Rust C++ interop refers to the process of integrating Rust code with C++ applications, allowing developers to leverage the strengths of both languages for better performance and safety.
Here's a simple code snippet demonstrating FFI (Foreign Function Interface) between Rust and C++:
// C++ Code (main.cpp)
extern "C" {
void rust_function();
}
int main() {
rust_function();
return 0;
}
// Rust Code (lib.rs)
#[no_mangle]
pub extern "C" fn rust_function() {
println!("Hello from Rust!");
}
To compile and link, you would typically build the Rust code as a shared library and link it to your C++ application.
Understanding Rust and C++
What is Rust?
Rust is a systems programming language that emphasizes memory safety, speed, and concurrency. Unlike C++, Rust adopts a stricter approach to memory management through its ownership model, ensuring that data races and memory leaks are minimized. Rust's key features include:
- Ownership and Borrowing: These concepts allow Rust to manage memory without a garbage collector, thus providing high performance and safety.
- Concurrency Support: Rust's type system enforces thread safety, making it easier to build concurrent applications without the common pitfalls of data races.
- Rich Type System: With features like pattern matching and algebraic data types, Rust enables developers to express complex ideas succinctly and safely.
What is C++?
C++ is a versatile and powerful systems programming language that extends the capabilities of C with support for object-oriented programming. Its most significant features include:
- Object-Oriented: C++ supports encapsulation, inheritance, and polymorphism, allowing developers to create modular and reusable code.
- Low-Level Memory Manipulation: C++ gives programmers direct access to memory management, which is crucial for performance in systems-level programming.
- Extensive Standard Library: The C++ Standard Library provides numerous data structures and algorithms that simplify development tasks.
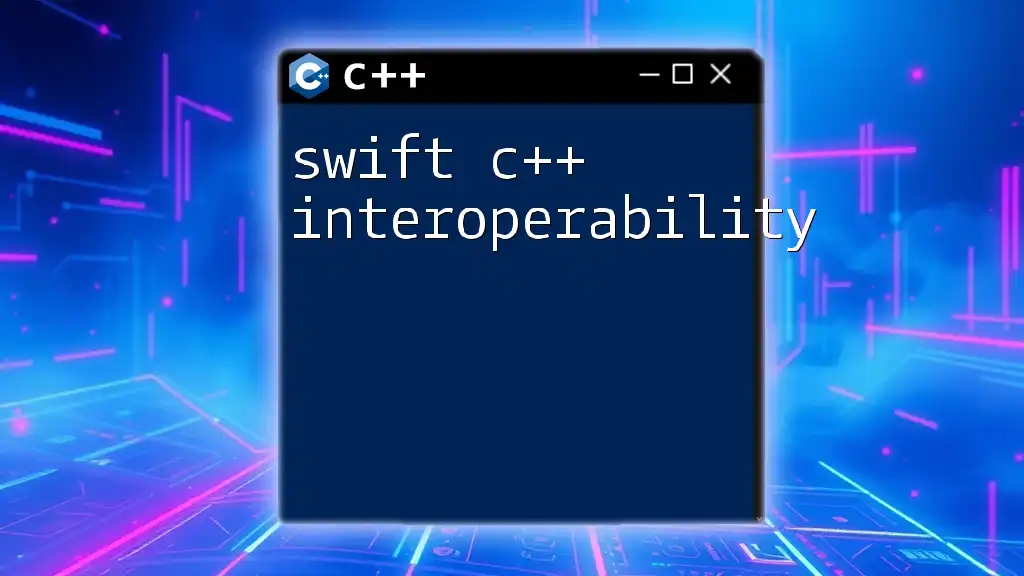
The Importance of Interoperability
What is Interoperability?
Interoperability refers to the capability of different systems or programming languages to work together. In the context of Rust and C++, it means utilizing libraries or components written in one language within the other, thereby taking advantage of both languages' strengths.
Key Benefits of Rust C++ Interoperability
- Utilizing Existing Libraries: Many legacy systems and performance-critical applications are developed in C++. By integrating with these systems, developers can leverage powerful libraries without having to rewrite them in Rust.
- Enhanced Safety: By using Rust's memory-safe constructs alongside C++ code, developers can improve the safety of their applications. Rust enforces strict ownership rules which can help prevent common bugs related to memory management.
