The `continue` statement in C++ is used within loops to skip the current iteration and continue with the next iteration of the loop.
Here's a simple example:
#include <iostream>
using namespace std;
int main() {
for (int i = 1; i <= 10; i++) {
if (i % 2 == 0) continue; // Skip even numbers
cout << i << " "; // Print odd numbers
}
return 0;
}
What is the Continue Statement?
The continue statement in C++ is a control flow statement that allows you to skip the current iteration of a loop and proceed to the next iteration. This can be particularly helpful when you want to avoid executing certain pieces of code based on specific conditions.
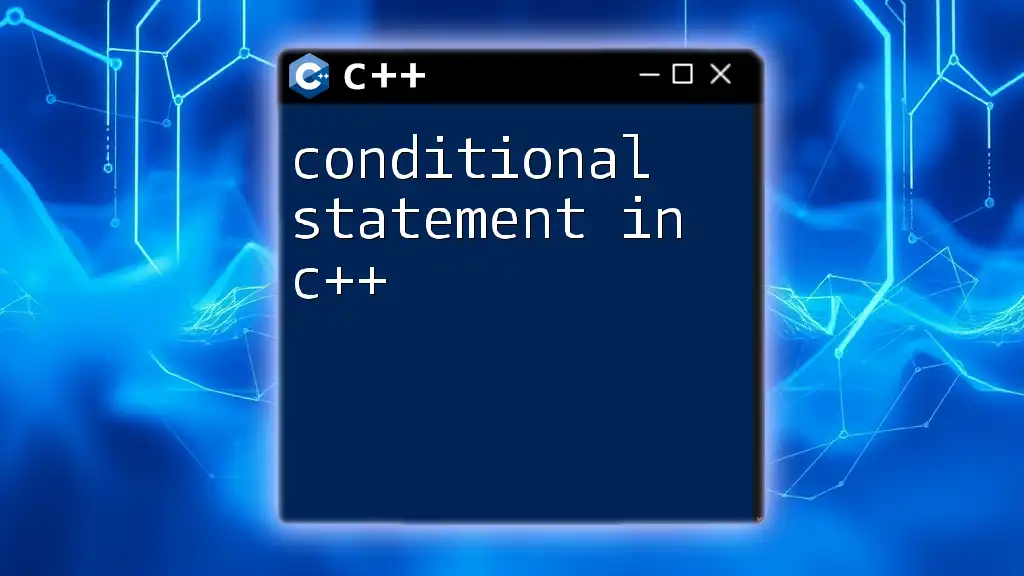
How the Continue Statement Works
The behavior of the continue statement in C++ differs slightly based on the type of loop you are using—whether it's a `for`, `while`, or `do-while` loop. When the `continue` statement is encountered, control is immediately transferred to the next iteration of the loop, and the remaining code in the loop block is skipped for that iteration.
Here’s a simplified flow of control when using the continue statement:
- In a `for` loop, it will skip the part after the `continue` and go directly to the expression that updates the loop variable.
- In `while` and `do-while` loops, it will skip to the condition check of the loop.
