C++ is a powerful, low-level programming language that offers fine control over system resources and memory management, while C# is a higher-level, object-oriented language primarily used for developing Windows applications and web services through the .NET framework.
Here’s a simple example demonstrating the syntax differences in defining a class and a method in both languages:
// C++ Example
class MyClass {
public:
void MyMethod() {
std::cout << "Hello from C++!" << std::endl;
}
};
// C# Example
class MyClass {
public void MyMethod() {
Console.WriteLine("Hello from C#!");
}
}
Understanding the Basics
What is C++?
C++ is a general-purpose programming language created by Bjarne Stroustrup in the early 1980s as an enhancement of the C language. Renowned for its performance and ability to allow fine-grained control over system resources, C++ is a multi-paradigm language that supports both procedural and object-oriented programming. This versatility makes it especially useful for resource-intensive applications such as:
- Game development: Powerful graphics engines and real-time performance are often built with C++.
- System/software applications: Operating systems and real-time systems commonly use C++ for its efficiency.
What is C#?
C#, pronounced "C-sharp," was developed by Microsoft and released as part of the .NET framework in the early 2000s. It is often praised for its simplicity and modern syntax that facilitates rapid application development. C# is a purely object-oriented language, meaning it emphasizes the use of classes and objects to organize code, making it an excellent choice for:
- Web applications: Using ASP.NET, developers can create dynamic websites quickly and efficiently.
- Enterprise software: C# is favored in large business environments for its robustness and extensive support through libraries.
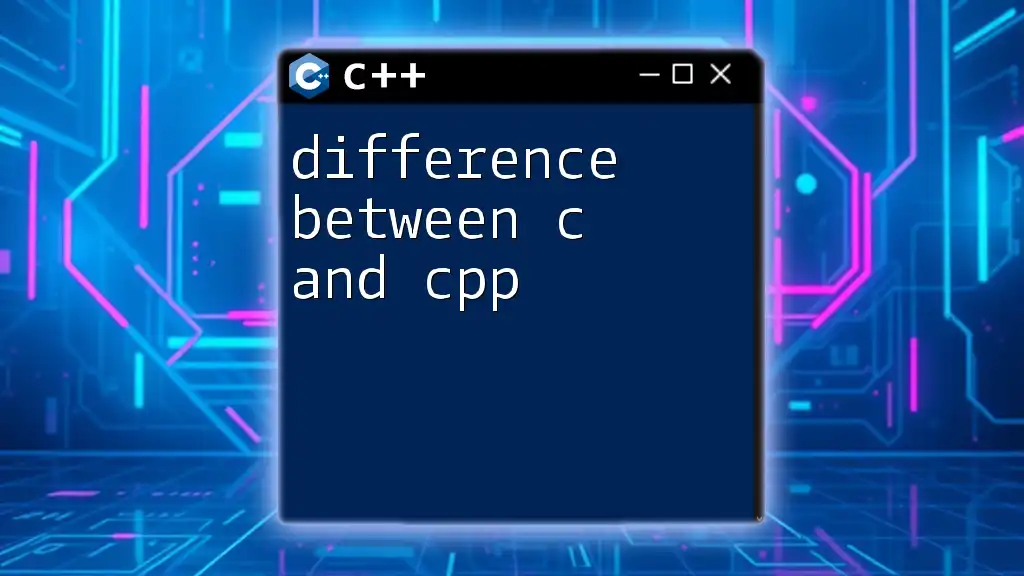
C++ vs C#: A Comparative Analysis
Syntax Differences
Basic Syntax Overview
The syntax of C++ can be more complex compared to C#. For example, the inclusion of headers and the use of namespaces in C++ may initially seem cumbersome to beginners. Here's how the "Hello, World!" program stands in both languages:
// C++ Example
#include <iostream>
using namespace std;
int main() {
cout << "Hello, World!";
return 0;
}
// C# Example
using System;
class Program {
static void Main() {
Console.WriteLine("Hello, World!");
}
}
Notice that C# requires less boilerplate code, making it easier for beginners to dive into programming.
