C++ can be used to create graphical user interfaces (GUIs) through various libraries, such as Qt or wxWidgets, enabling developers to build visually appealing and interactive applications.
Here’s a simple example using Qt to create a basic window:
#include <QApplication>
#include <QPushButton>
int main(int argc, char *argv[]) {
QApplication app(argc, argv);
QPushButton button("Hello, World!");
button.resize(200, 100);
button.show();
return app.exec();
}
Understanding GUI Concepts
What is a GUI?
A Graphical User Interface (GUI) provides users with an interactive interface that enables them to operate software visually instead of through text-based commands. It allows users to engage with the application using graphical elements like windows, icons, buttons, and menus. For example, operating systems such as Windows or macOS utilize GUIs for seamless user interaction, making software more accessible to a wider range of users, including those who might not be comfortable operating through command lines.
Key Components of a GUI
When developing a GUI, familiarity with its key components is essential:
- Windows serve as frames where applications are displayed. They encapsulate all GUI elements and provide a coordinated interface for user interactions.
- Buttons allow users to perform actions, and their design significantly influences user experience. Their responsiveness and arrangement can dictate how effectively a user accomplishes their goals.
- Menus offer a list of options or commands that can be easily accessed. They are fundamental in providing navigation throughout the application.
- Input Controls, such as text boxes and sliders, allow users to provide data or preferences. These controls need to be intuitive and user-friendly.
Understanding event handling is also critical. Events are user actions like button clicks or keystrokes. The GUI must handle these events effectively through callbacks, which are functions that execute in response to events.
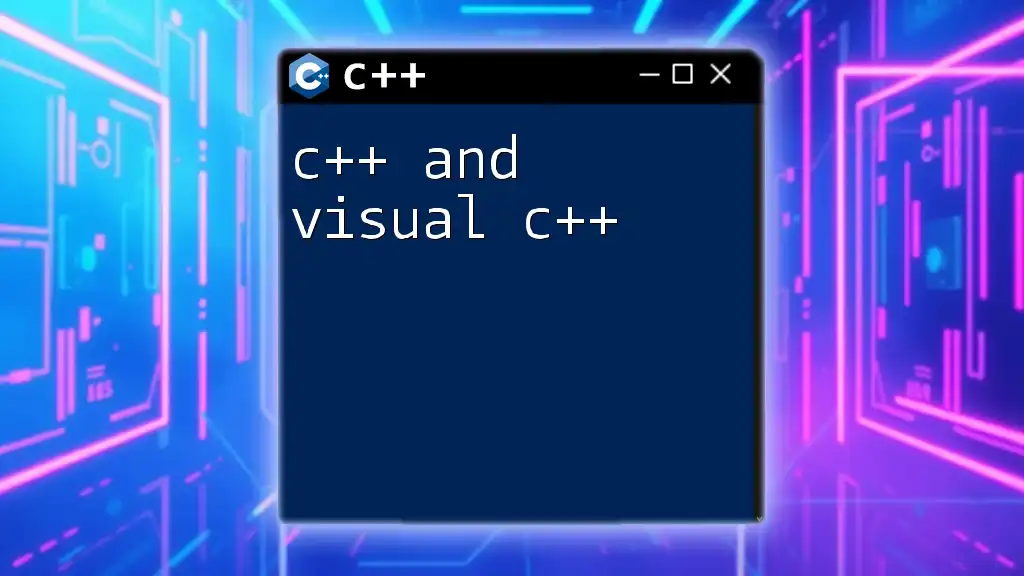
Popular GUI Frameworks for C++
When it comes to implementing GUIs in C++, several frameworks can help you create visually appealing applications. Each framework has unique capabilities, which makes it essential to choose one that fits your project’s requirements.
Qt Framework
Qt is one of the most popular C++ frameworks for GUI application development, known for its robustness and cross-platform capabilities. It provides a comprehensive library of components that enable developers to create rich GUIs with less effort.
Key Features and Components
- Signal and Slot Mechanism: This powerful feature facilitates communication between objects. It allows you to connect signals (events) to slots (functions) seamlessly.
