The `std::find` function in C++ is used to search for a specific value within a range of elements in a container, returning an iterator to the first occurrence of that value or the end of the range if not found.
Here’s a code snippet demonstrating its use:
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
auto it = std::find(numbers.begin(), numbers.end(), 3);
if (it != numbers.end()) {
std::cout << "Found: " << *it << std::endl;
} else {
std::cout << "Not found." << std::endl;
}
return 0;
}
What is std::find?
`std::find` is a function provided by the C++ Standard Template Library (STL) that is used to locate a specific element within a range defined by two iterators. It traverses the range one element at a time, searching for a match with the specified value. If the value is found, it returns an iterator pointing to the first occurrence of that value; if not, it returns the end iterator.
This utility makes it easier for developers to perform search operations in various STL containers, including vectors, lists, and arrays.
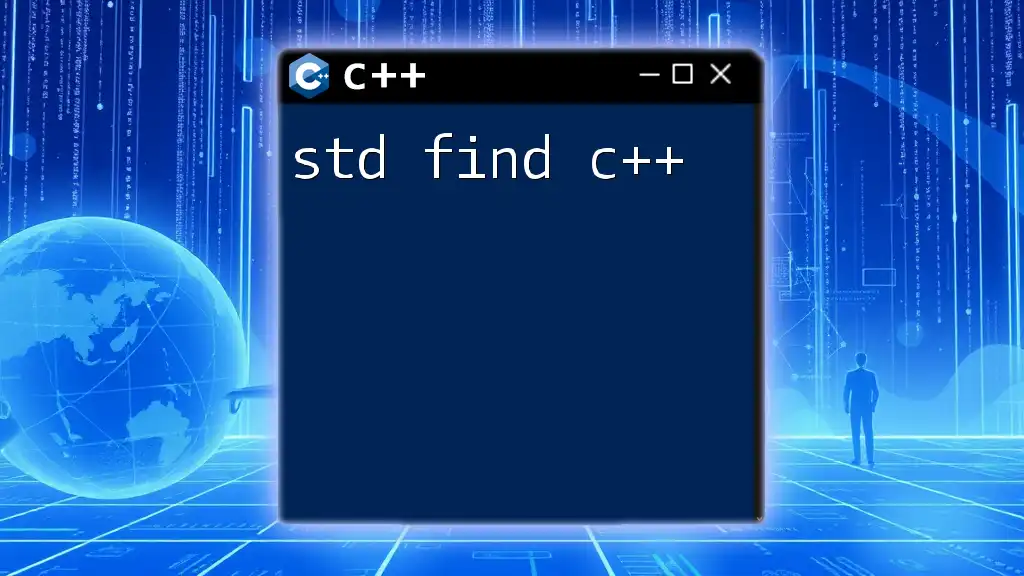
Syntax and Parameters
The Syntax of std::find
The syntax for `std::find` is as follows:
template<class ForwardIt, class T>
ForwardIt find(ForwardIt first, ForwardIt last, const T& value);
Parameters Explained
- `ForwardIt first`: A forward iterator pointing to the beginning of the range to be searched.
- `ForwardIt last`: A forward iterator pointing to the end of the range (the search will exclude this element).
- `const T& value`: The value you wish to find within the specified range.
