In C++, the `std::accumulate` function from the `<numeric>` header can be used to compute the sum (or sigma) of elements in a container, such as an array or vector.
Here’s a code snippet that demonstrates how to use `std::accumulate` to calculate the sum of elements in a vector:
#include <iostream>
#include <vector>
#include <numeric>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
int sum = std::accumulate(numbers.begin(), numbers.end(), 0);
std::cout << "The sum is: " << sum << std::endl;
return 0;
}
Understanding Sigma in C++
What is Sigma?
Sigma is often used to represent summation in mathematics, particularly giving a concise way to express the sum of a series of terms. In the context of C++, understanding sigma is essential for efficiently writing code that processes numerical data. It highlights the role of summation within algorithms, allowing programmers to create clear and optimized solutions.
The Sigma Notation
Definition and Explanation
The sigma notation uses the Greek letter sigma (Σ) to denote the sum of a sequence of terms. It is a powerful tool that simplifies mathematical expressions involving addition, enabling coders to translate mathematical concepts into effective programming implementations.
Real-world Applications of Sigma Notation
Sigma is frequently utilized in various domains, including data analysis, financial calculations, and statistical functions. Understanding sigma allows developers to harness its power for efficient computations.
Mathematical Background
Summation Basics
Summation forms the backbone of many algorithms and calculations. By efficiently summarizing a series of numbers, programmers can derive insights from data and facilitate further computations.
The Relevance of Sigma in Algorithms
In algorithm design, sigma notation often provides a basis for assessing an algorithm's time complexity. Understanding how frequently an operation executes (often determined by summing iterations) can significantly impact performance.
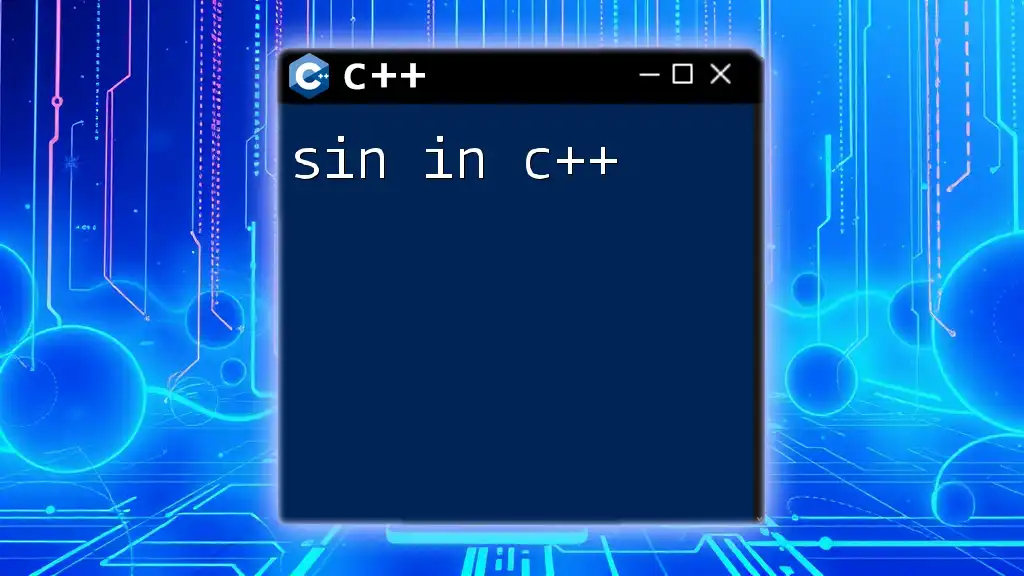
Implementing Sigma in C++
Using Loops for Summation
For Loop Example
A common straightforward method to calculate the summation of a sequence is through the use of a for loop. This method is efficient and easy to understand.
int sum = 0;
for (int i = 1; i <= n; i++) {
sum += i;
}
This code snippet initializes a variable `sum` to zero, then iterates from 1 to `n`, adding each successive number to `sum`. It effectively calculates the value of \( \sigma(n) = 1 + 2 + ... + n \).
While Loop Example
Another way to achieve the same result is by using a while loop, which can sometimes provide clearer logic for certain conditions.
int sum = 0, i = 1;
while (i <= n) {
sum += i;
i++;
}
In this example, a sum is maintained while incrementing `i` until it surpasses `n`. Both techniques yield the same result, showing how sigma can be represented through simple iterative processes.
