In C++, `size()` returns the number of elements in a container while `length()` is typically used with strings to return the number of characters, although for standard containers like `std::vector`, `length()` is not applicable and `size()` should be used instead.
#include <iostream>
#include <vector>
#include <string>
int main() {
std::string str = "Hello, World!";
std::vector<int> vec = {1, 2, 3, 4, 5};
std::cout << "Length of string: " << str.length() << std::endl; // Returns 13
std::cout << "Size of vector: " << vec.size() << std::endl; // Returns 5
return 0;
}
What is the C++ Standard Library?
The C++ Standard Library is a powerful set of classes and functions that streamline many programming tasks. It includes key components such as containers, algorithms, and input/output facilities. Among these, containers like `std::vector`, `std::list`, and `std::string` are crucial for managing collections of values or characters. Understanding how to effectively utilize these containers is foundational for writing efficient C++ programs. Key to this understanding are the concepts of length and size, which play a significant role in the manipulation of these containers.
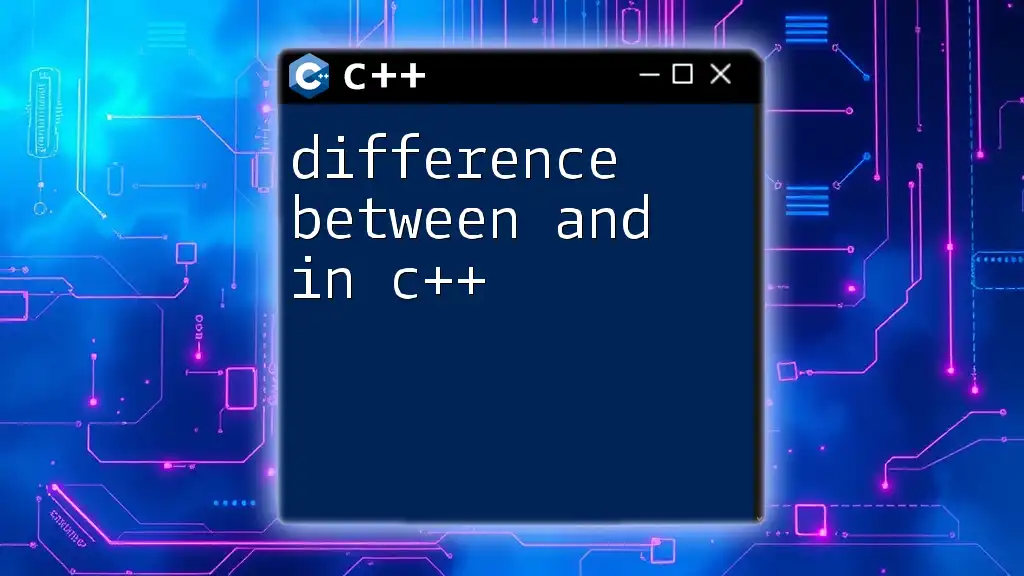
Understanding Length and Size in C++
What is Size?
In C++, size refers to the number of elements in a container. The size() method is implemented across a wide range of standard containers, and it returns the current number of elements held in that container.
For example, when working with a vector, calling `size()` provides an immediate understanding of how many items exist in that vector. Here's a simple code snippet:
#include <iostream>
#include <vector>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
std::cout << "Size of the vector: " << numbers.size() << std::endl; // Output: 5
return 0;
}
This code initializes a vector and retrieves its size using the size() method, printing the result to the console.
What is Length?
On the other hand, length is primarily associated with strings in C++. The length() method retrieves the number of characters in a string. While you may find other container types that also have the length() method, its primary association remains with the `std::string` class.
Here’s an example of how to use length() with a string:
#include <iostream>
#include <string>
int main() {
std::string text = "Hello, World!";
std::cout << "Length of the string: " << text.length() << std::endl; // Output: 13
return 0;
}
In this case, the `length()` method returns the number of characters in the provided string, including spaces and punctuation. It’s crucial to note that despite seemingly similar functionality, length and size serve different types of data structures.