Programming Paradigms
Object-Oriented vs. Procedural
C++ supports multiple programming paradigms, meaning that you can adopt a procedural approach or a purely object-oriented one. This flexibility allows developers to choose the paradigm that best suits their project needs. For instance, C++ allows the use of class and struct interchangeably, giving developers more options.
Conversely, C# enforces an object-oriented structure. Everything in C# revolves around classes and objects. Here’s an example demonstrating a simple class in both languages:
// C++ Example
class Dog {
public:
void bark() {
cout << "Woof!";
}
};
// C# Example
class Dog {
public void Bark() {
Console.WriteLine("Woof!");
}
}
The structural differences are pronounced; in C#, class and method declarations are more straightforward and easier to grasp for new programmers.
Memory Management
Manual vs. Automatic
One of the notable distinctions between C++ and C# is their approach to memory management. In C++, developers are responsible for manual memory management, often using `new` and `delete` to allocate and free memory. This provides great control but can lead to memory leaks if not managed properly.
// C++ Example
int* arr = new int[10];
// ... use arr
delete[] arr;
In contrast, C# employs garbage collection, which automatically manages memory allocation and deallocation. This means developers can focus more on their code rather than on memory management.
// C# Example
int[] arr = new int[10];
// No need to manually free memory
Performance Metrics
Speed and Efficiency
When it comes to performance metrics, C++ is generally considered faster because it compiles directly to machine code. This makes it a top choice for applications where performance is critical, such as gaming and systems programming.
C#, while optimized for productivity and usability, may introduce additional overhead due to its managed runtime environment. In scenarios such as enterprise applications where performance trade-offs are acceptable for faster development cycles, C# shines.
Compilation and Execution
C++ is a compiled language, meaning source code is transformed into machine code by a compiler before execution. This compilation step can be lengthy, but it results in faster execution speeds.
C#, on the other hand, uses Just-In-Time (JIT) compilation, which translates code into machine language at runtime. This can lead to slower startup times, but once the code is running, the performance often improves, making it feasible for large-scale applications.
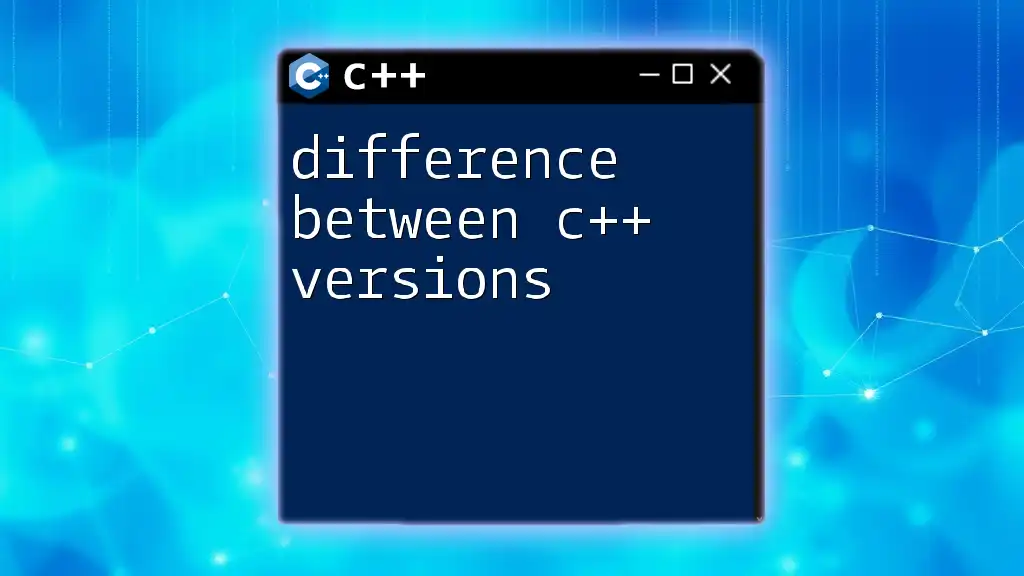
Key Libraries and Ecosystem
Standard Libraries
The Standard Template Library (STL) in C++ provides a rich set of features including algorithms, iterators, and data structures like lists and vectors. This allows developers to write efficient and reusable code.
