A C++ POD (Plain Old Data) is a type that is compatible with C's data structures, meaning it has no user-defined constructors, destructors, or virtual functions, making it suitable for low-level operations.
Here's a code snippet demonstrating a simple POD structure:
struct Point {
int x;
int y;
};
Understanding POD in C++
Definition of POD
Plain Old Data (POD) in C++ refers to data structures that are compatible with C-style data types. It implies a simple structure without non-trivial constructors, destructors, or copy assignment operators. POD types allow for predictable behavior in terms of memory layout and initialization, making them invaluable in low-level programming, systems interfaces, and high-performance scenarios.
Historical Context
The term "POD" has its roots in the early days of programming languages, when data structures were primarily simple and straightforward. As programming languages evolved, the introduction of concepts such as classes in C++ brought about complexity. Understanding POD provides a bridge between simple C-style programming and the complexities introduced by object-oriented paradigms in C++.
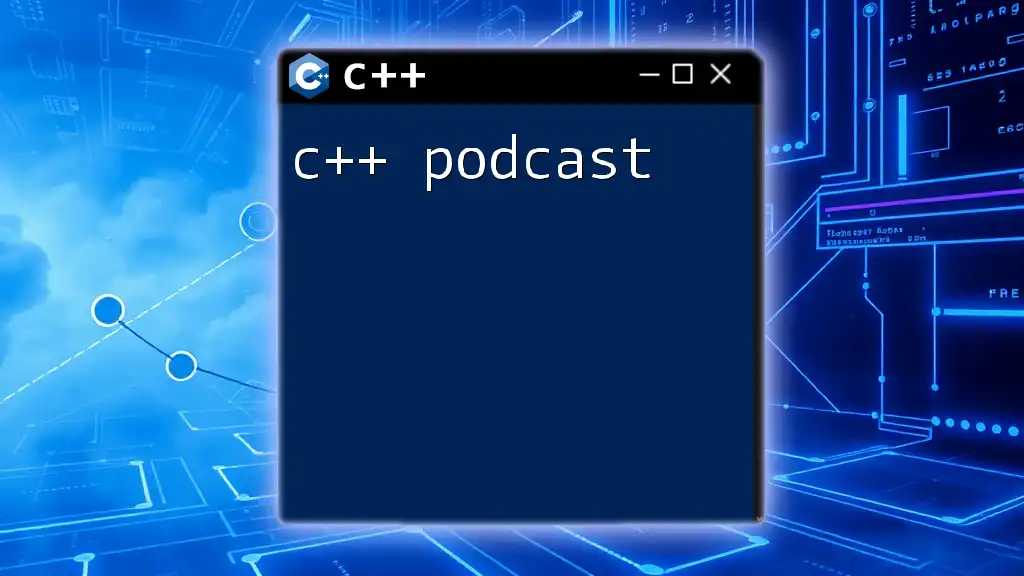
Characteristics of POD Types
Basic Features of POD
POD types in C++ exhibit two primary characteristics:
-
Triviality: A trivial type is a type that has no user-defined constructors, destructors, or copy/move assignment operators. This allows for a straightforward memory representation that can be copied with `memcpy` without any concern for virtual tables or non-trivial construction.
-
Standard Layout: A standard layout type adheres to certain restrictions ensuring that its memory layout is predictable. This means that data members must have types that are themselves standard layout types and the ordering of member variables should adhere to the order they are declared.
Differences Between POD and Non-POD Types
POD types differ significantly from non-POD types, which may include classes that manage resource lifetimes or implement complex behaviors. Non-POD types can have:
- Constructors and destructors that manage resources.
- Virtual functions introducing complexity in memory layout.
- Non-trivial copy operations, which complicate memory copy operations.
Understanding these differences is crucial for efficient programming and understanding the potential overhead that may be introduced by non-POD types.