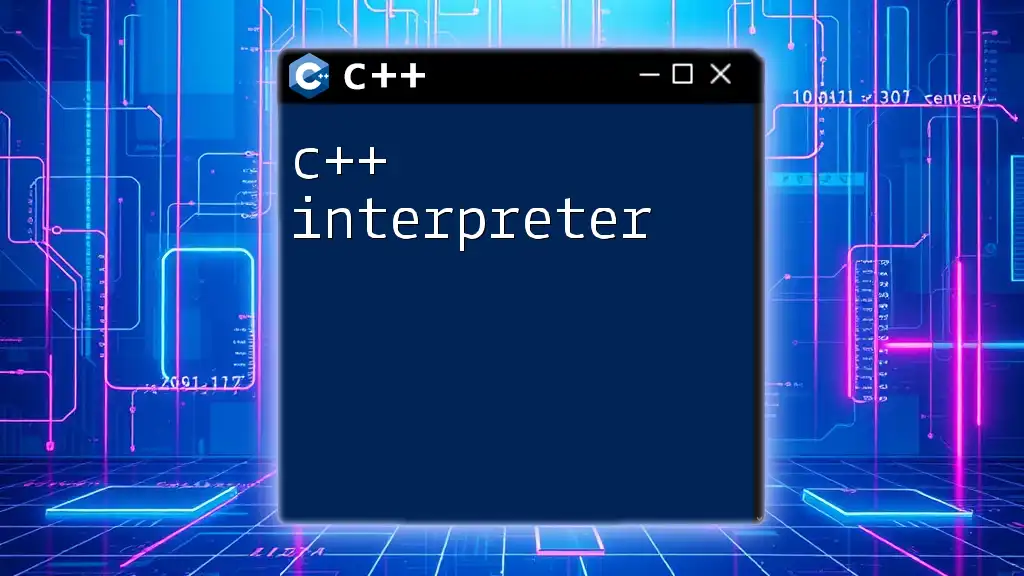
Setting Up Your Environment
Installing Rust
To begin using Rust, you can easily install it via `rustup`, an installer for the Rust programming language. Here’s how to get started:
- Open your terminal and run the command:
curl --proto '=https' --tlsv1.2 -sSf https://sh.rustup.rs | sh
- Follow the on-screen instructions to complete the installation.
To confirm the installation, execute:
rustc --version
Setting Up C++
To set up a C++ development environment, you will typically need a C++ compiler. For example, with `g++` (GNU C++ Compiler), you can follow these steps:
- Install `g++` on your system. On Ubuntu, you can run:
sudo apt-get install build-essential
- Create a simple C++ source file:
touch example.cpp
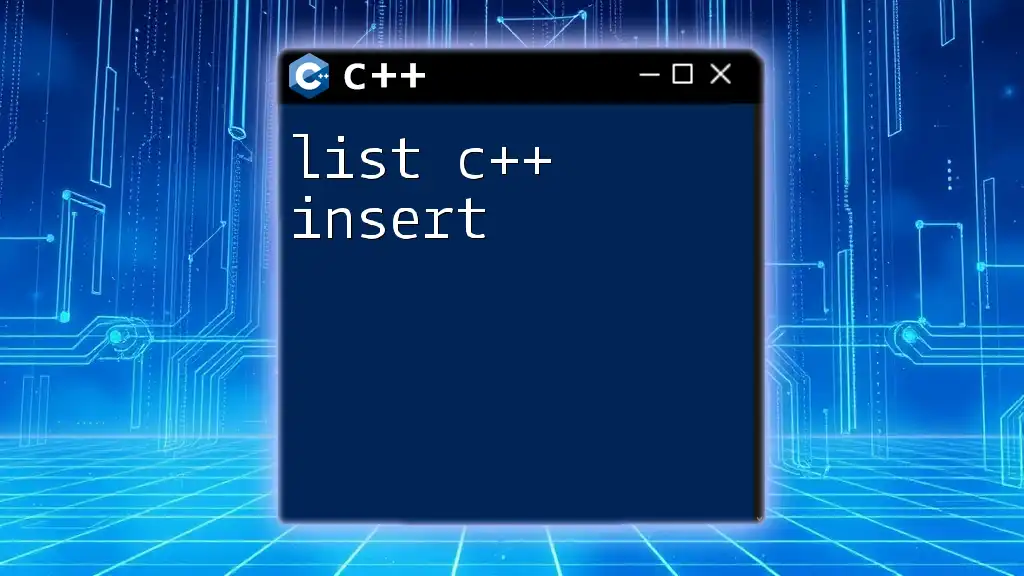
Interfacing Rust and C++
Understanding FFI (Foreign Function Interface)
The Foreign Function Interface (FFI) allows Rust to call C and C++ code and vice versa. Interfacing with C++ is predominantly conducted through an `extern "C"` declaration, allowing Rust to link against C-style functions. This is essential for ensuring that the C++ compiler does not alter function names, which can occur due to name mangling in C++.