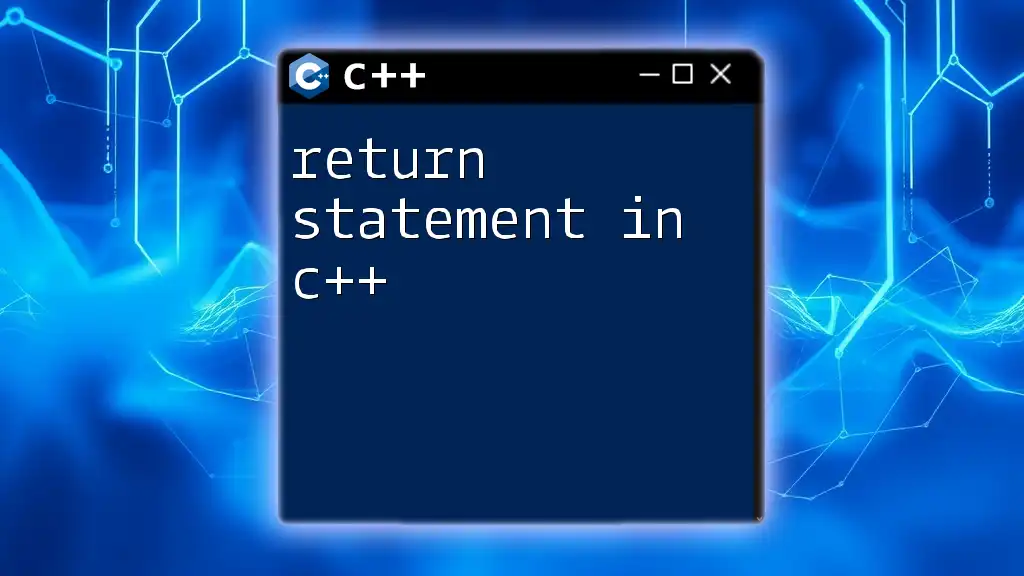
Syntax of the Continue Statement
The syntax for using the continue statement in C++ is straightforward. It simply consists of the word `continue`:
continue;
You can place this statement inside any loop, immediately followed by a condition or any logical structure that guides the flow of your program.
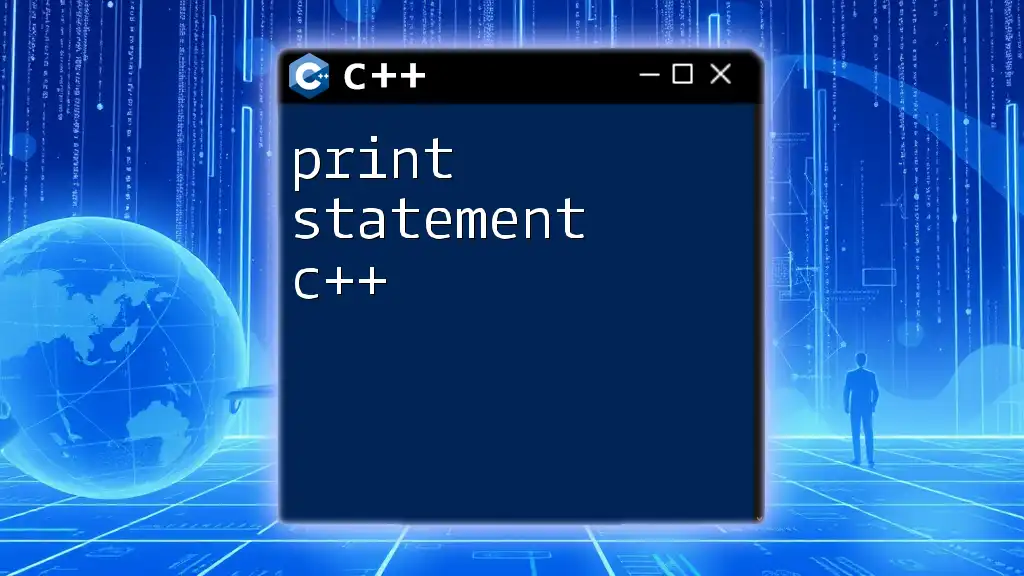
Usage of the Continue Statement
Using Continue in For Loops
Consider this example:
#include <iostream>
using namespace std;
int main() {
for (int i = 0; i < 10; i++) {
if (i % 2 == 0) {
continue; // Skip even numbers
}
cout << i << " "; // Output odd numbers
}
return 0;
}
In this `for` loop, we iterate from 0 to 9. The `if` statement checks if `i` is even using the modulus operator. If it is, the `continue` statement is executed, skipping the `cout` line and moving to the next iteration of the loop. This results in the output of odd numbers only (1, 3, 5, 7, 9).
Using `continue` in a `for` loop helps keep your code clean and clear, especially when you want to filter out specific values from being processed further.
