The Standard Library (stdlib) in C++ provides a rich set of functions and classes that facilitate common programming tasks such as input/output, data manipulation, and memory management.
Here’s a simple code snippet demonstrating the use of the `iostream` and `vector` components from the C++ Standard Library:
#include <iostream>
#include <vector>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
for (int num : numbers) {
std::cout << num << " ";
}
return 0;
}
Overview of C++ Standard Library Components
Containers
Containers are fundamental building blocks in C++ that allow developers to store and manage collections of data. The C++ standard library (stdlib) offers a variety of container types, each tailored for specific use cases.
Types of Containers
Sequence Containers store elements in a linear arrangement and allow access through indices or iterators.
-
Vector: A dynamic array that can grow or shrink in size. Vector offers fast random access and is generally more efficient than a list for indexed access.
#include <iostream> #include <vector> int main() { std::vector<int> numbers = {1, 2, 3}; numbers.push_back(4); // Add element for (int num : numbers) { std::cout << num << " "; // Output: 1 2 3 4 } return 0; }
The above code demonstrates how to create a vector, add an element, and iterate over its contents.
-
List: A doubly linked list that allows efficient insertions and deletions from any position in the list. However, it lacks the random access capability of vectors.
#include <iostream> #include <list> int main() { std::list<int> myList = {1, 2, 3}; myList.push_back(4); // Add element at the end for (int num : myList) { std::cout << num << " "; // Output: 1 2 3 4 } return 0; }
-
Deque: A double-ended queue that supports fast insertions and deletions at both ends. It's versatile, accommodating various access patterns.
Associative Containers
These containers facilitate organized storage and retrieval of data based on keys.
-
Set: A collection that stores unique elements following a specific order (usually ascending).
#include <iostream> #include <set> int main() { std::set<int> mySet = {3, 1, 2}; mySet.insert(4); for (int num : mySet) { std::cout << num << " "; // Output: 1 2 3 4 } return 0; }
-
Map: A collection of key-value pairs, where each key is unique. This is particularly useful for associating data.
#include <iostream> #include <map> int main() { std::map<std::string, int> myMap; myMap["one"] = 1; myMap["two"] = 2; std::cout << myMap["one"]; // Output: 1 return 0; }
Unordered Containers
These containers do not maintain any specific order, prioritizing faster access.
-
Unordered Set allows for unique elements but with no specific order.
#include <iostream> #include <unordered_set> int main() { std::unordered_set<int> mySet = {2, 1, 3}; mySet.insert(4); for (int num : mySet) { std::cout << num << " "; // Output can vary in order } return 0; }
-
Unordered Map is similar to a map but, like the unordered set, does not maintain any order.
#include <iostream> #include <unordered_map> int main() { std::unordered_map<std::string, int> myMap; myMap["apple"] = 1; myMap["banana"] = 2; std::cout << myMap["banana"]; // Output: 2 return 0; }
Algorithms
The C++ standard library also comprises a vast array of algorithms that facilitate operations on containers, such as searching, sorting, and modifying data.
Common Algorithms
-
Sorting: The `std::sort` function enables sorting elements in containers rapidly.
#include <iostream> #include <vector> #include <algorithm> int main() { std::vector<int> numbers = {4, 3, 2, 1}; std::sort(numbers.begin(), numbers.end()); for (int num : numbers) { std::cout << num << " "; // Output: 1 2 3 4 } return 0; }
-
Searching: The `std::binary_search` function is effective for checking if an element exists in a sorted container.
#include <iostream> #include <vector> #include <algorithm> int main() { std::vector<int> numbers = {1, 2, 3, 4}; bool found = std::binary_search(numbers.begin(), numbers.end(), 3); std::cout << found; // Output: 1 (true) return 0; }
-
Modification: Using the `std::transform` function allows for applying a transformation to elements of a container.
#include <iostream> #include <vector> #include <algorithm> int main() { std::vector<int> numbers = {1, 2, 3}; std::transform(numbers.begin(), numbers.end(), numbers.begin(), [](int n) { return n * 2; }); for (int num : numbers) { std::cout << num << " "; // Output: 2 4 6 } return 0; }
Iterators
Iterators are pointers pointing to elements in a container, playing a critical role in traversing and manipulating data.
Types of Iterators
-
Input Iterators: Allow reading data in a single-pass manner.
#include <vector> #include <iostream> #include <iterator> int main() { std::vector<int> vec = {1, 2, 3}; std::vector<int>::iterator it = vec.begin(); std::cout << *it; // Output: 1 return 0; }
-
Output Iterators: Are used for writing data back to a container.
#include <vector> #include <iostream> int main() { std::vector<int> vec; std::back_inserter(vec) = 5; std::cout << vec[0]; // Output: 5 return 0; }
-
Forward Iterators: Allow moving forward through a container and can be used multiple times.