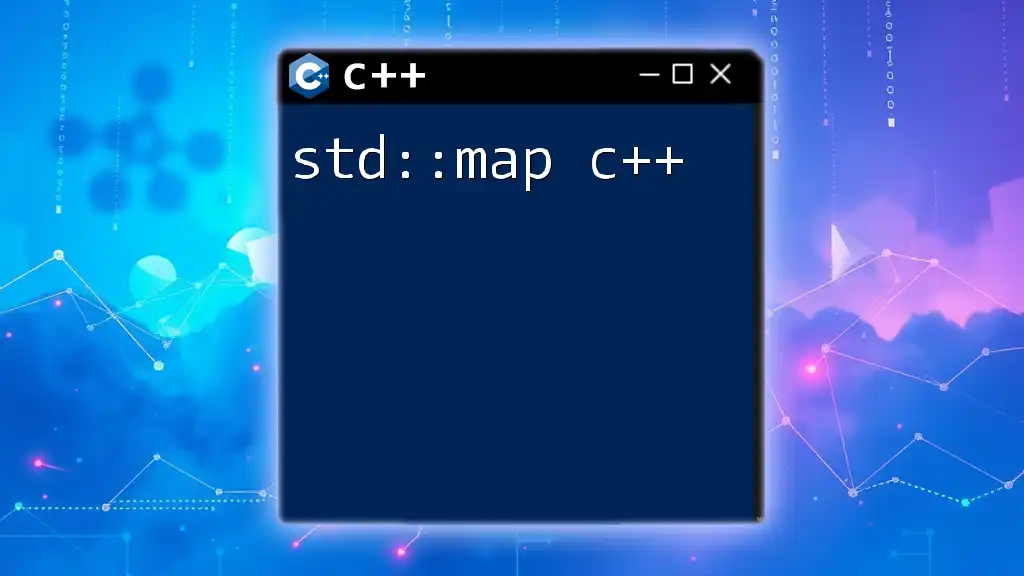
How std::find Works
Iterative Search Mechanism
`std::find` uses an iterative approach to search through the range of elements. It checks each element starting from the `first` iterator and compares it to the specified `value`. If it encounters a match, it immediately returns an iterator pointing to that element. If the end of the range is reached without finding the value, it returns the `last` iterator.
Return value
The return value of `std::find` is crucial for utilizing the function effectively:
- If the specified value is found, the function returns an iterator pointing to that value.
- If the specified value is not found, it returns the same iterator as `last`.
Here is a simple example:
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
std::vector<int> vec = {1, 2, 3, 4, 5};
int target = 3;
auto it = std::find(vec.begin(), vec.end(), target);
if (it != vec.end()) {
std::cout << "Element found: " << *it << "\n";
} else {
std::cout << "Element not found\n";
}
return 0;
}
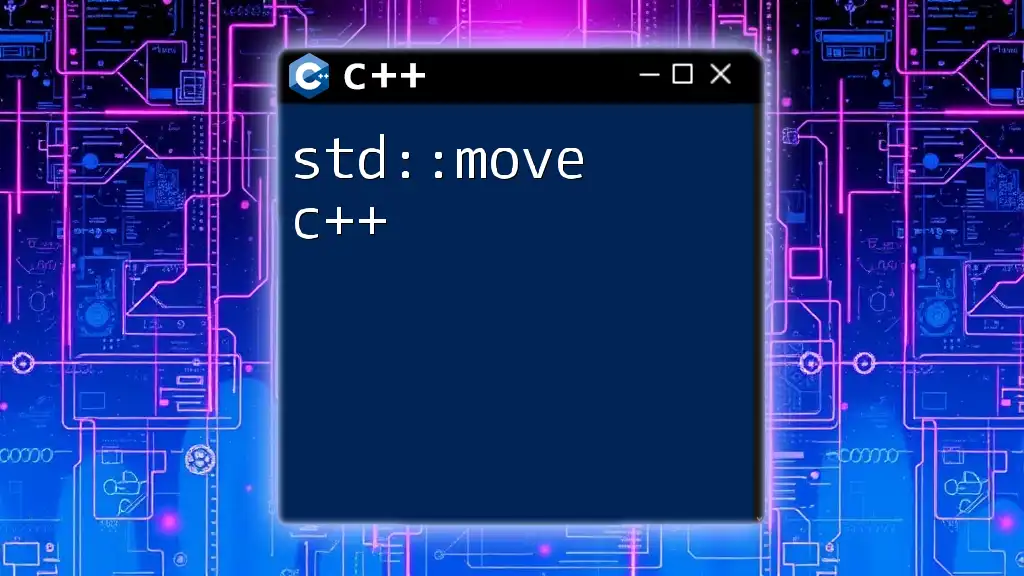
Example Use Cases
Searching in Arrays
`std::find` works seamlessly with C++ arrays as well. Here’s a basic example:
#include <iostream>
#include <algorithm>
int main() {
int arr[] = {10, 20, 30, 40, 50};
int target = 30;
auto it = std::find(std::begin(arr), std::end(arr), target);
if (it != std::end(arr)) {
std::cout << "Element found: " << *it << "\n";
} else {
std::cout << "Element not found\n";
}
return 0;
}
In this example, `std::begin` and `std::end` are used to get the iterators that represent the range of the array.