- Rich Set of Widgets: Qt comes packed with a variety of pre-defined widgets, such as buttons, labels, and advanced ones like charts.
Setting Up a Simple Qt Application
Getting started with Qt involves setting up your environment:
- Installation Steps: You can download Qt from the official Qt website and install Qt Creator, the IDE for Qt development.
- Example Code for a Basic Qt Application:
#include <QApplication>
#include <QPushButton>
int main(int argc, char *argv[]) {
QApplication app(argc, argv);
QPushButton button("Hello, World!");
button.resize(200, 100);
button.show();
return app.exec();
}
This simple program creates a button titled "Hello, World!" that displays when the application runs.
wxWidgets
wxWidgets is another powerful GUI framework that focuses on native controls and appearance, making your application look like a native program on each supported platform.
Key Features and Components
- Native Look and Feel: wxWidgets utilizes the platform’s native API, giving applications a consistent appearance across different operating systems.
- Extensive Documentation: It comes with thorough documentation, making it easier to learn and implement.
Creating a Simple wxWidgets Application
- Installation Steps: Download wxWidgets from its official website and include it in your project.
- Example Code for a Basic wxWidgets Application:
#include <wx/wx.h>
class MyApp : public wxApp {
public:
virtual bool OnInit();
};
class MyFrame : public wxFrame {
public:
MyFrame(const wxString& title);
};
wxIMPLEMENT_APP(MyApp);
bool MyApp::OnInit() {
MyFrame *frame = new MyFrame("Hello, wxWidgets!");
frame->Show(true);
return true;
}
MyFrame::MyFrame(const wxString& title) : wxFrame(NULL, wxID_ANY, title) {}
This code creates a basic wxWidgets application that opens a window saying "Hello, wxWidgets!".
GTKmm
GTKmm is the official C++ interface for the popular GTK+ GUI toolkit. It is primarily aimed at developing graphical applications on Linux but also supports Windows and macOS.
Key Features and Components
- Intuitive API: GTKmm offers an easy-to-use programming interface, making it approachable for both beginners and advanced programmers alike.
- Rich Set of Widgets: Like Qt and wxWidgets, GTKmm provides numerous widgets for creating functional user interfaces.
Developing a Simple GTKmm Application
- Installation Steps: Install GTKmm using your package manager or download it from gtkmm.org.
- Example Code for a Basic GTKmm Application:
#include <gtkmm.h>
int main(int argc, char *argv[]) {
auto app = Gtk::Application::create(argc, argv, "org.gtkmm.example");
Gtk::Window window;
window.set_title("Hello, GTKmm!");
return app->run(window);
}
This simple program creates a window titled "Hello, GTKmm!" using GTKmm.
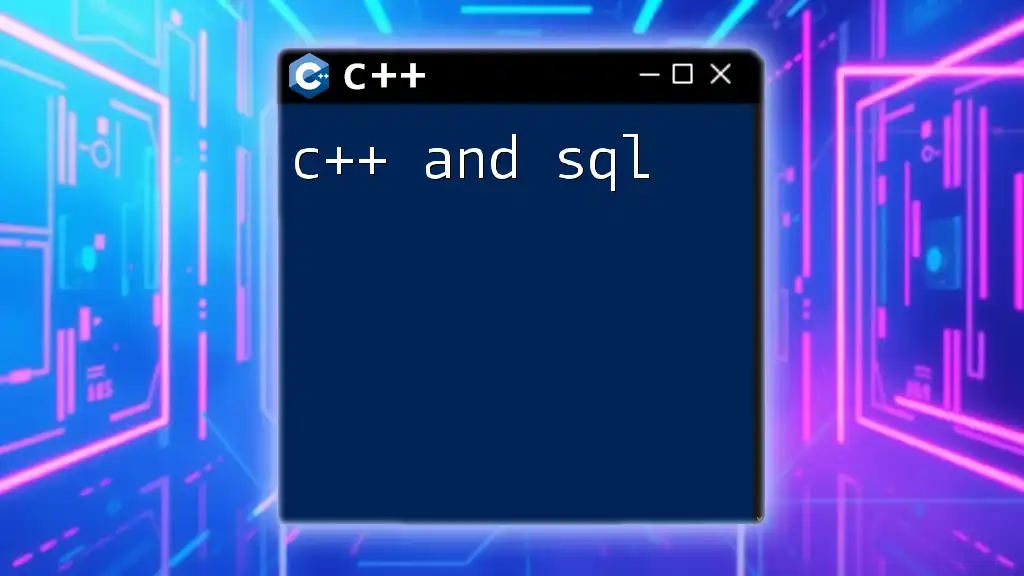
Choosing the Right GUI Framework
When selecting a GUI framework for C++ programming, it's essential to consider various factors that can affect your development process significantly.
