The `pow` function in C++ is used to raise a number to the power of another number, and it can be found in the `<cmath>` library.
Here’s a simple example using the `pow` function:
#include <iostream>
#include <cmath>
int main() {
double base = 2.0;
double exponent = 3.0;
double result = pow(base, exponent);
std::cout << base << " raised to the power of " << exponent << " is " << result << std::endl;
return 0;
}
Understanding the pow Function
The `pow` function in C++ is a versatile tool used for performing power calculations. Its straightforward syntax is as follows:
double pow(double base, double exponent);
In this declaration, `base` is the number you're raising to a power, and `exponent` represents the power itself. The `pow` function will return a `double` value that is the result of the operation.
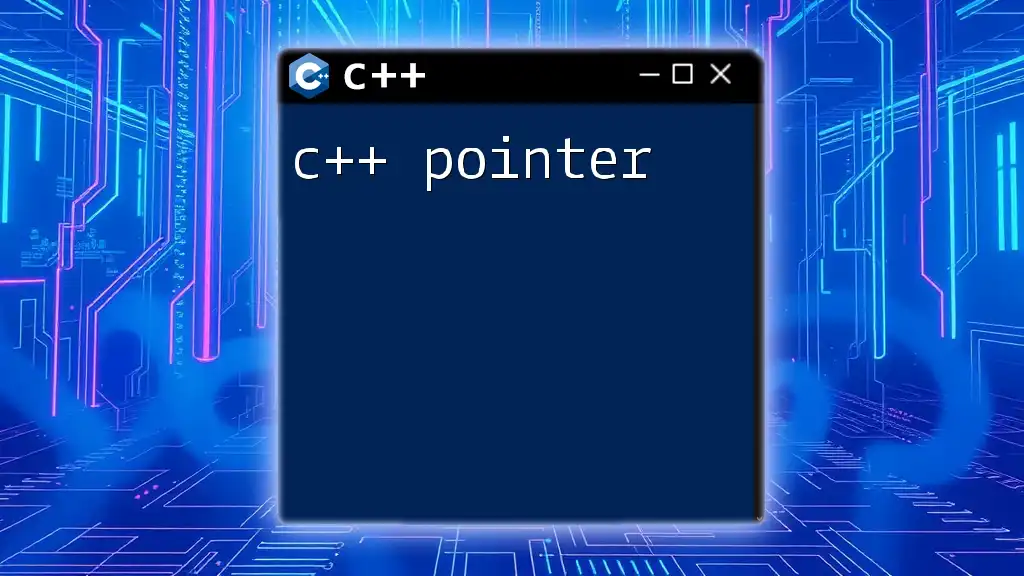
How to Use C++ pow
To illustrate the basic usage of the `pow` function, consider the following example where we raise a number to a power.
#include <iostream>
#include <cmath> // Required for pow function
int main() {
double result = pow(2, 3); // Calculates 2^3
std::cout << "2 raised to the power of 3 is: " << result << std::endl; // Outputs 8
return 0;
}
In this example, we calculate \(2^3\), which equals 8.
Using pow with Negative Exponents
The `pow` function also handles negative exponents seamlessly. This means that when you raise a number to a negative power, you are effectively calculating its reciprocal raised to the absolute value of the exponent:
int main() {
double result = pow(2, -2); // Calculates 2^-2
std::cout << "2 raised to the power of -2 is: " << result << std::endl; // Outputs 0.25
return 0;
}
Here, \(2^{-2}\) results in 0.25, demonstrating that `pow` can manage negative values effortlessly.
