In C++, the `&` operator is used for bitwise AND operations and to obtain the address of a variable, while the `&&` operator represents the logical AND operation used to evaluate boolean expressions.
// Example of bitwise AND and logical AND
int a = 5; // 0101 in binary
int b = 3; // 0011 in binary
int bitwise_result = a & b; // bitwise AND result is 1 (0001)
bool x = true;
bool y = false;
bool logical_result = x && y; // logical AND result is false
Understanding Operators in C++
What are Operators?
Operators are special symbols in programming that tell the compiler to perform specific mathematical or logical manipulations on operands. In C++, operators play a crucial role in carrying out calculations, comparisons, and even memory operations.
Types of Operators in C++
C++ has a variety of operators categorized into several groups, including:
- Arithmetic Operators: Used for mathematical operations (e.g., `+`, `-`, `*`, `/`).
- Relational Operators: Used for comparisons (e.g., `>`, `<`, `==`).
- Logical Operators: Used to combine boolean expressions (e.g., `&&`, `||`).
- Bitwise Operators: Used for manipulating bits (e.g., `&`, `|`, `^`).
In this article, we will specifically focus on the bitwise AND operator (`&`) and the logical AND operator (`&&`), examining their functions and the difference between them in C++.
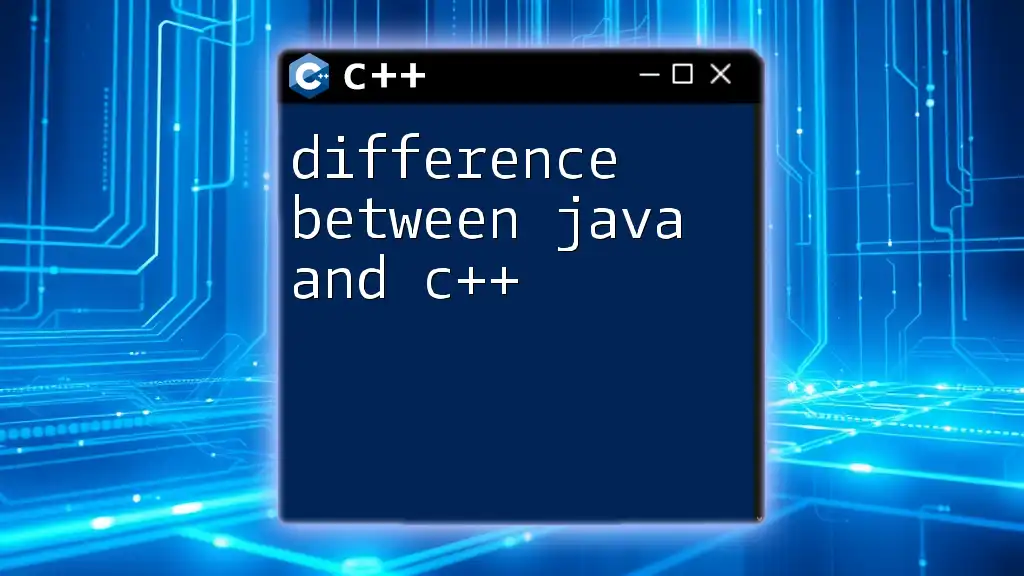
Exploration of `&` Operator
Definition of `&`
The `&` operator in C++ serves two principal functions: it acts as a bitwise AND operator in binary operations and as an address-of operator when applied to variables.
Uses of `&`
Bitwise AND Operator
The bitwise AND operation compares each bit of its operands, returning a new number where bits are set to `1` in the result if both corresponding bits of the operands are also `1`. If either bit is `0`, the result bit is set to `0`.
Example of a Bitwise AND Operation:
int a = 5; // (binary: 0101)
int b = 3; // (binary: 0011)
int result = a & b; // result is 1 (binary: 0001)
In this example, only the least significant bit is set to `1`, resulting in a final value of `1`.
Address-of Operator
The address-of operator retrieves the memory address of a variable. This is particularly useful in pointer manipulation and dynamic memory situations.
Example Showing How to Use the Address-of Operator:
int var = 10;
int* ptr = &var; // ptr contains the address of var
In this code, `ptr` stores the memory address of `var`, allowing for direct manipulation of the variable's value through pointers.