Using Continue in While Loops
Here’s an example of how the continue statement in C++ can be used in a `while` loop:
#include <iostream>
using namespace std;
int main() {
int i = 0;
while (i < 10) {
i++;
if (i % 2 == 0) {
continue; // Skip even numbers
}
cout << i << " "; // Output odd numbers
}
return 0;
}
In this `while` loop, the variable `i` is incremented on each iteration. The `if` statement checks if `i` is even once again. If true, the `continue` statement executes, skipping the `cout` statement and prompting the next iteration immediately. The outcome remains consistent: it prints only odd numbers from 1 to 9.
Using Continue in Do-While Loops
The continue statement in C++ can also be applied in `do-while` loops:
#include <iostream>
using namespace std;
int main() {
int i = 0;
do {
i++;
if (i % 2 == 0) {
continue; // Skip even numbers
}
cout << i << " "; // Output odd numbers
} while (i < 10);
return 0;
}
In this scenario, even though we are using `do-while`, the logic remains the same. The loop ensures that the body executes at least once before the condition is tested. The `continue` statement causes the loop to skip printing even numbers, and thus the output will again be the odd numbers from 1 to 9.
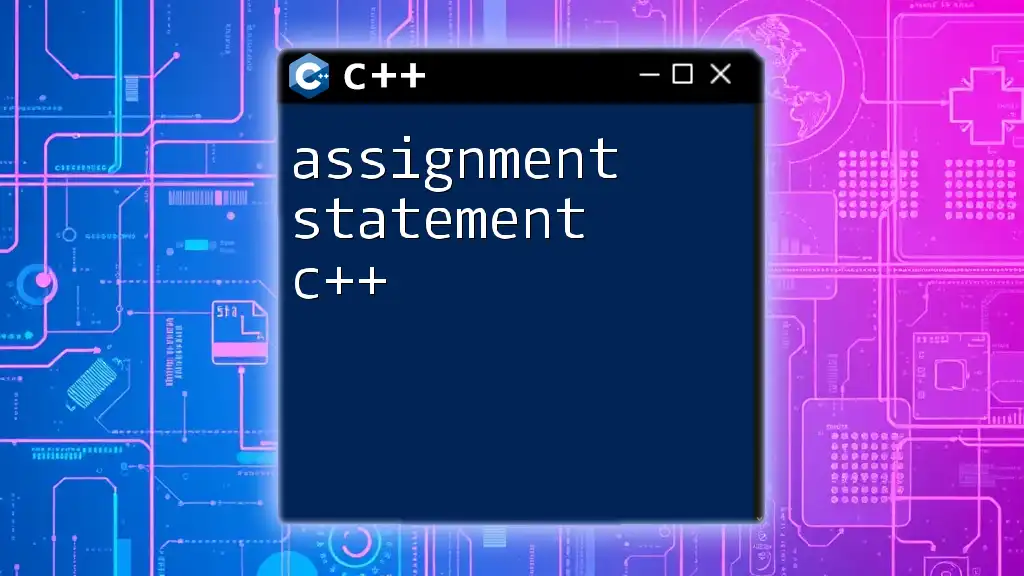
Best Practices for Using the Continue Statement
When employing the continue statement in C++, consider these best practices:
- Use Sparingly: Overusing the `continue` statement can make your code harder to read and debug. Use it only when it results in cleaner logic.
- Keep It Clear: Always aim for clarity. If a `continue` statement makes your logic convoluted, consider refactoring your conditional statements instead.
- Maintain Loop Integrity: Ensure that the loop variable is updated appropriately—failure to do so might lead to infinite loops.