Factors to Consider
- Project Requirements: Assess the complexity and size of your project. For small-scale projects, a lighter framework may suffice, while larger, more intricate applications may benefit from the rich features of Qt.
- Platform Compatibility: Identify your intended platforms. If targeting multiple operating systems, frameworks like Qt and wxWidgets offer excellent cross-platform support.
- Community Support and Documentation: A strong community and comprehensive documentation can substantially ease your learning curve and help you troubleshoot issues more efficiently.
Pros and Cons of Each Framework
Framework | Pros | Cons |
---|---|---|
Qt | Cross-platform, rich features | Steeper learning curve |
wxWidgets | Native appearance, good documentation | Less modern look compared to Qt |
GTKmm | Intuitive API, strong Linux support | Limited support on Windows/macOS |
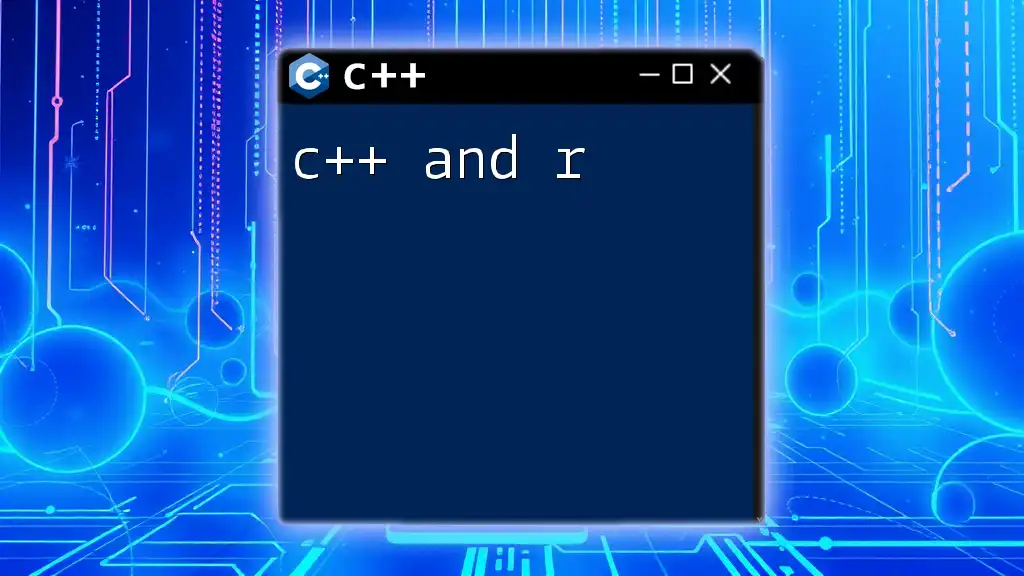
Developing a Basic GUI Application with C++
Creating a GUI application in C++ involves several critical steps, from setting up your development environment to coding the functionality.
Setting Up Your Development Environment
To get started, you need the right tools and IDE for developing C++ GUI applications. Recommended tools include:
- Qt Creator for Qt development.
- Code::Blocks or Visual Studio for wxWidgets applications.
- Code::Blocks or GNOME Builder for GTKmm.
Configuration Steps vary based on the chosen framework but generally involve ensuring libraries are linked correctly and dependencies installed.
Hands-On: Create a Simple To-Do List Application
Let’s build a simple GUI to-do list application using Qt as a demonstration.
Step-by-Step Guide
-
Designing the GUI Layout: Create a window with input fields for new tasks, a button to add tasks, and a list area to show added tasks.
