In C++, the `[[nodiscard]]` attribute warns programmers when the return value of a function should not be ignored, promoting better code practices.
Here's a code snippet demonstrating its usage:
#include <iostream>
[[nodiscard]] int computeValue() {
return 42;
}
int main() {
computeValue(); // This will trigger a warning if the return value is ignored
return 0;
}
Understanding c++ nodiscard
What is c++ nodiscard?
The `nodiscard` attribute in C++ is a feature introduced in C++17 that signifies the return value of a function should not be ignored. This means if a function is marked with `[[nodiscard]]`, the compiler will generate a warning when its return value is not captured or utilized. This feature underscores the importance of values returned from functions—encouraging developers to acknowledge and handle crucial data.
The importance of returning values
In many programming scenarios, return values are vital for the correctness of an application. For example, functions that perform calculations, validate inputs, or manage resources typically yield significant outputs. Ignoring these outputs can lead to logic errors or bugs in software, potentially cascading into larger issues. Therefore, `nodiscard` helps enforce a discipline of checking return values where necessary.
Context of using nodiscard in modern C++
With the evolution of C++, especially in its recent versions, the language has sought to enhance code safety and maintainability. Attributes like `nodiscard` serve as tools that guide developers towards safer coding practices, improving code readability and robustness.
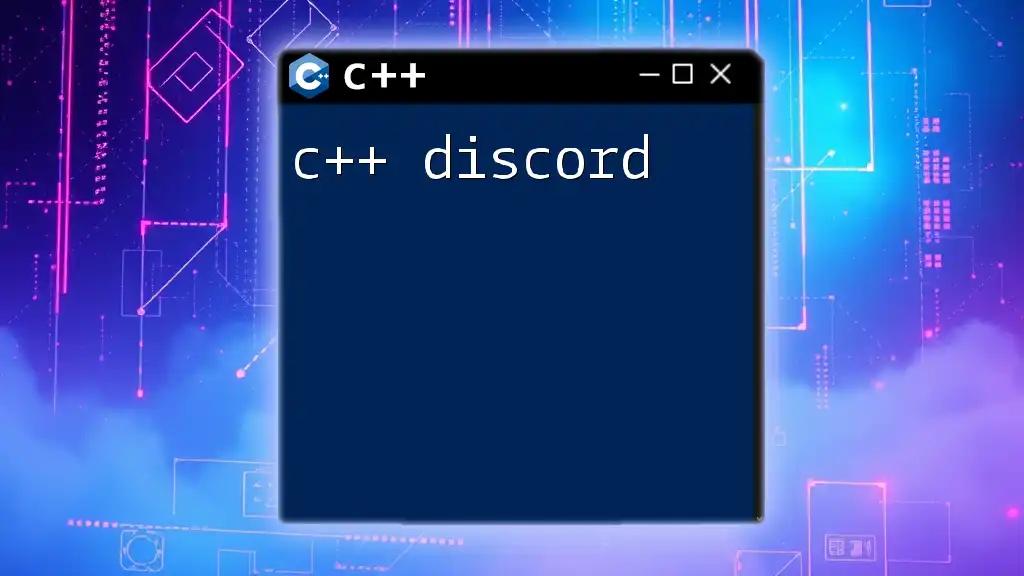
Understanding nodiscard Attribute
Definition and Purpose
What does nodiscard mean? In essence, `nodiscard` indicates that the return value of a function is significant and should be actively used. Failing to do so may result in unintended consequences in the program’s flow.
Purpose of using nodiscard in function design is multifaceted. It primarily serves to warn developers about ignored return values, thus minimizing the risk of errors caused by neglect. By marking functions with this attribute, developers can create cleaner, more understandable APIs, reducing cognitive load for anyone who interfaces with their code.
How nodiscard Works in C++
Syntax of nodiscard
To use the `nodiscard` attribute in a function, simply place `[[nodiscard]]` before the return type. Here’s how it looks:
[[nodiscard]] int calculateValue() {
return 42;
}
This succinct code snippet demonstrates a function that returns an integer. The `[[nodiscard]]` attribute indicates that the return value (42) should not be ignored.
