An assignment statement in C++ is used to assign a value to a variable using the assignment operator (`=`).
Here’s an example:
int number = 5;
Understanding Assignment Statements
What is an Assignment Statement?
An assignment statement in programming is a fundamental operation that assigns a value to a variable. It is essential for storing and manipulating data throughout a program. In C++, the assignment statement uses the single equals sign (`=`) as an operator to set the value of a variable.
Syntax of an Assignment Statement in C++
The general syntax for an assignment statement in C++ can be illustrated as follows:
variable = expression;
Here’s a breakdown of each component:
- Variable: This is the name of the storage location that will hold the value.
- Operator: In this case, it is the assignment operator `=`, which assigns the value on its right to the variable on its left.
- Expression: This is any valid expression that results in a value, which could be a constant, variable, or the result of a calculation.
For example:
int x = 5;
In this statement, the integer variable `x` is being assigned the value `5`.
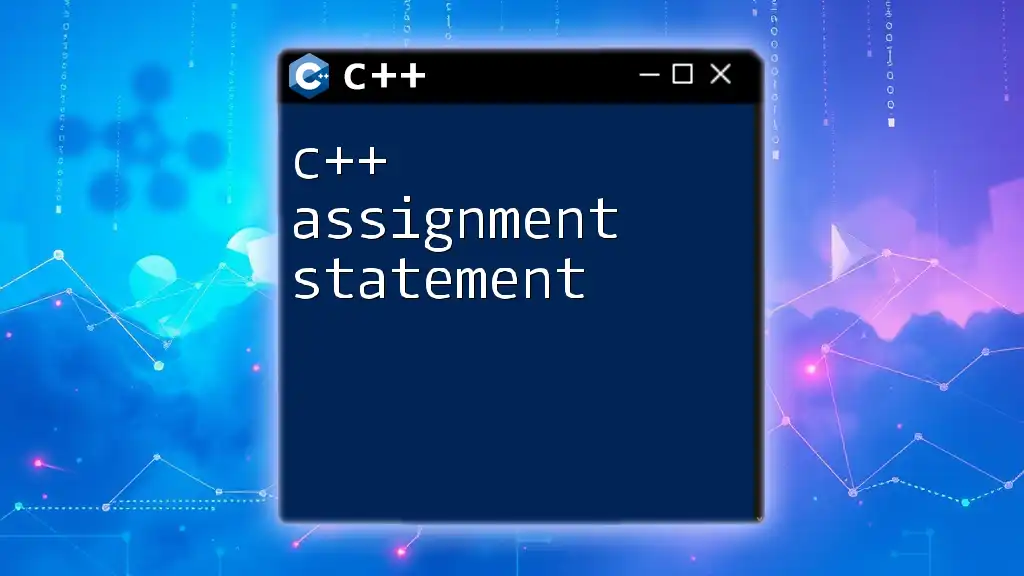
Types of Assignment Statements
Simple Assignment
Simple assignment assigns a single value to a variable. In C++, this is straightforward and looks like this:
int a = 10;
float b = 5.5;
In this example:
- The integer variable `a` is assigned the value `10`.
- The floating-point variable `b` is assigned the value `5.5`.
Compound Assignment
Compound assignment combines the assignment with an arithmetic operation. C++ provides several compound assignment operators, which include `+=`, `-=`, `*=`, and `/=`.
For instance, consider the compound assignment with addition:
int c = 10;
c += 5; // c is now 15
In this example, `c` is increased by `5`. The expression `c += 5` is equivalent to `c = c + 5`.
Multiple Assignments
C++ also allows multiple assignments in one statement. This can streamline code but should be used with care to maintain clarity.
int x, y, z;
x = y = z = 0;
In this statement, all three variables, `x`, `y`, and `z`, are assigned the value of `0` in a single line. The assignments are evaluated from right to left.