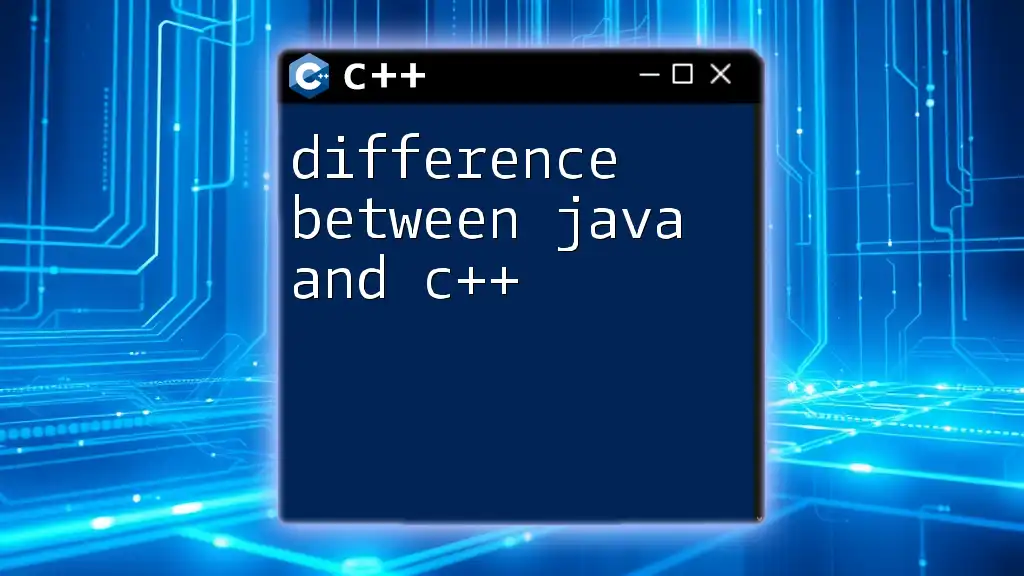
Comparing Length and Size in C++
Size vs Length: Key Differences
One of the most fundamental aspects of professional C++ programming lies in recognizing the differences between size and length. While length() is tailored for strings, size() is applicable across various containers, including vectors, lists, and arrays.
When utilizing these methods, consider the following:
-
Usage Context:
- Use size() for standard containers like `std::vector`, `std::list`, etc.
- Use length() specifically with `std::string`, although size() can also be used with it.
-
Return Value:
- Both methods return a value of type `size_type`, which typically is an unsigned integer.
Practical Code Comparison
To concretely illustrate the differences, here’s an example that uses both size() and length():
#include <iostream>
#include <vector>
#include <string>
int main() {
std::string str = "Sample text";
std::vector<int> vec = {1, 2, 3};
// Using size and length
std::cout << "Size of vector: " << vec.size() << std::endl; // Output: 3
std::cout << "Length of string: " << str.length() << std::endl; // Output: 11
return 0;
}
In the above code, the size() method is invoked on a vector to retrieve the number of integer elements, while length() is called on the string to determine the number of characters.
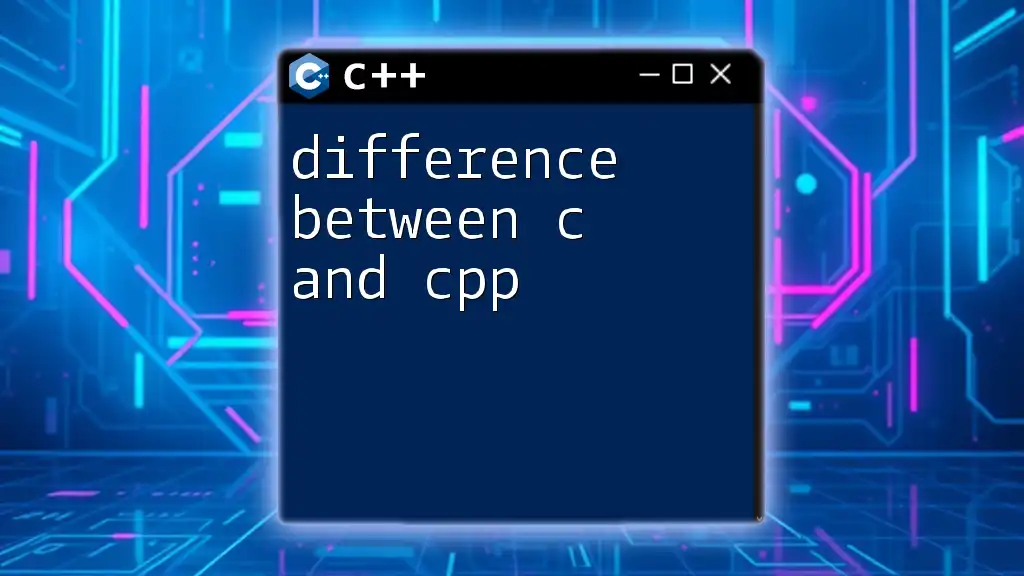
Implications of Using Length and Size
Why Choose One Over the Other?
