The C++ linker is a tool that combines multiple object files generated by the compiler into a single executable, ensuring that all necessary references to functions and variables are resolved.
// Example of how to compile and link C++ files
g++ main.cpp utils.cpp -o my_program
What is a Linker?
The linker is a crucial tool in the C++ development process, often overlooked by beginners. At its core, the linker is responsible for taking one or more object files generated by the compiler and combining them into a single executable program. This process is essential for transforming the code written in high-level languages like C++ into machine-readable format.
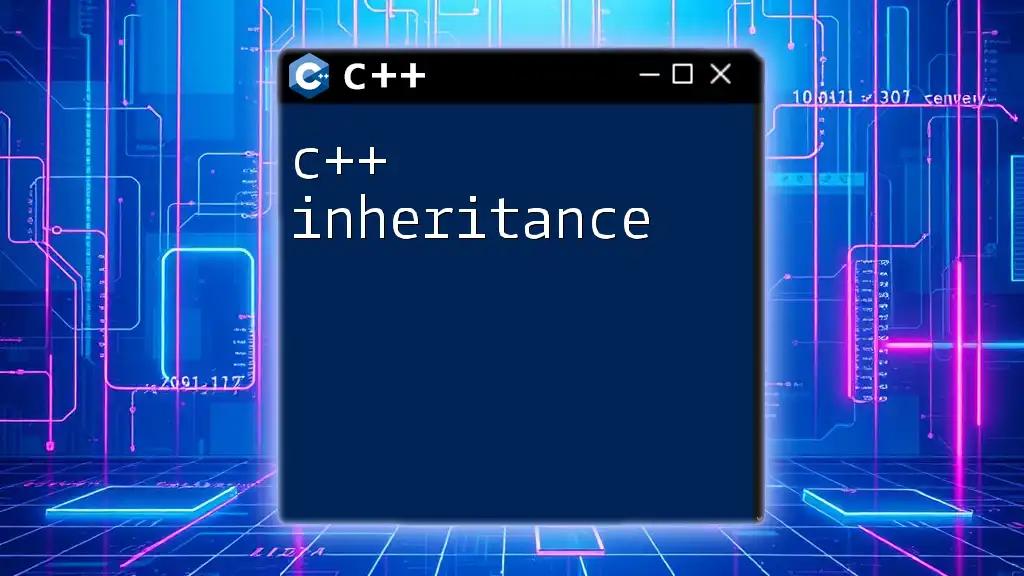
Compilation vs. Linking
To fully understand the role of the C++ linker, it's important to delineate between compilation and linking.
Explanation of the Compilation Process
When you write a C++ program and compile it, the compiler translates your code into one or more object files. Each object file contains the machine code that corresponds to the functions and variables defined in your source files, but it doesn’t yet form a complete executable.
Transition from Object Files to Executable
Once the compilation phase is complete, the linker takes over. It will process the object files, ensuring that function calls and variable references are correctly linked to their definitions. This is crucial for creating a functional and cohesive executable program.
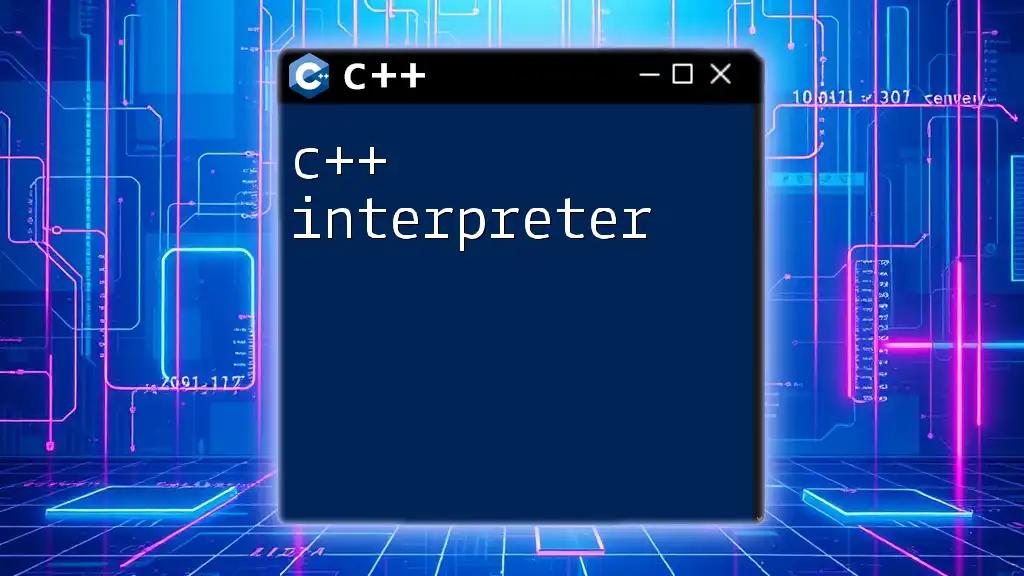
The Linking Process
The linking process can be divided into several stages, each serving a specific purpose.
