The `unistd.h` header in C++ provides access to the POSIX operating system API, allowing for functions that deal with file operations, process control, and various other system calls.
#include <iostream>
#include <unistd.h>
int main() {
std::cout << "Sleeping for 2 seconds..." << std::endl;
sleep(2); // Pauses the program for 2 seconds
std::cout << "Awake now!" << std::endl;
return 0;
}
What is unistd.h?
`unistd.h` is a header file that provides access to the POSIX (Portable Operating System Interface) operating system API. This interface is essential for developing applications in UNIX-like systems and is an integral part of C++ when working in such environments. By including `unistd.h`, you gain access to a variety of functions that facilitate system calls for file operations, process control, and more. Understanding how to leverage these functions can enhance your programming skills significantly in C++.
Key Features of unistd.h
- Cross-Platform Compatibility: Programs written using `unistd.h` can easily be ported across UNIX-like operating systems, thereby enhancing code reusability.
- System-Level Operations: It provides low-level functionalities that allow developers to interact more closely with the operating system.
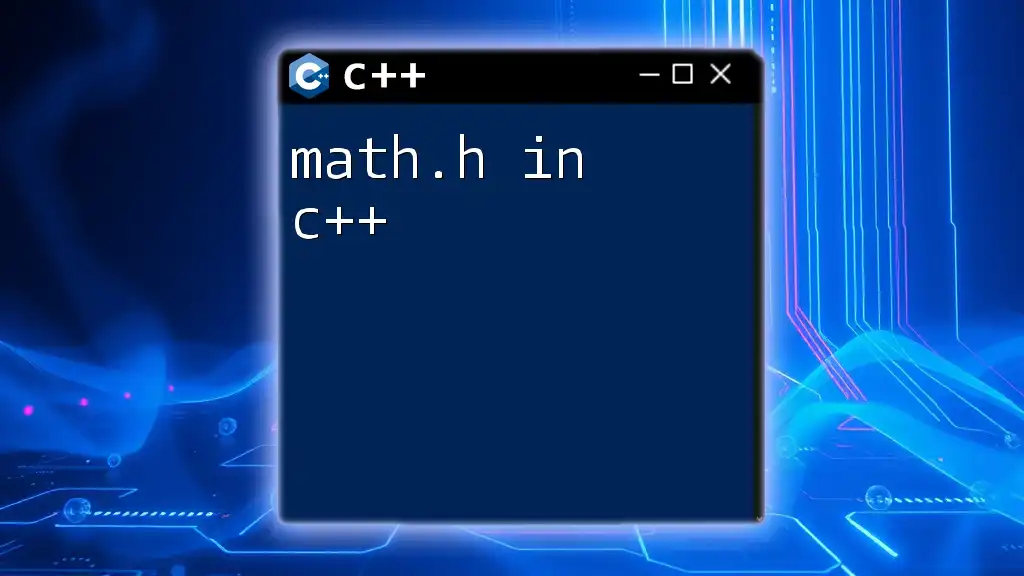
Basic Functions in unistd.h
File Operations
read
The `read` function allows you to read data from a file descriptor, enabling you to obtain information stored in files or devices.
Syntax:
ssize_t read(int fd, void *buf, size_t count);
Example:
#include <unistd.h>
#include <fcntl.h>
#include <iostream>
int main() {
int fd = open("example.txt", O_RDONLY);
char buffer[100];
ssize_t bytesRead = read(fd, buffer, sizeof(buffer));
std::cout << "Bytes read: " << bytesRead << " - Content: " << buffer << std::endl;
close(fd);
return 0;
}
Explanation: In this example, we first open a file named `example.txt` in read-only mode. We then use the `read` function to read a specified number of bytes into a buffer. The function returns the number of bytes actually read, which can be less than the requested count. Finally, we print the content of the buffer and close the file descriptor.
write
The `write` function is employed to write data to a file descriptor. This is critical for storing output or logging information.
Syntax:
ssize_t write(int fd, const void *buf, size_t count);
Example:
#include <unistd.h>
#include <fcntl.h>
#include <iostream>
int main() {
int fd = open("output.txt", O_WRONLY | O_CREAT, S_IRUSR | S_IWUSR);
const char *text = "Hello, World!";
write(fd, text, sizeof(text));
close(fd);
return 0;
}
Explanation: Here, we open (or create) a file named `output.txt` for writing. The `write` function takes care of sending the content from the buffer to the specified file. In this case, we are writing "Hello, World!" into the file. We also specify file permissions, ensuring that the user has read and write access. Finally, we close the file descriptor.