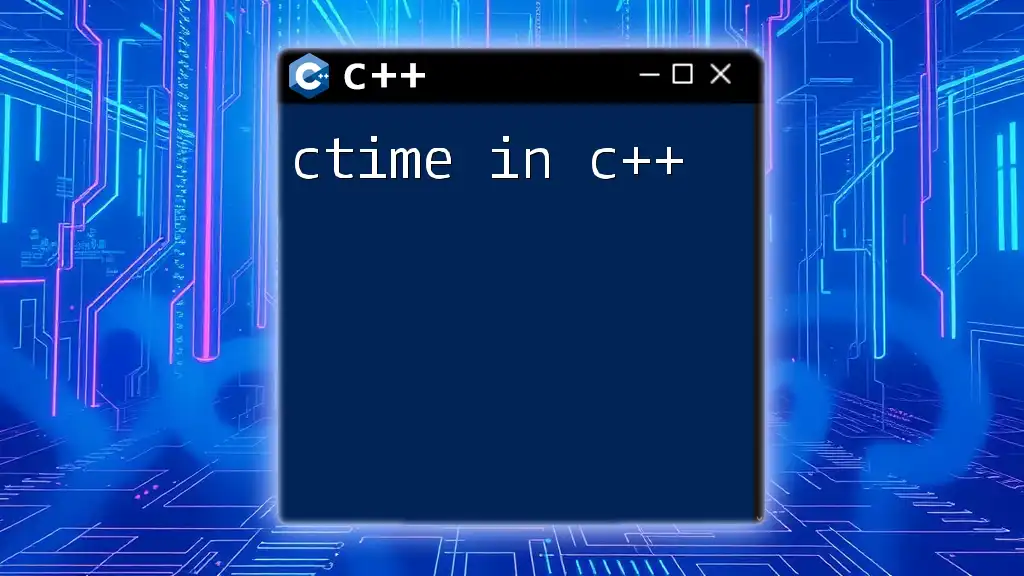
Advanced Sigma Techniques
Recursive Summation
What is Recursion?
Recursion is a method in programming where a function calls itself to break down complex problems into simpler ones. This technique is especially useful when working with mathematical formulations.
Recursive Function Example
A recursive function can elegantly compute summation through self-reference:
int sigma(int n) {
if (n <= 0) return 0;
return n + sigma(n - 1);
}
Here, the function calls itself each time by decrementing `n` until it reaches the base case. While recursion may be less intuitive than iterative approaches, it often results in cleaner and more modular code.
Using STL Algorithms
Introduction to the Standard Template Library (STL)
C++'s Standard Template Library offers built-in functions, vastly simplifying operations like summation.
std::accumulate Usage
The `std::accumulate` function provides an elegant solution for deriving sums within containers.
#include <numeric> // Required for std::accumulate
#include <vector>
std::vector<int> vec = {1, 2, 3, 4, 5};
int sum = std::accumulate(vec.begin(), vec.end(), 0);
This snippet demonstrates how to accumulate values in a vector. The third argument initializes the sum; in this case, it starts from `0`. Utilizing STL not only enhances code readability but also optimizes performance, allowing developers to focus on solving higher-level problems.
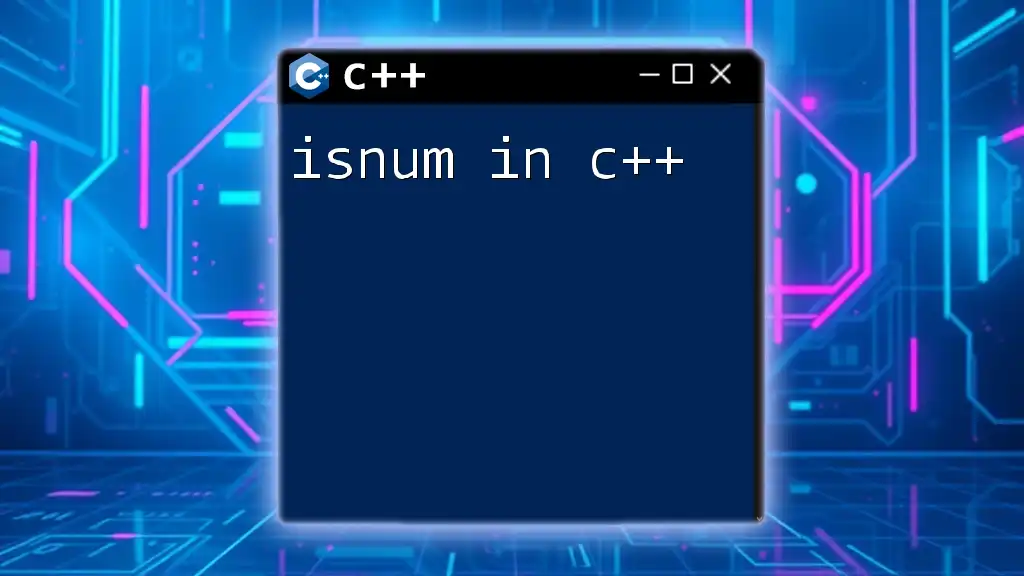
Practical Applications of Sigma in C++
Calculating Factorials using Sigma
Sigma's Role in Factorial Calculations
Factorials are fundamental in many computing tasks, especially in combination and permutation calculations. Here, sigma assists in the iterative buildup of products.