Behavior when ignoring a nodiscard value
If a developer attempts to call a function marked as `[[nodiscard]]` and does not capture its return value, most modern compilers will emit a warning. This compiler feedback helps retain the integrity of the code, preventing silent failures.
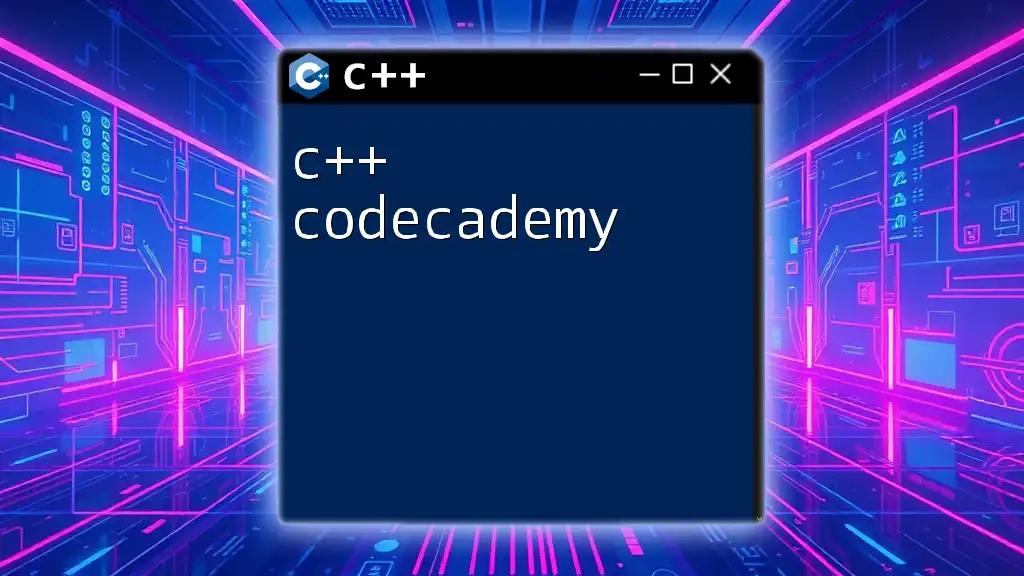
When to Use nodiscard
Scenarios for nodiscard Application
Functions that return important values are prime candidates for marking with `[[nodiscard]]`. For instance, functions like those validating inputs, performing calculations, or returning resource handles should significantly benefit from this practice. Ignoring such return values might lead to shallow errors or malfunctioning code.
Designing APIs for clarity and safety requires a careful thought process regarding function attributes. When developing libraries or large projects, ensuring that other developers are warned about critical functional outputs fosters a considerate design approach.
Best Practices for Using nodiscard
-
When to enforce return checking: Apply `nodiscard` judiciously where the return value is vital for subsequent logic. Avoid marking every function indiscriminately; instead, focus on functions whose outputs are indispensable.
-
Considerations for usability vs. strictness: The balance between being strict (ensuring all significant return values are checked) and usability (not overwhelming the developer with warnings) is crucial. Define clear guidelines for your projects on how to apply `nodiscard`.
