In C++, `std::forward` is a utility that allows you to preserve the value category (lvalue or rvalue) of a function argument when forwarding it to another function, which is particularly useful in template programming.
Here’s a simple code snippet demonstrating its use:
#include <iostream>
#include <utility>
template<typename T>
void process(T&& arg) {
handle(std::forward<T>(arg)); // Forwarding the argument
}
void handle(int& value) {
std::cout << "Lvalue: " << value << std::endl;
}
void handle(int&& value) {
std::cout << "Rvalue: " << value << std::endl;
}
int main() {
int x = 10;
process(x); // Calls handle(int&)
process(20); // Calls handle(int&&)
return 0;
}
What is the concept of forwarding in C++?
C++ forwarding refers to the practice of passing arguments to functions in a way that preserves their value categories, which ultimately enhances performance by avoiding unnecessary copies. Understanding the concept of forwarding is crucial for effective resource management and optimized code execution in C++.
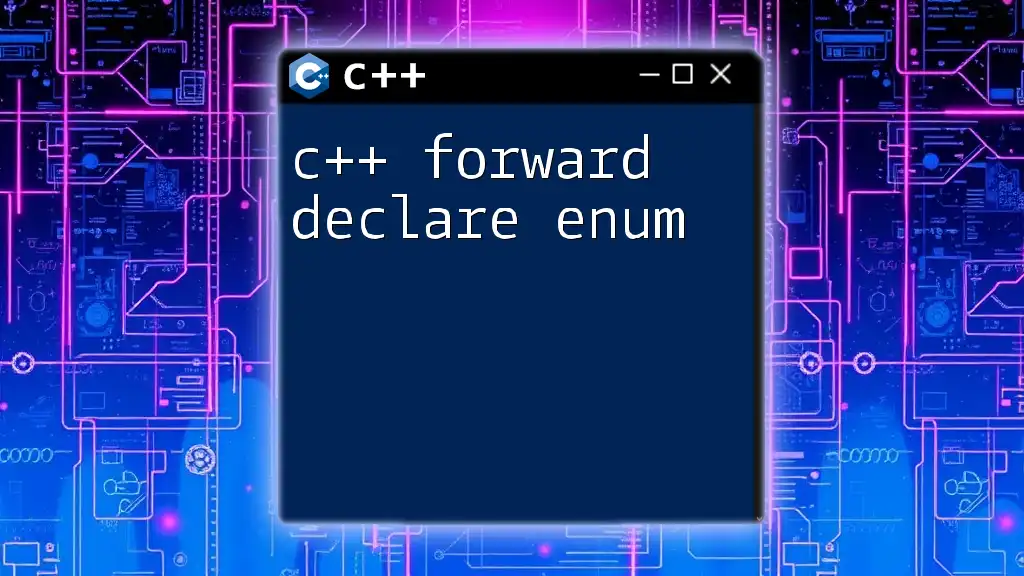
Why Use Forwarding in C++?
Using forwarding in C++ provides significant benefits:
- Efficiency: Forwarding allows the programmer to avoid unnecessary copies and moves, thus improving the efficiency of the program, especially for large objects.
- Resource Management: It helps manage resources effectively by preserving the original behavior of the entities being passed around.
