In C++, the print statement is commonly achieved using the `std::cout` stream to output text to the console.
Here's a simple code snippet demonstrating this:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Understanding Basics of Input and Output in C++
In C++, the print statement is fundamental to managing input and output (I/O) operations. Every program requires some form of communication with the user, and understanding how to effectively print data to the console is critical.
Standard Input/Output Streams
C++ uses streams for its input and output operations. The primary streams are:
- `std::cout`: Used for output to the console.
- `std::cin`: Used for input from the user.
To use these streams, you need to include the `<iostream>` header file at the beginning of your program. For example:
#include <iostream>
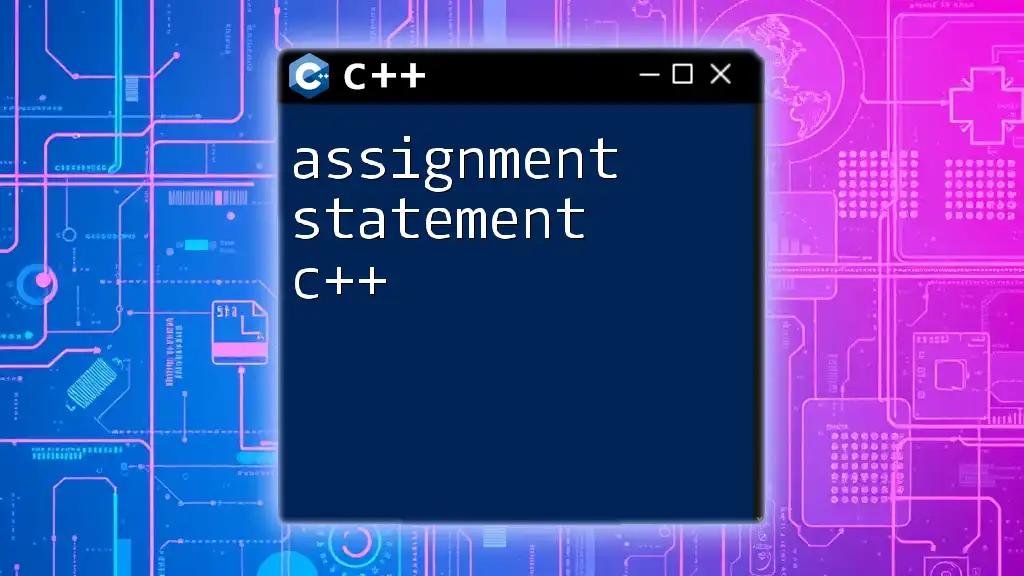
The Syntax of the C++ Print Statement
The syntax of a basic print statement in C++ generally looks like this:
std::cout << "Your text here";
Where:
- `std::cout` indicates that you are using the standard output stream.
- `<<` is known as the stream insertion operator, which directs the output to the specified stream.
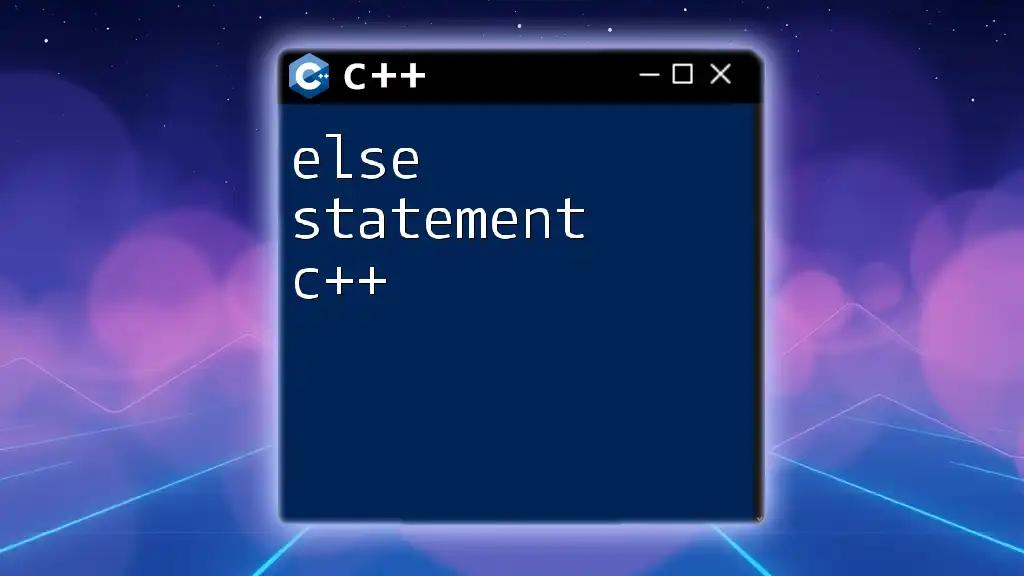
Different Ways to Print in C++
Printing Strings
You can effortlessly print standard strings using the print statement in C++. Here’s a simple example right from the heart of the C++ world:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
In this snippet, `std::endl` is used to insert a newline after printing. This ensures your output is clean and organized.