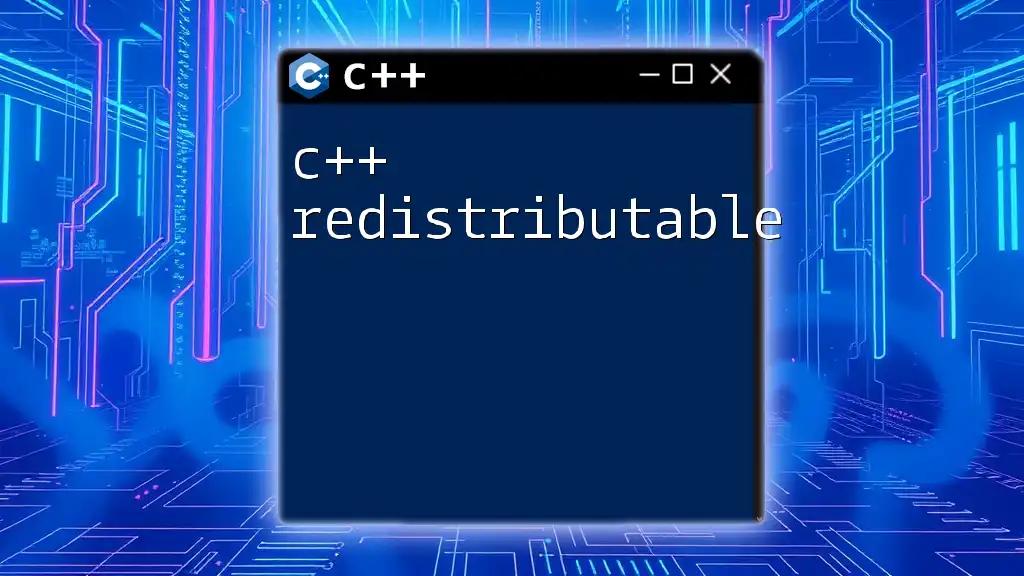
Practical Examples of c++ nodiscard
Example 1: Simple Function Implementation
Here’s a straightforward implementation of a function utilizing `[[nodiscard]]`:
[[nodiscard]] int getUserInput() {
int value;
std::cin >> value;
return value;
}
In this illustration, `getUserInput` is marked with `[[nodiscard]]`. It requests a user input, returning it as an integer. To handle the return value properly, users must activate checks:
int userValue = getUserInput(); // Proper usage
Ignoring the return value would lead to compiler warnings, alerting developers to handle the user’s input appropriately.
Example 2: Complex Use Case
class Widget {
public:
[[nodiscard]] std::string getName() const {
return "A Widget";
}
};
In this code, the method `getName()` inside the `Widget` class returns the name of the widget. Marking this method with `[[nodiscard]]` communicates that the output is essential for any operation using the class.
Attempting to use this method without capturing its return value would raise a compiler warning, highlighting potential misuse:
Widget widget;
widget.getName(); // This will generate a warning
Here, the warning suggests the developer should capture the return from `getName()`, ensuring the state of the program remains predictable and robust.

Compiler Support and Compatibility
Supported Compilers
Most popular compilers, including GCC, Clang, and MSVC, support `[[nodiscard]]`, typically in their versions that promote C++17 or later standards. This widespread compatibility underscores the attribute's importance in contemporary C++ development.
Limitations and Workarounds
Older C++ standards lack native support for `[[nodiscard]]`, which means developers working with legacy codebases might need to create workarounds.
Pointing to custom macros or defining coding guidelines can help maintain clarity in such cases. Writing clear documentation or providing alternative designs ensures that developers are still alerted to crucial return values even in the absence of `[[nodiscard]]`.