Process Control
fork
The `fork` function is utilized to create a new process by duplicating the calling process. This is a fundamental aspect of process management in UNIX.
Syntax:
pid_t fork();
Example:
#include <unistd.h>
#include <iostream>
int main() {
pid_t pid = fork();
if (pid < 0) {
std::cerr << "Fork failed" << std::endl;
return 1;
} else if (pid == 0) {
std::cout << "I'm the child process!" << std::endl;
} else {
std::cout << "I'm the parent process with child pid: " << pid << std::endl;
}
return 0;
}
Explanation: In this snippet, the `fork` function splits the current process into two: the parent process and the child process. Each one gets a unique process ID (PID). Here, we check if the fork operation failed (indicated by a return value less than zero), and if successful, we differentiate between the child and parent processes, outputting corresponding messages.
getpid
The `getpid` function retrieves the process ID of the calling process, which can be useful in various scenarios such as debugging and logging.
Syntax:
pid_t getpid();
Example:
#include <unistd.h>
#include <iostream>
int main() {
std::cout << "Current Process ID: " << getpid() << std::endl;
return 0;
}
Explanation: This simple example demonstrates how to use `getpid` to print the current process ID to the console. Such information can be vital when monitoring and managing processes in a system.
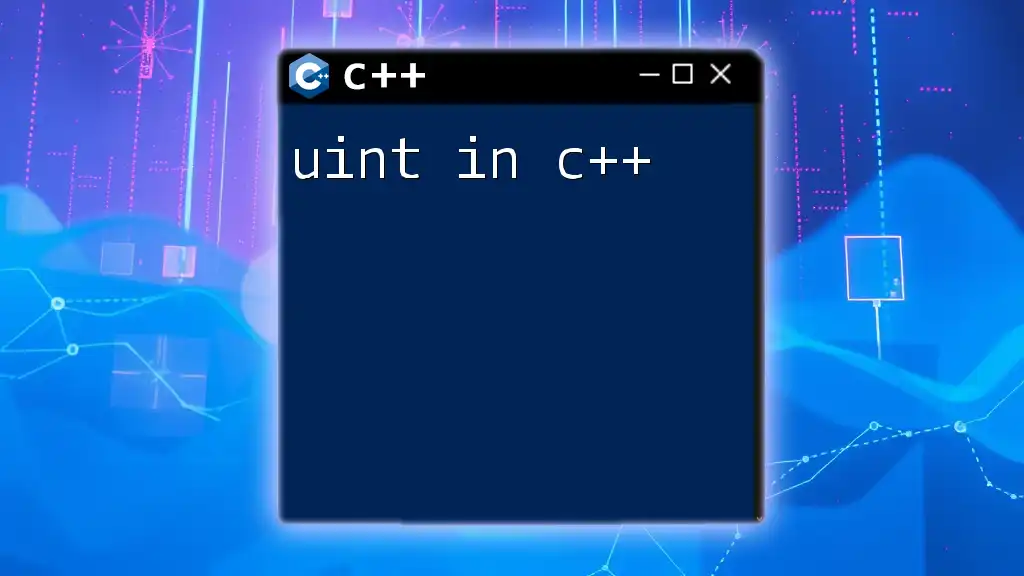
Working with Environment Variables
getenv
The `getenv` function allows you to access environment variables, which can be crucial for configuring application settings based on the environment it runs in.
Syntax:
char *getenv(const char *name);
Example:
#include <unistd.h>
#include <iostream>
int main() {
char *path = getenv("PATH");
std::cout << "System PATH: " << path << std::endl;
return 0;
}
Explanation: In this code snippet, we use `getenv` to extract the value of the `PATH` environment variable, which tells the system where to look for executables. Printing this information can help you understand how the system is configured and can assist in debugging path-related issues.