int factorial(int n) {
if (n < 0) return -1; // Error for negative numbers
return sigma(n); // Assuming sigma is defined as in an earlier example
}
This use of sigma may prompt a realization of its versatility—not just summation, but as a building block for more complex operations.
Summation in Data Analysis
Considering applications in data analysis, sigma is crucial for statistical computations—such as deriving the mean, variance, and standard deviation of datasets. By using summation methods, programmers can achieve insightful statistical analyses that are essential for decision-making processes.
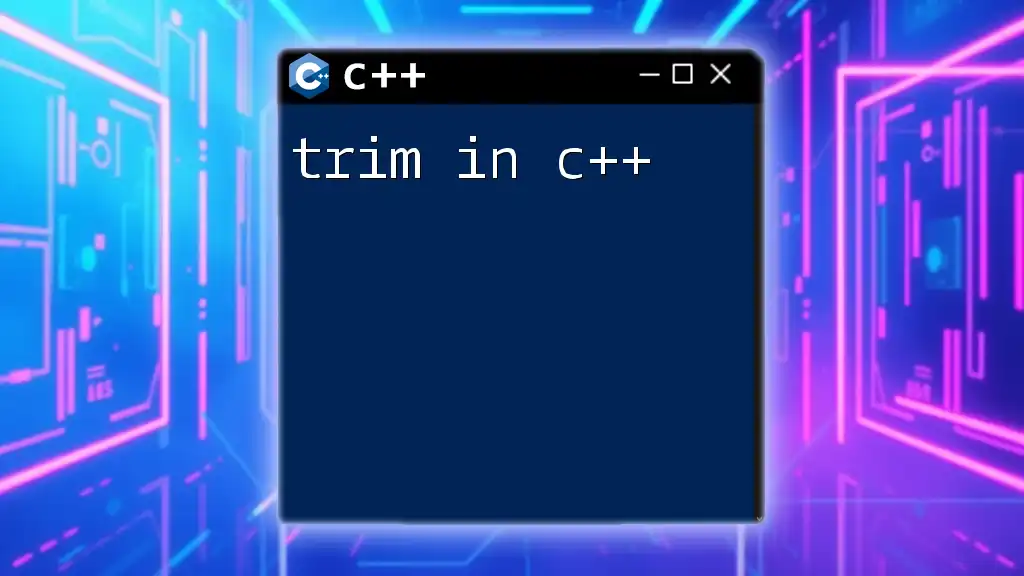
Common Errors and Debugging Tips
Common Mistakes in Sigma Implementation
Off-by-One Errors
When implementing sigma, a frequent error arises when indices start at `1` instead of `0` in loops, which can lead to incorrect sums. It is important to validate these bounds carefully.
Infinite Recursion in Recursive Functions
Failing to establish a base case in recursive functions can lead to infinite cycles. Ensuring a proper exit condition is crucial for maintaining system performance and preventing crashes.
Debugging Techniques
Effective debugging of sigma implementations may involve introducing print statements to trace variable states within loops or using dedicated debugging tools. These methods help isolate faults and improve understanding throughout development.