Creating a Simple C++ Library
To demonstrate interoperation, we will create a simple C++ library that contains a function:
- Write a simple function in your `example.cpp` file:
extern "C" int add(int a, int b) { return a + b; }
- Compile the function as a shared library:
g++ -shared -o libexample.so -fPIC example.cpp
Calling C++ Functions from Rust
Next, we will call the C++ function from a Rust application.
-
Set up a new Rust project:
cargo new rust_cpp_interop cd rust_cpp_interop
-
Edit the `Cargo.toml` file to specify the library:
[dependencies] libc = "0.2"
-
In `src/main.rs`, we will import the function:
#[link(name = "example", kind = "dylib")] extern "C" { fn add(a: i32, b: i32) -> i32; } fn main() { unsafe { let result = add(5, 7); println!("Result: {}", result); } }
In this example, we declare the C++ function `add` using `extern "C"` and call it inside an `unsafe` block. This is necessary because FFI interactions can lead to undefined behavior if not handled correctly.
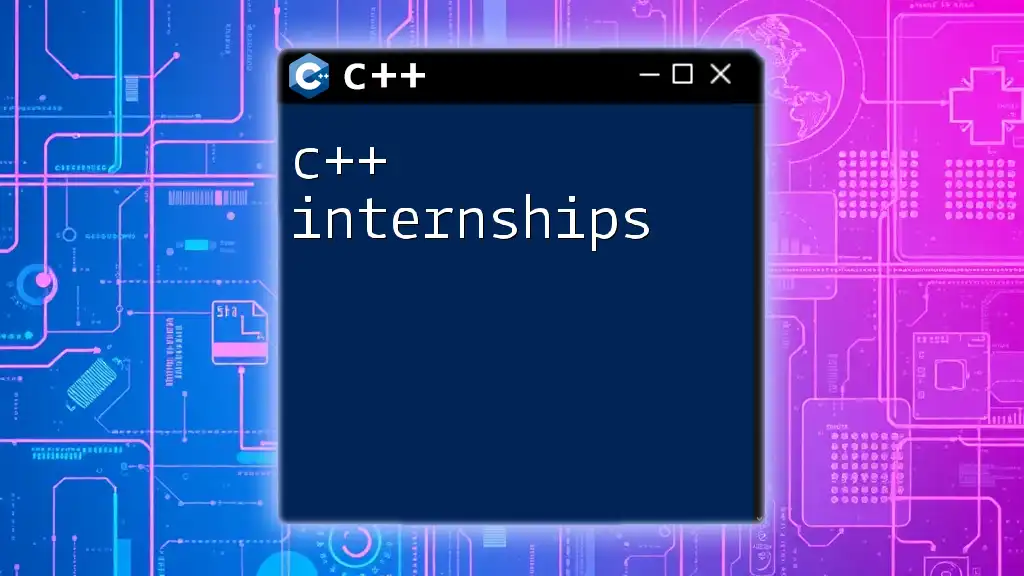
Handling Complex Data Types
Passing Structures Between Rust and C++
When passing custom data types like structs between Rust and C++, you must properly align the structures in memory.
-
Define a C++ struct and modification to our library:
struct Point { int x; int y; }; extern "C" Point create_point(int x, int y) { return Point{x, y}; }
-
In the Rust code, we declare the struct as follows:
#[repr(C)] struct Point { x: i32, y: i32, } extern "C" { fn create_point(x: i32, y: i32) -> Point; } fn main() { unsafe { let point = create_point(3, 4); println!("Point: ({}, {})", point.x, point.y); } }
Managing Memory Across Languages
One critical aspect of Rust C++ interop involves memory management. Rust’s ownership model prevents dangling pointers and double frees, while C++ allows for manual memory management.
When passing ownership between Rust and C++, it is crucial to clearly define who is responsible for freeing the memory. Proper documentation for external functions should specify whether the caller or the callee is responsible for memory management.
