C++ backend development involves utilizing C++ to create server-side applications that handle data processing and business logic efficiently. Here's a simple example of a C++ backend server using the Boost.Beast library:
#include <boost/asio.hpp>
#include <boost/beast.hpp>
namespace beast = boost::beast;
namespace http = beast::http;
namespace net = boost::asio;
using tcp = net::ip::tcp;
void start_server() {
net::io_context io_context{};
tcp::acceptor acceptor{io_context, {tcp::v4(), 8080}};
for(;;) {
tcp::socket socket{io_context};
acceptor.accept(socket);
http::response<http::string_body> response{
http::status::ok, 11};
response.set(http::field::server, "C++ Beast");
response.set(http::field::content_type, "text/plain");
response.body() = "Hello from C++ backend!";
response.prepare_payload();
http::write(socket, response);
}
}
What is C++?
C++ is a powerful, high-performance programming language that builds on the foundation of C by adding object-oriented features. It has gained prominence in system programming, game development, and, notably, backend development due to its efficiency, performance, and flexibility.
The language evolved from its humble beginnings in the early 1980s, and its robust type system and ability to directly manipulate hardware resources make it an optimal choice for developing backend systems. C++ developers can harness fine control over system resources—beneficial in building applications that require both speed and efficiency.
When we compare C++ with other programming languages used for backend development, such as Python, Ruby, or Java, C++ stands out for applications needing high performance and real-time processing. While languages like Python may enable quick application development, C++ remains unparalleled when performance optimization is critical.
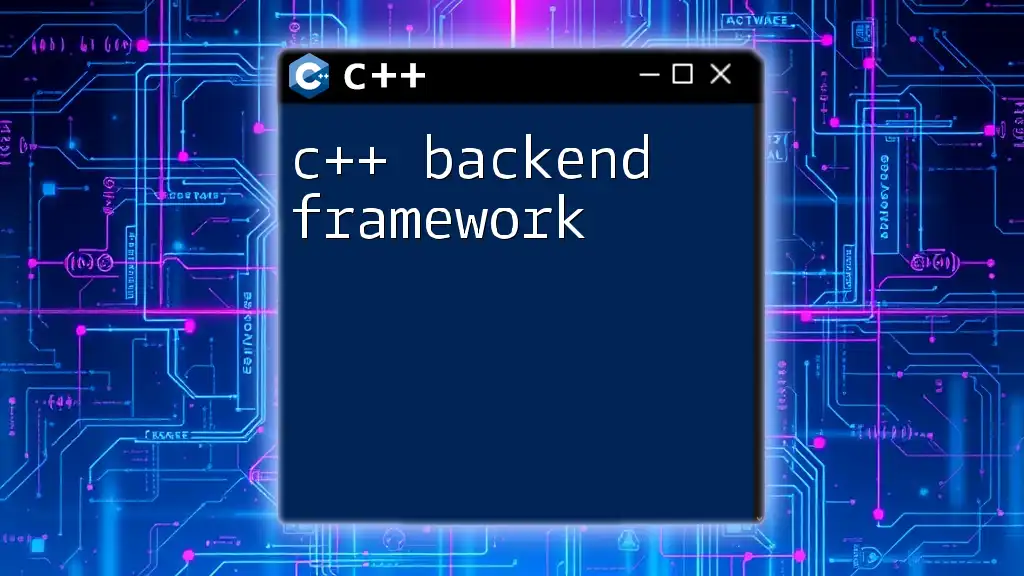
Setting Up Your C++ Development Environment
To develop efficient C++ backend applications, you first need to set up a robust development environment.
Choosing the Right IDE
Selecting a powerful Integrated Development Environment (IDE) can greatly enhance productivity. Some popular IDE options include:
- Visual Studio: Preferred for Windows development, offering extensive debugging and compiling tools.
- CLion: Known for its user-friendly interface and advanced refactoring features.
- Eclipse CDT: A versatile, cross-platform IDE with a strong plugin ecosystem.
Installing C++ Compiler
A C++ compiler is essential for converting your C++ code into executable programs. The three most prominent compilers are:
- GCC (GNU Compiler Collection): Widely used in various platforms, particularly Linux.
- Clang: Known for its speed and superior error messages.
- MSVC (Microsoft Visual C++): Optimized for Windows, part of Visual Studio.
Writing Your First C++ Program
Once your environment is set up, you can start coding. Here's an example of a simple C++ program that outputs "Hello, World!" to the console:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
This program introduces you to the essentials of C++ syntax: including libraries, defining the main function, and basic output commands.
