To install C++ on Ubuntu, simply use the following command in the terminal to install the GNU C++ compiler (g++).
sudo apt update && sudo apt install g++
What You Need Before Installing C++
Before diving into the installation process, it’s essential to ensure that your system meets the necessary requirements for a smooth C++ setup. Ubuntu typically runs well on a variety of hardware, but ensuring the latest version provides the best compatibility and support is crucial. Any version of Ubuntu from 18.04 and onwards is compatible with C++.
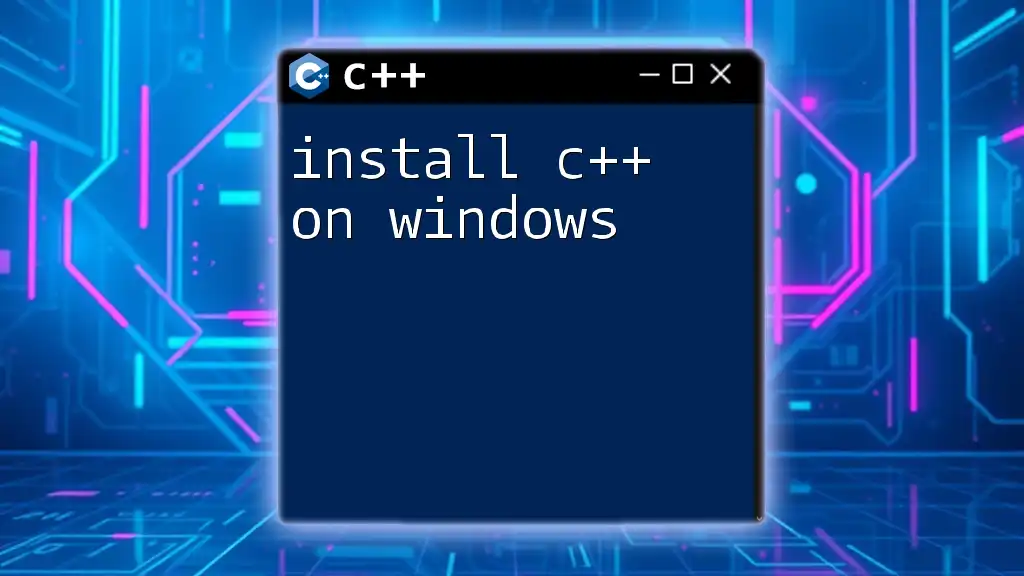
Installing C++ on Ubuntu
Installing the Build-Essential Package
To begin using C++ on Ubuntu, the very first step is to install the build-essential package. This package includes essential tools for compiling software, such as compiler and libraries. It sets up your environment efficiently.
You can install the build-essential package by opening a terminal and running the following commands:
sudo apt update
sudo apt install build-essential
This process downloads and installs the necessary components. Once installed, you can verify its presence and ensure everything is updated. To double-check that the package has been successfully installed, you can run:
dpkg --get-selections | grep build-essential
Installing a Specific C++ Compiler
Installing GCC (GNU Compiler Collection)
GCC is widely regarded as the default and most commonly used C++ compiler on Ubuntu. Installing GCC is straightforward.
Use the following command to install the core components of GCC, specifically the C++ compiler:
sudo apt install g++
After the installation is complete, you can verify that GCC is working correctly by checking the version. Run this command:
g++ --version
You should see the version information displayed, confirming installation success.
Installing Clang
If you prefer an alternative to GCC, Clang is a robust and widely-used C++ compiler that many developers enjoy due to its speed and diagnostic capabilities.