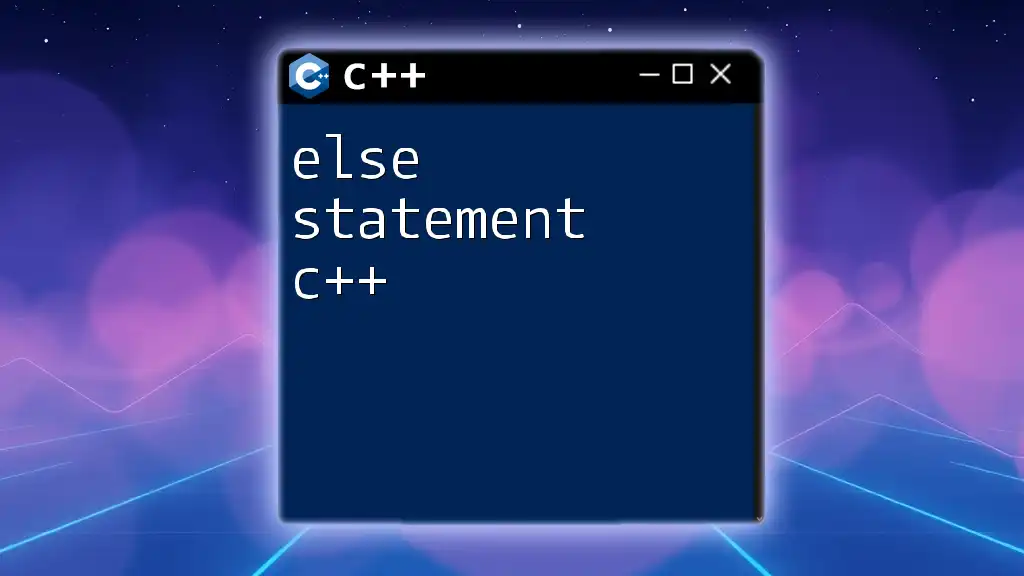
Common Mistakes to Avoid
While using the continue statement in C++ can streamline your code, several pitfalls can occur:
- Inappropriate Context: Using `continue` within nested loops can be confusing. Ensure you are aware of which loop you are affecting.
- Complexity: Avoid excessive nesting of loops with `continue` statements, as this can decrease overall readability.
- Condition Management: Ensure that the conditions you have set up do not lead to scenarios where critical code is consistently skipped unintentionally.
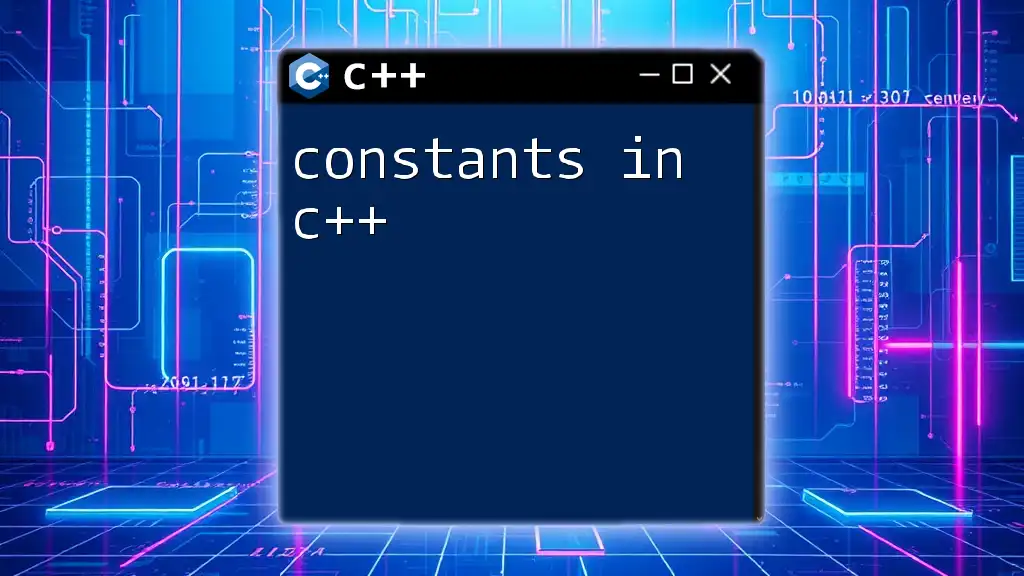
Conclusion
The continue statement in C++ is a powerful tool for managing loop iterations effectively. When used properly, it allows developers to skip unnecessary processing, thus keeping the code clean and efficient. Practice implementing the `continue` statement in various loop constructs to reinforce your understanding and make the most out of your control flow statements.
By internalizing this concept, you'll be better positioned to handle more complex coding scenarios in C++. Don't hesitate to explore various examples and modify them to see how the `continue` statement influences execution flow.