In C++, you can generate a random integer within a specified range using the `<cstdlib>` library along with the `rand()` function and a simple formula to scale the random number accordingly. Here's an example code snippet:
#include <iostream>
#include <cstdlib>
#include <ctime>
int main() {
std::srand(std::time(0)); // Seed for random number generation
int lower = 1, upper = 100;
int randomInteger = lower + std::rand() % (upper - lower + 1);
std::cout << "Random Integer: " << randomInteger << std::endl;
return 0;
}
Understanding Random Numbers in C++
What is a Random Number?
A random number is a value generated in a manner that ensures it cannot be accurately predicted. Randomness can be categorized into two types: pseudorandom numbers, which are generated algorithmically and appear random, and true random numbers, which are derived from inherently unpredictable natural processes. In C++, we typically use pseudorandom numbers, which are generated through algorithms that utilize algorithms with seed values.
Importance of Randomness in C++
Random numbers play a significant role across various applications, including games, simulations, and cryptography. For instance, in gaming, random numbers can determine outcomes like dice rolls or card shuffles. In simulations, they allow the modeling of unpredictable events, such as stock market fluctuations or weather changes. Cryptography relies heavily on randomness for key generation, ensuring secure communication.
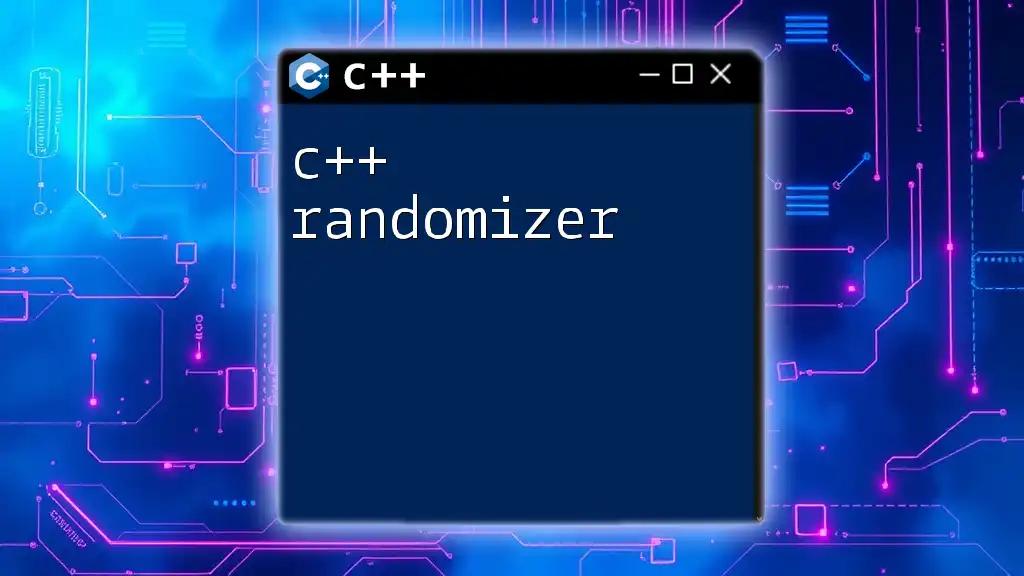
Setting Up the Environment
Required Libraries
To generate random integers in C++, you need to include specific libraries. The most commonly used libraries for this purpose are `<random>`, which facilitates advanced random generation, and `<ctime>`, which allows us to work with time to seed our generator.
To include these libraries in your code, use the following syntax:
#include <random>
#include <ctime>
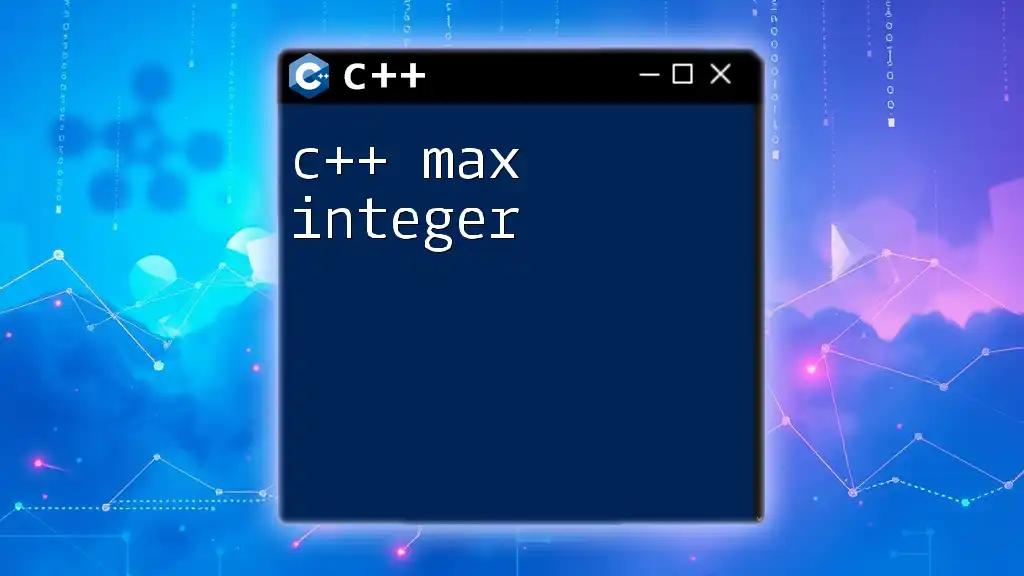
Generating Random Integers in C++
Basic Method for Random Integers
The simplest way to generate a random integer in C++ is by using the `rand()` function from the `<cstdlib>` library. However, it's essential to note that `rand()` has certain limitations, including its predictable sequence if not seeded.