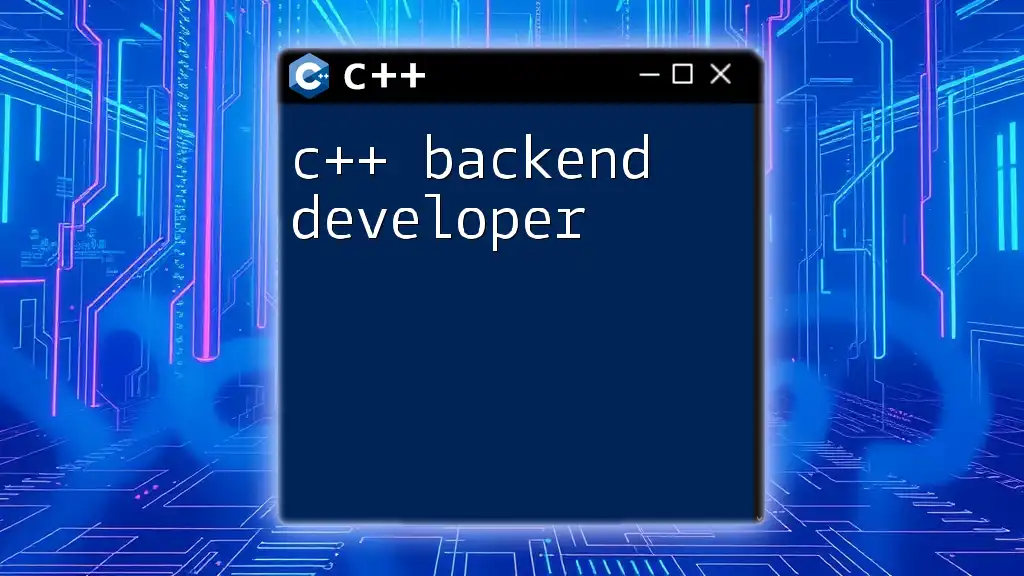
Key Concepts in C++ for Backend
Memory Management
Understanding memory management is critical in C++. Unlike languages that provide automatic garbage collection, C++ requires developers to manage memory manually. This involves understanding the use of pointers and references, as well as dynamic versus static memory allocation.
Pointers are variables that hold memory addresses, while references act as an alias to another variable. Here’s an example of dynamic memory allocation:
int* arr = new int[5]; // Dynamically allocate an array of integers
delete[] arr; // Always deallocate to prevent memory leaks
In this snippet, `new` is used to allocate memory, and it must be matched with `delete` to avoid memory leaks.
Object-Oriented Programming
Object-oriented programming (OOP) is a fundamental paradigm in C++. OOP allows developers to create classes and objects, inherit properties, and leverage polymorphism to write more maintainable code.
For instance, consider the following code snippet demonstrating class inheritance:
class Animal {
public:
virtual void sound() = 0; // Pure virtual function
};
class Dog : public Animal {
public:
void sound() override {
std::cout << "Woof!" << std::endl;
}
};
In this example, the `Animal` class is abstract, requiring derived classes like `Dog` to provide specific implementations.
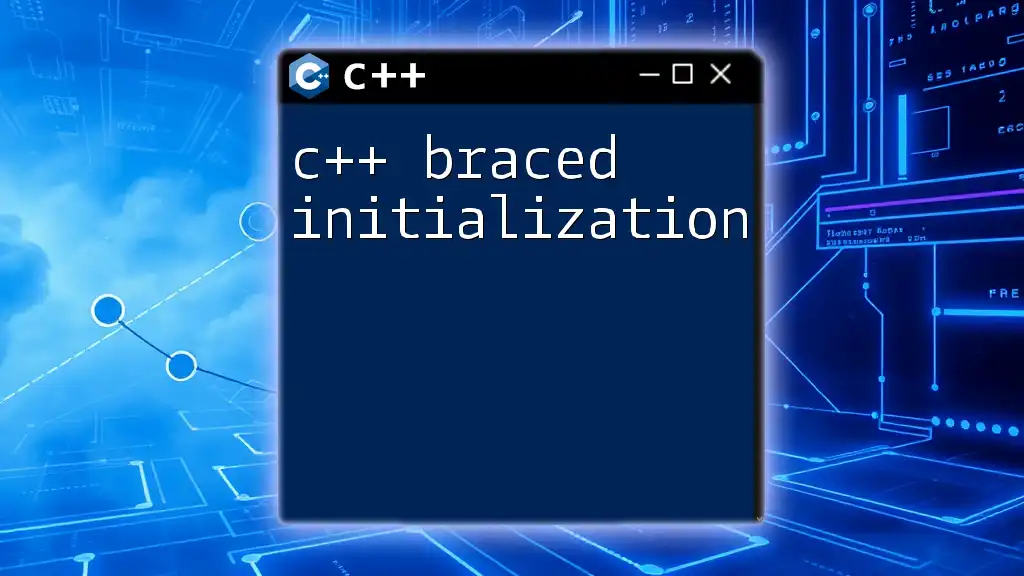
Frameworks and Libraries for C++ Backend Development
Overview of Popular C++ Frameworks
Using frameworks can significantly reduce development time and boost performance. A few notable C++ frameworks include:
- Boost: A widely used collection of libraries that extend the functionality of C++. It provides everything from smart pointers to thread management.
