The `isspace` function in C++ checks if a character is a whitespace character, returning a non-zero value if true and zero otherwise.
Here’s a code snippet demonstrating its usage:
#include <iostream>
#include <cctype>
int main() {
char ch = ' ';
if (isspace(ch)) {
std::cout << "'" << ch << "' is a whitespace character." << std::endl;
} else {
std::cout << "'" << ch << "' is not a whitespace character." << std::endl;
}
return 0;
}
What is isspace in C++?
The `isspace` function is a part of the C++ Standard Library that is crucial for character classification. Specifically, it checks whether a given character is a whitespace character. Whitespace characters include spaces, tabs, vertical tabs, form feeds, and newline characters.
To utilize `isspace`, it's important to include the header file `<cctype>` in your C++ program. This inclusion ensures that all character classification functions, including `isspace`, are available for use.

How does isspace Work?
Function Signature
The function is defined in the standard library as follows:
int isspace(int ch);
This means that the function takes a single parameter of type `int`, which represents a character, and returns a non-zero value (typically `1`) if the character is a whitespace character, or zero if it is not.
Returns True for Whitespace
The characters that `isspace` identifies as whitespace include:
- Space (` `)
- Horizontal Tab (`\t`)
- Vertical Tab (`\v`)
- New Line (`\n`)
- Form Feed (`\f`)
- Carriage Return (`\r`)
Understanding how `isspace` classifies these characters is fundamental for effective string manipulation and input handling in C++.

Using isspace: Syntax and Parameters
The syntax for using `isspace` is straightforward:
std::isspace(int ch);
Parameter Explanation
The parameter `ch` accepts an integer value that corresponds to the ASCII value of the character you wish to evaluate. It is important to cast character types to integers, especially when using standard C++ string or character classes. The ASCII values map as follows:
- The space character (' ') has an ASCII value of 32.
- The newline character ('\n') has an ASCII value of 10.
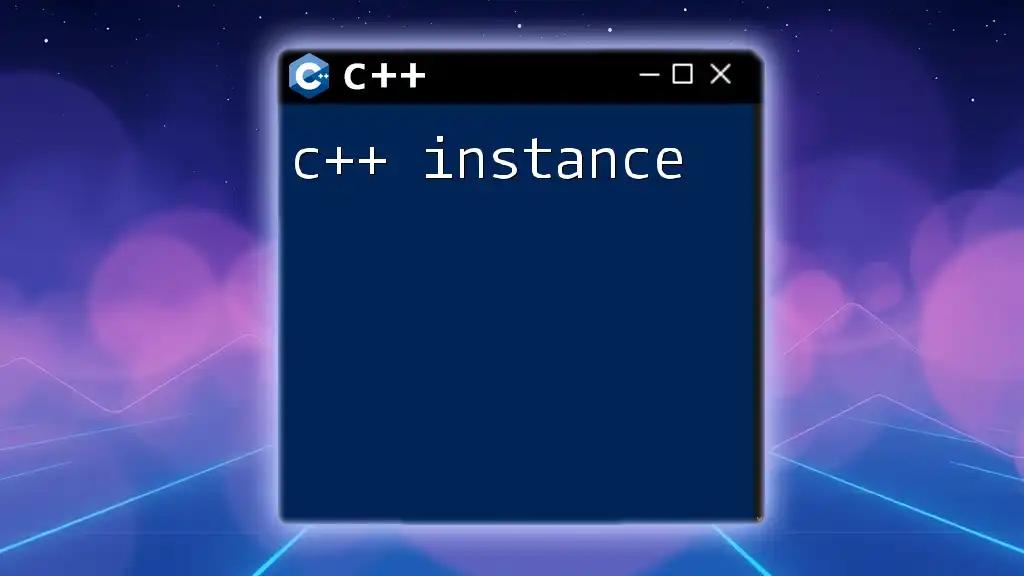
Examples of Using isspace in C++
Basic Example
Here's a simple example demonstrating `isspace`:
#include <iostream>
#include <cctype>
int main() {
char ch = ' ';
if (std::isspace(ch)) {
std::cout << "'" << ch << "' is a whitespace character." << std::endl;
}
return 0;
}
In this example, we initialize a character `ch` with a space. The `if` statement utilizes `isspace(ch)` to verify whether `ch` is a whitespace character. Upon evaluation, the program prints that the character is indeed whitespace.
Advanced Example
`isspace` can also be invaluable when handling strings. Let’s explore an example where we iterate through a string to identify whitespace characters:
#include <iostream>
#include <cctype>
#include <string>
int main() {
std::string str = "C++ is amazing!";
for (char ch : str) {
if (std::isspace(ch)) {
std::cout << "'" << ch << "' is a whitespace character." << std::endl;
}
}
return 0;
}
In this example, we created a string that contains a space. We loop through each character in the string and check each one with `isspace`. If it’s a whitespace character, we print a notification. This ability to check characters in a string context is one of the powerful applications of `isspace` in C++ programming.
