C++ internships provide practical experience for aspiring developers to enhance their coding skills while working on real-world projects, often involving collaborative programming and exposure to industry-standard practices.
Here's a simple code snippet demonstrating a basic C++ program structure:
#include <iostream>
int main() {
std::cout << "Hello, C++ Interns!" << std::endl;
return 0;
}
What is a C++ Internship?
A C++ internship is a temporary position where individuals, typically students or recent graduates, gain practical experience by working on projects that use C++. These internships are designed to help you develop your skills in a professional environment while applying theoretical knowledge in real-world situations.
Internships play a vital role in a developer's career path, as they offer a direct glimpse into the industry and the daily tasks of a C++ developer. This valuable experience can significantly enhance your resume and improve job prospects post-internship.
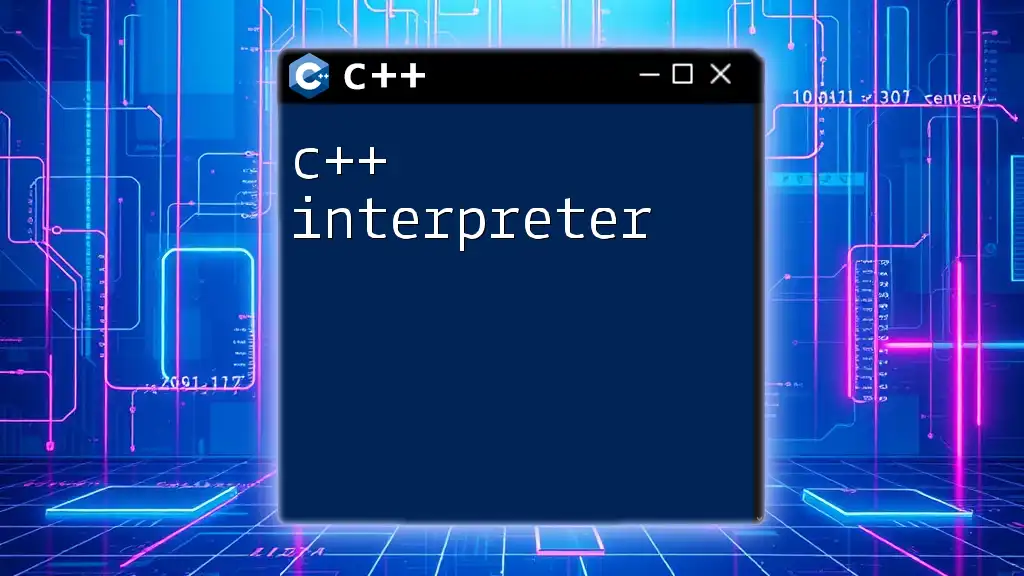
Benefits of C++ Internships
The advantages of securing a C++ internship are manifold:
Real-World Experience
Internships provide hands-on experience that classroom education often lacks. Engaging in actual coding projects allows interns to apply their knowledge in a practical setting, developing a deeper understanding of software development processes.
Networking Opportunities
Working as an intern gives you the chance to meet professionals in your field. Establishing connections can lead to mentorship opportunities, recommendations, and even job offers down the road.
Skill Enhancement
C++ internships often challenge interns to learn new languages, tools, or frameworks. The fast-paced environment allows you to enhance both technical and soft skills, making you a more competent developer.