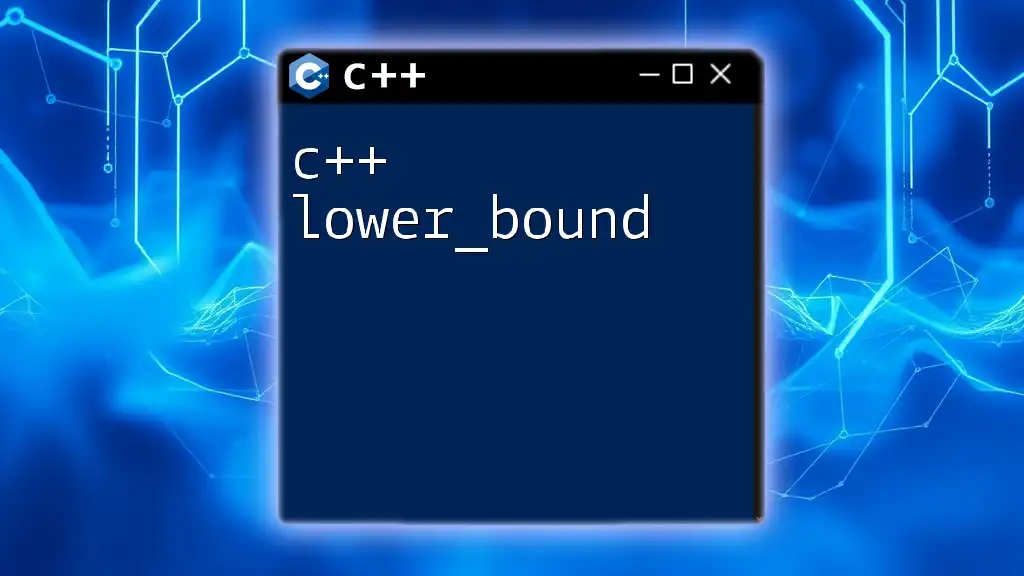
Advanced Usage of pow
pow with Floating-Point Numbers
The `pow` function can also be applied to floating-point numbers. Let's consider an example of raising a decimal to a power:
int main() {
double result = pow(3.5, 2); // Calculates 3.5^2
std::cout << "3.5 raised to the power of 2 is: " << result << std::endl; // Outputs 12.25
return 0;
}
In this instance, \(3.5^2\) gives 12.25, showcasing the function's versatility with decimal numbers.
pow with Large Numbers
When working with large numbers, the `pow` function remains robust. However, care must be taken due to the potential for overflow:
int main() {
double result = pow(10, 10); // Calculates 10^10
std::cout << "10 raised to the power of 10 is: " << result << std::endl; // Outputs 10000000000
return 0;
}
The output of \(10^{10}\) is 10,000,000,000. It's essential to be aware of the limitations of the `double` data type and the possibility of precision issues or overflow with exceedingly large results.
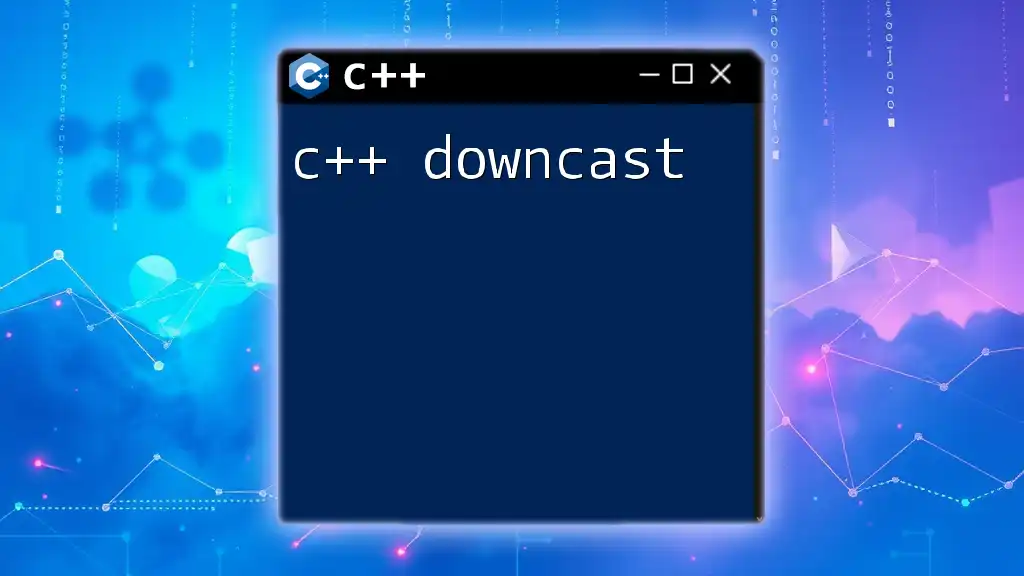
Error Handling in C++ pow
Understanding the potential errors that can arise with the `pow` function is crucial for robust programming. For example, raising negative numbers to fractional exponents can lead to math errors:
To guard against such issues, we can check for errors like this:
#include <iostream>
#include <cmath>
#include <cerrno>
int main() {
double base = -4;
double exponent = 0.5; // Attempting to calculate the square root of -4
double result = pow(base, exponent);
if (errno == EDOM) {
std::cout << "Math error: domain error in pow function" << std::endl;
} else {
std::cout << "-4 raised to the power of 0.5 is: " << result << std::endl;
}
return 0;
}
In this code, if an error occurs, we catch it using the `errno` global variable to prevent further issues in our application. Here, attempting to compute the square root of -4 results in a math error.