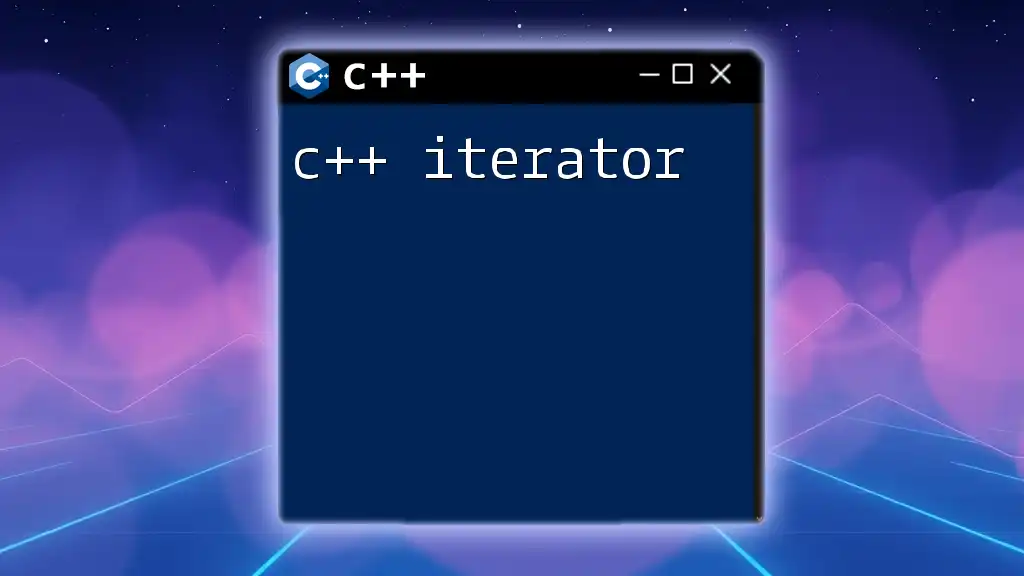
Error Handling in Rust and C++
Error Handling Strategies
Rust implements a robust error handling mechanism with `Result` and `Option` types, in contrast to C++'s exception handling approach. When dealing with FFI, it’s important to handle errors correctly to prevent crashes and undefined behavior.
- In Rust, you can use the `panic!` macro to signal an unhandled error state during unsafe calls.
- Alternatively, C++ functions should return error codes to indicate failure or success.
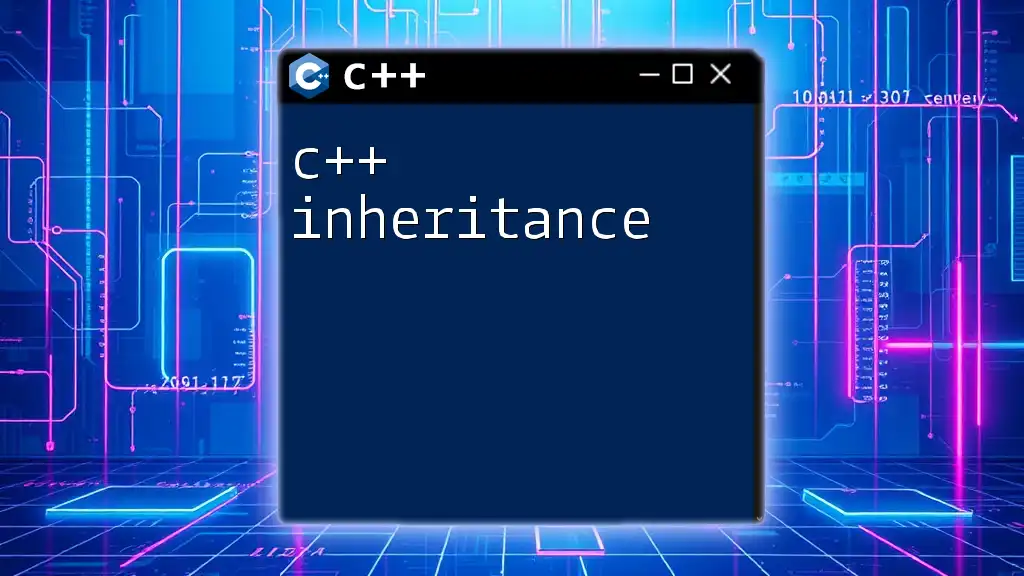
Common Pitfalls and Best Practices
Common Issues
Interacting between Rust and C++ can lead to several issues if not properly managed:
- Mismatched Data Types: Make sure that data sizes and types match across language boundaries.
- Memory Leaks: Always ensure that memory allocations are properly handled in both languages to avoid leaks.
- Thread Safety Concerns: When using threads, be mindful of accessing shared resources across Rust and C++.
Best Practices
- Design Clean Interfaces: Abstract away complex logic in C++ before exposing it to Rust. This will simplify the API and reduce potential errors.
- Testing Interoperability: Always conduct thorough testing to ensure that FFI calls work accurately. Unit tests are highly recommended to catch any issues early.