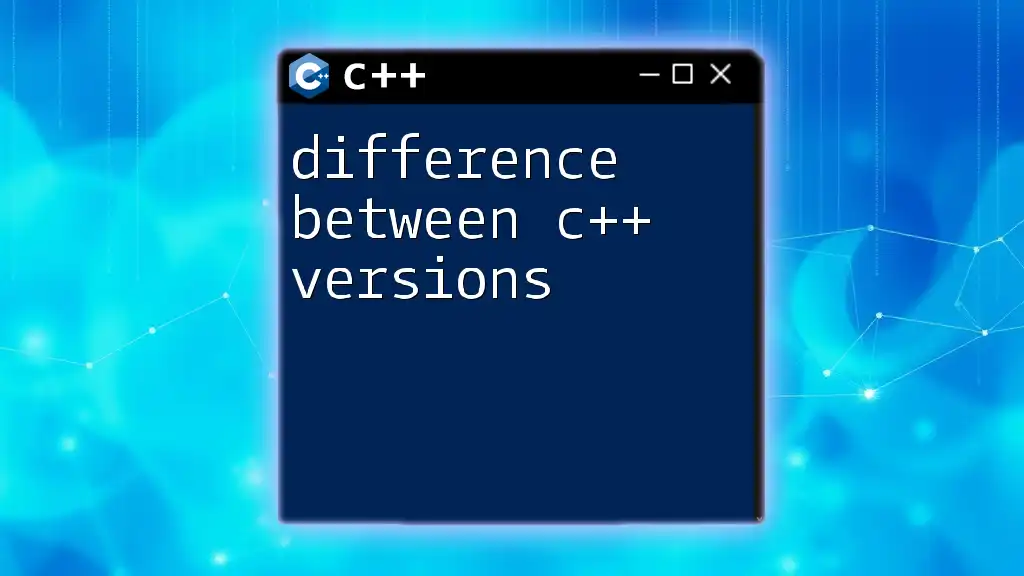
Exploration of `&&` Operator
Definition of `&&`
The `&&` operator is chiefly known as the logical AND operator in C++. It's essential for evaluating boolean expressions, returning `true` only if both operands are `true`.
Uses of `&&`
Logical AND Operator
The logical AND evaluates two boolean expressions and produces a boolean result. If both expressions on either side of the `&&` are true, the overall result is `true`.
Example Illustrating Logical AND Usage:
bool x = true;
bool y = false;
bool result = x && y; // result is false
In this case, the outcome is `false` because one of the operands (`y`) is `false`.
Short-Circuit Evaluation
One key feature of the `&&` operator is short-circuit evaluation. This means if the first operand is `false`, the second operand is not evaluated since the overall result will already be `false`.
Example Illustrating Short-Circuit Evaluation:
int a = 10;
int b = 20;
if (a < 15 && ++b > 25) {
// b is not incremented since the first condition is false
}
Here, because the condition `(a < 15)` is `true`, the second operand `(b > 25)` will not be executed, preserving the original value of `b`.
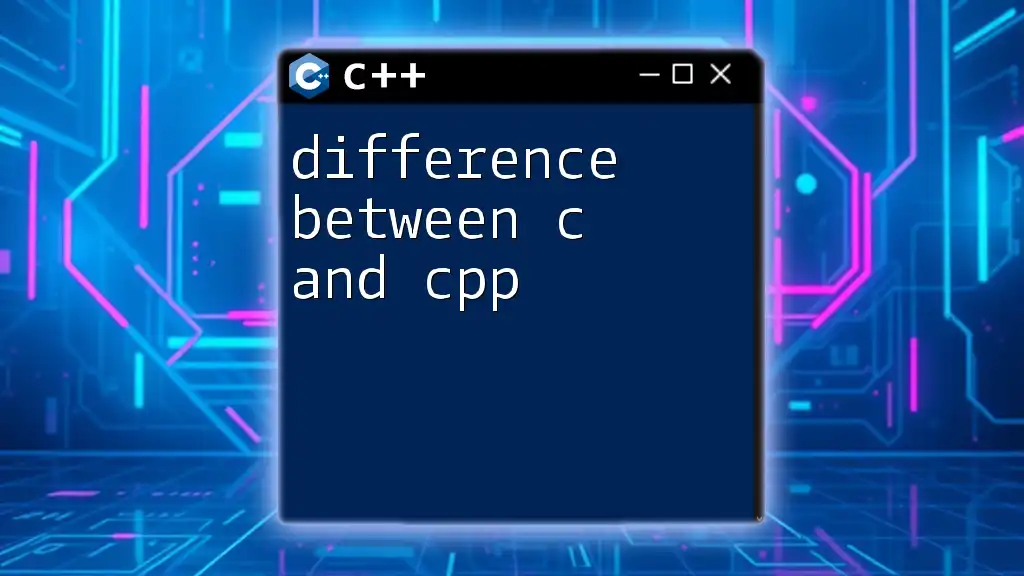
Key Differences Between `&` and `&&`
Context of Use
The critical distinction between `&` and `&&` lies in their context. The `&` operator is used for both bitwise operations and obtaining addresses, while `&&` is exclusively a logical operator for boolean evaluations.
Performance Considerations
In terms of performance, the bitwise AND operation (`&`) executes without any conditional checks, meaning it evaluates both operands entirely. Conversely, the logical AND (`&&`) can terminate the evaluation early if the first condition proves to be false, which can save time in more complex expressions.
Behavior in Different Scenarios
To illustrate the difference further, consider the following examples:
Using `&`:
int x = 6; // (binary: 0110)
int y = 3; // (binary: 0011)
int bitwise_res = x & y; // Output is 2 (binary: 0010)
This expression evaluates the bitwise AND between `x` and `y`, yielding `2`.