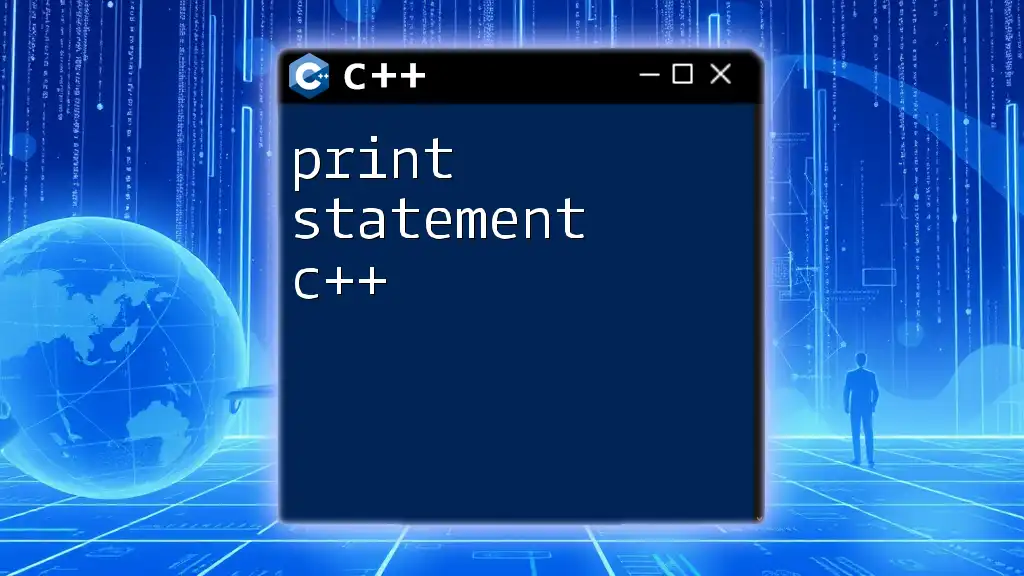
Special Assignment Scenarios
Assignment with Expressions
Expressions can also be assigned to variables. When using expressions, the calculation is processed before the assignment takes place.
int result = (5 + 3) * 2; // result is 16
Here, the expression `(5 + 3)` is calculated first, resulting in `8`, which is then multiplied by `2` to give `16` before being assigned to `result`.
Assignment with Function Returns
A C++ assignment statement can also work with function return values. When a function returns a value, it can be directly assigned to a variable.
int getValue() { return 42; }
int value = getValue(); // value is now 42
In this snippet, the function `getValue()` returns `42`, which is then assigned to the variable `value`.
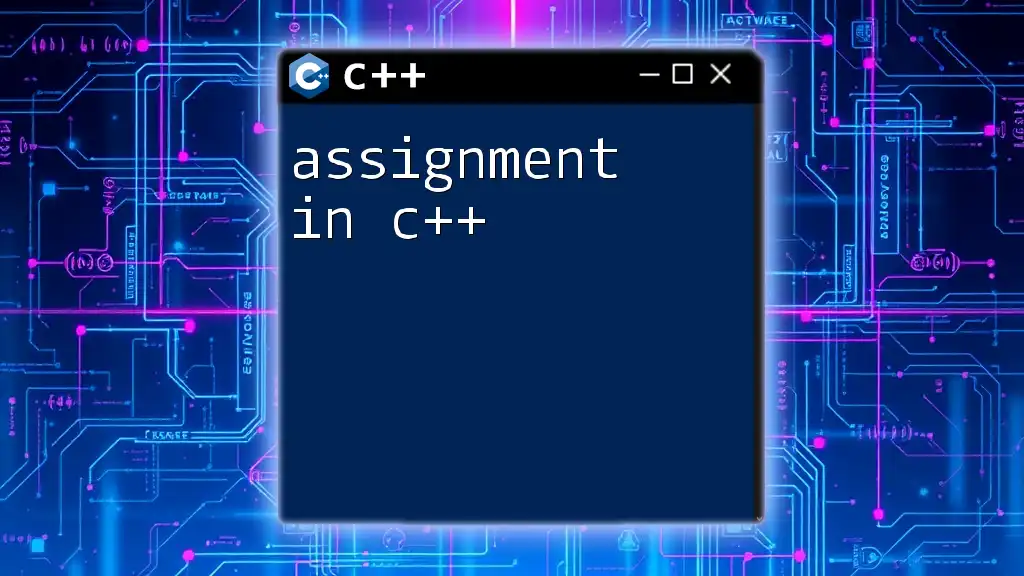
Common Errors with Assignment Statements
Type Mismatches
One of the most common pitfalls when using assignment statements is a type mismatch, where the variable type does not match the type of the assigned value.