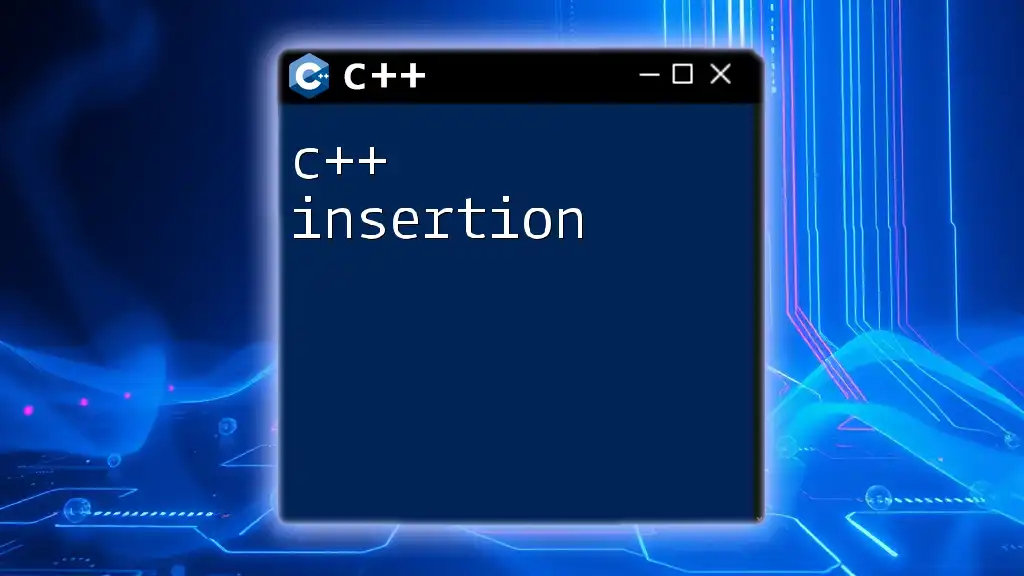
Real-World Scenarios
Use Cases for Rust C++ Interoperability
In the real world, many software systems can benefit from Rust C++ integration:
- Game Development: Utilizing C++ libraries for graphics rendering while leveraging Rust for game logic can improve both performance and safety.
- Embedded Systems: Many embedded applications rely on C++. Rust can be introduced gradually to enhance safety and reliability without disrupting existing codebases.
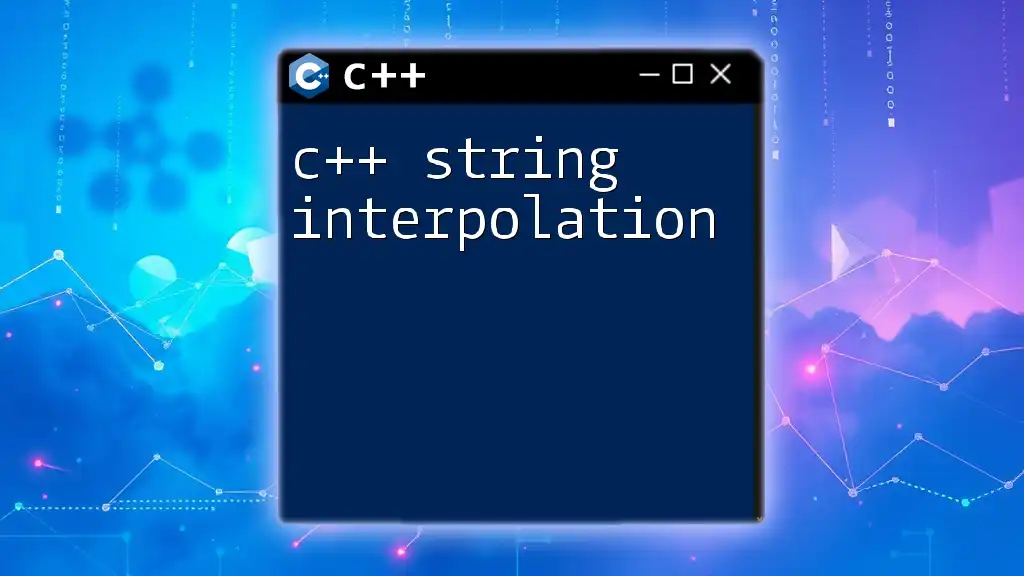
Conclusion
Rust C++ interoperability enables developers to harness the unique strengths of both languages, leading to safer and more efficient software solutions. By following best practices and understanding the intricacies of FFI, you can create robust applications that leverage existing C++ code while benefiting from Rust's safety features. As you delve into this exciting fusion of programming languages, consider experimenting with examples and expanding your skillset through hands-on practice.