Here's how you can use `rand()`:
#include <cstdlib>
#include <iostream>
int main() {
std::cout << "Random Number: " << rand() % 100 << std::endl; // Generates a number between 0 and 99
return 0;
}
In this example, `rand() % 100` means we limit the random integer generation to numbers between 0 and 99. However, using `rand()` without seeding can lead to the same sequence of numbers appearing every time the program runs.
Using the `<random>` Library
Using the `<random>` library provides a more sophisticated and robust method for generating random integers. The `std::mt19937` class, which implements the Mersenne Twister algorithm, offers better randomness compared to `rand()`.
Creating a Random Integer Generator
To set up a random integer generator, initialize `std::mt19937` and define the distribution:
#include <random>
#include <iostream>
int main() {
std::mt19937 generator(static_cast<unsigned int>(std::time(nullptr))); // Seeding with current time
std::uniform_int_distribution<int> distribution(1, 100); // Range of numbers
std::cout << "Random Number: " << distribution(generator) << std::endl;
return 0;
}
In this code snippet, we seed the random number generator with the current time, ensuring different results each time the program runs. The `std::uniform_int_distribution<int>` allows us to specify a range for the random integers, in this case from 1 to 100.
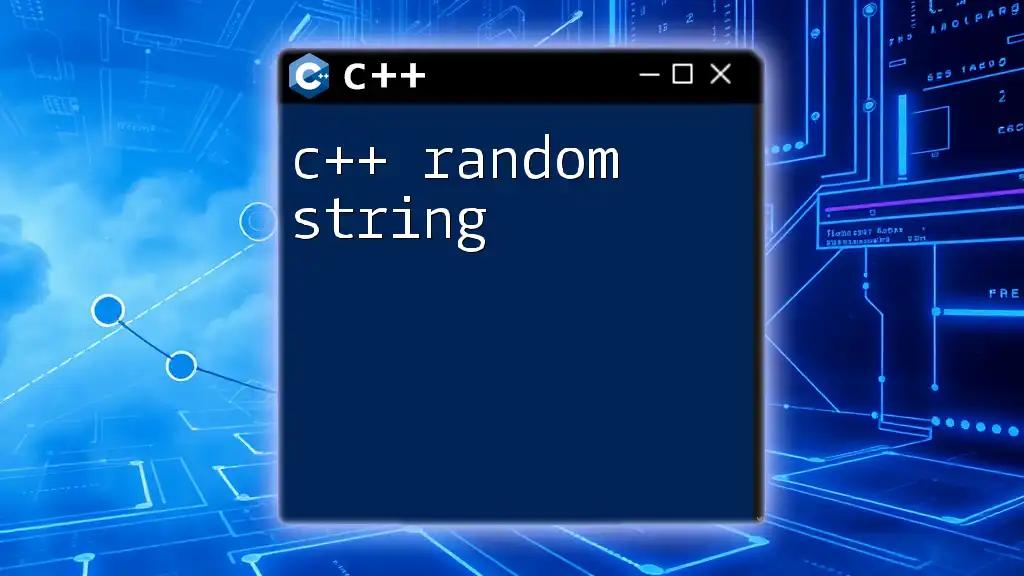
Controlling Random Number Distribution
Uniform Distribution
Uniform distribution means that every number in a given range has an equal probability of being generated.
Here’s an example of generating uniform random integers:
#include <random>
#include <iostream>
int main() {
std::mt19937 generator(static_cast<unsigned int>(std::time(nullptr)));
std::uniform_int_distribution<int> distribution(1, 100); // Range 1-100
for (int i = 0; i < 5; ++i) {
std::cout << "Random Number: " << distribution(generator) << std::endl;
}
return 0;
}
In this example, five random numbers between 1 and 100 are produced, each having an equal chance of appearing.