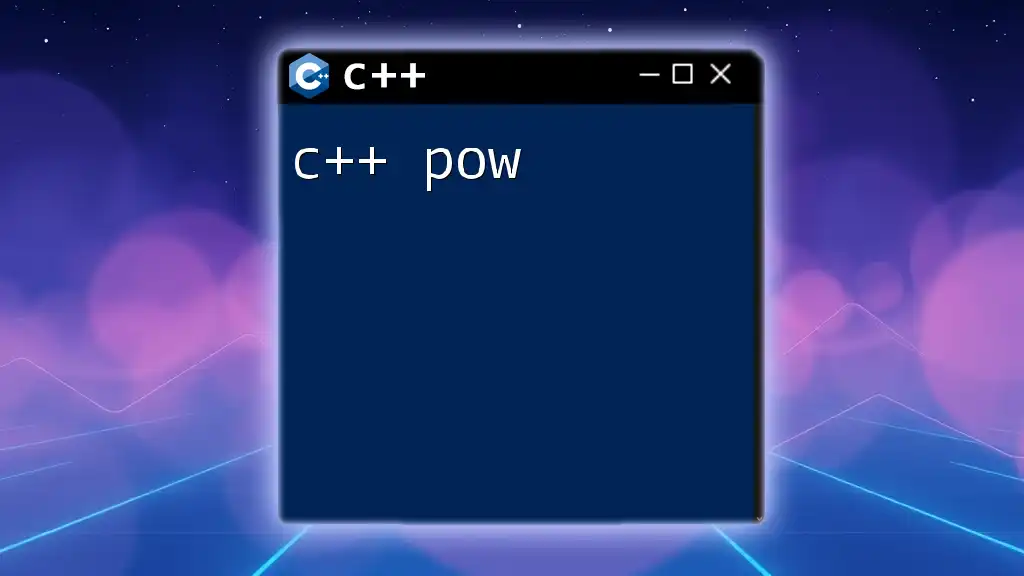
Creating POD Types
Defining POD Structures and Classes
To create a POD type, you must adhere to the rules of triviality and standard layout. Here’s an example of how to define a simple POD structure:
struct PODStruct {
int x;
double y;
};
This structure meets the POD requirements since it has no user-defined constructors and a standard layout, making it efficient for memory operations.
Nested POD Types
A nested POD type is special in that it encapsulates structured data types. You can define a POD structure that contains another POD structure:
struct NestedPOD {
struct InnerPOD {
char c;
long l;
};
int a;
InnerPOD inner;
};
In this example, both `NestedPOD` and `InnerPOD` are considered POD types, allowing for efficient memory access and manipulation.
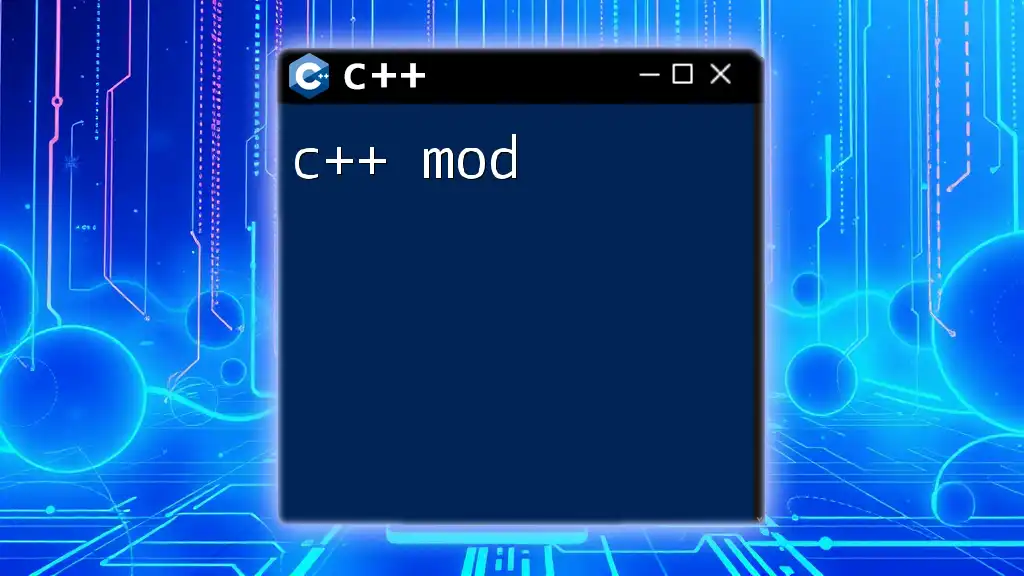
Best Practices for Using POD
When to Use POD Types
Choosing to use POD types is particularly beneficial in:
- Performance-critical applications: POD types can be manipulated more efficiently compared to non-POD types.
