In C++, alignment refers to the way data is arranged and accessed in memory, and it can be controlled using the `alignas` specifier to ensure that variables are aligned to specific byte boundaries for performance optimization.
Here's a code snippet demonstrating the use of `alignas`:
#include <iostream>
#include <cstdalign>
alignas(16) int alignedArray[4]; // Aligns the array to a 16-byte boundary
int main() {
std::cout << "Alignment of alignedArray: " << alignof(alignedArray) << " bytes\n";
return 0;
}
Understanding Memory Alignment
What is Memory Alignment?
Memory alignment is a critical concept in C++ programming, referring to how data is arranged and accessed in memory. Most CPUs operate more efficiently when data is located at specific memory addresses that are multiples of a particular size, known as the alignment. For instance, a 4-byte integer is ideally stored at addresses that are multiples of 4 (0x0, 0x4, 0x8, etc.).
The Basics of Data Alignment
In C++, each data type is associated with a default alignment requirement based on its size. This is consistent across different platforms, though the exact alignment can vary. The concept of "natural alignment" suggests that data types should be aligned in a way that their addresses are multiples of their size. For example:
- `char` is aligned to 1 byte
- `int` is aligned to 4 bytes
- `double` is often aligned to 8 bytes
Proper alignment helps in optimizing RAM usage, making programs run faster and more efficiently by enabling the CPU to fetch data from memory in an optimal way.
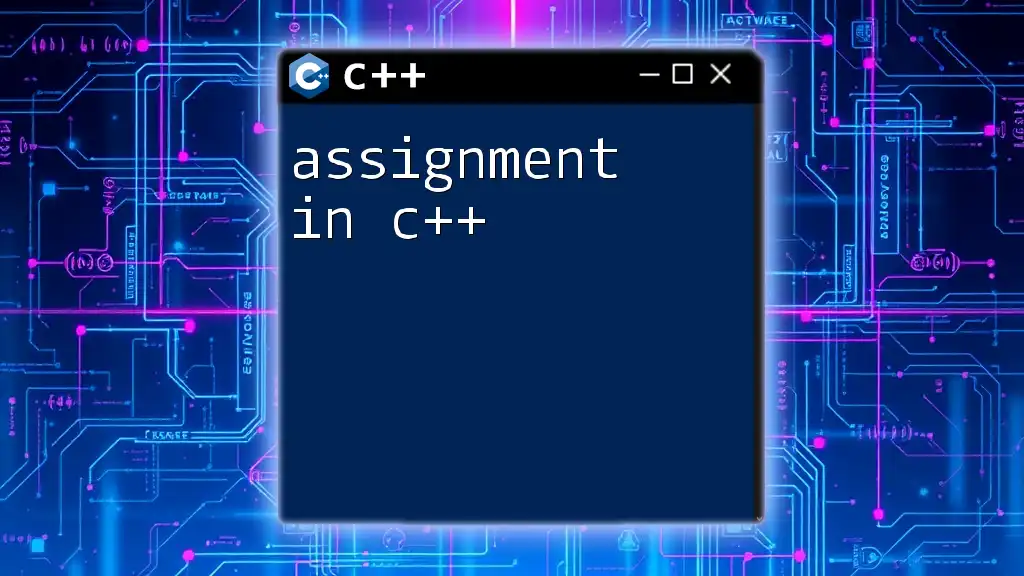
C++ Alignment Concepts
C++ Alignment Attributes
C++ provides specific alignment attributes that allow programmers to control how their data is aligned in memory precisely. Two key attributes to be aware of are `alignas` and `alignof`.
Example Code: Using `alignas`
You can specify the alignment of a variable or structure using `alignas`. Here’s how it works:
alignas(16) double myDouble;
In this example, `myDouble` is guaranteed to be aligned on a 16-byte boundary, ensuring that it can be accessed quickly by the CPU.
The `alignof` Operator
Using `alignof`, you can query the alignment requirements of any type or variable in C++. This operator returns the alignment value in bytes.