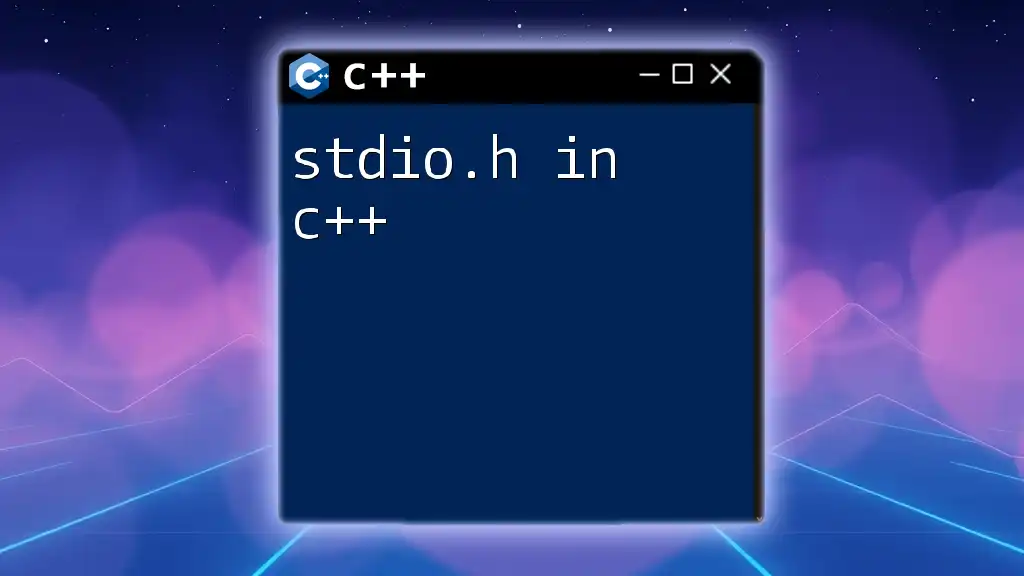
Error Handling with unistd.h Functions
Error handling is a critical part of any programming language, especially when making system calls that might fail.
When using functions from `unistd.h`, it's essential to check the return values for errors. For instance, if a file cannot be opened or read, the related function may return `-1`. Always inspect the `errno` variable to understand the type of error encountered.
Example of Error Handling
#include <unistd.h>
#include <fcntl.h>
#include <iostream>
#include <cerrno>
#include <cstring>
int main() {
int fd = open("nonexistent.txt", O_RDONLY);
if (fd == -1) {
std::cerr << "Error opening file: " << strerror(errno) << std::endl;
return 1;
}
close(fd);
return 0;
}
Explanation: In this example, we attempt to open a file that does not exist. Since `open` returns `-1`, we capture the error and print a descriptive message using `strerror`, which translates the error code stored in `errno` to a human-readable string. This kind of error handling is vital for robust application development.
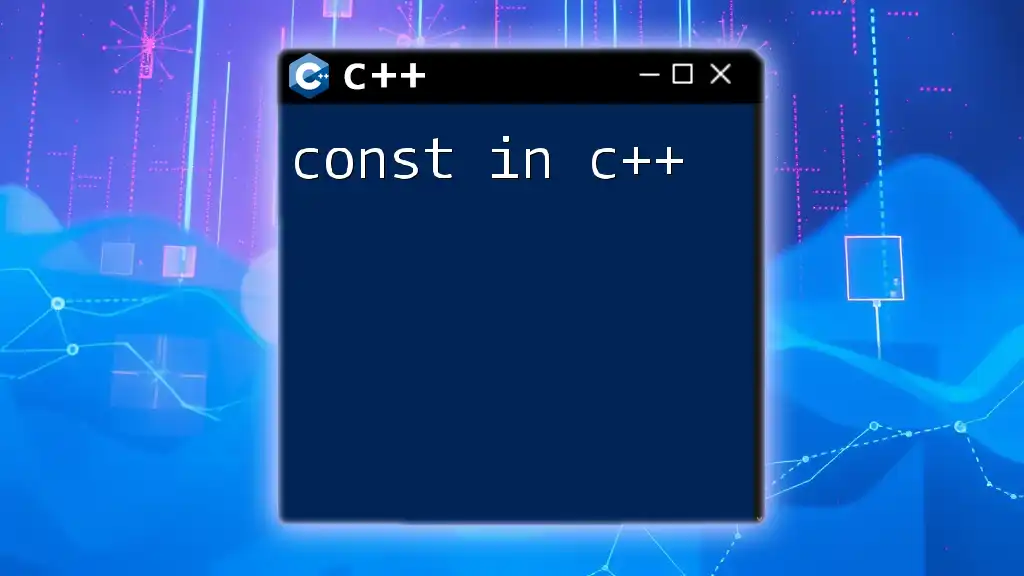
Conclusion
Understanding the capabilities of `unistd.h` in C++ opens up endless possibilities for developing effective and portable applications. From basic file operations to intricate process management and environment manipulation, mastering these functions can significantly enhance your programming repertoire.
The examples provided illustrate how to work with some of the most commonly used functions in the header file. Remember to experiment with these functions on your own and explore further to uncover the full potential of `unistd.h`. With practice, you’ll become proficient at utilizing these tools for developing robust C++ applications in a UNIX environment.