Searching in Lists
`std::find` is not limited to arrays and vectors; it can also be applied to C++ lists, where it can be beneficial. Consider this example:
#include <iostream>
#include <list>
#include <algorithm>
int main() {
std::list<std::string> names = {"Alice", "Bob", "Charlie"};
std::string target = "Charlie";
auto it = std::find(names.begin(), names.end(), target);
if (it != names.end()) {
std::cout << "Element found: " << *it << "\n";
} else {
std::cout << "Element not found\n";
}
return 0;
}
This illustrates how to use `std::find` effectively with type-safe, ordered collections like lists.
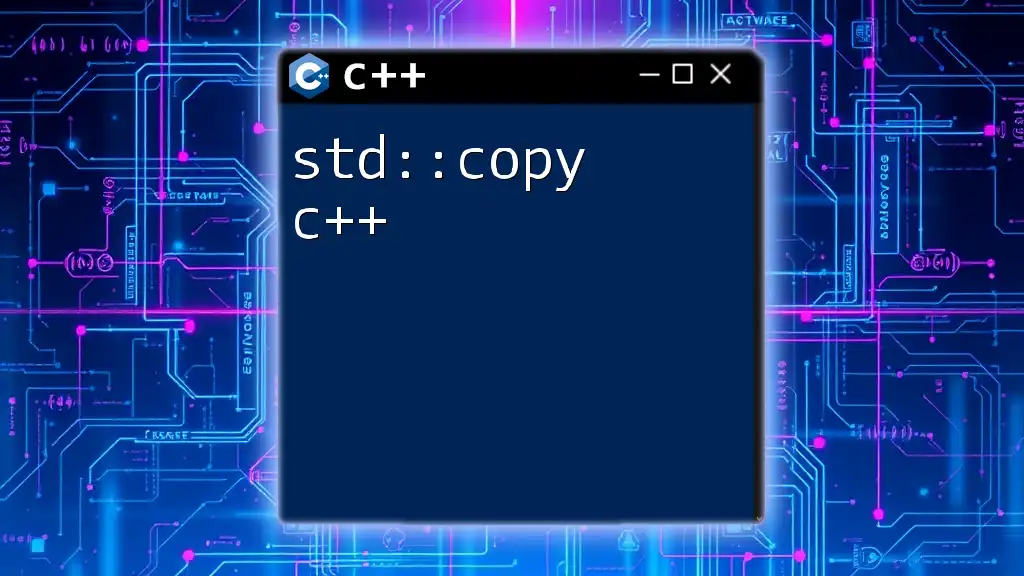
Practical Tips
Best Practices for Using std::find
To leverage `std::find` efficiently, keep these best practices in mind:
- Use `std::find` when you need to search a simple element in a container with a linear search.
- Ensure you are aware of the container’s iterator type, as misusing iterators can lead to runtime errors.
- Consider using more specialized algorithms for sorted data (like `std::binary_search`) if performance is a priority.
Common Mistakes to Avoid
-
Forgetting to include the necessary header: Always include the header `<algorithm>` at the top of your CPP file when using `std::find`.
#include <algorithm> // Necessary for std::find
-
Confusing `std::find` with `std::find_if`: `std::find_if` allows you to use a predicate to determine if an element satisfies certain conditions, offering more flexibility.