To install Clang, you can execute the following command:
sudo apt install clang
Just like with GCC, you can check the version of Clang after installation to ensure everything is good:
clang --version
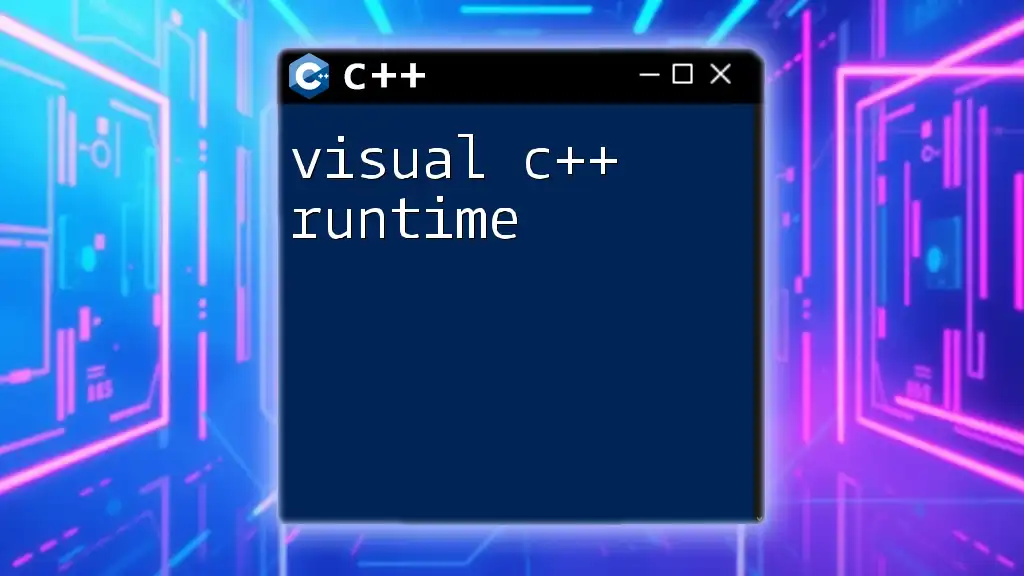
Setting Up Your Development Environment
Choosing a Code Editor
Selecting a code editor suited for your development workflow enhances productivity. Some popular code editors for Ubuntu include Visual Studio Code, Atom, and Sublime Text. Each has its unique features, so you may want to try a couple before settling on one.
For example, to install Visual Studio Code, which is highly regarded for its extensions and debugging capabilities, you can run:
sudo snap install --classic code
This command installs Visual Studio Code via Snap, making the setup seamless.
Writing Your First C++ Program
After you have your compiler and code editor set up, you’re ready to write your very first C++ program! Here’s a simple "Hello, World!" example that introduces you to the basic structure of a C++ program:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
- Open your code editor and create a new file named hello.cpp.
- Copy the code above into your editor and save the file.
- To compile the program, return to your terminal and navigate to the directory where your hello.cpp file is located. Then run:
g++ -o hello hello.cpp
This command compiles your code and produces an executable named hello. To execute the program, type:
./hello
You should see the output:
Hello, World!
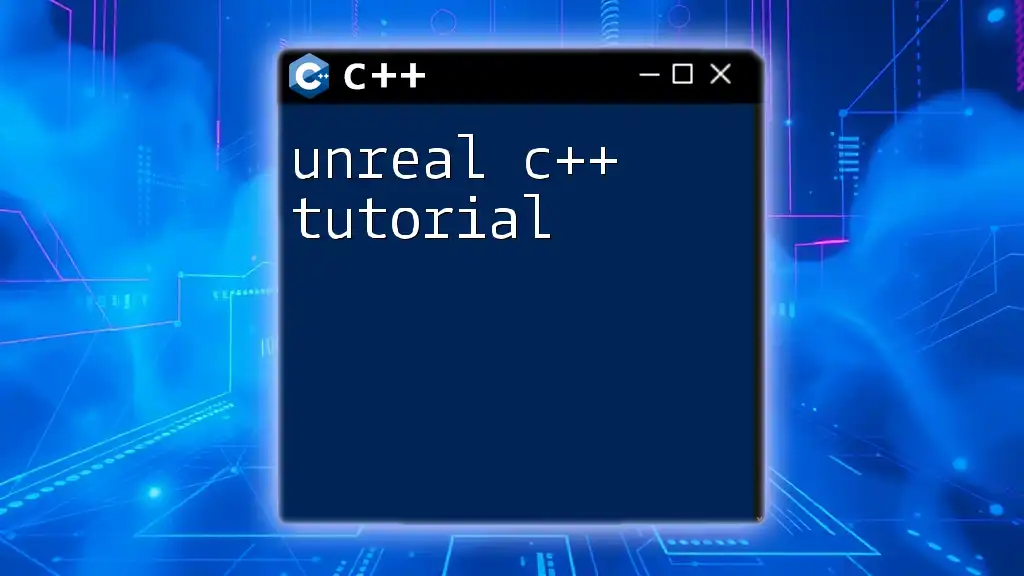
Troubleshooting Common Issues
Compiler Not Found
In some cases, you may encounter errors indicating that the compiler cannot be found. This issue can arise due to an improper installation or path configuration. Make sure that you’ve correctly installed the compiler and check your system PATH. Use the following command to check if the compiler installation path is included:
echo $PATH
If it’s not correctly included, you can add it by editing your ~/.bashrc file.
Installation Errors
Errors during the installation process can occur for a variety of reasons. Ensure that your package list is up to date using the `sudo apt update` command before starting the installation. If you still face issues, consider searching for the specific error message in forums or Ubuntu's help community.