Non-Uniform Distribution
C++ also allows for non-uniform distributions, such as normal distribution. A normal distribution produces numbers centered around a mean, where values further from the mean become increasingly rare.
To implement a normal distribution for generating random integers, use:
#include <random>
#include <iostream>
int main() {
std::mt19937 generator(static_cast<unsigned int>(std::time(nullptr)));
std::normal_distribution<double> distribution(50.0, 15.0); // mean=50, stddev=15
for (int i = 0; i < 10; ++i) {
std::cout << "Random Number: " << static_cast<int>(distribution(generator)) << std::endl;
}
return 0;
}
In this scenario, the numbers generated will cluster around the mean (50) with a standard deviation of 15. This distribution helps model scenarios where outcomes are more likely to occur around a central value.
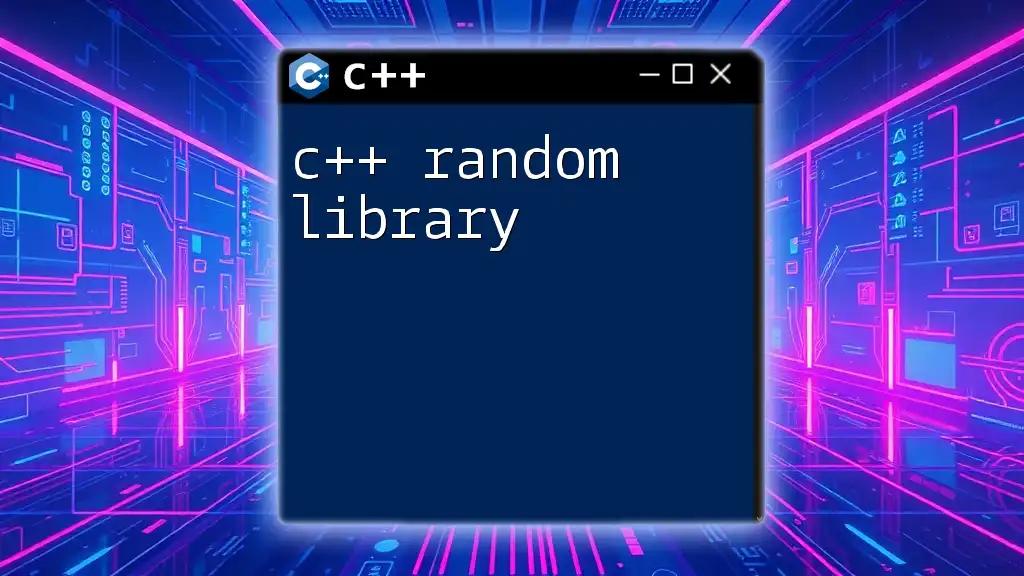
Seeding the Random Number Generator
What is Seeding?
Seeding is a critical aspect of random number generation in C++. It defines the initial value for the random number generator, which influences the subsequent random number sequence. If you use the same seed repeatedly, the sequence generated will be identical each time, leading to less randomness.
Practical Examples of Seeding
To ensure varied outputs, you can seed the generator with time or another varying value:
std::mt19937 generator(static_cast<unsigned int>(std::time(nullptr))); // Different output each execution
If you replace `std::time(nullptr)` with a fixed number, like `42`, the output will be the same across different executions, thereby reducing randomness.
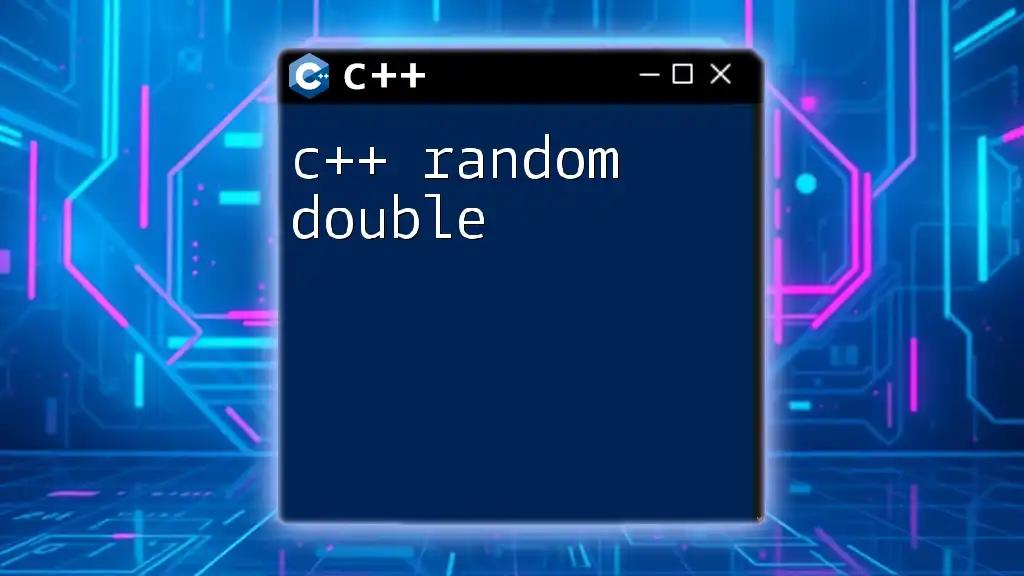
Common Mistakes and Best Practices
Common Mistakes
Many beginners make the mistake of using `rand()` without seeding. This oversight leads to repetitive outputs across program runs, which can hinder effective testing and deployment. Another frequent error is not setting a proper range for generated numbers, leading to unexpected results.