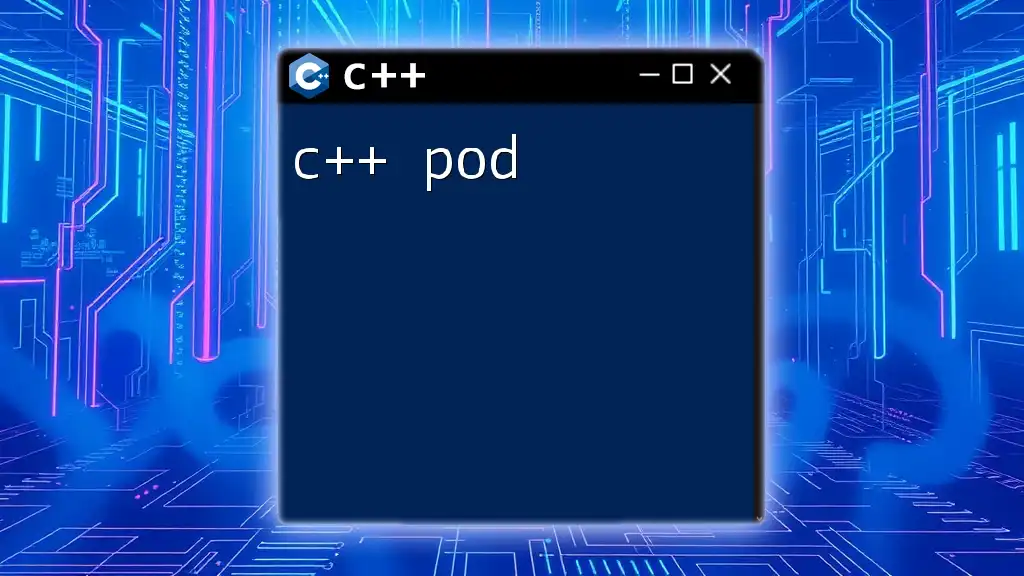
Power Functions in C++ Libraries
The `pow` function is just one of many functions provided in the `<cmath>` library, which contains a variety of mathematical functions. This library also includes complementary functions like `sqrt` for square roots, `exp` for exponentials, and various trigonometric operations. Utilizing these functions can simplify your code and enhance its functionality.
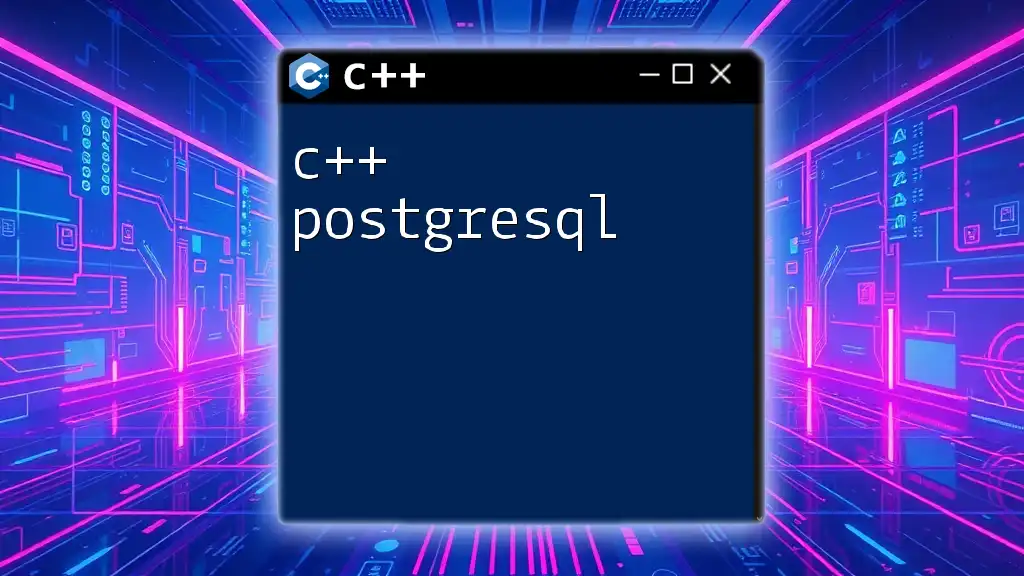
Real-Life Applications of C++ pow
The `pow` function plays a significant role across various fields, especially in scientific computing, simulations, and algorithm development. For instance, in physics simulations, `pow` can be employed to calculate potential energy or gravitational force based on various power laws. In data science, it may be instrumental in algorithms that rely on polynomial regression, classification, or clustering tasks.