-
Implementing Functionality with C++ Code:
- Add New Task: Connect the button’s clicked signal to a function that retrieves text from the input field and adds it to the list.
-
Sample Code Snippets for Each Step:
#include <QApplication>
#include <QVBoxLayout>
#include <QPushButton>
#include <QLineEdit>
#include <QListWidget>
#include <QWidget>
class TodoApp : public QWidget {
public:
TodoApp();
private:
QLineEdit *taskInput;
QListWidget *taskList;
};
TodoApp::TodoApp() {
taskInput = new QLineEdit(this);
QPushButton *addButton = new QPushButton("Add Task", this);
taskList = new QListWidget(this);
QVBoxLayout *layout = new QVBoxLayout(this);
layout->addWidget(taskInput);
layout->addWidget(addButton);
layout->addWidget(taskList);
connect(addButton, &QPushButton::clicked, [this]() {
taskList->addItem(taskInput->text());
taskInput->clear();
});
}
int main(int argc, char *argv[]) {
QApplication app(argc, argv);
TodoApp window;
window.setWindowTitle("Simple To-Do List");
window.show();
return app.exec();
}
This code sets up a basic to-do list GUI application where you can enter tasks and add them to the list.
Debugging and Testing
As with any programming endeavor, encountering bugs is expected. Common issues in GUI applications include:
- Incorrectly set event handlers that prevent interactions.
- Layout issues where widgets overlap or do not render as intended.
Tips for Effective Debugging:
- Use debugging features in your IDE to step through code.
- Print debug statements to console for tracking variable states and event triggers.
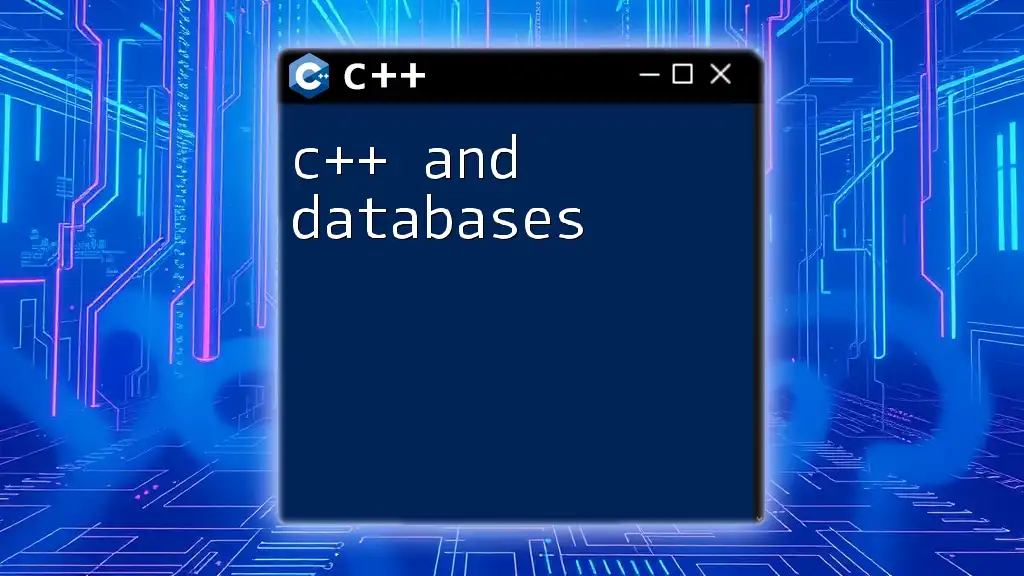
Advanced GUI Topics
Customizing GUI Elements
One key aspect of GUI development is customization. The look and feel of your application should resonate with your target users.
- Styling Buttons and Windows: Change styles using stylesheets in Qt or properties in wxWidgets.
- Using Resource Files: Enhance UI aesthetics with icons and images stored in resource files integrated within your application.
Implementing MVC Pattern in GUI Applications
Understanding design patterns is crucial in creating scalable applications. The Model-View-Controller (MVC) pattern is widely adopted in GUI development.
- Explanation of MVC: The Model represents the data, the View is the user interface, and the Controller manages user input, often bridging the two.
