In C++, the ellipsis `...` can be used in function parameters to indicate that the function can accept a variable number of arguments, often referred to as a variadic function.
Here’s a simple code snippet demonstrating its usage:
#include <iostream>
#include <cstdarg>
void printNumbers(int count, ...) {
va_list args;
va_start(args, count);
for (int i = 0; i < count; ++i) {
std::cout << va_arg(args, int) << " ";
}
va_end(args);
}
int main() {
printNumbers(4, 10, 20, 30, 40); // Output: 10 20 30 40
return 0;
}
Understanding Ellipsis in C++
What is Ellipsis?
In C++, the ellipsis (`...`) is a powerful feature that allows developers to create functions capable of accepting a variable number of arguments. This capability is particularly useful when the exact number of parameters isn't known beforehand, enabling greater flexibility in function design.
Ellipsis Syntax
The ellipsis is introduced in a function declaration to indicate that the function can take a variable number of arguments. The syntax looks like this:
void myFunction(int count, ...);
In this case, `myFunction` can accept `count` as the first parameter along with a flexible number of additional arguments.
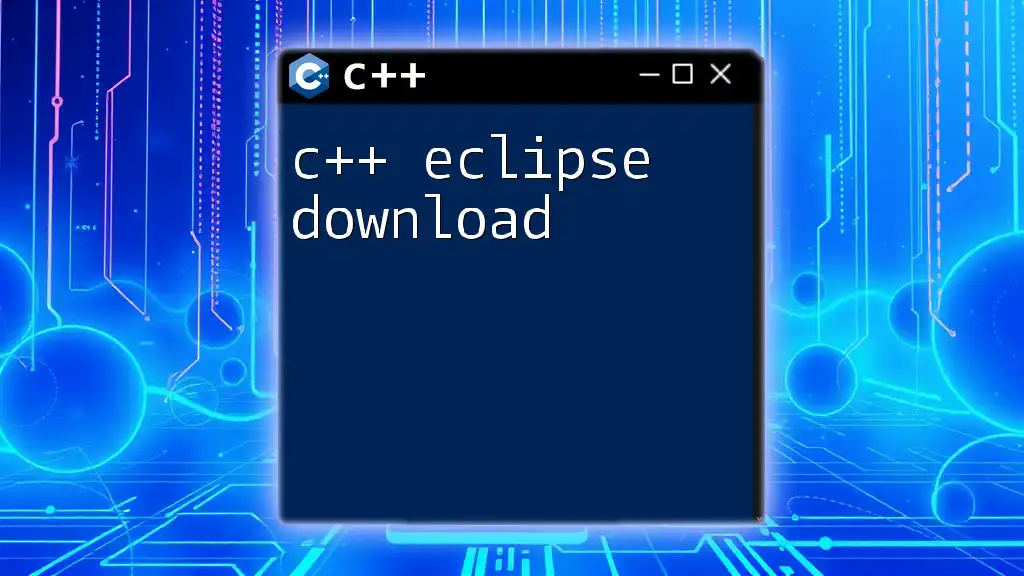
Uses of Ellipsis in C++
Variadic Functions
A core application of the C++ ellipsis is in variadic functions. These functions allow you to pass a varying number of arguments to them, making them incredibly versatile and useful in many programming scenarios.
Here’s a simple code snippet that demonstrates how to create a variadic function to print numbers:
#include <iostream>
#include <cstdarg>
void printNumbers(int count, ...) {
va_list args;
va_start(args, count);
for (int i = 0; i < count; i++) {
std::cout << va_arg(args, int) << " ";
}
va_end(args);
}
In this example, `printNumbers` takes multiple integer arguments. The `va_list`, `va_start`, and `va_arg` macros are used to handle the variable number of inputs effectively.
Flexible Argument Lists
The key advantage of using ellipsis in C++ is the creation of flexible argument lists. By allowing functions to accept a varying number of arguments, developers can create more adaptable and reusable code. For instance, if you are designing a logging function, it would be cumbersome to define every possible combination of arguments individually. Using ellipsis, you can seamlessly log different numbers of messages:
#include <iostream>
#include <cstdarg>
void log(const char* format, ...) {
va_list args;
va_start(args, format);
vfprintf(stdout, format, args);
va_end(args);
}
Here, the `log` function can accept any format string and a variable number of additional arguments corresponding to placeholders in that string, providing a flexible logging mechanism.