Example Code: Finding the Alignment of Data Types
#include <iostream>
std::cout << "Alignment of int: " << alignof(int) << std::endl;
std::cout << "Alignment of double: " << alignof(double) << std::endl;
This snippet will print out the alignment requirements for `int` and `double`, typically revealing that `int` might require 4 bytes while `double` may require 8 bytes.
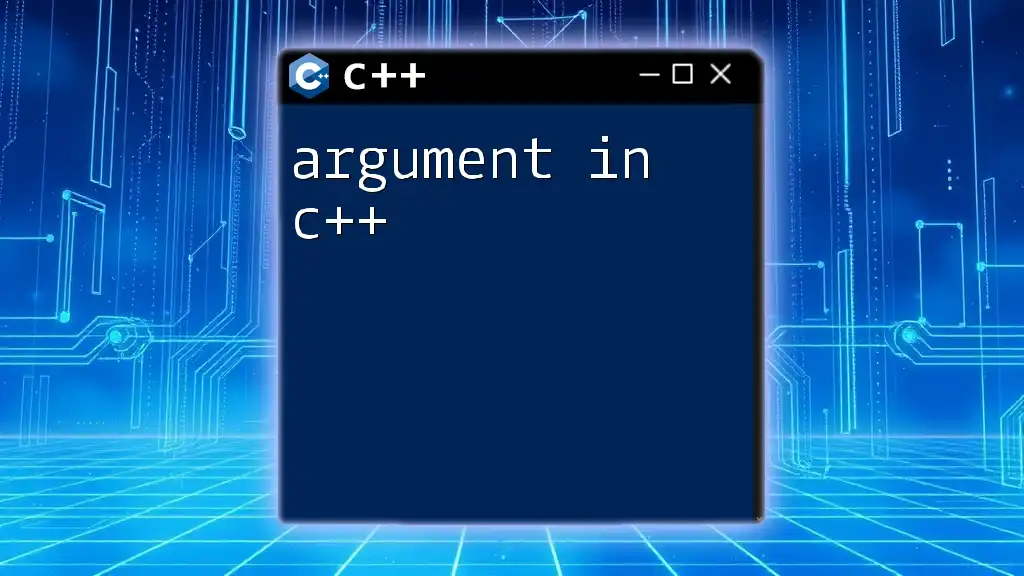
Advanced Alignment Techniques
Custom Alignment
Custom alignment allows developers to impose specific alignment constraints for their types and objects. This can enhance performance in certain applications, especially those dealing with hardware-level programming or performance-critical applications.
Example Code: Custom Struct Alignment
You can define a structure with a custom alignment by using `alignas`:
struct alignas(32) MyStruct {
int x;
double y;
};
In this example, `MyStruct` is aligned to a 32-byte boundary, which can be beneficial for SIMD (Single Instruction, Multiple Data) operations.
Working with Memory Alignment in Classes
When working with class members, understanding alignment becomes paramount. Class inheritance can introduce additional alignment concerns as the derived classes inherit the base class's alignment.
Example Code: Class Member Alignment
Here's how to manage alignment in class hierarchies:
class Base {
alignas(64) int data;
};
class Derived : public Base {
alignas(16) double value;
};
In this scenario, `Base` is aligned to 64 bytes, while `Derived` maintains its own alignment requirement of 16 bytes for its `value`.