int a = 10;
// a = 3.14; // Error: cannot assign a float to an int
In this scenario, attempting to assign a `float` value to an `int` variable will result in a compilation error, emphasizing the importance of ensuring that data types align.
Use of Assignment vs. Equality
Another frequent mistake involves confusing assignment (`=`) with equality checking (`==`). This error can lead to unintended behavior within conditional statements.
int b = 5;
if (b = 10) { /* This assigns 10 to b instead of comparing */ }
In this code, rather than checking if `b` is equal to `10`, the statement actually assigns `10` to `b`. Understanding the distinction is crucial for avoiding logical errors.
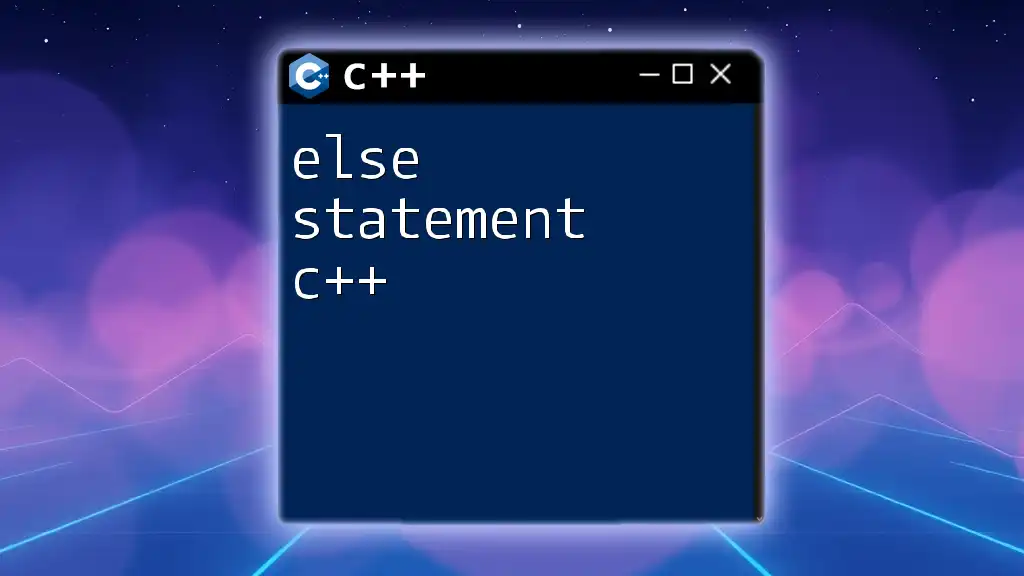
Best Practices for Declaring Assignment Statements
Use of Meaningful Variable Names
Choosing clear, descriptive variable names enhances the readability and maintainability of the code. For example:
int totalScore = 100; // Meaningful context is clear
In this case, `totalScore` provides insight into what the variable represents.
Code Commenting
Including comments to explain complex assignment statements can prevent confusion and aid understanding for future readers or collaborators.
// Calculate the total price after tax
float totalPrice = basePrice + (basePrice * taxRate);
The comment clarifies the intention behind the calculation, making it easier for someone reading the code to understand the logic.