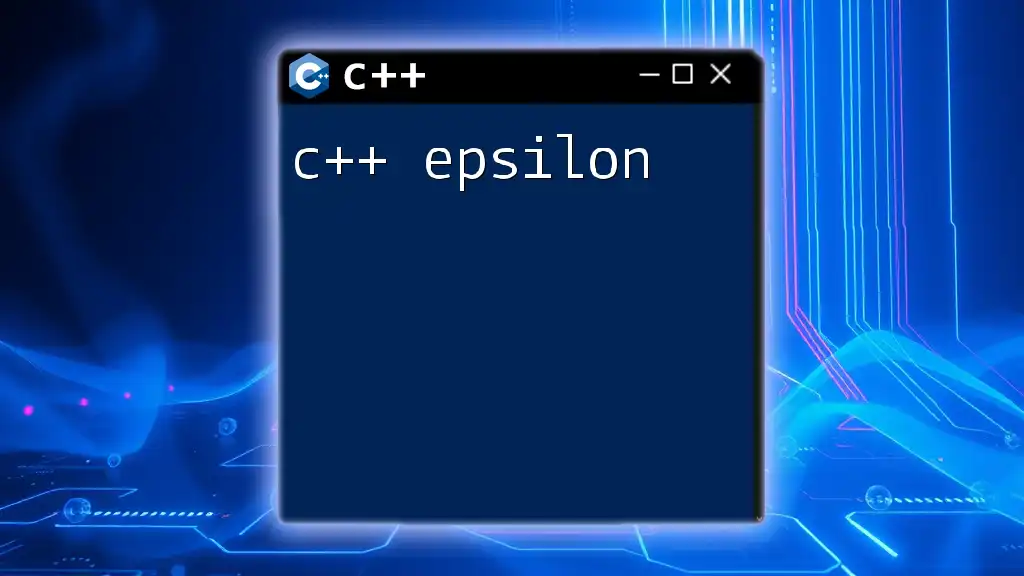
Implementation Details
Including Header Files
When utilizing variadic functions, it’s crucial to include the necessary header files. You generally need:
#include <cstdarg>
This header file provides access to the macros necessary for manipulating variable arguments.
Using `va_list`, `va_start`, and `va_end`
These macros are essential for managing the argument list in a variadic function:
- `va_list`: This type is used to declare a variable that can hold the address of the next argument.
- `va_start`: This macro initializes the `va_list` variable, pointing it to the first variable argument.
- `va_arg`: This macro takes two arguments: the `va_list` and the type of the next argument, returning the next argument from the list.
- `va_end`: This macro cleans up the `va_list`, ending its use.
Each of these macros plays a critical role in safely and effectively traversing the variable argument list within your function.
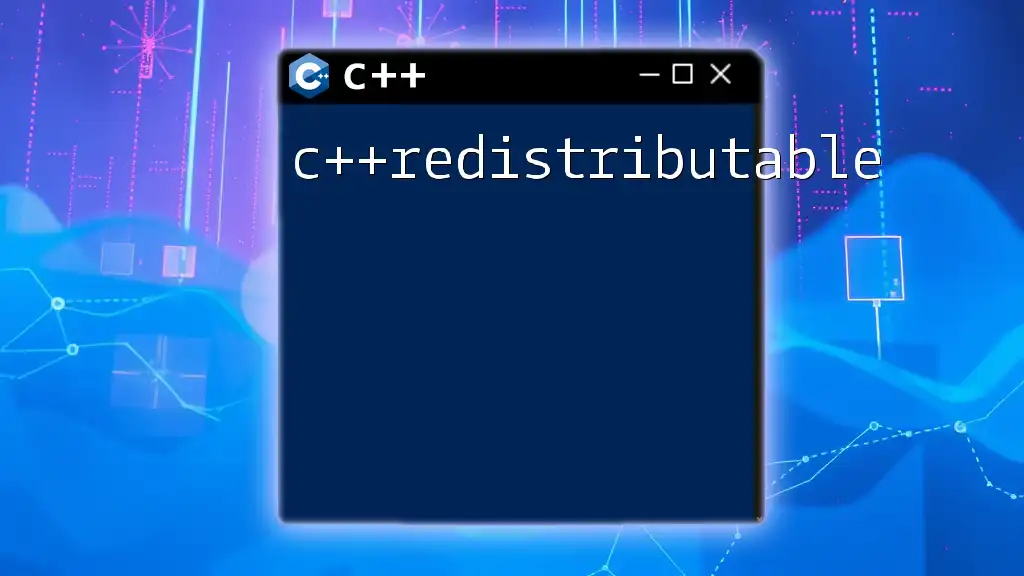
Error Handling and Best Practices
Limitations of Ellipsis
Even though ellipsis provides flexibility, it comes with several limitations. One major drawback is type safety. By using ellipsis, the compiler does not know the number or types of the arguments, which can lead to runtime errors if not managed carefully. For example, if you expect to receive only integers but mistakenly pass a string, you could encounter unpredictable behavior.