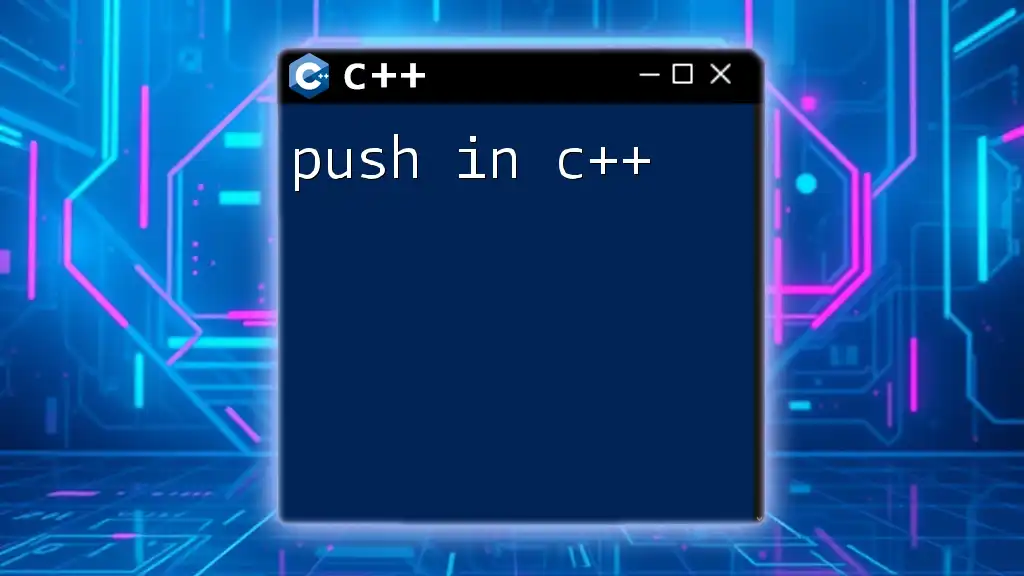
Additional Resources
For further learning, you may consider consulting official documentation, participating in coding forums, or engaging with online communities focused on C++ programming in UNIX systems. These resources can provide valuable insights and support as you delve deeper into the intricacies of `unistd.h` and beyond.
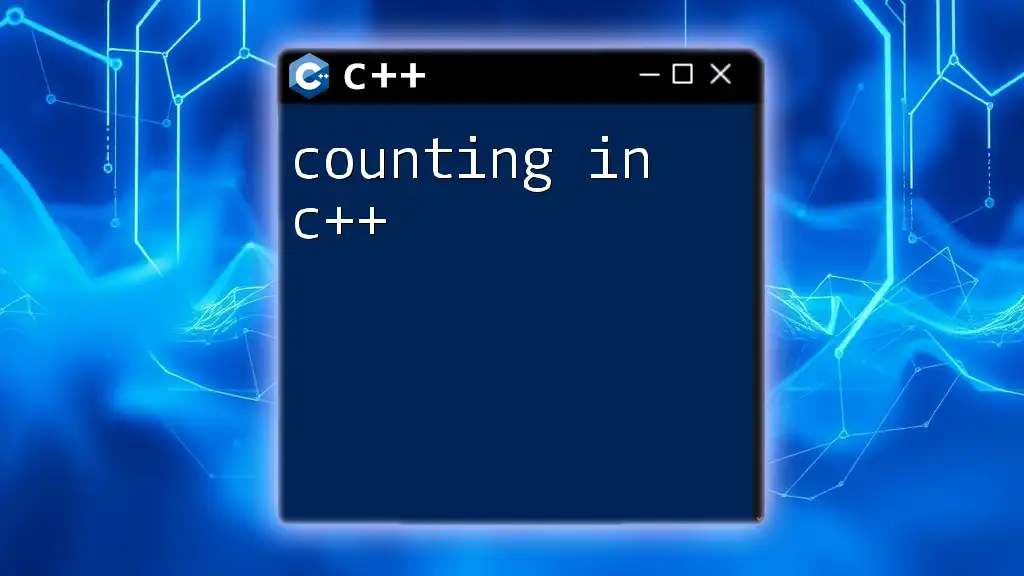
FAQs
What is the difference between `unistd.h` and standard C++ libraries?
`unistd.h` provides low-level access to system calls, while standard C++ libraries are focused on general-purpose programming tasks.
Can `unistd.h` be used in Windows systems?
No, `unistd.h` is specific to UNIX-like systems. For Windows, you would typically use `<windows.h>` for similar functionalities.
What to do if certain functions in `unistd.h` are not available?
If the function is not available, check if your development environment supports POSIX standards; alternatively, consider using equivalent standard C++ solutions or libraries that provide similar functionalities.