Using `&&`:
bool success = (x > 5) && (y < 5); // Evaluated as true or false
In this case, the logical AND operation checks the validity of both conditions, determining the boolean outcome.
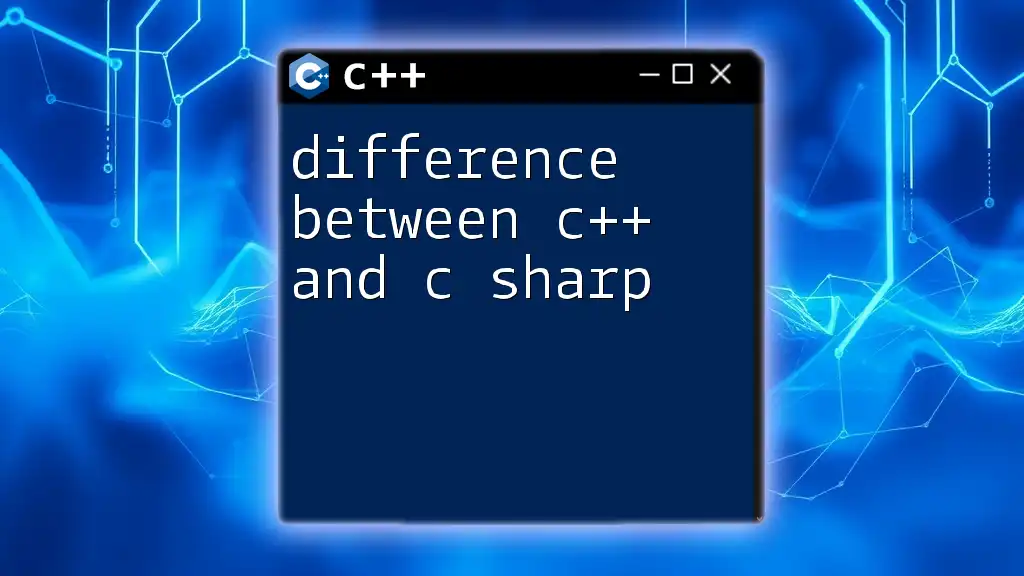
Common Mistakes When Using `&` and `&&`
Misunderstanding the Context
A common misunderstanding occurs when programmers mistakenly use `&` instead of `&&` in boolean contexts or vice versa. This can lead to unexpected results, especially when dealing with conditions in `if` statements or loops.
Debugging Tips
To mitigate confusion:
- Always check the context of the code to ensure the correct operator is used.
- Utilize compiler warnings and errors that can point out type mismatches or logical errors.
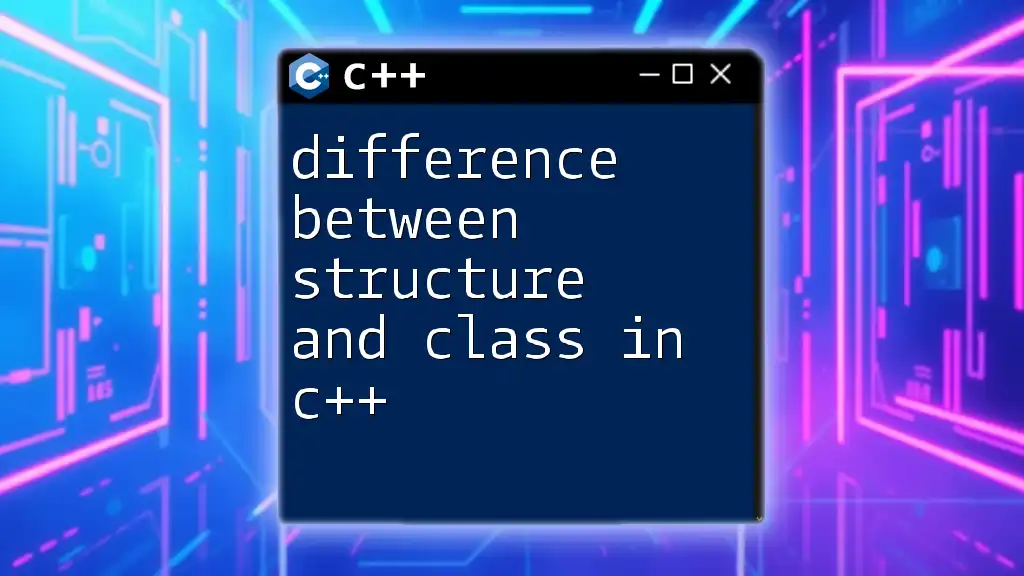
Conclusion
Understanding the difference between `&` and `&&` in C++ is fundamental for efficient programming. The `&` operator has its own use cases in bitwise operations and addressing, while `&&` caters to logical operations, enhancing code readability and efficiency through short-circuit evaluation.
Developing a strong grasp of these operators will undoubtedly improve your coding ability in C++. Practice various scenarios to solidify your understanding and become proficient in manipulating data and performing evaluations accurately.