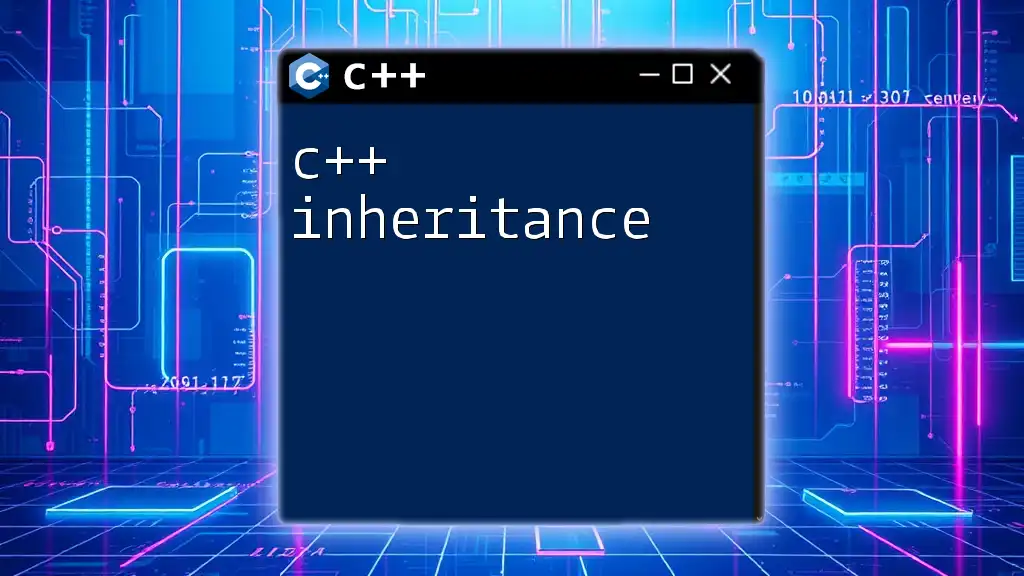
How to Find C++ Internship Opportunities
Finding a C++ internship requires research and persistence. Here are effective ways to discover potential opportunities:
Online Job Portals
Websites like LinkedIn, Indeed, and Glassdoor are excellent resources for finding C++ internships. Use specific search terms to narrow down the results and filter by location, company, and salary.
Company Websites
Major tech companies often list internship opportunities on their own careers page. Regularly check these websites to discover new postings or application requirements.
University Career Centers
If you're in school, leverage your institution's career center. Many universities have job boards specifically for students, featuring companies eager to recruit interns.
Networking Events and Meetups
Attend coding meetups, workshops, or hackathons to connect with professionals in the field. These events often have companies scouting for interns; showcasing your skills can put you on their radar.
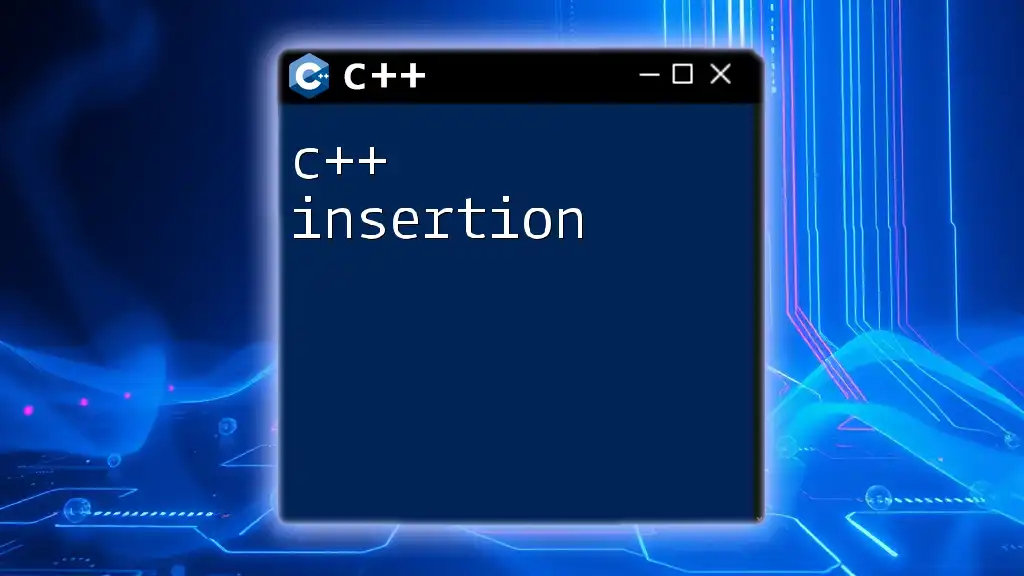
Preparing for a C++ Internship
To stand out when applying for internships, you need to prepare effectively:
Resume Writing Tips
Your resume should highlight your relevant skills and experience clearly. Include specific projects where you've applied C++, demonstrating your capability to handle real-world tasks.
Crafting an Effective Cover Letter
A well-written cover letter allows you to express your enthusiasm for the position. Emphasize your C++ skills and how they align with the company's goals.
Building a Portfolio
Create a portfolio showcasing your C++ projects. This could include coursework, personal projects, or contributions to open-source initiatives. A robust portfolio speaks volumes about your skills and dedication.