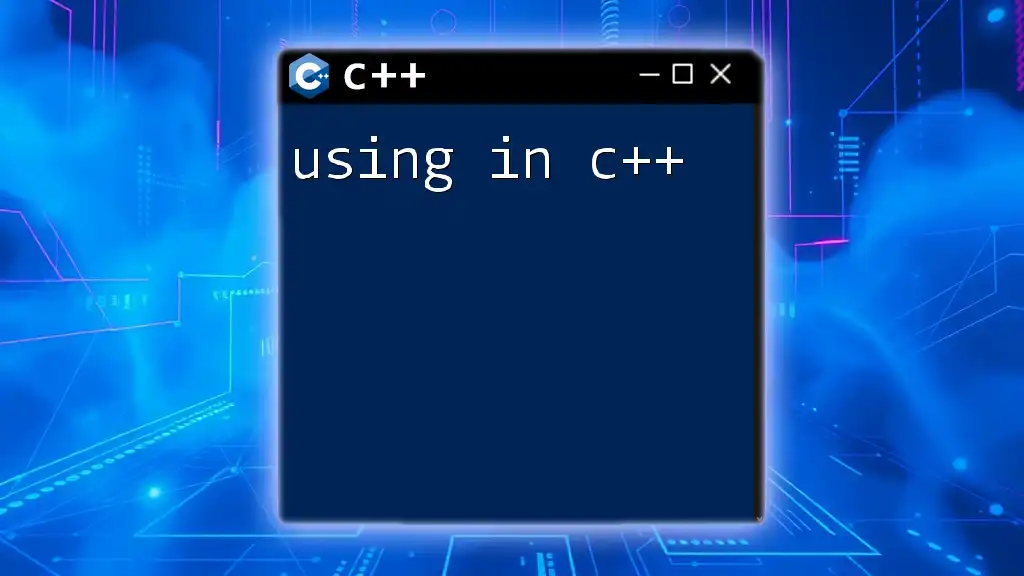
Performance Considerations
Comparing Loop Methods
When comparing loop methods, particularly for larger `n`, overall performance becomes apparent. Iterative loops tend to be more efficient than recursive methods, as each recursive call adds overhead in terms of function calls and memory stack usage.
Optimizing Summation Techniques
For large datasets, mathematic shortcuts can enhance performance. For example, using the formula for the sum of the first `n` natural numbers, \( \text{sum} = \frac{n(n + 1)}{2} \), can significantly reduce computational complexity.
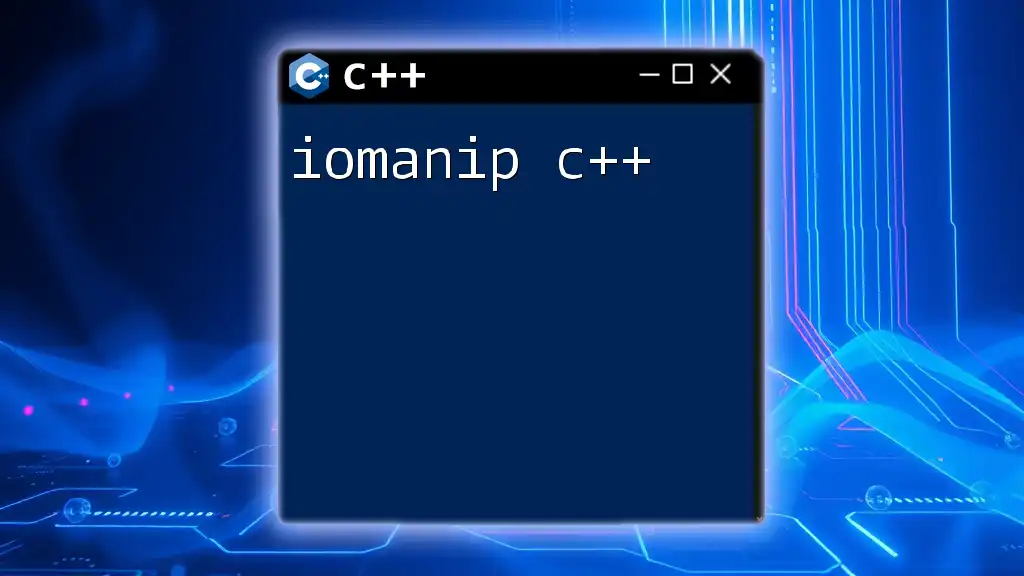
Conclusion
Understanding sigma in C++ is integral for any programmer interested in numerical computations or algorithm design. By leveraging various sigma techniques, developers can write concise, efficient code while avoiding common pitfalls that can impact performance. Practicing these methods can sharpen one's programming skills and optimize solutions across multiple domains.
