The `sockaddr` structure in C++ is used to define a socket address that includes address-family information as well as the actual IP address and port number for network communication.
#include <sys/socket.h>
#include <netinet/in.h>
#include <arpa/inet.h>
struct sockaddr_in addr;
addr.sin_family = AF_INET; // Address family
addr.sin_port = htons(8080); // Port number
addr.sin_addr.s_addr = inet_addr("192.168.1.1"); // IP address
Understanding the sockaddr Structure
Definition of sockaddr
The sockaddr structure is a fundamental component in C++ networking, primarily used to define an endpoint address for socket communication. It serves as a generic container for various types of socket addresses, making it an essential part of socket programming.
The basic definition of the sockaddr structure looks like this:
struct sockaddr {
unsigned short sa_family; // address family
char sa_data[14]; // protocol address
};
The sa_family field specifies the address family, which indicates the type of addresses that the socket can communicate with (e.g., IPv4, IPv6). The sa_data field contains the actual address data.
Common sockaddr Variants
sockaddr_in
The sockaddr_in structure is a specialized version of sockaddr used for IPv4 addresses. Here’s what the structure typically looks like:
struct sockaddr_in {
short sin_family; // address family (AF_INET)
unsigned short sin_port; // port number (in network byte order)
struct in_addr sin_addr; // internet address
};
- Fields of sockaddr_in:
- sin_family: Set to AF_INET for IPv4.
- sin_port: This should be converted to network byte order using `htons()`.
- sin_addr: Represents the IP address, set using functions like `inet_pton()`.
Example usage of sockaddr_in to create a server socket:
struct sockaddr_in server_addr;
server_addr.sin_family = AF_INET;
server_addr.sin_port = htons(8080);
server_addr.sin_addr.s_addr = INADDR_ANY;
sockaddr_in6
The sockaddr_in6 structure is the counterpart for IPv6 addresses, providing an extended address format for modern network applications:
struct sockaddr_in6 {
short sin6_family; // address family (AF_INET6)
unsigned short sin6_port; // port number (in network byte order)
uint32_t sin6_flowinfo; // IPv6 flow information
struct in6_addr sin6_addr; // IPv6 address
uint32_t sin6_scope_id; // scope ID
};
- Fields of sockaddr_in6:
- sin6_family: Set to AF_INET6 for IPv6.
- sin6_port: Again converted to network byte order with `htons()`.
- sin6_addr: Contains the IPv6 address.
Example usage of sockaddr_in6:
struct sockaddr_in6 server_addr6;
server_addr6.sin6_family = AF_INET6;
server_addr6.sin6_port = htons(8080);
inet_pton(AF_INET6, "::1", &server_addr6.sin6_addr);
sockaddr_un
The sockaddr_un structure is used for UNIX domain sockets, facilitating inter-process communication on the same host:
struct sockaddr_un {
sa_family_t sun_family; // address family (AF_UNIX)
char sun_path[108]; // path name
};
- Fields of sockaddr_un:
- sun_family: Set to AF_UNIX.
- sun_path: The path to the socket file.
Example usage of sockaddr_un:
struct sockaddr_un unix_addr;
unix_addr.sun_family = AF_UNIX;
strncpy(unix_addr.sun_path, "/tmp/my_socket", sizeof(unix_addr.sun_path) - 1);
Purpose of sockaddr
The primary purpose of the sockaddr structure is to facilitate binding, connecting, and listening for sockets in various protocols, notably IPv4 and IPv6. Understanding how to utilize the sockaddr structure across different variations is vital for establishing efficient communication channels in network programming.