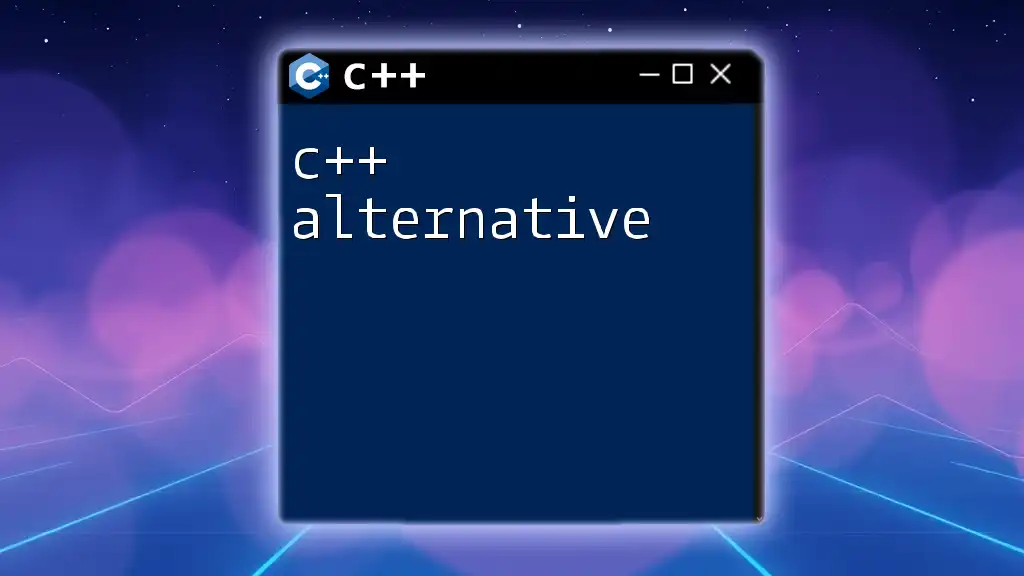
Essential C++ Skills for Interns
Equipping yourself with the necessary skills will make you a valuable candidate for C++ internships:
Core C++ Concepts
A solid understanding of basic C++ concepts is essential. You should be comfortable using variables, data types, control structures, and functions. For example, consider this simple C++ program that prints "Hello, World!":
#include <iostream>
using namespace std;
int main() {
cout << "Hello, World!" << endl;
return 0;
}
Object-Oriented Programming
C++ is renowned for its object-oriented features. Being proficient in OOP allows you to structure your code more effectively. A simple class definition might look like this:
class Dog {
public:
void bark() {
cout << "Woof!" << endl;
}
};
Data Structures and Algorithms
Familiarity with common data structures, such as arrays and vectors, is crucial. Understanding algorithms is equally important; consider a bubble sort algorithm:
void bubbleSort(int arr[], int n) {
for (int i = 0; i < n-1; i++)
for (int j = 0; j < n-i-1; j++)
if (arr[j] > arr[j+1]) {
swap(arr[j], arr[j+1]);
}
}
Version Control Systems
Proficiency in Git and understanding version control is imperative for effective collaboration within a software development team. Familiarize yourself with commands like `git commit`, `git push`, and `git pull`.
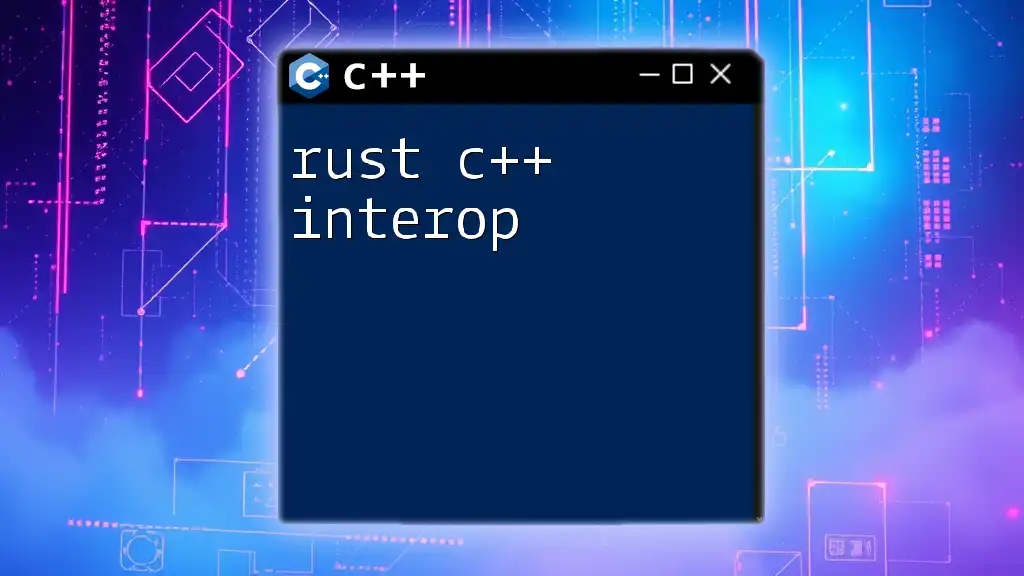
Interview Preparation for C++ Internships
The interview process can be daunting, but careful preparation can help you excel.