-
Bidirectional Iterators: Can move in both directions throughout a container.
#include <list> #include <iostream> int main() { std::list<int> lst = {1, 2, 3}; std::list<int>::iterator it = lst.begin(); std::cout << *it; // Output: 1 ++it; std::cout << *it; // Output: 2 --it; std::cout << *it; // Output: 1 return 0; }
-
Random Access Iterators: These provide full pointer semantics, allowing jumps to arbitrary locations with ease.
Function Objects and Lambdas
Function objects, or functors, encapsulate behaviors and can be invoked like functions. This feature is pervasive within the C++ standard library.
Lambdas in C++ STL
Lambda expressions provide a concise mechanism for defining anonymous functions directly in the code. This is particularly useful when working with STL algorithms.
Syntax:
[ capture_clause ] ( parameters ) -> return_type { body }
A practical example of using lambda with an STL algorithm is shown below:
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
std::vector<int> vec = {1, 2, 3, 4};
std::for_each(vec.begin(), vec.end(), [](int n) { std::cout << n * 2 << " "; }); // Output: 2 4 6 8
return 0;
}
In this example, the lambda function doubles each element in the vector and prints it.
Utilities
Utility components in the stdlib C++ serve a variety of practical functions beyond containers and algorithms.
Common Utility Components
-
Pair: A container that holds two heterogeneous objects. It finds utility in scenarios such as returning multiple values from functions.
#include <iostream> #include <utility> int main() { std::pair<int, std::string> myPair = {1, "apple"}; std::cout << myPair.first << " " << myPair.second; // Output: 1 apple return 0; }
-
Tuple: Similar to pair but can hold a fixed number of elements of varying types.
#include <iostream> #include <tuple> int main() { std::tuple<int, double, std::string> myTuple = {1, 2.5, "banana"}; std::cout << std::get<2>(myTuple); // Output: banana return 0; }
-
Optional: An innovative way to handle optional values without the use of pointers or nullable types. This can evoke clarity and safety in code.
#include <iostream> #include <optional> int main() { std::optional<int> myOpt = 5; if (myOpt.has_value()) { std::cout << myOpt.value(); // Output: 5 } return 0; }
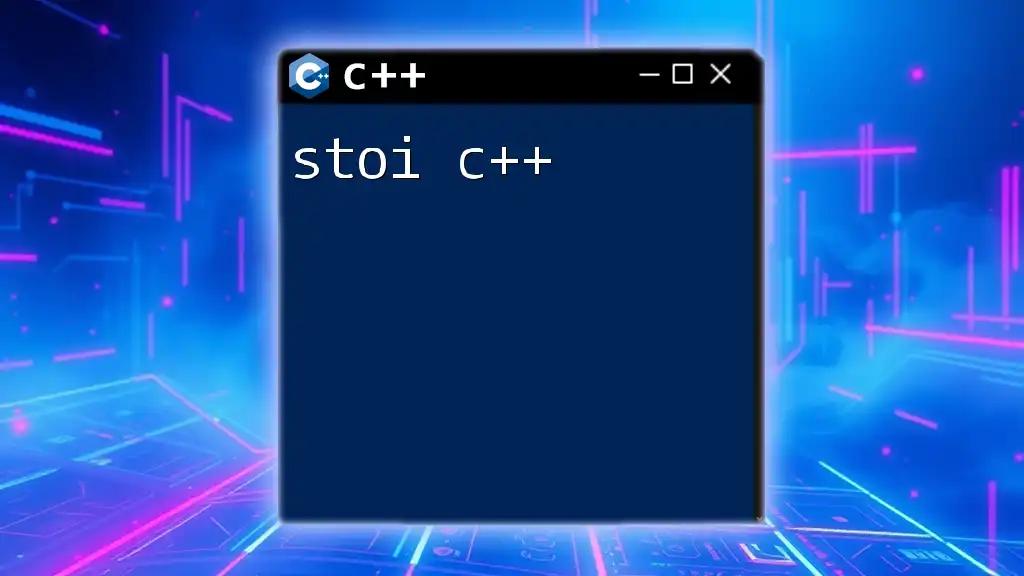
Best Practices when Using C++ Standard Library
Leveraging the stdlib C++ effectively can dramatically enhance your coding experience and program performance. Here are some best practices:
-
Understand the Container's Nature: Choose the right container based on your performance needs—whether it's for fast access, order preservation, or a collection of unique elements.
-
Avoid Unnecessary Copies: When passing objects to functions, prefer using references to avoid costly copies. Use `const` references where appropriate.
-
Utilize `std::move`: Move semantics can optimize memory usage, reducing overhead when creating temporary objects.
-
Favor STL algorithms: Instead of rolling your own algorithm, harness the power of built-in STL algorithms that are tried and tested.