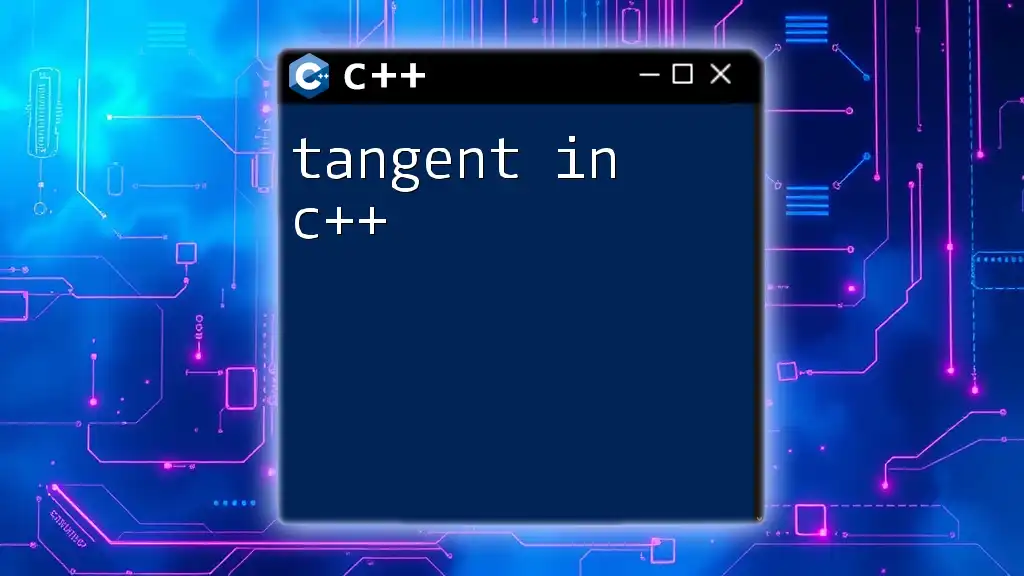
Memory Management and Alignment
Alignment and Dynamic Memory
Alignment is also crucial when allocating memory dynamically. If alignment issues arise in dynamic memory, accessing misaligned data can lead to performance penalties or even runtime errors.
Example Code: New/Delete with Alignment
When allocating aligned memory, you use:
double* aligned_ptr = new(std::align_val_t{64}) double[10];
This code snippet shows how to allocate a dynamically sized array of doubles that is aligned to 64 bytes.
Using `std::aligned_alloc`
Introduced in C++17, `std::aligned_alloc` offers a standardized way to allocate memory with specific alignment constraints.
Example Code: Allocating Aligned Memory
An example usage of `std::aligned_alloc` is as follows:
#include <cstdlib>
void* ptr = std::aligned_alloc(64, 1024);
This allocates 1024 bytes of memory with a required alignment of 64 bytes.
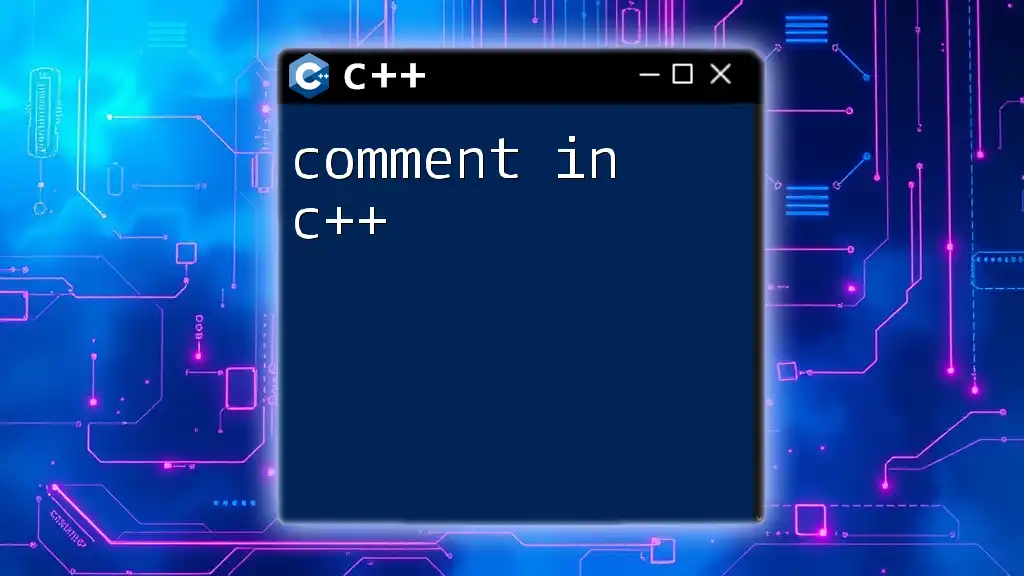
Performance Implications of Data Alignment
How Misalignment Affects Performance
Data misalignment can severely degrade performance. When a CPU accesses misaligned data, it may incur additional cycles to read the data compared to aligned accesses. Misaligned accesses can disrupt cache line utilization, leading to inefficiencies during memory operations.