- Pistache: A modern and easy-to-use HTTP and REST framework for handling requests.
Understanding Database Connectivity
Building backend applications often involves databases. C++ provides several database management systems (DBMS) that offer robust features for data storage and retrieval. Utilizing libraries like SQLite makes it straightforward to work with SQL databases.
Here's a simple example of how to connect to a SQLite database:
#include <sqlite3.h>
int main() {
sqlite3* DB;
int exit = sqlite3_open("test.db", &DB); // Open database
if (exit != SQLITE_OK) {
std::cerr << "Error opening database! " << sqlite3_errmsg(DB);
}
sqlite3_close(DB); // Close database
return 0;
}
This code demonstrates the fundamental steps in connecting to a SQLite database, checking for errors, and closing the connection.
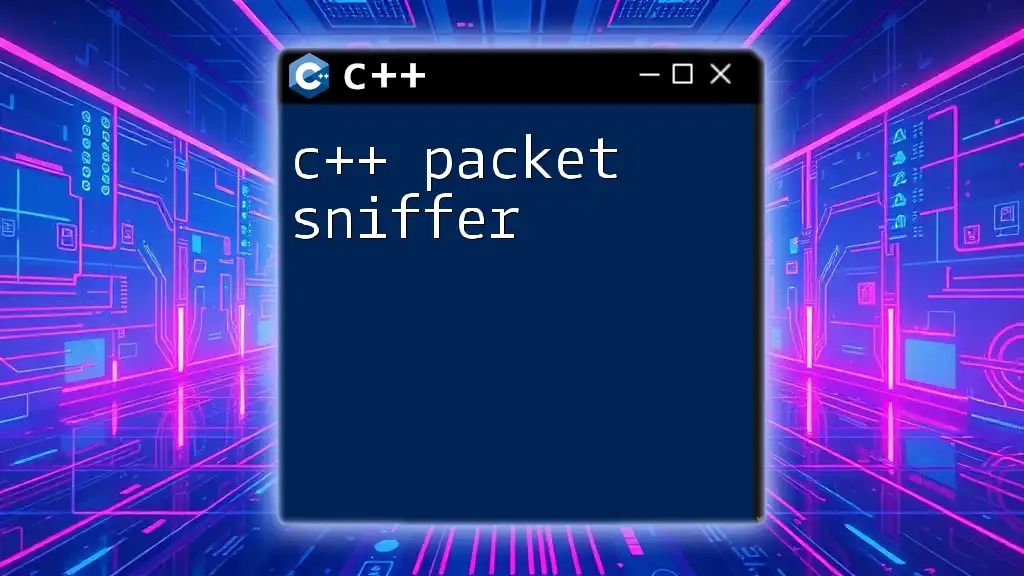
Building RESTful APIs with C++
Principles of REST
REST (Representational State Transfer) is an architectural style for designing networked applications. It utilizes standard HTTP methods like GET, POST, PUT, and DELETE for CRUD operations. Understanding REST principles is crucial for backend developers as they create services that communicate over the web.
Creating Basic RESTful API
Using C++ libraries like Pistache, developers can effortlessly set up HTTP endpoints for RESTful APIs. Here’s a basic example of an endpoint that fetches user data:
void getUserData(const Http::Request& request, Http::ResponseWriter response) {
// Your logic to fetch user data based on request goes here
response.send(Http::Code::Ok, "User data response");
}
This example highlights a simple function handling user data requests, which can easily be expanded to query from a database.