This approach promotes a clean separation of concerns, making the application easier to manage and test.
Multithreading in GUI Applications
Responsiveness is vital in GUI applications, especially when processing heavy tasks.
-
The Importance of Responsiveness: Keeping the GUI responsive while performing background tasks helps improve user experience.
-
Managing Threads in C++ for GUI Applications: Use worker threads for long-running tasks and signal back to the GUI thread to update the interface, utilizing the threading capabilities in frameworks like Qt.
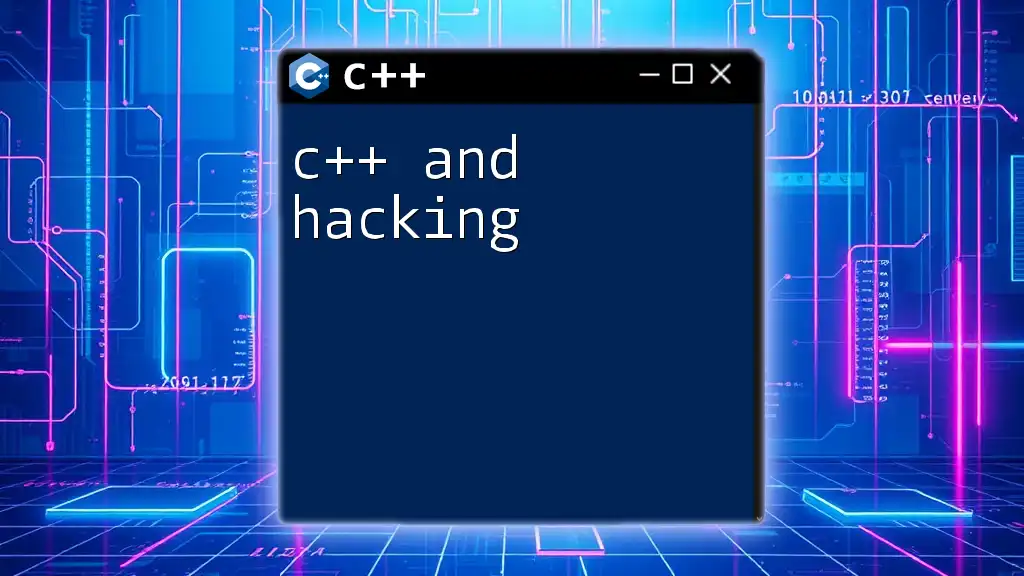
Best Practices for C++ GUI Programming
Writing Readable and Maintainable Code
Maintainability is essential for long-term project success. Follow these guidelines:
- Code Style Guidelines: Adhere to consistent naming conventions and formatting styles. This practice facilitates team collaboration and code reviews.
- Commenting and Documentation: Clearly comment on your code and keep documentation updated to reflect ongoing changes, aiding future maintenance efforts.
Performance Optimization
Performance is a crucial aspect of any application. To optimize your GUI application:
-
Reducing Resource Usage: Optimize images and control rendering to minimize memory consumption and improve load times.
-
Lazy Loading and Asynchronous Operations: Load heavy components only when needed using asynchronous programming to improve initial response times of your application.