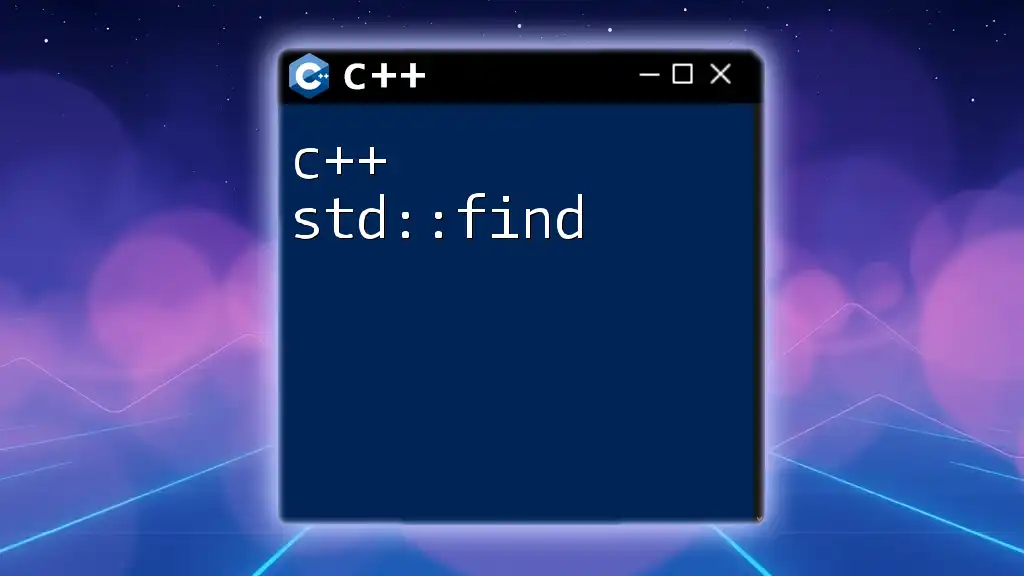
Alternatives to std::find
std::find_if and std::find_if_not
If you need to search based on conditions, consider using `std::find_if`. This function takes an additional predicate that dictates the search criteria:
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
std::vector<int> vec = {1, 2, 3, 4, 5};
auto it = std::find_if(vec.begin(), vec.end(), [](int x) { return x > 3; });
if (it != vec.end()) {
std::cout << "Element found: " << *it << "\n";
} else {
std::cout << "Element not found\n";
}
return 0;
}
Using a lambda function allows you to customize your search logic.
std::find_if_not comparison
Similarly, `std::find_if_not` helps in finding the first element that does not satisfy a specific condition. This offers even greater control over your search operations.
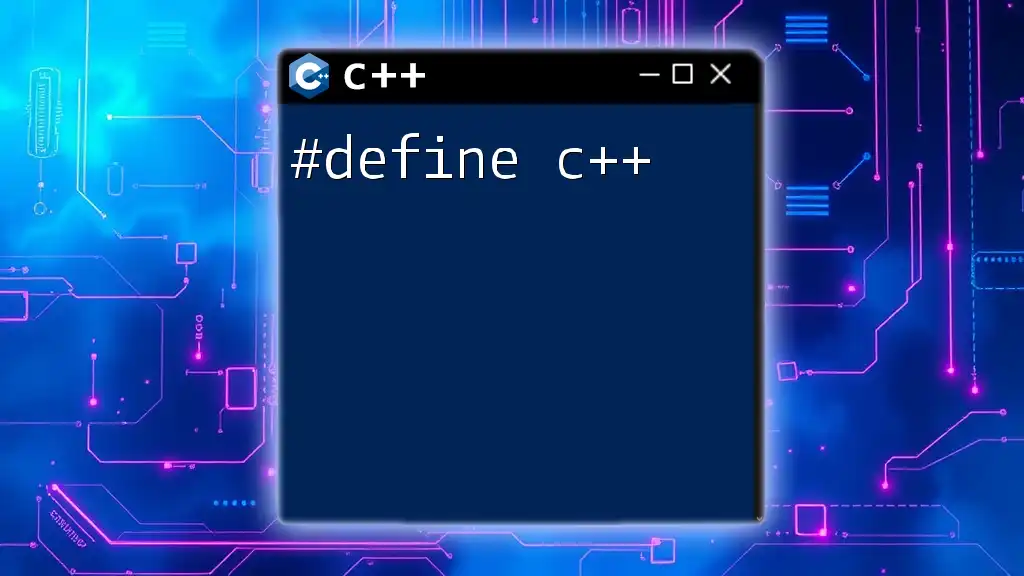
Conclusion
In summary, `std::find` is an essential standard algorithm that offers a straightforward way to search through elements in various container types in C++. By understanding the syntax, return values, and practical uses, developers can efficiently utilize this algorithm in their projects. Encouragement to practice using `std::find` will deepen your understanding of both C++ algorithms and STL containers.