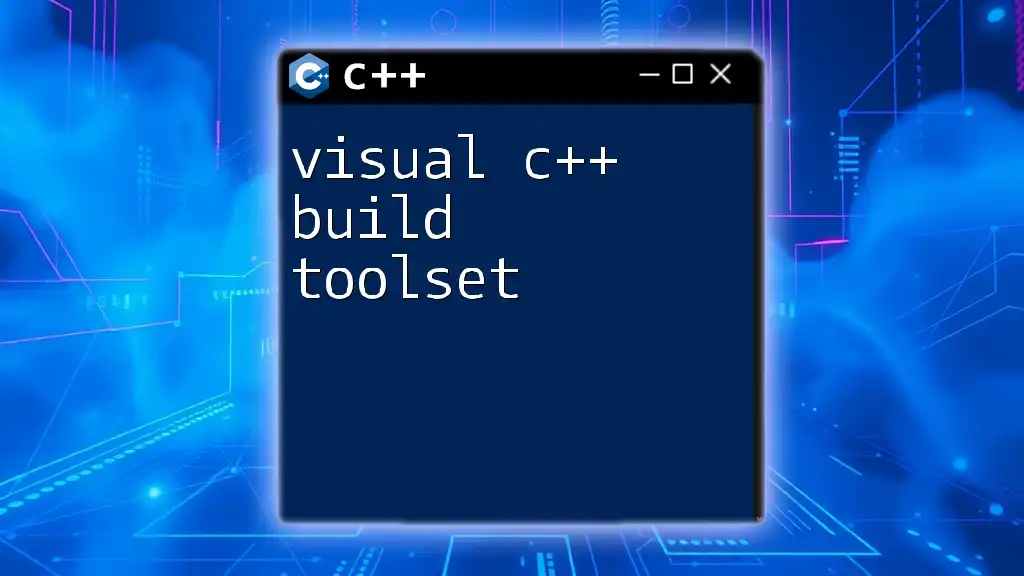
Conclusion
In this guide, we’ve walked through the essential steps to install C++ on Ubuntu, covering the installation of both GCC and Clang, setting up a development environment, and even writing a simple C++ program. With your compiler set up and your development environment ready, you can now explore the world of C++ programming right from your Ubuntu system.
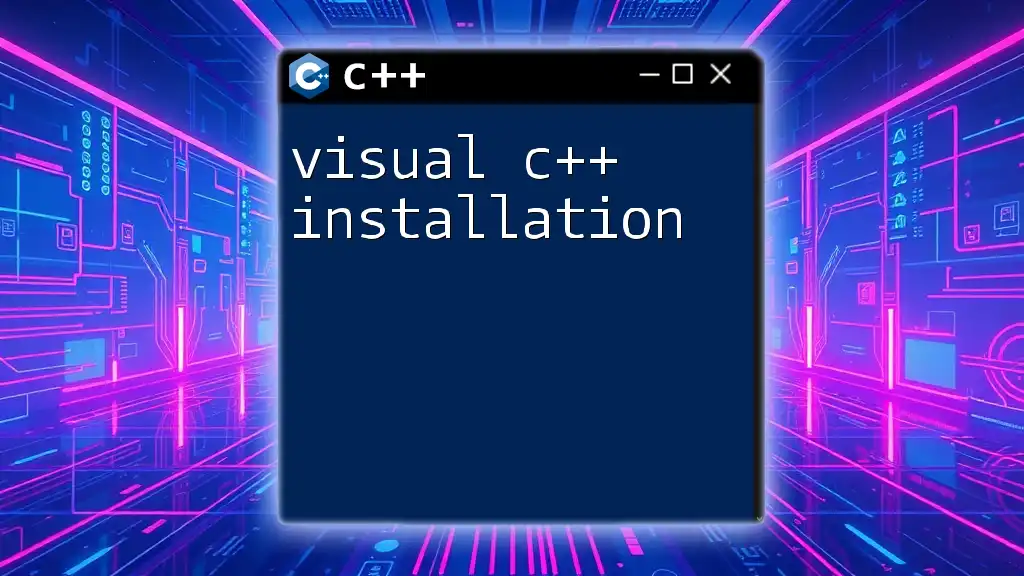
Additional Resources
To further enhance your C++ skills, consider exploring the official C++ documentation, online programming forums, and various educational platforms offering courses tailored to your needs. Whether you're a beginner or looking to deepen your understanding, there are countless resources available to support your journey into C++ development.