Common Interview Questions
Expect both behavioral and technical questions. Prepare for queries that assess not just your knowledge of C++, but also your problem-solving abilities and teamwork skills.
Coding Challenges
Practice coding challenges on platforms like LeetCode or HackerRank to hone your skills. These platforms simulate actual technical interviews and can help you become more comfortable with common problem types.
Mock Interviews
Engage in mock interviews with peers or mentors. This practice can help reduce anxiety and improve your performance under pressure.
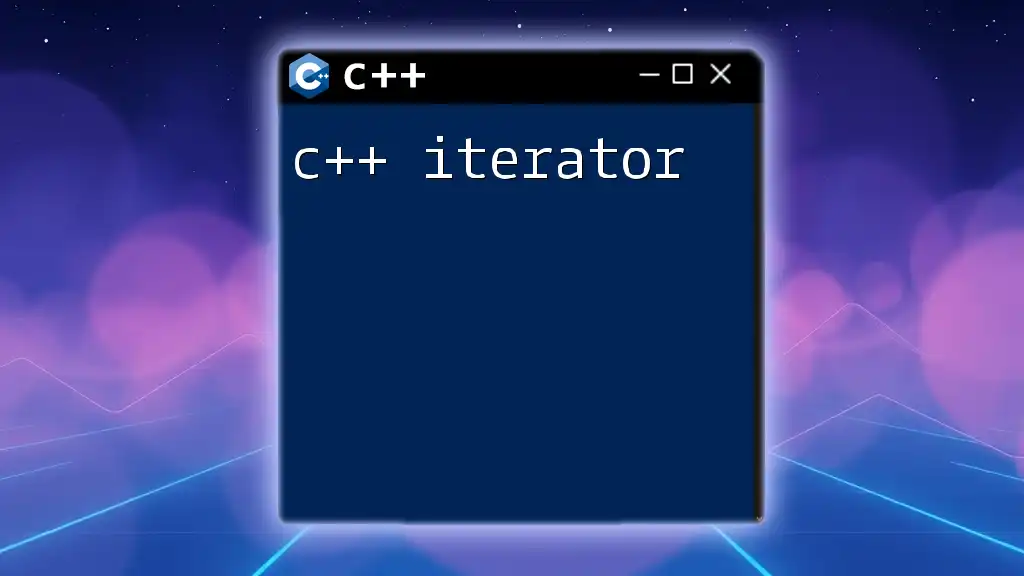
What to Expect During a C++ Internship
When you land a C++ internship, it's crucial to have realistic expectations about your role:
Internship Roles and Responsibilities
Intern duties may vary widely depending on the company and project. You might be asked to assist in debugging, writing code, or collaborating on design meetings. Be prepared for challenges and learning experiences.
Mentorship and Guidance
Most internships offer a mentorship component. A mentor can provide invaluable insight and assistance, guiding you through complexities you may encounter.