Profiling and Measuring Alignment Effects
Tools such as profilers can help detect alignment issues. By analyzing memory allocation patterns, developers can find and refactor misaligned data to enhance performance. A careful examination of memory usage often reveals areas where slight adjustments to alignment can yield significant performance gains.
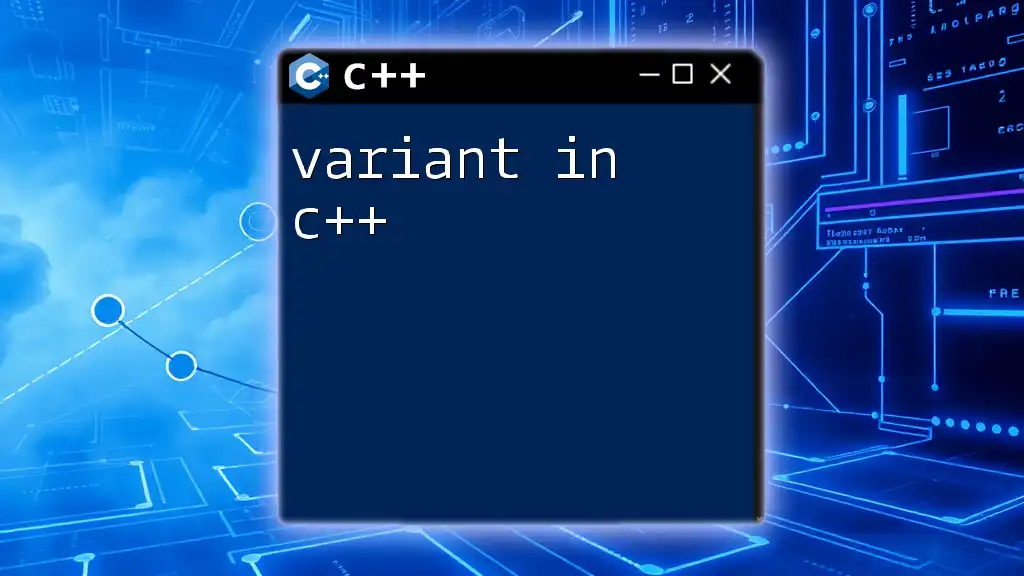
Best Practices for Alignment in C++
Guidelines for Using Alignment Attributes
- Use `alignas` for defining specific alignment where necessary, especially in performance-sensitive applications.
- Regularly check alignment with `alignof` to ensure types meet your performance requirements.
- Avoid excessive custom alignment, as it may introduce complex behavior and platform-specific issues.
Future-Proofing Your Code for Alignment
As hardware progresses, it's vital to consider future compatibility when hardcoding alignment specifications. Adopting flexible coding practices while maintaining awareness of alignment rules across platforms will help ensure software longevity.