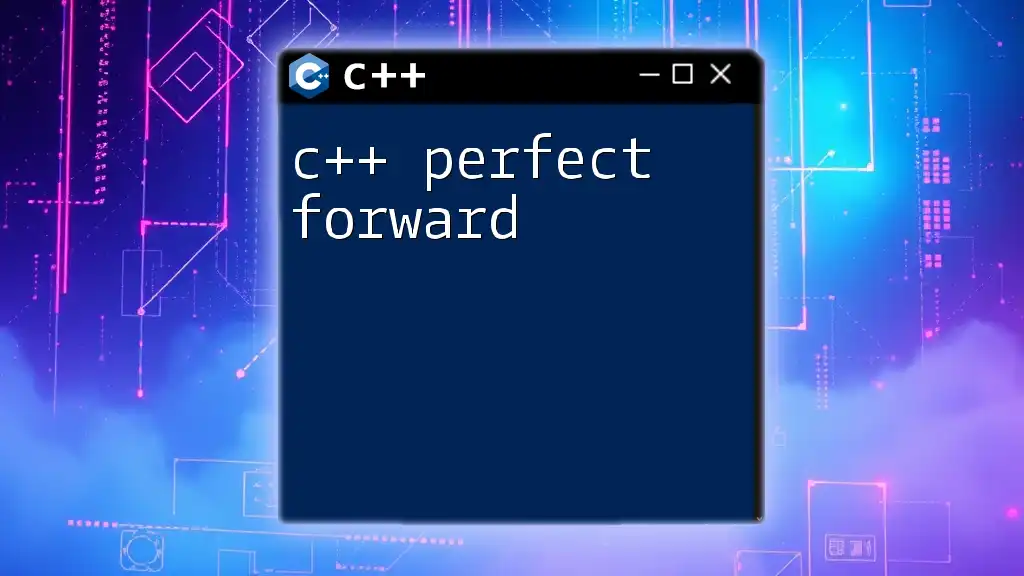
Understanding lvalue and rvalue
Distinction Between lvalue and rvalue
What is an lvalue?
An lvalue (locator value) represents an object that occupies some identifiable location in memory. In simpler terms, it’s anything that has an identifiable memory address. For instance:
int x = 10;
int &lvalueRef = x; // lvalueRef is an lvalue
In this example, `x` is an lvalue because it has a fixed address.
What is an rvalue?
An rvalue (read value) is a temporary object that does not occupy a specific location in memory. While lvalues can be assigned a value, rvalues cannot be assigned to a non-const reference. Example:
int&& rvalueRef = 20; // 20 is an rvalue
Here, `20` is an rvalue because it’s a temporary value that doesn’t have a persistent memory address.
Significance of lvalues and rvalues in Forwarding
Understanding lvalues and rvalues is critical in forwarding because it allows the developer to pass parameters correctly, ensuring optimal performance. In forwarding, it becomes essential to distinguish between the two to maintain efficiency in function calls.
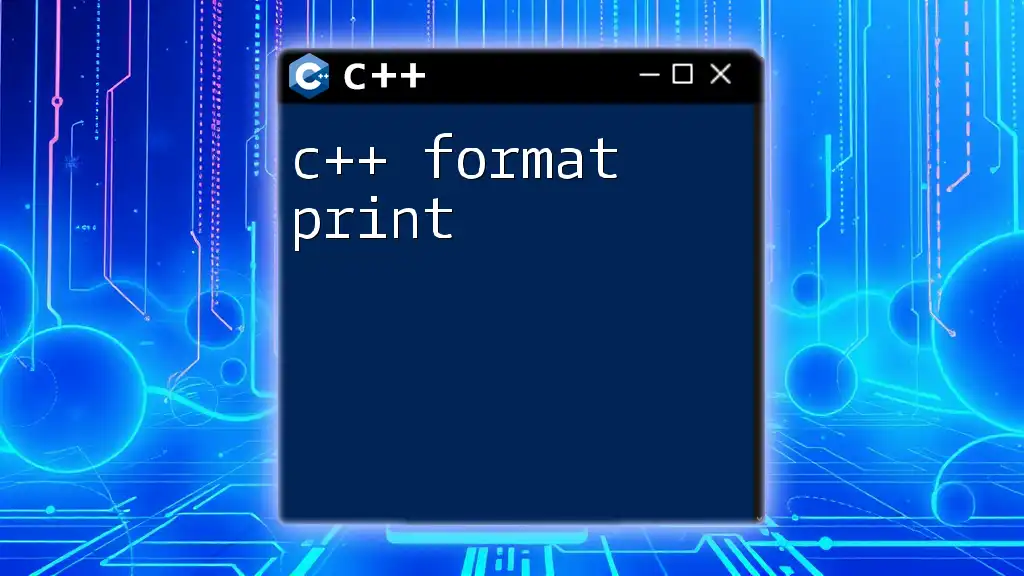
What is std::forward?