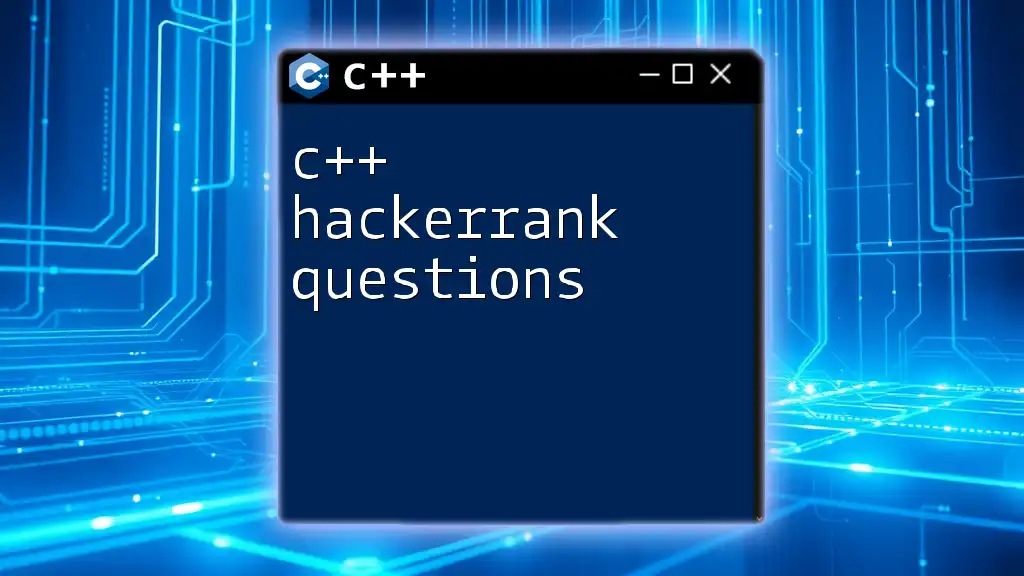
Best Practices for C++ Backend Development
Code Quality and Maintainability
Writing clean, maintainable code is essential for any programming project, particularly backend development. Use meaningful names for functions and variables and adhere to a consistent naming convention. Commenting on complex logic and creating documentation can also benefit future maintenance.
Performance Optimization
C++ excels in performance optimization. To enhance the speed of your backend applications, consider employing profiling tools to identify bottlenecks. Proper memory management is also vital—use smart pointers where applicable to avoid memory leaks and reduce the complexity of ownership responsibilities.
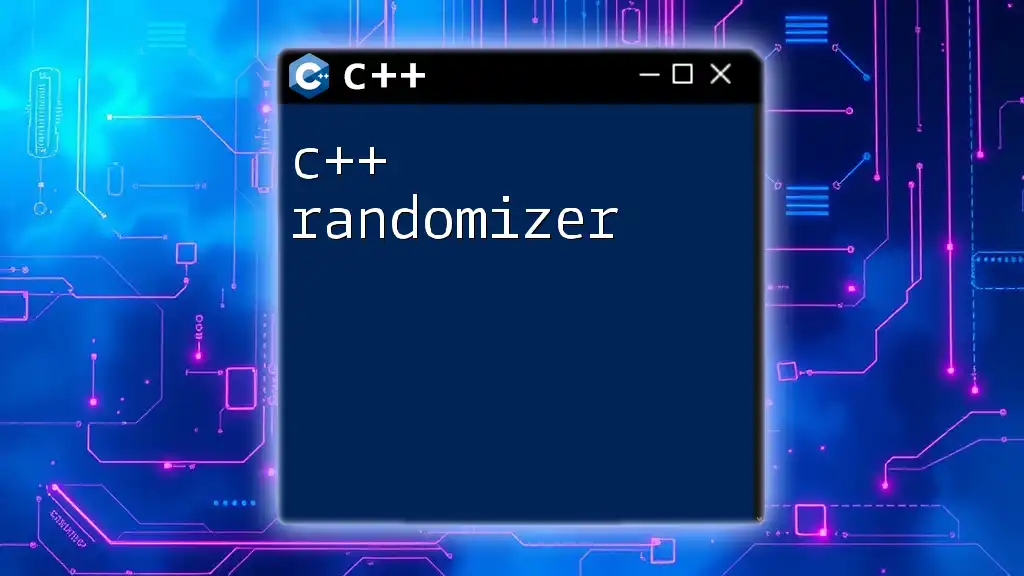
Challenges in C++ Backend Development
C++ backend developers face unique challenges, including memory management complexities and undefined behavior issues. Ensuring thread safety can also be quite challenging in multithreaded applications. Using modern C++ features, such as `std::thread`, can help manage concurrency more effectively.