Static Linking
Static linking involves combining all the necessary code into a single executable. This means that all the required libraries and functions are included directly in the final output file.
- Advantages: Static linking results in a single, standalone executable. This makes distribution easier, as there are no external dependencies that need to be installed on the target machine.
- Disadvantages: On the downside, static linking can result in larger executable sizes and may lead to longer build times.
Example of Static Linking
Here’s a simple example to show how static linking works:
// main.cpp
#include "math_functions.cpp"
int main() {
int result = add(3, 4);
return 0;
}
In this case, the implementation of `add` from `math_functions.cpp` is directly included in `main.cpp`, and the linker processes this to create a single executable.
Dynamic Linking
Dynamic linking is a more flexible approach where the executable relies on external library files at runtime rather than including them directly.
- Advantages: Dynamic linking leads to smaller executable sizes as common libraries can be shared across different programs.
- Disadvantages: However, it requires that the necessary libraries are available on the system where the program is run, which can complicate deployment.
Example of Dynamic Linking
Here’s how you would use dynamic linking in a simple program:
// main.cpp
extern "C" int add(int a, int b);
int main() {
int result = add(3, 4);
return 0;
}
In this example, the function `add` would be defined in a separate shared library, and the linker resolves this reference during runtime.
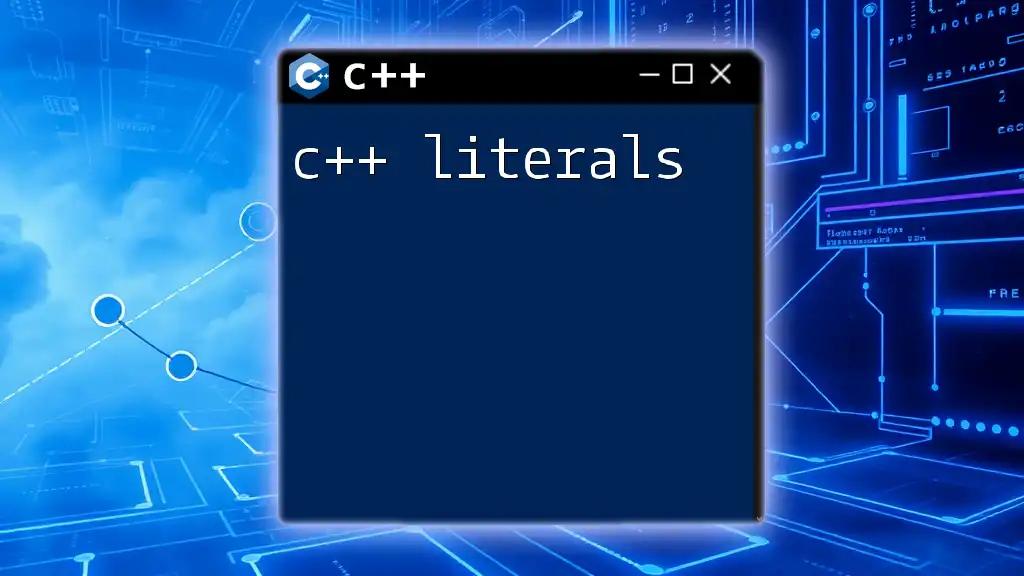
Types of Linkers
The two primary types of linkers used in C++ programming are static linkers and dynamic linkers.
Static Linkers
Static linkers operate by creating a complete executable at compile time. They do this by analyzing the object files and libraries needed for the program, and then packaging them into the final output.
Dynamic Linkers
Dynamic linkers, on the other hand, work at runtime. They look for the required libraries when the executable is launched, loading them into memory as needed. This allows for more flexibility and smaller file sizes.

The Role of Libraries in Linking
Libraries are essential components in the linking process, providing reusable code that can be shared across multiple projects.