Overview of std::forward in C++
std::forward is a utility function that was introduced in C++11 as part of the standard library. Its primary purpose is to preserve the value category of an argument when forwarding it to another function.
How std::forward Works
The mechanism of `std::forward` is deeply tied to template parameters and type deduction. It enables perfect forwarding by ensuring that an lvalue remains an lvalue, and an rvalue remains an rvalue when passed through functions.
Example of how `std::forward` is used:
template<typename T>
void process(T&& arg) {
handle(std::forward<T>(arg)); // Preserves the value category
}
In this example, `arg` can be either an lvalue or rvalue, and `std::forward<T>(arg)` will ensure that the original type is retained.
Usage Scenarios for std::forward
Utilizing std::forward in function templates
When writing function templates, using `std::forward` can be extremely beneficial. Consider the following code:
#include <iostream>
template<typename T>
void display(T&& value) {
std::cout << std::forward<T>(value) << std::endl;
}
int main() {
int x = 42;
display(x); // Forwarding lvalue
display(100); // Forwarding rvalue
return 0;
}
Here, calling `display(x)` will correctly pass `x` as an lvalue, while `display(100)` will pass `100` as an rvalue.
Combining std::forward with std::move
`std::move` transforms an lvalue into an rvalue. This can work hand-in-hand with `std::forward` to pass along objects. Consider this example:
#include <iostream>
#include <utility>
class MyClass {
public:
MyClass(int data) : data(data) {}
void show() const {
std::cout << "Data: " << data << std::endl;
}
private:
int data;
};
template<typename T>
void processObject(T&& obj) {
auto newObj = std::forward<T>(obj); // Forwards correctly based on T's type
newObj.show();
}
int main() {
MyClass obj(10);
processObject(std::move(obj)); // Pass obj as rvalue
return 0;
}
In this example, `processObject` uses `std::forward` to maintain the value category of `obj`.
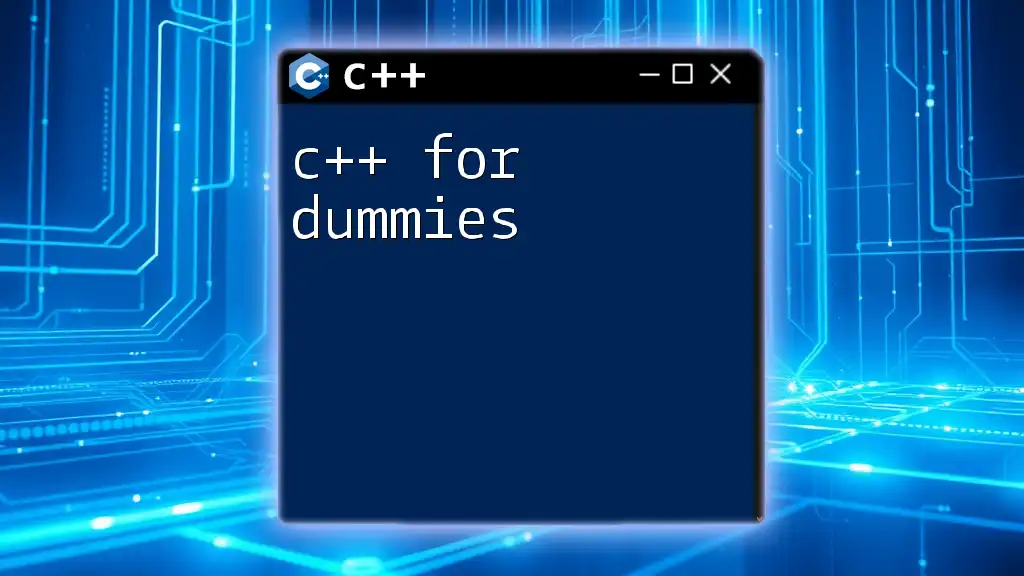
Implementing Forwarding in C++
Creating Functions with Forwarding
To design a function that implements forwarding, follow a simple structure. Here's a step-by-step approach to creating a forwarding function:
- Define a template function that accepts a universal reference.