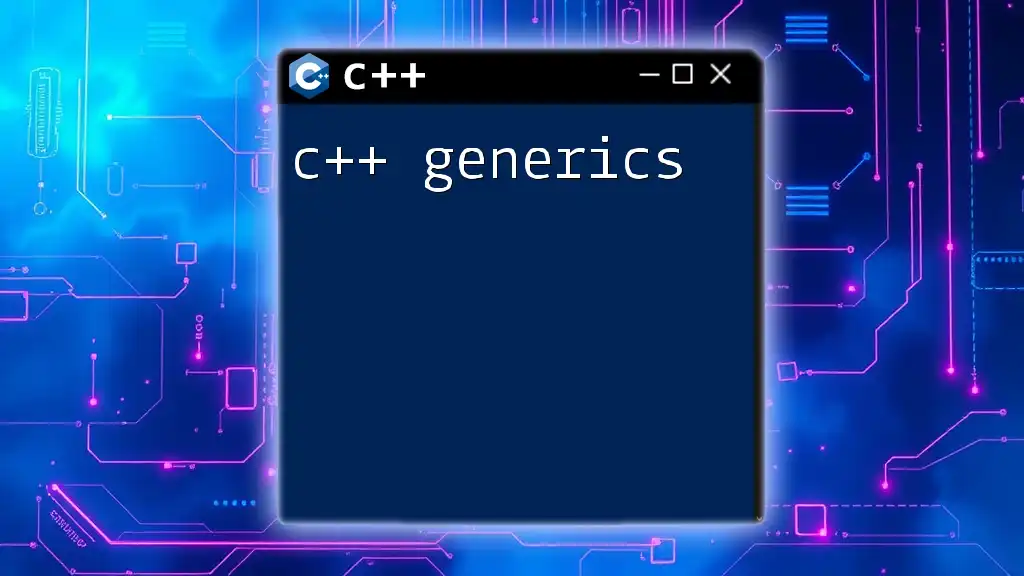
Maximizing Your Internship Experience
To get the most out of your internship, consider these tips:
Setting Goals
Establish personal and professional development goals. This approach not only gives you direction but also allows you to measure your growth throughout the internship period.
Feedback and Self-Assessment
Seek constructive feedback regularly. This can help you identify areas for improvement early, allowing you to become a more effective developer.
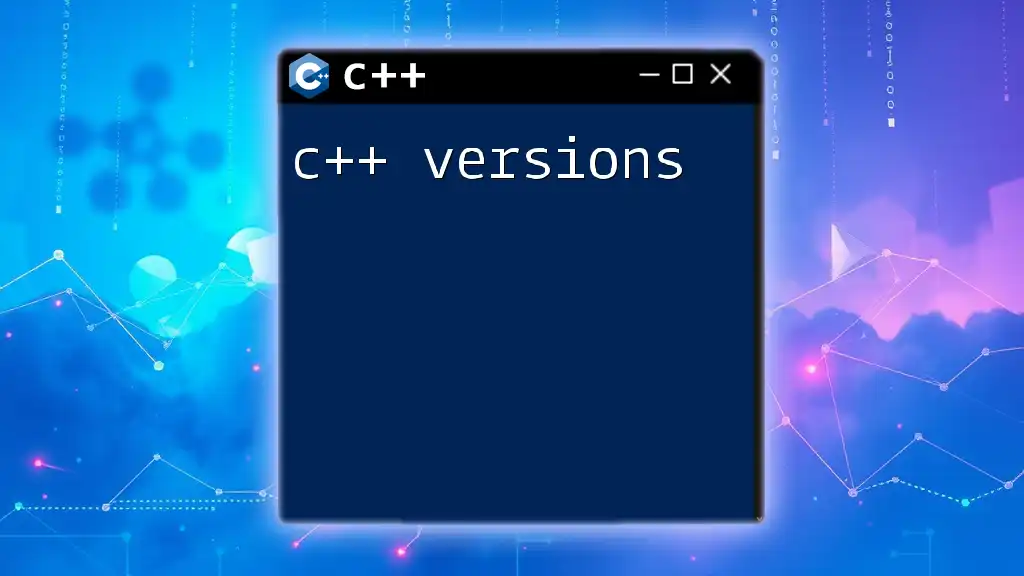
Transitioning from Internship to Full-Time Role
As your internship period comes to a close, consider how to transition into a full-time position:
How to Stand Out
Deliver quality work and show initiative. Demonstrate your passion for the role and willingness to learn, as these attributes can set you apart.
Conversations with Your Supervisor
Begin discussing potential job offers before your internship concludes. Express your interest in full-time opportunities within the company; this proactive approach might pay off.