Best Practices
Using the `<random>` library over `rand()` is strongly recommended for better reliability and diversity in random numbers. When implementing your random integer generator, ensure to seed it with a varying value to maintain randomness and avoid generating predictable sequences.
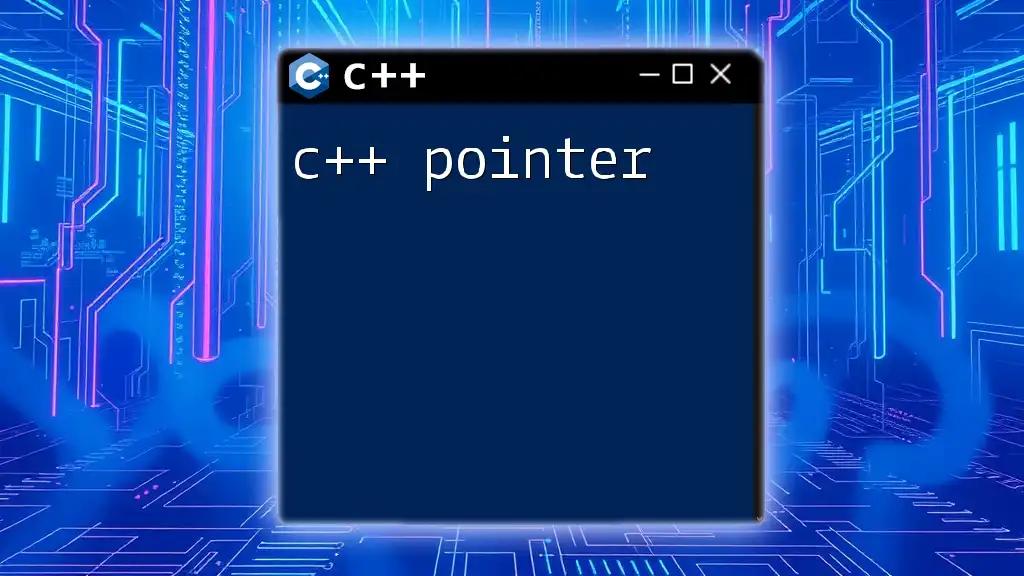
Use Cases for Random Integers
Games
In game development, random integers are utilized to simulate elements like random enemy spawns, loot drops, and procedural level generation, providing engaging and unpredictable player experiences.
Simulations
Random integers are essential in running simulations, allowing for the modeling of realistic scenarios, such as random weather patterns or traffic flows in city planning simulations.
Cryptography
In security applications, random integers play a crucial role in generating keys for encryption, ensuring that communications remain secure and unpredictable against potential intrusions.

Conclusion
In this article, we explored various methods for generating C++ random integers, ranging from basic usage of the `rand()` function to more sophisticated implementations using the `<random>` library. Understanding how to control distributions and properly seed random number generators enhances the effectiveness and flexibility of random number applications in programming. Experimenting with these concepts is vital in mastering random integer generation, leading to better software development practices.

Additional Resources
For those looking to dive deeper into the C++ random integer topic, consider exploring recommended books and articles on C++ programming, as well as online tutorials and courses focusing on advanced random number techniques and distributions.

FAQs
Q: What is the difference between `rand()` and `<random>`?
A: `rand()` is a simpler, older function that lacks flexibility and predictability when not seeded. The `<random>` library provides more powerful and customizable options for generating pseudorandom numbers.
Q: How can I ensure a unique random number each time my program runs?
A: To obtain unique outputs, you should seed your random number generator with a changing value, such as the current time using `std::time(nullptr)`. This ensures different sequences on each execution.