- Interfacing with C libraries or APIs: When working with C libraries, using POD types ensures compatibility.
- Serialization and deserialization: POD types can be easily serialized or deserialized with predictable behavior.
Potential Pitfalls
When using POD types, several pitfalls can occur:
- Memory Management Considerations: Don’t forget that POD types don’t manage resources by themselves. If your program uses dynamic memory, always remember to handle allocations properly.
- Alignment Issues: Be cautious of alignment, particularly in complex systems where hardware-specific data structures may impose additional constraints.
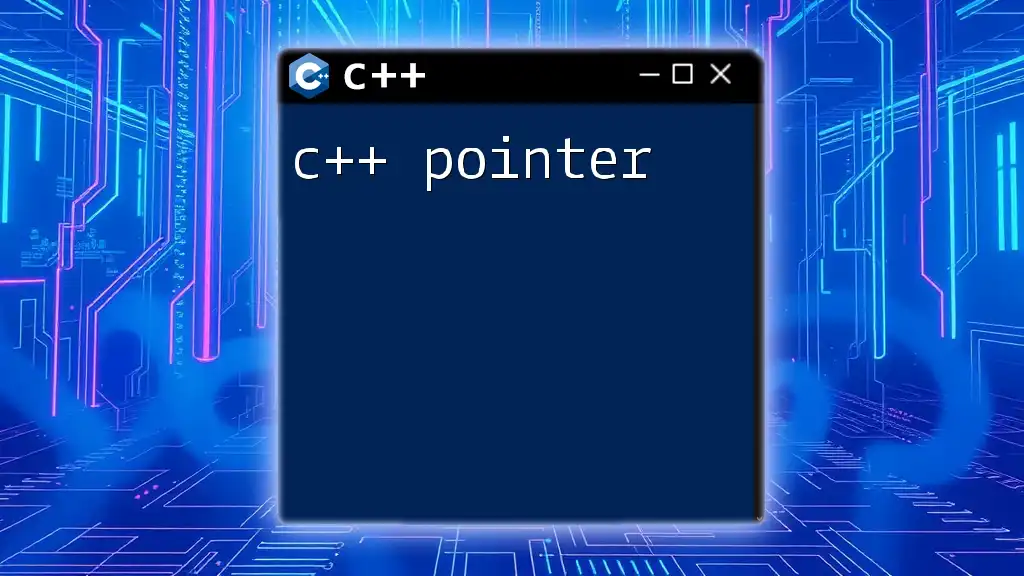
Advanced Concepts Related to POD
POD in Modern C++
As C++ evolves, new features enhance how we work with POD types. Modern C++ introduces constructs like `std::variant` and `std::tuple`, which can hold POD types, but often involve complexity not seen in simple struct declarations. Understanding their interactions with POD helps programmers maximize performance without introducing overhead.
C++17 and std::optional
The introduction of `std::optional` in C++17 allows for better handling of optional values while still interfacing with POD types. Unlike POD, `std::optional` manages the state of the contained type, which requires extra considerations during memory allocation and deallocation.
Using POD with Templates
POD types work seamlessly with templates—an essential feature of C++. Consider the following function template:
template <typename T>
void processPOD(T podType) {
static_assert(std::is_pod<T>::value, "T must be POD");
// process POD type
}
This function template guarantees that only POD types can be passed, leveraging `static_assert` for compile-time checks. This ensures the safety and predictability of operations performed on the passed type.