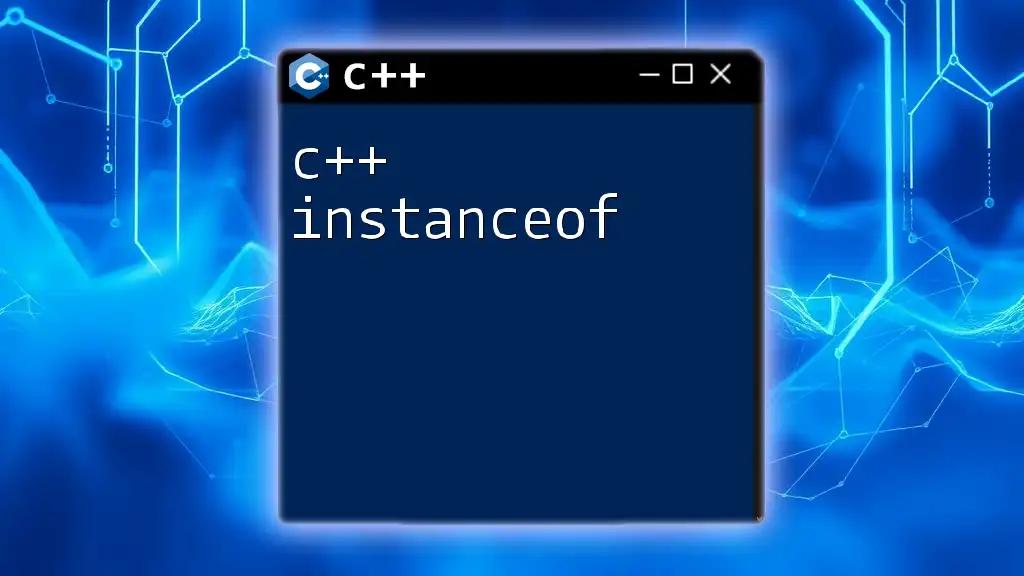
Common Mistakes with isspace
One common pitfall when using `isspace` is passing non-character data types as arguments. For example, inadvertently passing a floating-point or string type instead of a character may lead to unexpected behavior or compile errors. Always ensure that the argument is either a character or its corresponding ASCII integer value.
// Incorrect usage
double num = 4.5;
if (std::isspace(num)) { // This will lead to errors.
// ...
}
This scenario highlights the importance of understanding the expected input type for `isspace`.
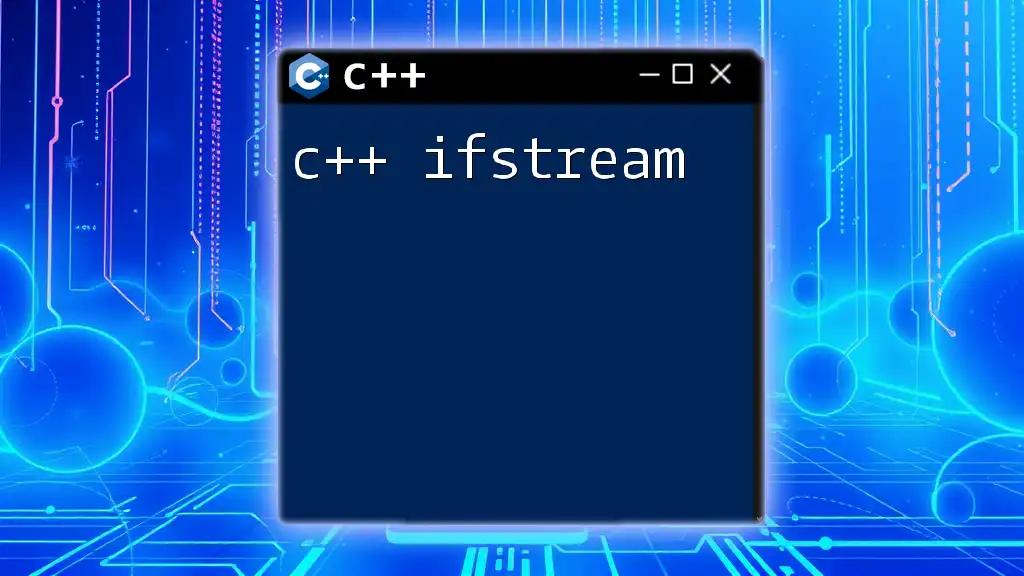
Performance Considerations
Using built-in functions like `isspace` is often more efficient than creating custom checks for whitespace. These built-in functions are optimized for performance within the standard library, making them a reliable choice for character classification. Custom checks, while sometimes necessary, can introduce performance overhead and complexity.