Common pitfalls to avoid
-
Misunderstanding the performance characteristics of different containers can lead to significant inefficiencies. Make sure to read documentation carefully.
-
Forgetting to specify `#include <iostream>` or similar directives leads to compilation errors. Always ensure necessary headers are included.
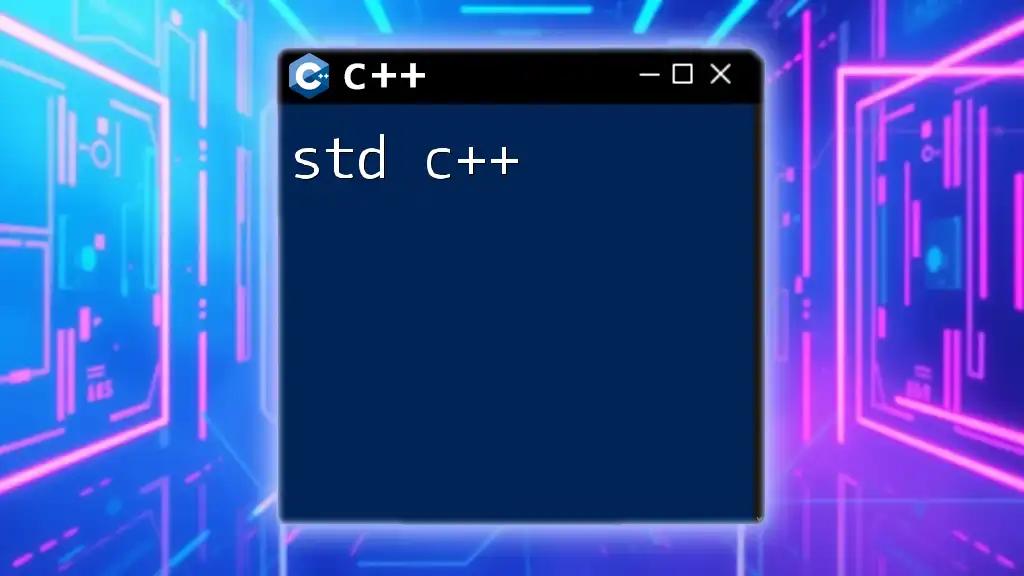
Conclusion
The C++ Standard Library (stdlib) is an indispensable resource for programmers, offering a suite of containers, algorithms, and utilities that streamline programming tasks and enhance code clarity and performance. Mastering these components will greatly empower you in your C++ programming journey. Practice using these features and refer to the comprehensive documentation to further deepen your understanding.
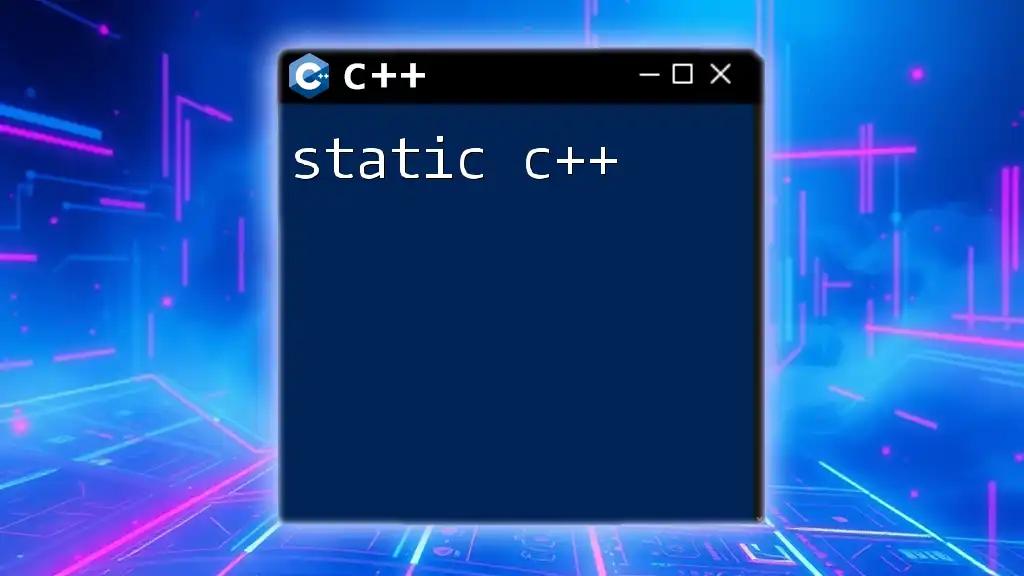
Additional Resources
To continue your learning, consider exploring:
- Recommended books such as "The C++ Programming Language" by Bjarne Stroustrup.
- Online platforms like Codecademy and LeetCode for practical coding exercises.
- Community forums like Stack Overflow for interactive discussions and solutions to your queries.