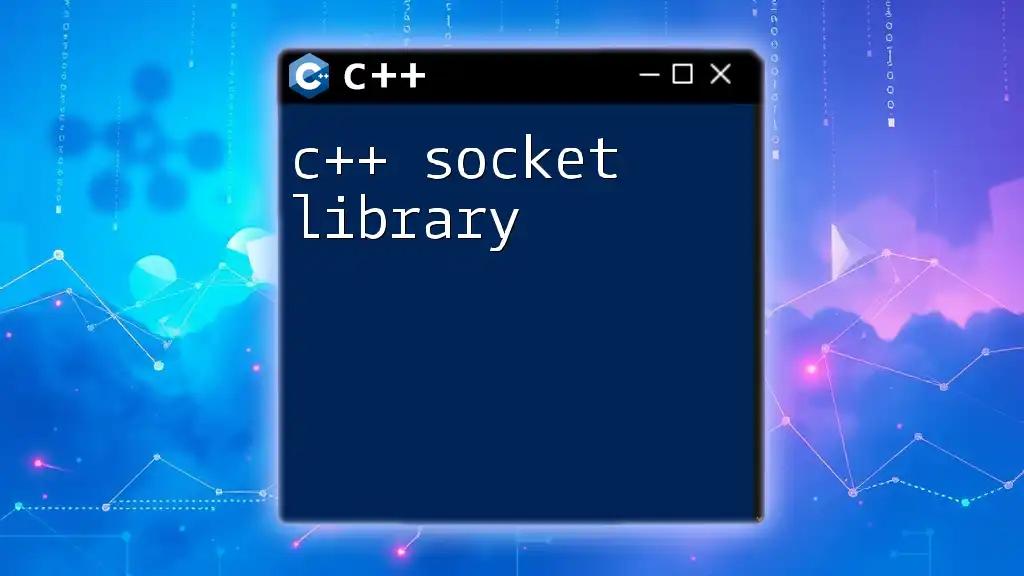
Working with sockaddr in C++
Including Necessary Headers
Before working with sockets in C++, you need to include the appropriate headers that provide socket functionality. The following is a typical inclusion for socket programming:
#include <sys/socket.h>
#include <netinet/in.h>
#include <arpa/inet.h>
#include <unistd.h>
#include <cstring>
These headers provide declarations for the socket API, including structures like sockaddr and other crucial network functions.
Creating and Using Socket
Creating a Socket
To initiate socket programming, the first step is to create a socket:
int sockfd = socket(AF_INET, SOCK_STREAM, 0);
This creates a socket that uses the IPv4 protocol (AF_INET) and supports TCP (SOCK_STREAM).
Binding to an Address
After creating a socket, you typically bind it to a specific address and port. This step is crucial as it informs the operating system which address and port the socket should listen to:
struct sockaddr_in server_addr;
server_addr.sin_family = AF_INET;
server_addr.sin_port = htons(12345);
server_addr.sin_addr.s_addr = INADDR_ANY;
bind(sockfd, (struct sockaddr*)&server_addr, sizeof(server_addr));
In this code snippet, the socket is bound to all available network interfaces on port 12345.
Listening for Connections
In the case of a TCP server, the next step is to listen for incoming connections:
listen(sockfd, 5);
The number 5 indicates the maximum number of queued connections.
Accepting Connections
Once the server is listening, it can accept incoming connections. This sets up a new socket for interacting with the client:
struct sockaddr_in client_addr;
socklen_t client_len = sizeof(client_addr);
int newsockfd = accept(sockfd, (struct sockaddr*)&client_addr, &client_len);
In this example, `accept()` blocks until a client connects, at which point it returns a new socket descriptor.
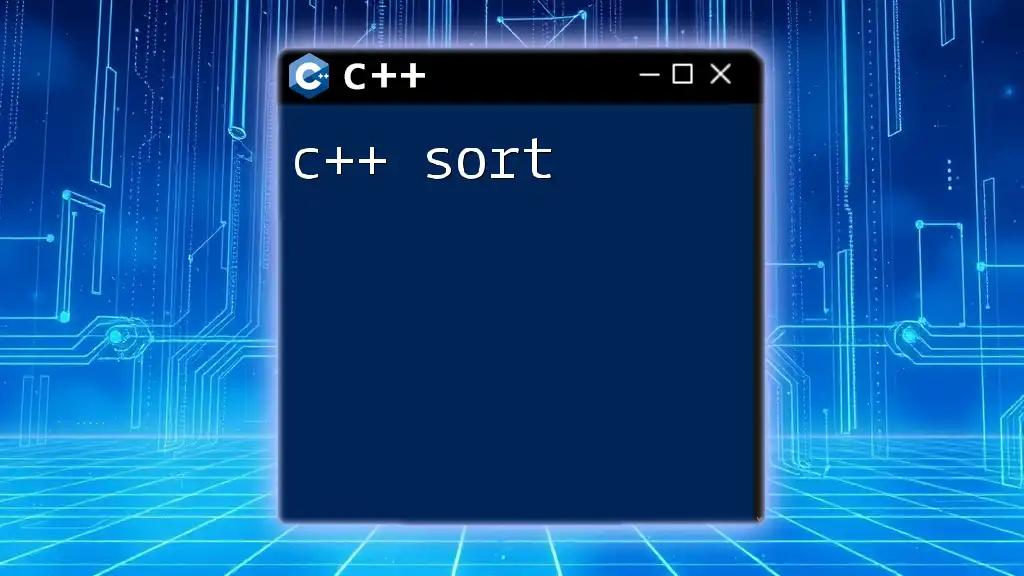
Handling sockaddr Safely
Type Safety with sockaddr
When dealing with the sockaddr and its variants, typecasting is essential. You often need to use casting to convert from one type to another when passing around the sockaddr structure. For example, when binding or connecting, you should cast your specific sockaddr structure to a pointer of type sockaddr:
bind(sockfd, (struct sockaddr*)&server_addr, sizeof(server_addr));
This type safety is crucial to ensure the correct handling of socket structures.