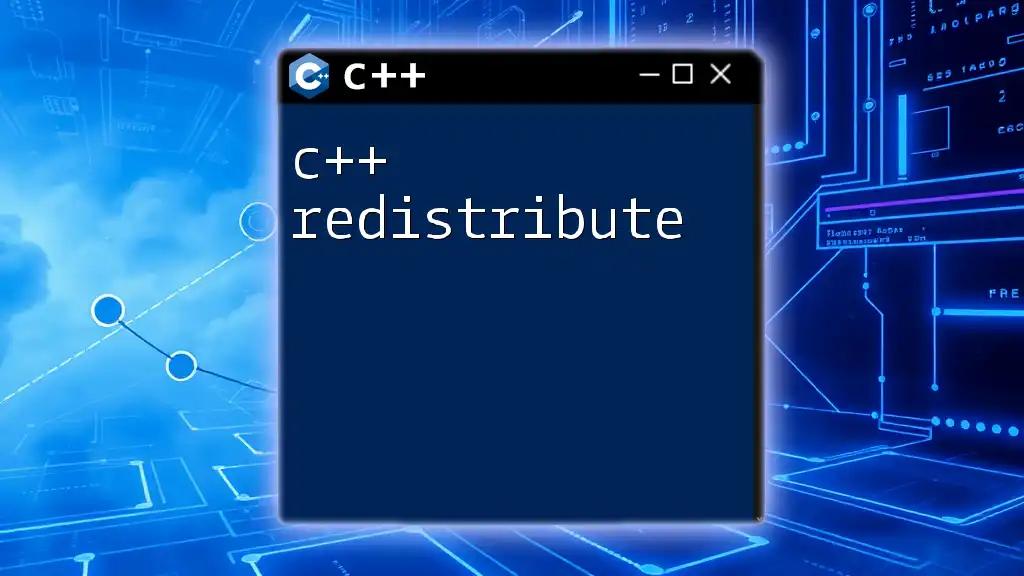
Conclusion
Utilizing `c++ nodiscard` offers developers a powerful method to guide their code towards safety and robustness. By emphasizing the importance of return values, and encouraging diligent coding practices, it minimizes the risk of bugs and improves software quality.
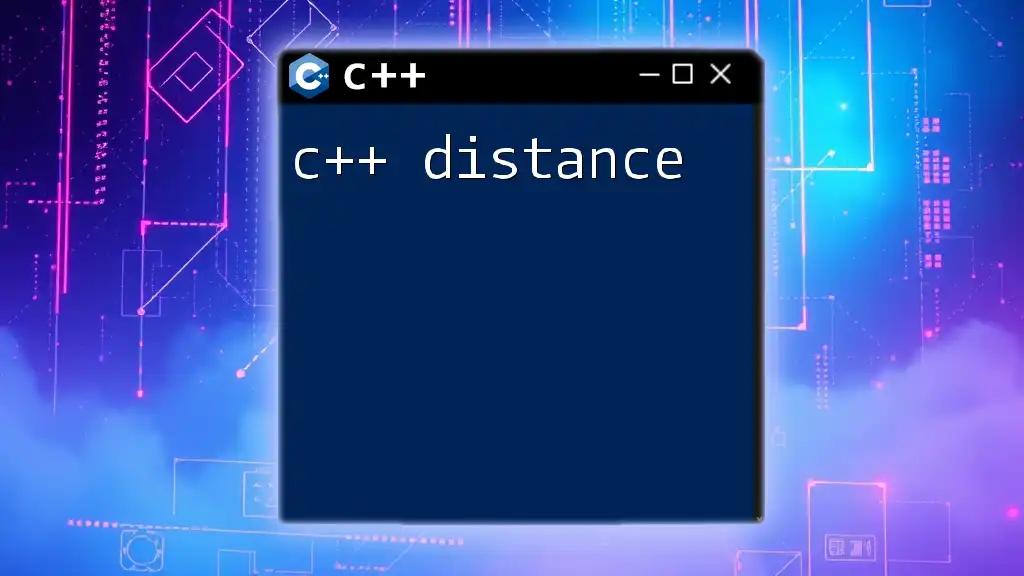
FAQs about c++ nodiscard
What happens if I ignore a nodiscard return value? The compiler will typically generate a warning, prompting you to address the overlooked return value.
Can I use nodiscard with custom types? Absolutely! The `[[nodiscard]]` attribute is compatible with any return type—whether it be built-in types, custom classes, or structures.
Is nodiscard useful in every project? While `nodiscard` can enhance code safety, each project is unique. Use cases should be evaluated based on the significance of return values and the potential impact on usability and readability.

Call to Action
Practice using `[[nodiscard]]` in your code! By applying this attribute where appropriate, you can foster better software design and increase maintainability. For further reading, explore additional C++ features or dive deeper into best practices for modern programming.