Best Practices
To ensure your code is safe and effective when using C++ ellipsis, consider the following best practices:
- Specify the number and types of expected arguments whenever possible. You might add an initial integer parameter that specifies how many arguments are being passed.
- Avoid overusing ellipsis. If your function’s parameters can be encapsulated within a structured type (like a struct or a class) or through template functions, those options may be safer and more maintainable.
- Consider alternatives. With modern C++, consider using templates or standard containers (e.g., `std::vector`) to handle arguments instead of relying solely on ellipsis.
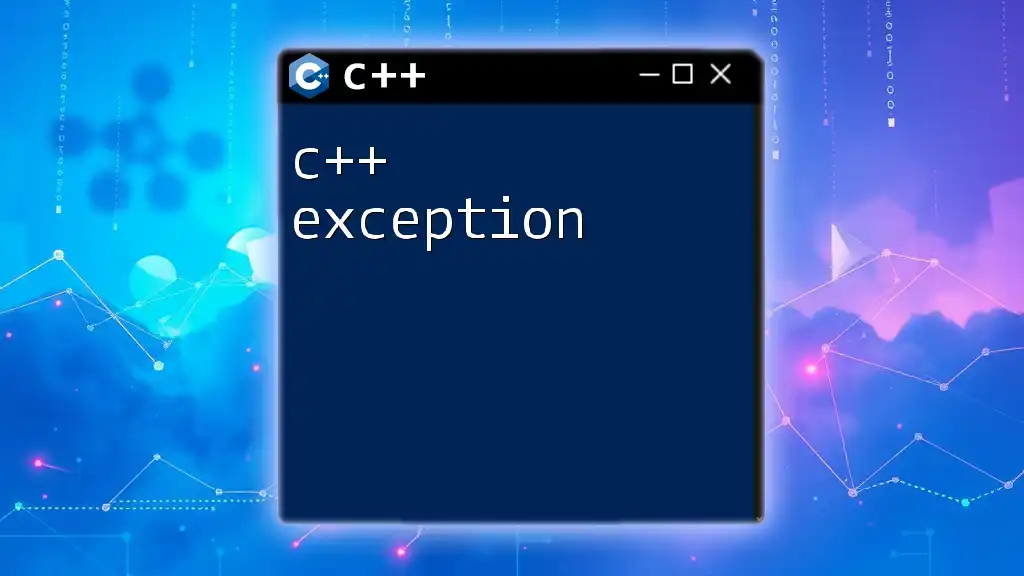
Advanced Topics
Using Ellipsis with Templates
Another fascinating aspect of C++ ellipsis is its compatibility with template functions. This combination allows for the creation of functions that can handle any number of arguments of different types. Here’s an example:
template<typename... Args>
void myTemplateFunction(Args... args) {
// Implementation
}
This function can accept any number of parameters, and thanks to templates, you can maintain type safety.
Ellipsis in C++17 (Fold Expressions)
With the introduction of C++17, fold expressions extend the usability of ellipsis even further, allowing developers to perform operations on parameter packs more elegantly. Here’s a quick demonstration of summing values using fold expressions:
template<typename... Args>
auto sum(Args... args) {
return (args + ...);
}
This code leverages the fold expression syntax to sum all the values passed to the `sum` function, showcasing a clear and concise way to manage multiple arguments.
