C++ inter-process communication (IPC) allows different processes to communicate with each other, enabling data exchange and coordination through various mechanisms such as pipes, sockets, and shared memory.
Here’s a simple example of using named pipes for IPC in C++:
#include <iostream>
#include <sys/types.h>
#include <sys/stat.h>
#include <fcntl.h>
#include <unistd.h>
#include <cstring>
int main() {
const char* pipePath = "/tmp/my_pipe";
mkfifo(pipePath, 0666); // Create a named pipe
// Write to the pipe
int fd = open(pipePath, O_WRONLY);
const char* msg = "Hello from process A!";
write(fd, msg, strlen(msg) + 1);
close(fd);
// Read from the pipe
fd = open(pipePath, O_RDONLY);
char buffer[128];
read(fd, buffer, sizeof(buffer));
std::cout << "Received message: " << buffer << std::endl;
close(fd);
unlink(pipePath); // Remove the named pipe
return 0;
}
What is Interprocess Communication (IPC)?
Interprocess Communication (IPC) is a fundamental concept in computing that allows different processes to communicate with one another. In the context of C++, IPC enables multiple processes to exchange data and synchronize their operations, which can be essential for applications that require cooperation among separate programs or threads.
IPC is particularly vital in scenarios where processes need to share resources or data. Without IPC, processes operate in isolation, making it difficult to achieve complex tasks that depend on collaboration. Its significance lies in enabling efficient resource utilization, enhancing program functionality, and improving overall application performance.
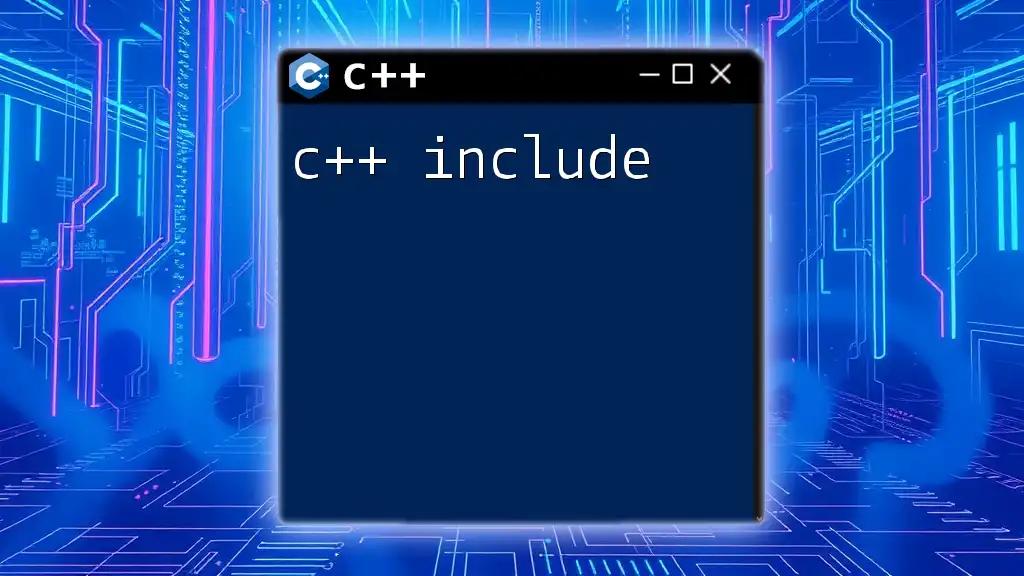
Types of IPC Mechanisms in C++
Pipes
Pipes are a straightforward IPC mechanism that allows for one-way communication between processes. They can be of two types: anonymous pipes and named pipes.
-
Anonymous Pipes are typically used for communication between a parent and child process. They do not have a name and can only be used within the lifetime of the processes that created them.
-
Named Pipes, on the other hand, can be used between any processes and persist beyond the terminating processes. This makes them more flexible for various applications.
Example of Using Pipes:
#include <unistd.h>
#include <iostream>
int main() {
int pipefd[2];
pid_t cpid;
char buf;
pipe(pipefd);
cpid = fork();
if (cpid == 0) { // Child process
close(pipefd[1]); // Close unused write end
read(pipefd[0], &buf, 1);
std::cout << "Received: " << buf << std::endl;
close(pipefd[0]);
} else { // Parent process
close(pipefd[0]); // Close unused read end
write(pipefd[1], "A", 1);
close(pipefd[1]);
}
return 0;
}
Message Queues
Message queues facilitate the storage of messages in a queue format, allowing processes to communicate asynchronously. This means a sender can send a message without requiring the receiver to be ready, making message queues particularly advantageous for multitasking environments.
This mechanism supports various characteristics, such as priority levels for messages, ensuring that more critical messages are processed first.