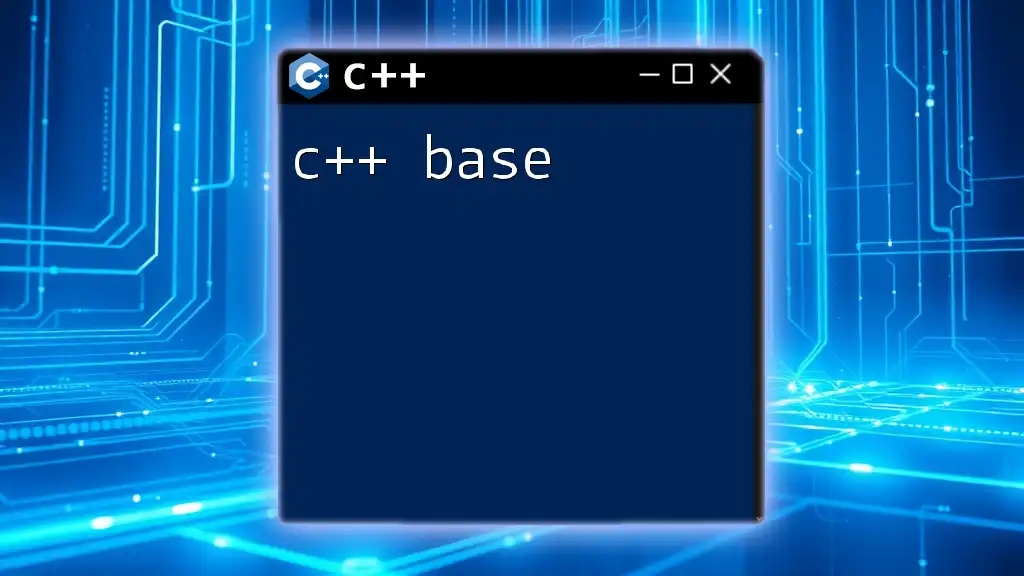
Future of C++ in Backend Development
The future of C++ in backend development looks promising. As systems grow more complex and performance demands increase, C++ is likely to remain a critical language in the backend landscape. Growing interest in cloud computing and IoT provides opportunities for robust C++ applications.
As emerging technologies, such as machine learning and artificial intelligence, evolve, C++ can provide the necessary performance and efficiency for developing real-time systems.
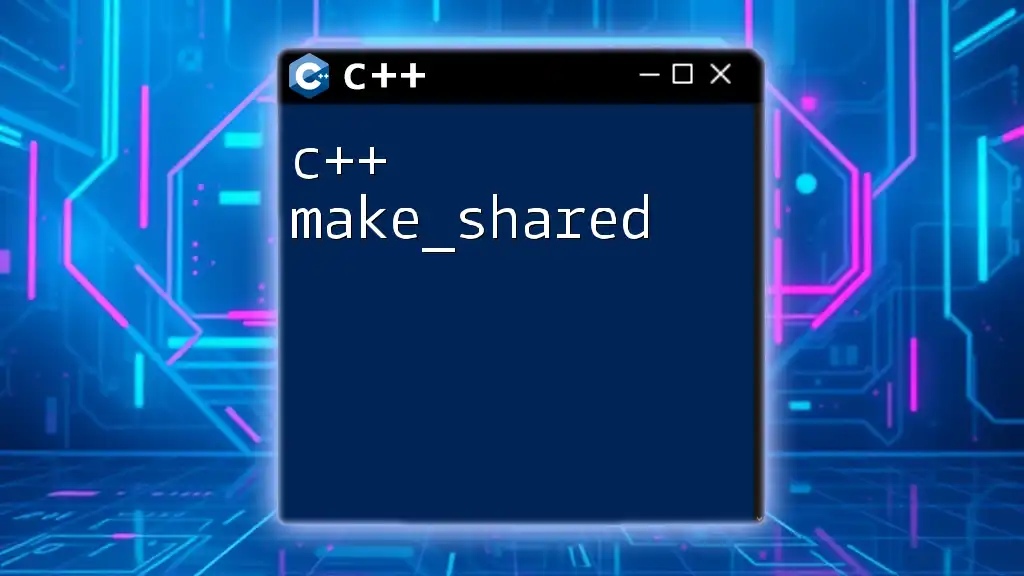
Conclusion
C++ is a formidable choice for backend development, enabling developers to create high-performance applications that can handle vast amounts of data and complex logic. The language's strengths lie in its object-oriented features, memory management capabilities, and wide-ranging libraries and frameworks.
By diving deeper into both the foundational and advanced topics covered in this guide, you can gain the expertise needed to excel in C++ backend development. Be sure to explore additional resources, engage with the community, and continue enhancing your skills as you embark on this exciting journey into C++ backend programming.