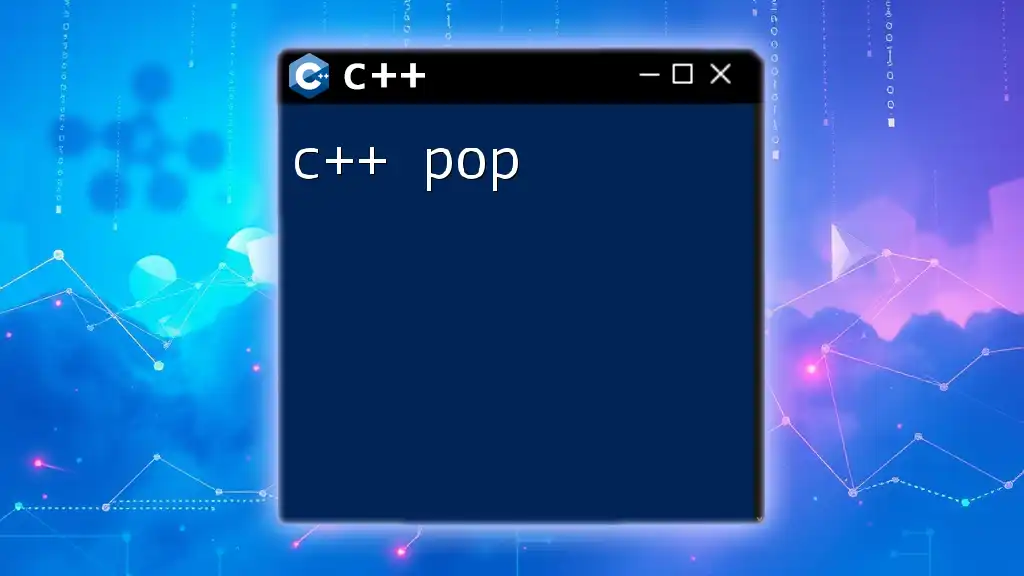
Tips for Optimizing Power Calculations
While the `pow` function is incredibly handy, there are scenarios where optimizing calculations can save time and resources. One common strategy involves exploiting properties of exponents. Instead of using `pow(base, n)`, we can sometimes reduce the number of multiplications needed:
- Example of Squaring: When computing powers that are power of two (like \(base^4\)), it can be calculated as \(pow(base, 2) * pow(base, 2)\), or more efficiently, \(pow(base, 2) * pow(base, 2)\).
By understanding and applying these properties, developers can enhance efficiency and reduce computational overhead.
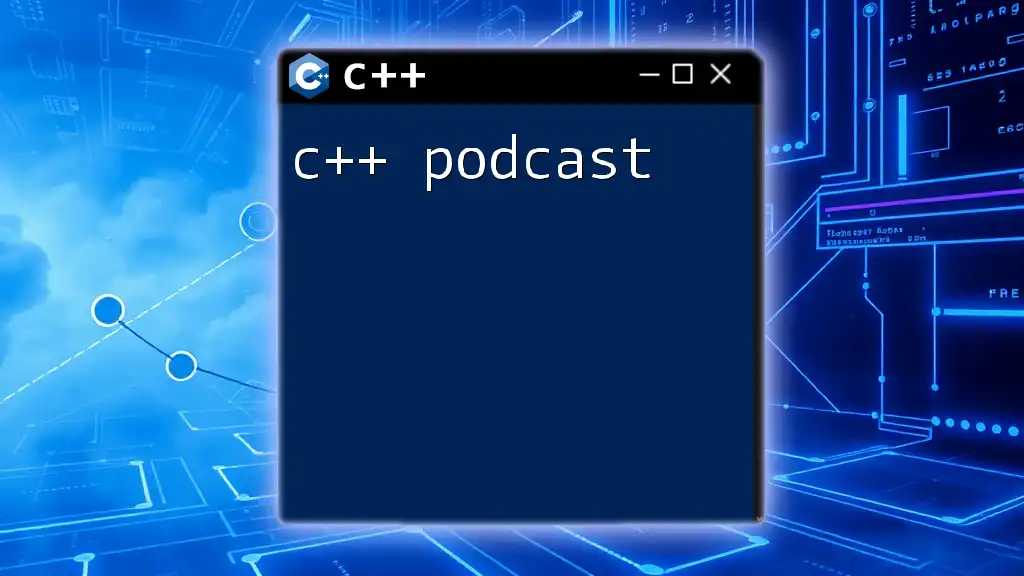
Conclusion
The `c++ pow` function is an indispensable tool in the programmer's toolkit, enabling quick and efficient power calculations. From basic uses to tackling complex mathematical operations, mastering `pow` can significantly improve your programming prowess.