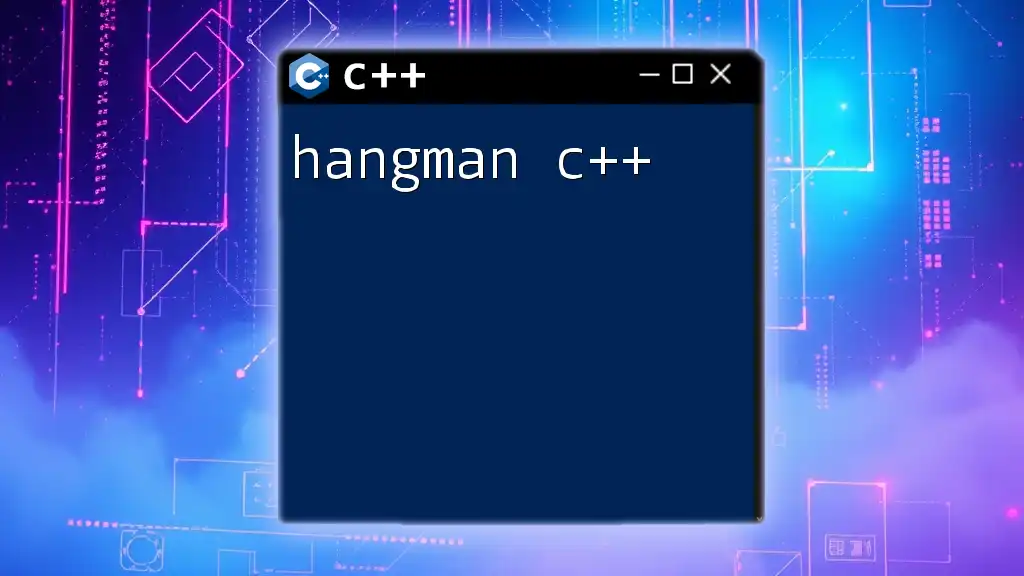
Additional Resources
To further deepen your knowledge of sigma and summation techniques, consider exploring online tutorials, reading foundational books on C++, and engaging in community forums where like-minded individuals share insights and support.
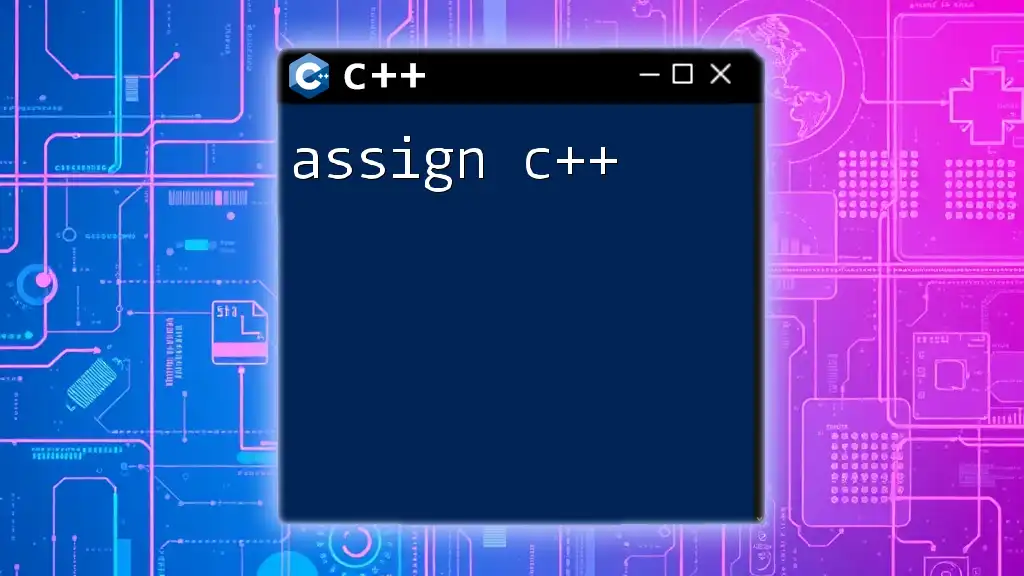
FAQs
What are some common applications of Sigma in programming?
Sigma finds use in statistical analysis, algorithm complexity measurements, and financial calculations, making it versatile across domains.
How does Sigma improve coding efficiency?
By providing clear and concise mathematical representations, sigma allows programmers to improve write times and reduce logical complexity in their code.
Are there any libraries in C++ specifically for Sigma-related calculations?
While not exclusive to sigma, the Standard Template Library (STL) offers functionalities like `std::accumulate`, which streamline summation processes in C++.
By exploring these concepts, you can effectively incorporate the power of sigma into your C++ projects!