C#, within the .NET framework, offers a wealth of libraries and frameworks that simplify many programming tasks, like ASP.NET for web development and Entity Framework for data handling. The ease of use and comprehensive features of C# libraries can greatly speed up the development process.
Third-Party Libraries
C++ has a rich ecosystem of third-party libraries such as Boost and Qt, which enhance its functionality and efficiency. However, integrating these libraries may require additional effort compared to C#.
C#, especially through its association with .NET, has extensive libraries and frameworks that are easy to integrate, allowing for quick development cycles and diverse application capabilities.
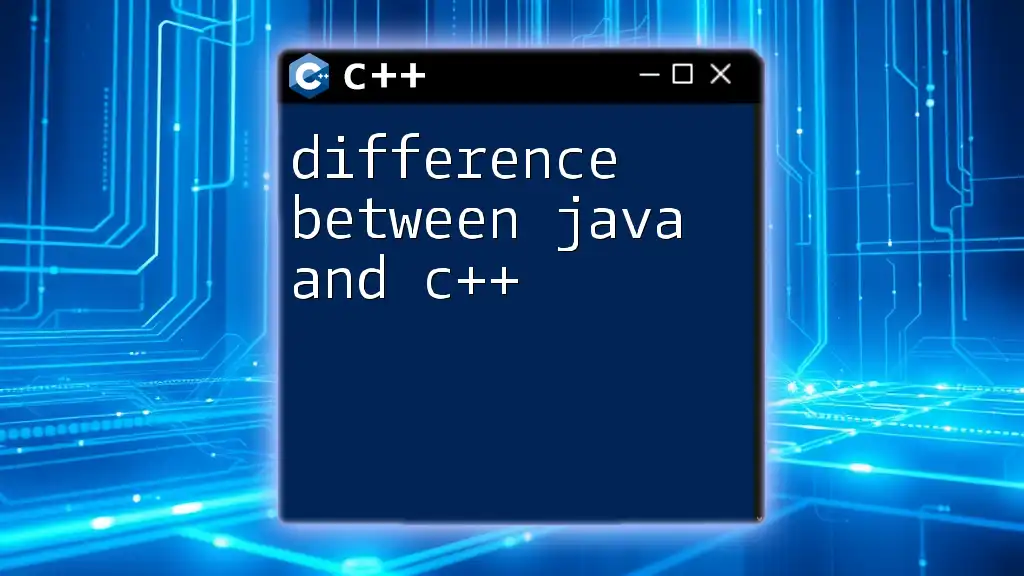
Development Environment
IDE Support
C++ is supported by multiple Integrated Development Environments (IDEs) such as Code::Blocks, Eclipse, and Visual Studio. While these IDEs offer powerful features, the setup may be more complex due to the language's versatility.
C#, primarily used with Visual Studio, provides a streamlined experience for developers. Visual Studio is revered for its user-friendly interface, comprehensive debugging tools, and strong support for C# development.
Community and Resources
Both languages boast strong community support. C++ has a long-standing history and robust resources available through forums, books, and online courses. C# benefits from Microsoft’s extensive documentation and a rapidly growing community that supports modern application development.