Understanding when to use size versus length is critical for writing clear and effective code. Use size() to determine the content size for containers like vectors where the concern is often about elements rather than characters. Conversely, choose length() when dealing with strings to clarify your intentions to anyone reading your code, making it evident that you are referring specifically to the character count.
Common Mistakes to Avoid
While using length and size might seem straightforward, developers can occasionally encounter pitfalls:
-
Forgetting to Use the Correct Method: Mistakenly using size() when working with strings can lead to confusion. Stick to length() for strings to maintain code clarity.
-
Type Confusion: Both methods return similar types; however, failing to understand that they may yield different contexts can lead to misinterpretation of data.
-
Mixing Containers: Using length() on a container type that does not support it will result in a compilation error. Ensuring that the type supports the desired method is essential.
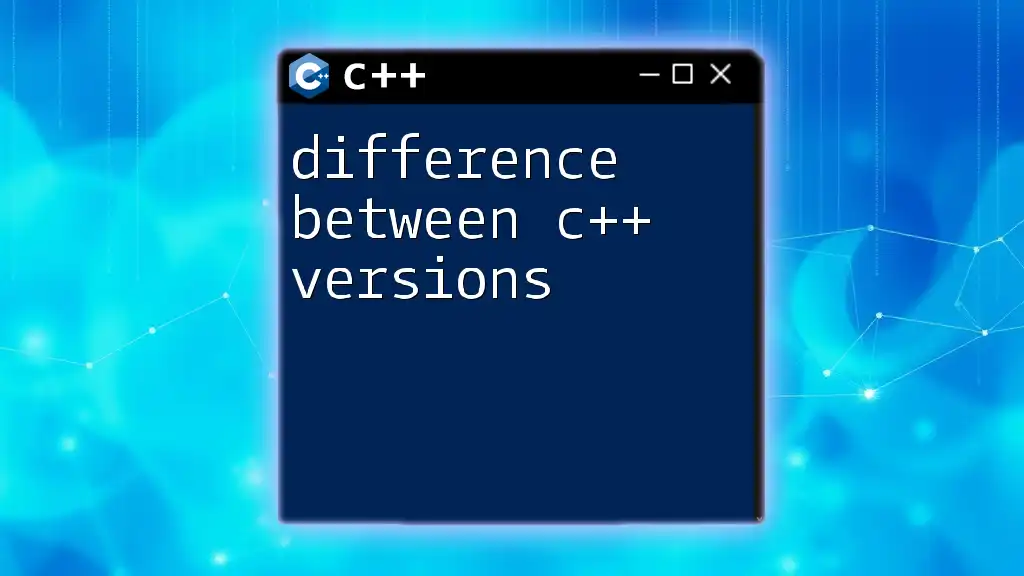
Conclusion
Understanding the difference between length and size in C++ is vital for any programmer aiming to write efficient and readable code. Size() is universally applicable to various C++ containers, while length() is specifically designated for strings, providing clarity in your code's intentions. By grasping these concepts, programmers can write cleaner, more maintainable code and facilitate better communication among peers.