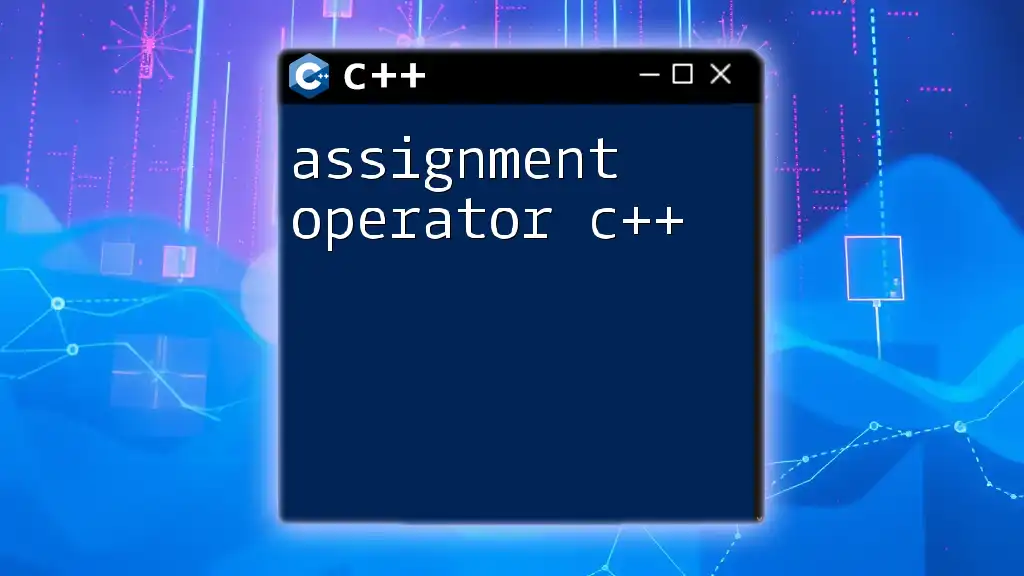
Conclusion
Assignment statements in C++ are a foundational aspect of programming, enabling developers to store and manipulate data effectively. Whether through simple or compound assignments, and even in assignments involving expressions or function returns, understanding how to use these statements is essential to writing robust C++ code.
Continued learning and practice are encouraged to master the nuances of assignment statements and their role within the broader context of C++ programming.
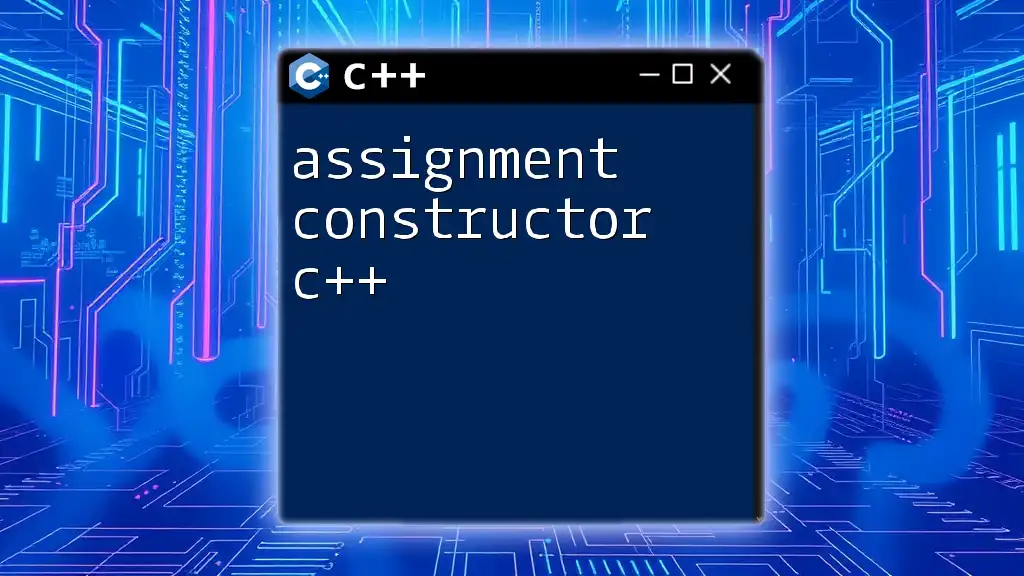
Additional Resources
For further exploration, consider checking C++ documentation and interactive tutorials, which can provide deeper insight and hands-on experience. Community forums are also excellent places to ask questions and share knowledge on the intricacies of assignment statements in C++.