Printing Numbers
Printing numbers, whether integers or floating-point values, works similarly. Here’s how you can print both types of values:
#include <iostream>
int main() {
int age = 30;
double salary = 50000.50;
std::cout << "Age: " << age << ", Salary: " << salary << "\n";
return 0;
}
In this example, multiple data types are printed in one line by chaining them with the `<<` operator, demonstrating the flexibility of the print statement in C++.
Printing Multiple Values
You can also print several values at once, demonstrating the chaining capability of `std::cout`. For example:
#include <iostream>
int main() {
std::cout << "This is " << 7 << " and " << 3.14 << "\n";
return 0;
}
This illustrates how you can insert a mixture of string literals and numeric values into the output seamlessly.

Formatting Output in C++
Using `std::endl` vs `\n`
Both `std::endl` and `\n` can be used to insert new lines in the output. However, they serve slightly different purposes. While `std::endl` flushes the output buffer, `\n` only creates a new line. Using `\n` is generally more performant in rapid output scenarios where flushing is unnecessary.
Example of both techniques:
#include <iostream>
int main() {
std::cout << "Output using std::endl" << std::endl;
std::cout << "Output using \\n" << "\n";
return 0;
}
Setting Precision and Fixed Format
Control over the formatting of numeric output is crucial, especially when dealing with floating-point numbers. You can set the precision of your output using the `<iomanip>` library. Here’s an example that sets the precision of a floating-point number:
#include <iostream>
#include <iomanip>
int main() {
double pi = 3.14159265359;
std::cout << std::fixed << std::setprecision(2) << pi << "\n";
return 0;
}
In this case, `std::fixed` ensures that the number is printed in a fixed decimal format, while `std::setprecision(2)` limits the output to two decimal places.

Advanced Printing Techniques
Printing Special Characters
Printing special characters may require some knowledge of escape sequences. For example, to include quotes or backslashes in the output, you can use:
std::cout << "She said, \"Hello!\" and I replied, \"Hi!\"\n";
Double quotes are escaped with a backslash (`\`) to prevent confusion with string delimiters.
Using Escape Sequences
Escape sequences are a powerful feature for controlling complex formatting in C++. Here are some common ones:
- `\n`: New line
- `\t`: Tab
- `\\`: Backslash
Here’s an example that utilizes escape sequences:
#include <iostream>
int main() {
std::cout << "Column1\tColumn2\nValue1\tValue2\n";
return 0;
}
This demonstrates how to create a tabulated layout in the console output.

Error Handling in Output Statements
While the print statement itself typically doesn’t directly raise errors, you can check for issues related to output buffering or stream states. Using the stream's `fail()` function can help manage error states gracefully:
#include <iostream>
int main() {
if (std::cout.fail()) {
std::cerr << "Output error occurred!" << std::endl;
} else {
std::cout << "Output is successful!" << std::endl;
}
return 0;
}
Here, `std::cerr` is used to print error messages to the console when issues arise.
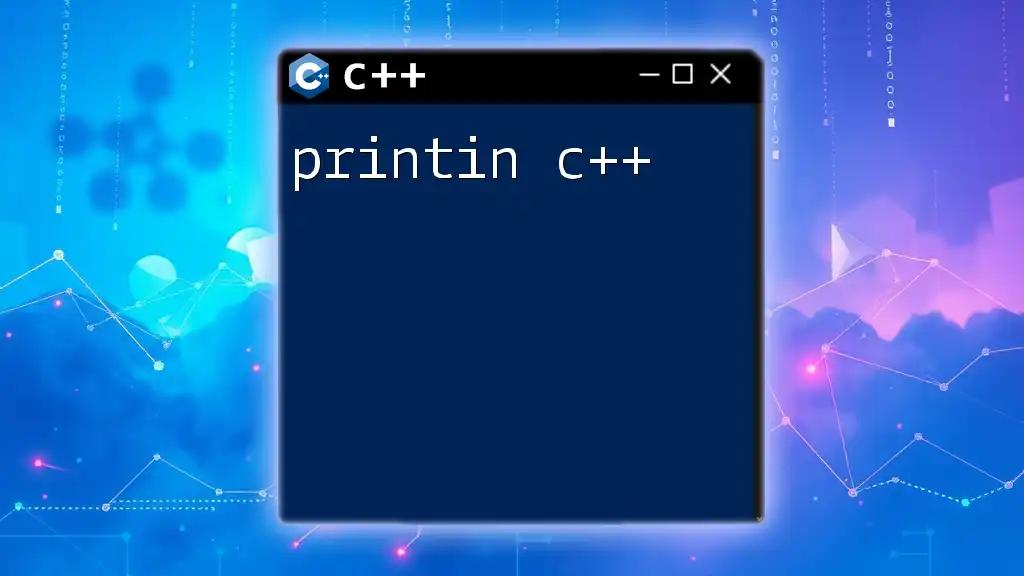
Conclusion
Mastering the print statement in C++ is essential for effective data output and user interaction in your programs. With a solid understanding of `std::cout`, formatted printing, and advanced techniques, you will enhance your coding capabilities significantly. Continuous practice with various examples will help solidify your knowledge.
