In C++, you can generate a random number between 1 and 10 using the `rand()` function along with some arithmetic to scale the output appropriately. Here's a code snippet to demonstrate this:
#include <iostream>
#include <cstdlib>
#include <ctime>
int main() {
std::srand(std::time(0)); // Seed for random number generation
int random_number = std::rand() % 10 + 1; // Random number between 1 and 10
std::cout << random_number << std::endl;
return 0;
}
Understanding Random Number Generation in C++
Random number generation (RNG) is a core concept in programming and computational fields. It allows developers to create unpredictable outputs, which are essential in various applications such as simulations, cryptography, gaming, and statistical sampling. The key to effective RNG in C++ lies in leveraging the capabilities of the C++ Standard Library, which houses a robust set of tools for generating random numbers.
The C++ Standard Library
The C++ Standard Library includes several powerful features that facilitate random number generation. Specifically, it provides dedicated classes and functions under the `<random>` header, which assists in producing both pseudo-random and more sophisticated random sequences. Utilizing these built-in functionalities not only enhances code readability but also ensures the randomness of generated numbers in a verified manner.

Setting Up RNG in C++
To generate a random number between 1 and 10 in C++, we first need to ensure we have the right components in place.
Required Headers for Random Number Generation
The principal header for accessing random number functionalities in C++ is `<random>`. Additionally, you'll still need `<iostream>` for input and output operations. This combination gives you access to the necessary tools for effective RNG.
Initializing a Random Number Generator
Before we can generate a random number, we must set up our generator. A commonly used generator in modern C++ is the Mersenne Twister (`std::mt19937`), which offers a good balance of speed and randomness.
Code Snippet: Basic Setup
#include <iostream>
#include <random>
std::mt19937 generateRandomNumber() {
std::random_device rd; // Seed
std::mt19937 gen(rd()); // Mersenne twister
return gen;
}
In the code above, `std::random_device` is used to provide a seed for the generator, which helps in ensuring that each run produces different sequences of random numbers. The `std::mt19937` is a popular choice among developers for general-purpose randomness due to its excellent performance characteristics.

Generating Random Numbers within a Specified Range
When we need a random integer within a specific range, we utilize the concept of distributions. C++ offers the `std::uniform_int_distribution`, which provides a way to output random integers uniformly distributed across a specified range.
Using `std::uniform_int_distribution`
The `std::uniform_int_distribution` class is designed to generate randomly selected integers within a defined interval, making it ideal for generating a c++ random number between 1 and 10.
Code Snippet: Generating Random Numbers from 1 to 10
#include <iostream>
#include <random>
int getRandomNumber(int min, int max) {
std::random_device rd; // Seed
std::mt19937 gen(rd()); // Mersenne twister
std::uniform_int_distribution<> distr(min, max); // Define the range
return distr(gen); // Generate and return the random number
}
int main() {
std::cout << "Random number between 1 and 10: " << getRandomNumber(1, 10) << std::endl;
return 0;
}
In this example, the function `getRandomNumber` takes two parameters, `min` and `max`, which define the range for our random number. The `std::uniform_int_distribution<>` is utilized to create a distribution for integers between `min` and `max`, which is then called within the `gen` function, returning a random number.