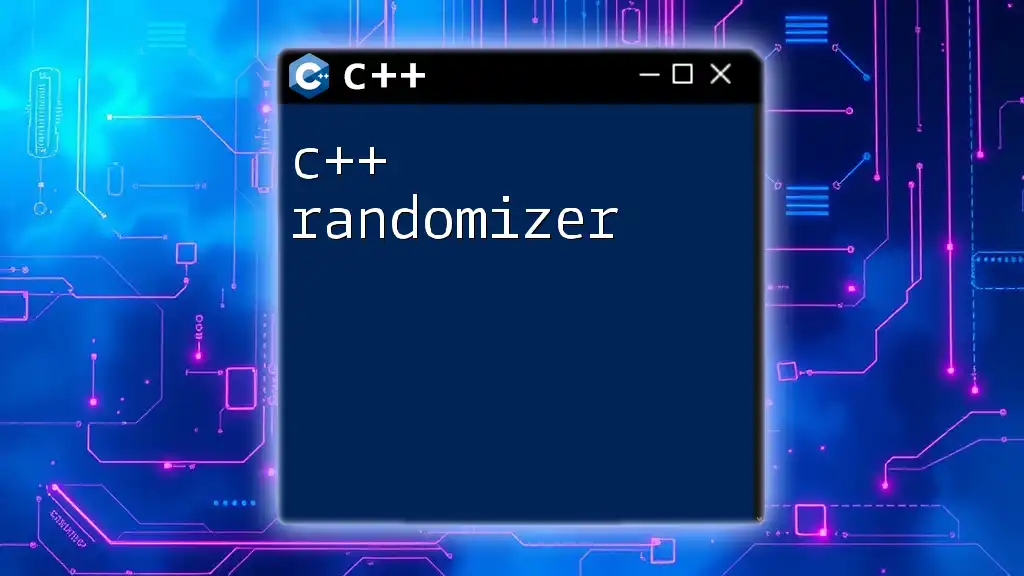
Conclusion
C++ and GUI programming open up exciting opportunities for creating user-friendly software applications. By exploring frameworks like Qt, wxWidgets, and GTKmm, developers are equipped to build visually engaging and functional interfaces. Understanding the basics, from GUI concepts to advanced topics, allows for a solid foundation in GUI development. Whether you're a beginner or an experienced coder, the world of C++ and GUI offers a wealth of knowledge and possibilities. Exploring further through additional resources will enhance your skills and confidence in GUI programming.
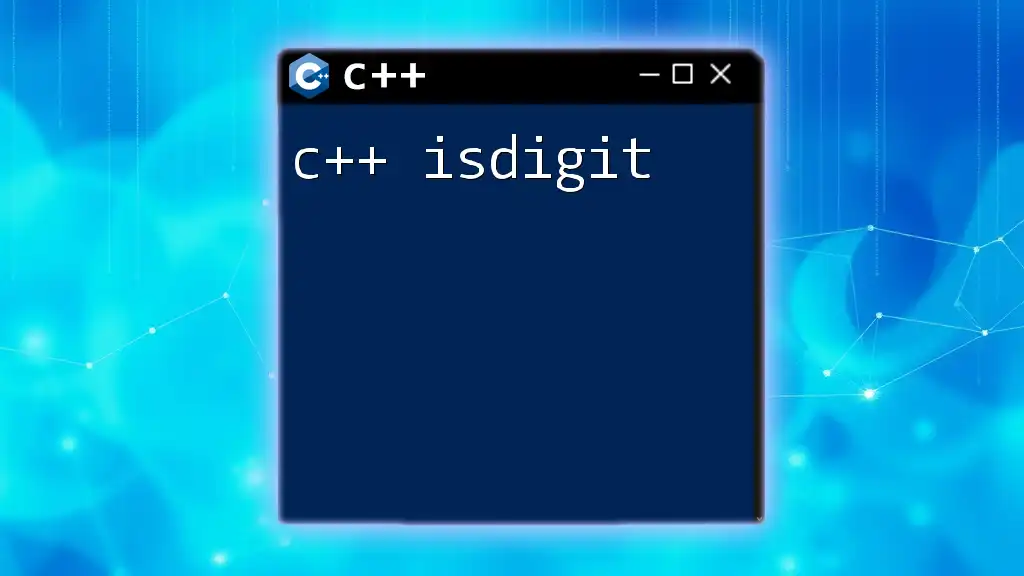
Frequently Asked Questions (FAQs)
Can I Use C++ for Web-based GUI Applications?
Yes, while C++ is not primarily used for web-based applications, frameworks like Emscripten allow you to compile C++ applications to WebAssembly, enabling C++ programs to run in web browsers.
What is the Future of C++ and GUI Development?
The future of C++ in GUI development looks promising, with advancements in frameworks and libraries continuously emerging. As applications become more sophisticated, C++ will be critical in delivering performance and advanced functionalities.
How Do I Get Started with GUI Programming in C++?
Start by choosing a GUI framework that fits your needs, install it, and follow beginner tutorials and projects. Building simple applications will significantly enhance your understanding and skills.