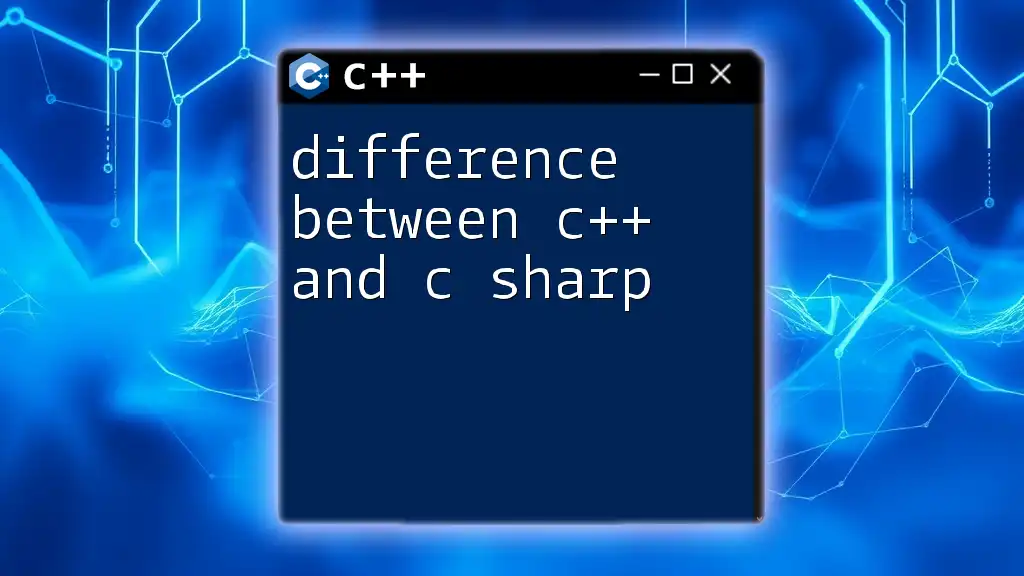
Further Reading and Resources
For those seeking a deeper understanding of these concepts, consider diving into comprehensive C++ resources such as:
- Official C++ documentation
- Online tutorials focused on standard containers
- Books dedicated to C++ programming and best practices
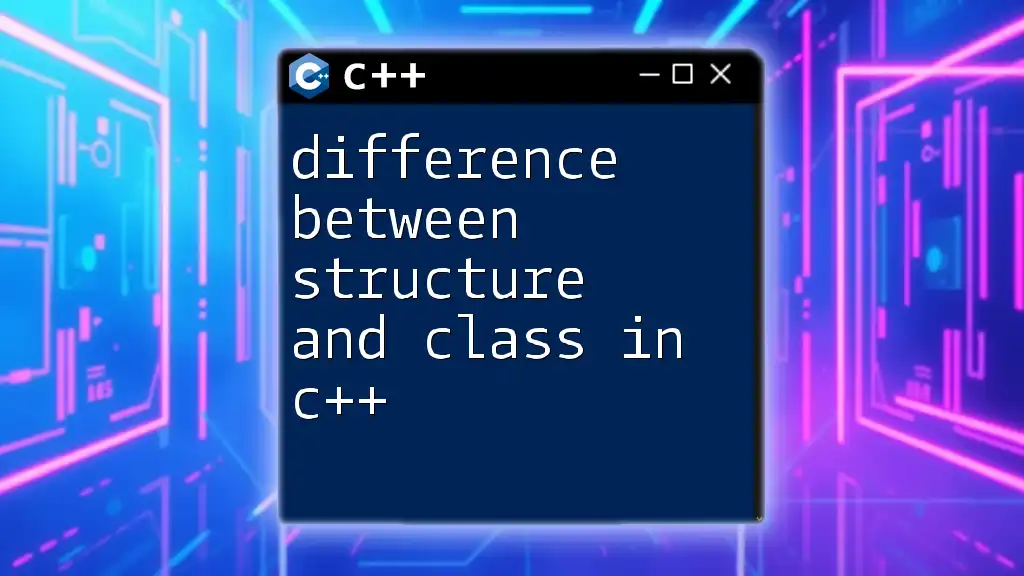
Call to Action
Stay tuned for more tips and tricks on mastering C++, and feel free to reach out for guidance on specific programming challenges or questions relating to efficient code practices!