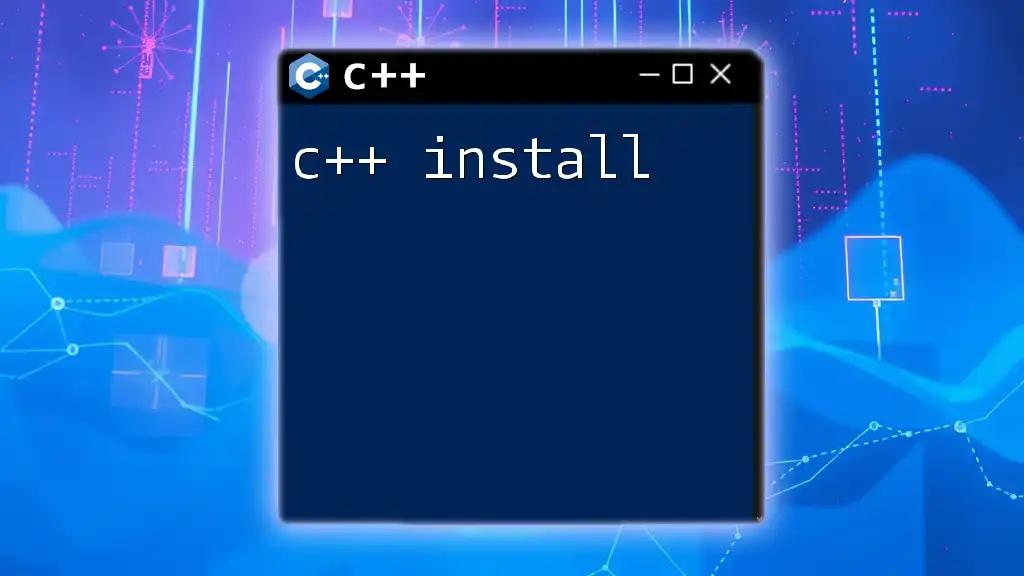
Best Practices
Knowing when to use `isspace` is vital for efficient programming. Use `isspace` when you need to validate user input, parse strings, or preprocess data before further manipulation. It’s also recommended to include a quick check and handle cases where your input might lead to invalid characters.
Alternatives to isspace
While `isspace` serves its purpose well, you may also come across other character classification functions in C++, such as `isalpha` for alphabetic characters and `isdigit` for digit characters. Knowing all these functions enhances your toolkit for string handling in C++.

Conclusion
The `isspace` function in C++ is a powerful and versatile tool for checking whitespace characters in your programs. Its ability to streamline character classification tasks makes it an essential function within the C++ standard library. By understanding its use cases, syntax, and potential pitfalls, you can effectively utilize `isspace` to enhance your C++ programming practices.
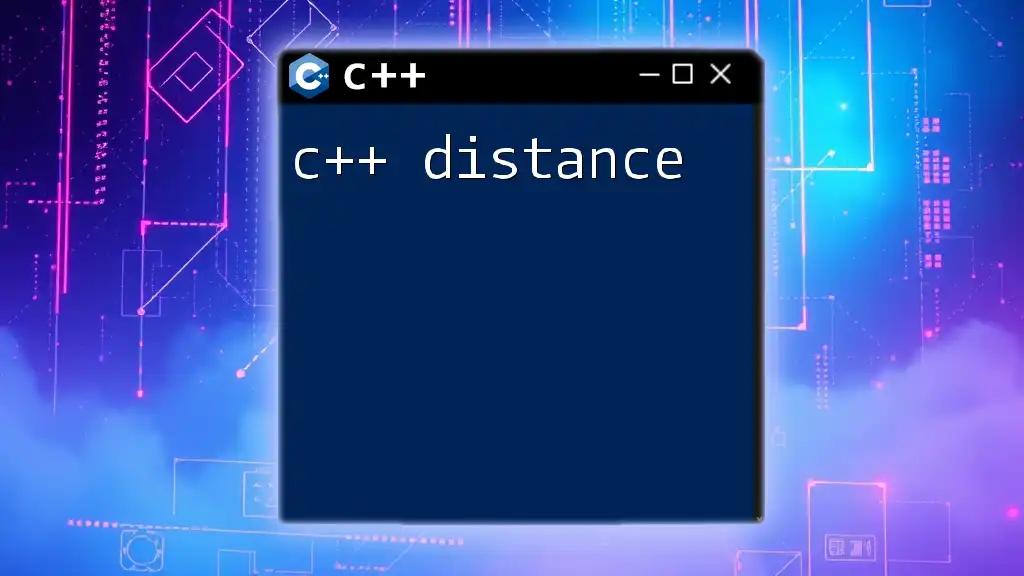
Additional Resources
To deepen your understanding of `isspace`, consult the [official C++ documentation](https://en.cppreference.com/w/cpp/string/byte/isspace) for comprehensive insights. Consider exploring recommended books or online courses designed to expand your knowledge of C++ programming.
Call to Action
If you're eager to dive deeper into mastering C++ commands and character manipulation, join our community and partake in our specialized programs designed to streamline your learning experience!