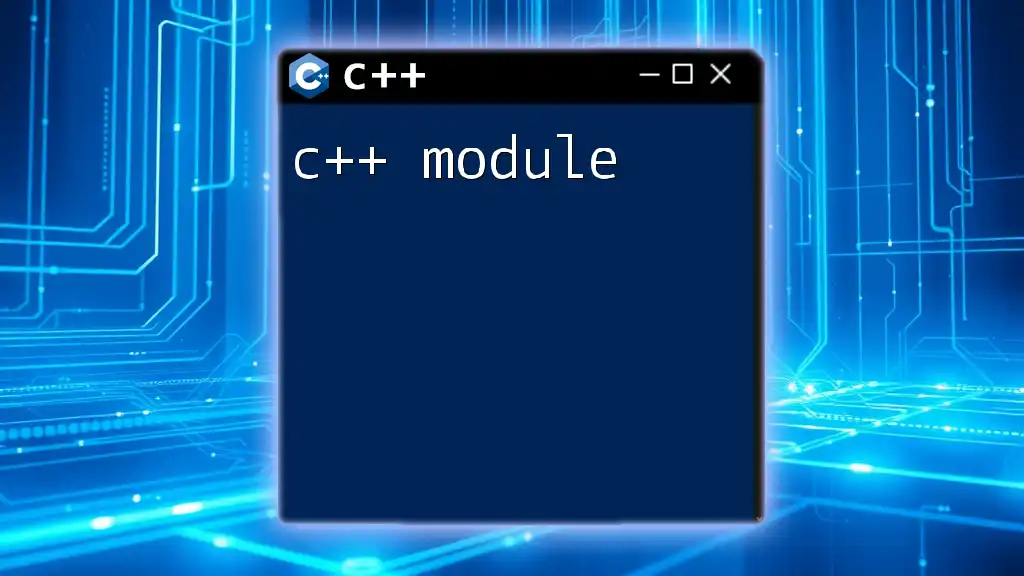
Conclusion
Recap of Key Points
Understanding POD types in C++ is essential for efficient system-level programming and interfacing with other programming languages. Their straightforward, predictable characteristics make them ideal for situations where performance is critical, and resource usage must be managed manually.
Further Reading and Resources
For those interested in deepening their understanding:
- Review the C++ Standard for intricate definitions and rules about POD types.
- Explore pertinent books like "Effective C++" by Scott Meyers for advanced memory management techniques.
- Look into online courses that cover modern C++ best practices.
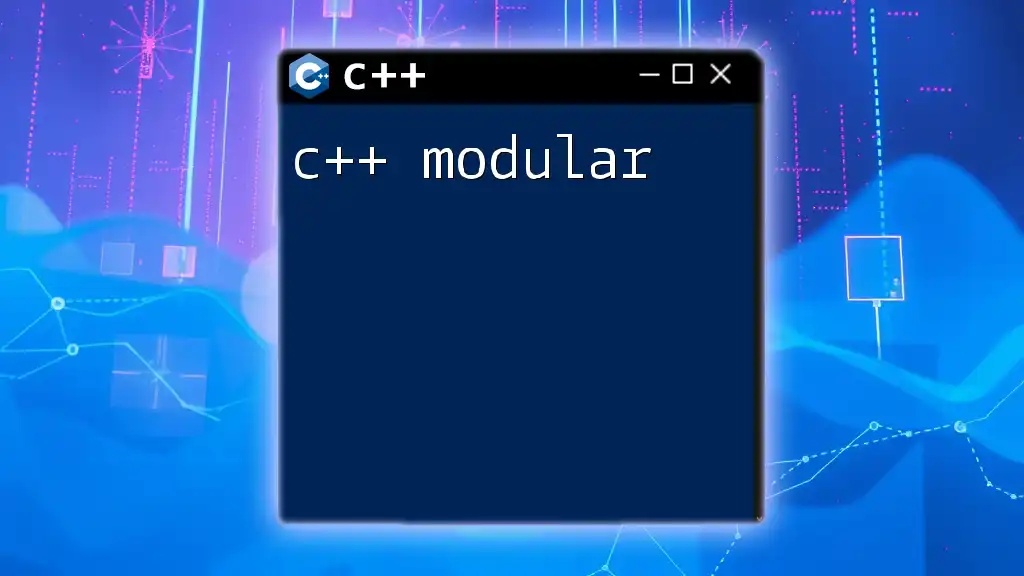
FAQs About C++ POD
Common Questions
One question often asked revolves around the applicability of PODs in modern design patterns. While PODs are essential for performance, newer designs can benefit from modern C++ features, encouraging a balance between simplicity and complexity.
Additional Examples and Scenarios
Real-world situations, such as writing drivers or working directly with hardware, often require mastery over POD data structures. A solid grasp of POD ensures efficient data handling, leading to optimized program behavior and resource management.