- Use `std::forward` to preserve the value category when calling another function.
Code example:
template<typename T>
void forwardingFunction(T&& arg) {
// Process with forwarding
anotherFunction(std::forward<T>(arg));
}
void anotherFunction(int&& x) {
std::cout << "Rvalue forwarded: " << x << std::endl;
}
Using Forwarding with Classes
Forwarding can also be effectively used within class constructors. This is often referred to as perfect forwarding.
class Wrapper {
public:
template<typename T>
Wrapper(T&& obj) : object(std::forward<T>(obj)) {}
void display() const {
object.show();
}
private:
MyClass object; // Assume MyClass is defined elsewhere
};
In this example, the constructor of `Wrapper` captures any type of object and forwards it correctly, preserving its value category.
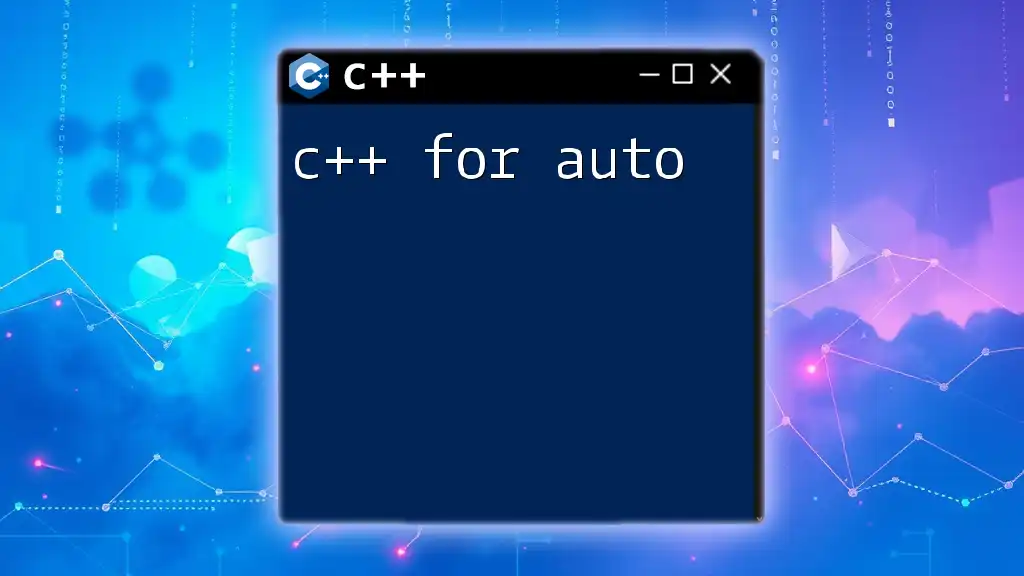
Best Practices for Using Forwarding in C++
When to Use Forwarding
Forwarding is particularly beneficial in scenarios such as:
- Templates: When creating generic functions that can handle different types efficiently.
- Constructor Initializers: When you want to initialize a class member with perfect forwarding.
- Performance-Critical Code: To avoid unnecessary copies in high-performance applications.
Common Pitfalls to Avoid
When using `std::forward`, be cautious of the following pitfalls:
- Incorrect Use of std::forward: Avoid using `std::forward` on a non-deduced context, which can lead to unexpected behavior.
- Misunderstanding Value Categories: It's essential to have a strong grasp of lvalues and rvalues to prevent mistakes in forwarding logic.
Here’s an example of a common mistake:
void exampleFunction(int& arg) {
// Misusing std::forward on a non-reference
// std::forward<int>(arg); // Incorrect: arg is lvalue
}
Always ensure that you use `std::forward` appropriately to maintain the expected type.