Error Handling
Error handling is a vital part of networking. Common issues like failure to create a socket, bind errors, or connection issues will require appropriate checks. Utilize `errno` to diagnose issues:
if (sockfd < 0) {
perror("Error creating socket");
}
Implementing robust error handling will prevent many runtime problems and improve the reliability of your application.

Practical Example: A Simple TCP Echo Server
Setting Up the Environment
Before you can write and run an echo server, ensure you have the development tools installed, such as a C++ compiler and the necessary libraries for socket functionality.
Complete Code Example
Here is a simple TCP echo server that uses the sockaddr structure:
#include <iostream>
#include <sys/socket.h>
#include <netinet/in.h>
#include <unistd.h>
#include <cstring>
int main() {
int sockfd, newsockfd;
struct sockaddr_in server_addr, client_addr;
socklen_t client_len = sizeof(client_addr);
char buffer[256];
sockfd = socket(AF_INET, SOCK_STREAM, 0);
server_addr.sin_family = AF_INET;
server_addr.sin_port = htons(12345);
server_addr.sin_addr.s_addr = INADDR_ANY;
bind(sockfd, (struct sockaddr*)&server_addr, sizeof(server_addr));
listen(sockfd, 5);
newsockfd = accept(sockfd, (struct sockaddr*)&client_addr, &client_len);
while (true) {
int n = read(newsockfd, buffer, 255);
write(newsockfd, buffer, n);
}
close(newsockfd);
close(sockfd);
return 0;
}
The echo server listens on port 12345 and echoes back any message received from a client. This example demonstrates how to implement basic functionality using sockaddr structures seamlessly.

Conclusion
In summary, understanding the c++ sockaddr structure is imperative for any socket programming endeavor. This comprehensive guide has walked you through its various forms, utilization, and practical examples. By mastering sockaddr, you pave the way for robust network communication solutions.
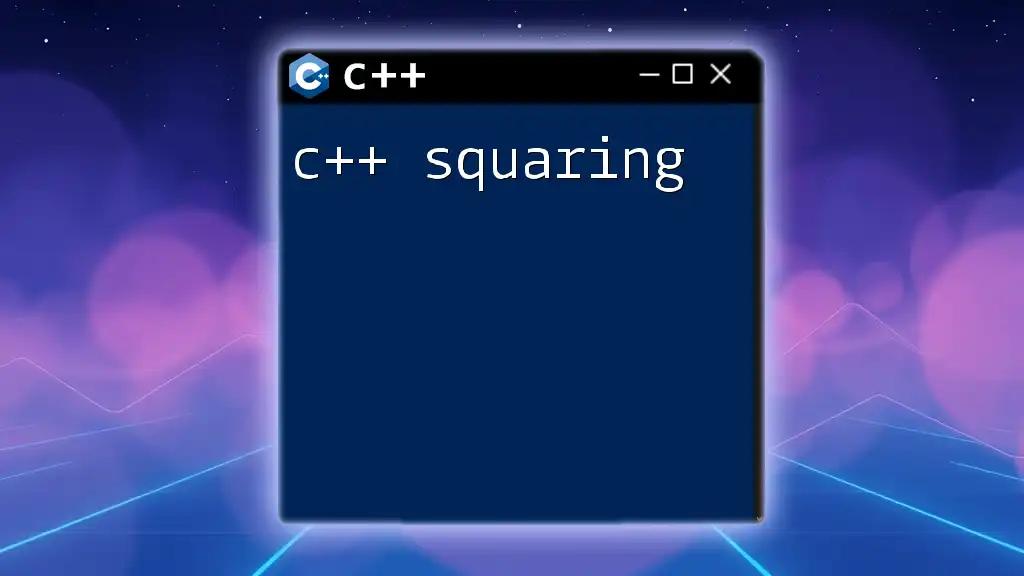
Further Resources
For those looking to deepen their understanding of C++ socket programming, several excellent resources are suggested. Comprehensive books, online courses, and documentation can provide additional insights. Also, consider engaging with community forums and discussion groups for real-world advice and best practices.

FAQs
It is normal to have questions when venturing into socket programming. Common inquiries often revolve around misconceptions about sockaddr usage, typical errors during socket operations, and best practices for implementing networked applications in C++. Always refer back to documentation and community advice when in doubt, as the landscape of networking can quickly evolve.