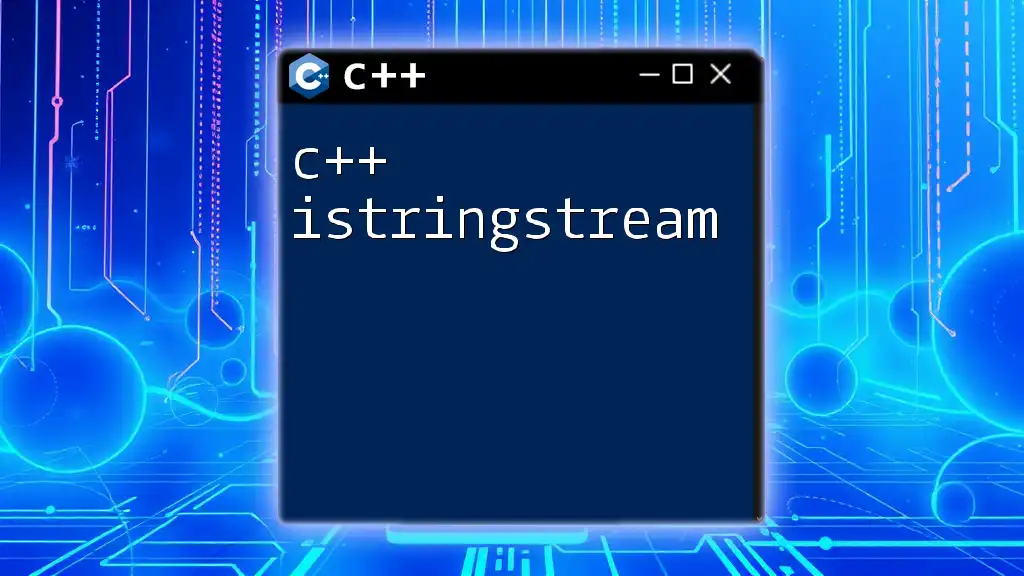
Conclusion
C++ internships serve as an essential stepping stone in your programming career. Not only do they provide valuable experience and skills, but they also open doors for future employment opportunities. Whether you’re currently in school or transitioning from another field, seeking a C++ internship is a proactive move that can lead to a successful career in software development.
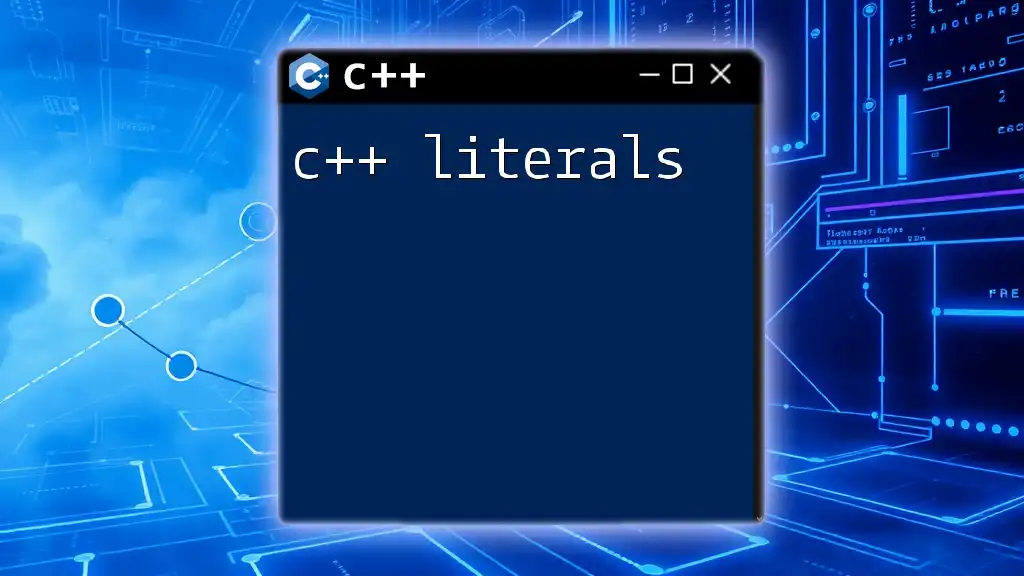
Additional Resources
To further enhance your skills, explore online courses and tutorials available on platforms like Codecademy and Coursera. Additionally, invest in books and references that cover advanced C++ concepts and programming practices. Continuous learning and practice are key to thriving in the technology landscape!