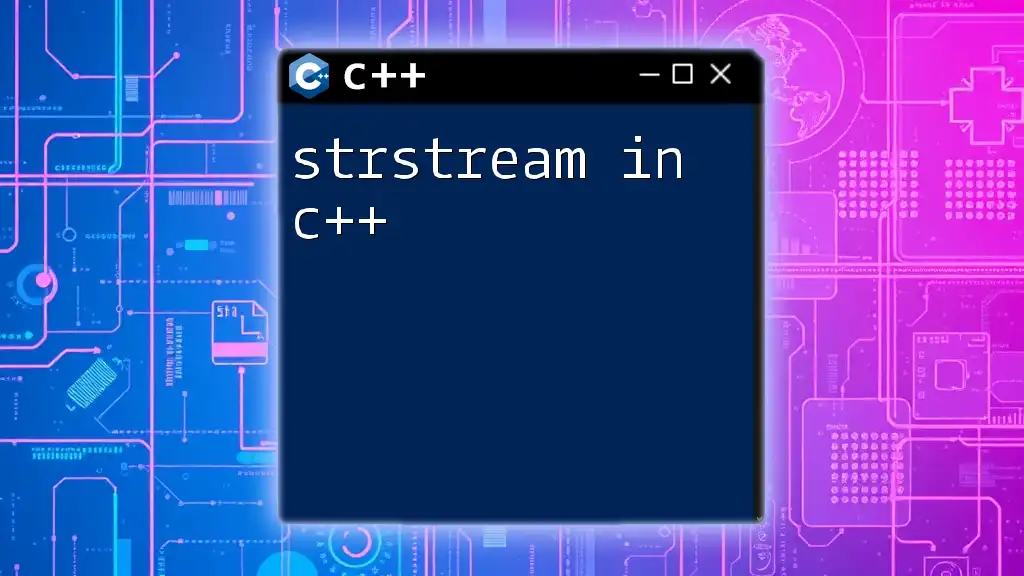
Performance Considerations
When comparing `strdup` with other methods, consider both performance and memory implications. `strdup` performs memory allocation and copying, which can be inefficient in high-performance applications where frequent allocations and deallocations may lead to fragmentation.
Generally, prefer `std::string` in modern C++ programming, as it manages memory automatically, reduces scope for errors, and offers various utility functions for string manipulation.
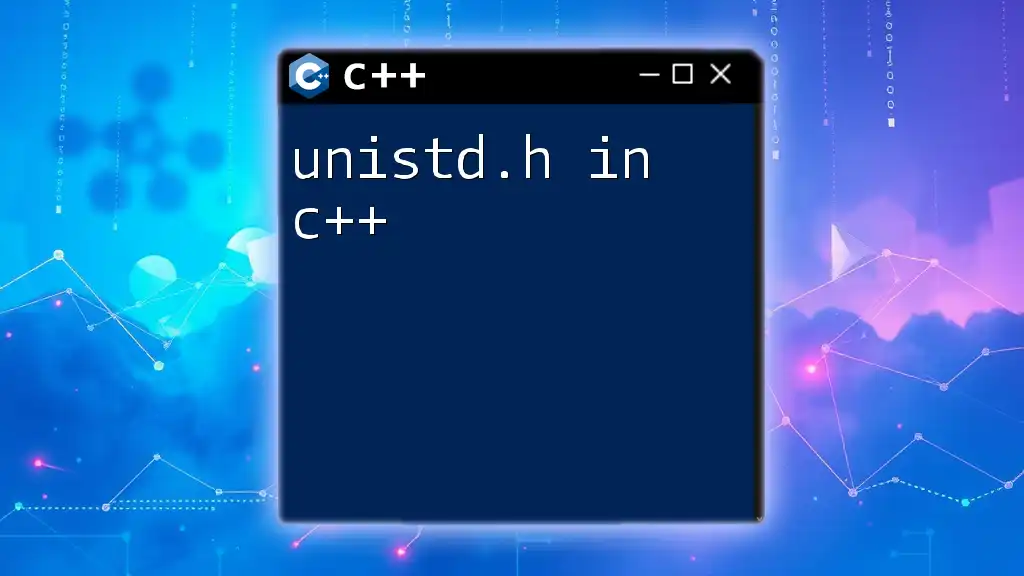
Conclusion
In conclusion, while `strdup in C++` provides a way to duplicate C-style strings, it is crucial to understand its memory allocation responsibilities. Always remember to free any memory allocated with `strdup` to prevent leaks. However, as C++ evolves, using `std::string` is recommended for modern applications due to its simplicity and safety features. By understanding both methods, developers can make informed decisions when it comes to string manipulation in their C++ projects.
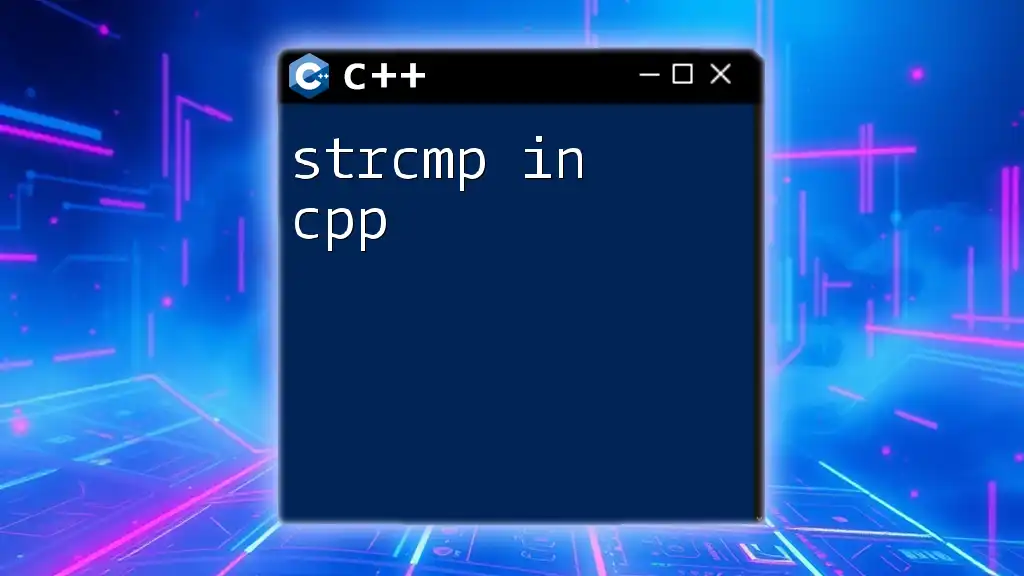
Frequently Asked Questions (FAQ)
Is `strdup` part of the C++ standard?
`strdup` is not part of the standard C++ library, but it is found in the C standard library, and most C++ compilers support it as part of compatibility with C.
When should I use `strdup`?
Use `strdup` when working directly with C-style strings, particularly in legacy systems or when interfacing with C libraries. For most new applications, consider using `std::string` to avoid direct memory management issues.
Can I safely use `strdup` in multithreaded programs?
`strdup` is not inherently thread-safe because it allocates memory; however, you can safely use it in multiple threads as long as each call to `strdup` operates independently and you manage the allocated memory correctly. Just ensure that each thread is responsible for its own memory management and freeing its allocated strings.