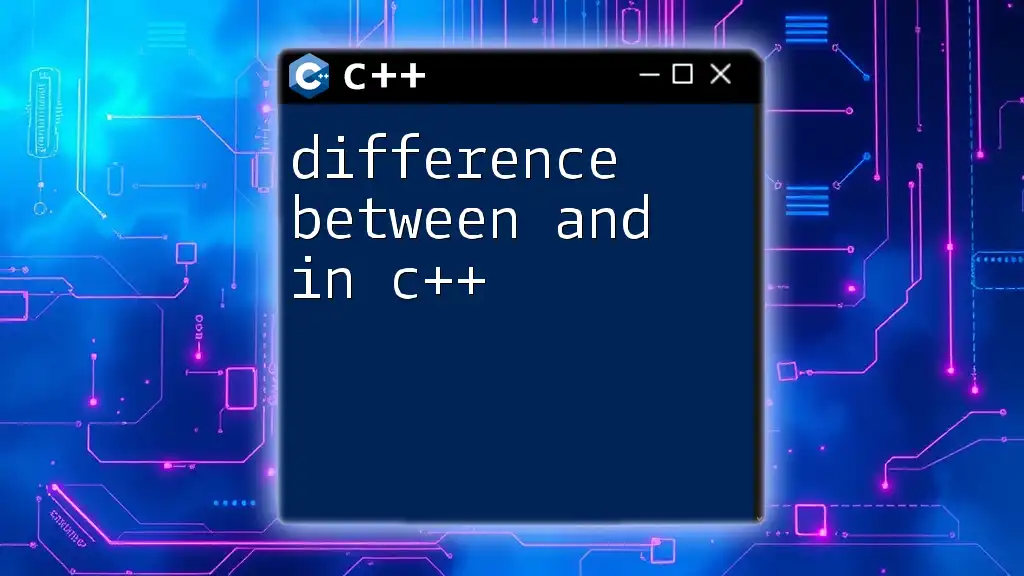
Conclusion
Understanding the difference between C++ and C# is crucial for developers looking to choose the right programming language for their projects. C++ offers unparalleled control and performance, ideal for system-level programming, while C# provides a productive environment for application development with strong support for modern programming concepts.
The choice between C++ and C# should be based on specific project requirements, programming paradigm preferences, and the desired level of control over system resources. Exploring both languages can equip developers with a versatile skill set suitable for a variety of programming challenges.
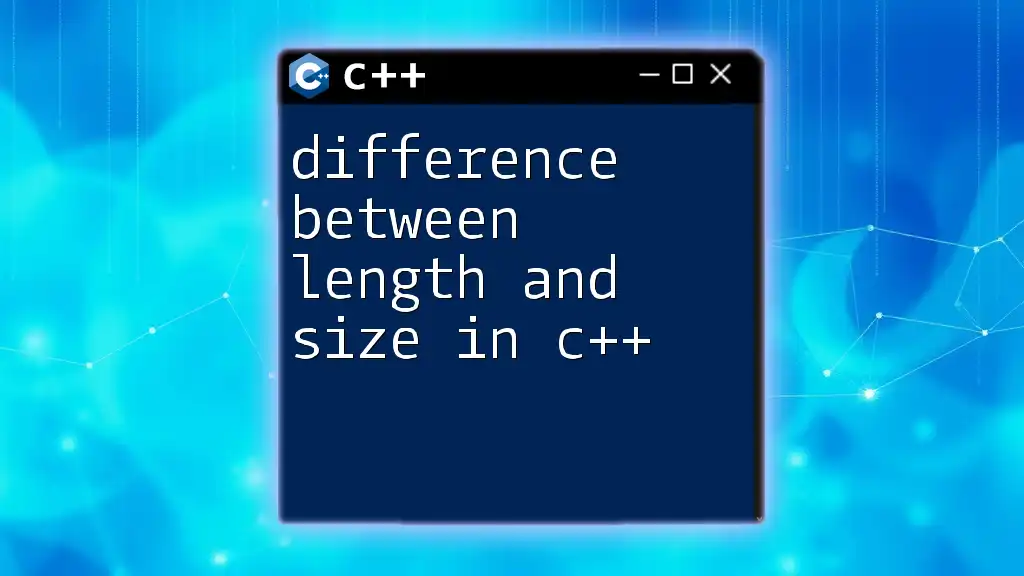
Additional Resources
Recommended Learning Paths
- Various online platforms offer comprehensive courses for both languages, from beginner to advanced levels.
- Books specific to C++ and C#, focusing on best practices, design patterns, and real-world applications, can provide deeper insights into both languages.
FAQs
Common queries often arise regarding the differences between C++ and C#. Beginners may be puzzled by nuances, such as why memory management differs or how the chosen paradigm affects application design. Addressing these questions can clear up misunderstandings and guide developers towards effective learning.