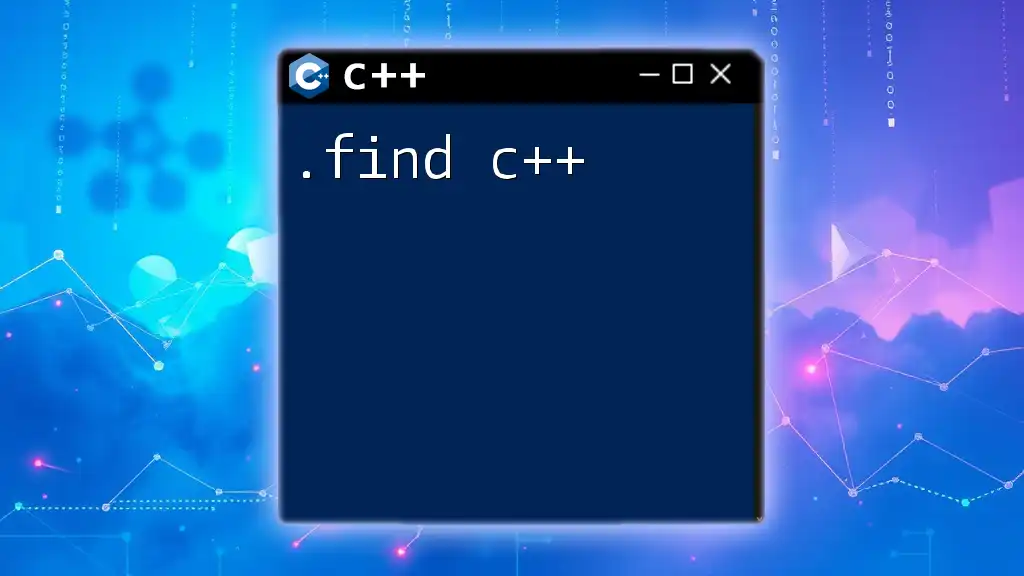
Additional Resources
For further learning and mastery of `std::find c++`, explore the official C++ documentation and consider recommended books and online courses that dive deeper into the C++ Standard Library and beyond.
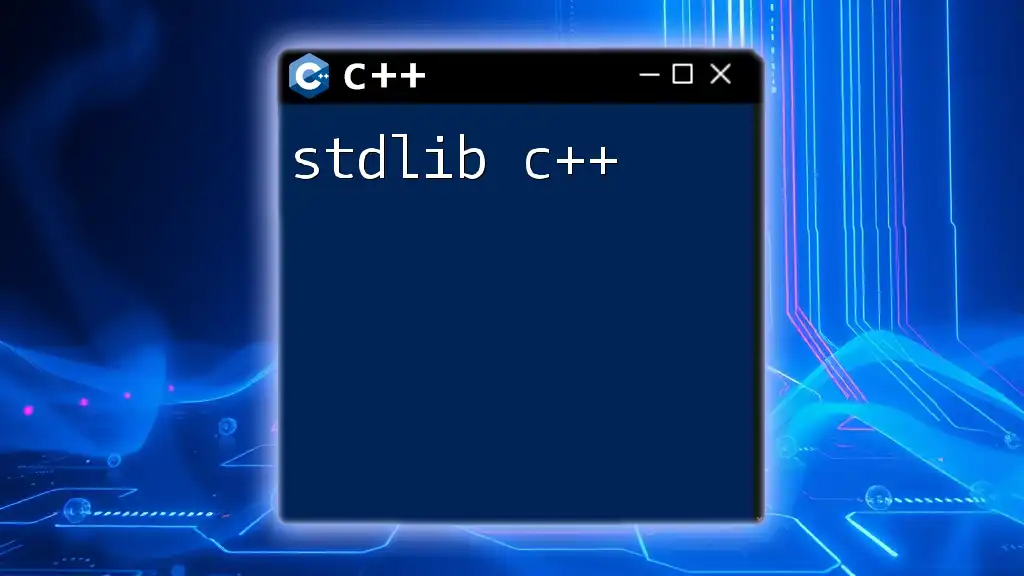
FAQs
- What is the complexity of std::find?
- `std::find` has a time complexity of O(n) because it may need to examine each element in the specified range.
- Can I use std::find with custom objects?
- Yes! Just make sure that your custom objects support comparison through the == operator for `std::find` to work.