Example of Using Message Queues:
#include <sys/ipc.h>
#include <sys/msg.h>
#include <iostream>
#include <cstring>
struct message {
long msg_type;
char msg_text[100];
};
int main() {
key_t key = ftok("progfile", 65);
int msgid = msgget(key, 0666 | IPC_CREAT);
message msg;
msg.msg_type = 1;
strcpy(msg.msg_text, "Hello World!");
msgsnd(msgid, &msg, sizeof(msg), 0);
std::cout << "Message sent: " << msg.msg_text << std::endl;
return 0;
}
Shared Memory
Shared memory is the fastest IPC technique available, allowing multiple processes to access a common memory space. With shared memory, processes can read and write data directly to a shared segment, which minimizes the overhead associated with copying data between the processes.
Because shared memory can lead to synchronization problems, it is typically used in conjunction with semaphores to manage access among processes effectively.
Example of Using Shared Memory:
#include <sys/ipc.h>
#include <sys/shm.h>
#include <iostream>
#include <cstring>
int main() {
key_t key = ftok("shmfile", 65);
int shmid = shmget(key, 1024, 0666 | IPC_CREAT);
char *str = (char*)shmat(shmid, (void*)0, 0);
strcpy(str, "Shared Memory Test");
std::cout << "Data written to shared memory: " << str << std::endl;
shmdt(str);
return 0;
}
Semaphores
Semaphores are primarily used to control access to shared resources in concurrent programming. They act as counters that manage resource availability, preventing race conditions and ensuring safe data sharing.
- Binary Semaphores allow access to a single resource.
- Counting Semaphores manage multiple instances of resources, providing a more flexible control compared to binary semaphores.
Example of Using Semaphores:
#include <semaphore.h>
#include <pthread.h>
#include <iostream>
void* task(void* arg) {
sem_t* sem = (sem_t*)arg;
sem_wait(sem);
std::cout << "Thread entering critical section." << std::endl;
sem_post(sem);
return NULL;
}
int main() {
sem_t semaphore;
sem_init(&semaphore, 0, 1);
pthread_t tid[2];
for (int i = 0; i < 2; i++) {
pthread_create(&tid[i], nullptr, task, (void*)&semaphore);
}
for (int i = 0; i < 2; i++) {
pthread_join(tid[i], nullptr);
}
sem_destroy(&semaphore);
return 0;
}
Sockets
Sockets are a network-based IPC mechanism that facilitates communication between processes either on the same machine or over a network. They come in two primary varieties: TCP (connection-oriented) and UDP (connectionless). Sockets allow for versatile communication setups.
Example of TCP Socket Communication:
#include <sys/types.h>
#include <sys/socket.h>
#include <netinet/in.h>
#include <unistd.h>
#include <iostream>
#include <string.h>
int main() {
int sockfd = socket(AF_INET, SOCK_STREAM, 0);
struct sockaddr_in serv_addr;
serv_addr.sin_family = AF_INET;
serv_addr.sin_port = htons(8080);
serv_addr.sin_addr.s_addr = INADDR_ANY;
bind(sockfd, (struct sockaddr*)&serv_addr, sizeof(serv_addr));
listen(sockfd, 3);
int new_socket = accept(sockfd, nullptr, nullptr);
const char* message = "Hello from server!";
send(new_socket, message, strlen(message), 0);
close(new_socket);
close(sockfd);
return 0;
}

Choosing the Right IPC Method
When it comes to selecting the appropriate IPC method for a C++ application, several factors must be considered:
Factors to Consider
-
Performance: Depending on the application's needs, some IPC methods may perform better than others. For instance, shared memory tends to be faster than message queues but comes with more complexity in synchronization.
-
Complexity: While some IPC mechanisms, like pipes, are easier to implement, they may lack the flexibility needed for more complex applications. Consider the trade-offs between simplicity and functionality.
-
Data Size: The size of data that processes must share can dictate the choice. For smaller pieces of information, message queues or pipes may suffice. For bulk data, shared memory would be more appropriate.

Implementing IPC in C++
Example: Using Pipes in C++
To effectively utilize pipes for communication, one must understand how to establish communication between a parent and child process.
Here's a deeper implementation of pipes in action. The example shared earlier demonstrates communication where the parent process writes to the pipe, and the child reads from it.
Example: Using Shared Memory in C++
For shared memory, the code shared previously shows data written to a shared segment. The process needs to attach to the segment and could be implemented in two separate programs for effective demonstration.
By correctly managing access through semaphores, you ensure safe data sharing among processes.
Example: Using Message Queues in C++
As illustrated in previous examples, using message queues requires configuring a queue and sending messages. Extending this, you can introduce multi-process message handling to visualize how asynchronous communication works.