Static Libraries vs. Dynamic Libraries
-
Static Libraries: These are collections of object files that are linked directly into the executable at compile time. Once linked, their code becomes part of the program.
-
Dynamic Libraries: Also known as shared libraries, these are separate files that the linker links at runtime. They allow multiple programs to share the same library code, reducing overall memory usage.
Linking with Libraries
To include a library in a C++ project, you will typically specify it during the compilation process. Here’s how you can link with a static library:
g++ -o my_app main.cpp math_functions.a
In this command, `math_functions.a` is the static library being linked to the program.

Common Linker Errors
Linker errors can be daunting for many developers, especially those new to C++. Understanding these errors is key to troubleshooting them effectively.
Types of Linker Errors
-
Undefined References: This occurs when the linker cannot find a definition for a function or variable that was declared in your code.
-
Duplicate Symbols: This error arises when two or more object files declare the same function or variable, causing ambiguity for the linker.
Example of an Undefined Reference Error
If you attempt to compile the following code without including the implementation of `add`:
g++ main.cpp -o my_app
You may encounter an output error like: `undefined reference to 'add(int, int)'`.
Troubleshooting Linker Errors
When you encounter linker errors, start by checking:
- Whether your function declarations and definitions match.
- If all necessary object files or libraries are included in your compile command.
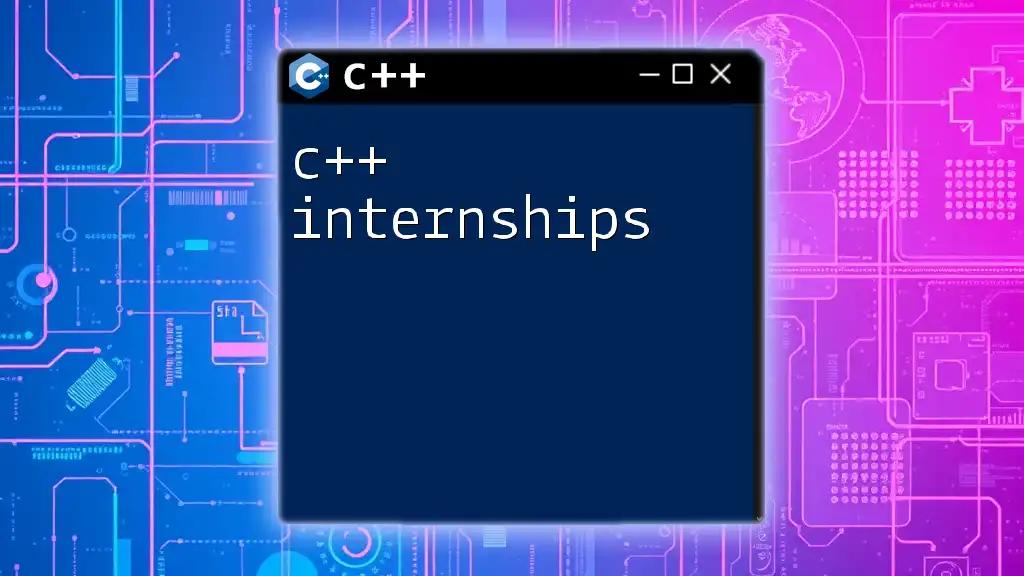
Best Practices for Effective Linking
To ensure a smooth linking process, consider adopting the following best practices:
Keeping Your Code Modular
Modular code helps in managing dependencies better. By breaking your application into distinct modules, you promote reusability and make the linking process more manageable.
Using Makefiles for Managing Builds
A Makefile can streamline the build process and manage dependencies effectively. Here’s an example of a simple Makefile for a C++ project:
all: main
main: main.o math_functions.o
g++ -o main main.o math_functions.o
clean:
rm -f *.o main
This Makefile will compile the source files and link them together into a single executable, while also providing a clean command to remove generated files.

Conclusion
In conclusion, mastering the C++ linker significantly enhances your ability to build efficient, functional applications. By understanding how the linking process operates, the types of linkers available, and how to troubleshoot common errors, you can optimize your C++ development workflow. Take the time to practice these concepts, as they are foundational to becoming a proficient C++ developer.

Additional Resources
For those looking to delve deeper into the topic, consider exploring books and online resources that focus on C++ programming and compilation processes. The official compiler documentation is also invaluable for understanding specific linker options and behaviors. Happy coding!