Understanding the Code: Line-by-Line Breakdown
- Line 1: `#include <iostream>` - This inclusion facilitates the use of input and output streams, essential for displaying results on the console.
- Line 2: `#include <random>` - This line is crucial, giving access to the random number generation library which includes the necessary classes and functions.
- Lines 3-6: These lines define `getRandomNumber` function which initializes our random number generator and the distribution range.
- Lines 7-10: The `main` function serves as the entry point of the program, invoking `getRandomNumber` and printing the result to the console for user visibility.
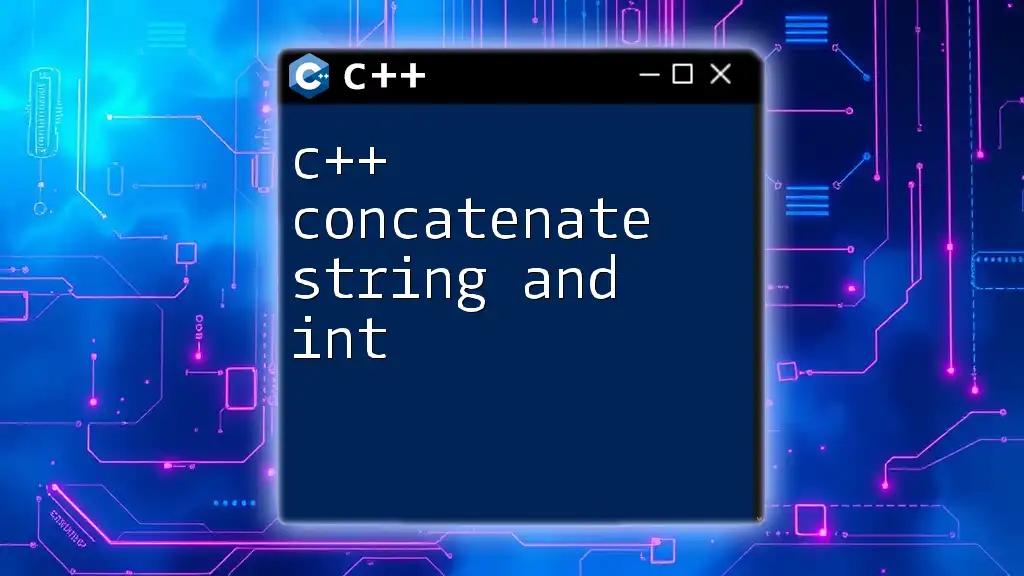
Common Pitfalls in Random Number Generation
When dealing with random number generation, several common mistakes can lead to unintended outcomes:
-
Not seeding your random number generator: If you don’t seed your random number generator, successive runs of the program might produce the exact same set of random numbers. This defeats the primary purpose of having randomness.
-
Relying on the default seed: Using the same seed every time can produce predictable results. Using `std::random_device` mitigates this by providing a random seed for each execution, ensuring diversity in your outputs.
Possible Solutions and Best Practices
To avoid the above pitfalls, always ensure to initialize your RNG with a unique seed using `std::random_device`. Additionally, familiarize yourself with different distributions offered by C++, as well as their use-cases.

Best Practices for Random Number Generation
-
Choose the Right RNG Algorithm: Select a suitable random number generator for your specific needs. While `std::mt19937` is a solid choice for general cases, some scenarios might call for a different generator.
-
Proper Seeding: Always seed your generator each time you run your program, especially for applications where true randomness is critical, like in games.
-
Understand Distribution: Familiarize yourself with the different types of distributions available in C++, such as normal distribution (`std::normal_distribution`) and uniform distribution. Knowing when to use each can dramatically improve both functionality and application reliability.

Conclusion
In summary, generating a c++ random number between 1 and 10 can be easily accomplished using the features of the C++ Standard Library. Through proper setup and understanding of the components, one can achieve great versatility in random number generation. Experimenting with this setup can further enhance your programming skills, enabling you to dive deeper into more advanced RNG techniques.
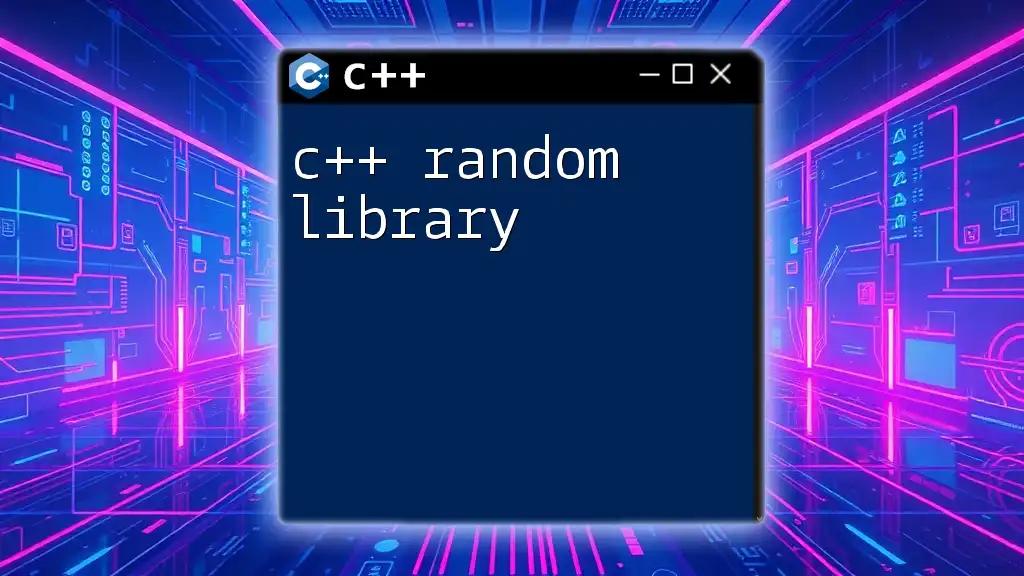
Additional Resources
For more in-depth information, you may want to consult the official C++ documentation for the `<random>` library. Engaging with online courses or textbooks focused on advanced C++ can greatly enhance your understanding of both CSC and practical applications of random number generation.