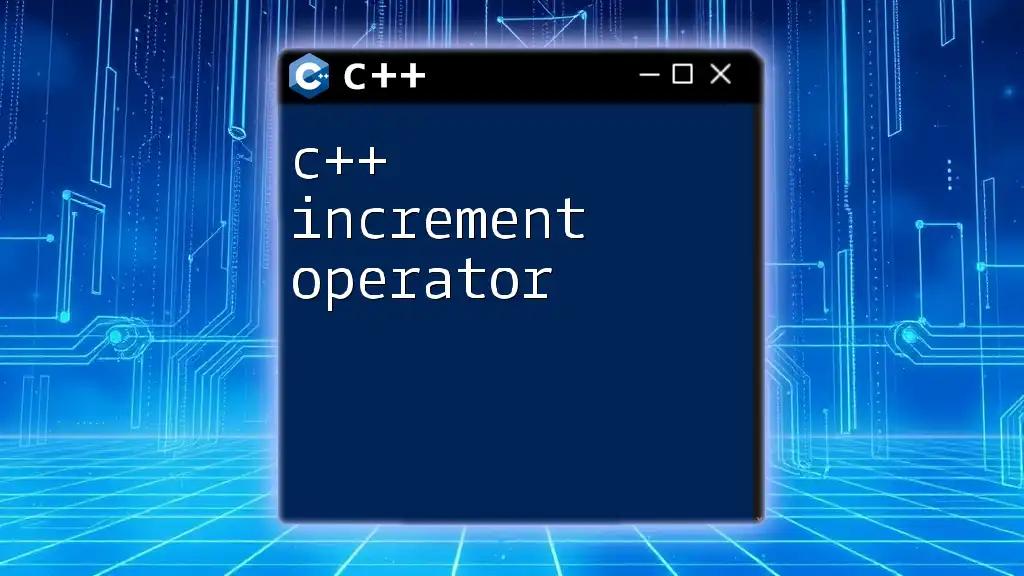
Common Pitfalls in C++ IPC
Synchronization Issues
With shared resources, data corruption can occur due to race conditions if proper synchronization is not maintained. Understanding and implementing synchronization mechanisms like semaphores and mutexes is key to avoiding these issues.
Error Handling
IPC can often fail due to various issues like memory allocation failures, permission errors, or data corruption. Always ensure you incorporate error checking in your IPC code to handle such cases gracefully.

Best Practices for IPC in C++
Performance Optimization
Consider the following techniques for optimizing IPC performance:
- Minimize Context Switching: Reduce the frequency of context switches by keeping communications efficient.
- Batch Communication: Send data in bulk rather than piecemeal, especially with message queues.
Code Modularization
To maintain clean and manageable IPC code, adopt a modular approach. This helps you in:
- Isolating IPC functionality from the main application logic.
- Reusing IPC components across different parts of your application or in different projects.
Documentation and Testing
Documenting your IPC code makes it easier for others (and your future self) to understand. Ensure that you include:
- Clear usage examples.
- Test cases that cover various IPC scenarios to validate that your implementation works as intended.
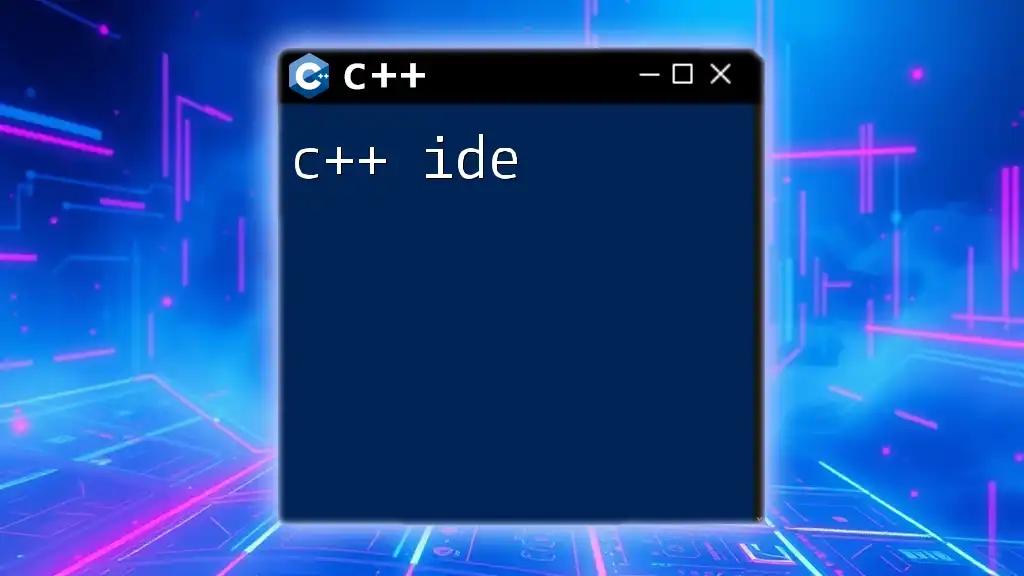
Conclusion
In summary, mastering C++ IPC is essential for creating efficient and responsive applications that require inter-process communications. By understanding the different IPC mechanisms, their advantages and disadvantages, and implementing best practices, you can enhance the performance and reliability of your applications significantly.
Whether you are developing a small utility or a large-scale system, leveraging the power of IPC can lead you to achieve complex tasks efficiently, enabling a smooth operating environment across processes.