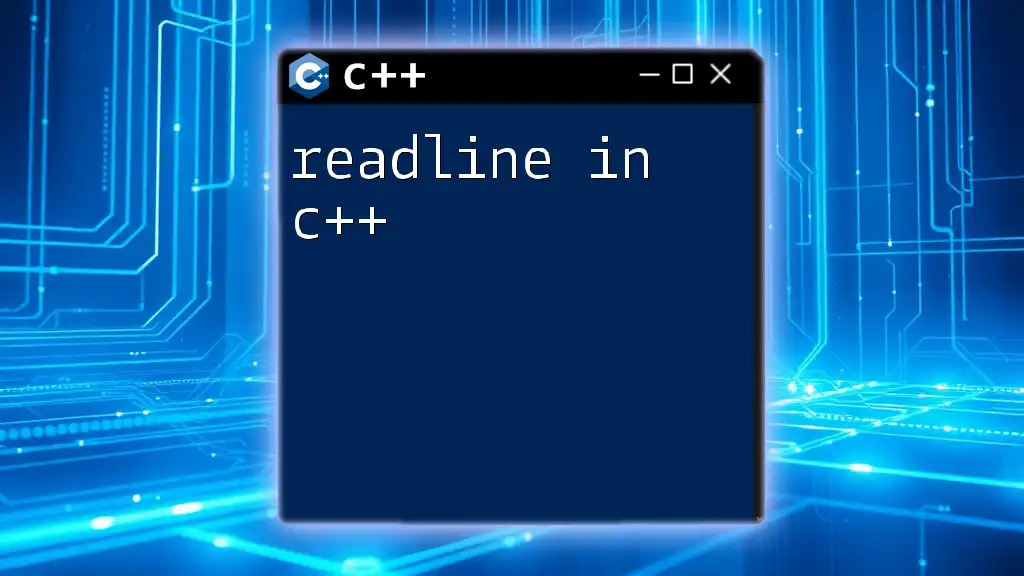
Conclusion
Alignment in C++ constitutes an essential aspect of efficient programming, deeply impacting performance and memory management. By understanding and applying best practices concerning alignment attributes, developers can optimize their code, ensuring both correctness and speed.
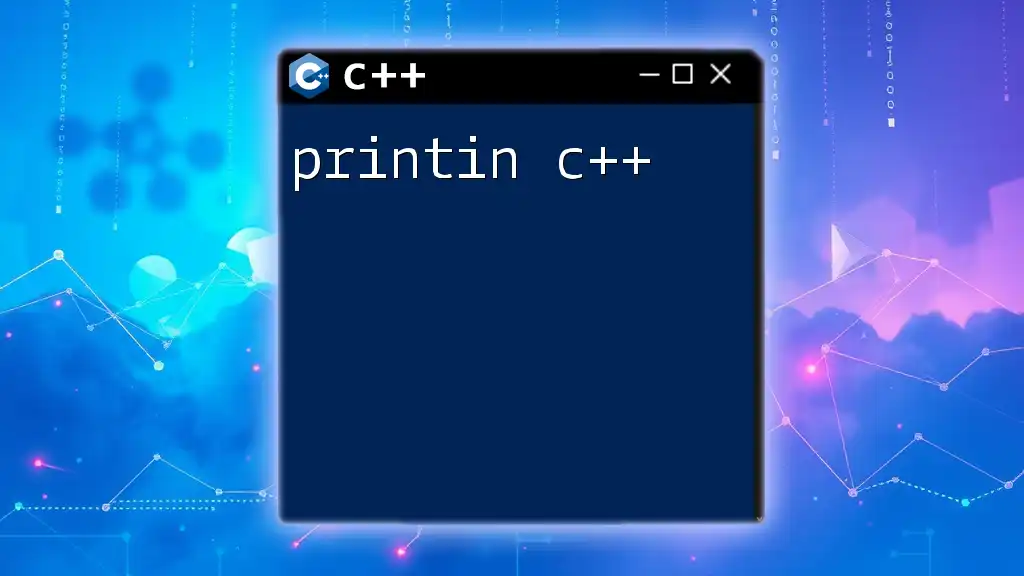
Frequently Asked Questions about C++ Alignment
C++ alignment can also raise many questions. Common inquiries include how to handle multiple data types in a single array and ensuring cross-platform consistency. Developing a strong foundation in alignment principles will help you navigate these complexities with confidence, guiding your C++ coding practices toward greater efficiency and reliability.