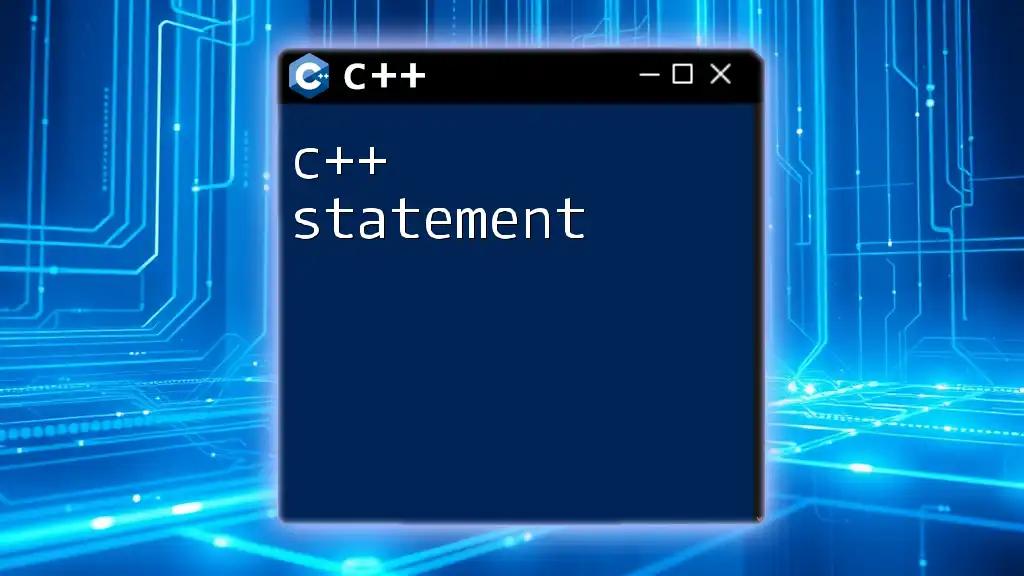
Additional Resources
To further your learning, consider exploring recommended books, online courses, and coding platforms dedicated to C++. Engaging with multiple resources will widen your understanding of C++ programming and its nuances.
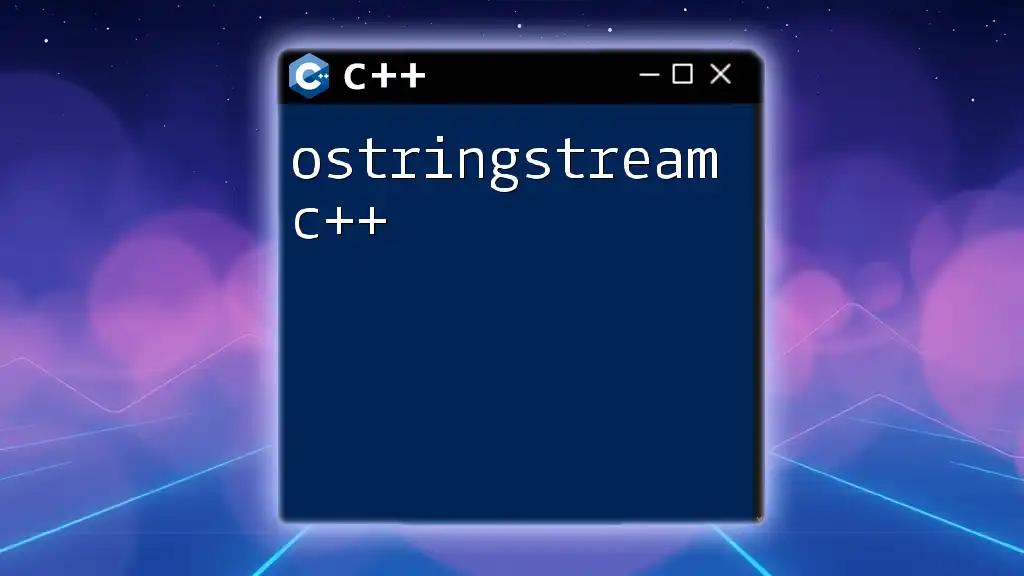
FAQs about Printing in C++
-
What is the difference between `std::cout` and `printf`?
- While both can print to the console, `printf` is a part of the C library and uses format specifiers, whereas `std::cout` is C++-style output and utilizes stream insertion.
-
Can I print objects using `std::cout`?
- Yes, but you must overload the `<<` operator for custom types to define how they should be represented as output.
With these insights, you are ready to utilize C++ print statements effectively in your projects!