Experimenting with various bases, exponents, and error handling scenarios can deepen your understanding and improve your coding skills. As you dive deeper into C++, consider exploring additional mathematical concepts and functions that can complement your knowledge of the `pow` function for a more comprehensive understanding of computational mathematics in programming.
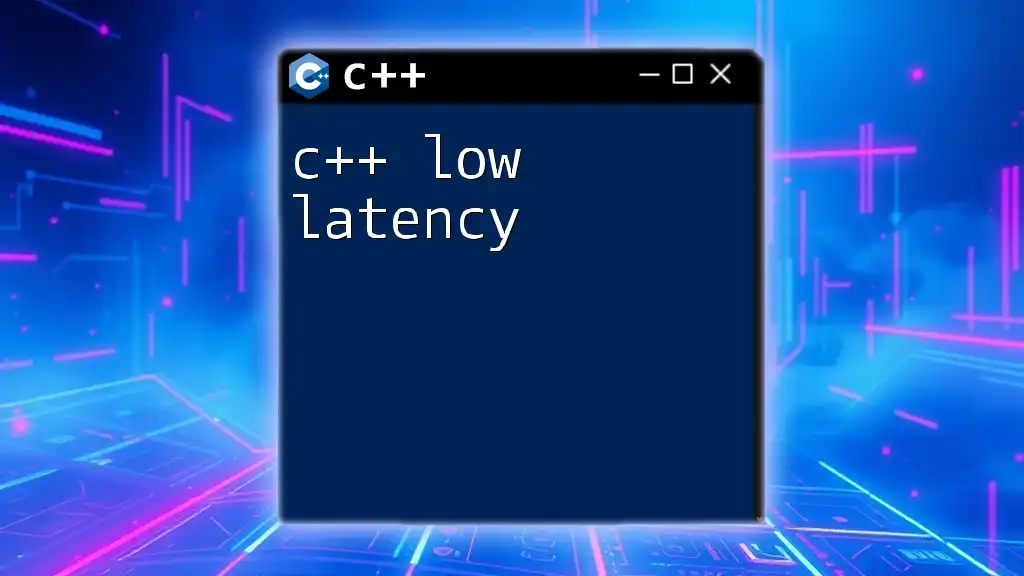
Additional Resources
For further exploration of function details, refer to the C++ Standard Library documentation. Books on advanced C++ programming or mathematical computations can provide more examples and strategies for effectively using the `pow` function and its associated mathematical techniques.
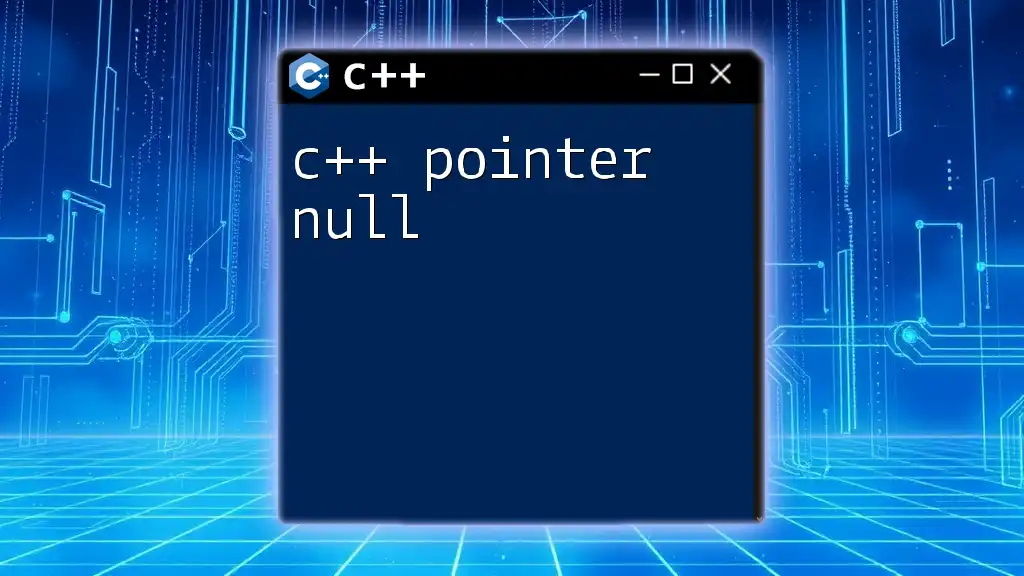
FAQs
In case you encounter common questions or run into issues while using `c++ pow`, remember to consult the documentation or community forums for troubleshooting tips and shared knowledge on best practices.