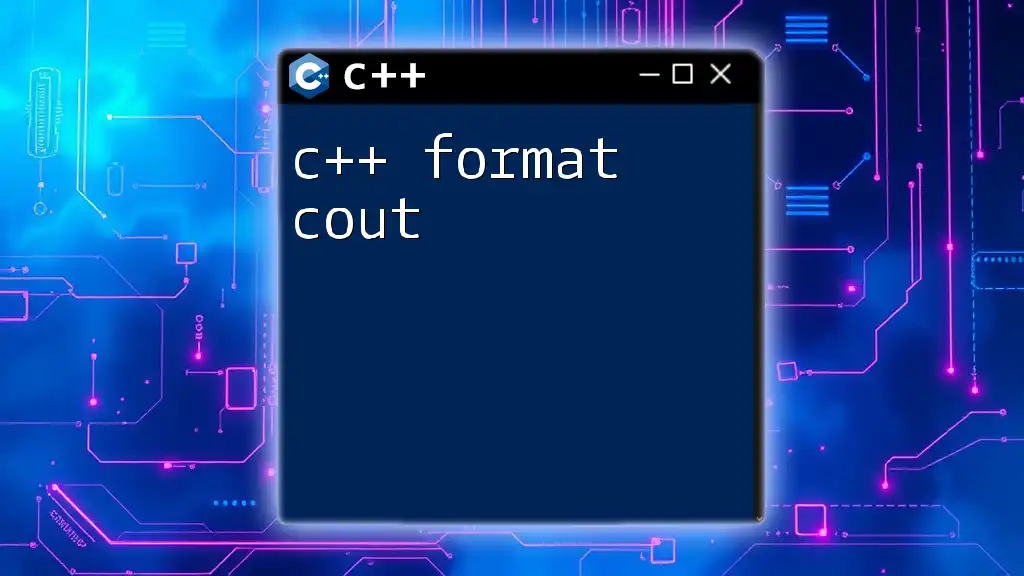
Conclusion
In summary, C++ forwarding is a powerful feature that enables efficient parameter passing by preserving value categories. Utilizing `std::forward`, you can design functions and constructors that are both flexible and performance-optimized. Remember to practice with forwarding in your own C++ projects to grasp its full potential!
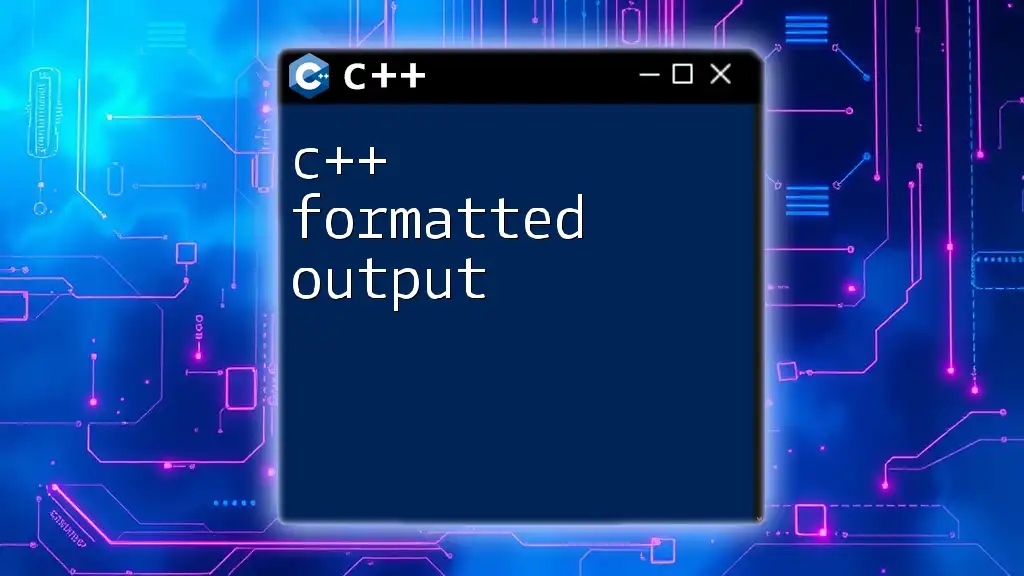
Additional Resources
To further enhance your understanding of C++ forwarding, consider exploring these additional resources:
- Books on C++ programming.
- Online tutorials and documentation specific to C++11 and later standards.
- Community forums and coding practice sites to engage with other C++ developers and refine your skills.