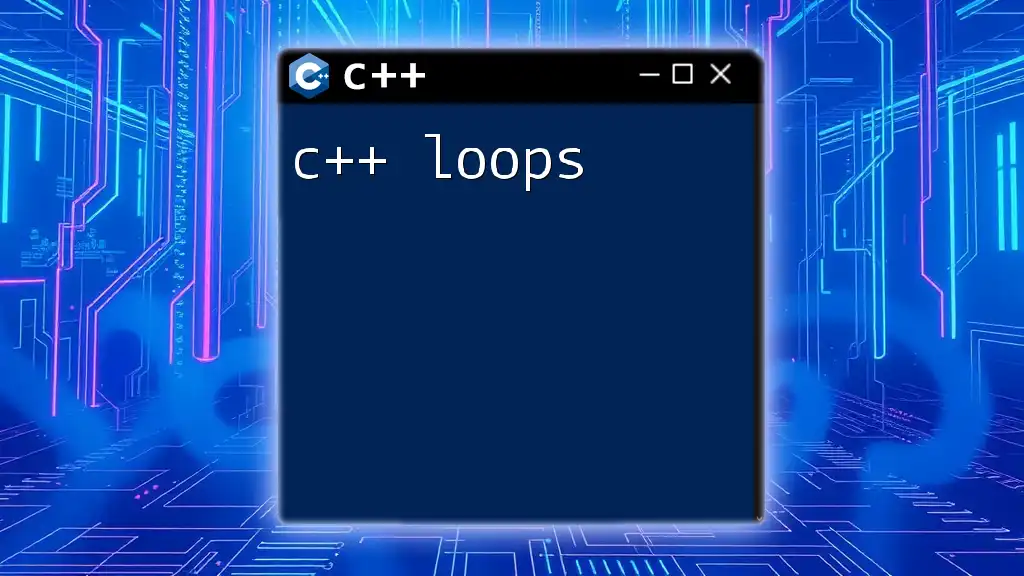
Conclusion
The C++ ellipsis is an indispensable tool for developers, allowing for the crafting of flexible and reusable functions. By embracing ellipsis and understanding its implications in variadic functions, argument handling, and templates, programmers can significantly enhance their coding efficiency.
As you explore this versatile feature, practice with examples and dive deeper into using variadic templates for even more robust designs. The ability to handle a variable number of arguments opens up countless possibilities, enabling powerful and adaptable C++ programming techniques.
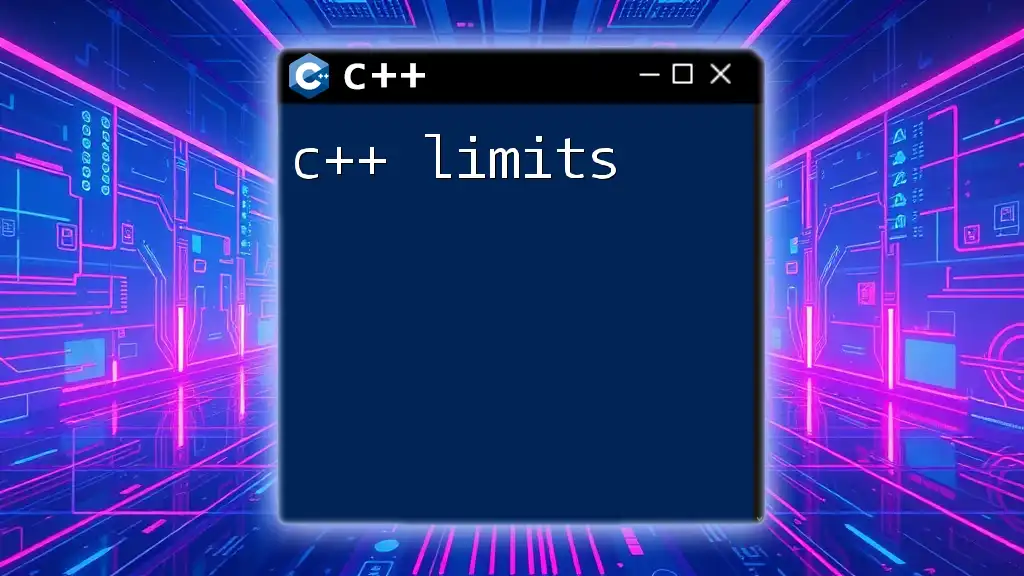
Additional Resources
For further reading, consider delving into well-regarded C++ books and online courses, as well as participating in C++ community forums and discussions. These resources will strengthen your mastery of C++ and expand your understanding of advanced features like ellipsis.